Linked List Stack Question in Regards to the Stack Being Empty
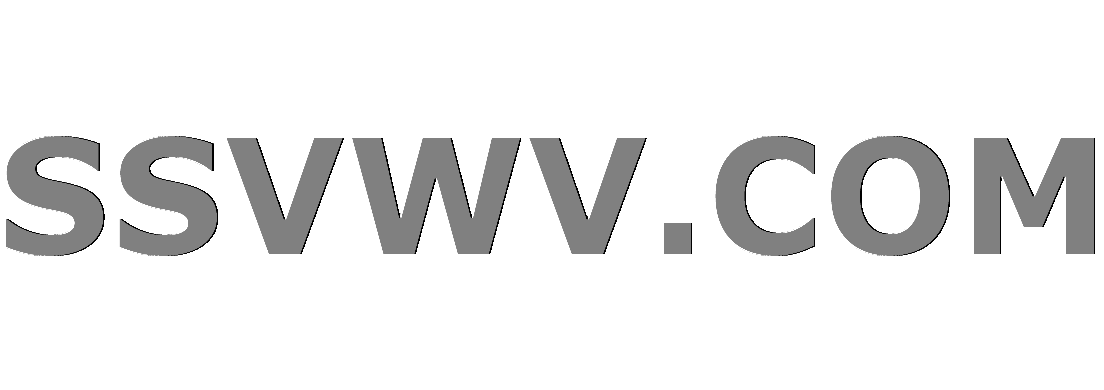
Multi tool use
up vote
0
down vote
favorite
I am worked on a Linked list stack and a bunch of functions for it. What I do not understand currently is how come my "isEmpty" function is not working correctly. I believe that the way I have it written makes sense. By nature if Front is Null then the list should have to be empty which would mean that "isEmpty" would return false. The problem that I am having is that my program says that the list is always empty whether or not it actually is or not. I am not sure what the issue is. Any help would be appreciated.
struct node
{
int data;
node *next;
}*front = NULL, *rear = NULL, *p = NULL, *np = NULL;
void push(int x)
{
np = new node;
np->data = x;
np->next = NULL;
if(front == NULL)
{
front = rear = np;
rear->next = NULL;
}
else
{
rear->next = np;
rear = np;
rear->next = NULL;
}
}
int pop()
{
int x;
if (front == NULL)
{
cout<<"empty queuen";
}
else
{
p = front;
x = p->data;
front = front->next;
delete(p);
return(x);
}
}
int peek()
{
int x;
if (front == NULL)
{
cout<<"empty queuen";
}
else
{
p = front;
x = p->data;
front = front->next;
return(x);
}
}
bool isEmpty()
{
if (front == NULL)
{
return true;
}
else if (front != NULL)
{
return false;
}
}
void Display()
{
cout << front;
}
int main()
{
int n, c = 0, x;
bool is_empty = isEmpty();
cout<<"Enter the number of values to be pushed into queuen";
cin>>n;
while (c < n)
{
cout<<"Enter the value to be entered into queuen";
cin>>x;
push(x);
c++;
}
cout<<endl<<"Pop value: ";
if (front != NULL)
cout<<pop()<<endl;
cout<<endl<<"Peak value: ";
if (front != NULL)
cout<<peek()<<endl;
if (is_empty == true)
{
cout<<endl<<"The list is empty";
}
else if (is_empty == false)
{
cout<<endl<<"The list is not empty";
}
cout << endl << "The current contents of the stack are: ";
while(front != NULL)
{
Display();
if(front == NULL)
cout << "The stack is empty";
break;
}
getch();
}
c++ linked-list stack
|
show 14 more comments
up vote
0
down vote
favorite
I am worked on a Linked list stack and a bunch of functions for it. What I do not understand currently is how come my "isEmpty" function is not working correctly. I believe that the way I have it written makes sense. By nature if Front is Null then the list should have to be empty which would mean that "isEmpty" would return false. The problem that I am having is that my program says that the list is always empty whether or not it actually is or not. I am not sure what the issue is. Any help would be appreciated.
