Tensor flow throws an uninitialized error even after the variable is initialized
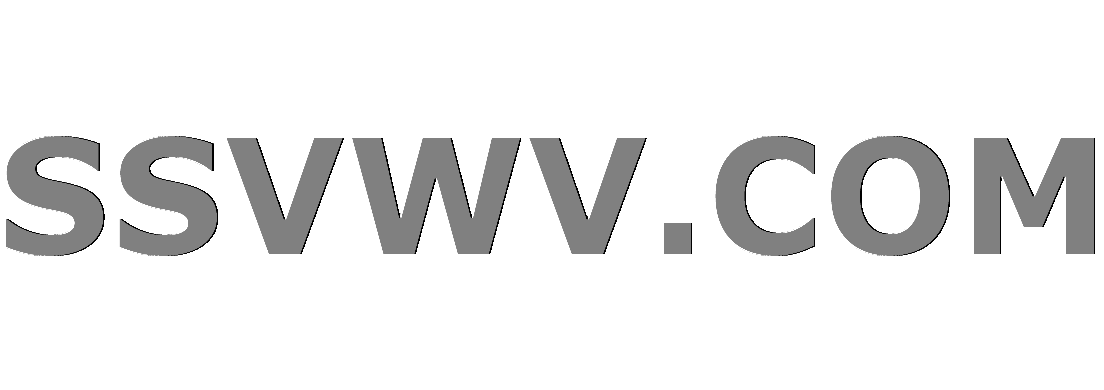
Multi tool use
up vote
0
down vote
favorite
I am trying to write a program in python using tensorflow to fit data with different patterns using a neural network with a hidden layer. I am facing an error with the code which states that the variable b2 is uninitialized. But I have initialized it already and dont understand what I am missing here.
This is part of an assignment (the dataset can be understood here) and got stuck here while solving it.
The link to the colab notebook is here.
The initialization lines look like
W1 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(hidden, 2)), name="W1")
b1 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(hidden, 1)), name="b1")
W2 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(classes, hidden)), name="W2")
b2 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(classes, 1)), name="b2")
The code snippet that shows the computation graph is below.
operation = "ReLU" # "Sigmoid"
o = tf.add(tf.matmul(W1, p), b1)
# ReLU or Sigmoid
if operation == "ReLU":
z = tf.zeros((hidden, 1), dtype=tf.float64)
output = tf.maximum(o, z)
else:
output = tf.sigmoid(o)
foutput = tf.add(tf.matmul(W2, output), b2)
crossentropy = tf.log(tf.exp(foutput) / tf.reduce_sum(tf.exp(foutput), 0))
init = tf.initialize_all_variables()
with tf.Session() as sess:
ce = sess.run([crossentropy], feed_dict={p : inputs, t : targets, lr : 0.01})
print(ce)
Error message
FailedPreconditionError Traceback (most recent call last)
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _do_call(self, fn, *args)
1333 try:
-> 1334 return fn(*args)
1335 except errors.OpError as e:
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _run_fn(feed_dict, fetch_list, target_list, options, run_metadata)
1318 return self._call_tf_sessionrun(
-> 1319 options, feed_dict, fetch_list, target_list, run_metadata)
1320
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _call_tf_sessionrun(self, options, feed_dict, fetch_list, target_list, run_metadata)
1406 self._session, options, feed_dict, fetch_list, target_list,
-> 1407 run_metadata)
1408
FailedPreconditionError: Attempting to use uninitialized value b2
[[{{node b2/read}} = Identity[T=DT_DOUBLE, _device="/job:localhost/replica:0/task:0/device:CPU:0"](b2)]]
python tensorflow
add a comment |
up vote
0
down vote
favorite
I am trying to write a program in python using tensorflow to fit data with different patterns using a neural network with a hidden layer. I am facing an error with the code which states that the variable b2 is uninitialized. But I have initialized it already and dont understand what I am missing here.
This is part of an assignment (the dataset can be understood here) and got stuck here while solving it.
The link to the colab notebook is here.
