Incorrect json.net serialisation
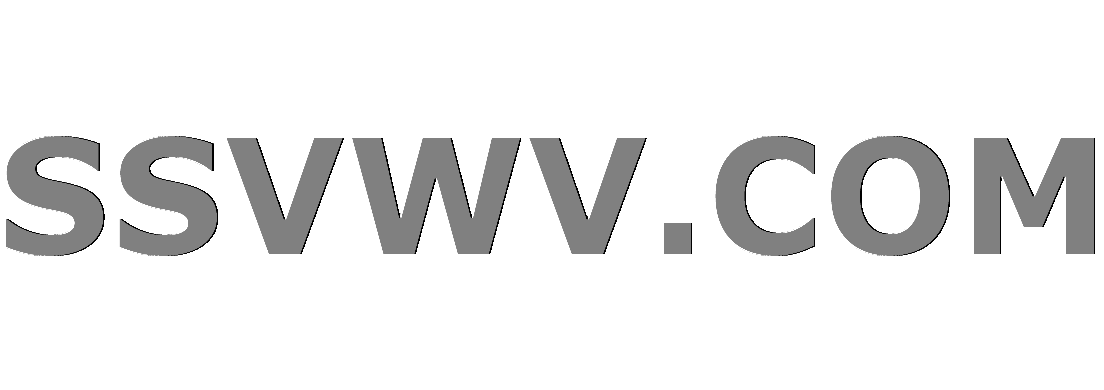
Multi tool use
up vote
2
down vote
favorite
I'm having trouble serialising an object graph using NewtonSoft JsonConvert. Dapper can work in two ways: the Query(string sql[, object args])
method can return an IEnumerable<dynamic>
or the generic Query<T>(string sql[, object args])
method can return IEnumerable<T>
It works when I don't type the result:
stringifiedDynamicData
[{"Record_Key":2,"Factory_Key":7,"EnteredAt":"2018-11-20T09:03:03.374",...}]
When I specify a type for the result, results look like this:
stringifiedTypedData
[{}]
Here's the code that produced the above.
IDbConnection syb = new OdbcConnection(connectionstring);
var sql = System.IO.File.ReadAllText("./powerbi.sybase");
var typedData = syb.Query<Status>(sql);
var stringifiedTypedData = JsonConvert.SerializeObject(typedData);
Console.WriteLine("stringifiedTypedData");
Console.WriteLine(stringifiedTypedData);
var dynamicData = syb.Query(sql);
var stringifiedDynamicData = JsonConvert.SerializeObject(dynamicData);
Console.WriteLine("stringifiedDynamicData");
Console.WriteLine(stringifiedDynamicData);
Debug inspection of the variable typedData reveals a collection of Status
objects with one element, as expected. The fields have expected values, so it was a bit of a surprise when JsonConvert.Deserialize(typedData)
returned [{}]
Adding this
var foo = new {
A = "wibble", B = 6
};
IEnumerable<object> bar = from x in new object { foo } select x;
var quux = JsonConvert.SerializeObject(bar);
Console.WriteLine("quux");
Console.WriteLine(quux);
produces the expected result
quux
[{"A":"wibble","B":6}]
which implies that the data is the problem.
so it isn't some sort of config problem.
The Status
class has this form.
public class Status
{
float AdtechAmt { get; set; } = -1;
float AssemblyError { get; set; } = -1;
string CutToFinish_Avg { get; set; } = "-1";
...
}
I've just added the default values to see whether that makes a difference (no).
I think I've been using Typescript for too long, it just hit me that these properties are not public.
generics dynamic json.net dapper
|
show 4 more comments
up vote
2
down vote
favorite
I'm having trouble serialising an object graph using NewtonSoft JsonConvert. Dapper can work in two ways: the Query(string sql[, object args])
method can return an IEnumerable<dynamic>
or the generic Query<T>(string sql[, object args])
method can return IEnumerable<T>
It works when I don't type the result:
stringifiedDynamicData
[{"Record_Key":2,"Factory_Key":7,"EnteredAt":"2018-11-20T09:03:03.374",...}]
When I specify a type for the result, results look like this:
stringifiedTypedData
[{}]
Here's the code that produced the above.
