How to condense Yup “when” validations
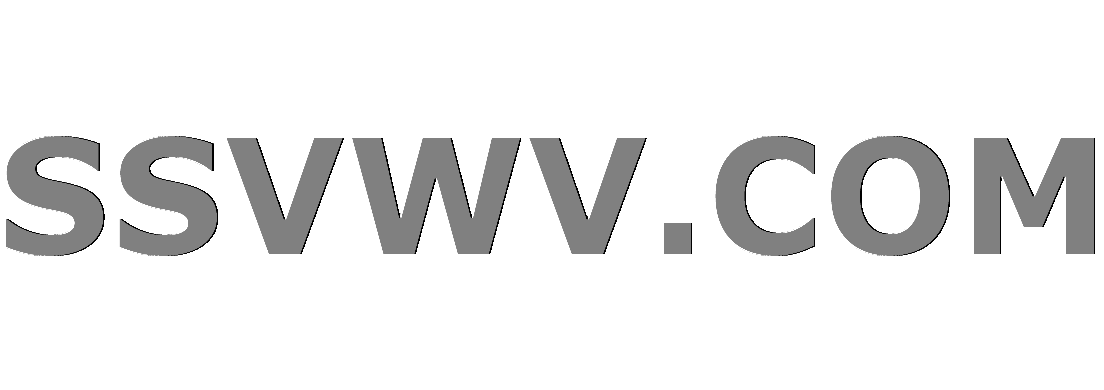
Multi tool use
up vote
0
down vote
favorite
I have several fields that are required if a single condition is true. Is there a better way to condense this code to avoid repeating the when
for all of these fields?
const requiredForDiffAddress = {
is: false,
then: Yup.string().required()
};
export const BillingAddressYupValidationSchemaShape = {
useShippingAddress: Yup.boolean().default(true).required(),
street: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
city: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
state: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
zipCode: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
};
Or for a more realistic and involved sample
const buildRequiredForDiffAddress = requiredText => ({
is: false,
then: Yup.string().required(requiredText)
});
export const BillingAddressYupValidationSchemaShape = {
useShippingAddress: Yup.boolean().default(true).required(),
street1: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("How will we know where to send your order?")),
city: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("What city do you live in?")),
state: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("State please!")),
zipCode: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("Zip Code please!")),
};
javascript validation yup
add a comment |
up vote
0
down vote
favorite
I have several fields that are required if a single condition is true. Is there a better way to condense this code to avoid repeating the when
for all of these fields?
const requiredForDiffAddress = {
is: false,
then: Yup.string().required()
};
export const BillingAddressYupValidationSchemaShape = {
useShippingAddress: Yup.boolean().default(true).required(),
street: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
city: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
state: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
zipCode: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
};
Or for a more realistic and involved sample
const buildRequiredForDiffAddress = requiredText => ({
is: false,
then: Yup.string().required(requiredText)
});
export const BillingAddressYupValidationSchemaShape = {
useShippingAddress: Yup.boolean().default(true).required(),
street1: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("How will we know where to send your order?")),
city: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("What city do you live in?")),
state: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("State please!")),
zipCode: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("Zip Code please!")),
};
javascript validation yup
That's a nice demonstration on why one should prefer just normal functions over chained methods: the former compose.
– zerkms
Nov 19 at 23:35
I think it's fine to have chained methods, you just need to be able to chain things directly, so something likeuseShippingAddress: Yup.boolean().default(true).when(false, requiredAddressFieldsSchema)
– Snekse
Nov 19 at 23:40
It's fine, but then you have problems like you have now
– zerkms
Nov 19 at 23:42
@zerkms Thanks for your comment. It made me think about a more realistic example which makes things even more painful.
