Nested string interpolation
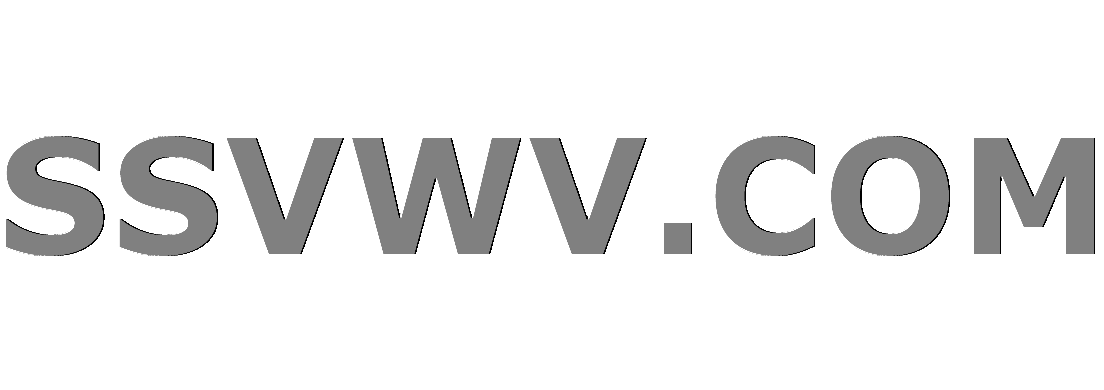
Multi tool use
up vote
0
down vote
favorite
I've faced an issue with nested string interpolation in C# 6.
For example, there is a string:
string test = "StartText MiddleText1 MiddleText2 EndText";
If I want to apply ToUpper() method for MiddleText1 only, I can do this way:
string test = $@"StartText {"MiddleText1".ToUpper()} MiddleText2 EndText";
But what if I want to apply a string method, for example Replace() for this part of string:
{"Middletext1".ToUpper()} MiddleText2
I expected that something like this will work:
string test = $@"StartText {"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
But this syntax is wrong - I've tried a lot variations, played with quotas but I couldn't get correct syntax for this purpose.
I'd wish to not split the string in a different parts. Is there a way to solve it using interpolation feature only?
c# string interpolation
add a comment |
up vote
0
down vote
favorite
I've faced an issue with nested string interpolation in C# 6.
For example, there is a string:
string test = "StartText MiddleText1 MiddleText2 EndText";
If I want to apply ToUpper() method for MiddleText1 only, I can do this way:
string test = $@"StartText {"MiddleText1".ToUpper()} MiddleText2 EndText";
But what if I want to apply a string method, for example Replace() for this part of string:
{"Middletext1".ToUpper()} MiddleText2
I expected that something like this will work:
string test = $@"StartText {"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
But this syntax is wrong - I've tried a lot variations, played with quotas but I couldn't get correct syntax for this purpose.
I'd wish to not split the string in a different parts. Is there a way to solve it using interpolation feature only?
c# string interpolation
2
Just shooting from the hip here...string test = $@"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
... that said, this looks just painful to read and really defeats the purpose of string interpolation.
– Glorin Oakenfoot
Nov 19 at 23:35
2
Stop trying to do everything in one line, if you broke this apart you would know the problem
– TheGeneral
Nov 19 at 23:42
Thank Glorin, nice shot. And could you suggest another way for this task without such bad readability - to involve interim variable?
– Vladimir
Nov 19 at 23:45
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I've faced an issue with nested string interpolation in C# 6.
For example, there is a string:
string test = "StartText MiddleText1 MiddleText2 EndText";
If I want to apply ToUpper() method for MiddleText1 only, I can do this way:
string test = $@"StartText {"MiddleText1".ToUpper()} MiddleText2 EndText";
But what if I want to apply a string method, for example Replace() for this part of string:
{"Middletext1".ToUpper()} MiddleText2
I expected that something like this will work:
string test = $@"StartText {"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
But this syntax is wrong - I've tried a lot variations, played with quotas but I couldn't get correct syntax for this purpose.
I'd wish to not split the string in a different parts. Is there a way to solve it using interpolation feature only?
c# string interpolation
I've faced an issue with nested string interpolation in C# 6.
For example, there is a string:
string test = "StartText MiddleText1 MiddleText2 EndText";
If I want to apply ToUpper() method for MiddleText1 only, I can do this way:
string test = $@"StartText {"MiddleText1".ToUpper()} MiddleText2 EndText";
But what if I want to apply a string method, for example Replace() for this part of string:
{"Middletext1".ToUpper()} MiddleText2
I expected that something like this will work:
string test = $@"StartText {"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
But this syntax is wrong - I've tried a lot variations, played with quotas but I couldn't get correct syntax for this purpose.
