Why react native redux reducer is called twice sometimes and sometimes once only?
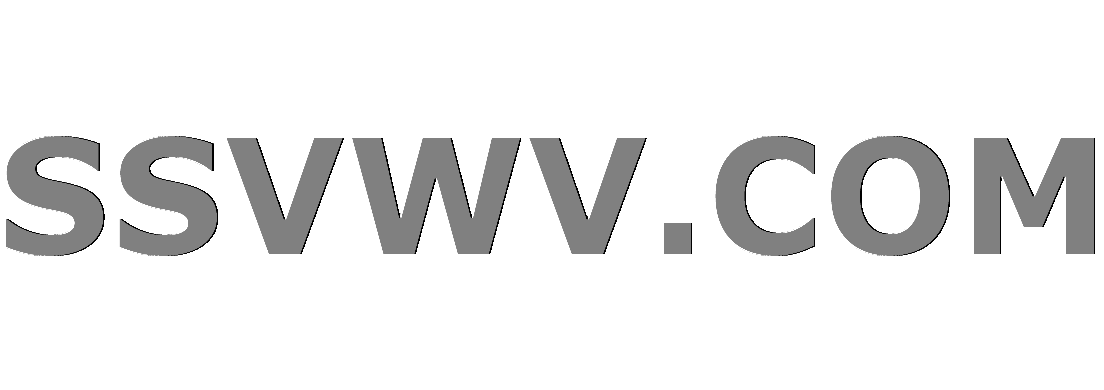
Multi tool use
I am working on React Native app. I am still new to react native and react world so I might be doing something wrong here that I don't know but I need help. So what is happening right now in my app when I try to update reducer state, it is calling it twice updating it twice.
Here is what I am trying to do,
1) When app opens, set default `deviceData` object.
2) Check for internet connection and update device data.
3) I disable internet that updates `deviceData` object once.
4) I enable internet and that updates `deviceData` object twice where it comes back as false.
Here is my code,
NoInternet.js
file
import React, {PureComponent} from 'react';
import {View, Text, NetInfo, Dimensions, StyleSheet} from 'react-native';
import {statusBarHeight} from "./AppBar";
import {RED_DARKEN_THREE} from "./colors";
import {connect} from "react-redux";
import {updateInternetState} from '../actions/internetStateChangeAction';
const {width} = Dimensions.get('window');
function MiniOfflineSign() {
return (
<View style={styles.offlineContainer}>
<Text style={styles.offlineText}>No Internet Connection</Text>
</View>
);
}
class OfflineNotice extends PureComponent {
state = {
isConnected: true
};
componentDidMount() {
NetInfo.isConnected.addEventListener('connectionChange', this.handleConnectivityChange);
}
componentWillUnmount() {
NetInfo.isConnected.removeEventListener('connectionChange', this.handleConnectivityChange);
}
handleConnectivityChange = isConnected => {
this.setState({isConnected});
this.props.updateConnectivityChange({isConnected});
};
render() {
if (!this.state.isConnected) {
return <MiniOfflineSign/>;
}
return null;
}
}
const styles = StyleSheet.create({
offlineContainer: {
backgroundColor: RED_DARKEN_THREE,
height: 30,
justifyContent: 'center',
alignItems: 'center',
flexDirection: 'row',
width,
position: 'absolute',
top: statusBarHeight,
},
offlineText: {
color: '#fff',
fontFamily: "Muli-Regular",
}
});
function mapStateToProps(state, {navigation}) {
return {
state,
navigation
};
}
const mapDispatchToProps = dispatch => ({
updateConnectivityChange: ({isConnected}) => {
dispatch(updateInternetState({isConnected}));
}
});
export default connect(mapStateToProps, mapDispatchToProps)(OfflineNotice);
internetStateChangeAction.js
file
export const UPDATE_INTERNET_STATE = 'UPDATE_INTERNET_STATE';
export const updateInternetState = data => ({
type: UPDATE_INTERNET_STATE,
data,
});
deviceReducer.js
file
import {Platform} from 'react-native';
import {UPDATE_INTERNET_STATE} from '../actions/internetStateChangeAction';
const deviceData = {
deviceType: Platform.OS,
deviceID: null,
isInternetAvailable: true,
};
const deviceDataReducer = (state = deviceData, action) => {
switch (action.type) {
case UPDATE_INTERNET_STATE:
return {
...state,
isInternetAvailable: action.data.isConnected
};
default:
return state;
}
};
export default deviceDataReducer;
And here is my debug log,
As you can see, there are four logs of actions.
