Update Azure AD Application keys or secrets using Microsoft Graph API - BadRequest Error
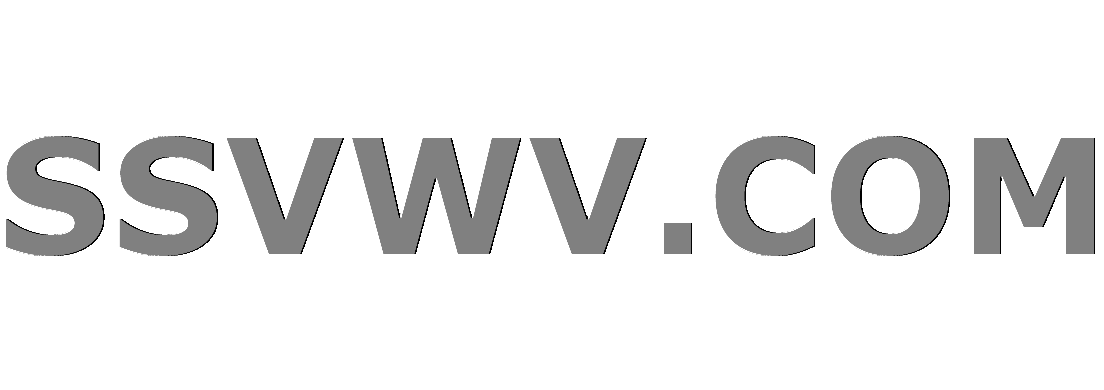
Multi tool use
up vote
1
down vote
favorite
I am trying to patch password credentials for an application using Microsoft Graph API beta endpoint for application resource type.
https://graph.microsoft.com/beta/applications/{applicationId}
The content variable is a JSON-serialized representation of something like this:
[{
"customKeyIdentifier":null,
"endDateTime":"2019-11-19T23:16:24.2602448Z",
"keyId":"47fde652-8b60-4384-b630-8e5f8f6e24b1",
"startDateTime":"2018-11-19T23:16:24.2602448Z",
"secretText":"SomeGeneratedPassword",
"hint":null
}]
Calling code is this:
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri("https://graph.microsoft.com");
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", authHeaderValue.Result.AccessToken);
client.DefaultRequestHeaders
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var method = new HttpMethod("PATCH");
var requestUri = $"https://graph.microsoft.com/beta/applications/{applicationId}";
var content = GeneratePasswordCredentials(passwordHint);
var request = new HttpRequestMessage(method, requestUri)
{
Content = new StringContent(
content,
System.Text.Encoding.UTF8,
"application/json")
};
request.Headers
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var resultApi = await client.SendAsync(request);
response = await resultApi.Content.ReadAsStringAsync();
}
Auth appears to be working fine, but the response is this (inner error removed for brevity):
{
"error": {
"code": "BadRequest",
"message": "Empty Payload. JSON content expected.",
}
}
What is wrong with the above code?


add a comment |
up vote
1
down vote
favorite
I am trying to patch password credentials for an application using Microsoft Graph API beta endpoint for application resource type.
https://graph.microsoft.com/beta/applications/{applicationId}
The content variable is a JSON-serialized representation of something like this:
[{
"customKeyIdentifier":null,
"endDateTime":"2019-11-19T23:16:24.2602448Z",
"keyId":"47fde652-8b60-4384-b630-8e5f8f6e24b1",
"startDateTime":"2018-11-19T23:16:24.2602448Z",
"secretText":"SomeGeneratedPassword",
"hint":null
}]
Calling code is this:
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri("https://graph.microsoft.com");
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", authHeaderValue.Result.AccessToken);
client.DefaultRequestHeaders
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var method = new HttpMethod("PATCH");
var requestUri = $"https://graph.microsoft.com/beta/applications/{applicationId}";
var content = GeneratePasswordCredentials(passwordHint);
var request = new HttpRequestMessage(method, requestUri)
{
Content = new StringContent(
content,
System.Text.Encoding.UTF8,
"application/json")
};
request.Headers
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var resultApi = await client.SendAsync(request);
response = await resultApi.Content.ReadAsStringAsync();
}
Auth appears to be working fine, but the response is this (inner error removed for brevity):
{
"error": {
"code": "BadRequest",
"message": "Empty Payload. JSON content expected.",
}
}
What is wrong with the above code?


