C++ Multinomial distribution
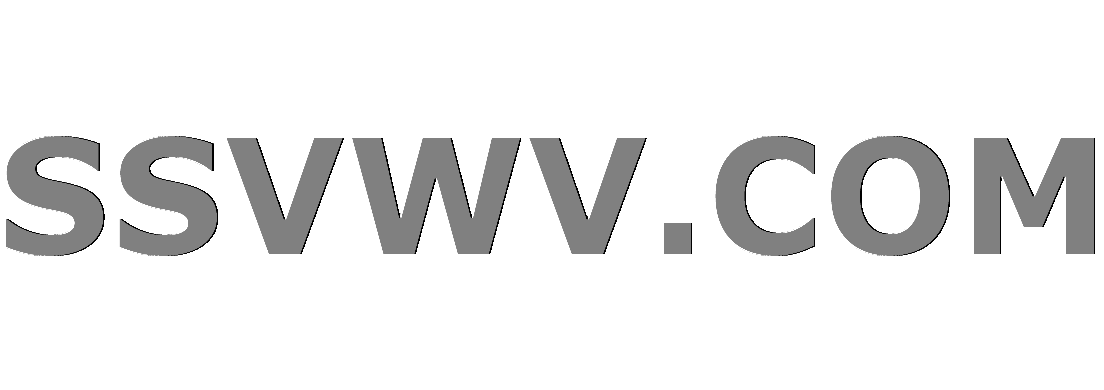
Multi tool use
I'm trying to code a multinomial algorithm that will basically apply a binomial distribution to each value of an input vector, knowing the values of all previous ones. It's aimed here to generate a new population for multiple alleles knowing the initial population.
To achieve this, I'm using this recursive algorithm :
This is what my code looks like right now :
void RandomNumbers::multinomial(std::vector<unsigned int>& alleleNumbers) {
/* In this function we need two different records of the size.
* We need the size from the old populations, ( N - n1 - ... - nA )
* and we also need the size from the newly created population,
* ( N - k1 - ... - kA ).
* In order to achieve such a task, we'll use the integer "temp" to store
* the value n1 before modifying it to k1 and so on.
*
*
*/
double totalSize = 0;
for(auto n : alleleNumbers) totalSize+=n;
double newTotalSize(totalSize);
std::cout<< newTotalSize;
for(size_t i = 0; i < alleleNumbers.size(); ++i){
size_t temp = alleleNumbers[i];
alleleNumbers[i] = binomial(newTotalSize,
(alleleNumbers[i])/(totalSize));
newTotalSize-= alleleNumbers[i];
totalSize = temp;
}
}
But I'm not sure at all about this, and I was wondering if there was an already existing multinomial algorithm of that kind...
Thank you very much.
c++ algorithm recursion probability multinomial
add a comment |
I'm trying to code a multinomial algorithm that will basically apply a binomial distribution to each value of an input vector, knowing the values of all previous ones. It's aimed here to generate a new population for multiple alleles knowing the initial population.
To achieve this, I'm using this recursive algorithm :
This is what my code looks like right now :
void RandomNumbers::multinomial(std::vector<unsigned int>& alleleNumbers) {
/* In this function we need two different records of the size.
* We need the size from the old populations, ( N - n1 - ... - nA )
* and we also need the size from the newly created population,
* ( N - k1 - ... - kA ).
* In order to achieve such a task, we'll use the integer "temp" to store
* the value n1 before modifying it to k1 and so on.
*
*
*/
double totalSize = 0;
for(auto n : alleleNumbers) totalSize+=n;
double newTotalSize(totalSize);
std::cout<< newTotalSize;
for(size_t i = 0; i < alleleNumbers.size(); ++i){
size_t temp = alleleNumbers[i];
alleleNumbers[i] = binomial(newTotalSize,
(alleleNumbers[i])/(totalSize));
newTotalSize-= alleleNumbers[i];
totalSize = temp;
}
}
But I'm not sure at all about this, and I was wondering if there was an already existing multinomial algorithm of that kind...
Thank you very much.
c++ algorithm recursion probability multinomial
There is no direct implementation of this in the standard library and since questions suggesting a library are off-topic here, have a look e.g. here: scicomp.stackexchange.com/questions/382/…
– user10605163
Nov 24 '18 at 16:15
There is std::discrete_distribution.
– eozd
Nov 24 '18 at 16:16
add a comment |
I'm trying to code a multinomial algorithm that will basically apply a binomial distribution to each value of an input vector, knowing the values of all previous ones. It's aimed here to generate a new population for multiple alleles knowing the initial population.
To achieve this, I'm using this recursive algorithm :
This is what my code looks like right now :
void RandomNumbers::multinomial(std::vector<unsigned int>& alleleNumbers) {
/* In this function we need two different records of the size.
* We need the size from the old populations, ( N - n1 - ... - nA )
* and we also need the size from the newly created population,
* ( N - k1 - ... - kA ).
