Displaying PHP variables within Javascript loop
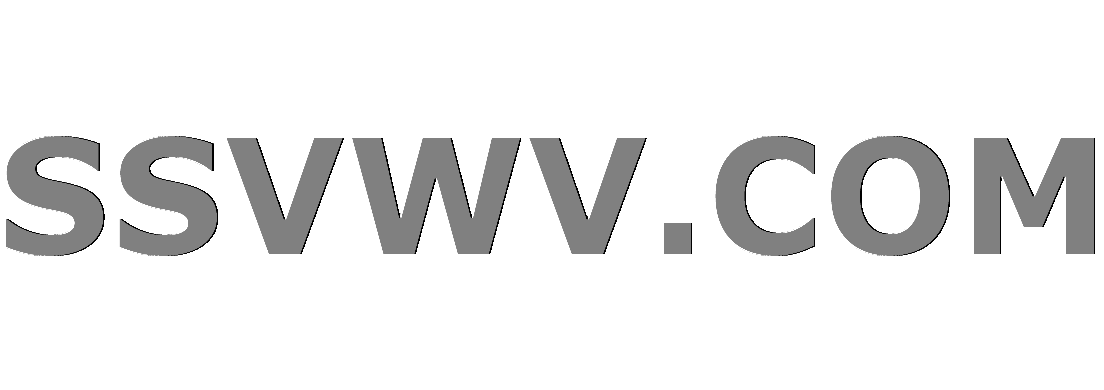
Multi tool use
I am currently developing a stock market simulator and I am attempting to display the first generated price for each virtual company. To do this, I have generated my prices in PHP and added them to the array '$firstValue'. I have also generated IDs for my HTML elements in which these values will be displayed and these are stored in '$priceIdentifiers'. Below is the code used to generate the values:
PHP:
$priceIdentifiers = array("Prices1", "Prices2", "Prices3");
$normalDistChangers = array("-1000", "1000");
$highValues = array("2500", "4850", "1780");
$lowValues = array("2200", "4300", "1400");
$firstValue = array();
for ($x = 0, $length = 3; $x < $length; $x++)
{
$tempValue = (rand($lowValues[$x], $highValues[$x]) / 100);
$tempMean = log(($tempValue) / (rand($lowValues[$x], $highValues[$x]) / 100));
$tempAnnStdDev = sqrt(365 * ($tempMean * $tempMean));
$tempNormalDist = (rand($normalDistChangers[0], $normalDistChangers[1]) / 1000);
$tempPrice = number_format(($tempValue * (1 + ($tempMean * (1/100000)) + $tempAnnStdDev * sqrt(1 / 100000) * $tempNormalDist)), 2, '.', '');
array_push($firstValue, $tempPrice);
}
Below is the code for the HTML elements in which the prices will be displayed. As I am working with 3 companies at the moment, there are 3 rows generated.
HTML and PHP:
<td style = "text-align:center;font-size:15pt" id = "<?php echo $priceIdentifiers[$a]; ?>"></td>
Where $a is a variable equal to 0 and increments until its value is equal to 2.
Generating the values has worked and I have seen in the console log that, when displaying the contents of the list, the 3 prices for the different companies are in the list but when attempting to display them using the following Javascript code, it ends up displaying the whole array and not the individual element:
Javascript:
var tempPriceOne = <?php echo json_encode($firstValue); ?>;
var a = 0;
var highPrice = tempPriceOne;
var inv = setInterval(function() {
if (a < 50)
{
document.getElementById("Prices1").innerHTML = tempPriceOne;
At the moment, when looking on the website: http://leonid.chashchin.net/stockMarket.php , it displays the whole array in the first 'Price' row. What do I need to modify so that the first element is displayed in the first row, the second in the second and so on?
