How do I create a new collection?
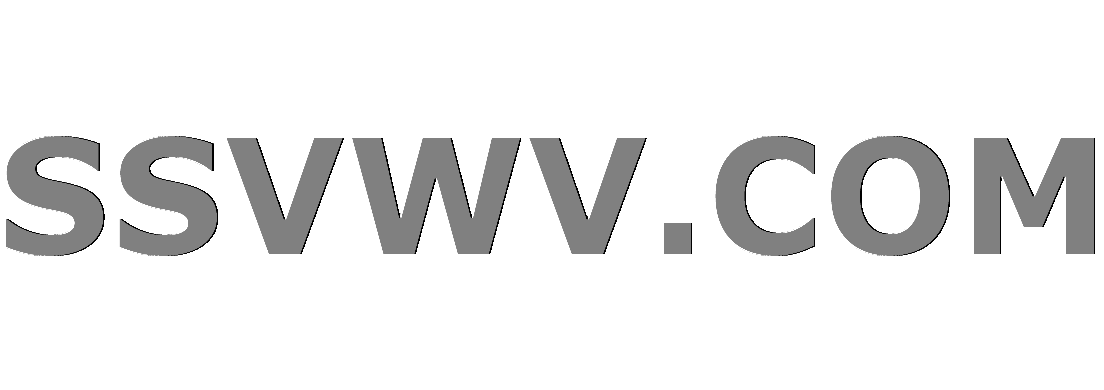
Multi tool use
up vote
1
down vote
favorite
I'm building a chat app where there are GroupConversations
and GroupMessages
. Following the example of some of the Firestore YouTube videos, I've chosen to structure my data this way:
The ID of the GroupConversation is the ID for the collection of GroupMessages associated with that GroupConversation. Then inside of that document should be a new collection called Messages
which holds documents.
Does that make sense or am I overcomplicating things? I can't seem to create that Messages
collection or set anything to it in my Swift code
GroupConversations
id
title
desc
memberIds
GroupMessages
GroupConversationID
createdAt: Date // just because I need a key and a document?
Messages (new collection)
Message1
Message2
Message3
Thanks
Edit: Added Swift code
let db = Firestore.firestore()
var data: [String: Any] = [
"text": title,
"senderUsername": username,
"createdAt": Date(),
"updatedAt": Date()
]
let creationData: [String: Any] = [
"text": "Group Created",
"createdAt": Date()
]
let doc = db.collection("GroupMessages").addDocument(data: creationData)
db.document("GroupMessages/(groupConvo.documentId)/(doc.documentID)/messages").setData(data) { error in
if error == nil {
completion()
}
}
In my console, I'm seeing a new GroupMessage object created but not sub-collection of that GroupMessage called messages
ios swift google-cloud-firestore
add a comment |
up vote
1
down vote
favorite
I'm building a chat app where there are GroupConversations
and GroupMessages
. Following the example of some of the Firestore YouTube videos, I've chosen to structure my data this way:
The ID of the GroupConversation is the ID for the collection of GroupMessages associated with that GroupConversation. Then inside of that document should be a new collection called Messages
which holds documents.
Does that make sense or am I overcomplicating things? I can't seem to create that Messages
collection or set anything to it in my Swift code
GroupConversations
id
title
desc
memberIds
GroupMessages
GroupConversationID
createdAt: Date // just because I need a key and a document?
Messages (new collection)
Message1
Message2
Message3
Thanks
Edit: Added Swift code
let db = Firestore.firestore()
var data: [String: Any] = [
"text": title,
"senderUsername": username,
"createdAt": Date(),
"updatedAt": Date()
]
let creationData: [String: Any] = [
"text": "Group Created",
"createdAt": Date()
]
let doc = db.collection("GroupMessages").addDocument(data: creationData)
db.document("GroupMessages/(groupConvo.documentId)/(doc.documentID)/messages").setData(data) { error in
if error == nil {
completion()
}
}
In my console, I'm seeing a new GroupMessage object created but not sub-collection of that GroupMessage called messages
ios swift google-cloud-firestore
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm building a chat app where there are GroupConversations
and GroupMessages
. Following the example of some of the Firestore YouTube videos, I've chosen to structure my data this way:
The ID of the GroupConversation is the ID for the collection of GroupMessages associated with that GroupConversation. Then inside of that document should be a new collection called Messages
which holds documents.
Does that make sense or am I overcomplicating things? I can't seem to create that Messages
collection or set anything to it in my Swift code
GroupConversations
id
title
desc
memberIds
GroupMessages
GroupConversationID
createdAt: Date // just because I need a key and a document?
Messages (new collection)
Message1
Message2
Message3
Thanks
Edit: Added Swift code
let db = Firestore.firestore()
var data: [String: Any] = [
"text": title,
"senderUsername": username,
"createdAt": Date(),
"updatedAt": Date()
]
let creationData: [String: Any] = [
"text": "Group Created",
"createdAt": Date()
]
let doc = db.collection("GroupMessages").addDocument(data: creationData)
db.document("GroupMessages/(groupConvo.documentId)/(doc.documentID)/messages").setData(data) { error in
if error == nil {
completion()
}
}
In my console, I'm seeing a new GroupMessage object created but not sub-collection of that GroupMessage called messages
ios swift google-cloud-firestore
I'm building a chat app where there are GroupConversations
and GroupMessages
. Following the example of some of the Firestore YouTube videos, I've chosen to structure my data this way:
The ID of the GroupConversation is the ID for the collection of GroupMessages associated with that GroupConversation. Then inside of that document should be a new collection called Messages
which holds documents.