* In order to achieve such a task, we'll use the integer "temp" to store
* the value n1 before modifying it to k1 and so on.
*
*
*/
double totalSize = 0;
for(auto n : alleleNumbers) totalSize+=n;
double newTotalSize(totalSize);
std::cout<< newTotalSize;
for(size_t i = 0; i < alleleNumbers.size(); ++i){
size_t temp = alleleNumbers[i];
alleleNumbers[i] = binomial(newTotalSize,
(alleleNumbers[i])/(totalSize));
newTotalSize-= alleleNumbers[i];
totalSize = temp;
}
}
But I'm not sure at all about this, and I was wondering if there was an already existing multinomial algorithm of that kind...
Thank you very much.
c++ algorithm recursion probability multinomial
I'm trying to code a multinomial algorithm that will basically apply a binomial distribution to each value of an input vector, knowing the values of all previous ones. It's aimed here to generate a new population for multiple alleles knowing the initial population.
To achieve this, I'm using this recursive algorithm :
This is what my code looks like right now :
void RandomNumbers::multinomial(std::vector<unsigned int>& alleleNumbers) {
/* In this function we need two different records of the size.
* We need the size from the old populations, ( N - n1 - ... - nA )
* and we also need the size from the newly created population,
* ( N - k1 - ... - kA ).
* In order to achieve such a task, we'll use the integer "temp" to store
* the value n1 before modifying it to k1 and so on.
*
*
*/
double totalSize = 0;
for(auto n : alleleNumbers) totalSize+=n;
double newTotalSize(totalSize);
std::cout<< newTotalSize;
for(size_t i = 0; i < alleleNumbers.size(); ++i){
size_t temp = alleleNumbers[i];
alleleNumbers[i] = binomial(newTotalSize,
(alleleNumbers[i])/(totalSize));
newTotalSize-= alleleNumbers[i];
totalSize = temp;
}
}
But I'm not sure at all about this, and I was wondering if there was an already existing multinomial algorithm of that kind...
Thank you very much.
c++ algorithm recursion probability multinomial
c++ algorithm recursion probability multinomial
edited Nov 24 '18 at 17:47


eozd
9041515
9041515
asked Nov 24 '18 at 16:09


Camil HamdaneCamil Hamdane
111
111
There is no direct implementation of this in the standard library and since questions suggesting a library are off-topic here, have a look e.g. here: scicomp.stackexchange.com/questions/382/…
– user10605163
Nov 24 '18 at 16:15
There is std::discrete_distribution.
– eozd
Nov 24 '18 at 16:16
add a comment |
There is no direct implementation of this in the standard library and since questions suggesting a library are off-topic here, have a look e.g. here: scicomp.stackexchange.com/questions/382/…
– user10605163
Nov 24 '18 at 16:15
There is std::discrete_distribution.
– eozd
Nov 24 '18 at 16:16
There is no direct implementation of this in the standard library and since questions suggesting a library are off-topic here, have a look e.g. here: scicomp.stackexchange.com/questions/382/…
– user10605163
Nov 24 '18 at 16:15
There is no direct implementation of this in the standard library and since questions suggesting a library are off-topic here, have a look e.g. here: scicomp.stackexchange.com/questions/382/…
– user10605163
Nov 24 '18 at 16:15
There is std::discrete_distribution.
– eozd
Nov 24 '18 at 16:16
There is std::discrete_distribution.
– eozd
Nov 24 '18 at 16:16
add a comment |
1 Answer
1
active
oldest
votes
You could try using the GNU Scientific Library's gsl_ran_multinomial
command.
The function is called as:
gsl_ran_multinomial (const gsl_rng * r, size_t K, unsigned int N, const double p, unsigned int n)
where (n_1, n_2, ..., n_K)
are nonnegative integers with sum_{k=1}^K n_k = N, and (p_1, p_2, ..., p_K)
is a probability distribution with sum(p_i) = 1
. If the array p[K]
is not normalized then its entries will be treated as weights and normalized appropriately. The arrays n
and p
must both be of length K
.
The function implements the conditional binomial method from C.S. Davis's "The computer generation of multinomial random variates" (Comp. Stat. Data Anal. 16, 1993. link), so you could implement using that approach. Let me know if you need a copy of the paper.
Hey,Thank you for this amazing response, since then I installed GSL, however, I cannot seem to manage to compile with it, and I only get a "gsl_rng does not name a type"... I will try to fix my problem, but thanks a lot !
– Camil Hamdane
Nov 25 '18 at 15:17
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53459990%2fc-multinomial-distribution%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could try using the GNU Scientific Library's gsl_ran_multinomial
command.
The function is called as:
gsl_ran_multinomial (const gsl_rng * r, size_t K, unsigned int N, const double p, unsigned int n)
where (n_1, n_2, ..., n_K)
are nonnegative integers with sum_{k=1}^K n_k = N, and (p_1, p_2, ..., p_K)
is a probability distribution with sum(p_i) = 1
. If the array p[K]
is not normalized then its entries will be treated as weights and normalized appropriately. The arrays n
and p
must both be of length K
.
