Simple countdown programs to print “3, 2, 1, Action!” using while loop
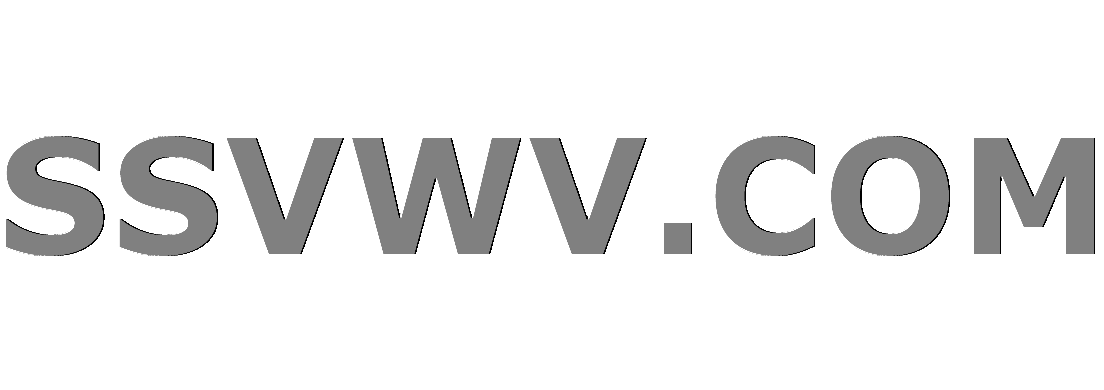
Multi tool use
$begingroup$
My program is a simple while loop making a countdown starting from 3 and shouts "Action!" upon reaching zero and then breaks. I would like to know which of the following ways is better in means of clarity and functionality. I am aware that there are other ways to achieve the same thing. I would like to hear your thoughts and suggestions.
countDown = 3
while (countDown >= 0):
print(countDown)
countDown = countDown - 1
if countDown == 0:
print("Action!")
break
or
countDown = 3
while (countDown >= 0):
if countDown != 0:
print(countDown)
countDown = countDown - 1
else:
print("Action!")
break
python python-3.x comparative-review
$endgroup$
add a comment |
$begingroup$
My program is a simple while loop making a countdown starting from 3 and shouts "Action!" upon reaching zero and then breaks. I would like to know which of the following ways is better in means of clarity and functionality. I am aware that there are other ways to achieve the same thing. I would like to hear your thoughts and suggestions.
countDown = 3
while (countDown >= 0):
print(countDown)
countDown = countDown - 1
if countDown == 0:
print("Action!")
break
or
countDown = 3
while (countDown >= 0):
if countDown != 0:
print(countDown)
countDown = countDown - 1
else:
print("Action!")
break
python python-3.x comparative-review
$endgroup$
$begingroup$
Are you saying it has to be a while loop?
$endgroup$
– dangee1705
Oct 3 '17 at 15:28
add a comment |
$begingroup$
My program is a simple while loop making a countdown starting from 3 and shouts "Action!" upon reaching zero and then breaks. I would like to know which of the following ways is better in means of clarity and functionality. I am aware that there are other ways to achieve the same thing. I would like to hear your thoughts and suggestions.
countDown = 3
while (countDown >= 0):
print(countDown)
countDown = countDown - 1
if countDown == 0:
print("Action!")
break
or
countDown = 3
while (countDown >= 0):
if countDown != 0:
print(countDown)
countDown = countDown - 1
else:
print("Action!")
break
python python-3.x comparative-review
$endgroup$
My program is a simple while loop making a countdown starting from 3 and shouts "Action!" upon reaching zero and then breaks. I would like to know which of the following ways is better in means of clarity and functionality. I am aware that there are other ways to achieve the same thing. I would like to hear your thoughts and suggestions.
countDown = 3
while (countDown >= 0):
print(countDown)
countDown = countDown - 1
if countDown == 0:
print("Action!")
break
or
countDown = 3
while (countDown >= 0):
if countDown != 0:
print(countDown)
countDown = countDown - 1
else:
print("Action!")
break
python python-3.x comparative-review
python python-3.x comparative-review
edited Oct 2 '17 at 22:22


200_success
129k15153416
129k15153416
asked Oct 2 '17 at 8:23
U.MarvinU.Marvin
41112
41112
$begingroup$
Are you saying it has to be a while loop?
