In C, how would you save different lines of a text file to different variables
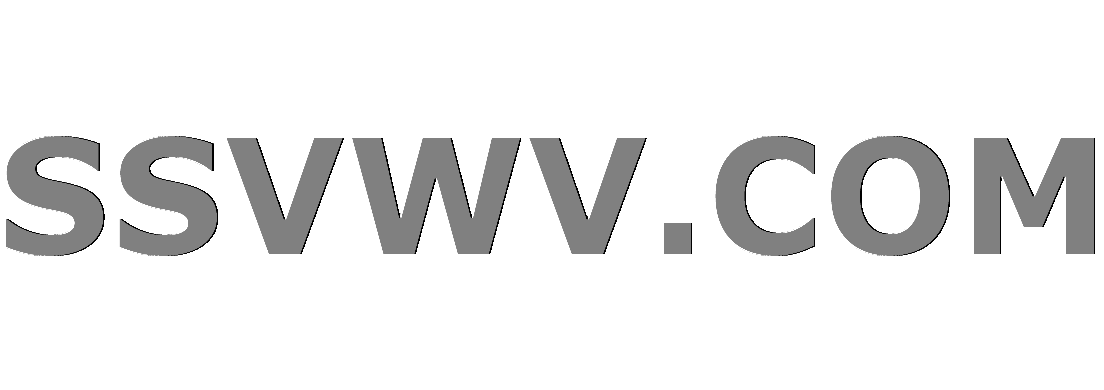
Multi tool use
How would I save different lines from a text File to different variables of different datatypes; all of these variables make up a struct (in my example a flight struct with the following).
struct Flight
{
int flightNum;
char desination[30];
char departDay[15];
};
An Example of the information that I would like to add via text file would be.
111
NYC
Monday
I obviously want to save the words NYC and Monday to a char array, but I want to save 111 to an integer variable
So far I have
while (fscanf(flightInfo, "%s", tempName) != EOF)
{
fscanf(flightInfo, "%dn", &tempNum);
flight.flightNumber = tempNum;
fscanf(flightInfo, "%sn", tempName);
strcpy(flight.desination, tempName);
fscanf(flightInfo, "%sn", tempName)
strcpy(flight.departDay, tempName);
}
Assume that flightInfo is a pointer to a filename, tempNum is an integer, and tempName is a char array
c file file-io
add a comment |
How would I save different lines from a text File to different variables of different datatypes; all of these variables make up a struct (in my example a flight struct with the following).
struct Flight
{
int flightNum;
char desination[30];
char departDay[15];
};
An Example of the information that I would like to add via text file would be.
111
NYC
Monday
I obviously want to save the words NYC and Monday to a char array, but I want to save 111 to an integer variable
So far I have
while (fscanf(flightInfo, "%s", tempName) != EOF)
{
fscanf(flightInfo, "%dn", &tempNum);
flight.flightNumber = tempNum;
fscanf(flightInfo, "%sn", tempName);
strcpy(flight.desination, tempName);
fscanf(flightInfo, "%sn", tempName)
strcpy(flight.departDay, tempName);
}
Assume that flightInfo is a pointer to a filename, tempNum is an integer, and tempName is a char array
c file file-io
2
geeksforgeeks.org/fgets-gets-c-language
– Bwebb
Nov 20 at 23:09
1
@Joshua - fgets() isn't a bad idea; using fscanf() isn't intrinstically evil. I just wanted to stay as close to your original code as possible. Please let me know if you'd like me to update my example; please let us know if you have any further questions.
– paulsm4
Nov 21 at 0:51
add a comment |
How would I save different lines from a text File to different variables of different datatypes; all of these variables make up a struct (in my example a flight struct with the following).
struct Flight
{
int flightNum;
char desination[30];
char departDay[15];
};
An Example of the information that I would like to add via text file would be.
111
NYC
Monday
I obviously want to save the words NYC and Monday to a char array, but I want to save 111 to an integer variable
So far I have
while (fscanf(flightInfo, "%s", tempName) != EOF)
{
fscanf(flightInfo, "%dn", &tempNum);
flight.flightNumber = tempNum;
fscanf(flightInfo, "%sn", tempName);
strcpy(flight.desination, tempName);
fscanf(flightInfo, "%sn", tempName)
strcpy(flight.departDay, tempName);
}
Assume that flightInfo is a pointer to a filename, tempNum is an integer, and tempName is a char array
c file file-io
How would I save different lines from a text File to different variables of different datatypes; all of these variables make up a struct (in my example a flight struct with the following).
struct Flight
{
int flightNum;
char desination[30];
char departDay[15];
};
An Example of the information that I would like to add via text file would be.
