The ViewData item that has the key 'XXX' is of type 'System.Int32' but must be of type 'IEnumerable'
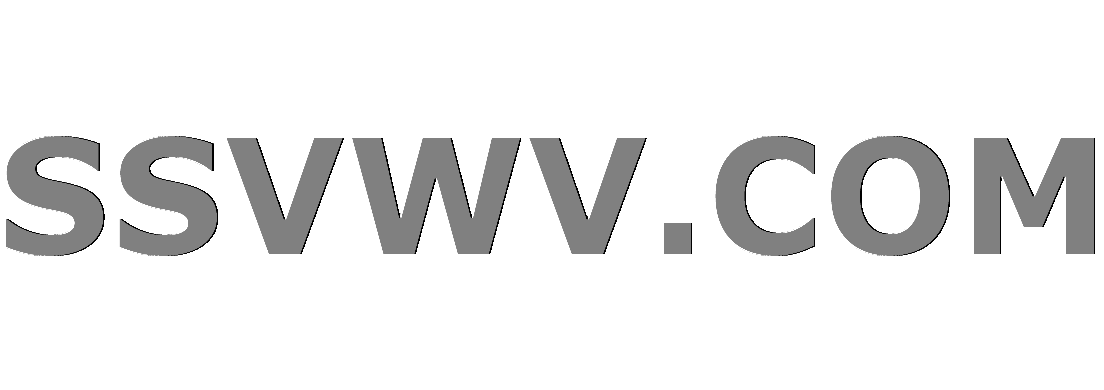
Multi tool use
up vote
80
down vote
favorite
I have the following view model
public class ProjectVM
{
....
[Display(Name = "Category")]
[Required(ErrorMessage = "Please select a category")]
public int CategoryID { get; set; }
public IEnumerable<SelectListItem> CategoryList { get; set; }
....
}
and the following controller method to create a new Project and assign a Category
public ActionResult Create()
{
ProjectVM model = new ProjectVM
{
CategoryList = new SelectList(db.Categories, "ID", "Name")
}
return View(model);
}
public ActionResult Create(ProjectVM model)
{
if (!ModelState.IsValid)
{
return View(model);
}
// Save and redirect
}
and in the view
@model ProjectVM
....
@using (Html.BeginForm())
{
....
@Html.LabelFor(m => m.CategoryID)
@Html.DropDownListFor(m => m.CategoryID, Model.CategoryList, "-Please select-")
@Html.ValidationMessageFor(m => m.CategoryID)
....
<input type="submit" value="Create" />
}
The view displays correctly but when submitting the form, I get the following error message
InvalidOperationException: The ViewData item that has the key 'CategoryID' is of type 'System.Int32' but must be of type 'IEnumerable<SelectListItem>'.
The same error occurs using the @Html.DropDownList()
method, and if I pass the SelectList using a ViewBag
or ViewData
.
c# asp.net-mvc
add a comment |
up vote
80
down vote
favorite
I have the following view model
public class ProjectVM
{
....
[Display(Name = "Category")]
[Required(ErrorMessage = "Please select a category")]
public int CategoryID { get; set; }
public IEnumerable<SelectListItem> CategoryList { get; set; }
....
}
and the following controller method to create a new Project and assign a Category
public ActionResult Create()
{
ProjectVM model = new ProjectVM
{
CategoryList = new SelectList(db.Categories, "ID", "Name")
}
return View(model);
}
public ActionResult Create(ProjectVM model)
{
if (!ModelState.IsValid)
{
return View(model);
}
// Save and redirect
}
and in the view
@model ProjectVM
....
@using (Html.BeginForm())
{
....
@Html.LabelFor(m => m.CategoryID)
@Html.DropDownListFor(m => m.CategoryID, Model.CategoryList, "-Please select-")
@Html.ValidationMessageFor(m => m.CategoryID)
....
<input type="submit" value="Create" />
}
The view displays correctly but when submitting the form, I get the following error message
InvalidOperationException: The ViewData item that has the key 'CategoryID' is of type 'System.Int32' but must be of type 'IEnumerable<SelectListItem>'.