add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am trying to patch password credentials for an application using Microsoft Graph API beta endpoint for application resource type.
https://graph.microsoft.com/beta/applications/{applicationId}
The content variable is a JSON-serialized representation of something like this:
[{
"customKeyIdentifier":null,
"endDateTime":"2019-11-19T23:16:24.2602448Z",
"keyId":"47fde652-8b60-4384-b630-8e5f8f6e24b1",
"startDateTime":"2018-11-19T23:16:24.2602448Z",
"secretText":"SomeGeneratedPassword",
"hint":null
}]
Calling code is this:
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri("https://graph.microsoft.com");
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", authHeaderValue.Result.AccessToken);
client.DefaultRequestHeaders
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var method = new HttpMethod("PATCH");
var requestUri = $"https://graph.microsoft.com/beta/applications/{applicationId}";
var content = GeneratePasswordCredentials(passwordHint);
var request = new HttpRequestMessage(method, requestUri)
{
Content = new StringContent(
content,
System.Text.Encoding.UTF8,
"application/json")
};
request.Headers
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var resultApi = await client.SendAsync(request);
response = await resultApi.Content.ReadAsStringAsync();
}
Auth appears to be working fine, but the response is this (inner error removed for brevity):
{
"error": {
"code": "BadRequest",
"message": "Empty Payload. JSON content expected.",
}
}
What is wrong with the above code?


I am trying to patch password credentials for an application using Microsoft Graph API beta endpoint for application resource type.
https://graph.microsoft.com/beta/applications/{applicationId}
The content variable is a JSON-serialized representation of something like this:
[{
"customKeyIdentifier":null,
"endDateTime":"2019-11-19T23:16:24.2602448Z",
"keyId":"47fde652-8b60-4384-b630-8e5f8f6e24b1",
"startDateTime":"2018-11-19T23:16:24.2602448Z",
"secretText":"SomeGeneratedPassword",
"hint":null
}]
Calling code is this:
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri("https://graph.microsoft.com");
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", authHeaderValue.Result.AccessToken);
client.DefaultRequestHeaders
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var method = new HttpMethod("PATCH");
var requestUri = $"https://graph.microsoft.com/beta/applications/{applicationId}";
var content = GeneratePasswordCredentials(passwordHint);
var request = new HttpRequestMessage(method, requestUri)
{
Content = new StringContent(
content,
System.Text.Encoding.UTF8,
"application/json")
};
request.Headers
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var resultApi = await client.SendAsync(request);
response = await resultApi.Content.ReadAsStringAsync();
}
Auth appears to be working fine, but the response is this (inner error removed for brevity):
{
"error": {
"code": "BadRequest",
"message": "Empty Payload. JSON content expected.",
}
}
What is wrong with the above code?




edited Nov 20 at 3:56


Rohit Saigal
2,4722216
2,4722216
asked Nov 19 at 23:36
William Verner
61
61
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
The body content format should be
{
"passwordCredentials":
[
{"customKeyIdentifier":"YWJjZA==",
"startDateTime":"2018-11-20T02:37:07.3963006Z",
"endDateTime":"2019-11-20T02:37:07.3963006Z",
"secretText":"The passwords must be 16-64 characters in length",
"keyId":"aeda515d-dc58-4ce6-a452-3bc3d84f58a3",
"hint":"xxx"}
]
}
The following the demo code to Generate PasswordCredentials body content
public static string GeneratePasswordCredentials(string passwordHint)
{
var passwordCredential = new JObject
{
new JProperty("customKeyIdentifier",Encoding.UTF8.GetBytes(passwordHint)),
new JProperty("startDateTime",DateTime.UtcNow),
new JProperty("endDateTime", DateTime.UtcNow.AddYears(1)),
new JProperty("secretText", "The passwords must be 16-64 characters in length"),
new JProperty("keyId", Guid.NewGuid().ToString()),
new JProperty("hint", passwordHint)
};
JArray jArray = new JArray
{
passwordCredential
};
var jsonObject = new JObject
{
new JProperty("passwordCredentials",jArray)
};
var json = JsonConvert.SerializeObject(jsonObject);
return json;
}
Note: The request url should be $"https://graph.microsoft.com/beta/applications/{ApplicationObjectId}"
add a comment |
up vote
0
down vote
The issue is with JSON string specified for Update Application Microsoft Graph API. It's missing which property you're trying to update for the application. I've added "passwordCredentials" property and given it the JSON as a collection. See jsonContent variable at the very beginning of my code.