1) First action is default when app is opened and it is called to check internet connection.
2) Second action is when I disable internet in my iMac by disconnecting from wifi (I am developing this app on iMac and using iOS simulator)
3) HERE IS THE MOST IMPORTANT PART, I should see only one action when I enable wifi access in my iMac and connect to internet. Which happens as third action and internet connection as true
4) But there is fourth action called automatically which returns false and that fails everything.
And if I disable internet again and enable again, it gets called twice again and I get following result,
I don't know where I am doing wrong and what!
Thanks everyone!
javascript reactjs react-native redux react-redux
|
show 1 more comment
I am working on React Native app. I am still new to react native and react world so I might be doing something wrong here that I don't know but I need help. So what is happening right now in my app when I try to update reducer state, it is calling it twice updating it twice.
Here is what I am trying to do,
1) When app opens, set default `deviceData` object.
2) Check for internet connection and update device data.
3) I disable internet that updates `deviceData` object once.
4) I enable internet and that updates `deviceData` object twice where it comes back as false.
Here is my code,
NoInternet.js
file
import React, {PureComponent} from 'react';
import {View, Text, NetInfo, Dimensions, StyleSheet} from 'react-native';
import {statusBarHeight} from "./AppBar";
import {RED_DARKEN_THREE} from "./colors";
import {connect} from "react-redux";
import {updateInternetState} from '../actions/internetStateChangeAction';
const {width} = Dimensions.get('window');
function MiniOfflineSign() {
return (
<View style={styles.offlineContainer}>
<Text style={styles.offlineText}>No Internet Connection</Text>
</View>
);
}
class OfflineNotice extends PureComponent {
state = {
isConnected: true
};
componentDidMount() {
NetInfo.isConnected.addEventListener('connectionChange', this.handleConnectivityChange);
}
componentWillUnmount() {
NetInfo.isConnected.removeEventListener('connectionChange', this.handleConnectivityChange);
}
handleConnectivityChange = isConnected => {
this.setState({isConnected});
this.props.updateConnectivityChange({isConnected});
};
render() {
if (!this.state.isConnected) {
return <MiniOfflineSign/>;
}
return null;
}
}
const styles = StyleSheet.create({
offlineContainer: {
backgroundColor: RED_DARKEN_THREE,
height: 30,
justifyContent: 'center',
alignItems: 'center',
flexDirection: 'row',
width,
position: 'absolute',
top: statusBarHeight,
},
offlineText: {
color: '#fff',
fontFamily: "Muli-Regular",
}
});
function mapStateToProps(state, {navigation}) {
return {
state,
navigation
};
}
const mapDispatchToProps = dispatch => ({
updateConnectivityChange: ({isConnected}) => {
dispatch(updateInternetState({isConnected}));
}
});
export default connect(mapStateToProps, mapDispatchToProps)(OfflineNotice);
internetStateChangeAction.js
file
export const UPDATE_INTERNET_STATE = 'UPDATE_INTERNET_STATE';
export const updateInternetState = data => ({
type: UPDATE_INTERNET_STATE,
data,
});
deviceReducer.js
file
import {Platform} from 'react-native';
import {UPDATE_INTERNET_STATE} from '../actions/internetStateChangeAction';
const deviceData = {
deviceType: Platform.OS,
deviceID: null,
isInternetAvailable: true,
};
const deviceDataReducer = (state = deviceData, action) => {
switch (action.type) {
case UPDATE_INTERNET_STATE:
return {
...state,
isInternetAvailable: action.data.isConnected
};
default:
return state;
}
};
export default deviceDataReducer;
And here is my debug log,
As you can see, there are four logs of actions.
1) First action is default when app is opened and it is called to check internet connection.
2) Second action is when I disable internet in my iMac by disconnecting from wifi (I am developing this app on iMac and using iOS simulator)
3) HERE IS THE MOST IMPORTANT PART, I should see only one action when I enable wifi access in my iMac and connect to internet. Which happens as third action and internet connection as true
4) But there is fourth action called automatically which returns false and that fails everything.
And if I disable internet again and enable again, it gets called twice again and I get following result,
I don't know where I am doing wrong and what!