struct node
{
int data;
node *next;
}*front = NULL, *rear = NULL, *p = NULL, *np = NULL;
void push(int x)
{
np = new node;
np->data = x;
np->next = NULL;
if(front == NULL)
{
front = rear = np;
rear->next = NULL;
}
else
{
rear->next = np;
rear = np;
rear->next = NULL;
}
}
int pop()
{
int x;
if (front == NULL)
{
cout<<"empty queuen";
}
else
{
p = front;
x = p->data;
front = front->next;
delete(p);
return(x);
}
}
int peek()
{
int x;
if (front == NULL)
{
cout<<"empty queuen";
}
else
{
p = front;
x = p->data;
front = front->next;
return(x);
}
}
bool isEmpty()
{
if (front == NULL)
{
return true;
}
else if (front != NULL)
{
return false;
}
}
void Display()
{
cout << front;
}
int main()
{
int n, c = 0, x;
bool is_empty = isEmpty();
cout<<"Enter the number of values to be pushed into queuen";
cin>>n;
while (c < n)
{
cout<<"Enter the value to be entered into queuen";
cin>>x;
push(x);
c++;
}
cout<<endl<<"Pop value: ";
if (front != NULL)
cout<<pop()<<endl;
cout<<endl<<"Peak value: ";
if (front != NULL)
cout<<peek()<<endl;
if (is_empty == true)
{
cout<<endl<<"The list is empty";
}
else if (is_empty == false)
{
cout<<endl<<"The list is not empty";
}
cout << endl << "The current contents of the stack are: ";
while(front != NULL)
{
Display();
if(front == NULL)
cout << "The stack is empty";
break;
}
getch();
}
c++ linked-list stack
What do you thinkis_empty
does?
– Fei Xiang
Nov 19 at 23:39
You setis_empty
1 time at the beginning of yourmain()
it does not change its value after regardless of the condition of the stack. Instead callisEmpty()
each time you want to check.
– drescherjm
Nov 19 at 23:42
@drescherjm thank you that was very helpful.
– Jenny Nicky
Nov 19 at 23:49
@drescherjm do you also know why this code does not properly display the contents of the list and only displays the list if the list is empty.
– Jenny Nicky
Nov 19 at 23:50
Did you put at least 2 items in the stack?if (front != NULL) cout<<pop()<<endl;
removes the first item if there is one.
– drescherjm
Nov 19 at 23:54
|
show 14 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am worked on a Linked list stack and a bunch of functions for it. What I do not understand currently is how come my "isEmpty" function is not working correctly. I believe that the way I have it written makes sense. By nature if Front is Null then the list should have to be empty which would mean that "isEmpty" would return false. The problem that I am having is that my program says that the list is always empty whether or not it actually is or not. I am not sure what the issue is. Any help would be appreciated.
struct node
{
int data;
node *next;
}*front = NULL, *rear = NULL, *p = NULL, *np = NULL;
void push(int x)
{
np = new node;
np->data = x;
np->next = NULL;
if(front == NULL)
{
front = rear = np;
rear->next = NULL;
}
else
{
rear->next = np;
rear = np;
rear->next = NULL;
}
}
int pop()
{
int x;
if (front == NULL)
{
cout<<"empty queuen";
}
else
{
p = front;
x = p->data;
front = front->next;
delete(p);
return(x);
}
}
int peek()
{
int x;
if (front == NULL)
{
cout<<"empty queuen";
}
else
{
p = front;
x = p->data;
front = front->next;
return(x);
}
}
bool isEmpty()
{
if (front == NULL)
{
return true;
}
else if (front != NULL)
{
return false;
}
}
void Display()
{
cout << front;
}
int main()
{
int n, c = 0, x;
bool is_empty = isEmpty();
cout<<"Enter the number of values to be pushed into queuen";
cin>>n;
while (c < n)
{
cout<<"Enter the value to be entered into queuen";
cin>>x;
push(x);
c++;
}
cout<<endl<<"Pop value: ";
if (front != NULL)
cout<<pop()<<endl;
cout<<endl<<"Peak value: ";
if (front != NULL)
cout<<peek()<<endl;
if (is_empty == true)
{
cout<<endl<<"The list is empty";
}
else if (is_empty == false)
{
cout<<endl<<"The list is not empty";
}
cout << endl << "The current contents of the stack are: ";
while(front != NULL)
{
Display();
if(front == NULL)
cout << "The stack is empty";
break;
}
getch();
}
c++ linked-list stack
I am worked on a Linked list stack and a bunch of functions for it. What I do not understand currently is how come my "isEmpty" function is not working correctly. I believe that the way I have it written makes sense. By nature if Front is Null then the list should have to be empty which would mean that "isEmpty" would return false. The problem that I am having is that my program says that the list is always empty whether or not it actually is or not. I am not sure what the issue is. Any help would be appreciated.
