angularjs update button text based $http responses ng-click
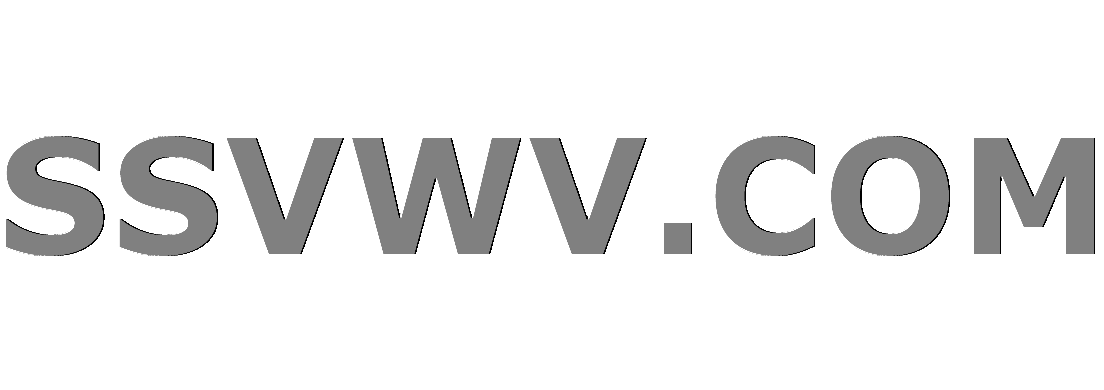
Multi tool use
up vote
0
down vote
favorite
I'm trying to update button value on-click based $http responses. I want to change button value to Processing... while making $http request with ng-click function. And based on Success/Error response, need to update text Success and Try Again etc.
I'm using ui-router and I've different form elements distributed in different states, e.g. Email, User, Password etc.
In my View, I've following code in Email State.
<div ng-controller="RegisterEmailController">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ErrorMsg}}</span>
</div>
<button type="button" ng-click="RegisterEmailController.nexStep()">{{buttonTxt}}</button>
</div>
In my Controller
var controller = this;
$scope.buttonTxt = 'Next Step';
controller.nexStep = function() {
$scope.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
$scope.buttonTxt = 'Success';
}, function error(response) {
$scope.buttonTxt = 'Try Again';
return;
});
} else {
$scope.buttonTxt = 'Try Again';
return;
}
}
With above code i can't be able to change button text on click, i tried with controller.buttonTxt
as well but it doesn't print value at all on init. I also tried ng-bind directive but no luck.
Please assist me where I'm doing wrong. As far as i can estimate that $scope
might conflicting with this
(var controller = this;) in controller.
Also how can i add success/error class to input parent element based on response?
Thanks for reading my query and i appreciate your help in advance.
javascript angularjs
add a comment |
up vote
0
down vote
favorite
I'm trying to update button value on-click based $http responses. I want to change button value to Processing... while making $http request with ng-click function. And based on Success/Error response, need to update text Success and Try Again etc.
I'm using ui-router and I've different form elements distributed in different states, e.g. Email, User, Password etc.
In my View, I've following code in Email State.
<div ng-controller="RegisterEmailController">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ErrorMsg}}</span>
</div>
<button type="button" ng-click="RegisterEmailController.nexStep()">{{buttonTxt}}</button>
</div>
In my Controller
var controller = this;
$scope.buttonTxt = 'Next Step';
controller.nexStep = function() {
$scope.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
$scope.buttonTxt = 'Success';
}, function error(response) {
$scope.buttonTxt = 'Try Again';
return;
});
} else {
$scope.buttonTxt = 'Try Again';
return;
}
}
With above code i can't be able to change button text on click, i tried with controller.buttonTxt
as well but it doesn't print value at all on init. I also tried ng-bind directive but no luck.
Please assist me where I'm doing wrong. As far as i can estimate that $scope
might conflicting with this
(var controller = this;) in controller.