The same error occurs using the @Html.DropDownList()
method, and if I pass the SelectList using a ViewBag
or ViewData
.
c# asp.net-mvc
add a comment |
up vote
80
down vote
favorite
up vote
80
down vote
favorite
I have the following view model
public class ProjectVM
{
....
[Display(Name = "Category")]
[Required(ErrorMessage = "Please select a category")]
public int CategoryID { get; set; }
public IEnumerable<SelectListItem> CategoryList { get; set; }
....
}
and the following controller method to create a new Project and assign a Category
public ActionResult Create()
{
ProjectVM model = new ProjectVM
{
CategoryList = new SelectList(db.Categories, "ID", "Name")
}
return View(model);
}
public ActionResult Create(ProjectVM model)
{
if (!ModelState.IsValid)
{
return View(model);
}
// Save and redirect
}
and in the view
@model ProjectVM
....
@using (Html.BeginForm())
{
....
@Html.LabelFor(m => m.CategoryID)
@Html.DropDownListFor(m => m.CategoryID, Model.CategoryList, "-Please select-")
@Html.ValidationMessageFor(m => m.CategoryID)
....
<input type="submit" value="Create" />
}
The view displays correctly but when submitting the form, I get the following error message
InvalidOperationException: The ViewData item that has the key 'CategoryID' is of type 'System.Int32' but must be of type 'IEnumerable<SelectListItem>'.
The same error occurs using the @Html.DropDownList()
method, and if I pass the SelectList using a ViewBag
or ViewData
.
c# asp.net-mvc
I have the following view model
public class ProjectVM
{
....
[Display(Name = "Category")]
[Required(ErrorMessage = "Please select a category")]
public int CategoryID { get; set; }
public IEnumerable<SelectListItem> CategoryList { get; set; }
....
}
and the following controller method to create a new Project and assign a Category
public ActionResult Create()
{
ProjectVM model = new ProjectVM
{
CategoryList = new SelectList(db.Categories, "ID", "Name")
}
return View(model);
}
public ActionResult Create(ProjectVM model)
{
if (!ModelState.IsValid)
{
return View(model);
}
// Save and redirect
}
and in the view
@model ProjectVM
....
@using (Html.BeginForm())
{
....
@Html.LabelFor(m => m.CategoryID)
@Html.DropDownListFor(m => m.CategoryID, Model.CategoryList, "-Please select-")
@Html.ValidationMessageFor(m => m.CategoryID)
....
<input type="submit" value="Create" />
}
The view displays correctly but when submitting the form, I get the following error message
InvalidOperationException: The ViewData item that has the key 'CategoryID' is of type 'System.Int32' but must be of type 'IEnumerable<SelectListItem>'.
The same error occurs using the @Html.DropDownList()
method, and if I pass the SelectList using a ViewBag
or ViewData
.
c# asp.net-mvc
c# asp.net-mvc
asked Dec 19 '15 at 1:26
user3559349
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
77
down vote
accepted
The error means that the value of CategoryList
is null (and as a result the DropDownListFor()
method expects that the first parameter is of type IEnumerable<SelectListItem>
).
You are not generating an input for each property of each SelectListItem
in CategoryList
(and nor should you) so no values for the SelectList
are posted to the controller method, and therefore the value of model.CategoryList
in the POST method is null
. If you return the view, you must first reassign the value of CategoryList
, just as you did in the GET method.
public ActionResult Create(ProjectVM model)
{
if (!ModelState.IsValid)
{
model.CategoryList = new SelectList(db.Categories, "ID", "Name"); // add this
return View(model);
}
// Save and redirect
}
To explain the inner workings (the source code can be seen here)
Each overload of DropDownList()
and DropDownListFor()
eventually calls the following method
private static MvcHtmlString SelectInternal(this HtmlHelper htmlHelper, ModelMetadata metadata,
string optionLabel, string name, IEnumerable<SelectListItem> selectList, bool allowMultiple,
IDictionary<string, object> htmlAttributes)
which checks if the selectList
(the second parameter of @Html.DropDownListFor()
) is null
// If we got a null selectList, try to use ViewData to get the list of items.
if (selectList == null)
{
selectList = htmlHelper.GetSelectData(name);
usedViewData = true;
}
which in turn calls
private static IEnumerable<SelectListItem> GetSelectData(this HtmlHelper htmlHelper, string name)
which evaluates the the first parameter of @Html.DropDownListFor()
(in this case CategoryID
)
....
o = htmlHelper.ViewData.Eval(name);
....