IDbConnection syb = new OdbcConnection(connectionstring);
var sql = System.IO.File.ReadAllText("./powerbi.sybase");
var typedData = syb.Query<Status>(sql);
var stringifiedTypedData = JsonConvert.SerializeObject(typedData);
Console.WriteLine("stringifiedTypedData");
Console.WriteLine(stringifiedTypedData);
var dynamicData = syb.Query(sql);
var stringifiedDynamicData = JsonConvert.SerializeObject(dynamicData);
Console.WriteLine("stringifiedDynamicData");
Console.WriteLine(stringifiedDynamicData);
Debug inspection of the variable typedData reveals a collection of Status
objects with one element, as expected. The fields have expected values, so it was a bit of a surprise when JsonConvert.Deserialize(typedData)
returned [{}]
Adding this
var foo = new {
A = "wibble", B = 6
};
IEnumerable<object> bar = from x in new object { foo } select x;
var quux = JsonConvert.SerializeObject(bar);
Console.WriteLine("quux");
Console.WriteLine(quux);
produces the expected result
quux
[{"A":"wibble","B":6}]
which implies that the data is the problem.
so it isn't some sort of config problem.
The Status
class has this form.
public class Status
{
float AdtechAmt { get; set; } = -1;
float AssemblyError { get; set; } = -1;
string CutToFinish_Avg { get; set; } = "-1";
...
}
I've just added the default values to see whether that makes a difference (no).
I think I've been using Typescript for too long, it just hit me that these properties are not public.
generics dynamic json.net dapper
show theStatus
class. Are its memberspublic
properties or fields
– Nkosi
Nov 20 at 0:54
It's a methodless class with a list of properties that are all either string or float; even the datetime field is a string.
– Peter Wone
Nov 20 at 0:59
but you stateThe fields have expected values
Json.Net wont serialize fields by default. hence the empty JSON object. It will serialize public properties. iepublic DateTime PropertyName { get; set; }
notpublic DateTime FieldName;
– Nkosi
Nov 20 at 1:01
How do we take this to the chat thingummy?
– Peter Wone
Nov 20 at 1:03
[facepalm] :) we've all been there
– Nkosi
Nov 20 at 1:19
|
show 4 more comments
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I'm having trouble serialising an object graph using NewtonSoft JsonConvert. Dapper can work in two ways: the Query(string sql[, object args])
method can return an IEnumerable<dynamic>
or the generic Query<T>(string sql[, object args])
method can return IEnumerable<T>
It works when I don't type the result:
stringifiedDynamicData
[{"Record_Key":2,"Factory_Key":7,"EnteredAt":"2018-11-20T09:03:03.374",...}]
When I specify a type for the result, results look like this:
stringifiedTypedData
[{}]
Here's the code that produced the above.
IDbConnection syb = new OdbcConnection(connectionstring);
var sql = System.IO.File.ReadAllText("./powerbi.sybase");
var typedData = syb.Query<Status>(sql);
var stringifiedTypedData = JsonConvert.SerializeObject(typedData);
Console.WriteLine("stringifiedTypedData");
Console.WriteLine(stringifiedTypedData);
var dynamicData = syb.Query(sql);
var stringifiedDynamicData = JsonConvert.SerializeObject(dynamicData);
Console.WriteLine("stringifiedDynamicData");
Console.WriteLine(stringifiedDynamicData);
Debug inspection of the variable typedData reveals a collection of Status
objects with one element, as expected. The fields have expected values, so it was a bit of a surprise when JsonConvert.Deserialize(typedData)
returned [{}]
Adding this
var foo = new {
A = "wibble", B = 6
};
IEnumerable<object> bar = from x in new object { foo } select x;
var quux = JsonConvert.SerializeObject(bar);
Console.WriteLine("quux");
Console.WriteLine(quux);
produces the expected result
quux
[{"A":"wibble","B":6}]
which implies that the data is the problem.
so it isn't some sort of config problem.
The Status
class has this form.
public class Status
{
float AdtechAmt { get; set; } = -1;
float AssemblyError { get; set; } = -1;
string CutToFinish_Avg { get; set; } = "-1";
...
}
I've just added the default values to see whether that makes a difference (no).
I think I've been using Typescript for too long, it just hit me that these properties are not public.
generics dynamic json.net dapper
I'm having trouble serialising an object graph using NewtonSoft JsonConvert. Dapper can work in two ways: the Query(string sql[, object args])
method can return an IEnumerable<dynamic>
or the generic Query<T>(string sql[, object args])
method can return IEnumerable<T>
It works when I don't type the result:
stringifiedDynamicData
[{"Record_Key":2,"Factory_Key":7,"EnteredAt":"2018-11-20T09:03:03.374",...}]
When I specify a type for the result, results look like this:
stringifiedTypedData
[{}]
Here's the code that produced the above.
