Converting multiple activites to fragments [closed]
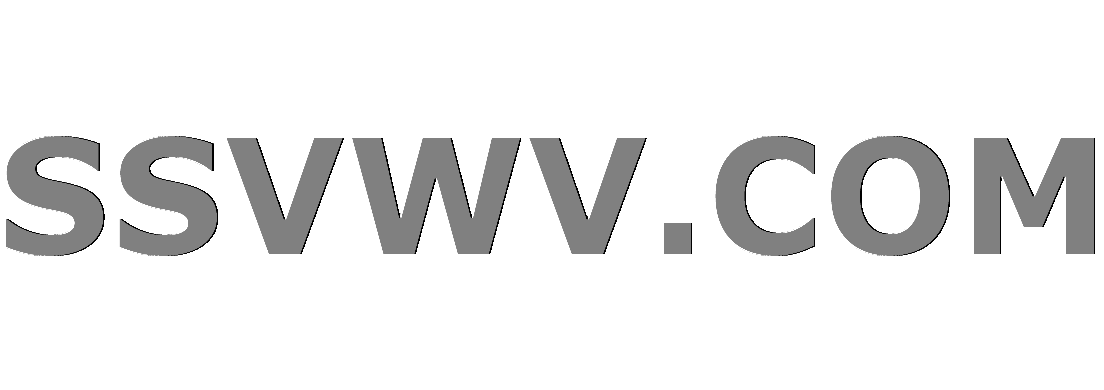
Multi tool use
I just got an idea to implement a BottomNavigationView
to my app.
But the problem is that how/where do i start converting my existing activities, class and adapter to be fragments?
Here is a picture demonstrating what i have now
I have 3 properties in my BottomNavigationView
: Main (list of songs), Radio (empty - not begun on yet) and Collections (my playlist is gonna appear here)
These are my java classes i want to convert to a fragment
These are my layout(XML) files
Any help is appreciated as im really confused on how to accomplish this with my current setup.
This is my CustomAdapter
class:
public class ListViewAdapter extends BaseAdapter {
//Create variables
MediaPlayer mediaPlayer;
int layout;
Song currentSong;
ArrayList<Song> arrayList;
Context context;
public ListViewAdapter(int layout, ArrayList<Song> arrayList, Context
context) {
this.layout = layout;
this.arrayList = arrayList;
this.context = context;
}
private class Viewholder {
TextView artistTxt, songNameTxt;
ImageView playB, stopB;
}
@Override
public int getCount() {
return arrayList.size();
}
@Override
public Object getItem(int position) {
return null;
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View view, ViewGroup parent) {
final Viewholder viewholder;
if (view == null) {
viewholder = new Viewholder();
LayoutInflater layoutInflater = (LayoutInflater)
context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = layoutInflater.inflate(layout, null);
viewholder.artistTxt = (TextView)
view.findViewById(R.id.artistTxt);
viewholder.songNameTxt = (TextView)
view.findViewById(R.id.songNameTxt);
viewholder.playB = (ImageView) view.findViewById(R.id.playB);
viewholder.stopB = (ImageView) view.findViewById(R.id.stopB);
view.setTag(viewholder);
} else {
viewholder = (Viewholder) view.getTag();
}
final Song song = arrayList.get(position);
viewholder.artistTxt.setText(song.getArtist());
viewholder.songNameTxt.setText(song.getSongName());
//get all songs
mediaPlayer = MediaPlayer.create(context, song.getSong());
viewholder.playB.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (currentSong == null) {
mediaPlayer = MediaPlayer.create(context, song.getSong());
}
//if mediaplayer is not null and my current song is not equal
to the new song i clicked on
if (mediaPlayer != null && currentSong != song) {
//resets the mediaplayer and creates a new song from the
position in the list
mediaPlayer.reset();
mediaPlayer = MediaPlayer.create(context, song.getSong());
viewholder.playB.setImageResource(R.drawable.play_black);
mediaPlayer.start();
viewholder.playB.setImageResource(R.drawable.pause_black);
} else {
mediaPlayer.pause();
viewholder.playB.setImageResource(R.drawable.play_black);
}
//check if current song is null or the newly clicked song is
equal to my current song
//if true then assign the newly clicked song as my CURRENT one
//--so it doesnt play the same song for every single one
if (currentSong == null || song != currentSong) {
currentSong = song;
}
}
});
//stop song
viewholder.stopB.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//stops my current song and make it null
if (currentSong != null) {
mediaPlayer.stop();
mediaPlayer.release();
currentSong = null;
viewholder.playB.setImageResource(R.drawable.play_black);
}
}
});
return view;
}
And this is my SongListActivity
where i add my songs and set my adapter:
public class SongListActivity extends AppCompatActivity {
ArrayList<Song> arrayList;
ListView listView;
ListViewAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_song_list);
BottomNavigationView bottomNavigationView = (BottomNavigationView) findViewById(R.id.navigationBar);
bottomNavigationView.setOnNavigationItemSelectedListener(new BottomNavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem menuItem) {
switch (menuItem.getItemId()) {
case R.id.action_main:
startActivity(new Intent(getApplicationContext(), SongListActivity.class));
break;
case R.id.action_collection:
startActivity(new Intent(getApplicationContext(), CollectionActivity.class));
break;
}
return true;
}
});
//Actionbar modified
getSupportActionBar().setTitle("Song list");
//Find my listview
listView = (ListView) findViewById(R.id.listView);
//create a new arraylist object
arrayList = new ArrayList<>();
//Add songs to my list
arrayList.add(new Song("Beyonce", "-Formation", R.raw.beyonce_formation));
arrayList.add(new Song("Chris Brown", "-Hope You Do", R.raw.chrisbrown_hopeyoudo));
arrayList.add(new Song("Akon ft. Colby'O'Donis", "-Beautiful", R.raw.akon_beautiful_ft_colbyodonis_kardinaloffishall));
arrayList.add(new Song("Akon", "-Beautiful", R.raw.akon_dontmatter));
arrayList.add(new Song("Akon", "-Locked Up", R.raw.akon_lockedup_ft_stylesp));
arrayList.add(new Song("Ava Max", "-Sweet but Psycho", R.raw.avamax_sweetbutpsycho));
arrayList.add(new Song("Tupac and Biggie ft. Akon Remix", "-Ghetto", R.raw.tupacbiggieakon_ghetto));
//Create a new adapter of my custom adapter and assign its values
adapter = new ListViewAdapter(R.layout.listview_customlayout, arrayList, this);
//Set my listview to my custom adapter
listView.setAdapter(adapter);
}
}
My CustomListViewLayout
is like this:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/white">
<ImageView
android:id="@+id/musicLogo"
android:layout_width="40sp"
android:layout_height="40sp"
android:src="@drawable/musiclogo" />
<TextView
android:id="@+id/artistTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toRightOf="@id/musicLogo"
android:text="Artist"
android:textColor="@color/black"
android:textSize="15sp" />
<TextView
android:id="@+id/songNameTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/artistTxt"
android:layout_toRightOf="@id/musicLogo"
android:text="Song Name"
android:textColor="#11ca6b" />
<ImageView
android:id="@+id/playB"
android:layout_width="30sp"
android:layout_height="30sp"
android:layout_marginTop="4sp"
android:layout_toLeftOf="@id/stopB"
android:src="@drawable/play_black" />
<ImageView
android:id="@+id/stopB"
android:layout_width="30sp"
android:layout_height="30sp"
android:layout_alignParentRight="true"
android:layout_marginTop="4sp"
android:src="@drawable/stop_black" />
</RelativeLayout>
Thank you.



closed as too broad by Mike M., sideshowbarker, Sotirios Delimanolis, eyllanesc, Machavity Nov 21 at 2:25
Please edit the question to limit it to a specific problem with enough detail to identify an adequate answer. Avoid asking multiple distinct questions at once. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
I just got an idea to implement a BottomNavigationView
to my app.
But the problem is that how/where do i start converting my existing activities, class and adapter to be fragments?
Here is a picture demonstrating what i have now
I have 3 properties in my BottomNavigationView
: Main (list of songs), Radio (empty - not begun on yet) and Collections (my playlist is gonna appear here)
These are my java classes i want to convert to a fragment
These are my layout(XML) files
Any help is appreciated as im really confused on how to accomplish this with my current setup.