/*Only change here from original JSON is to add the passwordCredentials node*/
{
"passwordCredentials":[
{
"customKeyIdentifier": null,
"endDateTime": "2019-11-19T23:16:24.2602448Z",
"keyId": "47fde652-8b60-4384-b630-8e5f8f6e24b1",
"startDateTime": "2018-11-19T23:16:24.2602448Z",
"secretText": "SomeGeneratedPassword",
"hint": null
}
]
}
I started with your code and the 400 bad response error reproduced for me as well.
Below is the final working code and now I get back a 204 response status. I can also see the new key added to Application keys collection from Azure Portal > App Registrations > My app > Settings > Keys
string jsonContent = "{"passwordCredentials":[{"customKeyIdentifier":null,"endDateTime":"2019-11-19T23:16:24.2602448Z","keyId":"47fde652-8b60-4384-b630-8e5f8f6e24b1","startDateTime":"2018-11-19T23:16:24.2602448Z","secretText":"somegeneratedpassword","hint":null}]}";
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri("https://graph.microsoft.com");
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", authHeaderValue.Result.AccessToken);
client.DefaultRequestHeaders
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var method = new HttpMethod("PATCH");
var requestUri = $"https://graph.microsoft.com/beta/applications/{applicationId}";
// I have commented out this method and passed in my JSON instead.
//var content = GeneratePasswordCredentials(passwordHint);
var content = jsonContent;
var request = new HttpRequestMessage(method, requestUri)
{
Content = new StringContent(
content,
System.Text.Encoding.UTF8,
"application/json")
};
request.Headers
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var resultApi = client.SendAsync(request).GetAwaiter().GetResult();
//response = await resultApi.Content.ReadAsStringAsync();
var response = resultApi.Content.ReadAsStringAsync().GetAwaiter().GetResult();
}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
The body content format should be
{
"passwordCredentials":
[
{"customKeyIdentifier":"YWJjZA==",
"startDateTime":"2018-11-20T02:37:07.3963006Z",
"endDateTime":"2019-11-20T02:37:07.3963006Z",
"secretText":"The passwords must be 16-64 characters in length",
"keyId":"aeda515d-dc58-4ce6-a452-3bc3d84f58a3",
"hint":"xxx"}
]
}
The following the demo code to Generate PasswordCredentials body content
public static string GeneratePasswordCredentials(string passwordHint)
{
var passwordCredential = new JObject
{
new JProperty("customKeyIdentifier",Encoding.UTF8.GetBytes(passwordHint)),
new JProperty("startDateTime",DateTime.UtcNow),
new JProperty("endDateTime", DateTime.UtcNow.AddYears(1)),
new JProperty("secretText", "The passwords must be 16-64 characters in length"),
new JProperty("keyId", Guid.NewGuid().ToString()),
new JProperty("hint", passwordHint)
};
JArray jArray = new JArray
{
passwordCredential
};
var jsonObject = new JObject
{
new JProperty("passwordCredentials",jArray)
};
var json = JsonConvert.SerializeObject(jsonObject);
return json;
}
Note: The request url should be $"https://graph.microsoft.com/beta/applications/{ApplicationObjectId}"
add a comment |
up vote
0
down vote
The body content format should be
{
"passwordCredentials":
[
{"customKeyIdentifier":"YWJjZA==",
"startDateTime":"2018-11-20T02:37:07.3963006Z",
"endDateTime":"2019-11-20T02:37:07.3963006Z",
"secretText":"The passwords must be 16-64 characters in length",
"keyId":"aeda515d-dc58-4ce6-a452-3bc3d84f58a3",
"hint":"xxx"}
]
}
The following the demo code to Generate PasswordCredentials body content