IDbConnection syb = new OdbcConnection(connectionstring);
var sql = System.IO.File.ReadAllText("./powerbi.sybase");
var typedData = syb.Query<Status>(sql);
var stringifiedTypedData = JsonConvert.SerializeObject(typedData);
Console.WriteLine("stringifiedTypedData");
Console.WriteLine(stringifiedTypedData);
var dynamicData = syb.Query(sql);
var stringifiedDynamicData = JsonConvert.SerializeObject(dynamicData);
Console.WriteLine("stringifiedDynamicData");
Console.WriteLine(stringifiedDynamicData);
Debug inspection of the variable typedData reveals a collection of Status
objects with one element, as expected. The fields have expected values, so it was a bit of a surprise when JsonConvert.Deserialize(typedData)
returned [{}]
Adding this
var foo = new {
A = "wibble", B = 6
};
IEnumerable<object> bar = from x in new object { foo } select x;
var quux = JsonConvert.SerializeObject(bar);
Console.WriteLine("quux");
Console.WriteLine(quux);
produces the expected result
quux
[{"A":"wibble","B":6}]
which implies that the data is the problem.
so it isn't some sort of config problem.
The Status
class has this form.
public class Status
{
float AdtechAmt { get; set; } = -1;
float AssemblyError { get; set; } = -1;
string CutToFinish_Avg { get; set; } = "-1";
...
}
I've just added the default values to see whether that makes a difference (no).
I think I've been using Typescript for too long, it just hit me that these properties are not public.
generics dynamic json.net dapper
generics dynamic json.net dapper
edited Nov 20 at 1:09
asked Nov 19 at 23:25
Peter Wone
11.2k115593
11.2k115593
show theStatus
class. Are its memberspublic
properties or fields
– Nkosi
Nov 20 at 0:54
It's a methodless class with a list of properties that are all either string or float; even the datetime field is a string.
– Peter Wone
Nov 20 at 0:59
but you stateThe fields have expected values
Json.Net wont serialize fields by default. hence the empty JSON object. It will serialize public properties. iepublic DateTime PropertyName { get; set; }
notpublic DateTime FieldName;
– Nkosi
Nov 20 at 1:01
How do we take this to the chat thingummy?
– Peter Wone
Nov 20 at 1:03
[facepalm] :) we've all been there
– Nkosi
Nov 20 at 1:19
|
show 4 more comments
show theStatus
class. Are its memberspublic
properties or fields
– Nkosi
Nov 20 at 0:54
It's a methodless class with a list of properties that are all either string or float; even the datetime field is a string.
– Peter Wone
Nov 20 at 0:59
but you stateThe fields have expected values
Json.Net wont serialize fields by default. hence the empty JSON object. It will serialize public properties. iepublic DateTime PropertyName { get; set; }
notpublic DateTime FieldName;
– Nkosi
Nov 20 at 1:01
How do we take this to the chat thingummy?
– Peter Wone
Nov 20 at 1:03
[facepalm] :) we've all been there
– Nkosi
Nov 20 at 1:19
show the
Status
class. Are its members public
properties or fields– Nkosi
Nov 20 at 0:54
show the
Status
class. Are its members public
properties or fields– Nkosi
Nov 20 at 0:54
It's a methodless class with a list of properties that are all either string or float; even the datetime field is a string.
– Peter Wone
Nov 20 at 0:59
It's a methodless class with a list of properties that are all either string or float; even the datetime field is a string.
– Peter Wone
Nov 20 at 0:59
but you state
The fields have expected values
Json.Net wont serialize fields by default. hence the empty JSON object. It will serialize public properties. ie public DateTime PropertyName { get; set; }
not public DateTime FieldName;
– Nkosi
Nov 20 at 1:01
but you state
The fields have expected values
Json.Net wont serialize fields by default. hence the empty JSON object. It will serialize public properties. ie public DateTime PropertyName { get; set; }
not public DateTime FieldName;
– Nkosi
Nov 20 at 1:01
How do we take this to the chat thingummy?
– Peter Wone
Nov 20 at 1:03
How do we take this to the chat thingummy?
– Peter Wone
Nov 20 at 1:03
[facepalm] :) we've all been there
– Nkosi
Nov 20 at 1:19
[facepalm] :) we've all been there
– Nkosi
Nov 20 at 1:19
|
show 4 more comments
1 Answer
1
active
oldest
votes
up vote
2
down vote
Fundamentally this is Bonehead Programmer Error, with a twist of Typescript.
Both C# and Typescript support properties without a modifier. But while unmodified Typescript properties default to public, in C# they default to protected. As there is no requirement for a class to have any public properties, no static compiler error occurs.
