Clean object from nulls and undefined
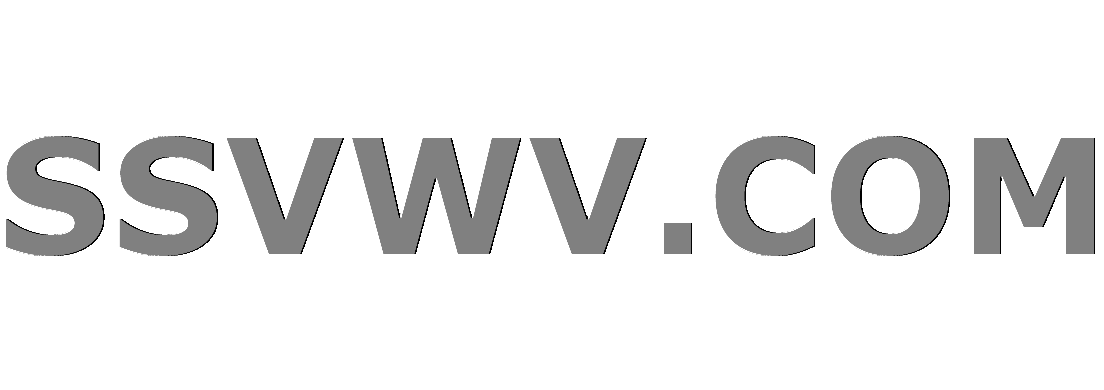
Multi tool use
I've written the following function which cleans nulls and undefined from an object, but for some reason it also removes key where the value is 0.
For example:
{
key1: 'value1',
key2: 0
}
The function will remove key2, even though it should not.
Here is the function:
const cleanJson = function cleanJson (obj) {
if (Object.prototype.toString.call(obj) !== '[object Object]') return obj;
return Object.keys(obj).filter(key => obj[key] && obj[key] !== 'delete').reduce((newObj, key) => {
newObj[key] = Object.prototype.toString.call(obj[key]) !== '[object Object]' ? obj[key] : cleanJson(obj[key]);
return newObj;
}, {});
};
Please advise.
javascript node.js
add a comment |
I've written the following function which cleans nulls and undefined from an object, but for some reason it also removes key where the value is 0.
For example:
{
key1: 'value1',
key2: 0
}
The function will remove key2, even though it should not.
Here is the function:
const cleanJson = function cleanJson (obj) {
if (Object.prototype.toString.call(obj) !== '[object Object]') return obj;
return Object.keys(obj).filter(key => obj[key] && obj[key] !== 'delete').reduce((newObj, key) => {
newObj[key] = Object.prototype.toString.call(obj[key]) !== '[object Object]' ? obj[key] : cleanJson(obj[key]);
return newObj;
}, {});
};
Please advise.
javascript node.js
1
Please read the description of thejson
tag, especially what is in capitals.
– trincot
Nov 25 '18 at 20:53
2
0
is "falsy", so it gets removed. Your function also cleans up things that evaluate to false.
– Houseman
Nov 25 '18 at 20:56
add a comment |
I've written the following function which cleans nulls and undefined from an object, but for some reason it also removes key where the value is 0.
For example:
{
key1: 'value1',
key2: 0
}
The function will remove key2, even though it should not.
Here is the function:
const cleanJson = function cleanJson (obj) {
if (Object.prototype.toString.call(obj) !== '[object Object]') return obj;
return Object.keys(obj).filter(key => obj[key] && obj[key] !== 'delete').reduce((newObj, key) => {
newObj[key] = Object.prototype.toString.call(obj[key]) !== '[object Object]' ? obj[key] : cleanJson(obj[key]);
return newObj;
}, {});
};
Please advise.
javascript node.js
I've written the following function which cleans nulls and undefined from an object, but for some reason it also removes key where the value is 0.
For example:
{
key1: 'value1',
key2: 0
}
The function will remove key2, even though it should not.
