Creating a GUI with PyQt5 to interact with an Excel file, trouble loading sheets names and subject list into...
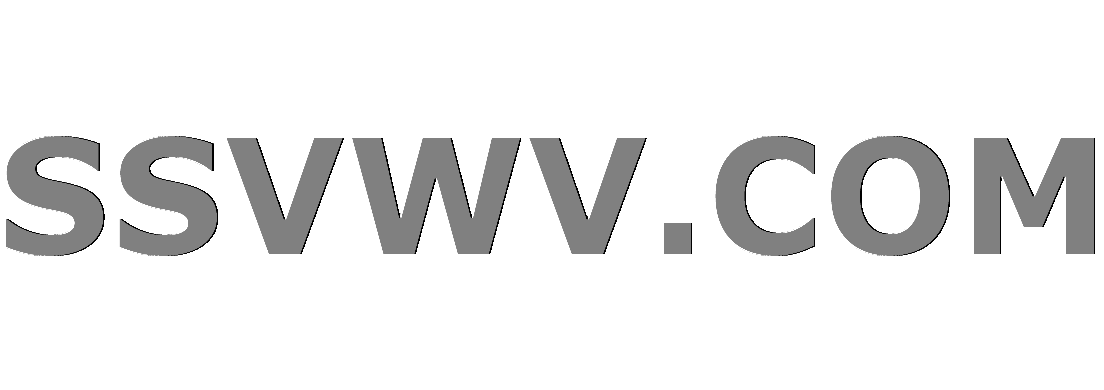
Multi tool use
up vote
0
down vote
favorite
Goal of the app: open an excel file, allow the user to select a sheet, generate a searchable list of subjects, return specific cells from that row
Essentially, the department I work for has excel outputs that they have but don't know how to use, and I'm trying to create a simple app to return the data they need. I'm new to coding, and I've tried a lot of ways recently to build this application. I have settled on using PyQt5 to create the GUI and pandas to read the excel file.
Right now, I'm trying to use comboboxes to allow the user to select the tab and the subject, but I can't get the information from the opened file into the comboboxes.
Currently, the code for my boxes is as follows:
class MainWindow(QMainWindow):
def __init__(self, parent=None):
super().__init__()
self.title = 'NU Forceplate Data'
self.setWindowIcon(QIcon('image.png'))
QApplication.setStyle(QStyleFactory.create('Fusion'))
self.initUI()
# ...
def initUI(self):
# ...
self.sheetsBox()
# ...
def sheetsBox(self):
self.sheetselectionbox = QComboBox(self)
sheetsBox.move(50, 75)
sheetsBox.activated[str].connect(self.sheets) #I still don't understand this
def sheets(self, text):
self.sheetselection.setText(text) #or this
#if I don't pass on this, the kernel doesn't crash but the box won't show up
def file_open(self):
import_excel_name, _ = QFileDialog.getOpenFileName(self, "Please select file:")
if import_excel_name:
with pd.ExcelFile(selected_file) as xls:
sheet_tabnames = xls.sheet_names
self.sheetselectionbox.clear()
self.sheetselectionbox.addItems(sheet_tabnames)
# ...
if __name__ == "__main__":
app = QApplication(sys.argv)
mainWin = MainWindow()
sys.exit( app.exec_() )
I can't figure out what to to put in sheets, so I've passed on its definition.
I'm not sure if this is enough information, happy to provide more if needed. I'm not sure if this is even the best way to do this, so I'm open to any other suggestions.
python excel user-interface combobox pyqt
add a comment |
up vote
0
down vote
favorite
Goal of the app: open an excel file, allow the user to select a sheet, generate a searchable list of subjects, return specific cells from that row
Essentially, the department I work for has excel outputs that they have but don't know how to use, and I'm trying to create a simple app to return the data they need. I'm new to coding, and I've tried a lot of ways recently to build this application. I have settled on using PyQt5 to create the GUI and pandas to read the excel file.
Right now, I'm trying to use comboboxes to allow the user to select the tab and the subject, but I can't get the information from the opened file into the comboboxes.
Currently, the code for my boxes is as follows:
class MainWindow(QMainWindow):
def __init__(self, parent=None):
super().__init__()
self.title = 'NU Forceplate Data'
self.setWindowIcon(QIcon('image.png'))
QApplication.setStyle(QStyleFactory.create('Fusion'))
self.initUI()
# ...
def initUI(self):
# ...
self.sheetsBox()
# ...
def sheetsBox(self):
self.sheetselectionbox = QComboBox(self)
sheetsBox.move(50, 75)
sheetsBox.activated[str].connect(self.sheets) #I still don't understand this
def sheets(self, text):
self.sheetselection.setText(text) #or this
#if I don't pass on this, the kernel doesn't crash but the box won't show up
def file_open(self):
import_excel_name, _ = QFileDialog.getOpenFileName(self, "Please select file:")
if import_excel_name:
with pd.ExcelFile(selected_file) as xls:
sheet_tabnames = xls.sheet_names
self.sheetselectionbox.clear()
self.sheetselectionbox.addItems(sheet_tabnames)
# ...
if __name__ == "__main__":
app = QApplication(sys.argv)
mainWin = MainWindow()
sys.exit( app.exec_() )
I can't figure out what to to put in sheets, so I've passed on its definition.