I'd wish to not split the string in a different parts. Is there a way to solve it using interpolation feature only?
c# string interpolation
c# string interpolation
asked Nov 19 at 23:31
Vladimir
129211
129211
2
Just shooting from the hip here...string test = $@"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
... that said, this looks just painful to read and really defeats the purpose of string interpolation.
– Glorin Oakenfoot
Nov 19 at 23:35
2
Stop trying to do everything in one line, if you broke this apart you would know the problem
– TheGeneral
Nov 19 at 23:42
Thank Glorin, nice shot. And could you suggest another way for this task without such bad readability - to involve interim variable?
– Vladimir
Nov 19 at 23:45
add a comment |
2
Just shooting from the hip here...string test = $@"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
... that said, this looks just painful to read and really defeats the purpose of string interpolation.
– Glorin Oakenfoot
Nov 19 at 23:35
2
Stop trying to do everything in one line, if you broke this apart you would know the problem
– TheGeneral
Nov 19 at 23:42
Thank Glorin, nice shot. And could you suggest another way for this task without such bad readability - to involve interim variable?
– Vladimir
Nov 19 at 23:45
2
2
Just shooting from the hip here...
string test = $@"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
... that said, this looks just painful to read and really defeats the purpose of string interpolation.– Glorin Oakenfoot
Nov 19 at 23:35
Just shooting from the hip here...
string test = $@"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
... that said, this looks just painful to read and really defeats the purpose of string interpolation.– Glorin Oakenfoot
Nov 19 at 23:35
2
2
Stop trying to do everything in one line, if you broke this apart you would know the problem
– TheGeneral
Nov 19 at 23:42
Stop trying to do everything in one line, if you broke this apart you would know the problem
– TheGeneral
Nov 19 at 23:42
Thank Glorin, nice shot. And could you suggest another way for this task without such bad readability - to involve interim variable?
– Vladimir
Nov 19 at 23:45
Thank Glorin, nice shot. And could you suggest another way for this task without such bad readability - to involve interim variable?
– Vladimir
Nov 19 at 23:45
add a comment |
1 Answer
1
active
oldest
votes
up vote
6
down vote
accepted
Stop trying to do everything in one line is my suggestion
The following is the answer
var middle = "MiddleText1";
middle = middle.ToUpper();
var middle2 = $"{middle} MiddleText2";
middle2 = middle2.Replace("x", "y");
string test = $"StartText {middle2} EndText";
Which, when you add it all together.
string test = $"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
In short, you were just missing a $
However, Even this is messy as i am not sure what all the replaces are for, where this text comes from, and what the issue is you are trying to solve
This is good approach, but original text is large and I need to make many similar method invocations, that's why I tried to get one-line solution to avoid creating a lot of additional variables.
– Vladimir
Nov 19 at 23:54
1
@Vladimir that's fair enough, however you were just missing a$
– TheGeneral
Nov 19 at 23:54
Actually this question came from work with some kind of "html page builder". It uses string extension methods to create html page from some volume of text, returning inner strings embedded in different html tags. For example, it will return some parts in <td> tag, then result embedded in <tr>, then result in <table> tag etc. Now I see It will be really hard readable. Maybe you could suggest another approach?
– Vladimir
Nov 20 at 0:04
1
@Vladimir codereview.stackexchange.com
– TheGeneral
Nov 20 at 0:18
1
@Vladimir: Generally the only thing you get for squeezing a lot of operations into a single line, is a horrible time debugging. Because you can not figure out wich of those 3-10 operations is even causing the issue. Do not be to worried about performance. The JiT is pretty good at cutting out dead code and adding/removing Temporary variables in Release builds to improove performance.
– Christopher
Nov 20 at 0:48
|
show 3 more comments
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
6
down vote
accepted
Stop trying to do everything in one line is my suggestion
The following is the answer
var middle = "MiddleText1";
middle = middle.ToUpper();
var middle2 = $"{middle} MiddleText2";
middle2 = middle2.Replace("x", "y");
string test = $"StartText {middle2} EndText";
Which, when you add it all together.
string test = $"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
In short, you were just missing a $
However, Even this is messy as i am not sure what all the replaces are for, where this text comes from, and what the issue is you are trying to solve
This is good approach, but original text is large and I need to make many similar method invocations, that's why I tried to get one-line solution to avoid creating a lot of additional variables.