IEnumerable<SelectListItem> selectList = o as IEnumerable<SelectListItem>;
if (selectList == null)
{
throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture,
MvcResources.HtmlHelper_WrongSelectDataType,
name, o.GetType().FullName, "IEnumerable<SelectListItem>"));
}
Because property CategoryID
is typeof int
, it cannot be cast to IEnumerable<SelectListItem>
and the exception is thrown (which is defined in the MvcResources.resx
file as)
<data name="HtmlHelper_WrongSelectDataType" xml:space="preserve">
<value>The ViewData item that has the key '{0}' is of type '{1}' but must be of type '{2}'.</value>
</data>
7
@Shyju, Yes, I asked and answered it (as community wiki) purely for the purposes of dupe-hammering many other similar question on SO which remain unanswered or unaccepted. But I see the revenge voters have already started - the first one was less than 2 seconds after posting - not enough time to even read it let alone the answer.
– user3559349
Dec 19 '15 at 1:49
1
I see. There are 100's of questions with the same problem. Usually the people who ask those questions does not do proper search ( or they copied and pasted an existing answer word by word, but did not work!) So i am not sure this might really help. :) Nicely written BTW.
– Shyju
Dec 19 '15 at 2:15
@Stephen this is not right way u are asking and u are answering
– Dilip
Dec 19 '15 at 5:45
8
@DilipN, What do you mean not the right way? Its actually encouraged on SO. You should read this and spend some time on meta.
– user3559349
Dec 19 '15 at 5:46
3
@DilipN, Because I will use it to mark numerous similar questions as a duplicates which have either been either been left unanswered, or answered but not accepted so they can be closed out (and so others do not waste their time). I have also made it a community wiki so anyone can edit and improve it over time.
– user3559349
Dec 19 '15 at 5:54
|
show 4 more comments
up vote
0
down vote
I had the same problem, I was getting an invalid ModelState when I tried to post the form. For me, this was caused by setting CategoryId to int, when I changed it to string the ModelState was valid and the Create method worked as expected.
add a comment |
up vote
0
down vote
according to stephen answer, this can be useful:
@Html.DropDownListFor(m => m.CategoryID, Model.CategoryList ?? new List<SelectListItem>(), "-Please select-")
or in ProjectVM:
public class ProjectVM
{
public ProjectVM()
{
CategoryList = new List<SelectListItem>();
}
...
}
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
77
down vote
accepted
The error means that the value of CategoryList
is null (and as a result the DropDownListFor()
method expects that the first parameter is of type IEnumerable<SelectListItem>
).
You are not generating an input for each property of each SelectListItem
in CategoryList
(and nor should you) so no values for the SelectList
are posted to the controller method, and therefore the value of model.CategoryList
in the POST method is null
. If you return the view, you must first reassign the value of CategoryList
, just as you did in the GET method.
public ActionResult Create(ProjectVM model)
{
if (!ModelState.IsValid)
{
model.CategoryList = new SelectList(db.Categories, "ID", "Name"); // add this
return View(model);
}
// Save and redirect
}
To explain the inner workings (the source code can be seen here)
Each overload of DropDownList()
and DropDownListFor()
eventually calls the following method
private static MvcHtmlString SelectInternal(this HtmlHelper htmlHelper, ModelMetadata metadata,
string optionLabel, string name, IEnumerable<SelectListItem> selectList, bool allowMultiple,
IDictionary<string, object> htmlAttributes)
which checks if the selectList
(the second parameter of @Html.DropDownListFor()
) is null
// If we got a null selectList, try to use ViewData to get the list of items.
if (selectList == null)
{
selectList = htmlHelper.GetSelectData(name);
usedViewData = true;
}
which in turn calls
private static IEnumerable<SelectListItem> GetSelectData(this HtmlHelper htmlHelper, string name)
which evaluates the the first parameter of @Html.DropDownListFor()
(in this case CategoryID
)
....
o = htmlHelper.ViewData.Eval(name);
....
