How to call static method from a static variable inside a class?
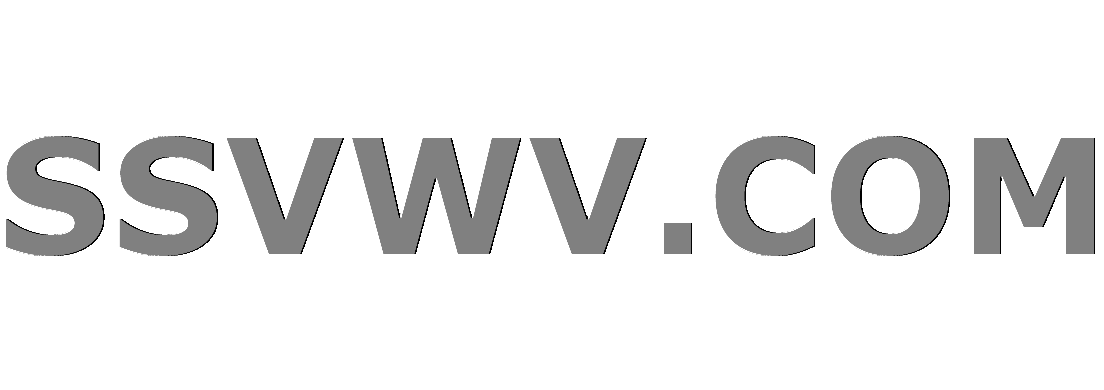
Multi tool use
I'm trying to implement a simple class with static vars and static methods:
class X(object):
@staticmethod
def do_something(par):
# do something with parameter par
#...
return something
static_var = X.do_something(5) #<-- that's how I call the function
But I have the error NameError: name 'X' is not defined
.
How to do call this static function?
python-3.x oop
|
show 9 more comments
I'm trying to implement a simple class with static vars and static methods:
class X(object):
@staticmethod
def do_something(par):
# do something with parameter par
#...
return something
static_var = X.do_something(5) #<-- that's how I call the function
But I have the error NameError: name 'X' is not defined
.
How to do call this static function?
python-3.x oop
Where do you definepar
?
– Red Cricket
Nov 23 '18 at 22:19
What are you trying to do?par
is a parameter indo_something
and you're trying to usepar
outside of that definition.
– ggorlen
Nov 23 '18 at 22:32
@RedCricket I don't know where to define it. I hope somebody can help.
– mimic
Nov 23 '18 at 22:36
@ggorlen How to define the ANS properly? I'm trying to call static function from the static variable and definitely I'm not doing properly but I don't know how to do it, that's the question.
– mimic
Nov 23 '18 at 22:38
You are not usingpar
in your method so just get rid of it.
– Red Cricket
Nov 23 '18 at 22:38
|
show 9 more comments
I'm trying to implement a simple class with static vars and static methods:
class X(object):
@staticmethod
def do_something(par):
# do something with parameter par
#...
return something
static_var = X.do_something(5) #<-- that's how I call the function
But I have the error NameError: name 'X' is not defined
.
How to do call this static function?
python-3.x oop
I'm trying to implement a simple class with static vars and static methods:
class X(object):
@staticmethod
def do_something(par):
# do something with parameter par
#...
return something
static_var = X.do_something(5) #<-- that's how I call the function
But I have the error NameError: name 'X' is not defined
.
How to do call this static function?
python-3.x oop
python-3.x oop
edited Nov 23 '18 at 23:31
ggorlen
7,1883825
7,1883825
asked Nov 23 '18 at 22:09
mimicmimic
1,22622455
1,22622455
Where do you definepar
?
– Red Cricket
Nov 23 '18 at 22:19
What are you trying to do?par
is a parameter indo_something
and you're trying to usepar
outside of that definition.
– ggorlen
Nov 23 '18 at 22:32
@RedCricket I don't know where to define it. I hope somebody can help.
– mimic
Nov 23 '18 at 22:36
@ggorlen How to define the ANS properly? I'm trying to call static function from the static variable and definitely I'm not doing properly but I don't know how to do it, that's the question.
– mimic
Nov 23 '18 at 22:38
You are not usingpar
in your method so just get rid of it.
– Red Cricket
Nov 23 '18 at 22:38
|
show 9 more comments
Where do you definepar
?
– Red Cricket
Nov 23 '18 at 22:19
What are you trying to do?par
is a parameter indo_something
and you're trying to usepar
outside of that definition.
– ggorlen
Nov 23 '18 at 22:32
@RedCricket I don't know where to define it. I hope somebody can help.
– mimic
Nov 23 '18 at 22:36
@ggorlen How to define the ANS properly? I'm trying to call static function from the static variable and definitely I'm not doing properly but I don't know how to do it, that's the question.
– mimic
Nov 23 '18 at 22:38
You are not usingpar
in your method so just get rid of it.