Here is the function:
const cleanJson = function cleanJson (obj) {
if (Object.prototype.toString.call(obj) !== '[object Object]') return obj;
return Object.keys(obj).filter(key => obj[key] && obj[key] !== 'delete').reduce((newObj, key) => {
newObj[key] = Object.prototype.toString.call(obj[key]) !== '[object Object]' ? obj[key] : cleanJson(obj[key]);
return newObj;
}, {});
};
Please advise.
javascript node.js
javascript node.js
edited Nov 25 '18 at 20:57


trincot
126k1688122
126k1688122
asked Nov 25 '18 at 20:51
David FaizDavid Faiz
92892247
92892247
1
Please read the description of thejson
tag, especially what is in capitals.
– trincot
Nov 25 '18 at 20:53
2
0
is "falsy", so it gets removed. Your function also cleans up things that evaluate to false.
– Houseman
Nov 25 '18 at 20:56
add a comment |
1
Please read the description of thejson
tag, especially what is in capitals.
– trincot
Nov 25 '18 at 20:53
2
0
is "falsy", so it gets removed. Your function also cleans up things that evaluate to false.
– Houseman
Nov 25 '18 at 20:56
1
1
Please read the description of the
json
tag, especially what is in capitals.– trincot
Nov 25 '18 at 20:53
Please read the description of the
json
tag, especially what is in capitals.– trincot
Nov 25 '18 at 20:53
2
2
0
is "falsy", so it gets removed. Your function also cleans up things that evaluate to false.– Houseman
Nov 25 '18 at 20:56
0
is "falsy", so it gets removed. Your function also cleans up things that evaluate to false.– Houseman
Nov 25 '18 at 20:56
add a comment |
4 Answers
4
active
oldest
votes
Here is one option using Object.entries
:
const myJSON = {key1:'value1', key2:0, key3:null, key4:undefined, key5:""};
const myCleanJSON = Object.entries(myJSON)
.filter(([key, value]) => (value !== null && typeof value !== 'undefined'))
.reduce((acc, b) => ((!acc.length) ? {...acc, [b[0]]: b[1] } : { [acc[0]] : acc[1], [b[0]]: b[1] }));
console.log(myCleanJSON);
add a comment |
The problem is within
obj[key] && obj[key] !== 'delete'
When obj[key] == 0, would return false.
add a comment |
Because 0
(as well as the empty string
""
) evaluates to false when cast to a Boolean, you need to explicitly check for undefined
and null
:
let a = {
key1: 'value1',
key2: 0,
key3: null
}
function cleanProps(o) {
if (Object.prototype.toString.call(o) !== '[object Object]') return o
for (key in o) {
if([undefined, null].includes(o[key])) delete o[key]
}
return o
}
console.log(cleanProps(a))
add a comment |
I think that would solve your issue:
obj[key] !== null && obj[key] !== undefined && obj[key] !== 'delete'
You can just use!=
to catch bothnull
andundefined
– Mark Meyer
Nov 25 '18 at 21:11
And pick one or the other?