Thanks everyone!
javascript reactjs react-native redux react-redux
your code looks fine for me. on the other side timestamps in your logs looks suspicious: according to themisConnected: true
comes just in 9ms afterisConnected: false
in both cases. is it possible you are so fast to switch connection off and on again? can it be some bug in emulator?
– skyboyer
Nov 25 '18 at 0:49
@skyboyer, thanks for replying me. I disconnect my iMac from wifi and it shows up 2nd action. I connect my machine and it takes about 3-5 seconds to properly connect to wifi and all and than about after 1-2 seconds, simulator gets connection and meanwhile I am not doing anything but looking at logs.
– Viral Joshi
Nov 25 '18 at 1:15
could you addconsole.log(isConnected)
inhandleConnectivityChange
? it is not unclear to me howEvent
object should come there transforms into booleantrue/false
in reducer later
– skyboyer
Nov 25 '18 at 1:40
@skyboyer, I will try to follow answer that I got from @Helmer. I will also post a screen shot ofisConnected
for you. :D
– Viral Joshi
Nov 25 '18 at 4:07
2
Hi Viral, please copy and paste your console logs instead of posting screenshots! Some users can't see images, and we also might want to copy and paste from your logs in the answer.
– stone
Nov 25 '18 at 8:37
|
show 1 more comment
I am working on React Native app. I am still new to react native and react world so I might be doing something wrong here that I don't know but I need help. So what is happening right now in my app when I try to update reducer state, it is calling it twice updating it twice.
Here is what I am trying to do,
1) When app opens, set default `deviceData` object.
2) Check for internet connection and update device data.
3) I disable internet that updates `deviceData` object once.
4) I enable internet and that updates `deviceData` object twice where it comes back as false.
Here is my code,
NoInternet.js
file
import React, {PureComponent} from 'react';
import {View, Text, NetInfo, Dimensions, StyleSheet} from 'react-native';
import {statusBarHeight} from "./AppBar";
import {RED_DARKEN_THREE} from "./colors";
import {connect} from "react-redux";
import {updateInternetState} from '../actions/internetStateChangeAction';
const {width} = Dimensions.get('window');
function MiniOfflineSign() {
return (
<View style={styles.offlineContainer}>
<Text style={styles.offlineText}>No Internet Connection</Text>
</View>
);
}
class OfflineNotice extends PureComponent {
state = {
isConnected: true
};
componentDidMount() {
NetInfo.isConnected.addEventListener('connectionChange', this.handleConnectivityChange);
}
componentWillUnmount() {
NetInfo.isConnected.removeEventListener('connectionChange', this.handleConnectivityChange);
}
handleConnectivityChange = isConnected => {
this.setState({isConnected});
this.props.updateConnectivityChange({isConnected});
};
render() {
if (!this.state.isConnected) {
return <MiniOfflineSign/>;
}
return null;
}
}
const styles = StyleSheet.create({
offlineContainer: {
backgroundColor: RED_DARKEN_THREE,
height: 30,
justifyContent: 'center',
alignItems: 'center',
flexDirection: 'row',
width,
position: 'absolute',
top: statusBarHeight,
},
offlineText: {
color: '#fff',
fontFamily: "Muli-Regular",
}
});
function mapStateToProps(state, {navigation}) {
return {
state,
navigation
};
}
const mapDispatchToProps = dispatch => ({
updateConnectivityChange: ({isConnected}) => {
dispatch(updateInternetState({isConnected}));
}
});
export default connect(mapStateToProps, mapDispatchToProps)(OfflineNotice);
internetStateChangeAction.js
file
export const UPDATE_INTERNET_STATE = 'UPDATE_INTERNET_STATE';
export const updateInternetState = data => ({
type: UPDATE_INTERNET_STATE,
data,
});
deviceReducer.js
file
import {Platform} from 'react-native';
import {UPDATE_INTERNET_STATE} from '../actions/internetStateChangeAction';
const deviceData = {
deviceType: Platform.OS,
deviceID: null,
isInternetAvailable: true,
};
const deviceDataReducer = (state = deviceData, action) => {
switch (action.type) {
case UPDATE_INTERNET_STATE:
return {
...state,
isInternetAvailable: action.data.isConnected
};
default:
return state;
}
};
export default deviceDataReducer;
And here is my debug log,
As you can see, there are four logs of actions.
1) First action is default when app is opened and it is called to check internet connection.