111
NYC
Monday
I obviously want to save the words NYC and Monday to a char array, but I want to save 111 to an integer variable
So far I have
while (fscanf(flightInfo, "%s", tempName) != EOF)
{
fscanf(flightInfo, "%dn", &tempNum);
flight.flightNumber = tempNum;
fscanf(flightInfo, "%sn", tempName);
strcpy(flight.desination, tempName);
fscanf(flightInfo, "%sn", tempName)
strcpy(flight.departDay, tempName);
}
Assume that flightInfo is a pointer to a filename, tempNum is an integer, and tempName is a char array
c file file-io
c file file-io
asked Nov 20 at 22:58
Joshua
71
71
2
geeksforgeeks.org/fgets-gets-c-language
– Bwebb
Nov 20 at 23:09
1
@Joshua - fgets() isn't a bad idea; using fscanf() isn't intrinstically evil. I just wanted to stay as close to your original code as possible. Please let me know if you'd like me to update my example; please let us know if you have any further questions.
– paulsm4
Nov 21 at 0:51
add a comment |
2
geeksforgeeks.org/fgets-gets-c-language
– Bwebb
Nov 20 at 23:09
1
@Joshua - fgets() isn't a bad idea; using fscanf() isn't intrinstically evil. I just wanted to stay as close to your original code as possible. Please let me know if you'd like me to update my example; please let us know if you have any further questions.
– paulsm4
Nov 21 at 0:51
2
2
geeksforgeeks.org/fgets-gets-c-language
– Bwebb
Nov 20 at 23:09
geeksforgeeks.org/fgets-gets-c-language
– Bwebb
Nov 20 at 23:09
1
1
@Joshua - fgets() isn't a bad idea; using fscanf() isn't intrinstically evil. I just wanted to stay as close to your original code as possible. Please let me know if you'd like me to update my example; please let us know if you have any further questions.
– paulsm4
Nov 21 at 0:51
@Joshua - fgets() isn't a bad idea; using fscanf() isn't intrinstically evil. I just wanted to stay as close to your original code as possible. Please let me know if you'd like me to update my example; please let us know if you have any further questions.
– paulsm4
Nov 21 at 0:51
add a comment |
2 Answers
2
active
oldest
votes
It sounds like you're on the right track.
What about something like this:
#define MAX_FLIGHTS 100
...
struct Flight flights[MAX_FLIGHTS ];
int n_flights = 0;
...
while (!feof(fp) && (n_flights < MAX_FLIGHTS-1))
{
if (fscanf(fp, "%dn", &flights[n_flights].flightNum) != 1)
error_handler();
if (fscanf(fp, "%29sn", flights[n_flights].destination) != 1)
error_handler();
if (fscanf(fp, "%14sn", flights[n_flights].departDay) != 1)
error_handler();
++n_flights;
}
...
ADDENDUM:
Per Chux's suggestion, I've modified the code to mitigate against potential buffer overruns, by setting scanf max string length to 29 (1 less than char[30] buffer size).
Here is a more detailed explanation:
SonarSource: "scanf()" and "fscanf()" format strings should specify a field width for the "%s" string placeholder
1
Suggestfscanf(fp, "%29sn", flights[n_flights].destination)
instead offscanf(fp, "%sn", flights[n_flights].destination)
– chux
Nov 21 at 4:41
1
@Chux - Thank you - good, constructive suggestion.
– paulsm4
Nov 21 at 5:43
add a comment |
The first question you have to answer is this: how important is it for the file to be readable by people, or on other platforms?
If it isn't that important, then I recommend serializing with fwrite()
and fread()
. That is easier to code for each record, and - as long as your structs are all the same size - allows O(1) access to any record in the file.