struct node
{
int data;
node *next;
}*front = NULL, *rear = NULL, *p = NULL, *np = NULL;
void push(int x)
{
np = new node;
np->data = x;
np->next = NULL;
if(front == NULL)
{
front = rear = np;
rear->next = NULL;
}
else
{
rear->next = np;
rear = np;
rear->next = NULL;
}
}
int pop()
{
int x;
if (front == NULL)
{
cout<<"empty queuen";
}
else
{
p = front;
x = p->data;
front = front->next;
delete(p);
return(x);
}
}
int peek()
{
int x;
if (front == NULL)
{
cout<<"empty queuen";
}
else
{
p = front;
x = p->data;
front = front->next;
return(x);
}
}
bool isEmpty()
{
if (front == NULL)
{
return true;
}
else if (front != NULL)
{
return false;
}
}
void Display()
{
cout << front;
}
int main()
{
int n, c = 0, x;
bool is_empty = isEmpty();
cout<<"Enter the number of values to be pushed into queuen";
cin>>n;
while (c < n)
{
cout<<"Enter the value to be entered into queuen";
cin>>x;
push(x);
c++;
}
cout<<endl<<"Pop value: ";
if (front != NULL)
cout<<pop()<<endl;
cout<<endl<<"Peak value: ";
if (front != NULL)
cout<<peek()<<endl;
if (is_empty == true)
{
cout<<endl<<"The list is empty";
}
else if (is_empty == false)
{
cout<<endl<<"The list is not empty";
}
cout << endl << "The current contents of the stack are: ";
while(front != NULL)
{
Display();
if(front == NULL)
cout << "The stack is empty";
break;
}
getch();
}
c++ linked-list stack
c++ linked-list stack
edited Nov 20 at 0:08
asked Nov 19 at 23:26
Jenny Nicky
113
113
What do you thinkis_empty
does?
– Fei Xiang
Nov 19 at 23:39
You setis_empty
1 time at the beginning of yourmain()
it does not change its value after regardless of the condition of the stack. Instead callisEmpty()
each time you want to check.
– drescherjm
Nov 19 at 23:42
@drescherjm thank you that was very helpful.
– Jenny Nicky
Nov 19 at 23:49
@drescherjm do you also know why this code does not properly display the contents of the list and only displays the list if the list is empty.
– Jenny Nicky
Nov 19 at 23:50
Did you put at least 2 items in the stack?if (front != NULL) cout<<pop()<<endl;
removes the first item if there is one.
– drescherjm
Nov 19 at 23:54
|
show 14 more comments
What do you thinkis_empty
does?
– Fei Xiang
Nov 19 at 23:39
You setis_empty
1 time at the beginning of yourmain()
it does not change its value after regardless of the condition of the stack. Instead callisEmpty()
each time you want to check.
– drescherjm
Nov 19 at 23:42
@drescherjm thank you that was very helpful.
– Jenny Nicky
Nov 19 at 23:49
@drescherjm do you also know why this code does not properly display the contents of the list and only displays the list if the list is empty.
– Jenny Nicky
Nov 19 at 23:50
Did you put at least 2 items in the stack?if (front != NULL) cout<<pop()<<endl;
removes the first item if there is one.
– drescherjm
Nov 19 at 23:54
What do you think
is_empty
does?– Fei Xiang
Nov 19 at 23:39
What do you think
is_empty
does?– Fei Xiang
Nov 19 at 23:39
You set
is_empty
1 time at the beginning of your main()
it does not change its value after regardless of the condition of the stack. Instead call isEmpty()
each time you want to check.– drescherjm
Nov 19 at 23:42
You set
is_empty
1 time at the beginning of your main()
it does not change its value after regardless of the condition of the stack. Instead call isEmpty()
each time you want to check.– drescherjm
Nov 19 at 23:42
@drescherjm thank you that was very helpful.
– Jenny Nicky
Nov 19 at 23:49
@drescherjm thank you that was very helpful.
– Jenny Nicky
Nov 19 at 23:49
@drescherjm do you also know why this code does not properly display the contents of the list and only displays the list if the list is empty.
– Jenny Nicky
Nov 19 at 23:50
@drescherjm do you also know why this code does not properly display the contents of the list and only displays the list if the list is empty.
– Jenny Nicky
Nov 19 at 23:50
Did you put at least 2 items in the stack?
if (front != NULL) cout<<pop()<<endl;
removes the first item if there is one.– drescherjm
Nov 19 at 23:54
Did you put at least 2 items in the stack?
if (front != NULL) cout<<pop()<<endl;
removes the first item if there is one.– drescherjm
Nov 19 at 23:54
|
show 14 more comments
1 Answer
1
active
oldest
votes
up vote
0
down vote
There are some issues with your code.