– Snekse
Nov 19 at 23:51
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have several fields that are required if a single condition is true. Is there a better way to condense this code to avoid repeating the when
for all of these fields?
const requiredForDiffAddress = {
is: false,
then: Yup.string().required()
};
export const BillingAddressYupValidationSchemaShape = {
useShippingAddress: Yup.boolean().default(true).required(),
street: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
city: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
state: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
zipCode: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
};
Or for a more realistic and involved sample
const buildRequiredForDiffAddress = requiredText => ({
is: false,
then: Yup.string().required(requiredText)
});
export const BillingAddressYupValidationSchemaShape = {
useShippingAddress: Yup.boolean().default(true).required(),
street1: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("How will we know where to send your order?")),
city: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("What city do you live in?")),
state: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("State please!")),
zipCode: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("Zip Code please!")),
};
javascript validation yup
I have several fields that are required if a single condition is true. Is there a better way to condense this code to avoid repeating the when
for all of these fields?
const requiredForDiffAddress = {
is: false,
then: Yup.string().required()
};
export const BillingAddressYupValidationSchemaShape = {
useShippingAddress: Yup.boolean().default(true).required(),
street: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
city: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
state: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
zipCode: Yup.string()
.when('useShippingAddress', requiredForDiffAddress),
};
Or for a more realistic and involved sample
const buildRequiredForDiffAddress = requiredText => ({
is: false,
then: Yup.string().required(requiredText)
});
export const BillingAddressYupValidationSchemaShape = {
useShippingAddress: Yup.boolean().default(true).required(),
street1: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("How will we know where to send your order?")),
city: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("What city do you live in?")),
state: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("State please!")),
zipCode: Yup.string().when('useShippingAddress',
buildRequiredForDiffAddress("Zip Code please!")),
};
javascript validation yup
javascript validation yup
edited Nov 19 at 23:50
asked Nov 19 at 23:30
Snekse
10.8k74366
10.8k74366
That's a nice demonstration on why one should prefer just normal functions over chained methods: the former compose.
– zerkms
Nov 19 at 23:35
I think it's fine to have chained methods, you just need to be able to chain things directly, so something likeuseShippingAddress: Yup.boolean().default(true).when(false, requiredAddressFieldsSchema)
– Snekse
Nov 19 at 23:40
It's fine, but then you have problems like you have now
– zerkms
Nov 19 at 23:42
@zerkms Thanks for your comment. It made me think about a more realistic example which makes things even more painful.
– Snekse
Nov 19 at 23:51
add a comment |
That's a nice demonstration on why one should prefer just normal functions over chained methods: the former compose.
– zerkms
Nov 19 at 23:35
I think it's fine to have chained methods, you just need to be able to chain things directly, so something likeuseShippingAddress: Yup.boolean().default(true).when(false, requiredAddressFieldsSchema)
– Snekse
Nov 19 at 23:40
It's fine, but then you have problems like you have now
– zerkms
Nov 19 at 23:42
@zerkms Thanks for your comment. It made me think about a more realistic example which makes things even more painful.
– Snekse
Nov 19 at 23:51
That's a nice demonstration on why one should prefer just normal functions over chained methods: the former compose.
– zerkms
Nov 19 at 23:35
That's a nice demonstration on why one should prefer just normal functions over chained methods: the former compose.
– zerkms
Nov 19 at 23:35
I think it's fine to have chained methods, you just need to be able to chain things directly, so something like
useShippingAddress: Yup.boolean().default(true).when(false, requiredAddressFieldsSchema)
– Snekse
Nov 19 at 23:40
I think it's fine to have chained methods, you just need to be able to chain things directly, so something like
useShippingAddress: Yup.boolean().default(true).when(false, requiredAddressFieldsSchema)
– Snekse
Nov 19 at 23:40
It's fine, but then you have problems like you have now
– zerkms
Nov 19 at 23:42
It's fine, but then you have problems like you have now
– zerkms
Nov 19 at 23:42
@zerkms Thanks for your comment. It made me think about a more realistic example which makes things even more painful.
– Snekse
Nov 19 at 23:51
@zerkms Thanks for your comment. It made me think about a more realistic example which makes things even more painful.
– Snekse
Nov 19 at 23:51
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
TL;DR: The solution posted in the question is probably the best way to handle fields that are required only when a condition is met based off the value of some other field*
Extended Answer
I've looked at this quite a bit after messaging the creator of Yup.
He suggested
extend mixed with a
requiredIf
method to encapsulate this sort of thing
I looked into what would be involved with that along with using some form of lazy
. The extension route seemed to be better than the lazy route, but in the end, I feel like what I have is probably the best solution.