If you do want to store these as individual lines, the best way to read a line in from a file is with fgets()
Pseudocode follows:
typedef struct flight {
int flightNum;
char desination[30];
char departDay[15];
} flight;
typedef struct flightSet {
flight *flights;
size_t n; /* number of flights */
size_t nAlloc; /* number of flights you have space for */
} flightSet;
#define FLIGHTSET_INIT_SIZE 16
#define MAX_LINE_LENGTH 128
#define FILENAME "file.txt"
// Create a new flightSet, calling it F
// Allocate FLIGHTSET_INIT_ALLOC number of flight structures for F->flights
// Set F->n to 0
// Set F->nAlloc to FLIGHTSET_INIT_ALLOC
/* Set up other variables */
size_t i = 0; // iterator */
char buffer[MAX_LINE_LENGTH]; // for reading with fgets() */
flights *temp; // for realloc()ing when we have more flights to read
// after reaching nAlloc flights
char *endptr; // for using strtol() to get a number from buffer
FILE *fp; // for reading from the file
// Open FILENAME with fp for reading
//MAIN LOOP
// If i == F->nAlloc, use realloc() to double the allocation of F->flights
// If successful, double F->nAlloc
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file
// Use break to get out of the main loop
}
F->flights[i]->flightNum = (int)strtol(buffer, &endptr, 10);
if (endptr == buffer) {
// The first invalid character that can't be converted to a number is at the very beginning
// of the buffer, so this is not a valid numerical character and your data file is corrupt
// Print out an error message
break;
}
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file when expecting new line; file format error
// Use break to get out of the main loop
} else {
F->flights[i]->destination = strdup(buffer); // If your system has strdup()
// Check for memory allocation
}
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file when expecting new line; file format error
// Use break to get out of the main loop
} else {
F->flights[i]->departDay = strdup(buffer); // If your system has strdup()
// Check for memory allocation
}
// If you've gotten here so far without errors, great!
// Increment F->n to reflect the number of successful records we have in F.
// Increment i, the loop iterator
//Final cleanup. Should include closing the file, and freeing any allocated
//memory that didn't end up in a valid record.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402869%2fin-c-how-would-you-save-different-lines-of-a-text-file-to-different-variables%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
It sounds like you're on the right track.
What about something like this:
#define MAX_FLIGHTS 100
...
struct Flight flights[MAX_FLIGHTS ];
int n_flights = 0;
...
while (!feof(fp) && (n_flights < MAX_FLIGHTS-1))
{
if (fscanf(fp, "%dn", &flights[n_flights].flightNum) != 1)
error_handler();
if (fscanf(fp, "%29sn", flights[n_flights].destination) != 1)
error_handler();
if (fscanf(fp, "%14sn", flights[n_flights].departDay) != 1)
error_handler();
++n_flights;
}
...
ADDENDUM:
Per Chux's suggestion, I've modified the code to mitigate against potential buffer overruns, by setting scanf max string length to 29 (1 less than char[30] buffer size).
Here is a more detailed explanation:
SonarSource: "scanf()" and "fscanf()" format strings should specify a field width for the "%s" string placeholder
1
Suggestfscanf(fp, "%29sn", flights[n_flights].destination)
instead offscanf(fp, "%sn", flights[n_flights].destination)
– chux
Nov 21 at 4:41
1
@Chux - Thank you - good, constructive suggestion.
– paulsm4
Nov 21 at 5:43
add a comment |
It sounds like you're on the right track.
What about something like this:
#define MAX_FLIGHTS 100
...
struct Flight flights[MAX_FLIGHTS ];
int n_flights = 0;
...
while (!feof(fp) && (n_flights < MAX_FLIGHTS-1))
{
if (fscanf(fp, "%dn", &flights[n_flights].flightNum) != 1)
error_handler();
if (fscanf(fp, "%29sn", flights[n_flights].destination) != 1)
error_handler();
if (fscanf(fp, "%14sn", flights[n_flights].departDay) != 1)
error_handler();
++n_flights;
}
...
ADDENDUM:
Per Chux's suggestion, I've modified the code to mitigate against potential buffer overruns, by setting scanf max string length to 29 (1 less than char[30] buffer size).
Here is a more detailed explanation:
SonarSource: "scanf()" and "fscanf()" format strings should specify a field width for the "%s" string placeholder
1
Suggestfscanf(fp, "%29sn", flights[n_flights].destination)
instead offscanf(fp, "%sn", flights[n_flights].destination)
– chux
Nov 21 at 4:41
1
@Chux - Thank you - good, constructive suggestion.
– paulsm4
Nov 21 at 5:43
add a comment |
It sounds like you're on the right track.