$endgroup$
– dangee1705
Oct 3 '17 at 15:28
add a comment |
$begingroup$
Are you saying it has to be a while loop?
$endgroup$
– dangee1705
Oct 3 '17 at 15:28
$begingroup$
Are you saying it has to be a while loop?
$endgroup$
– dangee1705
Oct 3 '17 at 15:28
$begingroup$
Are you saying it has to be a while loop?
$endgroup$
– dangee1705
Oct 3 '17 at 15:28
add a comment |
5 Answers
5
active
oldest
votes
$begingroup$
Why does it has to be a while
?
Maybe it is preference but for
loops look cleaner.
Reversing can be done by using reversed
function which reverses a iteration
countdown = 3
for count in reversed(range(1, countdown+1)):
print(count)
print('action!')
Or you could use the step
parameter in range(start, end, step)
and rewrite the for loop to
for count in range(countdown, 0, -1):
$endgroup$
$begingroup$
Very good point, howeverlist(range(1, 3, -1)) ==
.reversed(range(1, countdown + 1))
may be easier to understand, and reason with.
$endgroup$
– Peilonrayz
Oct 2 '17 at 9:34
10
$begingroup$
IMO,range(countdown, 0, -1)
looks cleaner and more idiomatic thanreversed(range(1, countdown+1))
. Opinions may vary, of course.
$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:45
add a comment |
$begingroup$
You can get rid of some instructions by writing this instead:
count_down = 3
while (count_down):
print(count_down)
count_down -= 1
print('Action!')
Note that I have replaced countDown
by count_down
to comply with PEP8' naming conventions.
Code explanation:
count_down -= 1
is equivalent to count_down = count_down - 1
. You can read more on Python basic operators.
You do not need to check within the while loop if count_down
reached the 0 value because it is already done when you coded while (countDown>=0)
. I mean you are duplicating the checking. In order to keep DRY, I just decrement the value of count_down
by 1 and the break
instruction is done by default as I am testing while(count_down)
meaning if count_down != 0
in this context (because it also means while count_down
is not False
or None
).
$endgroup$
10
$begingroup$
Why the parentheses inwhile (count_down):
? And while it's somewhat a matter of taste, I'd argue thatwhile count_down > 0:
would express the implied intent better in this case. (In particular, it ensures that the loop will still terminate even if someone changes the initial value ofcount_down
to, say, 3.5 or -1.)
$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:40
add a comment |
$begingroup$
Why not try recursion? Clean and simple.
num = 10
def countdown(num):
if num == 0:
print("Action!")
return
print(num)
countdown(num-1)
countdown(num)
$endgroup$
add a comment |
$begingroup$
I think your first example is better in terms of clarity, although you could replace while (countDown >= 0):
with while (countDown > 0):
, allowing you to remove your break statement.
Ex:
countDown = 3
while (countDown > 0):
print(countDown)
countDown = countDown - 1
if countDown == 0:
print("Action!")
$endgroup$
add a comment |
$begingroup$
This is how a counter should be...
import time
def countdown(n):
while n > 0:
print(n)
n = n - 1
if n == 0:
print('Times up')
countdown(50)
New contributor
jerald king is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
You importtime
but don't even use it in your answer. Also, this will never work, since the if statement is inside the loop, whenn = 0
, the loop will be skipped and will just end without printingTimes up
$endgroup$
– David White
2 mins ago
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f176965%2fsimple-countdown-programs-to-print-3-2-1-action-using-while-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Why does it has to be a while
?
Maybe it is preference but for
loops look cleaner.
Reversing can be done by using reversed
function which reverses a iteration
countdown = 3
for count in reversed(range(1, countdown+1)):
print(count)
print('action!')
Or you could use the step
parameter in range(start, end, step)
and rewrite the for loop to
for count in range(countdown, 0, -1):
$endgroup$
$begingroup$
Very good point, howeverlist(range(1, 3, -1)) ==
.reversed(range(1, countdown + 1))
may be easier to understand, and reason with.
$endgroup$
– Peilonrayz
Oct 2 '17 at 9:34
10
$begingroup$
IMO,range(countdown, 0, -1)
looks cleaner and more idiomatic thanreversed(range(1, countdown+1))
. Opinions may vary, of course.
$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:45
add a comment |
$begingroup$
Why does it has to be a while
?
Maybe it is preference but for
loops look cleaner.