You have defined an isEmpty()
function, but you don't really it. You have declared a separate variable is_empty
that you set to the return value of isEmpty()
one time before you populate the list, and never update is_empty
after making any changes to the list. That is why your code is always reporting the list is "empty" even if it is really not. You need to get rid of the is_empty
variable and call isEmpty()
every time you need to check for empty.
Also, the return value of peek()
and pop()
are indeterminate if front
is NULL.
And peek()
pops the front
node from the list. Only pop()
should be doing that.
And pop()
does not check if rear
is pointing at the node being popped. You are not resetting rear
to NULL in that case.
And you don't free any nodes remaining in the list before exiting the program.
Try something more like this instead:
#include <iostream>
#include <limits>
struct node
{
int data;
node *next;
node(int value): data(value), next(NULL) {}
};
node *front = NULL;
node *rear = NULL;
void push(int x)
{
node **np = (rear) ? &(rear->next) : &front;
*np = new node(x);
rear = *np;
}
int peek()
{
if (front)
return front->data;
return -1;
}
int pop()
{
int x = peek();
if (front)
{
node *p = front;
front = front->next;
if (p == rear) rear = NULL;
delete p;
}
return x;
}
bool isEmpty()
{
return !front;
}
void clear()
{
while (front)
{
node *p = front;
front = front->next;
delete p;
}
rear = NULL;
}
void display()
{
node *n = front;
if (n)
{
cout << n->data;
while (n = n->next)
cout << ' ' << n->data;
}
}
int askForNumber(const char *prompt)
{
int n;
cout << prompt << ": ";
cin >> n;
cin.ignore(numeric_limits<streamsize>::max(), 'n');
return n;
}
int main()
{
int n, x;
n = askForNumber("Enter the number of values to be pushed into queue");
for(int c = 0; c < n; ++c)
{
x = askForNumber("Enter the value to be entered into queue");
push(x);
}
cout << endl;
cout << "Pop value: ";
if (!isEmpty())
cout << pop();
else
cout << "empty queue";
cout << endl;
cout << "Peak value: ";
if (!isEmpty())
cout << peek();
else
cout << "empty queue";
cout << endl;
if (isEmpty())
cout << "The list is empty";
else
cout << "The list is not empty";
cout << endl;
cout << "The current contents of the stack are:" << endl;
display();
cout << endl;
clear();
cin.get();
return 0;
}
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
There are some issues with your code.
You have defined an isEmpty()
function, but you don't really it. You have declared a separate variable is_empty
that you set to the return value of isEmpty()
one time before you populate the list, and never update is_empty
after making any changes to the list. That is why your code is always reporting the list is "empty" even if it is really not. You need to get rid of the is_empty
variable and call isEmpty()
every time you need to check for empty.
Also, the return value of peek()
and pop()
are indeterminate if front
is NULL.
And peek()
pops the front
node from the list. Only pop()
should be doing that.
And pop()
does not check if rear
is pointing at the node being popped. You are not resetting rear
to NULL in that case.
And you don't free any nodes remaining in the list before exiting the program.
Try something more like this instead:
#include <iostream>
#include <limits>
struct node
{
int data;
node *next;
node(int value): data(value), next(NULL) {}
};
node *front = NULL;
node *rear = NULL;
void push(int x)
{
node **np = (rear) ? &(rear->next) : &front;
*np = new node(x);
rear = *np;
}
int peek()
{
if (front)
return front->data;
return -1;
}
int pop()
{
int x = peek();
if (front)
{
node *p = front;
front = front->next;
if (p == rear) rear = NULL;
delete p;
}
return x;
}
bool isEmpty()
{
return !front;
}
void clear()
{
while (front)
{
node *p = front;
front = front->next;
delete p;
}
rear = NULL;
}
void display()
{
node *n = front;
if (n)
{
cout << n->data;
while (n = n->next)
cout << ' ' << n->data;
}
}
int askForNumber(const char *prompt)
{
int n;
cout << prompt << ": ";
cin >> n;
cin.ignore(numeric_limits<streamsize>::max(), 'n');
return n;
}
int main()
{
int n, x;
n = askForNumber("Enter the number of values to be pushed into queue");
for(int c = 0; c < n; ++c)
{
x = askForNumber("Enter the value to be entered into queue");
push(x);
}
cout << endl;
cout << "Pop value: ";
if (!isEmpty())
cout << pop();
else
cout << "empty queue";
cout << endl;
cout << "Peak value: ";
if (!isEmpty())
cout << peek();
else
cout << "empty queue";
cout << endl;
if (isEmpty())
cout << "The list is empty";
else
cout << "The list is not empty";
cout << endl;
cout << "The current contents of the stack are:" << endl;
display();
cout << endl;
clear();
cin.get();
return 0;
}
add a comment |
up vote
0
down vote
There are some issues with your code.