What about something like this:
#define MAX_FLIGHTS 100
...
struct Flight flights[MAX_FLIGHTS ];
int n_flights = 0;
...
while (!feof(fp) && (n_flights < MAX_FLIGHTS-1))
{
if (fscanf(fp, "%dn", &flights[n_flights].flightNum) != 1)
error_handler();
if (fscanf(fp, "%29sn", flights[n_flights].destination) != 1)
error_handler();
if (fscanf(fp, "%14sn", flights[n_flights].departDay) != 1)
error_handler();
++n_flights;
}
...
ADDENDUM:
Per Chux's suggestion, I've modified the code to mitigate against potential buffer overruns, by setting scanf max string length to 29 (1 less than char[30] buffer size).
Here is a more detailed explanation:
SonarSource: "scanf()" and "fscanf()" format strings should specify a field width for the "%s" string placeholder
It sounds like you're on the right track.
What about something like this:
#define MAX_FLIGHTS 100
...
struct Flight flights[MAX_FLIGHTS ];
int n_flights = 0;
...
while (!feof(fp) && (n_flights < MAX_FLIGHTS-1))
{
if (fscanf(fp, "%dn", &flights[n_flights].flightNum) != 1)
error_handler();
if (fscanf(fp, "%29sn", flights[n_flights].destination) != 1)
error_handler();
if (fscanf(fp, "%14sn", flights[n_flights].departDay) != 1)
error_handler();
++n_flights;
}
...
ADDENDUM:
Per Chux's suggestion, I've modified the code to mitigate against potential buffer overruns, by setting scanf max string length to 29 (1 less than char[30] buffer size).
Here is a more detailed explanation:
SonarSource: "scanf()" and "fscanf()" format strings should specify a field width for the "%s" string placeholder
edited Nov 21 at 6:28
answered Nov 20 at 23:11
paulsm4
76.8k999126
76.8k999126
1
Suggestfscanf(fp, "%29sn", flights[n_flights].destination)
instead offscanf(fp, "%sn", flights[n_flights].destination)
– chux
Nov 21 at 4:41
1
@Chux - Thank you - good, constructive suggestion.
– paulsm4
Nov 21 at 5:43
add a comment |
1
Suggestfscanf(fp, "%29sn", flights[n_flights].destination)
instead offscanf(fp, "%sn", flights[n_flights].destination)
– chux
Nov 21 at 4:41
1
@Chux - Thank you - good, constructive suggestion.
– paulsm4
Nov 21 at 5:43
1
1
Suggest
fscanf(fp, "%29sn", flights[n_flights].destination)
instead of fscanf(fp, "%sn", flights[n_flights].destination)
– chux
Nov 21 at 4:41
Suggest
fscanf(fp, "%29sn", flights[n_flights].destination)
instead of fscanf(fp, "%sn", flights[n_flights].destination)
– chux
Nov 21 at 4:41
1
1
@Chux - Thank you - good, constructive suggestion.
– paulsm4
Nov 21 at 5:43
@Chux - Thank you - good, constructive suggestion.
– paulsm4
Nov 21 at 5:43
add a comment |
The first question you have to answer is this: how important is it for the file to be readable by people, or on other platforms?
If it isn't that important, then I recommend serializing with fwrite()
and fread()
. That is easier to code for each record, and - as long as your structs are all the same size - allows O(1) access to any record in the file.
If you do want to store these as individual lines, the best way to read a line in from a file is with fgets()
Pseudocode follows:
typedef struct flight {
int flightNum;
char desination[30];
char departDay[15];
} flight;
typedef struct flightSet {
flight *flights;
size_t n; /* number of flights */
size_t nAlloc; /* number of flights you have space for */
} flightSet;
#define FLIGHTSET_INIT_SIZE 16
#define MAX_LINE_LENGTH 128
#define FILENAME "file.txt"
// Create a new flightSet, calling it F
// Allocate FLIGHTSET_INIT_ALLOC number of flight structures for F->flights
// Set F->n to 0
// Set F->nAlloc to FLIGHTSET_INIT_ALLOC
/* Set up other variables */
size_t i = 0; // iterator */
char buffer[MAX_LINE_LENGTH]; // for reading with fgets() */
flights *temp; // for realloc()ing when we have more flights to read
// after reaching nAlloc flights
char *endptr; // for using strtol() to get a number from buffer
FILE *fp; // for reading from the file
// Open FILENAME with fp for reading
//MAIN LOOP
// If i == F->nAlloc, use realloc() to double the allocation of F->flights
// If successful, double F->nAlloc
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file
// Use break to get out of the main loop
}
F->flights[i]->flightNum = (int)strtol(buffer, &endptr, 10);
if (endptr == buffer) {
// The first invalid character that can't be converted to a number is at the very beginning
// of the buffer, so this is not a valid numerical character and your data file is corrupt
// Print out an error message
break;
}
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file when expecting new line; file format error
// Use break to get out of the main loop
} else {
F->flights[i]->destination = strdup(buffer); // If your system has strdup()
// Check for memory allocation
}
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file when expecting new line; file format error
// Use break to get out of the main loop
} else {
F->flights[i]->departDay = strdup(buffer); // If your system has strdup()
// Check for memory allocation
}
// If you've gotten here so far without errors, great!