public static string GeneratePasswordCredentials(string passwordHint)
{
var passwordCredential = new JObject
{
new JProperty("customKeyIdentifier",Encoding.UTF8.GetBytes(passwordHint)),
new JProperty("startDateTime",DateTime.UtcNow),
new JProperty("endDateTime", DateTime.UtcNow.AddYears(1)),
new JProperty("secretText", "The passwords must be 16-64 characters in length"),
new JProperty("keyId", Guid.NewGuid().ToString()),
new JProperty("hint", passwordHint)
};
JArray jArray = new JArray
{
passwordCredential
};
var jsonObject = new JObject
{
new JProperty("passwordCredentials",jArray)
};
var json = JsonConvert.SerializeObject(jsonObject);
return json;
}
Note: The request url should be $"https://graph.microsoft.com/beta/applications/{ApplicationObjectId}"
add a comment |
up vote
0
down vote
up vote
0
down vote
The body content format should be
{
"passwordCredentials":
[
{"customKeyIdentifier":"YWJjZA==",
"startDateTime":"2018-11-20T02:37:07.3963006Z",
"endDateTime":"2019-11-20T02:37:07.3963006Z",
"secretText":"The passwords must be 16-64 characters in length",
"keyId":"aeda515d-dc58-4ce6-a452-3bc3d84f58a3",
"hint":"xxx"}
]
}
The following the demo code to Generate PasswordCredentials body content
public static string GeneratePasswordCredentials(string passwordHint)
{
var passwordCredential = new JObject
{
new JProperty("customKeyIdentifier",Encoding.UTF8.GetBytes(passwordHint)),
new JProperty("startDateTime",DateTime.UtcNow),
new JProperty("endDateTime", DateTime.UtcNow.AddYears(1)),
new JProperty("secretText", "The passwords must be 16-64 characters in length"),
new JProperty("keyId", Guid.NewGuid().ToString()),
new JProperty("hint", passwordHint)
};
JArray jArray = new JArray
{
passwordCredential
};
var jsonObject = new JObject
{
new JProperty("passwordCredentials",jArray)
};
var json = JsonConvert.SerializeObject(jsonObject);
return json;
}
Note: The request url should be $"https://graph.microsoft.com/beta/applications/{ApplicationObjectId}"
The body content format should be
{
"passwordCredentials":
[
{"customKeyIdentifier":"YWJjZA==",
"startDateTime":"2018-11-20T02:37:07.3963006Z",
"endDateTime":"2019-11-20T02:37:07.3963006Z",
"secretText":"The passwords must be 16-64 characters in length",
"keyId":"aeda515d-dc58-4ce6-a452-3bc3d84f58a3",
"hint":"xxx"}
]
}
The following the demo code to Generate PasswordCredentials body content
public static string GeneratePasswordCredentials(string passwordHint)
{
var passwordCredential = new JObject
{
new JProperty("customKeyIdentifier",Encoding.UTF8.GetBytes(passwordHint)),
new JProperty("startDateTime",DateTime.UtcNow),
new JProperty("endDateTime", DateTime.UtcNow.AddYears(1)),
new JProperty("secretText", "The passwords must be 16-64 characters in length"),
new JProperty("keyId", Guid.NewGuid().ToString()),
new JProperty("hint", passwordHint)
};
JArray jArray = new JArray
{
passwordCredential
};
var jsonObject = new JObject
{
new JProperty("passwordCredentials",jArray)
};
var json = JsonConvert.SerializeObject(jsonObject);
return json;
}
Note: The request url should be $"https://graph.microsoft.com/beta/applications/{ApplicationObjectId}"
edited Nov 20 at 2:49
answered Nov 20 at 2:43
Tom Sun
15.9k2921
15.9k2921
add a comment |
add a comment |
up vote
0
down vote
The issue is with JSON string specified for Update Application Microsoft Graph API. It's missing which property you're trying to update for the application. I've added "passwordCredentials" property and given it the JSON as a collection. See jsonContent variable at the very beginning of my code.