– Vladimir
Nov 19 at 23:54
1
@Vladimir that's fair enough, however you were just missing a$
– TheGeneral
Nov 19 at 23:54
Actually this question came from work with some kind of "html page builder". It uses string extension methods to create html page from some volume of text, returning inner strings embedded in different html tags. For example, it will return some parts in <td> tag, then result embedded in <tr>, then result in <table> tag etc. Now I see It will be really hard readable. Maybe you could suggest another approach?
– Vladimir
Nov 20 at 0:04
1
@Vladimir codereview.stackexchange.com
– TheGeneral
Nov 20 at 0:18
1
@Vladimir: Generally the only thing you get for squeezing a lot of operations into a single line, is a horrible time debugging. Because you can not figure out wich of those 3-10 operations is even causing the issue. Do not be to worried about performance. The JiT is pretty good at cutting out dead code and adding/removing Temporary variables in Release builds to improove performance.
– Christopher
Nov 20 at 0:48
|
show 3 more comments
up vote
6
down vote
accepted
Stop trying to do everything in one line is my suggestion
The following is the answer
var middle = "MiddleText1";
middle = middle.ToUpper();
var middle2 = $"{middle} MiddleText2";
middle2 = middle2.Replace("x", "y");
string test = $"StartText {middle2} EndText";
Which, when you add it all together.
string test = $"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
In short, you were just missing a $
However, Even this is messy as i am not sure what all the replaces are for, where this text comes from, and what the issue is you are trying to solve
This is good approach, but original text is large and I need to make many similar method invocations, that's why I tried to get one-line solution to avoid creating a lot of additional variables.
– Vladimir
Nov 19 at 23:54
1
@Vladimir that's fair enough, however you were just missing a$
– TheGeneral
Nov 19 at 23:54
Actually this question came from work with some kind of "html page builder". It uses string extension methods to create html page from some volume of text, returning inner strings embedded in different html tags. For example, it will return some parts in <td> tag, then result embedded in <tr>, then result in <table> tag etc. Now I see It will be really hard readable. Maybe you could suggest another approach?
– Vladimir
Nov 20 at 0:04
1
@Vladimir codereview.stackexchange.com
– TheGeneral
Nov 20 at 0:18
1
@Vladimir: Generally the only thing you get for squeezing a lot of operations into a single line, is a horrible time debugging. Because you can not figure out wich of those 3-10 operations is even causing the issue. Do not be to worried about performance. The JiT is pretty good at cutting out dead code and adding/removing Temporary variables in Release builds to improove performance.
– Christopher
Nov 20 at 0:48
|
show 3 more comments
up vote
6
down vote
accepted
up vote
6
down vote
accepted
Stop trying to do everything in one line is my suggestion
The following is the answer
var middle = "MiddleText1";
middle = middle.ToUpper();
var middle2 = $"{middle} MiddleText2";
middle2 = middle2.Replace("x", "y");
string test = $"StartText {middle2} EndText";
Which, when you add it all together.
string test = $"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
In short, you were just missing a $
However, Even this is messy as i am not sure what all the replaces are for, where this text comes from, and what the issue is you are trying to solve
Stop trying to do everything in one line is my suggestion
The following is the answer
var middle = "MiddleText1";
middle = middle.ToUpper();
var middle2 = $"{middle} MiddleText2";
middle2 = middle2.Replace("x", "y");
string test = $"StartText {middle2} EndText";
Which, when you add it all together.
string test = $"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
In short, you were just missing a $
However, Even this is messy as i am not sure what all the replaces are for, where this text comes from, and what the issue is you are trying to solve
edited Nov 20 at 0:06
answered Nov 19 at 23:46


TheGeneral
26.4k63163
26.4k63163
This is good approach, but original text is large and I need to make many similar method invocations, that's why I tried to get one-line solution to avoid creating a lot of additional variables.
– Vladimir
Nov 19 at 23:54
1
@Vladimir that's fair enough, however you were just missing a$
– TheGeneral
Nov 19 at 23:54
Actually this question came from work with some kind of "html page builder". It uses string extension methods to create html page from some volume of text, returning inner strings embedded in different html tags. For example, it will return some parts in <td> tag, then result embedded in <tr>, then result in <table> tag etc. Now I see It will be really hard readable. Maybe you could suggest another approach?