The initialization lines look like
W1 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(hidden, 2)), name="W1")
b1 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(hidden, 1)), name="b1")
W2 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(classes, hidden)), name="W2")
b2 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(classes, 1)), name="b2")
The code snippet that shows the computation graph is below.
operation = "ReLU" # "Sigmoid"
o = tf.add(tf.matmul(W1, p), b1)
# ReLU or Sigmoid
if operation == "ReLU":
z = tf.zeros((hidden, 1), dtype=tf.float64)
output = tf.maximum(o, z)
else:
output = tf.sigmoid(o)
foutput = tf.add(tf.matmul(W2, output), b2)
crossentropy = tf.log(tf.exp(foutput) / tf.reduce_sum(tf.exp(foutput), 0))
init = tf.initialize_all_variables()
with tf.Session() as sess:
ce = sess.run([crossentropy], feed_dict={p : inputs, t : targets, lr : 0.01})
print(ce)
Error message
FailedPreconditionError Traceback (most recent call last)
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _do_call(self, fn, *args)
1333 try:
-> 1334 return fn(*args)
1335 except errors.OpError as e:
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _run_fn(feed_dict, fetch_list, target_list, options, run_metadata)
1318 return self._call_tf_sessionrun(
-> 1319 options, feed_dict, fetch_list, target_list, run_metadata)
1320
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _call_tf_sessionrun(self, options, feed_dict, fetch_list, target_list, run_metadata)
1406 self._session, options, feed_dict, fetch_list, target_list,
-> 1407 run_metadata)
1408
FailedPreconditionError: Attempting to use uninitialized value b2
[[{{node b2/read}} = Identity[T=DT_DOUBLE, _device="/job:localhost/replica:0/task:0/device:CPU:0"](b2)]]
python tensorflow
For newer tensorflow versions you should usetf.global_variables_initializer()
. Does that work?
– Tobias Ernst
Nov 19 at 23:40
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to write a program in python using tensorflow to fit data with different patterns using a neural network with a hidden layer. I am facing an error with the code which states that the variable b2 is uninitialized. But I have initialized it already and dont understand what I am missing here.
This is part of an assignment (the dataset can be understood here) and got stuck here while solving it.
The link to the colab notebook is here.
The initialization lines look like
W1 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(hidden, 2)), name="W1")
b1 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(hidden, 1)), name="b1")
W2 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(classes, hidden)), name="W2")
b2 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(classes, 1)), name="b2")
The code snippet that shows the computation graph is below.
operation = "ReLU" # "Sigmoid"
o = tf.add(tf.matmul(W1, p), b1)
# ReLU or Sigmoid
if operation == "ReLU":
z = tf.zeros((hidden, 1), dtype=tf.float64)
output = tf.maximum(o, z)
else:
output = tf.sigmoid(o)
foutput = tf.add(tf.matmul(W2, output), b2)
crossentropy = tf.log(tf.exp(foutput) / tf.reduce_sum(tf.exp(foutput), 0))
init = tf.initialize_all_variables()
with tf.Session() as sess:
ce = sess.run([crossentropy], feed_dict={p : inputs, t : targets, lr : 0.01})
print(ce)
Error message
FailedPreconditionError Traceback (most recent call last)
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _do_call(self, fn, *args)
1333 try:
-> 1334 return fn(*args)
1335 except errors.OpError as e:
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _run_fn(feed_dict, fetch_list, target_list, options, run_metadata)
1318 return self._call_tf_sessionrun(
-> 1319 options, feed_dict, fetch_list, target_list, run_metadata)
1320
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _call_tf_sessionrun(self, options, feed_dict, fetch_list, target_list, run_metadata)
1406 self._session, options, feed_dict, fetch_list, target_list,
-> 1407 run_metadata)
1408
FailedPreconditionError: Attempting to use uninitialized value b2
[[{{node b2/read}} = Identity[T=DT_DOUBLE, _device="/job:localhost/replica:0/task:0/device:CPU:0"](b2)]]
python tensorflow
I am trying to write a program in python using tensorflow to fit data with different patterns using a neural network with a hidden layer. I am facing an error with the code which states that the variable b2 is uninitialized. But I have initialized it already and dont understand what I am missing here.
This is part of an assignment (the dataset can be understood here) and got stuck here while solving it.
The link to the colab notebook is here.
The initialization lines look like
W1 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(hidden, 2)), name="W1")
b1 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(hidden, 1)), name="b1")
W2 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(classes, hidden)), name="W2")
b2 = tf.Variable(np.random.uniform(low=-0.01, high=0.01, size=(classes, 1)), name="b2")
The code snippet that shows the computation graph is below.