IEnumerable<SelectListItem> selectList = o as IEnumerable<SelectListItem>;
if (selectList == null)
{
throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture,
MvcResources.HtmlHelper_WrongSelectDataType,
name, o.GetType().FullName, "IEnumerable<SelectListItem>"));
}
Because property CategoryID
is typeof int
, it cannot be cast to IEnumerable<SelectListItem>
and the exception is thrown (which is defined in the MvcResources.resx
file as)
<data name="HtmlHelper_WrongSelectDataType" xml:space="preserve">
<value>The ViewData item that has the key '{0}' is of type '{1}' but must be of type '{2}'.</value>
</data>
7
@Shyju, Yes, I asked and answered it (as community wiki) purely for the purposes of dupe-hammering many other similar question on SO which remain unanswered or unaccepted. But I see the revenge voters have already started - the first one was less than 2 seconds after posting - not enough time to even read it let alone the answer.
– user3559349
Dec 19 '15 at 1:49
1
I see. There are 100's of questions with the same problem. Usually the people who ask those questions does not do proper search ( or they copied and pasted an existing answer word by word, but did not work!) So i am not sure this might really help. :) Nicely written BTW.
– Shyju
Dec 19 '15 at 2:15
@Stephen this is not right way u are asking and u are answering
– Dilip
Dec 19 '15 at 5:45
8
@DilipN, What do you mean not the right way? Its actually encouraged on SO. You should read this and spend some time on meta.
– user3559349
Dec 19 '15 at 5:46
3
@DilipN, Because I will use it to mark numerous similar questions as a duplicates which have either been either been left unanswered, or answered but not accepted so they can be closed out (and so others do not waste their time). I have also made it a community wiki so anyone can edit and improve it over time.
– user3559349
Dec 19 '15 at 5:54
|
show 4 more comments
up vote
77
down vote
accepted
The error means that the value of CategoryList
is null (and as a result the DropDownListFor()
method expects that the first parameter is of type IEnumerable<SelectListItem>
).
You are not generating an input for each property of each SelectListItem
in CategoryList
(and nor should you) so no values for the SelectList
are posted to the controller method, and therefore the value of model.CategoryList
in the POST method is null
. If you return the view, you must first reassign the value of CategoryList
, just as you did in the GET method.
public ActionResult Create(ProjectVM model)
{
if (!ModelState.IsValid)
{
model.CategoryList = new SelectList(db.Categories, "ID", "Name"); // add this
return View(model);
}
// Save and redirect
}
To explain the inner workings (the source code can be seen here)
Each overload of DropDownList()
and DropDownListFor()
eventually calls the following method
private static MvcHtmlString SelectInternal(this HtmlHelper htmlHelper, ModelMetadata metadata,
string optionLabel, string name, IEnumerable<SelectListItem> selectList, bool allowMultiple,
IDictionary<string, object> htmlAttributes)
which checks if the selectList
(the second parameter of @Html.DropDownListFor()
) is null
// If we got a null selectList, try to use ViewData to get the list of items.
if (selectList == null)
{
selectList = htmlHelper.GetSelectData(name);
usedViewData = true;
}
which in turn calls
private static IEnumerable<SelectListItem> GetSelectData(this HtmlHelper htmlHelper, string name)
which evaluates the the first parameter of @Html.DropDownListFor()
(in this case CategoryID
)
....
o = htmlHelper.ViewData.Eval(name);
....
IEnumerable<SelectListItem> selectList = o as IEnumerable<SelectListItem>;
if (selectList == null)
{
throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture,
MvcResources.HtmlHelper_WrongSelectDataType,
name, o.GetType().FullName, "IEnumerable<SelectListItem>"));
}
Because property CategoryID
is typeof int
, it cannot be cast to IEnumerable<SelectListItem>
and the exception is thrown (which is defined in the MvcResources.resx
file as)
<data name="HtmlHelper_WrongSelectDataType" xml:space="preserve">
<value>The ViewData item that has the key '{0}' is of type '{1}' but must be of type '{2}'.</value>
</data>
7
@Shyju, Yes, I asked and answered it (as community wiki) purely for the purposes of dupe-hammering many other similar question on SO which remain unanswered or unaccepted. But I see the revenge voters have already started - the first one was less than 2 seconds after posting - not enough time to even read it let alone the answer.