This is my CustomAdapter
class:
public class ListViewAdapter extends BaseAdapter {
//Create variables
MediaPlayer mediaPlayer;
int layout;
Song currentSong;
ArrayList<Song> arrayList;
Context context;
public ListViewAdapter(int layout, ArrayList<Song> arrayList, Context
context) {
this.layout = layout;
this.arrayList = arrayList;
this.context = context;
}
private class Viewholder {
TextView artistTxt, songNameTxt;
ImageView playB, stopB;
}
@Override
public int getCount() {
return arrayList.size();
}
@Override
public Object getItem(int position) {
return null;
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View view, ViewGroup parent) {
final Viewholder viewholder;
if (view == null) {
viewholder = new Viewholder();
LayoutInflater layoutInflater = (LayoutInflater)
context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = layoutInflater.inflate(layout, null);
viewholder.artistTxt = (TextView)
view.findViewById(R.id.artistTxt);
viewholder.songNameTxt = (TextView)
view.findViewById(R.id.songNameTxt);
viewholder.playB = (ImageView) view.findViewById(R.id.playB);
viewholder.stopB = (ImageView) view.findViewById(R.id.stopB);
view.setTag(viewholder);
} else {
viewholder = (Viewholder) view.getTag();
}
final Song song = arrayList.get(position);
viewholder.artistTxt.setText(song.getArtist());
viewholder.songNameTxt.setText(song.getSongName());
//get all songs
mediaPlayer = MediaPlayer.create(context, song.getSong());
viewholder.playB.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (currentSong == null) {
mediaPlayer = MediaPlayer.create(context, song.getSong());
}
//if mediaplayer is not null and my current song is not equal
to the new song i clicked on
if (mediaPlayer != null && currentSong != song) {
//resets the mediaplayer and creates a new song from the
position in the list
mediaPlayer.reset();
mediaPlayer = MediaPlayer.create(context, song.getSong());
viewholder.playB.setImageResource(R.drawable.play_black);
mediaPlayer.start();
viewholder.playB.setImageResource(R.drawable.pause_black);
} else {
mediaPlayer.pause();
viewholder.playB.setImageResource(R.drawable.play_black);
}
//check if current song is null or the newly clicked song is
equal to my current song
//if true then assign the newly clicked song as my CURRENT one
//--so it doesnt play the same song for every single one
if (currentSong == null || song != currentSong) {
currentSong = song;
}
}
});
//stop song
viewholder.stopB.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//stops my current song and make it null
if (currentSong != null) {
mediaPlayer.stop();
mediaPlayer.release();
currentSong = null;
viewholder.playB.setImageResource(R.drawable.play_black);
}
}
});
return view;
}
And this is my SongListActivity
where i add my songs and set my adapter:
public class SongListActivity extends AppCompatActivity {
ArrayList<Song> arrayList;
ListView listView;
ListViewAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_song_list);
BottomNavigationView bottomNavigationView = (BottomNavigationView) findViewById(R.id.navigationBar);
bottomNavigationView.setOnNavigationItemSelectedListener(new BottomNavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem menuItem) {
switch (menuItem.getItemId()) {
case R.id.action_main:
startActivity(new Intent(getApplicationContext(), SongListActivity.class));
break;
case R.id.action_collection:
startActivity(new Intent(getApplicationContext(), CollectionActivity.class));
break;
}
return true;
}
});
//Actionbar modified
getSupportActionBar().setTitle("Song list");
//Find my listview
listView = (ListView) findViewById(R.id.listView);
//create a new arraylist object
arrayList = new ArrayList<>();
//Add songs to my list
arrayList.add(new Song("Beyonce", "-Formation", R.raw.beyonce_formation));
arrayList.add(new Song("Chris Brown", "-Hope You Do", R.raw.chrisbrown_hopeyoudo));
arrayList.add(new Song("Akon ft. Colby'O'Donis", "-Beautiful", R.raw.akon_beautiful_ft_colbyodonis_kardinaloffishall));
arrayList.add(new Song("Akon", "-Beautiful", R.raw.akon_dontmatter));
arrayList.add(new Song("Akon", "-Locked Up", R.raw.akon_lockedup_ft_stylesp));
arrayList.add(new Song("Ava Max", "-Sweet but Psycho", R.raw.avamax_sweetbutpsycho));
arrayList.add(new Song("Tupac and Biggie ft. Akon Remix", "-Ghetto", R.raw.tupacbiggieakon_ghetto));
//Create a new adapter of my custom adapter and assign its values
adapter = new ListViewAdapter(R.layout.listview_customlayout, arrayList, this);
//Set my listview to my custom adapter
listView.setAdapter(adapter);
}
}
My CustomListViewLayout
is like this:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/white">
<ImageView
android:id="@+id/musicLogo"
android:layout_width="40sp"
android:layout_height="40sp"
android:src="@drawable/musiclogo" />
<TextView
android:id="@+id/artistTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toRightOf="@id/musicLogo"
android:text="Artist"
android:textColor="@color/black"
android:textSize="15sp" />
<TextView
android:id="@+id/songNameTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/artistTxt"
android:layout_toRightOf="@id/musicLogo"
android:text="Song Name"
android:textColor="#11ca6b" />
<ImageView
android:id="@+id/playB"
android:layout_width="30sp"
android:layout_height="30sp"
android:layout_marginTop="4sp"
android:layout_toLeftOf="@id/stopB"
android:src="@drawable/play_black" />
<ImageView
android:id="@+id/stopB"
android:layout_width="30sp"
android:layout_height="30sp"
android:layout_alignParentRight="true"
android:layout_marginTop="4sp"
android:src="@drawable/stop_black" />
</RelativeLayout>
Thank you.