javascript php html
|
show 1 more comment
I am currently developing a stock market simulator and I am attempting to display the first generated price for each virtual company. To do this, I have generated my prices in PHP and added them to the array '$firstValue'. I have also generated IDs for my HTML elements in which these values will be displayed and these are stored in '$priceIdentifiers'. Below is the code used to generate the values:
PHP:
$priceIdentifiers = array("Prices1", "Prices2", "Prices3");
$normalDistChangers = array("-1000", "1000");
$highValues = array("2500", "4850", "1780");
$lowValues = array("2200", "4300", "1400");
$firstValue = array();
for ($x = 0, $length = 3; $x < $length; $x++)
{
$tempValue = (rand($lowValues[$x], $highValues[$x]) / 100);
$tempMean = log(($tempValue) / (rand($lowValues[$x], $highValues[$x]) / 100));
$tempAnnStdDev = sqrt(365 * ($tempMean * $tempMean));
$tempNormalDist = (rand($normalDistChangers[0], $normalDistChangers[1]) / 1000);
$tempPrice = number_format(($tempValue * (1 + ($tempMean * (1/100000)) + $tempAnnStdDev * sqrt(1 / 100000) * $tempNormalDist)), 2, '.', '');
array_push($firstValue, $tempPrice);
}
Below is the code for the HTML elements in which the prices will be displayed. As I am working with 3 companies at the moment, there are 3 rows generated.
HTML and PHP:
<td style = "text-align:center;font-size:15pt" id = "<?php echo $priceIdentifiers[$a]; ?>"></td>
Where $a is a variable equal to 0 and increments until its value is equal to 2.
Generating the values has worked and I have seen in the console log that, when displaying the contents of the list, the 3 prices for the different companies are in the list but when attempting to display them using the following Javascript code, it ends up displaying the whole array and not the individual element:
Javascript:
var tempPriceOne = <?php echo json_encode($firstValue); ?>;
var a = 0;
var highPrice = tempPriceOne;
var inv = setInterval(function() {
if (a < 50)
{
document.getElementById("Prices1").innerHTML = tempPriceOne;
At the moment, when looking on the website: http://leonid.chashchin.net/stockMarket.php , it displays the whole array in the first 'Price' row. What do I need to modify so that the first element is displayed in the first row, the second in the second and so on?
javascript php html
the tempPriceOne is an array of strings. From view source: var tempPriceOne = ["24.24","47.25","15.21"];
– Nawed Khan
Nov 25 '18 at 21:15
Welcome.$firstValue
is an array. What exactly do you expect the value of that cell to be?
– Jeto
Nov 25 '18 at 21:16
@Nawed Khan yes that is correct. What I am struggling with at the moment is displaying each element in that list individually as the rows go down and the element in priceIdentifiers increments.
– Leo Chashchin
Nov 25 '18 at 21:17
@Jeto I have researched other posts about implementing PHP array elements into Javascript and have tried to use the json_encode method. I had hoped that it would display each element in the list individually but, however, this did not work.
– Leo Chashchin
Nov 25 '18 at 21:19
@LeoChashchin What you did does put the array into a JS variable, and is the proper way to do it. Your issue is that you're not looping over the array (on JS side), but instead outputting it "as is" into a cell expecting a single (string) value.
– Jeto
Nov 25 '18 at 21:20
|
show 1 more comment
I am currently developing a stock market simulator and I am attempting to display the first generated price for each virtual company. To do this, I have generated my prices in PHP and added them to the array '$firstValue'. I have also generated IDs for my HTML elements in which these values will be displayed and these are stored in '$priceIdentifiers'. Below is the code used to generate the values:
PHP:
$priceIdentifiers = array("Prices1", "Prices2", "Prices3");
$normalDistChangers = array("-1000", "1000");
$highValues = array("2500", "4850", "1780");
$lowValues = array("2200", "4300", "1400");
$firstValue = array();
for ($x = 0, $length = 3; $x < $length; $x++)
{
$tempValue = (rand($lowValues[$x], $highValues[$x]) / 100);
$tempMean = log(($tempValue) / (rand($lowValues[$x], $highValues[$x]) / 100));
$tempAnnStdDev = sqrt(365 * ($tempMean * $tempMean));
$tempNormalDist = (rand($normalDistChangers[0], $normalDistChangers[1]) / 1000);
$tempPrice = number_format(($tempValue * (1 + ($tempMean * (1/100000)) + $tempAnnStdDev * sqrt(1 / 100000) * $tempNormalDist)), 2, '.', '');
array_push($firstValue, $tempPrice);
}
Below is the code for the HTML elements in which the prices will be displayed. As I am working with 3 companies at the moment, there are 3 rows generated.
