sliding window for linear regression using numpy as_strided
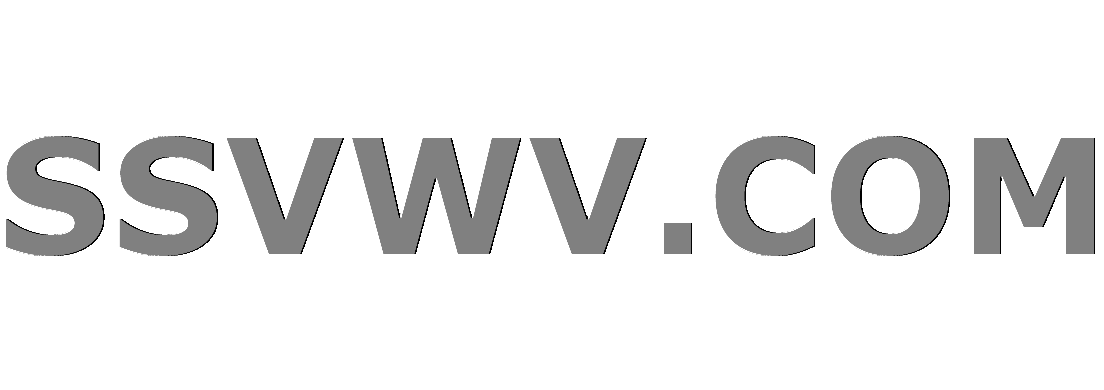
Multi tool use
I have leveraged the rolling window examples using as_strided to create various sliding versions of numpy functions.
def std(a,window):
shape = a.shape[:-1] + (a.shape[-1] - window + 1, window)
strides = a.strides + (a.strides[-1],)
return np.std(np.lib.stride_tricks.as_strided(a, shape=shape,strides=strides),axis=1)
Now I'm attempt to leverage the same as_strided method on a linear regression function. y = a + bx
def linear_regress(x,y):
sum_x = np.sum(x)
sum_y = np.sum(y)
sum_xy = np.sum(np.multiply(x,y))
sum_xx = np.sum(np.multiply(x,x))
sum_yy = np.sum(np.multiply(y,y))
number_of_records = len(x)
A = (sum_y*sum_xx - sum_x*sum_xy)/(number_of_records*sum_xx - sum_x*sum_x)
B = (number_of_records*sum_xy - sum_x*sum_y)/(number_of_records*sum_xx - sum_x*sum_x)
return A + B*number_of_records
You can get the same result using stats
from scipy import stats
slope, intercept, r_value, p_value, std_err = stats.linregress(x,p)
xValue = len(x)
y = (slope + intercept*xValue)
I am not sure how to fit the above functions into the as_strided method when two arrays are passed. I guess I would have to create two shapes and pass both through?
def rolling_lr(x,y,window):
shape = y.shape[:-1] + (y.shape[-1] - window + 1, window)
strides = y.strides + (y.strides[-1],)
return linear_regress(np.lib.stride_tricks.as_strided(x,y shape=shape, strides=strides))
Any help is appreciated.
python numpy regression
add a comment |
I have leveraged the rolling window examples using as_strided to create various sliding versions of numpy functions.
def std(a,window):
shape = a.shape[:-1] + (a.shape[-1] - window + 1, window)
strides = a.strides + (a.strides[-1],)
return np.std(np.lib.stride_tricks.as_strided(a, shape=shape,strides=strides),axis=1)
Now I'm attempt to leverage the same as_strided method on a linear regression function. y = a + bx
def linear_regress(x,y):
sum_x = np.sum(x)
sum_y = np.sum(y)
sum_xy = np.sum(np.multiply(x,y))
sum_xx = np.sum(np.multiply(x,x))
sum_yy = np.sum(np.multiply(y,y))
number_of_records = len(x)
A = (sum_y*sum_xx - sum_x*sum_xy)/(number_of_records*sum_xx - sum_x*sum_x)
B = (number_of_records*sum_xy - sum_x*sum_y)/(number_of_records*sum_xx - sum_x*sum_x)
return A + B*number_of_records
You can get the same result using stats
from scipy import stats
slope, intercept, r_value, p_value, std_err = stats.linregress(x,p)
xValue = len(x)
y = (slope + intercept*xValue)
I am not sure how to fit the above functions into the as_strided method when two arrays are passed. I guess I would have to create two shapes and pass both through?