// Increment F->n to reflect the number of successful records we have in F.
// Increment i, the loop iterator
//Final cleanup. Should include closing the file, and freeing any allocated
//memory that didn't end up in a valid record.
add a comment |
The first question you have to answer is this: how important is it for the file to be readable by people, or on other platforms?
If it isn't that important, then I recommend serializing with fwrite()
and fread()
. That is easier to code for each record, and - as long as your structs are all the same size - allows O(1) access to any record in the file.
If you do want to store these as individual lines, the best way to read a line in from a file is with fgets()
Pseudocode follows:
typedef struct flight {
int flightNum;
char desination[30];
char departDay[15];
} flight;
typedef struct flightSet {
flight *flights;
size_t n; /* number of flights */
size_t nAlloc; /* number of flights you have space for */
} flightSet;
#define FLIGHTSET_INIT_SIZE 16
#define MAX_LINE_LENGTH 128
#define FILENAME "file.txt"
// Create a new flightSet, calling it F
// Allocate FLIGHTSET_INIT_ALLOC number of flight structures for F->flights
// Set F->n to 0
// Set F->nAlloc to FLIGHTSET_INIT_ALLOC
/* Set up other variables */
size_t i = 0; // iterator */
char buffer[MAX_LINE_LENGTH]; // for reading with fgets() */
flights *temp; // for realloc()ing when we have more flights to read
// after reaching nAlloc flights
char *endptr; // for using strtol() to get a number from buffer
FILE *fp; // for reading from the file
// Open FILENAME with fp for reading
//MAIN LOOP
// If i == F->nAlloc, use realloc() to double the allocation of F->flights
// If successful, double F->nAlloc
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file
// Use break to get out of the main loop
}
F->flights[i]->flightNum = (int)strtol(buffer, &endptr, 10);
if (endptr == buffer) {
// The first invalid character that can't be converted to a number is at the very beginning
// of the buffer, so this is not a valid numerical character and your data file is corrupt
// Print out an error message
break;
}
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file when expecting new line; file format error
// Use break to get out of the main loop
} else {
F->flights[i]->destination = strdup(buffer); // If your system has strdup()
// Check for memory allocation
}
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file when expecting new line; file format error
// Use break to get out of the main loop
} else {
F->flights[i]->departDay = strdup(buffer); // If your system has strdup()
// Check for memory allocation
}
// If you've gotten here so far without errors, great!
// Increment F->n to reflect the number of successful records we have in F.
// Increment i, the loop iterator
//Final cleanup. Should include closing the file, and freeing any allocated
//memory that didn't end up in a valid record.
add a comment |
The first question you have to answer is this: how important is it for the file to be readable by people, or on other platforms?
If it isn't that important, then I recommend serializing with fwrite()
and fread()
. That is easier to code for each record, and - as long as your structs are all the same size - allows O(1) access to any record in the file.