closed as too broad by Mike M., sideshowbarker, Sotirios Delimanolis, eyllanesc, Machavity Nov 21 at 2:25
Please edit the question to limit it to a specific problem with enough detail to identify an adequate answer. Avoid asking multiple distinct questions at once. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
I just got an idea to implement a BottomNavigationView
to my app.
But the problem is that how/where do i start converting my existing activities, class and adapter to be fragments?
Here is a picture demonstrating what i have now
I have 3 properties in my BottomNavigationView
: Main (list of songs), Radio (empty - not begun on yet) and Collections (my playlist is gonna appear here)
These are my java classes i want to convert to a fragment
These are my layout(XML) files
Any help is appreciated as im really confused on how to accomplish this with my current setup.
This is my CustomAdapter
class:
public class ListViewAdapter extends BaseAdapter {
//Create variables
MediaPlayer mediaPlayer;
int layout;
Song currentSong;
ArrayList<Song> arrayList;
Context context;
public ListViewAdapter(int layout, ArrayList<Song> arrayList, Context
context) {
this.layout = layout;
this.arrayList = arrayList;
this.context = context;
}
private class Viewholder {
TextView artistTxt, songNameTxt;
ImageView playB, stopB;
}
@Override
public int getCount() {
return arrayList.size();
}
@Override
public Object getItem(int position) {
return null;
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View view, ViewGroup parent) {
final Viewholder viewholder;
if (view == null) {
viewholder = new Viewholder();
LayoutInflater layoutInflater = (LayoutInflater)
context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = layoutInflater.inflate(layout, null);
viewholder.artistTxt = (TextView)
view.findViewById(R.id.artistTxt);
viewholder.songNameTxt = (TextView)
view.findViewById(R.id.songNameTxt);
viewholder.playB = (ImageView) view.findViewById(R.id.playB);
viewholder.stopB = (ImageView) view.findViewById(R.id.stopB);
view.setTag(viewholder);
} else {
viewholder = (Viewholder) view.getTag();
}
final Song song = arrayList.get(position);
viewholder.artistTxt.setText(song.getArtist());
viewholder.songNameTxt.setText(song.getSongName());
//get all songs
mediaPlayer = MediaPlayer.create(context, song.getSong());
viewholder.playB.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (currentSong == null) {
mediaPlayer = MediaPlayer.create(context, song.getSong());
}
//if mediaplayer is not null and my current song is not equal
to the new song i clicked on
if (mediaPlayer != null && currentSong != song) {
//resets the mediaplayer and creates a new song from the
position in the list
mediaPlayer.reset();
mediaPlayer = MediaPlayer.create(context, song.getSong());
viewholder.playB.setImageResource(R.drawable.play_black);
mediaPlayer.start();
viewholder.playB.setImageResource(R.drawable.pause_black);
} else {
mediaPlayer.pause();
viewholder.playB.setImageResource(R.drawable.play_black);
}
//check if current song is null or the newly clicked song is
equal to my current song
//if true then assign the newly clicked song as my CURRENT one
//--so it doesnt play the same song for every single one
if (currentSong == null || song != currentSong) {
currentSong = song;
}
}
});
//stop song
viewholder.stopB.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//stops my current song and make it null
if (currentSong != null) {
mediaPlayer.stop();
mediaPlayer.release();
currentSong = null;
viewholder.playB.setImageResource(R.drawable.play_black);
}
}
});
return view;
}
And this is my SongListActivity
where i add my songs and set my adapter:
public class SongListActivity extends AppCompatActivity {
ArrayList<Song> arrayList;
ListView listView;
ListViewAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_song_list);
BottomNavigationView bottomNavigationView = (BottomNavigationView) findViewById(R.id.navigationBar);
bottomNavigationView.setOnNavigationItemSelectedListener(new BottomNavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem menuItem) {
switch (menuItem.getItemId()) {
case R.id.action_main:
startActivity(new Intent(getApplicationContext(), SongListActivity.class));
break;
case R.id.action_collection:
startActivity(new Intent(getApplicationContext(), CollectionActivity.class));
break;
}
return true;
}
});
//Actionbar modified
getSupportActionBar().setTitle("Song list");
//Find my listview
listView = (ListView) findViewById(R.id.listView);