HTML and PHP:
<td style = "text-align:center;font-size:15pt" id = "<?php echo $priceIdentifiers[$a]; ?>"></td>
Where $a is a variable equal to 0 and increments until its value is equal to 2.
Generating the values has worked and I have seen in the console log that, when displaying the contents of the list, the 3 prices for the different companies are in the list but when attempting to display them using the following Javascript code, it ends up displaying the whole array and not the individual element:
Javascript:
var tempPriceOne = <?php echo json_encode($firstValue); ?>;
var a = 0;
var highPrice = tempPriceOne;
var inv = setInterval(function() {
if (a < 50)
{
document.getElementById("Prices1").innerHTML = tempPriceOne;
At the moment, when looking on the website: http://leonid.chashchin.net/stockMarket.php , it displays the whole array in the first 'Price' row. What do I need to modify so that the first element is displayed in the first row, the second in the second and so on?
javascript php html
I am currently developing a stock market simulator and I am attempting to display the first generated price for each virtual company. To do this, I have generated my prices in PHP and added them to the array '$firstValue'. I have also generated IDs for my HTML elements in which these values will be displayed and these are stored in '$priceIdentifiers'. Below is the code used to generate the values:
PHP:
$priceIdentifiers = array("Prices1", "Prices2", "Prices3");
$normalDistChangers = array("-1000", "1000");
$highValues = array("2500", "4850", "1780");
$lowValues = array("2200", "4300", "1400");
$firstValue = array();
for ($x = 0, $length = 3; $x < $length; $x++)
{
$tempValue = (rand($lowValues[$x], $highValues[$x]) / 100);
$tempMean = log(($tempValue) / (rand($lowValues[$x], $highValues[$x]) / 100));
$tempAnnStdDev = sqrt(365 * ($tempMean * $tempMean));
$tempNormalDist = (rand($normalDistChangers[0], $normalDistChangers[1]) / 1000);
$tempPrice = number_format(($tempValue * (1 + ($tempMean * (1/100000)) + $tempAnnStdDev * sqrt(1 / 100000) * $tempNormalDist)), 2, '.', '');
array_push($firstValue, $tempPrice);
}
Below is the code for the HTML elements in which the prices will be displayed. As I am working with 3 companies at the moment, there are 3 rows generated.
HTML and PHP:
<td style = "text-align:center;font-size:15pt" id = "<?php echo $priceIdentifiers[$a]; ?>"></td>
Where $a is a variable equal to 0 and increments until its value is equal to 2.
Generating the values has worked and I have seen in the console log that, when displaying the contents of the list, the 3 prices for the different companies are in the list but when attempting to display them using the following Javascript code, it ends up displaying the whole array and not the individual element:
Javascript:
var tempPriceOne = <?php echo json_encode($firstValue); ?>;
var a = 0;
var highPrice = tempPriceOne;
var inv = setInterval(function() {
if (a < 50)
{
document.getElementById("Prices1").innerHTML = tempPriceOne;
At the moment, when looking on the website: http://leonid.chashchin.net/stockMarket.php , it displays the whole array in the first 'Price' row. What do I need to modify so that the first element is displayed in the first row, the second in the second and so on?
javascript php html
javascript php html
asked Nov 25 '18 at 21:04
Leo ChashchinLeo Chashchin
205
205
the tempPriceOne is an array of strings. From view source: var tempPriceOne = ["24.24","47.25","15.21"];
– Nawed Khan
Nov 25 '18 at 21:15
Welcome.$firstValue
is an array. What exactly do you expect the value of that cell to be?
– Jeto
Nov 25 '18 at 21:16
@Nawed Khan yes that is correct. What I am struggling with at the moment is displaying each element in that list individually as the rows go down and the element in priceIdentifiers increments.