/*Only change here from original JSON is to add the passwordCredentials node*/
{
"passwordCredentials":[
{
"customKeyIdentifier": null,
"endDateTime": "2019-11-19T23:16:24.2602448Z",
"keyId": "47fde652-8b60-4384-b630-8e5f8f6e24b1",
"startDateTime": "2018-11-19T23:16:24.2602448Z",
"secretText": "SomeGeneratedPassword",
"hint": null
}
]
}
I started with your code and the 400 bad response error reproduced for me as well.
Below is the final working code and now I get back a 204 response status. I can also see the new key added to Application keys collection from Azure Portal > App Registrations > My app > Settings > Keys
string jsonContent = "{"passwordCredentials":[{"customKeyIdentifier":null,"endDateTime":"2019-11-19T23:16:24.2602448Z","keyId":"47fde652-8b60-4384-b630-8e5f8f6e24b1","startDateTime":"2018-11-19T23:16:24.2602448Z","secretText":"somegeneratedpassword","hint":null}]}";
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri("https://graph.microsoft.com");
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", authHeaderValue.Result.AccessToken);
client.DefaultRequestHeaders
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var method = new HttpMethod("PATCH");
var requestUri = $"https://graph.microsoft.com/beta/applications/{applicationId}";
// I have commented out this method and passed in my JSON instead.
//var content = GeneratePasswordCredentials(passwordHint);
var content = jsonContent;
var request = new HttpRequestMessage(method, requestUri)
{
Content = new StringContent(
content,
System.Text.Encoding.UTF8,
"application/json")
};
request.Headers
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var resultApi = client.SendAsync(request).GetAwaiter().GetResult();
//response = await resultApi.Content.ReadAsStringAsync();
var response = resultApi.Content.ReadAsStringAsync().GetAwaiter().GetResult();
}
add a comment |
up vote
0
down vote
The issue is with JSON string specified for Update Application Microsoft Graph API. It's missing which property you're trying to update for the application. I've added "passwordCredentials" property and given it the JSON as a collection. See jsonContent variable at the very beginning of my code.
/*Only change here from original JSON is to add the passwordCredentials node*/
{
"passwordCredentials":[
{
"customKeyIdentifier": null,
"endDateTime": "2019-11-19T23:16:24.2602448Z",
"keyId": "47fde652-8b60-4384-b630-8e5f8f6e24b1",
"startDateTime": "2018-11-19T23:16:24.2602448Z",
"secretText": "SomeGeneratedPassword",
"hint": null
}
]
}
I started with your code and the 400 bad response error reproduced for me as well.
Below is the final working code and now I get back a 204 response status. I can also see the new key added to Application keys collection from Azure Portal > App Registrations > My app > Settings > Keys
string jsonContent = "{"passwordCredentials":[{"customKeyIdentifier":null,"endDateTime":"2019-11-19T23:16:24.2602448Z","keyId":"47fde652-8b60-4384-b630-8e5f8f6e24b1","startDateTime":"2018-11-19T23:16:24.2602448Z","secretText":"somegeneratedpassword","hint":null}]}";
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri("https://graph.microsoft.com");
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", authHeaderValue.Result.AccessToken);
client.DefaultRequestHeaders
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var method = new HttpMethod("PATCH");
var requestUri = $"https://graph.microsoft.com/beta/applications/{applicationId}";
// I have commented out this method and passed in my JSON instead.
//var content = GeneratePasswordCredentials(passwordHint);
var content = jsonContent;
var request = new HttpRequestMessage(method, requestUri)
{
Content = new StringContent(
content,
System.Text.Encoding.UTF8,
"application/json")
};
request.Headers
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var resultApi = client.SendAsync(request).GetAwaiter().GetResult();
//response = await resultApi.Content.ReadAsStringAsync();
var response = resultApi.Content.ReadAsStringAsync().GetAwaiter().GetResult();
}
add a comment |
up vote
0
down vote
up vote
0
down vote
The issue is with JSON string specified for Update Application Microsoft Graph API. It's missing which property you're trying to update for the application. I've added "passwordCredentials" property and given it the JSON as a collection. See jsonContent variable at the very beginning of my code.