Reversing can be done by using reversed
function which reverses a iteration
countdown = 3
for count in reversed(range(1, countdown+1)):
print(count)
print('action!')
Or you could use the step
parameter in range(start, end, step)
and rewrite the for loop to
for count in range(countdown, 0, -1):
$endgroup$
$begingroup$
Very good point, howeverlist(range(1, 3, -1)) ==
.reversed(range(1, countdown + 1))
may be easier to understand, and reason with.
$endgroup$
– Peilonrayz
Oct 2 '17 at 9:34
10
$begingroup$
IMO,range(countdown, 0, -1)
looks cleaner and more idiomatic thanreversed(range(1, countdown+1))
. Opinions may vary, of course.
$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:45
add a comment |
$begingroup$
Why does it has to be a while
?
Maybe it is preference but for
loops look cleaner.
Reversing can be done by using reversed
function which reverses a iteration
countdown = 3
for count in reversed(range(1, countdown+1)):
print(count)
print('action!')
Or you could use the step
parameter in range(start, end, step)
and rewrite the for loop to
for count in range(countdown, 0, -1):
$endgroup$
Why does it has to be a while
?
Maybe it is preference but for
loops look cleaner.
Reversing can be done by using reversed
function which reverses a iteration
countdown = 3
for count in reversed(range(1, countdown+1)):
print(count)
print('action!')
Or you could use the step
parameter in range(start, end, step)
and rewrite the for loop to
for count in range(countdown, 0, -1):
edited Oct 3 '17 at 9:04
answered Oct 2 '17 at 9:29


LudisposedLudisposed
7,96222060
7,96222060
$begingroup$
Very good point, howeverlist(range(1, 3, -1)) ==
.reversed(range(1, countdown + 1))
may be easier to understand, and reason with.
$endgroup$
– Peilonrayz
Oct 2 '17 at 9:34
10
$begingroup$
IMO,range(countdown, 0, -1)
looks cleaner and more idiomatic thanreversed(range(1, countdown+1))
. Opinions may vary, of course.
$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:45
add a comment |
$begingroup$
Very good point, howeverlist(range(1, 3, -1)) ==
.reversed(range(1, countdown + 1))
may be easier to understand, and reason with.
$endgroup$
– Peilonrayz
Oct 2 '17 at 9:34
10
$begingroup$
IMO,range(countdown, 0, -1)
looks cleaner and more idiomatic thanreversed(range(1, countdown+1))
. Opinions may vary, of course.
$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:45
$begingroup$
Very good point, however
list(range(1, 3, -1)) ==
. reversed(range(1, countdown + 1))
may be easier to understand, and reason with.$endgroup$
– Peilonrayz
Oct 2 '17 at 9:34
$begingroup$
Very good point, however
list(range(1, 3, -1)) ==
. reversed(range(1, countdown + 1))
may be easier to understand, and reason with.$endgroup$
– Peilonrayz
Oct 2 '17 at 9:34
10
10
$begingroup$
IMO,
range(countdown, 0, -1)
looks cleaner and more idiomatic than reversed(range(1, countdown+1))
. Opinions may vary, of course.$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:45
$begingroup$
IMO,
range(countdown, 0, -1)
looks cleaner and more idiomatic than reversed(range(1, countdown+1))
. Opinions may vary, of course.$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:45
add a comment |
$begingroup$
You can get rid of some instructions by writing this instead:
count_down = 3
while (count_down):
print(count_down)
count_down -= 1
print('Action!')
Note that I have replaced countDown
by count_down
to comply with PEP8' naming conventions.
Code explanation:
count_down -= 1
is equivalent to count_down = count_down - 1
. You can read more on Python basic operators.
You do not need to check within the while loop if count_down
reached the 0 value because it is already done when you coded while (countDown>=0)
. I mean you are duplicating the checking. In order to keep DRY, I just decrement the value of count_down
by 1 and the break
instruction is done by default as I am testing while(count_down)
meaning if count_down != 0
in this context (because it also means while count_down
is not False
or None
).
$endgroup$
10
$begingroup$
Why the parentheses inwhile (count_down):
? And while it's somewhat a matter of taste, I'd argue thatwhile count_down > 0:
would express the implied intent better in this case. (In particular, it ensures that the loop will still terminate even if someone changes the initial value ofcount_down
to, say, 3.5 or -1.)