You have defined an isEmpty()
function, but you don't really it. You have declared a separate variable is_empty
that you set to the return value of isEmpty()
one time before you populate the list, and never update is_empty
after making any changes to the list. That is why your code is always reporting the list is "empty" even if it is really not. You need to get rid of the is_empty
variable and call isEmpty()
every time you need to check for empty.
Also, the return value of peek()
and pop()
are indeterminate if front
is NULL.
And peek()
pops the front
node from the list. Only pop()
should be doing that.
And pop()
does not check if rear
is pointing at the node being popped. You are not resetting rear
to NULL in that case.
And you don't free any nodes remaining in the list before exiting the program.
Try something more like this instead:
#include <iostream>
#include <limits>
struct node
{
int data;
node *next;
node(int value): data(value), next(NULL) {}
};
node *front = NULL;
node *rear = NULL;
void push(int x)
{
node **np = (rear) ? &(rear->next) : &front;
*np = new node(x);
rear = *np;
}
int peek()
{
if (front)
return front->data;
return -1;
}
int pop()
{
int x = peek();
if (front)
{
node *p = front;
front = front->next;
if (p == rear) rear = NULL;
delete p;
}
return x;
}
bool isEmpty()
{
return !front;
}
void clear()
{
while (front)
{
node *p = front;
front = front->next;
delete p;
}
rear = NULL;
}
void display()
{
node *n = front;
if (n)
{
cout << n->data;
while (n = n->next)
cout << ' ' << n->data;
}
}
int askForNumber(const char *prompt)
{
int n;
cout << prompt << ": ";
cin >> n;
cin.ignore(numeric_limits<streamsize>::max(), 'n');
return n;
}
int main()
{
int n, x;
n = askForNumber("Enter the number of values to be pushed into queue");
for(int c = 0; c < n; ++c)
{
x = askForNumber("Enter the value to be entered into queue");
push(x);
}
cout << endl;
cout << "Pop value: ";
if (!isEmpty())
cout << pop();
else
cout << "empty queue";
cout << endl;
cout << "Peak value: ";
if (!isEmpty())
cout << peek();
else
cout << "empty queue";
cout << endl;
if (isEmpty())
cout << "The list is empty";
else
cout << "The list is not empty";
cout << endl;
cout << "The current contents of the stack are:" << endl;
display();
cout << endl;
clear();
cin.get();
return 0;
}
add a comment |
up vote
0
down vote
up vote
0
down vote
There are some issues with your code.
You have defined an isEmpty()
function, but you don't really it. You have declared a separate variable is_empty
that you set to the return value of isEmpty()
one time before you populate the list, and never update is_empty
after making any changes to the list. That is why your code is always reporting the list is "empty" even if it is really not. You need to get rid of the is_empty
variable and call isEmpty()
every time you need to check for empty.
Also, the return value of peek()
and pop()
are indeterminate if front
is NULL.
And peek()
pops the front
node from the list. Only pop()
should be doing that.
And pop()
does not check if rear
is pointing at the node being popped. You are not resetting rear
to NULL in that case.
And you don't free any nodes remaining in the list before exiting the program.