2) Second action is when I disable internet in my iMac by disconnecting from wifi (I am developing this app on iMac and using iOS simulator)
3) HERE IS THE MOST IMPORTANT PART, I should see only one action when I enable wifi access in my iMac and connect to internet. Which happens as third action and internet connection as true
4) But there is fourth action called automatically which returns false and that fails everything.
And if I disable internet again and enable again, it gets called twice again and I get following result,
I don't know where I am doing wrong and what!
Thanks everyone!
javascript reactjs react-native redux react-redux
I am working on React Native app. I am still new to react native and react world so I might be doing something wrong here that I don't know but I need help. So what is happening right now in my app when I try to update reducer state, it is calling it twice updating it twice.
Here is what I am trying to do,
1) When app opens, set default `deviceData` object.
2) Check for internet connection and update device data.
3) I disable internet that updates `deviceData` object once.
4) I enable internet and that updates `deviceData` object twice where it comes back as false.
Here is my code,
NoInternet.js
file
import React, {PureComponent} from 'react';
import {View, Text, NetInfo, Dimensions, StyleSheet} from 'react-native';
import {statusBarHeight} from "./AppBar";
import {RED_DARKEN_THREE} from "./colors";
import {connect} from "react-redux";
import {updateInternetState} from '../actions/internetStateChangeAction';
const {width} = Dimensions.get('window');
function MiniOfflineSign() {
return (
<View style={styles.offlineContainer}>
<Text style={styles.offlineText}>No Internet Connection</Text>
</View>
);
}
class OfflineNotice extends PureComponent {
state = {
isConnected: true
};
componentDidMount() {
NetInfo.isConnected.addEventListener('connectionChange', this.handleConnectivityChange);
}
componentWillUnmount() {
NetInfo.isConnected.removeEventListener('connectionChange', this.handleConnectivityChange);
}
handleConnectivityChange = isConnected => {
this.setState({isConnected});
this.props.updateConnectivityChange({isConnected});
};
render() {
if (!this.state.isConnected) {
return <MiniOfflineSign/>;
}
return null;
}
}
const styles = StyleSheet.create({
offlineContainer: {
backgroundColor: RED_DARKEN_THREE,
height: 30,
justifyContent: 'center',
alignItems: 'center',
flexDirection: 'row',
width,
position: 'absolute',
top: statusBarHeight,
},
offlineText: {
color: '#fff',
fontFamily: "Muli-Regular",
}
});
function mapStateToProps(state, {navigation}) {
return {
state,
navigation
};
}
const mapDispatchToProps = dispatch => ({
updateConnectivityChange: ({isConnected}) => {
dispatch(updateInternetState({isConnected}));
}
});
export default connect(mapStateToProps, mapDispatchToProps)(OfflineNotice);
internetStateChangeAction.js
file
export const UPDATE_INTERNET_STATE = 'UPDATE_INTERNET_STATE';
export const updateInternetState = data => ({
type: UPDATE_INTERNET_STATE,
data,
});
deviceReducer.js
file
import {Platform} from 'react-native';
import {UPDATE_INTERNET_STATE} from '../actions/internetStateChangeAction';
const deviceData = {
deviceType: Platform.OS,
deviceID: null,
isInternetAvailable: true,
};
const deviceDataReducer = (state = deviceData, action) => {
switch (action.type) {
case UPDATE_INTERNET_STATE:
return {
...state,
isInternetAvailable: action.data.isConnected
};
default:
return state;
}
};
export default deviceDataReducer;
And here is my debug log,
As you can see, there are four logs of actions.
1) First action is default when app is opened and it is called to check internet connection.
2) Second action is when I disable internet in my iMac by disconnecting from wifi (I am developing this app on iMac and using iOS simulator)
3) HERE IS THE MOST IMPORTANT PART, I should see only one action when I enable wifi access in my iMac and connect to internet. Which happens as third action and internet connection as true
4) But there is fourth action called automatically which returns false and that fails everything.
And if I disable internet again and enable again, it gets called twice again and I get following result,
I don't know where I am doing wrong and what!
Thanks everyone!
javascript reactjs react-native redux react-redux
javascript reactjs react-native redux react-redux
asked Nov 24 '18 at 23:51
Viral JoshiViral Joshi
87110
87110
your code looks fine for me. on the other side timestamps in your logs looks suspicious: according to themisConnected: true
comes just in 9ms afterisConnected: false
in both cases. is it possible you are so fast to switch connection off and on again? can it be some bug in emulator?