//create a new arraylist object
arrayList = new ArrayList<>();
//Add songs to my list
arrayList.add(new Song("Beyonce", "-Formation", R.raw.beyonce_formation));
arrayList.add(new Song("Chris Brown", "-Hope You Do", R.raw.chrisbrown_hopeyoudo));
arrayList.add(new Song("Akon ft. Colby'O'Donis", "-Beautiful", R.raw.akon_beautiful_ft_colbyodonis_kardinaloffishall));
arrayList.add(new Song("Akon", "-Beautiful", R.raw.akon_dontmatter));
arrayList.add(new Song("Akon", "-Locked Up", R.raw.akon_lockedup_ft_stylesp));
arrayList.add(new Song("Ava Max", "-Sweet but Psycho", R.raw.avamax_sweetbutpsycho));
arrayList.add(new Song("Tupac and Biggie ft. Akon Remix", "-Ghetto", R.raw.tupacbiggieakon_ghetto));
//Create a new adapter of my custom adapter and assign its values
adapter = new ListViewAdapter(R.layout.listview_customlayout, arrayList, this);
//Set my listview to my custom adapter
listView.setAdapter(adapter);
}
}
My CustomListViewLayout
is like this:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/white">
<ImageView
android:id="@+id/musicLogo"
android:layout_width="40sp"
android:layout_height="40sp"
android:src="@drawable/musiclogo" />
<TextView
android:id="@+id/artistTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toRightOf="@id/musicLogo"
android:text="Artist"
android:textColor="@color/black"
android:textSize="15sp" />
<TextView
android:id="@+id/songNameTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/artistTxt"
android:layout_toRightOf="@id/musicLogo"
android:text="Song Name"
android:textColor="#11ca6b" />
<ImageView
android:id="@+id/playB"
android:layout_width="30sp"
android:layout_height="30sp"
android:layout_marginTop="4sp"
android:layout_toLeftOf="@id/stopB"
android:src="@drawable/play_black" />
<ImageView
android:id="@+id/stopB"
android:layout_width="30sp"
android:layout_height="30sp"
android:layout_alignParentRight="true"
android:layout_marginTop="4sp"
android:src="@drawable/stop_black" />
</RelativeLayout>
Thank you.



I just got an idea to implement a BottomNavigationView
to my app.
But the problem is that how/where do i start converting my existing activities, class and adapter to be fragments?
Here is a picture demonstrating what i have now
I have 3 properties in my BottomNavigationView
: Main (list of songs), Radio (empty - not begun on yet) and Collections (my playlist is gonna appear here)
These are my java classes i want to convert to a fragment
These are my layout(XML) files
Any help is appreciated as im really confused on how to accomplish this with my current setup.
This is my CustomAdapter
class:
public class ListViewAdapter extends BaseAdapter {
//Create variables
MediaPlayer mediaPlayer;
int layout;
Song currentSong;
ArrayList<Song> arrayList;
Context context;
public ListViewAdapter(int layout, ArrayList<Song> arrayList, Context
context) {
this.layout = layout;
this.arrayList = arrayList;
this.context = context;
}
private class Viewholder {
TextView artistTxt, songNameTxt;
ImageView playB, stopB;
}
@Override
public int getCount() {
return arrayList.size();
}
@Override
public Object getItem(int position) {
return null;
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View view, ViewGroup parent) {
final Viewholder viewholder;
if (view == null) {
viewholder = new Viewholder();
LayoutInflater layoutInflater = (LayoutInflater)
context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = layoutInflater.inflate(layout, null);
viewholder.artistTxt = (TextView)
view.findViewById(R.id.artistTxt);
viewholder.songNameTxt = (TextView)
view.findViewById(R.id.songNameTxt);
viewholder.playB = (ImageView) view.findViewById(R.id.playB);
viewholder.stopB = (ImageView) view.findViewById(R.id.stopB);
view.setTag(viewholder);
} else {
viewholder = (Viewholder) view.getTag();
}
final Song song = arrayList.get(position);
viewholder.artistTxt.setText(song.getArtist());
viewholder.songNameTxt.setText(song.getSongName());
//get all songs
mediaPlayer = MediaPlayer.create(context, song.getSong());
viewholder.playB.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (currentSong == null) {
mediaPlayer = MediaPlayer.create(context, song.getSong());
}
//if mediaplayer is not null and my current song is not equal
to the new song i clicked on
if (mediaPlayer != null && currentSong != song) {
//resets the mediaplayer and creates a new song from the
position in the list
mediaPlayer.reset();
mediaPlayer = MediaPlayer.create(context, song.getSong());
viewholder.playB.setImageResource(R.drawable.play_black);