Also how can i add success/error class to input parent element based on response?
Thanks for reading my query and i appreciate your help in advance.
javascript angularjs
1
You need to assign a controller alias inng-controller
when not using $scope
– charlietfl
Nov 19 at 23:30
Can u make a plunkr with the code?
– ppollono
Nov 19 at 23:55
Thanks @charlietfl, by assinging controller alias my problem is resolved, though have to fix couple of more things.
– Umair Razzaq
Nov 20 at 5:37
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to update button value on-click based $http responses. I want to change button value to Processing... while making $http request with ng-click function. And based on Success/Error response, need to update text Success and Try Again etc.
I'm using ui-router and I've different form elements distributed in different states, e.g. Email, User, Password etc.
In my View, I've following code in Email State.
<div ng-controller="RegisterEmailController">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ErrorMsg}}</span>
</div>
<button type="button" ng-click="RegisterEmailController.nexStep()">{{buttonTxt}}</button>
</div>
In my Controller
var controller = this;
$scope.buttonTxt = 'Next Step';
controller.nexStep = function() {
$scope.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
$scope.buttonTxt = 'Success';
}, function error(response) {
$scope.buttonTxt = 'Try Again';
return;
});
} else {
$scope.buttonTxt = 'Try Again';
return;
}
}
With above code i can't be able to change button text on click, i tried with controller.buttonTxt
as well but it doesn't print value at all on init. I also tried ng-bind directive but no luck.
Please assist me where I'm doing wrong. As far as i can estimate that $scope
might conflicting with this
(var controller = this;) in controller.
Also how can i add success/error class to input parent element based on response?
Thanks for reading my query and i appreciate your help in advance.
javascript angularjs
I'm trying to update button value on-click based $http responses. I want to change button value to Processing... while making $http request with ng-click function. And based on Success/Error response, need to update text Success and Try Again etc.
I'm using ui-router and I've different form elements distributed in different states, e.g. Email, User, Password etc.
In my View, I've following code in Email State.
<div ng-controller="RegisterEmailController">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ErrorMsg}}</span>
</div>
<button type="button" ng-click="RegisterEmailController.nexStep()">{{buttonTxt}}</button>
</div>
In my Controller
var controller = this;
$scope.buttonTxt = 'Next Step';
controller.nexStep = function() {
$scope.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
$scope.buttonTxt = 'Success';
}, function error(response) {
$scope.buttonTxt = 'Try Again';
return;
});
} else {
$scope.buttonTxt = 'Try Again';
return;
}
}
With above code i can't be able to change button text on click, i tried with controller.buttonTxt
as well but it doesn't print value at all on init. I also tried ng-bind directive but no luck.
Please assist me where I'm doing wrong. As far as i can estimate that $scope
might conflicting with this
(var controller = this;) in controller.
Also how can i add success/error class to input parent element based on response?
Thanks for reading my query and i appreciate your help in advance.
javascript angularjs
javascript angularjs
asked Nov 19 at 23:18
Umair Razzaq
107215
107215
1
You need to assign a controller alias inng-controller
when not using $scope
– charlietfl
Nov 19 at 23:30
Can u make a plunkr with the code?
– ppollono
Nov 19 at 23:55
Thanks @charlietfl, by assinging controller alias my problem is resolved, though have to fix couple of more things.
– Umair Razzaq
Nov 20 at 5:37
add a comment |
1
You need to assign a controller alias inng-controller
when not using $scope
– charlietfl
Nov 19 at 23:30
Can u make a plunkr with the code?
– ppollono
Nov 19 at 23:55
Thanks @charlietfl, by assinging controller alias my problem is resolved, though have to fix couple of more things.
– Umair Razzaq
Nov 20 at 5:37
1
1
You need to assign a controller alias in
ng-controller
when not using $scope– charlietfl
Nov 19 at 23:30
You need to assign a controller alias in
ng-controller
when not using $scope– charlietfl
Nov 19 at 23:30
Can u make a plunkr with the code?