– skyboyer
Nov 25 '18 at 0:49
@skyboyer, thanks for replying me. I disconnect my iMac from wifi and it shows up 2nd action. I connect my machine and it takes about 3-5 seconds to properly connect to wifi and all and than about after 1-2 seconds, simulator gets connection and meanwhile I am not doing anything but looking at logs.
– Viral Joshi
Nov 25 '18 at 1:15
could you addconsole.log(isConnected)
inhandleConnectivityChange
? it is not unclear to me howEvent
object should come there transforms into booleantrue/false
in reducer later
– skyboyer
Nov 25 '18 at 1:40
@skyboyer, I will try to follow answer that I got from @Helmer. I will also post a screen shot ofisConnected
for you. :D
– Viral Joshi
Nov 25 '18 at 4:07
2
Hi Viral, please copy and paste your console logs instead of posting screenshots! Some users can't see images, and we also might want to copy and paste from your logs in the answer.
– stone
Nov 25 '18 at 8:37
|
show 1 more comment
your code looks fine for me. on the other side timestamps in your logs looks suspicious: according to themisConnected: true
comes just in 9ms afterisConnected: false
in both cases. is it possible you are so fast to switch connection off and on again? can it be some bug in emulator?
– skyboyer
Nov 25 '18 at 0:49
@skyboyer, thanks for replying me. I disconnect my iMac from wifi and it shows up 2nd action. I connect my machine and it takes about 3-5 seconds to properly connect to wifi and all and than about after 1-2 seconds, simulator gets connection and meanwhile I am not doing anything but looking at logs.
– Viral Joshi
Nov 25 '18 at 1:15
could you addconsole.log(isConnected)
inhandleConnectivityChange
? it is not unclear to me howEvent
object should come there transforms into booleantrue/false
in reducer later
– skyboyer
Nov 25 '18 at 1:40
@skyboyer, I will try to follow answer that I got from @Helmer. I will also post a screen shot ofisConnected
for you. :D
– Viral Joshi
Nov 25 '18 at 4:07
2
Hi Viral, please copy and paste your console logs instead of posting screenshots! Some users can't see images, and we also might want to copy and paste from your logs in the answer.
– stone
Nov 25 '18 at 8:37
your code looks fine for me. on the other side timestamps in your logs looks suspicious: according to them
isConnected: true
comes just in 9ms after isConnected: false
in both cases. is it possible you are so fast to switch connection off and on again? can it be some bug in emulator?– skyboyer
Nov 25 '18 at 0:49
your code looks fine for me. on the other side timestamps in your logs looks suspicious: according to them
isConnected: true
comes just in 9ms after isConnected: false
in both cases. is it possible you are so fast to switch connection off and on again? can it be some bug in emulator?– skyboyer
Nov 25 '18 at 0:49
@skyboyer, thanks for replying me. I disconnect my iMac from wifi and it shows up 2nd action. I connect my machine and it takes about 3-5 seconds to properly connect to wifi and all and than about after 1-2 seconds, simulator gets connection and meanwhile I am not doing anything but looking at logs.
– Viral Joshi
Nov 25 '18 at 1:15
@skyboyer, thanks for replying me. I disconnect my iMac from wifi and it shows up 2nd action. I connect my machine and it takes about 3-5 seconds to properly connect to wifi and all and than about after 1-2 seconds, simulator gets connection and meanwhile I am not doing anything but looking at logs.
– Viral Joshi
Nov 25 '18 at 1:15
could you add
console.log(isConnected)
in handleConnectivityChange
? it is not unclear to me how Event
object should come there transforms into boolean true/false
in reducer later– skyboyer
Nov 25 '18 at 1:40
could you add
console.log(isConnected)
in handleConnectivityChange
? it is not unclear to me how Event
object should come there transforms into boolean true/false
in reducer later– skyboyer
Nov 25 '18 at 1:40
@skyboyer, I will try to follow answer that I got from @Helmer. I will also post a screen shot of
isConnected
for you. :D– Viral Joshi
Nov 25 '18 at 4:07
@skyboyer, I will try to follow answer that I got from @Helmer. I will also post a screen shot of
isConnected
for you. :D– Viral Joshi
Nov 25 '18 at 4:07
2
2
Hi Viral, please copy and paste your console logs instead of posting screenshots! Some users can't see images, and we also might want to copy and paste from your logs in the answer.