mediaPlayer.start();
viewholder.playB.setImageResource(R.drawable.pause_black);
} else {
mediaPlayer.pause();
viewholder.playB.setImageResource(R.drawable.play_black);
}
//check if current song is null or the newly clicked song is
equal to my current song
//if true then assign the newly clicked song as my CURRENT one
//--so it doesnt play the same song for every single one
if (currentSong == null || song != currentSong) {
currentSong = song;
}
}
});
//stop song
viewholder.stopB.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//stops my current song and make it null
if (currentSong != null) {
mediaPlayer.stop();
mediaPlayer.release();
currentSong = null;
viewholder.playB.setImageResource(R.drawable.play_black);
}
}
});
return view;
}
And this is my SongListActivity
where i add my songs and set my adapter:
public class SongListActivity extends AppCompatActivity {
ArrayList<Song> arrayList;
ListView listView;
ListViewAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_song_list);
BottomNavigationView bottomNavigationView = (BottomNavigationView) findViewById(R.id.navigationBar);
bottomNavigationView.setOnNavigationItemSelectedListener(new BottomNavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem menuItem) {
switch (menuItem.getItemId()) {
case R.id.action_main:
startActivity(new Intent(getApplicationContext(), SongListActivity.class));
break;
case R.id.action_collection:
startActivity(new Intent(getApplicationContext(), CollectionActivity.class));
break;
}
return true;
}
});
//Actionbar modified
getSupportActionBar().setTitle("Song list");
//Find my listview
listView = (ListView) findViewById(R.id.listView);
//create a new arraylist object
arrayList = new ArrayList<>();
//Add songs to my list
arrayList.add(new Song("Beyonce", "-Formation", R.raw.beyonce_formation));
arrayList.add(new Song("Chris Brown", "-Hope You Do", R.raw.chrisbrown_hopeyoudo));
arrayList.add(new Song("Akon ft. Colby'O'Donis", "-Beautiful", R.raw.akon_beautiful_ft_colbyodonis_kardinaloffishall));
arrayList.add(new Song("Akon", "-Beautiful", R.raw.akon_dontmatter));
arrayList.add(new Song("Akon", "-Locked Up", R.raw.akon_lockedup_ft_stylesp));
arrayList.add(new Song("Ava Max", "-Sweet but Psycho", R.raw.avamax_sweetbutpsycho));
arrayList.add(new Song("Tupac and Biggie ft. Akon Remix", "-Ghetto", R.raw.tupacbiggieakon_ghetto));
//Create a new adapter of my custom adapter and assign its values
adapter = new ListViewAdapter(R.layout.listview_customlayout, arrayList, this);
//Set my listview to my custom adapter
listView.setAdapter(adapter);
}
}
My CustomListViewLayout
is like this:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/white">
<ImageView
android:id="@+id/musicLogo"
android:layout_width="40sp"
android:layout_height="40sp"
android:src="@drawable/musiclogo" />
<TextView
android:id="@+id/artistTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toRightOf="@id/musicLogo"
android:text="Artist"
android:textColor="@color/black"
android:textSize="15sp" />
<TextView
android:id="@+id/songNameTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/artistTxt"
android:layout_toRightOf="@id/musicLogo"
android:text="Song Name"
android:textColor="#11ca6b" />
<ImageView
android:id="@+id/playB"
android:layout_width="30sp"
android:layout_height="30sp"
android:layout_marginTop="4sp"
android:layout_toLeftOf="@id/stopB"
android:src="@drawable/play_black" />
<ImageView
android:id="@+id/stopB"
android:layout_width="30sp"
android:layout_height="30sp"
android:layout_alignParentRight="true"
android:layout_marginTop="4sp"
android:src="@drawable/stop_black" />
</RelativeLayout>
Thank you.






edited Nov 21 at 0:23
asked Nov 20 at 23:01


Delice
256
256
closed as too broad by Mike M., sideshowbarker, Sotirios Delimanolis, eyllanesc, Machavity Nov 21 at 2:25
Please edit the question to limit it to a specific problem with enough detail to identify an adequate answer. Avoid asking multiple distinct questions at once. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
closed as too broad by Mike M., sideshowbarker, Sotirios Delimanolis, eyllanesc, Machavity Nov 21 at 2:25
Please edit the question to limit it to a specific problem with enough detail to identify an adequate answer. Avoid asking multiple distinct questions at once. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
This is a question that can spawn diverse answers depending on how one looks at it, as in one wants to be pragmatic, or one wants to be architecturally good, etc.