– Red Cricket
Nov 23 '18 at 22:38
Where do you define
par
?– Red Cricket
Nov 23 '18 at 22:19
Where do you define
par
?– Red Cricket
Nov 23 '18 at 22:19
What are you trying to do?
par
is a parameter in do_something
and you're trying to use par
outside of that definition.– ggorlen
Nov 23 '18 at 22:32
What are you trying to do?
par
is a parameter in do_something
and you're trying to use par
outside of that definition.– ggorlen
Nov 23 '18 at 22:32
@RedCricket I don't know where to define it. I hope somebody can help.
– mimic
Nov 23 '18 at 22:36
@RedCricket I don't know where to define it. I hope somebody can help.
– mimic
Nov 23 '18 at 22:36
@ggorlen How to define the ANS properly? I'm trying to call static function from the static variable and definitely I'm not doing properly but I don't know how to do it, that's the question.
– mimic
Nov 23 '18 at 22:38
@ggorlen How to define the ANS properly? I'm trying to call static function from the static variable and definitely I'm not doing properly but I don't know how to do it, that's the question.
– mimic
Nov 23 '18 at 22:38
You are not using
par
in your method so just get rid of it.– Red Cricket
Nov 23 '18 at 22:38
You are not using
par
in your method so just get rid of it.– Red Cricket
Nov 23 '18 at 22:38
|
show 9 more comments
1 Answer
1
active
oldest
votes
It looks like you'd like to initialize the value of a static class variable using a static function from that same class as it's being defined. You can do this using the following syntax taken from this answer but with an added parameter:
class X:
@staticmethod
def do_something(par):
return par
static_var = do_something.__func__(5)
print(X.static_var)
Output:
5
Try it!
Referencing a static method of the class X
directly inside the X
definition fails because X
doesn't yet exist. However, since you have defined the @staticmethod do_something
, you can call its __func__
attribute with the parameter and assign the result to static_var
.
Having said that, more information about the underlying design goal you're trying to implement could reveal a better approach.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53453406%2fhow-to-call-static-method-from-a-static-variable-inside-a-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
It looks like you'd like to initialize the value of a static class variable using a static function from that same class as it's being defined. You can do this using the following syntax taken from this answer but with an added parameter:
class X:
@staticmethod
def do_something(par):
return par
static_var = do_something.__func__(5)
print(X.static_var)
Output:
5
Try it!
Referencing a static method of the class X
directly inside the X
definition fails because X
doesn't yet exist. However, since you have defined the @staticmethod do_something
, you can call its __func__
attribute with the parameter and assign the result to static_var
.
Having said that, more information about the underlying design goal you're trying to implement could reveal a better approach.
add a comment |
It looks like you'd like to initialize the value of a static class variable using a static function from that same class as it's being defined. You can do this using the following syntax taken from this answer but with an added parameter:
class X:
@staticmethod
def do_something(par):
return par
static_var = do_something.__func__(5)
print(X.static_var)
Output:
5
Try it!
Referencing a static method of the class X
directly inside the X
definition fails because X
doesn't yet exist. However, since you have defined the @staticmethod do_something
, you can call its __func__
attribute with the parameter and assign the result to static_var
.
Having said that, more information about the underlying design goal you're trying to implement could reveal a better approach.
add a comment |
It looks like you'd like to initialize the value of a static class variable using a static function from that same class as it's being defined. You can do this using the following syntax taken from this answer but with an added parameter:
class X:
@staticmethod
def do_something(par):
return par
static_var = do_something.__func__(5)
print(X.static_var)
Output:
5
Try it!
Referencing a static method of the class X
directly inside the X
definition fails because X
doesn't yet exist. However, since you have defined the @staticmethod do_something
, you can call its __func__
attribute with the parameter and assign the result to static_var
.
Having said that, more information about the underlying design goal you're trying to implement could reveal a better approach.
It looks like you'd like to initialize the value of a static class variable using a static function from that same class as it's being defined. You can do this using the following syntax taken from this answer but with an added parameter:
class X:
@staticmethod
def do_something(par):
return par
static_var = do_something.__func__(5)
print(X.static_var)
Output:
5
Try it!
Referencing a static method of the class X
directly inside the X
definition fails because X
doesn't yet exist. However, since you have defined the @staticmethod do_something
, you can call its __func__
attribute with the parameter and assign the result to static_var
.
Having said that, more information about the underlying design goal you're trying to implement could reveal a better approach.
answered Nov 23 '18 at 23:20
ggorlenggorlen
7,1883825
7,1883825
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53453406%2fhow-to-call-static-method-from-a-static-variable-inside-a-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PSn,d5k8BaOo,kFzZ 45X t64 6KVXM
Where do you define
par
?– Red Cricket
Nov 23 '18 at 22:19
What are you trying to do?
par
is a parameter indo_something
and you're trying to usepar
outside of that definition.– ggorlen
Nov 23 '18 at 22:32
@RedCricket I don't know where to define it. I hope somebody can help.
– mimic
Nov 23 '18 at 22:36
@ggorlen How to define the ANS properly? I'm trying to call static function from the static variable and definitely I'm not doing properly but I don't know how to do it, that's the question.
– mimic
Nov 23 '18 at 22:38
You are not using
par
in your method so just get rid of it.– Red Cricket
Nov 23 '18 at 22:38