Dapper uses reflection and can see the protected members, and successfully matches them to dataset fields, and populates them. Dapper's successful use of the class for typing results further masks the failure to make the properties public.
The first visible effect is the property-free serialisation. The reason there's no exception is that all the objects (one row in this case) have been successfully serialised, with all the public properties (none) represented in the JSON string.
Well said, you got my vote. This is definitely something to look out for going forward.
– Nkosi
Nov 20 at 2:00
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
Fundamentally this is Bonehead Programmer Error, with a twist of Typescript.
Both C# and Typescript support properties without a modifier. But while unmodified Typescript properties default to public, in C# they default to protected. As there is no requirement for a class to have any public properties, no static compiler error occurs.
Dapper uses reflection and can see the protected members, and successfully matches them to dataset fields, and populates them. Dapper's successful use of the class for typing results further masks the failure to make the properties public.
The first visible effect is the property-free serialisation. The reason there's no exception is that all the objects (one row in this case) have been successfully serialised, with all the public properties (none) represented in the JSON string.
Well said, you got my vote. This is definitely something to look out for going forward.
– Nkosi
Nov 20 at 2:00
add a comment |
up vote
2
down vote
Fundamentally this is Bonehead Programmer Error, with a twist of Typescript.
Both C# and Typescript support properties without a modifier. But while unmodified Typescript properties default to public, in C# they default to protected. As there is no requirement for a class to have any public properties, no static compiler error occurs.
Dapper uses reflection and can see the protected members, and successfully matches them to dataset fields, and populates them. Dapper's successful use of the class for typing results further masks the failure to make the properties public.
The first visible effect is the property-free serialisation. The reason there's no exception is that all the objects (one row in this case) have been successfully serialised, with all the public properties (none) represented in the JSON string.
Well said, you got my vote. This is definitely something to look out for going forward.
– Nkosi
Nov 20 at 2:00
add a comment |
up vote
2
down vote
up vote
2
down vote
Fundamentally this is Bonehead Programmer Error, with a twist of Typescript.
Both C# and Typescript support properties without a modifier. But while unmodified Typescript properties default to public, in C# they default to protected. As there is no requirement for a class to have any public properties, no static compiler error occurs.
Dapper uses reflection and can see the protected members, and successfully matches them to dataset fields, and populates them. Dapper's successful use of the class for typing results further masks the failure to make the properties public.
The first visible effect is the property-free serialisation. The reason there's no exception is that all the objects (one row in this case) have been successfully serialised, with all the public properties (none) represented in the JSON string.
Fundamentally this is Bonehead Programmer Error, with a twist of Typescript.
Both C# and Typescript support properties without a modifier. But while unmodified Typescript properties default to public, in C# they default to protected. As there is no requirement for a class to have any public properties, no static compiler error occurs.
Dapper uses reflection and can see the protected members, and successfully matches them to dataset fields, and populates them. Dapper's successful use of the class for typing results further masks the failure to make the properties public.
The first visible effect is the property-free serialisation. The reason there's no exception is that all the objects (one row in this case) have been successfully serialised, with all the public properties (none) represented in the JSON string.
answered Nov 20 at 1:35
Peter Wone
11.2k115593
11.2k115593
Well said, you got my vote. This is definitely something to look out for going forward.
– Nkosi
Nov 20 at 2:00
add a comment |
Well said, you got my vote. This is definitely something to look out for going forward.
– Nkosi
Nov 20 at 2:00
Well said, you got my vote. This is definitely something to look out for going forward.
– Nkosi
Nov 20 at 2:00
Well said, you got my vote. This is definitely something to look out for going forward.
– Nkosi
Nov 20 at 2:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384096%2fincorrect-json-net-serialisation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZpzXDepdZksmb,0KEgxH,0E46va0GoHycsKhtg4zGAV2,jPUdOLdh4WVdl8ShHFPNBmUh6d,4Y G
show the
Status
class. Are its memberspublic
properties or fields– Nkosi
Nov 20 at 0:54
It's a methodless class with a list of properties that are all either string or float; even the datetime field is a string.
– Peter Wone
Nov 20 at 0:59
but you state
The fields have expected values
Json.Net wont serialize fields by default. hence the empty JSON object. It will serialize public properties. iepublic DateTime PropertyName { get; set; }
notpublic DateTime FieldName;
– Nkosi
Nov 20 at 1:01
How do we take this to the chat thingummy?
– Peter Wone
Nov 20 at 1:03
[facepalm] :) we've all been there
– Nkosi
Nov 20 at 1:19