– ppollono
Nov 19 at 23:55
Can u make a plunkr with the code?
– ppollono
Nov 19 at 23:55
Thanks @charlietfl, by assinging controller alias my problem is resolved, though have to fix couple of more things.
– Umair Razzaq
Nov 20 at 5:37
Thanks @charlietfl, by assinging controller alias my problem is resolved, though have to fix couple of more things.
– Umair Razzaq
Nov 20 at 5:37
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
Try this
var controller = function ($http) {
var validation_conditions
var ApiURL = 'google.com'
var getData = ''
this.buttonTxt = 'Next Step';
this.nexStep = function() {
this.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
this.buttonTxt = 'Success';
}, function error(response) {
this.buttonTxt = 'Try Again';
return;
});
} else {
this.buttonTxt = 'Try Again';
return;
}
}
}
controller.$inject = ['$http']
angular
.module('app', )
.controller('RegisterEmailController',controller);
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.23/angular.min.js"></script>
<div ng-app="app" ng-controller="RegisterEmailController as ctrl">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ctrl.ErrorMsg}}</span>
</div>
<button type="button" ng-click="ctrl.nexStep()">{{ctrl.buttonTxt}}</button>
</div>
Thanks @ppollono, my issue is resolved. Further can you please assist how can i add valid/error class to input based on success/error responses?
– Umair Razzaq
Nov 20 at 5:42
To be able to help you with this you need an endpoint to get the responses (success/error)
– ppollono
Nov 20 at 11:03
yes you're right, i was able to add conditional classes with the response i get. Thanks for following up (thumbs-up)
– Umair Razzaq
Nov 21 at 13:33
add a comment |
up vote
1
down vote
Try to use:
<div ng-controller="RegisterEmailController as $registerEmail">
and then in button:
<button type="button" ng-click="RegisterEmailController.nexStep()">{{$registerEmail.buttonTxt}}</button>
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Try this
var controller = function ($http) {
var validation_conditions
var ApiURL = 'google.com'
var getData = ''
this.buttonTxt = 'Next Step';
this.nexStep = function() {
this.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
this.buttonTxt = 'Success';
}, function error(response) {
this.buttonTxt = 'Try Again';
return;
});
} else {
this.buttonTxt = 'Try Again';
return;
}
}
}
controller.$inject = ['$http']
angular
.module('app', )
.controller('RegisterEmailController',controller);
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.23/angular.min.js"></script>
<div ng-app="app" ng-controller="RegisterEmailController as ctrl">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ctrl.ErrorMsg}}</span>
</div>
<button type="button" ng-click="ctrl.nexStep()">{{ctrl.buttonTxt}}</button>
</div>
Thanks @ppollono, my issue is resolved. Further can you please assist how can i add valid/error class to input based on success/error responses?
– Umair Razzaq
Nov 20 at 5:42
To be able to help you with this you need an endpoint to get the responses (success/error)
– ppollono
Nov 20 at 11:03
yes you're right, i was able to add conditional classes with the response i get. Thanks for following up (thumbs-up)
– Umair Razzaq
Nov 21 at 13:33
add a comment |
up vote
1
down vote
accepted
Try this
var controller = function ($http) {
var validation_conditions
var ApiURL = 'google.com'
var getData = ''
this.buttonTxt = 'Next Step';
this.nexStep = function() {
this.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
this.buttonTxt = 'Success';
}, function error(response) {
this.buttonTxt = 'Try Again';
return;
});
} else {
this.buttonTxt = 'Try Again';
return;
}
}
}
controller.$inject = ['$http']
angular
.module('app', )
.controller('RegisterEmailController',controller);
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.23/angular.min.js"></script>
<div ng-app="app" ng-controller="RegisterEmailController as ctrl">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ctrl.ErrorMsg}}</span>
</div>
<button type="button" ng-click="ctrl.nexStep()">{{ctrl.buttonTxt}}</button>
</div>
Thanks @ppollono, my issue is resolved. Further can you please assist how can i add valid/error class to input based on success/error responses?