– stone
Nov 25 '18 at 8:37
Hi Viral, please copy and paste your console logs instead of posting screenshots! Some users can't see images, and we also might want to copy and paste from your logs in the answer.
– stone
Nov 25 '18 at 8:37
|
show 1 more comment
2 Answers
2
active
oldest
votes
There is a known problem with the NetInfo Component. It seems to return false after first reconnection. I would change your aproach. You need to keep in mind that NetInfo informs if there is a protocol connection to internet like WiFi or 3G, but not if data transit over internet is possible. You could try this by leaving WIFI connected and unplugging the data cabel from internet modem. Wifi signal would be still there, but not data transmit over internet would be possible however NetInfo should be returing true.
I had the same problem as you. Everytime the App start i wanted to make sure if there is internet or not. And my solution was calling www.google.com through fetch or axios
by first App launch. If it fails, means that there is no internet connection or data transit. You should also give a timeout for slow internet conections. Finally everytime that i had to make a request over internet i tried to handle that error in the catch method of a Promise.
I will try this approach and see if it works out for me. Thanks :D
– Viral Joshi
Nov 25 '18 at 4:06
add a comment |
Thank you all for helping me out here. As Helmer mentioned that it is known bug in react native, I ended up using Axios to check internet connection in handleConnectivityChange
everytime I get isConnected
= false
. And I run axios code in background every 5 seconds while my app is disconnected from the internet and trying to connect to Google and so if I run into any error or response is not 200, I call my handleConnectivityChange
function again after 5 seconds and keep repeating process until I can access Google.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463446%2fwhy-react-native-redux-reducer-is-called-twice-sometimes-and-sometimes-once-only%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is a known problem with the NetInfo Component. It seems to return false after first reconnection. I would change your aproach. You need to keep in mind that NetInfo informs if there is a protocol connection to internet like WiFi or 3G, but not if data transit over internet is possible. You could try this by leaving WIFI connected and unplugging the data cabel from internet modem. Wifi signal would be still there, but not data transmit over internet would be possible however NetInfo should be returing true.
I had the same problem as you. Everytime the App start i wanted to make sure if there is internet or not. And my solution was calling www.google.com through fetch or axios
by first App launch. If it fails, means that there is no internet connection or data transit. You should also give a timeout for slow internet conections. Finally everytime that i had to make a request over internet i tried to handle that error in the catch method of a Promise.
I will try this approach and see if it works out for me. Thanks :D
– Viral Joshi
Nov 25 '18 at 4:06
add a comment |
There is a known problem with the NetInfo Component. It seems to return false after first reconnection. I would change your aproach. You need to keep in mind that NetInfo informs if there is a protocol connection to internet like WiFi or 3G, but not if data transit over internet is possible. You could try this by leaving WIFI connected and unplugging the data cabel from internet modem. Wifi signal would be still there, but not data transmit over internet would be possible however NetInfo should be returing true.
I had the same problem as you. Everytime the App start i wanted to make sure if there is internet or not. And my solution was calling www.google.com through fetch or axios
by first App launch. If it fails, means that there is no internet connection or data transit. You should also give a timeout for slow internet conections. Finally everytime that i had to make a request over internet i tried to handle that error in the catch method of a Promise.
I will try this approach and see if it works out for me. Thanks :D
– Viral Joshi
Nov 25 '18 at 4:06
add a comment |
There is a known problem with the NetInfo Component. It seems to return false after first reconnection. I would change your aproach. You need to keep in mind that NetInfo informs if there is a protocol connection to internet like WiFi or 3G, but not if data transit over internet is possible. You could try this by leaving WIFI connected and unplugging the data cabel from internet modem. Wifi signal would be still there, but not data transmit over internet would be possible however NetInfo should be returing true.
I had the same problem as you. Everytime the App start i wanted to make sure if there is internet or not. And my solution was calling www.google.com through fetch or axios
by first App launch. If it fails, means that there is no internet connection or data transit. You should also give a timeout for slow internet conections. Finally everytime that i had to make a request over internet i tried to handle that error in the catch method of a Promise.