– user3559349
Dec 19 '15 at 1:49
1
I see. There are 100's of questions with the same problem. Usually the people who ask those questions does not do proper search ( or they copied and pasted an existing answer word by word, but did not work!) So i am not sure this might really help. :) Nicely written BTW.
– Shyju
Dec 19 '15 at 2:15
@Stephen this is not right way u are asking and u are answering
– Dilip
Dec 19 '15 at 5:45
8
@DilipN, What do you mean not the right way? Its actually encouraged on SO. You should read this and spend some time on meta.
– user3559349
Dec 19 '15 at 5:46
3
@DilipN, Because I will use it to mark numerous similar questions as a duplicates which have either been either been left unanswered, or answered but not accepted so they can be closed out (and so others do not waste their time). I have also made it a community wiki so anyone can edit and improve it over time.
– user3559349
Dec 19 '15 at 5:54
|
show 4 more comments
up vote
77
down vote
accepted
up vote
77
down vote
accepted
The error means that the value of CategoryList
is null (and as a result the DropDownListFor()
method expects that the first parameter is of type IEnumerable<SelectListItem>
).
You are not generating an input for each property of each SelectListItem
in CategoryList
(and nor should you) so no values for the SelectList
are posted to the controller method, and therefore the value of model.CategoryList
in the POST method is null
. If you return the view, you must first reassign the value of CategoryList
, just as you did in the GET method.
public ActionResult Create(ProjectVM model)
{
if (!ModelState.IsValid)
{
model.CategoryList = new SelectList(db.Categories, "ID", "Name"); // add this
return View(model);
}
// Save and redirect
}
To explain the inner workings (the source code can be seen here)
Each overload of DropDownList()
and DropDownListFor()
eventually calls the following method
private static MvcHtmlString SelectInternal(this HtmlHelper htmlHelper, ModelMetadata metadata,
string optionLabel, string name, IEnumerable<SelectListItem> selectList, bool allowMultiple,
IDictionary<string, object> htmlAttributes)
which checks if the selectList
(the second parameter of @Html.DropDownListFor()
) is null
// If we got a null selectList, try to use ViewData to get the list of items.
if (selectList == null)
{
selectList = htmlHelper.GetSelectData(name);
usedViewData = true;
}
which in turn calls
private static IEnumerable<SelectListItem> GetSelectData(this HtmlHelper htmlHelper, string name)
which evaluates the the first parameter of @Html.DropDownListFor()
(in this case CategoryID
)
....
o = htmlHelper.ViewData.Eval(name);
....
IEnumerable<SelectListItem> selectList = o as IEnumerable<SelectListItem>;
if (selectList == null)
{
throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture,
MvcResources.HtmlHelper_WrongSelectDataType,
name, o.GetType().FullName, "IEnumerable<SelectListItem>"));
}
Because property CategoryID
is typeof int
, it cannot be cast to IEnumerable<SelectListItem>
and the exception is thrown (which is defined in the MvcResources.resx
file as)
<data name="HtmlHelper_WrongSelectDataType" xml:space="preserve">
<value>The ViewData item that has the key '{0}' is of type '{1}' but must be of type '{2}'.</value>
</data>
The error means that the value of CategoryList
is null (and as a result the DropDownListFor()
method expects that the first parameter is of type IEnumerable<SelectListItem>
).
You are not generating an input for each property of each SelectListItem
in CategoryList
(and nor should you) so no values for the SelectList
are posted to the controller method, and therefore the value of model.CategoryList
in the POST method is null
. If you return the view, you must first reassign the value of CategoryList
, just as you did in the GET method.
public ActionResult Create(ProjectVM model)
{
if (!ModelState.IsValid)
{
model.CategoryList = new SelectList(db.Categories, "ID", "Name"); // add this
return View(model);
}
// Save and redirect
}
To explain the inner workings (the source code can be seen here)
Each overload of DropDownList()
and DropDownListFor()
eventually calls the following method
private static MvcHtmlString SelectInternal(this HtmlHelper htmlHelper, ModelMetadata metadata,
string optionLabel, string name, IEnumerable<SelectListItem> selectList, bool allowMultiple,
IDictionary<string, object> htmlAttributes)
which checks if the selectList
(the second parameter of @Html.DropDownListFor()
) is null
// If we got a null selectList, try to use ViewData to get the list of items.
if (selectList == null)
{
selectList = htmlHelper.GetSelectData(name);
usedViewData = true;
}
which in turn calls
private static IEnumerable<SelectListItem> GetSelectData(this HtmlHelper htmlHelper, string name)
which evaluates the the first parameter of @Html.DropDownListFor()
(in this case CategoryID
)
....
o = htmlHelper.ViewData.Eval(name);
....