– Umair Razzaq
Nov 20 at 5:42
To be able to help you with this you need an endpoint to get the responses (success/error)
– ppollono
Nov 20 at 11:03
yes you're right, i was able to add conditional classes with the response i get. Thanks for following up (thumbs-up)
– Umair Razzaq
Nov 21 at 13:33
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Try this
var controller = function ($http) {
var validation_conditions
var ApiURL = 'google.com'
var getData = ''
this.buttonTxt = 'Next Step';
this.nexStep = function() {
this.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
this.buttonTxt = 'Success';
}, function error(response) {
this.buttonTxt = 'Try Again';
return;
});
} else {
this.buttonTxt = 'Try Again';
return;
}
}
}
controller.$inject = ['$http']
angular
.module('app', )
.controller('RegisterEmailController',controller);
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.23/angular.min.js"></script>
<div ng-app="app" ng-controller="RegisterEmailController as ctrl">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ctrl.ErrorMsg}}</span>
</div>
<button type="button" ng-click="ctrl.nexStep()">{{ctrl.buttonTxt}}</button>
</div>
Try this
var controller = function ($http) {
var validation_conditions
var ApiURL = 'google.com'
var getData = ''
this.buttonTxt = 'Next Step';
this.nexStep = function() {
this.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
this.buttonTxt = 'Success';
}, function error(response) {
this.buttonTxt = 'Try Again';
return;
});
} else {
this.buttonTxt = 'Try Again';
return;
}
}
}
controller.$inject = ['$http']
angular
.module('app', )
.controller('RegisterEmailController',controller);
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.23/angular.min.js"></script>
<div ng-app="app" ng-controller="RegisterEmailController as ctrl">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ctrl.ErrorMsg}}</span>
</div>
<button type="button" ng-click="ctrl.nexStep()">{{ctrl.buttonTxt}}</button>
</div>
var controller = function ($http) {
var validation_conditions
var ApiURL = 'google.com'
var getData = ''
this.buttonTxt = 'Next Step';
this.nexStep = function() {
this.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
this.buttonTxt = 'Success';
}, function error(response) {
this.buttonTxt = 'Try Again';
return;
});
} else {
this.buttonTxt = 'Try Again';
return;
}
}
}
controller.$inject = ['$http']
angular
.module('app', )
.controller('RegisterEmailController',controller);
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.23/angular.min.js"></script>
<div ng-app="app" ng-controller="RegisterEmailController as ctrl">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ctrl.ErrorMsg}}</span>
</div>
<button type="button" ng-click="ctrl.nexStep()">{{ctrl.buttonTxt}}</button>
</div>
var controller = function ($http) {
var validation_conditions
var ApiURL = 'google.com'
var getData = ''
this.buttonTxt = 'Next Step';
this.nexStep = function() {
this.buttonTxt = 'Processing...';
if ( validation_conditions ) {
$http({
method: "POST",
url: ApiURL,
headers: { "Content-Type": "application/json" },
data: getData
}).then(function success(response) {
this.buttonTxt = 'Success';
}, function error(response) {
this.buttonTxt = 'Try Again';
return;
});
} else {
this.buttonTxt = 'Try Again';
return;
}
}
}
controller.$inject = ['$http']
angular
.module('app', )
.controller('RegisterEmailController',controller);
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.23/angular.min.js"></script>
<div ng-app="app" ng-controller="RegisterEmailController as ctrl">
<div class="form-group" style="position: relative;" ng-class="{ 'has-error': form.useremail.$dirty && form.useremail.$error.required && form.useremail.$invalid }">
<input type="email" name="useremail" id="useremail" ng-model="RegisterEmailController.userDetail.useremail" ng-required="true">
<span ng-show="form.useremail.$dirty && form.useremail.$error.required" class="help-block text-sm text-danger pl-4">Oops! Incorrect email address, or field is empty</span>
<span ng-hide="form.useremail.$dirty && form.useremail.$error.required" class="help-block d-block text-sm text-danger px-4">{{ctrl.ErrorMsg}}</span>
</div>
<button type="button" ng-click="ctrl.nexStep()">{{ctrl.buttonTxt}}</button>
</div>
answered Nov 20 at 0:19
ppollono
2,36111425
2,36111425
Thanks @ppollono, my issue is resolved. Further can you please assist how can i add valid/error class to input based on success/error responses?