$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:40
add a comment |
$begingroup$
You can get rid of some instructions by writing this instead:
count_down = 3
while (count_down):
print(count_down)
count_down -= 1
print('Action!')
Note that I have replaced countDown
by count_down
to comply with PEP8' naming conventions.
Code explanation:
count_down -= 1
is equivalent to count_down = count_down - 1
. You can read more on Python basic operators.
You do not need to check within the while loop if count_down
reached the 0 value because it is already done when you coded while (countDown>=0)
. I mean you are duplicating the checking. In order to keep DRY, I just decrement the value of count_down
by 1 and the break
instruction is done by default as I am testing while(count_down)
meaning if count_down != 0
in this context (because it also means while count_down
is not False
or None
).
$endgroup$
10
$begingroup$
Why the parentheses inwhile (count_down):
? And while it's somewhat a matter of taste, I'd argue thatwhile count_down > 0:
would express the implied intent better in this case. (In particular, it ensures that the loop will still terminate even if someone changes the initial value ofcount_down
to, say, 3.5 or -1.)
$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:40
add a comment |
$begingroup$
You can get rid of some instructions by writing this instead:
count_down = 3
while (count_down):
print(count_down)
count_down -= 1
print('Action!')
Note that I have replaced countDown
by count_down
to comply with PEP8' naming conventions.
Code explanation:
count_down -= 1
is equivalent to count_down = count_down - 1
. You can read more on Python basic operators.
You do not need to check within the while loop if count_down
reached the 0 value because it is already done when you coded while (countDown>=0)
. I mean you are duplicating the checking. In order to keep DRY, I just decrement the value of count_down
by 1 and the break
instruction is done by default as I am testing while(count_down)
meaning if count_down != 0
in this context (because it also means while count_down
is not False
or None
).
$endgroup$
You can get rid of some instructions by writing this instead:
count_down = 3
while (count_down):
print(count_down)
count_down -= 1
print('Action!')
Note that I have replaced countDown
by count_down
to comply with PEP8' naming conventions.
Code explanation:
count_down -= 1
is equivalent to count_down = count_down - 1
. You can read more on Python basic operators.
You do not need to check within the while loop if count_down
reached the 0 value because it is already done when you coded while (countDown>=0)
. I mean you are duplicating the checking. In order to keep DRY, I just decrement the value of count_down
by 1 and the break
instruction is done by default as I am testing while(count_down)
meaning if count_down != 0
in this context (because it also means while count_down
is not False
or None
).
edited Oct 2 '17 at 8:57
answered Oct 2 '17 at 8:34


Billal BegueradjBillal Begueradj
1
1
10
$begingroup$
Why the parentheses inwhile (count_down):
? And while it's somewhat a matter of taste, I'd argue thatwhile count_down > 0:
would express the implied intent better in this case. (In particular, it ensures that the loop will still terminate even if someone changes the initial value ofcount_down
to, say, 3.5 or -1.)
$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:40
add a comment |
10
$begingroup$
Why the parentheses inwhile (count_down):
? And while it's somewhat a matter of taste, I'd argue thatwhile count_down > 0:
would express the implied intent better in this case. (In particular, it ensures that the loop will still terminate even if someone changes the initial value ofcount_down
to, say, 3.5 or -1.)
$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:40
10
10
$begingroup$
Why the parentheses in
while (count_down):
? And while it's somewhat a matter of taste, I'd argue that while count_down > 0:
would express the implied intent better in this case. (In particular, it ensures that the loop will still terminate even if someone changes the initial value of count_down
to, say, 3.5 or -1.)$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:40
$begingroup$
Why the parentheses in
while (count_down):
? And while it's somewhat a matter of taste, I'd argue that while count_down > 0:
would express the implied intent better in this case. (In particular, it ensures that the loop will still terminate even if someone changes the initial value of count_down
to, say, 3.5 or -1.)$endgroup$
– Ilmari Karonen
Oct 2 '17 at 10:40
add a comment |
$begingroup$
Why not try recursion? Clean and simple.
num = 10
def countdown(num):
if num == 0:
print("Action!")
return
print(num)
countdown(num-1)
countdown(num)
$endgroup$
add a comment |
$begingroup$
Why not try recursion? Clean and simple.
num = 10
def countdown(num):
if num == 0:
print("Action!")
return
print(num)
countdown(num-1)
countdown(num)
$endgroup$
add a comment |
$begingroup$
Why not try recursion? Clean and simple.
num = 10
def countdown(num):
if num == 0:
print("Action!")
return
print(num)
countdown(num-1)
countdown(num)
$endgroup$
Why not try recursion? Clean and simple.
num = 10
def countdown(num):
if num == 0:
print("Action!")
return
print(num)
countdown(num-1)
countdown(num)
answered Oct 3 '17 at 13:24
amin.avanamin.avan
211
211
add a comment |
add a comment |
$begingroup$
I think your first example is better in terms of clarity, although you could replace while (countDown >= 0):
with while (countDown > 0):
, allowing you to remove your break statement.