def rolling_lr(x,y,window):
shape = y.shape[:-1] + (y.shape[-1] - window + 1, window)
strides = y.strides + (y.strides[-1],)
return linear_regress(np.lib.stride_tricks.as_strided(x,y shape=shape, strides=strides))
Any help is appreciated.
python numpy regression
This doesn't answer your question, but I recommend usingskimage.util.view_as_windows
for rolling windows.
– Nils Werner
Nov 23 '18 at 22:21
add a comment |
I have leveraged the rolling window examples using as_strided to create various sliding versions of numpy functions.
def std(a,window):
shape = a.shape[:-1] + (a.shape[-1] - window + 1, window)
strides = a.strides + (a.strides[-1],)
return np.std(np.lib.stride_tricks.as_strided(a, shape=shape,strides=strides),axis=1)
Now I'm attempt to leverage the same as_strided method on a linear regression function. y = a + bx
def linear_regress(x,y):
sum_x = np.sum(x)
sum_y = np.sum(y)
sum_xy = np.sum(np.multiply(x,y))
sum_xx = np.sum(np.multiply(x,x))
sum_yy = np.sum(np.multiply(y,y))
number_of_records = len(x)
A = (sum_y*sum_xx - sum_x*sum_xy)/(number_of_records*sum_xx - sum_x*sum_x)
B = (number_of_records*sum_xy - sum_x*sum_y)/(number_of_records*sum_xx - sum_x*sum_x)
return A + B*number_of_records
You can get the same result using stats
from scipy import stats
slope, intercept, r_value, p_value, std_err = stats.linregress(x,p)
xValue = len(x)
y = (slope + intercept*xValue)
I am not sure how to fit the above functions into the as_strided method when two arrays are passed. I guess I would have to create two shapes and pass both through?
def rolling_lr(x,y,window):
shape = y.shape[:-1] + (y.shape[-1] - window + 1, window)
strides = y.strides + (y.strides[-1],)
return linear_regress(np.lib.stride_tricks.as_strided(x,y shape=shape, strides=strides))
Any help is appreciated.
python numpy regression
I have leveraged the rolling window examples using as_strided to create various sliding versions of numpy functions.
def std(a,window):
shape = a.shape[:-1] + (a.shape[-1] - window + 1, window)
strides = a.strides + (a.strides[-1],)
return np.std(np.lib.stride_tricks.as_strided(a, shape=shape,strides=strides),axis=1)
Now I'm attempt to leverage the same as_strided method on a linear regression function. y = a + bx
def linear_regress(x,y):
sum_x = np.sum(x)
sum_y = np.sum(y)
sum_xy = np.sum(np.multiply(x,y))
sum_xx = np.sum(np.multiply(x,x))
sum_yy = np.sum(np.multiply(y,y))
number_of_records = len(x)
A = (sum_y*sum_xx - sum_x*sum_xy)/(number_of_records*sum_xx - sum_x*sum_x)
B = (number_of_records*sum_xy - sum_x*sum_y)/(number_of_records*sum_xx - sum_x*sum_x)
return A + B*number_of_records
You can get the same result using stats
from scipy import stats
slope, intercept, r_value, p_value, std_err = stats.linregress(x,p)
xValue = len(x)
y = (slope + intercept*xValue)
I am not sure how to fit the above functions into the as_strided method when two arrays are passed. I guess I would have to create two shapes and pass both through?
def rolling_lr(x,y,window):
shape = y.shape[:-1] + (y.shape[-1] - window + 1, window)
strides = y.strides + (y.strides[-1],)
return linear_regress(np.lib.stride_tricks.as_strided(x,y shape=shape, strides=strides))
Any help is appreciated.
python numpy regression
python numpy regression
edited Nov 25 '18 at 18:04
John Holmes
asked Nov 23 '18 at 22:05
John HolmesJohn Holmes
247
247
This doesn't answer your question, but I recommend usingskimage.util.view_as_windows
for rolling windows.