– Leo Chashchin
Nov 25 '18 at 21:17
@Jeto I have researched other posts about implementing PHP array elements into Javascript and have tried to use the json_encode method. I had hoped that it would display each element in the list individually but, however, this did not work.
– Leo Chashchin
Nov 25 '18 at 21:19
@LeoChashchin What you did does put the array into a JS variable, and is the proper way to do it. Your issue is that you're not looping over the array (on JS side), but instead outputting it "as is" into a cell expecting a single (string) value.
– Jeto
Nov 25 '18 at 21:20
|
show 1 more comment
the tempPriceOne is an array of strings. From view source: var tempPriceOne = ["24.24","47.25","15.21"];
– Nawed Khan
Nov 25 '18 at 21:15
Welcome.$firstValue
is an array. What exactly do you expect the value of that cell to be?
– Jeto
Nov 25 '18 at 21:16
@Nawed Khan yes that is correct. What I am struggling with at the moment is displaying each element in that list individually as the rows go down and the element in priceIdentifiers increments.
– Leo Chashchin
Nov 25 '18 at 21:17
@Jeto I have researched other posts about implementing PHP array elements into Javascript and have tried to use the json_encode method. I had hoped that it would display each element in the list individually but, however, this did not work.
– Leo Chashchin
Nov 25 '18 at 21:19
@LeoChashchin What you did does put the array into a JS variable, and is the proper way to do it. Your issue is that you're not looping over the array (on JS side), but instead outputting it "as is" into a cell expecting a single (string) value.
– Jeto
Nov 25 '18 at 21:20
the tempPriceOne is an array of strings. From view source: var tempPriceOne = ["24.24","47.25","15.21"];
– Nawed Khan
Nov 25 '18 at 21:15
the tempPriceOne is an array of strings. From view source: var tempPriceOne = ["24.24","47.25","15.21"];
– Nawed Khan
Nov 25 '18 at 21:15
Welcome.
$firstValue
is an array. What exactly do you expect the value of that cell to be?– Jeto
Nov 25 '18 at 21:16
Welcome.
$firstValue
is an array. What exactly do you expect the value of that cell to be?– Jeto
Nov 25 '18 at 21:16
@Nawed Khan yes that is correct. What I am struggling with at the moment is displaying each element in that list individually as the rows go down and the element in priceIdentifiers increments.
– Leo Chashchin
Nov 25 '18 at 21:17
@Nawed Khan yes that is correct. What I am struggling with at the moment is displaying each element in that list individually as the rows go down and the element in priceIdentifiers increments.
– Leo Chashchin
Nov 25 '18 at 21:17
@Jeto I have researched other posts about implementing PHP array elements into Javascript and have tried to use the json_encode method. I had hoped that it would display each element in the list individually but, however, this did not work.
– Leo Chashchin
Nov 25 '18 at 21:19
@Jeto I have researched other posts about implementing PHP array elements into Javascript and have tried to use the json_encode method. I had hoped that it would display each element in the list individually but, however, this did not work.
– Leo Chashchin
Nov 25 '18 at 21:19
@LeoChashchin What you did does put the array into a JS variable, and is the proper way to do it. Your issue is that you're not looping over the array (on JS side), but instead outputting it "as is" into a cell expecting a single (string) value.
– Jeto
Nov 25 '18 at 21:20
@LeoChashchin What you did does put the array into a JS variable, and is the proper way to do it. Your issue is that you're not looping over the array (on JS side), but instead outputting it "as is" into a cell expecting a single (string) value.
– Jeto
Nov 25 '18 at 21:20
|
show 1 more comment
1 Answer
1
active
oldest
votes
You are printing/assigning the whole array and not just the individual elements.
This is how you would print individual elements:
document.getElementById("Prices1").innerHTML = tempPriceOne[0];
document.getElementById("Prices2").innerHTML = tempPriceOne[1];
document.getElementById("Prices3").innerHTML = tempPriceOne[2];
1
This pretty much did the job for me. Quite surprised that I didn't see this as a solution but was just confused with being able to understand exactly what json_encode actually did. Thank you very much for your response.