IEnumerable<SelectListItem> selectList = o as IEnumerable<SelectListItem>;
if (selectList == null)
{
throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture,
MvcResources.HtmlHelper_WrongSelectDataType,
name, o.GetType().FullName, "IEnumerable<SelectListItem>"));
}
Because property CategoryID
is typeof int
, it cannot be cast to IEnumerable<SelectListItem>
and the exception is thrown (which is defined in the MvcResources.resx
file as)
<data name="HtmlHelper_WrongSelectDataType" xml:space="preserve">
<value>The ViewData item that has the key '{0}' is of type '{1}' but must be of type '{2}'.</value>
</data>
answered Dec 19 '15 at 1:26
community wiki
user3559349
7
@Shyju, Yes, I asked and answered it (as community wiki) purely for the purposes of dupe-hammering many other similar question on SO which remain unanswered or unaccepted. But I see the revenge voters have already started - the first one was less than 2 seconds after posting - not enough time to even read it let alone the answer.
– user3559349
Dec 19 '15 at 1:49
1
I see. There are 100's of questions with the same problem. Usually the people who ask those questions does not do proper search ( or they copied and pasted an existing answer word by word, but did not work!) So i am not sure this might really help. :) Nicely written BTW.
– Shyju
Dec 19 '15 at 2:15
@Stephen this is not right way u are asking and u are answering
– Dilip
Dec 19 '15 at 5:45
8
@DilipN, What do you mean not the right way? Its actually encouraged on SO. You should read this and spend some time on meta.
– user3559349
Dec 19 '15 at 5:46
3
@DilipN, Because I will use it to mark numerous similar questions as a duplicates which have either been either been left unanswered, or answered but not accepted so they can be closed out (and so others do not waste their time). I have also made it a community wiki so anyone can edit and improve it over time.
– user3559349
Dec 19 '15 at 5:54
|
show 4 more comments
7
@Shyju, Yes, I asked and answered it (as community wiki) purely for the purposes of dupe-hammering many other similar question on SO which remain unanswered or unaccepted. But I see the revenge voters have already started - the first one was less than 2 seconds after posting - not enough time to even read it let alone the answer.
– user3559349
Dec 19 '15 at 1:49
1
I see. There are 100's of questions with the same problem. Usually the people who ask those questions does not do proper search ( or they copied and pasted an existing answer word by word, but did not work!) So i am not sure this might really help. :) Nicely written BTW.
– Shyju
Dec 19 '15 at 2:15
@Stephen this is not right way u are asking and u are answering
– Dilip
Dec 19 '15 at 5:45
8
@DilipN, What do you mean not the right way? Its actually encouraged on SO. You should read this and spend some time on meta.
– user3559349
Dec 19 '15 at 5:46
3
@DilipN, Because I will use it to mark numerous similar questions as a duplicates which have either been either been left unanswered, or answered but not accepted so they can be closed out (and so others do not waste their time). I have also made it a community wiki so anyone can edit and improve it over time.
– user3559349
Dec 19 '15 at 5:54
7
7
@Shyju, Yes, I asked and answered it (as community wiki) purely for the purposes of dupe-hammering many other similar question on SO which remain unanswered or unaccepted. But I see the revenge voters have already started - the first one was less than 2 seconds after posting - not enough time to even read it let alone the answer.
– user3559349
Dec 19 '15 at 1:49
@Shyju, Yes, I asked and answered it (as community wiki) purely for the purposes of dupe-hammering many other similar question on SO which remain unanswered or unaccepted. But I see the revenge voters have already started - the first one was less than 2 seconds after posting - not enough time to even read it let alone the answer.
– user3559349
Dec 19 '15 at 1:49
1
1
I see. There are 100's of questions with the same problem. Usually the people who ask those questions does not do proper search ( or they copied and pasted an existing answer word by word, but did not work!) So i am not sure this might really help. :) Nicely written BTW.