I'm not sure if this is enough information, happy to provide more if needed. I'm not sure if this is even the best way to do this, so I'm open to any other suggestions.
python excel user-interface combobox pyqt
what is sheetsBox insheetsBox.move(50, 75)?In addition to that I have corrected a typo, is there an error in your original code?
– eyllanesc
Nov 20 at 18:02
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Goal of the app: open an excel file, allow the user to select a sheet, generate a searchable list of subjects, return specific cells from that row
Essentially, the department I work for has excel outputs that they have but don't know how to use, and I'm trying to create a simple app to return the data they need. I'm new to coding, and I've tried a lot of ways recently to build this application. I have settled on using PyQt5 to create the GUI and pandas to read the excel file.
Right now, I'm trying to use comboboxes to allow the user to select the tab and the subject, but I can't get the information from the opened file into the comboboxes.
Currently, the code for my boxes is as follows:
class MainWindow(QMainWindow):
def __init__(self, parent=None):
super().__init__()
self.title = 'NU Forceplate Data'
self.setWindowIcon(QIcon('image.png'))
QApplication.setStyle(QStyleFactory.create('Fusion'))
self.initUI()
# ...
def initUI(self):
# ...
self.sheetsBox()
# ...
def sheetsBox(self):
self.sheetselectionbox = QComboBox(self)
sheetsBox.move(50, 75)
sheetsBox.activated[str].connect(self.sheets) #I still don't understand this
def sheets(self, text):
self.sheetselection.setText(text) #or this
#if I don't pass on this, the kernel doesn't crash but the box won't show up
def file_open(self):
import_excel_name, _ = QFileDialog.getOpenFileName(self, "Please select file:")
if import_excel_name:
with pd.ExcelFile(selected_file) as xls:
sheet_tabnames = xls.sheet_names
self.sheetselectionbox.clear()
self.sheetselectionbox.addItems(sheet_tabnames)
# ...
if __name__ == "__main__":
app = QApplication(sys.argv)
mainWin = MainWindow()
sys.exit( app.exec_() )
I can't figure out what to to put in sheets, so I've passed on its definition.
I'm not sure if this is enough information, happy to provide more if needed. I'm not sure if this is even the best way to do this, so I'm open to any other suggestions.
python excel user-interface combobox pyqt
Goal of the app: open an excel file, allow the user to select a sheet, generate a searchable list of subjects, return specific cells from that row
Essentially, the department I work for has excel outputs that they have but don't know how to use, and I'm trying to create a simple app to return the data they need. I'm new to coding, and I've tried a lot of ways recently to build this application. I have settled on using PyQt5 to create the GUI and pandas to read the excel file.
Right now, I'm trying to use comboboxes to allow the user to select the tab and the subject, but I can't get the information from the opened file into the comboboxes.
Currently, the code for my boxes is as follows:
class MainWindow(QMainWindow):
def __init__(self, parent=None):
super().__init__()
self.title = 'NU Forceplate Data'
self.setWindowIcon(QIcon('image.png'))
QApplication.setStyle(QStyleFactory.create('Fusion'))
self.initUI()
# ...
def initUI(self):
# ...
self.sheetsBox()
# ...
def sheetsBox(self):
self.sheetselectionbox = QComboBox(self)
sheetsBox.move(50, 75)
sheetsBox.activated[str].connect(self.sheets) #I still don't understand this
def sheets(self, text):
self.sheetselection.setText(text) #or this
#if I don't pass on this, the kernel doesn't crash but the box won't show up
def file_open(self):
import_excel_name, _ = QFileDialog.getOpenFileName(self, "Please select file:")
if import_excel_name:
with pd.ExcelFile(selected_file) as xls:
sheet_tabnames = xls.sheet_names
self.sheetselectionbox.clear()
self.sheetselectionbox.addItems(sheet_tabnames)
# ...
if __name__ == "__main__":
app = QApplication(sys.argv)
mainWin = MainWindow()
sys.exit( app.exec_() )
I can't figure out what to to put in sheets, so I've passed on its definition.
I'm not sure if this is enough information, happy to provide more if needed. I'm not sure if this is even the best way to do this, so I'm open to any other suggestions.
python excel user-interface combobox pyqt
python excel user-interface combobox pyqt
edited Nov 20 at 18:06


eyllanesc
70.7k93052
70.7k93052
asked Nov 20 at 5:42
Anna Applegate
43
43
what is sheetsBox insheetsBox.move(50, 75)?In addition to that I have corrected a typo, is there an error in your original code?
– eyllanesc
Nov 20 at 18:02
add a comment |
what is sheetsBox insheetsBox.move(50, 75)?In addition to that I have corrected a typo, is there an error in your original code?
– eyllanesc
Nov 20 at 18:02
what is sheetsBox in
sheetsBox.move(50, 75)?In addition to that I have corrected a typo, is there an error in your original code?
– eyllanesc
Nov 20 at 18:02
what is sheetsBox in
sheetsBox.move(50, 75)?In addition to that I have corrected a typo, is there an error in your original code?
– eyllanesc
Nov 20 at 18:02
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53386896%2fcreating-a-gui-with-pyqt5-to-interact-with-an-excel-file-trouble-loading-sheets%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0EE6Jf5tv5r,xZEi,cq3jqkIyWq5vNSwve,Z1sOEXi6cMBio x8TLWFK88
what is sheetsBox in
sheetsBox.move(50, 75)?In addition to that I have corrected a typo, is there an error in your original code?
– eyllanesc
Nov 20 at 18:02