Does that make sense or am I overcomplicating things? I can't seem to create that Messages
collection or set anything to it in my Swift code
GroupConversations
id
title
desc
memberIds
GroupMessages
GroupConversationID
createdAt: Date // just because I need a key and a document?
Messages (new collection)
Message1
Message2
Message3
Thanks
Edit: Added Swift code
let db = Firestore.firestore()
var data: [String: Any] = [
"text": title,
"senderUsername": username,
"createdAt": Date(),
"updatedAt": Date()
]
let creationData: [String: Any] = [
"text": "Group Created",
"createdAt": Date()
]
let doc = db.collection("GroupMessages").addDocument(data: creationData)
db.document("GroupMessages/(groupConvo.documentId)/(doc.documentID)/messages").setData(data) { error in
if error == nil {
completion()
}
}
In my console, I'm seeing a new GroupMessage object created but not sub-collection of that GroupMessage called messages
ios swift google-cloud-firestore
ios swift google-cloud-firestore
edited Nov 19 at 23:57
asked Nov 19 at 23:35
Zack Shapiro
1,09673981
1,09673981
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
You're not building the path to the document correctly. Document paths always alternate between collection and document names. To build a path to a document in a subcollection, it would go collection/document/subcollection/document. It looks like you've mixed up the last two elements in the string you're building.
In my opinion, it's harder to go wrong if you build up the document using methods rather than trying to build a string. Something like this:
db
.collection("GroupMessages")
.document(groupConvo.documentId)
.collection("Messages")
.document(doc.documentId)
.setData(...)
Ahhh, had those backwards. Yeah that build method is a lot better than my string gibberish. Thanks again Doug!
– Zack Shapiro
Nov 20 at 0:12
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You're not building the path to the document correctly. Document paths always alternate between collection and document names. To build a path to a document in a subcollection, it would go collection/document/subcollection/document. It looks like you've mixed up the last two elements in the string you're building.
In my opinion, it's harder to go wrong if you build up the document using methods rather than trying to build a string. Something like this:
db
.collection("GroupMessages")
.document(groupConvo.documentId)
.collection("Messages")
.document(doc.documentId)
.setData(...)
Ahhh, had those backwards. Yeah that build method is a lot better than my string gibberish. Thanks again Doug!
– Zack Shapiro
Nov 20 at 0:12
add a comment |
up vote
1
down vote
accepted
You're not building the path to the document correctly. Document paths always alternate between collection and document names. To build a path to a document in a subcollection, it would go collection/document/subcollection/document. It looks like you've mixed up the last two elements in the string you're building.
In my opinion, it's harder to go wrong if you build up the document using methods rather than trying to build a string. Something like this:
db
.collection("GroupMessages")
.document(groupConvo.documentId)
.collection("Messages")
.document(doc.documentId)
.setData(...)
Ahhh, had those backwards. Yeah that build method is a lot better than my string gibberish. Thanks again Doug!
– Zack Shapiro
Nov 20 at 0:12
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You're not building the path to the document correctly. Document paths always alternate between collection and document names. To build a path to a document in a subcollection, it would go collection/document/subcollection/document. It looks like you've mixed up the last two elements in the string you're building.
In my opinion, it's harder to go wrong if you build up the document using methods rather than trying to build a string. Something like this:
db
.collection("GroupMessages")
.document(groupConvo.documentId)
.collection("Messages")
.document(doc.documentId)
.setData(...)
You're not building the path to the document correctly. Document paths always alternate between collection and document names. To build a path to a document in a subcollection, it would go collection/document/subcollection/document. It looks like you've mixed up the last two elements in the string you're building.
In my opinion, it's harder to go wrong if you build up the document using methods rather than trying to build a string. Something like this:
db
.collection("GroupMessages")
.document(groupConvo.documentId)
.collection("Messages")
.document(doc.documentId)
.setData(...)
answered Nov 20 at 0:05


Doug Stevenson
66.6k87997
66.6k87997
Ahhh, had those backwards. Yeah that build method is a lot better than my string gibberish. Thanks again Doug!
– Zack Shapiro
Nov 20 at 0:12
add a comment |
Ahhh, had those backwards. Yeah that build method is a lot better than my string gibberish. Thanks again Doug!
– Zack Shapiro
Nov 20 at 0:12
Ahhh, had those backwards. Yeah that build method is a lot better than my string gibberish. Thanks again Doug!
– Zack Shapiro
Nov 20 at 0:12
Ahhh, had those backwards. Yeah that build method is a lot better than my string gibberish. Thanks again Doug!
– Zack Shapiro
Nov 20 at 0:12
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384187%2fhow-do-i-create-a-new-collection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0POVE2m5 LfwGoX,W2XpPVJelbzNGoQ8vOjs1abiRo,Nd9C6m98n lC 2fJjaRnBOyTY1 1uf4cRutJPT4Mt12AAg6FowZST 8 U