Ex:
countDown = 3
while (countDown > 0):
print(countDown)
countDown = countDown - 1
if countDown == 0:
print("Action!")
$endgroup$
add a comment |
$begingroup$
I think your first example is better in terms of clarity, although you could replace while (countDown >= 0):
with while (countDown > 0):
, allowing you to remove your break statement.
Ex:
countDown = 3
while (countDown > 0):
print(countDown)
countDown = countDown - 1
if countDown == 0:
print("Action!")
$endgroup$
add a comment |
$begingroup$
I think your first example is better in terms of clarity, although you could replace while (countDown >= 0):
with while (countDown > 0):
, allowing you to remove your break statement.
Ex:
countDown = 3
while (countDown > 0):
print(countDown)
countDown = countDown - 1
if countDown == 0:
print("Action!")
$endgroup$
I think your first example is better in terms of clarity, although you could replace while (countDown >= 0):
with while (countDown > 0):
, allowing you to remove your break statement.
Ex:
countDown = 3
while (countDown > 0):
print(countDown)
countDown = countDown - 1
if countDown == 0:
print("Action!")
answered Oct 4 '17 at 2:40
ConfettimakerConfettimaker
145212
145212
add a comment |
add a comment |
$begingroup$
This is how a counter should be...
import time
def countdown(n):
while n > 0:
print(n)
n = n - 1
if n == 0:
print('Times up')
countdown(50)
New contributor
jerald king is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
You importtime
but don't even use it in your answer. Also, this will never work, since the if statement is inside the loop, whenn = 0
, the loop will be skipped and will just end without printingTimes up
$endgroup$
– David White
2 mins ago
add a comment |
$begingroup$
This is how a counter should be...
import time
def countdown(n):
while n > 0:
print(n)
n = n - 1
if n == 0:
print('Times up')
countdown(50)
New contributor
jerald king is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
You importtime
but don't even use it in your answer. Also, this will never work, since the if statement is inside the loop, whenn = 0
, the loop will be skipped and will just end without printingTimes up
$endgroup$
– David White
2 mins ago
add a comment |
$begingroup$
This is how a counter should be...
import time
def countdown(n):
while n > 0:
print(n)
n = n - 1
if n == 0:
print('Times up')
countdown(50)
New contributor
jerald king is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
This is how a counter should be...
import time
def countdown(n):
while n > 0:
print(n)
n = n - 1
if n == 0:
print('Times up')
countdown(50)
New contributor
jerald king is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
jerald king is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 13 mins ago


jerald kingjerald king
1
1
New contributor
jerald king is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
jerald king is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
jerald king is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$begingroup$
You importtime
but don't even use it in your answer. Also, this will never work, since the if statement is inside the loop, whenn = 0
, the loop will be skipped and will just end without printingTimes up
$endgroup$
– David White
2 mins ago
add a comment |
$begingroup$
You importtime
but don't even use it in your answer. Also, this will never work, since the if statement is inside the loop, whenn = 0
, the loop will be skipped and will just end without printingTimes up
$endgroup$
– David White
2 mins ago
$begingroup$
You import
time
but don't even use it in your answer. Also, this will never work, since the if statement is inside the loop, when n = 0
, the loop will be skipped and will just end without printing Times up
$endgroup$
– David White
2 mins ago
$begingroup$
You import
time
but don't even use it in your answer. Also, this will never work, since the if statement is inside the loop, when n = 0
, the loop will be skipped and will just end without printing Times up
$endgroup$
– David White
2 mins ago
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f176965%2fsimple-countdown-programs-to-print-3-2-1-action-using-while-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0a9Ypq jTU5QJ,gzE30i7
$begingroup$
Are you saying it has to be a while loop?
$endgroup$
– dangee1705
Oct 3 '17 at 15:28