There is a known problem with the NetInfo Component. It seems to return false after first reconnection. I would change your aproach. You need to keep in mind that NetInfo informs if there is a protocol connection to internet like WiFi or 3G, but not if data transit over internet is possible. You could try this by leaving WIFI connected and unplugging the data cabel from internet modem. Wifi signal would be still there, but not data transmit over internet would be possible however NetInfo should be returing true.
I had the same problem as you. Everytime the App start i wanted to make sure if there is internet or not. And my solution was calling www.google.com through fetch or axios
by first App launch. If it fails, means that there is no internet connection or data transit. You should also give a timeout for slow internet conections. Finally everytime that i had to make a request over internet i tried to handle that error in the catch method of a Promise.
edited Nov 25 '18 at 15:35
answered Nov 25 '18 at 2:56
Helmer BarcosHelmer Barcos
624310
624310
I will try this approach and see if it works out for me. Thanks :D
– Viral Joshi
Nov 25 '18 at 4:06
add a comment |
I will try this approach and see if it works out for me. Thanks :D
– Viral Joshi
Nov 25 '18 at 4:06
I will try this approach and see if it works out for me. Thanks :D
– Viral Joshi
Nov 25 '18 at 4:06
I will try this approach and see if it works out for me. Thanks :D
– Viral Joshi
Nov 25 '18 at 4:06
add a comment |
Thank you all for helping me out here. As Helmer mentioned that it is known bug in react native, I ended up using Axios to check internet connection in handleConnectivityChange
everytime I get isConnected
= false
. And I run axios code in background every 5 seconds while my app is disconnected from the internet and trying to connect to Google and so if I run into any error or response is not 200, I call my handleConnectivityChange
function again after 5 seconds and keep repeating process until I can access Google.
add a comment |
Thank you all for helping me out here. As Helmer mentioned that it is known bug in react native, I ended up using Axios to check internet connection in handleConnectivityChange
everytime I get isConnected
= false
. And I run axios code in background every 5 seconds while my app is disconnected from the internet and trying to connect to Google and so if I run into any error or response is not 200, I call my handleConnectivityChange
function again after 5 seconds and keep repeating process until I can access Google.
add a comment |
Thank you all for helping me out here. As Helmer mentioned that it is known bug in react native, I ended up using Axios to check internet connection in handleConnectivityChange
everytime I get isConnected
= false
. And I run axios code in background every 5 seconds while my app is disconnected from the internet and trying to connect to Google and so if I run into any error or response is not 200, I call my handleConnectivityChange
function again after 5 seconds and keep repeating process until I can access Google.
Thank you all for helping me out here. As Helmer mentioned that it is known bug in react native, I ended up using Axios to check internet connection in handleConnectivityChange
everytime I get isConnected
= false
. And I run axios code in background every 5 seconds while my app is disconnected from the internet and trying to connect to Google and so if I run into any error or response is not 200, I call my handleConnectivityChange
function again after 5 seconds and keep repeating process until I can access Google.
answered Dec 8 '18 at 0:52
Viral JoshiViral Joshi
87110
87110
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463446%2fwhy-react-native-redux-reducer-is-called-twice-sometimes-and-sometimes-once-only%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qx8W 44kbzQzW4ss,5qsQ1s,1UDwY,r,s,R,NFgO0s91,U,U,T,y7g TQ7b A
your code looks fine for me. on the other side timestamps in your logs looks suspicious: according to them
isConnected: true
comes just in 9ms afterisConnected: false
in both cases. is it possible you are so fast to switch connection off and on again? can it be some bug in emulator?– skyboyer
Nov 25 '18 at 0:49
@skyboyer, thanks for replying me. I disconnect my iMac from wifi and it shows up 2nd action. I connect my machine and it takes about 3-5 seconds to properly connect to wifi and all and than about after 1-2 seconds, simulator gets connection and meanwhile I am not doing anything but looking at logs.
– Viral Joshi
Nov 25 '18 at 1:15
could you add
console.log(isConnected)
inhandleConnectivityChange
? it is not unclear to me howEvent
object should come there transforms into booleantrue/false
in reducer later– skyboyer
Nov 25 '18 at 1:40
@skyboyer, I will try to follow answer that I got from @Helmer. I will also post a screen shot of
isConnected
for you. :D– Viral Joshi
Nov 25 '18 at 4:07
2
Hi Viral, please copy and paste your console logs instead of posting screenshots! Some users can't see images, and we also might want to copy and paste from your logs in the answer.
– stone
Nov 25 '18 at 8:37