I can suggest a quick (and easy) and not-so-quick (but fairly easy and good) way to tackle your problem.
Quick and easy approach; make a new activity, say, calledNavigationActivity
. Among other things, this can be the activity that:
- Will be your main launcher activity.
- Will implement an UI with a bottom navigation bar and a container for
child fragments. Now, the bottom navigation bar will be the
BottomNavigationView
and the container can be a ViewPager.
After that, convert your existing activities to fragments. It should be fairly straight forward.
And then the main trick is to use these converted fragments as the children of the NavigationActivity that you created above.
In summary, you convert your existing activities to fragments as it is. And then, create a new parent for them, where parent houses them and also provides navigation to them using a Bottom Navigation bar.
Not-so-quick, but suggested approach; this will require you to invest more time into your project but will be beneficial in the long run. It involves investing into a good architecture for your app.
Firstly, if you have not yet, then invest time familiarising yourself with app architectures like MVP, MVVM and more and make a decision on using one for your app. If you do not want to read all, then see Android Arch Components.
I'm sure you will have the right way of transforming your fragments once you are done with the above. You can still refactor the app using approach (1), but you will be doing more. Depending upon how much business logic you have housed in the individual activities, it will make sense to keep the NavigationActivity very lean, and then create ViewModel (or Presenter or something similar) for each new functionality (perhaps for each new fragment).
In summary, essentially, this approach is all about taking a more mature engineering route.
Thank you! The first approache helped me achieve my goal but there is something tricky about my app right now. When i play a song and click on another fragment e.gCollection
then the app still continues to play and when i get back to myMain
(where my song list is) theplay button
is onpause
visibily but it still plays. Any fix? EDIT: Im still using aCustomAdapter
who is not inside any fragment.
– Delice
Nov 21 at 15:07
I believe this should be easily fixable. May I remind you that when you switch fragments, they do not get destroyed. Things work a bit differently than mere finishing and creating the activity. And so you will need to make their UI slightly stateful, such as persist the state of button when fragment was switched and then restore it when the same fragment was switched back in.
– codeFood
Nov 22 at 0:33
So i should use something likeonPause()
?
– Delice
Nov 23 at 13:37
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is a question that can spawn diverse answers depending on how one looks at it, as in one wants to be pragmatic, or one wants to be architecturally good, etc.
I can suggest a quick (and easy) and not-so-quick (but fairly easy and good) way to tackle your problem.
Quick and easy approach; make a new activity, say, calledNavigationActivity
. Among other things, this can be the activity that:
- Will be your main launcher activity.
- Will implement an UI with a bottom navigation bar and a container for
child fragments. Now, the bottom navigation bar will be the
BottomNavigationView
and the container can be a ViewPager.
After that, convert your existing activities to fragments. It should be fairly straight forward.
And then the main trick is to use these converted fragments as the children of the NavigationActivity that you created above.
In summary, you convert your existing activities to fragments as it is. And then, create a new parent for them, where parent houses them and also provides navigation to them using a Bottom Navigation bar.
Not-so-quick, but suggested approach; this will require you to invest more time into your project but will be beneficial in the long run. It involves investing into a good architecture for your app.
Firstly, if you have not yet, then invest time familiarising yourself with app architectures like MVP, MVVM and more and make a decision on using one for your app. If you do not want to read all, then see Android Arch Components.
I'm sure you will have the right way of transforming your fragments once you are done with the above. You can still refactor the app using approach (1), but you will be doing more. Depending upon how much business logic you have housed in the individual activities, it will make sense to keep the NavigationActivity very lean, and then create ViewModel (or Presenter or something similar) for each new functionality (perhaps for each new fragment).
In summary, essentially, this approach is all about taking a more mature engineering route.
Thank you! The first approache helped me achieve my goal but there is something tricky about my app right now. When i play a song and click on another fragment e.gCollection
then the app still continues to play and when i get back to myMain
(where my song list is) theplay button
is onpause
visibily but it still plays. Any fix? EDIT: Im still using aCustomAdapter
who is not inside any fragment.
– Delice
Nov 21 at 15:07
I believe this should be easily fixable. May I remind you that when you switch fragments, they do not get destroyed. Things work a bit differently than mere finishing and creating the activity. And so you will need to make their UI slightly stateful, such as persist the state of button when fragment was switched and then restore it when the same fragment was switched back in.
– codeFood
Nov 22 at 0:33
So i should use something likeonPause()
?
– Delice
Nov 23 at 13:37
add a comment |
This is a question that can spawn diverse answers depending on how one looks at it, as in one wants to be pragmatic, or one wants to be architecturally good, etc.