Try something more like this instead:
#include <iostream>
#include <limits>
struct node
{
int data;
node *next;
node(int value): data(value), next(NULL) {}
};
node *front = NULL;
node *rear = NULL;
void push(int x)
{
node **np = (rear) ? &(rear->next) : &front;
*np = new node(x);
rear = *np;
}
int peek()
{
if (front)
return front->data;
return -1;
}
int pop()
{
int x = peek();
if (front)
{
node *p = front;
front = front->next;
if (p == rear) rear = NULL;
delete p;
}
return x;
}
bool isEmpty()
{
return !front;
}
void clear()
{
while (front)
{
node *p = front;
front = front->next;
delete p;
}
rear = NULL;
}
void display()
{
node *n = front;
if (n)
{
cout << n->data;
while (n = n->next)
cout << ' ' << n->data;
}
}
int askForNumber(const char *prompt)
{
int n;
cout << prompt << ": ";
cin >> n;
cin.ignore(numeric_limits<streamsize>::max(), 'n');
return n;
}
int main()
{
int n, x;
n = askForNumber("Enter the number of values to be pushed into queue");
for(int c = 0; c < n; ++c)
{
x = askForNumber("Enter the value to be entered into queue");
push(x);
}
cout << endl;
cout << "Pop value: ";
if (!isEmpty())
cout << pop();
else
cout << "empty queue";
cout << endl;
cout << "Peak value: ";
if (!isEmpty())
cout << peek();
else
cout << "empty queue";
cout << endl;
if (isEmpty())
cout << "The list is empty";
else
cout << "The list is not empty";
cout << endl;
cout << "The current contents of the stack are:" << endl;
display();
cout << endl;
clear();
cin.get();
return 0;
}
There are some issues with your code.
You have defined an isEmpty()
function, but you don't really it. You have declared a separate variable is_empty
that you set to the return value of isEmpty()
one time before you populate the list, and never update is_empty
after making any changes to the list. That is why your code is always reporting the list is "empty" even if it is really not. You need to get rid of the is_empty
variable and call isEmpty()
every time you need to check for empty.
Also, the return value of peek()
and pop()
are indeterminate if front
is NULL.
And peek()
pops the front
node from the list. Only pop()
should be doing that.
And pop()
does not check if rear
is pointing at the node being popped. You are not resetting rear
to NULL in that case.
And you don't free any nodes remaining in the list before exiting the program.
Try something more like this instead:
#include <iostream>
#include <limits>
struct node
{
int data;
node *next;
node(int value): data(value), next(NULL) {}
};
node *front = NULL;
node *rear = NULL;
void push(int x)
{
node **np = (rear) ? &(rear->next) : &front;
*np = new node(x);
rear = *np;
}
int peek()
{
if (front)
return front->data;
return -1;
}
int pop()
{
int x = peek();
if (front)
{
node *p = front;
front = front->next;
if (p == rear) rear = NULL;
delete p;
}
return x;
}
bool isEmpty()
{
return !front;
}
void clear()
{
while (front)
{
node *p = front;
front = front->next;
delete p;
}
rear = NULL;
}
void display()
{
node *n = front;
if (n)
{
cout << n->data;
while (n = n->next)
cout << ' ' << n->data;
}
}
int askForNumber(const char *prompt)
{
int n;
cout << prompt << ": ";
cin >> n;
cin.ignore(numeric_limits<streamsize>::max(), 'n');
return n;
}
int main()
{
int n, x;
n = askForNumber("Enter the number of values to be pushed into queue");
for(int c = 0; c < n; ++c)
{
x = askForNumber("Enter the value to be entered into queue");
push(x);
}
cout << endl;
cout << "Pop value: ";
if (!isEmpty())
cout << pop();
else
cout << "empty queue";
cout << endl;
cout << "Peak value: ";
if (!isEmpty())
cout << peek();
else
cout << "empty queue";
cout << endl;
if (isEmpty())
cout << "The list is empty";
else
cout << "The list is not empty";
cout << endl;
cout << "The current contents of the stack are:" << endl;
display();
cout << endl;
clear();
cin.get();
return 0;
}
edited Nov 20 at 3:06
answered Nov 20 at 2:51
Remy Lebeau
328k18246435
328k18246435
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384107%2flinked-list-stack-question-in-regards-to-the-stack-being-empty%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sl2Gupn AutLQWrso2DGsUA80J 4oE PUKZo1RRZ ZMFGFjwBXXapYHHN9gC7TUm3 kzTxKtf
What do you think
is_empty
does?– Fei Xiang
Nov 19 at 23:39
You set
is_empty
1 time at the beginning of yourmain()
it does not change its value after regardless of the condition of the stack. Instead callisEmpty()
each time you want to check.– drescherjm
Nov 19 at 23:42
@drescherjm thank you that was very helpful.
– Jenny Nicky
Nov 19 at 23:49
@drescherjm do you also know why this code does not properly display the contents of the list and only displays the list if the list is empty.
– Jenny Nicky
Nov 19 at 23:50
Did you put at least 2 items in the stack?
if (front != NULL) cout<<pop()<<endl;
removes the first item if there is one.– drescherjm
Nov 19 at 23:54