– Leo Chashchin
Nov 25 '18 at 21:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471972%2fdisplaying-php-variables-within-javascript-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are printing/assigning the whole array and not just the individual elements.
This is how you would print individual elements:
document.getElementById("Prices1").innerHTML = tempPriceOne[0];
document.getElementById("Prices2").innerHTML = tempPriceOne[1];
document.getElementById("Prices3").innerHTML = tempPriceOne[2];
1
This pretty much did the job for me. Quite surprised that I didn't see this as a solution but was just confused with being able to understand exactly what json_encode actually did. Thank you very much for your response.
– Leo Chashchin
Nov 25 '18 at 21:26
add a comment |
You are printing/assigning the whole array and not just the individual elements.
This is how you would print individual elements:
document.getElementById("Prices1").innerHTML = tempPriceOne[0];
document.getElementById("Prices2").innerHTML = tempPriceOne[1];
document.getElementById("Prices3").innerHTML = tempPriceOne[2];
1
This pretty much did the job for me. Quite surprised that I didn't see this as a solution but was just confused with being able to understand exactly what json_encode actually did. Thank you very much for your response.
– Leo Chashchin
Nov 25 '18 at 21:26
add a comment |
You are printing/assigning the whole array and not just the individual elements.
This is how you would print individual elements:
document.getElementById("Prices1").innerHTML = tempPriceOne[0];
document.getElementById("Prices2").innerHTML = tempPriceOne[1];
document.getElementById("Prices3").innerHTML = tempPriceOne[2];
You are printing/assigning the whole array and not just the individual elements.
This is how you would print individual elements:
document.getElementById("Prices1").innerHTML = tempPriceOne[0];
document.getElementById("Prices2").innerHTML = tempPriceOne[1];
document.getElementById("Prices3").innerHTML = tempPriceOne[2];
answered Nov 25 '18 at 21:21


Nawed KhanNawed Khan
2,8241618
2,8241618
1
This pretty much did the job for me. Quite surprised that I didn't see this as a solution but was just confused with being able to understand exactly what json_encode actually did. Thank you very much for your response.
– Leo Chashchin
Nov 25 '18 at 21:26
add a comment |
1
This pretty much did the job for me. Quite surprised that I didn't see this as a solution but was just confused with being able to understand exactly what json_encode actually did. Thank you very much for your response.
– Leo Chashchin
Nov 25 '18 at 21:26
1
1
This pretty much did the job for me. Quite surprised that I didn't see this as a solution but was just confused with being able to understand exactly what json_encode actually did. Thank you very much for your response.
– Leo Chashchin
Nov 25 '18 at 21:26
This pretty much did the job for me. Quite surprised that I didn't see this as a solution but was just confused with being able to understand exactly what json_encode actually did. Thank you very much for your response.
– Leo Chashchin
Nov 25 '18 at 21:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471972%2fdisplaying-php-variables-within-javascript-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hQmxOztG,GM k ZEVCpDeV7,3okte 63qEsZw Fi qK7s ooADYOZRvUoiUnFw
the tempPriceOne is an array of strings. From view source: var tempPriceOne = ["24.24","47.25","15.21"];
– Nawed Khan
Nov 25 '18 at 21:15
Welcome.
$firstValue
is an array. What exactly do you expect the value of that cell to be?– Jeto
Nov 25 '18 at 21:16
@Nawed Khan yes that is correct. What I am struggling with at the moment is displaying each element in that list individually as the rows go down and the element in priceIdentifiers increments.
– Leo Chashchin
Nov 25 '18 at 21:17
@Jeto I have researched other posts about implementing PHP array elements into Javascript and have tried to use the json_encode method. I had hoped that it would display each element in the list individually but, however, this did not work.
– Leo Chashchin
Nov 25 '18 at 21:19
@LeoChashchin What you did does put the array into a JS variable, and is the proper way to do it. Your issue is that you're not looping over the array (on JS side), but instead outputting it "as is" into a cell expecting a single (string) value.
– Jeto
Nov 25 '18 at 21:20