– Nils Werner
Nov 23 '18 at 22:21
add a comment |
This doesn't answer your question, but I recommend usingskimage.util.view_as_windows
for rolling windows.
– Nils Werner
Nov 23 '18 at 22:21
This doesn't answer your question, but I recommend using
skimage.util.view_as_windows
for rolling windows.– Nils Werner
Nov 23 '18 at 22:21
This doesn't answer your question, but I recommend using
skimage.util.view_as_windows
for rolling windows.– Nils Werner
Nov 23 '18 at 22:21
add a comment |
2 Answers
2
active
oldest
votes
Here is what i came up with. Not the prettiest but works.
def linear_regress(x,y,window):
x_shape = x.shape[:-1] + (x.shape[-1] - window + 1, window)
x_strides = x.strides + (x.strides[-1],)
y_shape = y.shape[:-1] + (y.shape[-1] - window + 1, window)
y_strides = y.strides + (y.strides[-1],)
sx = np.lib.stride_tricks.as_strided(x,shape=x_shape, strides=x_strides)
sy = np.lib.stride_tricks.as_strided(y,shape=y_shape, strides=y_strides)
sum_x = np.sum(sx,axis=1)
sum_y = np.sum(sy,axis=1)
sum_xy = np.sum(np.multiply(sx,sy),axis=1)
sum_xx = np.sum(np.multiply(sx,sx),axis=1)
sum_yy = np.sum(np.multiply(sy,sy),axis=1)
m = (sum_y*sum_xx - sum_x*sum_xy)/(window*sum_xx - sum_x*sum_x)
b = (window*sum_xy - sum_x*sum_y)/(window*sum_xx - sum_x*sum_x)
add a comment |
Interesting, I have never seen the stride function. The docs do warn about this method however. An alternative method would be using linear algebra to do the regression on the windows. Here is a trivial example:
from numpy import *
# generate points
N = 30
x = linspace(0, 10, N)[:, None]
X = ones((N, 1)) * x
Y = X * array([1, 30]) + random.randn(*X.shape)*1e-1
XX = concatenate((ones((N,1)), X), axis = 1)
# window data
windowLength = 10
windows = array([roll(
XX, -i * windowLength, axis = 0)[:windowLength, :]
for i in range(len(XX) - windowLength)])
windowsY = array([roll(Y, -i * windowLength, axis = 0)[:windowLength, :]
for i in range(len(Y) - windowLength)])
# linear regression on windows
reg = array([
((linalg.pinv(wx.T.dot(wx))).dot(wx.T)).dot(wy) for
wx, wy in zip(windows, windowsY)])
# plot regression on windows
from matplotlib import style
style.use('seaborn-poster')
from matplotlib.pyplot import subplots, cm
fig, ax = subplots()
colors = cm.tab20(linspace(0, 1, len(windows)))
for win, color, coeffs, yi in zip(windows, colors, reg, windowsY):
ax.plot(win, yi,'.', alpha = .5, color = color)
ax.plot(win[:, 1], win.dot(coeffs), alpha = .5, color = color)
x += 1
ax.set(**dict(xlabel = 'x', ylabel = 'y'))
Which produces:
thanks Global. I use as_strided for the speed. I have read the potential issues with using as_strided.