– Umair Razzaq
Nov 20 at 5:42
To be able to help you with this you need an endpoint to get the responses (success/error)
– ppollono
Nov 20 at 11:03
yes you're right, i was able to add conditional classes with the response i get. Thanks for following up (thumbs-up)
– Umair Razzaq
Nov 21 at 13:33
add a comment |
Thanks @ppollono, my issue is resolved. Further can you please assist how can i add valid/error class to input based on success/error responses?
– Umair Razzaq
Nov 20 at 5:42
To be able to help you with this you need an endpoint to get the responses (success/error)
– ppollono
Nov 20 at 11:03
yes you're right, i was able to add conditional classes with the response i get. Thanks for following up (thumbs-up)
– Umair Razzaq
Nov 21 at 13:33
Thanks @ppollono, my issue is resolved. Further can you please assist how can i add valid/error class to input based on success/error responses?
– Umair Razzaq
Nov 20 at 5:42
Thanks @ppollono, my issue is resolved. Further can you please assist how can i add valid/error class to input based on success/error responses?
– Umair Razzaq
Nov 20 at 5:42
To be able to help you with this you need an endpoint to get the responses (success/error)
– ppollono
Nov 20 at 11:03
To be able to help you with this you need an endpoint to get the responses (success/error)
– ppollono
Nov 20 at 11:03
yes you're right, i was able to add conditional classes with the response i get. Thanks for following up (thumbs-up)
– Umair Razzaq
Nov 21 at 13:33
yes you're right, i was able to add conditional classes with the response i get. Thanks for following up (thumbs-up)
– Umair Razzaq
Nov 21 at 13:33
add a comment |
up vote
1
down vote
Try to use:
<div ng-controller="RegisterEmailController as $registerEmail">
and then in button:
<button type="button" ng-click="RegisterEmailController.nexStep()">{{$registerEmail.buttonTxt}}</button>
add a comment |
up vote
1
down vote
Try to use:
<div ng-controller="RegisterEmailController as $registerEmail">
and then in button:
<button type="button" ng-click="RegisterEmailController.nexStep()">{{$registerEmail.buttonTxt}}</button>
add a comment |
up vote
1
down vote
up vote
1
down vote
Try to use:
<div ng-controller="RegisterEmailController as $registerEmail">
and then in button:
<button type="button" ng-click="RegisterEmailController.nexStep()">{{$registerEmail.buttonTxt}}</button>
Try to use:
<div ng-controller="RegisterEmailController as $registerEmail">
and then in button:
<button type="button" ng-click="RegisterEmailController.nexStep()">{{$registerEmail.buttonTxt}}</button>
answered Nov 19 at 23:50
uamanager
1557
1557
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384017%2fangularjs-update-button-text-based-http-responses-ng-click%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tRz3vq38,18Hi
1
You need to assign a controller alias in
ng-controller
when not using $scope– charlietfl
Nov 19 at 23:30
Can u make a plunkr with the code?
– ppollono
Nov 19 at 23:55
Thanks @charlietfl, by assinging controller alias my problem is resolved, though have to fix couple of more things.
– Umair Razzaq
Nov 20 at 5:37