operation = "ReLU" # "Sigmoid"
o = tf.add(tf.matmul(W1, p), b1)
# ReLU or Sigmoid
if operation == "ReLU":
z = tf.zeros((hidden, 1), dtype=tf.float64)
output = tf.maximum(o, z)
else:
output = tf.sigmoid(o)
foutput = tf.add(tf.matmul(W2, output), b2)
crossentropy = tf.log(tf.exp(foutput) / tf.reduce_sum(tf.exp(foutput), 0))
init = tf.initialize_all_variables()
with tf.Session() as sess:
ce = sess.run([crossentropy], feed_dict={p : inputs, t : targets, lr : 0.01})
print(ce)
Error message
FailedPreconditionError Traceback (most recent call last)
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _do_call(self, fn, *args)
1333 try:
-> 1334 return fn(*args)
1335 except errors.OpError as e:
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _run_fn(feed_dict, fetch_list, target_list, options, run_metadata)
1318 return self._call_tf_sessionrun(
-> 1319 options, feed_dict, fetch_list, target_list, run_metadata)
1320
/usr/local/lib/python3.6/dist-packages/tensorflow/python/client/session.py in _call_tf_sessionrun(self, options, feed_dict, fetch_list, target_list, run_metadata)
1406 self._session, options, feed_dict, fetch_list, target_list,
-> 1407 run_metadata)
1408
FailedPreconditionError: Attempting to use uninitialized value b2
[[{{node b2/read}} = Identity[T=DT_DOUBLE, _device="/job:localhost/replica:0/task:0/device:CPU:0"](b2)]]
python tensorflow
python tensorflow
asked Nov 19 at 23:35
suraj
52
52
For newer tensorflow versions you should usetf.global_variables_initializer()
. Does that work?
– Tobias Ernst
Nov 19 at 23:40
add a comment |
For newer tensorflow versions you should usetf.global_variables_initializer()
. Does that work?
– Tobias Ernst
Nov 19 at 23:40
For newer tensorflow versions you should use
tf.global_variables_initializer()
. Does that work?– Tobias Ernst
Nov 19 at 23:40
For newer tensorflow versions you should use
tf.global_variables_initializer()
. Does that work?– Tobias Ernst
Nov 19 at 23:40
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
You also need to run the init function in the graph before computing cross entropy:
with tf.Session() as sess:
sess.run(init)
ce = sess.run([crossentropy], feed_dict={p : inputs, t : targets, lr : 0.01})
Also, tf.initialize_all_variables()
is deprecated. Use tf.global_variables_initializer()
instead.
Thank you. This solution works for me. Does it mean that I cant initialize variables outside a session ?
– suraj
Nov 20 at 0:08
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You also need to run the init function in the graph before computing cross entropy:
with tf.Session() as sess:
sess.run(init)
ce = sess.run([crossentropy], feed_dict={p : inputs, t : targets, lr : 0.01})
Also, tf.initialize_all_variables()
is deprecated. Use tf.global_variables_initializer()
instead.
Thank you. This solution works for me. Does it mean that I cant initialize variables outside a session ?
– suraj
Nov 20 at 0:08
add a comment |
up vote
0
down vote
accepted
You also need to run the init function in the graph before computing cross entropy:
with tf.Session() as sess:
sess.run(init)
ce = sess.run([crossentropy], feed_dict={p : inputs, t : targets, lr : 0.01})
Also, tf.initialize_all_variables()
is deprecated. Use tf.global_variables_initializer()
instead.
Thank you. This solution works for me. Does it mean that I cant initialize variables outside a session ?
– suraj
Nov 20 at 0:08
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You also need to run the init function in the graph before computing cross entropy:
with tf.Session() as sess:
sess.run(init)
ce = sess.run([crossentropy], feed_dict={p : inputs, t : targets, lr : 0.01})
Also, tf.initialize_all_variables()
is deprecated. Use tf.global_variables_initializer()
instead.
You also need to run the init function in the graph before computing cross entropy:
with tf.Session() as sess:
sess.run(init)
ce = sess.run([crossentropy], feed_dict={p : inputs, t : targets, lr : 0.01})
Also, tf.initialize_all_variables()
is deprecated. Use tf.global_variables_initializer()
instead.
answered Nov 19 at 23:40


Gerges Dib
2,7231719
2,7231719
Thank you. This solution works for me. Does it mean that I cant initialize variables outside a session ?
– suraj
Nov 20 at 0:08
add a comment |
Thank you. This solution works for me. Does it mean that I cant initialize variables outside a session ?
– suraj
Nov 20 at 0:08
Thank you. This solution works for me. Does it mean that I cant initialize variables outside a session ?
– suraj
Nov 20 at 0:08
Thank you. This solution works for me. Does it mean that I cant initialize variables outside a session ?
– suraj
Nov 20 at 0:08
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384184%2ftensor-flow-throws-an-uninitialized-error-even-after-the-variable-is-initialized%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1mEjotZ eVyCZ71Yb,O7OjyMmZhLDR8EQEwOdbZriaa0ko
For newer tensorflow versions you should use
tf.global_variables_initializer()
. Does that work?– Tobias Ernst
Nov 19 at 23:40