/*Only change here from original JSON is to add the passwordCredentials node*/
{
"passwordCredentials":[
{
"customKeyIdentifier": null,
"endDateTime": "2019-11-19T23:16:24.2602448Z",
"keyId": "47fde652-8b60-4384-b630-8e5f8f6e24b1",
"startDateTime": "2018-11-19T23:16:24.2602448Z",
"secretText": "SomeGeneratedPassword",
"hint": null
}
]
}
I started with your code and the 400 bad response error reproduced for me as well.
Below is the final working code and now I get back a 204 response status. I can also see the new key added to Application keys collection from Azure Portal > App Registrations > My app > Settings > Keys
string jsonContent = "{"passwordCredentials":[{"customKeyIdentifier":null,"endDateTime":"2019-11-19T23:16:24.2602448Z","keyId":"47fde652-8b60-4384-b630-8e5f8f6e24b1","startDateTime":"2018-11-19T23:16:24.2602448Z","secretText":"somegeneratedpassword","hint":null}]}";
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri("https://graph.microsoft.com");
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", authHeaderValue.Result.AccessToken);
client.DefaultRequestHeaders
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var method = new HttpMethod("PATCH");
var requestUri = $"https://graph.microsoft.com/beta/applications/{applicationId}";
// I have commented out this method and passed in my JSON instead.
//var content = GeneratePasswordCredentials(passwordHint);
var content = jsonContent;
var request = new HttpRequestMessage(method, requestUri)
{
Content = new StringContent(
content,
System.Text.Encoding.UTF8,
"application/json")
};
request.Headers
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var resultApi = client.SendAsync(request).GetAwaiter().GetResult();
//response = await resultApi.Content.ReadAsStringAsync();
var response = resultApi.Content.ReadAsStringAsync().GetAwaiter().GetResult();
}
The issue is with JSON string specified for Update Application Microsoft Graph API. It's missing which property you're trying to update for the application. I've added "passwordCredentials" property and given it the JSON as a collection. See jsonContent variable at the very beginning of my code.
/*Only change here from original JSON is to add the passwordCredentials node*/
{
"passwordCredentials":[
{
"customKeyIdentifier": null,
"endDateTime": "2019-11-19T23:16:24.2602448Z",
"keyId": "47fde652-8b60-4384-b630-8e5f8f6e24b1",
"startDateTime": "2018-11-19T23:16:24.2602448Z",
"secretText": "SomeGeneratedPassword",
"hint": null
}
]
}
I started with your code and the 400 bad response error reproduced for me as well.
Below is the final working code and now I get back a 204 response status. I can also see the new key added to Application keys collection from Azure Portal > App Registrations > My app > Settings > Keys
string jsonContent = "{"passwordCredentials":[{"customKeyIdentifier":null,"endDateTime":"2019-11-19T23:16:24.2602448Z","keyId":"47fde652-8b60-4384-b630-8e5f8f6e24b1","startDateTime":"2018-11-19T23:16:24.2602448Z","secretText":"somegeneratedpassword","hint":null}]}";
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri("https://graph.microsoft.com");
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", authHeaderValue.Result.AccessToken);
client.DefaultRequestHeaders
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var method = new HttpMethod("PATCH");
var requestUri = $"https://graph.microsoft.com/beta/applications/{applicationId}";
// I have commented out this method and passed in my JSON instead.
//var content = GeneratePasswordCredentials(passwordHint);
var content = jsonContent;
var request = new HttpRequestMessage(method, requestUri)
{
Content = new StringContent(
content,
System.Text.Encoding.UTF8,
"application/json")
};
request.Headers
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var resultApi = client.SendAsync(request).GetAwaiter().GetResult();
//response = await resultApi.Content.ReadAsStringAsync();
var response = resultApi.Content.ReadAsStringAsync().GetAwaiter().GetResult();
}
edited Nov 20 at 2:54
answered Nov 20 at 2:41


Rohit Saigal
2,4722216
2,4722216
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384196%2fupdate-azure-ad-application-keys-or-secrets-using-microsoft-graph-api-badreque%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
aRPXSXjj,YKs47RlB3unBuu DwG y1V,M Lf6DCfzrfyTRnpzSSf SCcB7DPV7sxO jN,OiUQRuP3L