– Shyju
Dec 19 '15 at 2:15
I see. There are 100's of questions with the same problem. Usually the people who ask those questions does not do proper search ( or they copied and pasted an existing answer word by word, but did not work!) So i am not sure this might really help. :) Nicely written BTW.
– Shyju
Dec 19 '15 at 2:15
@Stephen this is not right way u are asking and u are answering
– Dilip
Dec 19 '15 at 5:45
@Stephen this is not right way u are asking and u are answering
– Dilip
Dec 19 '15 at 5:45
8
8
@DilipN, What do you mean not the right way? Its actually encouraged on SO. You should read this and spend some time on meta.
– user3559349
Dec 19 '15 at 5:46
@DilipN, What do you mean not the right way? Its actually encouraged on SO. You should read this and spend some time on meta.
– user3559349
Dec 19 '15 at 5:46
3
3
@DilipN, Because I will use it to mark numerous similar questions as a duplicates which have either been either been left unanswered, or answered but not accepted so they can be closed out (and so others do not waste their time). I have also made it a community wiki so anyone can edit and improve it over time.
– user3559349
Dec 19 '15 at 5:54
@DilipN, Because I will use it to mark numerous similar questions as a duplicates which have either been either been left unanswered, or answered but not accepted so they can be closed out (and so others do not waste their time). I have also made it a community wiki so anyone can edit and improve it over time.
– user3559349
Dec 19 '15 at 5:54
|
show 4 more comments
up vote
0
down vote
I had the same problem, I was getting an invalid ModelState when I tried to post the form. For me, this was caused by setting CategoryId to int, when I changed it to string the ModelState was valid and the Create method worked as expected.
add a comment |
up vote
0
down vote
I had the same problem, I was getting an invalid ModelState when I tried to post the form. For me, this was caused by setting CategoryId to int, when I changed it to string the ModelState was valid and the Create method worked as expected.
add a comment |
up vote
0
down vote
up vote
0
down vote
I had the same problem, I was getting an invalid ModelState when I tried to post the form. For me, this was caused by setting CategoryId to int, when I changed it to string the ModelState was valid and the Create method worked as expected.
I had the same problem, I was getting an invalid ModelState when I tried to post the form. For me, this was caused by setting CategoryId to int, when I changed it to string the ModelState was valid and the Create method worked as expected.
answered Nov 15 at 14:02
ardmark
267
267
add a comment |
add a comment |
up vote
0
down vote
according to stephen answer, this can be useful:
@Html.DropDownListFor(m => m.CategoryID, Model.CategoryList ?? new List<SelectListItem>(), "-Please select-")
or in ProjectVM:
public class ProjectVM
{
public ProjectVM()
{
CategoryList = new List<SelectListItem>();
}
...
}
add a comment |
up vote
0
down vote
according to stephen answer, this can be useful:
@Html.DropDownListFor(m => m.CategoryID, Model.CategoryList ?? new List<SelectListItem>(), "-Please select-")
or in ProjectVM:
public class ProjectVM
{
public ProjectVM()
{
CategoryList = new List<SelectListItem>();
}
...
}
add a comment |
up vote
0
down vote
up vote
0
down vote
according to stephen answer, this can be useful:
@Html.DropDownListFor(m => m.CategoryID, Model.CategoryList ?? new List<SelectListItem>(), "-Please select-")
or in ProjectVM:
public class ProjectVM
{
public ProjectVM()
{
CategoryList = new List<SelectListItem>();
}
...
}
according to stephen answer, this can be useful:
@Html.DropDownListFor(m => m.CategoryID, Model.CategoryList ?? new List<SelectListItem>(), "-Please select-")
or in ProjectVM:
public class ProjectVM
{
public ProjectVM()
{
CategoryList = new List<SelectListItem>();
}
...
}
answered Nov 25 at 12:59
Omid-RH
78411125
78411125
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f34366305%2fthe-viewdata-item-that-has-the-key-xxx-is-of-type-system-int32-but-must-be-o%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
y70f,3,HQ5PalrZ,DU4s,OPXsYNisvUlr2rZ0 CebhMRUG5GV9