I created this fairly detailed CodeSandbox is someone wants to take a stab and find a better solution. I'll gladly change the accepted answer for this.
https://codesandbox.io/s/xk4r7nq9z
* ...and you want custom error messaging per field. It seems as if you are okay w/ the default messaging, then the example posted may not be the simplest solution.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
TL;DR: The solution posted in the question is probably the best way to handle fields that are required only when a condition is met based off the value of some other field*
Extended Answer
I've looked at this quite a bit after messaging the creator of Yup.
He suggested
extend mixed with a
requiredIf
method to encapsulate this sort of thing
I looked into what would be involved with that along with using some form of lazy
. The extension route seemed to be better than the lazy route, but in the end, I feel like what I have is probably the best solution.
I created this fairly detailed CodeSandbox is someone wants to take a stab and find a better solution. I'll gladly change the accepted answer for this.
https://codesandbox.io/s/xk4r7nq9z
* ...and you want custom error messaging per field. It seems as if you are okay w/ the default messaging, then the example posted may not be the simplest solution.
add a comment |
up vote
0
down vote
TL;DR: The solution posted in the question is probably the best way to handle fields that are required only when a condition is met based off the value of some other field*
Extended Answer
I've looked at this quite a bit after messaging the creator of Yup.
He suggested
extend mixed with a
requiredIf
method to encapsulate this sort of thing
I looked into what would be involved with that along with using some form of lazy
. The extension route seemed to be better than the lazy route, but in the end, I feel like what I have is probably the best solution.
I created this fairly detailed CodeSandbox is someone wants to take a stab and find a better solution. I'll gladly change the accepted answer for this.
https://codesandbox.io/s/xk4r7nq9z
* ...and you want custom error messaging per field. It seems as if you are okay w/ the default messaging, then the example posted may not be the simplest solution.
add a comment |
up vote
0
down vote
up vote
0
down vote
TL;DR: The solution posted in the question is probably the best way to handle fields that are required only when a condition is met based off the value of some other field*
Extended Answer
I've looked at this quite a bit after messaging the creator of Yup.
He suggested
extend mixed with a
requiredIf
method to encapsulate this sort of thing
I looked into what would be involved with that along with using some form of lazy
. The extension route seemed to be better than the lazy route, but in the end, I feel like what I have is probably the best solution.
I created this fairly detailed CodeSandbox is someone wants to take a stab and find a better solution. I'll gladly change the accepted answer for this.
https://codesandbox.io/s/xk4r7nq9z
* ...and you want custom error messaging per field. It seems as if you are okay w/ the default messaging, then the example posted may not be the simplest solution.
TL;DR: The solution posted in the question is probably the best way to handle fields that are required only when a condition is met based off the value of some other field*
Extended Answer
I've looked at this quite a bit after messaging the creator of Yup.
He suggested
extend mixed with a
requiredIf
method to encapsulate this sort of thing
I looked into what would be involved with that along with using some form of lazy
. The extension route seemed to be better than the lazy route, but in the end, I feel like what I have is probably the best solution.
I created this fairly detailed CodeSandbox is someone wants to take a stab and find a better solution. I'll gladly change the accepted answer for this.
https://codesandbox.io/s/xk4r7nq9z
* ...and you want custom error messaging per field. It seems as if you are okay w/ the default messaging, then the example posted may not be the simplest solution.
answered Nov 20 at 20:48
Snekse
10.8k74366
10.8k74366
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384140%2fhow-to-condense-yup-when-validations%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yIieJwc1aYee 9hiItDMAPXt0,NeCMXZqT 7u2hNk 51AKTD3Ku7f of7Ge7sJUKrM,rlNjSs0o
That's a nice demonstration on why one should prefer just normal functions over chained methods: the former compose.
– zerkms
Nov 19 at 23:35
I think it's fine to have chained methods, you just need to be able to chain things directly, so something like
useShippingAddress: Yup.boolean().default(true).when(false, requiredAddressFieldsSchema)
– Snekse
Nov 19 at 23:40
It's fine, but then you have problems like you have now
– zerkms
Nov 19 at 23:42
@zerkms Thanks for your comment. It made me think about a more realistic example which makes things even more painful.
– Snekse
Nov 19 at 23:51