– Kevin Amiranoff
Nov 25 '18 at 21:14
Yes, bothundefined != null
andnull != undefined
arefalse
– Mark Meyer
Nov 25 '18 at 21:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471845%2fclean-object-from-nulls-and-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here is one option using Object.entries
:
const myJSON = {key1:'value1', key2:0, key3:null, key4:undefined, key5:""};
const myCleanJSON = Object.entries(myJSON)
.filter(([key, value]) => (value !== null && typeof value !== 'undefined'))
.reduce((acc, b) => ((!acc.length) ? {...acc, [b[0]]: b[1] } : { [acc[0]] : acc[1], [b[0]]: b[1] }));
console.log(myCleanJSON);
add a comment |
Here is one option using Object.entries
:
const myJSON = {key1:'value1', key2:0, key3:null, key4:undefined, key5:""};
const myCleanJSON = Object.entries(myJSON)
.filter(([key, value]) => (value !== null && typeof value !== 'undefined'))
.reduce((acc, b) => ((!acc.length) ? {...acc, [b[0]]: b[1] } : { [acc[0]] : acc[1], [b[0]]: b[1] }));
console.log(myCleanJSON);
add a comment |
Here is one option using Object.entries
:
const myJSON = {key1:'value1', key2:0, key3:null, key4:undefined, key5:""};
const myCleanJSON = Object.entries(myJSON)
.filter(([key, value]) => (value !== null && typeof value !== 'undefined'))
.reduce((acc, b) => ((!acc.length) ? {...acc, [b[0]]: b[1] } : { [acc[0]] : acc[1], [b[0]]: b[1] }));
console.log(myCleanJSON);
Here is one option using Object.entries
:
const myJSON = {key1:'value1', key2:0, key3:null, key4:undefined, key5:""};
const myCleanJSON = Object.entries(myJSON)
.filter(([key, value]) => (value !== null && typeof value !== 'undefined'))
.reduce((acc, b) => ((!acc.length) ? {...acc, [b[0]]: b[1] } : { [acc[0]] : acc[1], [b[0]]: b[1] }));
console.log(myCleanJSON);
const myJSON = {key1:'value1', key2:0, key3:null, key4:undefined, key5:""};
const myCleanJSON = Object.entries(myJSON)
.filter(([key, value]) => (value !== null && typeof value !== 'undefined'))
.reduce((acc, b) => ((!acc.length) ? {...acc, [b[0]]: b[1] } : { [acc[0]] : acc[1], [b[0]]: b[1] }));
console.log(myCleanJSON);
const myJSON = {key1:'value1', key2:0, key3:null, key4:undefined, key5:""};
const myCleanJSON = Object.entries(myJSON)
.filter(([key, value]) => (value !== null && typeof value !== 'undefined'))
.reduce((acc, b) => ((!acc.length) ? {...acc, [b[0]]: b[1] } : { [acc[0]] : acc[1], [b[0]]: b[1] }));
console.log(myCleanJSON);
answered Nov 25 '18 at 21:10


zvonazvona
12.6k14059
12.6k14059
add a comment |
add a comment |
The problem is within
obj[key] && obj[key] !== 'delete'
When obj[key] == 0, would return false.
add a comment |
The problem is within
obj[key] && obj[key] !== 'delete'
When obj[key] == 0, would return false.
add a comment |
The problem is within
obj[key] && obj[key] !== 'delete'
When obj[key] == 0, would return false.
The problem is within
obj[key] && obj[key] !== 'delete'
When obj[key] == 0, would return false.
answered Nov 25 '18 at 20:58


Guy YogevGuy Yogev
43239
43239
add a comment |
add a comment |
Because 0
(as well as the empty string
""
) evaluates to false when cast to a Boolean, you need to explicitly check for undefined
and null
:
let a = {
key1: 'value1',
key2: 0,
key3: null
}
function cleanProps(o) {
if (Object.prototype.toString.call(o) !== '[object Object]') return o
for (key in o) {
if([undefined, null].includes(o[key])) delete o[key]
}
return o
}
console.log(cleanProps(a))
add a comment |
Because 0
(as well as the empty string
""
) evaluates to false when cast to a Boolean, you need to explicitly check for undefined
and null
:
let a = {
key1: 'value1',
key2: 0,
key3: null
}
function cleanProps(o) {
if (Object.prototype.toString.call(o) !== '[object Object]') return o
for (key in o) {
if([undefined, null].includes(o[key])) delete o[key]
}
return o
}
console.log(cleanProps(a))