– John Holmes
Nov 25 '18 at 17:11
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53453383%2fsliding-window-for-linear-regression-using-numpy-as-strided%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here is what i came up with. Not the prettiest but works.
def linear_regress(x,y,window):
x_shape = x.shape[:-1] + (x.shape[-1] - window + 1, window)
x_strides = x.strides + (x.strides[-1],)
y_shape = y.shape[:-1] + (y.shape[-1] - window + 1, window)
y_strides = y.strides + (y.strides[-1],)
sx = np.lib.stride_tricks.as_strided(x,shape=x_shape, strides=x_strides)
sy = np.lib.stride_tricks.as_strided(y,shape=y_shape, strides=y_strides)
sum_x = np.sum(sx,axis=1)
sum_y = np.sum(sy,axis=1)
sum_xy = np.sum(np.multiply(sx,sy),axis=1)
sum_xx = np.sum(np.multiply(sx,sx),axis=1)
sum_yy = np.sum(np.multiply(sy,sy),axis=1)
m = (sum_y*sum_xx - sum_x*sum_xy)/(window*sum_xx - sum_x*sum_x)
b = (window*sum_xy - sum_x*sum_y)/(window*sum_xx - sum_x*sum_x)
add a comment |
Here is what i came up with. Not the prettiest but works.
def linear_regress(x,y,window):
x_shape = x.shape[:-1] + (x.shape[-1] - window + 1, window)
x_strides = x.strides + (x.strides[-1],)
y_shape = y.shape[:-1] + (y.shape[-1] - window + 1, window)
y_strides = y.strides + (y.strides[-1],)
sx = np.lib.stride_tricks.as_strided(x,shape=x_shape, strides=x_strides)
sy = np.lib.stride_tricks.as_strided(y,shape=y_shape, strides=y_strides)
sum_x = np.sum(sx,axis=1)
sum_y = np.sum(sy,axis=1)
sum_xy = np.sum(np.multiply(sx,sy),axis=1)
sum_xx = np.sum(np.multiply(sx,sx),axis=1)
sum_yy = np.sum(np.multiply(sy,sy),axis=1)
m = (sum_y*sum_xx - sum_x*sum_xy)/(window*sum_xx - sum_x*sum_x)
b = (window*sum_xy - sum_x*sum_y)/(window*sum_xx - sum_x*sum_x)
add a comment |
Here is what i came up with. Not the prettiest but works.
def linear_regress(x,y,window):
x_shape = x.shape[:-1] + (x.shape[-1] - window + 1, window)
x_strides = x.strides + (x.strides[-1],)
y_shape = y.shape[:-1] + (y.shape[-1] - window + 1, window)
y_strides = y.strides + (y.strides[-1],)
sx = np.lib.stride_tricks.as_strided(x,shape=x_shape, strides=x_strides)
sy = np.lib.stride_tricks.as_strided(y,shape=y_shape, strides=y_strides)
sum_x = np.sum(sx,axis=1)
sum_y = np.sum(sy,axis=1)
sum_xy = np.sum(np.multiply(sx,sy),axis=1)
sum_xx = np.sum(np.multiply(sx,sx),axis=1)
sum_yy = np.sum(np.multiply(sy,sy),axis=1)
m = (sum_y*sum_xx - sum_x*sum_xy)/(window*sum_xx - sum_x*sum_x)
b = (window*sum_xy - sum_x*sum_y)/(window*sum_xx - sum_x*sum_x)
Here is what i came up with. Not the prettiest but works.