If you do want to store these as individual lines, the best way to read a line in from a file is with fgets()
Pseudocode follows:
typedef struct flight {
int flightNum;
char desination[30];
char departDay[15];
} flight;
typedef struct flightSet {
flight *flights;
size_t n; /* number of flights */
size_t nAlloc; /* number of flights you have space for */
} flightSet;
#define FLIGHTSET_INIT_SIZE 16
#define MAX_LINE_LENGTH 128
#define FILENAME "file.txt"
// Create a new flightSet, calling it F
// Allocate FLIGHTSET_INIT_ALLOC number of flight structures for F->flights
// Set F->n to 0
// Set F->nAlloc to FLIGHTSET_INIT_ALLOC
/* Set up other variables */
size_t i = 0; // iterator */
char buffer[MAX_LINE_LENGTH]; // for reading with fgets() */
flights *temp; // for realloc()ing when we have more flights to read
// after reaching nAlloc flights
char *endptr; // for using strtol() to get a number from buffer
FILE *fp; // for reading from the file
// Open FILENAME with fp for reading
//MAIN LOOP
// If i == F->nAlloc, use realloc() to double the allocation of F->flights
// If successful, double F->nAlloc
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file
// Use break to get out of the main loop
}
F->flights[i]->flightNum = (int)strtol(buffer, &endptr, 10);
if (endptr == buffer) {
// The first invalid character that can't be converted to a number is at the very beginning
// of the buffer, so this is not a valid numerical character and your data file is corrupt
// Print out an error message
break;
}
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file when expecting new line; file format error
// Use break to get out of the main loop
} else {
F->flights[i]->destination = strdup(buffer); // If your system has strdup()
// Check for memory allocation
}
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file when expecting new line; file format error
// Use break to get out of the main loop
} else {
F->flights[i]->departDay = strdup(buffer); // If your system has strdup()
// Check for memory allocation
}
// If you've gotten here so far without errors, great!
// Increment F->n to reflect the number of successful records we have in F.
// Increment i, the loop iterator
//Final cleanup. Should include closing the file, and freeing any allocated
//memory that didn't end up in a valid record.
The first question you have to answer is this: how important is it for the file to be readable by people, or on other platforms?
If it isn't that important, then I recommend serializing with fwrite()
and fread()
. That is easier to code for each record, and - as long as your structs are all the same size - allows O(1) access to any record in the file.
If you do want to store these as individual lines, the best way to read a line in from a file is with fgets()
Pseudocode follows:
typedef struct flight {
int flightNum;
char desination[30];
char departDay[15];
} flight;
typedef struct flightSet {
flight *flights;
size_t n; /* number of flights */
size_t nAlloc; /* number of flights you have space for */
} flightSet;
#define FLIGHTSET_INIT_SIZE 16
#define MAX_LINE_LENGTH 128
#define FILENAME "file.txt"
// Create a new flightSet, calling it F
// Allocate FLIGHTSET_INIT_ALLOC number of flight structures for F->flights
// Set F->n to 0
// Set F->nAlloc to FLIGHTSET_INIT_ALLOC
/* Set up other variables */
size_t i = 0; // iterator */
char buffer[MAX_LINE_LENGTH]; // for reading with fgets() */
flights *temp; // for realloc()ing when we have more flights to read
// after reaching nAlloc flights
char *endptr; // for using strtol() to get a number from buffer
FILE *fp; // for reading from the file
// Open FILENAME with fp for reading
//MAIN LOOP
// If i == F->nAlloc, use realloc() to double the allocation of F->flights
// If successful, double F->nAlloc
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file
// Use break to get out of the main loop
}
F->flights[i]->flightNum = (int)strtol(buffer, &endptr, 10);
if (endptr == buffer) {
// The first invalid character that can't be converted to a number is at the very beginning
// of the buffer, so this is not a valid numerical character and your data file is corrupt
// Print out an error message
break;
}
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file when expecting new line; file format error
// Use break to get out of the main loop
} else {
F->flights[i]->destination = strdup(buffer); // If your system has strdup()
// Check for memory allocation
}
if (fgets(buffer, MAX_LINE_LENGTH, fp) == NULL) {
// End of file when expecting new line; file format error
// Use break to get out of the main loop
} else {
F->flights[i]->departDay = strdup(buffer); // If your system has strdup()
// Check for memory allocation
}
// If you've gotten here so far without errors, great!
// Increment F->n to reflect the number of successful records we have in F.
// Increment i, the loop iterator
//Final cleanup. Should include closing the file, and freeing any allocated
//memory that didn't end up in a valid record.
answered Nov 21 at 1:28


torstenvl
683613
683613
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402869%2fin-c-how-would-you-save-different-lines-of-a-text-file-to-different-variables%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mZ7e9gIjjoghzgro1 qU6VP1VM7o d6R,dP43N9tgaD W,0sG64dbFb
2
geeksforgeeks.org/fgets-gets-c-language
– Bwebb
Nov 20 at 23:09
1
@Joshua - fgets() isn't a bad idea; using fscanf() isn't intrinstically evil. I just wanted to stay as close to your original code as possible. Please let me know if you'd like me to update my example; please let us know if you have any further questions.
– paulsm4
Nov 21 at 0:51