add a comment |
Because 0
(as well as the empty string
""
) evaluates to false when cast to a Boolean, you need to explicitly check for undefined
and null
:
let a = {
key1: 'value1',
key2: 0,
key3: null
}
function cleanProps(o) {
if (Object.prototype.toString.call(o) !== '[object Object]') return o
for (key in o) {
if([undefined, null].includes(o[key])) delete o[key]
}
return o
}
console.log(cleanProps(a))
Because 0
(as well as the empty string
""
) evaluates to false when cast to a Boolean, you need to explicitly check for undefined
and null
:
let a = {
key1: 'value1',
key2: 0,
key3: null
}
function cleanProps(o) {
if (Object.prototype.toString.call(o) !== '[object Object]') return o
for (key in o) {
if([undefined, null].includes(o[key])) delete o[key]
}
return o
}
console.log(cleanProps(a))
let a = {
key1: 'value1',
key2: 0,
key3: null
}
function cleanProps(o) {
if (Object.prototype.toString.call(o) !== '[object Object]') return o
for (key in o) {
if([undefined, null].includes(o[key])) delete o[key]
}
return o
}
console.log(cleanProps(a))
let a = {
key1: 'value1',
key2: 0,
key3: null
}
function cleanProps(o) {
if (Object.prototype.toString.call(o) !== '[object Object]') return o
for (key in o) {
if([undefined, null].includes(o[key])) delete o[key]
}
return o
}
console.log(cleanProps(a))
answered Nov 25 '18 at 21:18


connexoconnexo
22.4k83561
22.4k83561
add a comment |
add a comment |
I think that would solve your issue:
obj[key] !== null && obj[key] !== undefined && obj[key] !== 'delete'
You can just use!=
to catch bothnull
andundefined
– Mark Meyer
Nov 25 '18 at 21:11
And pick one or the other?
– Kevin Amiranoff
Nov 25 '18 at 21:14
Yes, bothundefined != null
andnull != undefined
arefalse
– Mark Meyer
Nov 25 '18 at 21:16
add a comment |
I think that would solve your issue:
obj[key] !== null && obj[key] !== undefined && obj[key] !== 'delete'
You can just use!=
to catch bothnull
andundefined
– Mark Meyer
Nov 25 '18 at 21:11
And pick one or the other?
– Kevin Amiranoff
Nov 25 '18 at 21:14
Yes, bothundefined != null
andnull != undefined
arefalse
– Mark Meyer
Nov 25 '18 at 21:16
add a comment |
I think that would solve your issue:
obj[key] !== null && obj[key] !== undefined && obj[key] !== 'delete'
I think that would solve your issue:
obj[key] !== null && obj[key] !== undefined && obj[key] !== 'delete'
answered Nov 25 '18 at 21:06


Kevin AmiranoffKevin Amiranoff
2,92651338
2,92651338
You can just use!=
to catch bothnull
andundefined
– Mark Meyer
Nov 25 '18 at 21:11
And pick one or the other?
– Kevin Amiranoff
Nov 25 '18 at 21:14
Yes, bothundefined != null
andnull != undefined
arefalse
– Mark Meyer
Nov 25 '18 at 21:16
add a comment |
You can just use!=
to catch bothnull
andundefined
– Mark Meyer
Nov 25 '18 at 21:11
And pick one or the other?
– Kevin Amiranoff
Nov 25 '18 at 21:14
Yes, bothundefined != null
andnull != undefined
arefalse
– Mark Meyer
Nov 25 '18 at 21:16
You can just use
!=
to catch both null
and undefined
– Mark Meyer
Nov 25 '18 at 21:11
You can just use
!=
to catch both null
and undefined
– Mark Meyer
Nov 25 '18 at 21:11
And pick one or the other?
– Kevin Amiranoff
Nov 25 '18 at 21:14
And pick one or the other?
– Kevin Amiranoff
Nov 25 '18 at 21:14
Yes, both
undefined != null
and null != undefined
are false
– Mark Meyer
Nov 25 '18 at 21:16
Yes, both
undefined != null
and null != undefined
are false
– Mark Meyer
Nov 25 '18 at 21:16
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471845%2fclean-object-from-nulls-and-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xtGDDkdU P ykOg l5ofjJ5E,Z75HhJHi TJy
1
Please read the description of the
json
tag, especially what is in capitals.– trincot
Nov 25 '18 at 20:53
2
0
is "falsy", so it gets removed. Your function also cleans up things that evaluate to false.– Houseman
Nov 25 '18 at 20:56