– Vladimir
Nov 20 at 0:04
1
@Vladimir codereview.stackexchange.com
– TheGeneral
Nov 20 at 0:18
1
@Vladimir: Generally the only thing you get for squeezing a lot of operations into a single line, is a horrible time debugging. Because you can not figure out wich of those 3-10 operations is even causing the issue. Do not be to worried about performance. The JiT is pretty good at cutting out dead code and adding/removing Temporary variables in Release builds to improove performance.
– Christopher
Nov 20 at 0:48
|
show 3 more comments
This is good approach, but original text is large and I need to make many similar method invocations, that's why I tried to get one-line solution to avoid creating a lot of additional variables.
– Vladimir
Nov 19 at 23:54
1
@Vladimir that's fair enough, however you were just missing a$
– TheGeneral
Nov 19 at 23:54
Actually this question came from work with some kind of "html page builder". It uses string extension methods to create html page from some volume of text, returning inner strings embedded in different html tags. For example, it will return some parts in <td> tag, then result embedded in <tr>, then result in <table> tag etc. Now I see It will be really hard readable. Maybe you could suggest another approach?
– Vladimir
Nov 20 at 0:04
1
@Vladimir codereview.stackexchange.com
– TheGeneral
Nov 20 at 0:18
1
@Vladimir: Generally the only thing you get for squeezing a lot of operations into a single line, is a horrible time debugging. Because you can not figure out wich of those 3-10 operations is even causing the issue. Do not be to worried about performance. The JiT is pretty good at cutting out dead code and adding/removing Temporary variables in Release builds to improove performance.
– Christopher
Nov 20 at 0:48
This is good approach, but original text is large and I need to make many similar method invocations, that's why I tried to get one-line solution to avoid creating a lot of additional variables.
– Vladimir
Nov 19 at 23:54
This is good approach, but original text is large and I need to make many similar method invocations, that's why I tried to get one-line solution to avoid creating a lot of additional variables.
– Vladimir
Nov 19 at 23:54
1
1
@Vladimir that's fair enough, however you were just missing a
$
– TheGeneral
Nov 19 at 23:54
@Vladimir that's fair enough, however you were just missing a
$
– TheGeneral
Nov 19 at 23:54
Actually this question came from work with some kind of "html page builder". It uses string extension methods to create html page from some volume of text, returning inner strings embedded in different html tags. For example, it will return some parts in <td> tag, then result embedded in <tr>, then result in <table> tag etc. Now I see It will be really hard readable. Maybe you could suggest another approach?
– Vladimir
Nov 20 at 0:04
Actually this question came from work with some kind of "html page builder". It uses string extension methods to create html page from some volume of text, returning inner strings embedded in different html tags. For example, it will return some parts in <td> tag, then result embedded in <tr>, then result in <table> tag etc. Now I see It will be really hard readable. Maybe you could suggest another approach?
– Vladimir
Nov 20 at 0:04
1
1
@Vladimir codereview.stackexchange.com
– TheGeneral
Nov 20 at 0:18
@Vladimir codereview.stackexchange.com
– TheGeneral
Nov 20 at 0:18
1
1
@Vladimir: Generally the only thing you get for squeezing a lot of operations into a single line, is a horrible time debugging. Because you can not figure out wich of those 3-10 operations is even causing the issue. Do not be to worried about performance. The JiT is pretty good at cutting out dead code and adding/removing Temporary variables in Release builds to improove performance.
– Christopher
Nov 20 at 0:48
@Vladimir: Generally the only thing you get for squeezing a lot of operations into a single line, is a horrible time debugging. Because you can not figure out wich of those 3-10 operations is even causing the issue. Do not be to worried about performance. The JiT is pretty good at cutting out dead code and adding/removing Temporary variables in Release builds to improove performance.
– Christopher
Nov 20 at 0:48
|
show 3 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384153%2fnested-string-interpolation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
A2hLo2Jf5nfLcisYMoLBerLSfT,wC,fwl y UYJqkGl bslsnLB2iFegQ,TIvE5JmL4gyLKc,JGnfwJeBb2Auw 7 bBS,iz30L,x2
2
Just shooting from the hip here...
string test = $@"StartText {$"{"MiddleText1".ToUpper()} MiddleText2".Replace("x", "y")} EndText";
... that said, this looks just painful to read and really defeats the purpose of string interpolation.– Glorin Oakenfoot
Nov 19 at 23:35
2
Stop trying to do everything in one line, if you broke this apart you would know the problem
– TheGeneral
Nov 19 at 23:42
Thank Glorin, nice shot. And could you suggest another way for this task without such bad readability - to involve interim variable?
– Vladimir
Nov 19 at 23:45