def linear_regress(x,y,window):
x_shape = x.shape[:-1] + (x.shape[-1] - window + 1, window)
x_strides = x.strides + (x.strides[-1],)
y_shape = y.shape[:-1] + (y.shape[-1] - window + 1, window)
y_strides = y.strides + (y.strides[-1],)
sx = np.lib.stride_tricks.as_strided(x,shape=x_shape, strides=x_strides)
sy = np.lib.stride_tricks.as_strided(y,shape=y_shape, strides=y_strides)
sum_x = np.sum(sx,axis=1)
sum_y = np.sum(sy,axis=1)
sum_xy = np.sum(np.multiply(sx,sy),axis=1)
sum_xx = np.sum(np.multiply(sx,sx),axis=1)
sum_yy = np.sum(np.multiply(sy,sy),axis=1)
m = (sum_y*sum_xx - sum_x*sum_xy)/(window*sum_xx - sum_x*sum_x)
b = (window*sum_xy - sum_x*sum_y)/(window*sum_xx - sum_x*sum_x)
answered Nov 25 '18 at 1:17
John HolmesJohn Holmes
247
247
add a comment |
add a comment |
Interesting, I have never seen the stride function. The docs do warn about this method however. An alternative method would be using linear algebra to do the regression on the windows. Here is a trivial example:
from numpy import *
# generate points
N = 30
x = linspace(0, 10, N)[:, None]
X = ones((N, 1)) * x
Y = X * array([1, 30]) + random.randn(*X.shape)*1e-1
XX = concatenate((ones((N,1)), X), axis = 1)
# window data
windowLength = 10
windows = array([roll(
XX, -i * windowLength, axis = 0)[:windowLength, :]
for i in range(len(XX) - windowLength)])
windowsY = array([roll(Y, -i * windowLength, axis = 0)[:windowLength, :]
for i in range(len(Y) - windowLength)])
# linear regression on windows
reg = array([
((linalg.pinv(wx.T.dot(wx))).dot(wx.T)).dot(wy) for
wx, wy in zip(windows, windowsY)])
# plot regression on windows
from matplotlib import style
style.use('seaborn-poster')
from matplotlib.pyplot import subplots, cm
fig, ax = subplots()
colors = cm.tab20(linspace(0, 1, len(windows)))
for win, color, coeffs, yi in zip(windows, colors, reg, windowsY):
ax.plot(win, yi,'.', alpha = .5, color = color)
ax.plot(win[:, 1], win.dot(coeffs), alpha = .5, color = color)
x += 1
ax.set(**dict(xlabel = 'x', ylabel = 'y'))
Which produces:
thanks Global. I use as_strided for the speed. I have read the potential issues with using as_strided.
– John Holmes
Nov 25 '18 at 17:11
add a comment |
Interesting, I have never seen the stride function. The docs do warn about this method however. An alternative method would be using linear algebra to do the regression on the windows. Here is a trivial example:
from numpy import *
# generate points
N = 30
x = linspace(0, 10, N)[:, None]
X = ones((N, 1)) * x
Y = X * array([1, 30]) + random.randn(*X.shape)*1e-1
XX = concatenate((ones((N,1)), X), axis = 1)
# window data
windowLength = 10
windows = array([roll(
XX, -i * windowLength, axis = 0)[:windowLength, :]
for i in range(len(XX) - windowLength)])
windowsY = array([roll(Y, -i * windowLength, axis = 0)[:windowLength, :]
for i in range(len(Y) - windowLength)])
# linear regression on windows
reg = array([
((linalg.pinv(wx.T.dot(wx))).dot(wx.T)).dot(wy) for
wx, wy in zip(windows, windowsY)])
# plot regression on windows
from matplotlib import style
style.use('seaborn-poster')
from matplotlib.pyplot import subplots, cm
fig, ax = subplots()
colors = cm.tab20(linspace(0, 1, len(windows)))
for win, color, coeffs, yi in zip(windows, colors, reg, windowsY):
ax.plot(win, yi,'.', alpha = .5, color = color)
ax.plot(win[:, 1], win.dot(coeffs), alpha = .5, color = color)
x += 1
ax.set(**dict(xlabel = 'x', ylabel = 'y'))
Which produces:
thanks Global. I use as_strided for the speed. I have read the potential issues with using as_strided.