The function implements the conditional binomial method from C.S. Davis's "The computer generation of multinomial random variates" (Comp. Stat. Data Anal. 16, 1993. link), so you could implement using that approach. Let me know if you need a copy of the paper.
Hey,Thank you for this amazing response, since then I installed GSL, however, I cannot seem to manage to compile with it, and I only get a "gsl_rng does not name a type"... I will try to fix my problem, but thanks a lot !
– Camil Hamdane
Nov 25 '18 at 15:17
add a comment |
You could try using the GNU Scientific Library's gsl_ran_multinomial
command.
The function is called as:
gsl_ran_multinomial (const gsl_rng * r, size_t K, unsigned int N, const double p, unsigned int n)
where (n_1, n_2, ..., n_K)
are nonnegative integers with sum_{k=1}^K n_k = N, and (p_1, p_2, ..., p_K)
is a probability distribution with sum(p_i) = 1
. If the array p[K]
is not normalized then its entries will be treated as weights and normalized appropriately. The arrays n
and p
must both be of length K
.
The function implements the conditional binomial method from C.S. Davis's "The computer generation of multinomial random variates" (Comp. Stat. Data Anal. 16, 1993. link), so you could implement using that approach. Let me know if you need a copy of the paper.
Hey,Thank you for this amazing response, since then I installed GSL, however, I cannot seem to manage to compile with it, and I only get a "gsl_rng does not name a type"... I will try to fix my problem, but thanks a lot !
– Camil Hamdane
Nov 25 '18 at 15:17
add a comment |
You could try using the GNU Scientific Library's gsl_ran_multinomial
command.
The function is called as:
gsl_ran_multinomial (const gsl_rng * r, size_t K, unsigned int N, const double p, unsigned int n)
where (n_1, n_2, ..., n_K)
are nonnegative integers with sum_{k=1}^K n_k = N, and (p_1, p_2, ..., p_K)
is a probability distribution with sum(p_i) = 1
. If the array p[K]
is not normalized then its entries will be treated as weights and normalized appropriately. The arrays n
and p
must both be of length K
.
The function implements the conditional binomial method from C.S. Davis's "The computer generation of multinomial random variates" (Comp. Stat. Data Anal. 16, 1993. link), so you could implement using that approach. Let me know if you need a copy of the paper.
You could try using the GNU Scientific Library's gsl_ran_multinomial
command.
The function is called as:
gsl_ran_multinomial (const gsl_rng * r, size_t K, unsigned int N, const double p, unsigned int n)
where (n_1, n_2, ..., n_K)
are nonnegative integers with sum_{k=1}^K n_k = N, and (p_1, p_2, ..., p_K)
is a probability distribution with sum(p_i) = 1
. If the array p[K]
is not normalized then its entries will be treated as weights and normalized appropriately. The arrays n
and p
must both be of length K
.
The function implements the conditional binomial method from C.S. Davis's "The computer generation of multinomial random variates" (Comp. Stat. Data Anal. 16, 1993. link), so you could implement using that approach. Let me know if you need a copy of the paper.
answered Nov 24 '18 at 16:18
RichardRichard
27.2k19109167
27.2k19109167
Hey,Thank you for this amazing response, since then I installed GSL, however, I cannot seem to manage to compile with it, and I only get a "gsl_rng does not name a type"... I will try to fix my problem, but thanks a lot !
– Camil Hamdane
Nov 25 '18 at 15:17
add a comment |
Hey,Thank you for this amazing response, since then I installed GSL, however, I cannot seem to manage to compile with it, and I only get a "gsl_rng does not name a type"... I will try to fix my problem, but thanks a lot !
– Camil Hamdane
Nov 25 '18 at 15:17
Hey,Thank you for this amazing response, since then I installed GSL, however, I cannot seem to manage to compile with it, and I only get a "gsl_rng does not name a type"... I will try to fix my problem, but thanks a lot !
– Camil Hamdane
Nov 25 '18 at 15:17
Hey,Thank you for this amazing response, since then I installed GSL, however, I cannot seem to manage to compile with it, and I only get a "gsl_rng does not name a type"... I will try to fix my problem, but thanks a lot !
– Camil Hamdane
Nov 25 '18 at 15:17
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53459990%2fc-multinomial-distribution%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CouMEVCz,8DHJ,0sEqz7DHIKwnqSZnJLd,BJw7aRnx,sT8hi31xmeH,5cNnBYlL6P f,tvMjxvpSk0nQ,MjKJpv8bTqM i iCz
There is no direct implementation of this in the standard library and since questions suggesting a library are off-topic here, have a look e.g. here: scicomp.stackexchange.com/questions/382/…
– user10605163
Nov 24 '18 at 16:15
There is std::discrete_distribution.
– eozd
Nov 24 '18 at 16:16