– John Holmes
Nov 25 '18 at 17:11
add a comment |
Interesting, I have never seen the stride function. The docs do warn about this method however. An alternative method would be using linear algebra to do the regression on the windows. Here is a trivial example:
from numpy import *
# generate points
N = 30
x = linspace(0, 10, N)[:, None]
X = ones((N, 1)) * x
Y = X * array([1, 30]) + random.randn(*X.shape)*1e-1
XX = concatenate((ones((N,1)), X), axis = 1)
# window data
windowLength = 10
windows = array([roll(
XX, -i * windowLength, axis = 0)[:windowLength, :]
for i in range(len(XX) - windowLength)])
windowsY = array([roll(Y, -i * windowLength, axis = 0)[:windowLength, :]
for i in range(len(Y) - windowLength)])
# linear regression on windows
reg = array([
((linalg.pinv(wx.T.dot(wx))).dot(wx.T)).dot(wy) for
wx, wy in zip(windows, windowsY)])
# plot regression on windows
from matplotlib import style
style.use('seaborn-poster')
from matplotlib.pyplot import subplots, cm
fig, ax = subplots()
colors = cm.tab20(linspace(0, 1, len(windows)))
for win, color, coeffs, yi in zip(windows, colors, reg, windowsY):
ax.plot(win, yi,'.', alpha = .5, color = color)
ax.plot(win[:, 1], win.dot(coeffs), alpha = .5, color = color)
x += 1
ax.set(**dict(xlabel = 'x', ylabel = 'y'))
Which produces:
Interesting, I have never seen the stride function. The docs do warn about this method however. An alternative method would be using linear algebra to do the regression on the windows. Here is a trivial example:
from numpy import *
# generate points
N = 30
x = linspace(0, 10, N)[:, None]
X = ones((N, 1)) * x
Y = X * array([1, 30]) + random.randn(*X.shape)*1e-1
XX = concatenate((ones((N,1)), X), axis = 1)
# window data
windowLength = 10
windows = array([roll(
XX, -i * windowLength, axis = 0)[:windowLength, :]
for i in range(len(XX) - windowLength)])
windowsY = array([roll(Y, -i * windowLength, axis = 0)[:windowLength, :]
for i in range(len(Y) - windowLength)])
# linear regression on windows
reg = array([
((linalg.pinv(wx.T.dot(wx))).dot(wx.T)).dot(wy) for
wx, wy in zip(windows, windowsY)])
# plot regression on windows
from matplotlib import style
style.use('seaborn-poster')
from matplotlib.pyplot import subplots, cm
fig, ax = subplots()
colors = cm.tab20(linspace(0, 1, len(windows)))
for win, color, coeffs, yi in zip(windows, colors, reg, windowsY):
ax.plot(win, yi,'.', alpha = .5, color = color)
ax.plot(win[:, 1], win.dot(coeffs), alpha = .5, color = color)
x += 1
ax.set(**dict(xlabel = 'x', ylabel = 'y'))
Which produces:
answered Nov 25 '18 at 2:51
GlobalTravelerGlobalTraveler
57639
57639
thanks Global. I use as_strided for the speed. I have read the potential issues with using as_strided.
– John Holmes
Nov 25 '18 at 17:11
add a comment |
thanks Global. I use as_strided for the speed. I have read the potential issues with using as_strided.
– John Holmes
Nov 25 '18 at 17:11
thanks Global. I use as_strided for the speed. I have read the potential issues with using as_strided.
– John Holmes
Nov 25 '18 at 17:11
thanks Global. I use as_strided for the speed. I have read the potential issues with using as_strided.
– John Holmes
Nov 25 '18 at 17:11
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53453383%2fsliding-window-for-linear-regression-using-numpy-as-strided%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LbTXgtsCvZJzBhWJ2plfzAE,DPoXNZ h4U,n,3OebB4H0mS,a EdevZXphPW9wjIm,TGaQ33 5P,U,ZjnKBXf5RmuZV
This doesn't answer your question, but I recommend using
skimage.util.view_as_windows
for rolling windows.– Nils Werner
Nov 23 '18 at 22:21