I can suggest a quick (and easy) and not-so-quick (but fairly easy and good) way to tackle your problem.
Quick and easy approach; make a new activity, say, calledNavigationActivity
. Among other things, this can be the activity that:
- Will be your main launcher activity.
- Will implement an UI with a bottom navigation bar and a container for
child fragments. Now, the bottom navigation bar will be the
BottomNavigationView
and the container can be a ViewPager.
After that, convert your existing activities to fragments. It should be fairly straight forward.
And then the main trick is to use these converted fragments as the children of the NavigationActivity that you created above.
In summary, you convert your existing activities to fragments as it is. And then, create a new parent for them, where parent houses them and also provides navigation to them using a Bottom Navigation bar.
Not-so-quick, but suggested approach; this will require you to invest more time into your project but will be beneficial in the long run. It involves investing into a good architecture for your app.
Firstly, if you have not yet, then invest time familiarising yourself with app architectures like MVP, MVVM and more and make a decision on using one for your app. If you do not want to read all, then see Android Arch Components.
I'm sure you will have the right way of transforming your fragments once you are done with the above. You can still refactor the app using approach (1), but you will be doing more. Depending upon how much business logic you have housed in the individual activities, it will make sense to keep the NavigationActivity very lean, and then create ViewModel (or Presenter or something similar) for each new functionality (perhaps for each new fragment).
In summary, essentially, this approach is all about taking a more mature engineering route.
Thank you! The first approache helped me achieve my goal but there is something tricky about my app right now. When i play a song and click on another fragment e.gCollection
then the app still continues to play and when i get back to myMain
(where my song list is) theplay button
is onpause
visibily but it still plays. Any fix? EDIT: Im still using aCustomAdapter
who is not inside any fragment.
– Delice
Nov 21 at 15:07
I believe this should be easily fixable. May I remind you that when you switch fragments, they do not get destroyed. Things work a bit differently than mere finishing and creating the activity. And so you will need to make their UI slightly stateful, such as persist the state of button when fragment was switched and then restore it when the same fragment was switched back in.
– codeFood
Nov 22 at 0:33
So i should use something likeonPause()
?
– Delice
Nov 23 at 13:37
add a comment |
This is a question that can spawn diverse answers depending on how one looks at it, as in one wants to be pragmatic, or one wants to be architecturally good, etc.
I can suggest a quick (and easy) and not-so-quick (but fairly easy and good) way to tackle your problem.
Quick and easy approach; make a new activity, say, calledNavigationActivity
. Among other things, this can be the activity that:
- Will be your main launcher activity.
- Will implement an UI with a bottom navigation bar and a container for
child fragments. Now, the bottom navigation bar will be the
BottomNavigationView
and the container can be a ViewPager.
After that, convert your existing activities to fragments. It should be fairly straight forward.
And then the main trick is to use these converted fragments as the children of the NavigationActivity that you created above.
In summary, you convert your existing activities to fragments as it is. And then, create a new parent for them, where parent houses them and also provides navigation to them using a Bottom Navigation bar.
Not-so-quick, but suggested approach; this will require you to invest more time into your project but will be beneficial in the long run. It involves investing into a good architecture for your app.
Firstly, if you have not yet, then invest time familiarising yourself with app architectures like MVP, MVVM and more and make a decision on using one for your app. If you do not want to read all, then see Android Arch Components.
I'm sure you will have the right way of transforming your fragments once you are done with the above. You can still refactor the app using approach (1), but you will be doing more. Depending upon how much business logic you have housed in the individual activities, it will make sense to keep the NavigationActivity very lean, and then create ViewModel (or Presenter or something similar) for each new functionality (perhaps for each new fragment).
In summary, essentially, this approach is all about taking a more mature engineering route.
This is a question that can spawn diverse answers depending on how one looks at it, as in one wants to be pragmatic, or one wants to be architecturally good, etc.
I can suggest a quick (and easy) and not-so-quick (but fairly easy and good) way to tackle your problem.
Quick and easy approach; make a new activity, say, calledNavigationActivity
. Among other things, this can be the activity that:
- Will be your main launcher activity.
- Will implement an UI with a bottom navigation bar and a container for
child fragments. Now, the bottom navigation bar will be the
BottomNavigationView
and the container can be a ViewPager.
After that, convert your existing activities to fragments. It should be fairly straight forward.
And then the main trick is to use these converted fragments as the children of the NavigationActivity that you created above.
In summary, you convert your existing activities to fragments as it is. And then, create a new parent for them, where parent houses them and also provides navigation to them using a Bottom Navigation bar.
Not-so-quick, but suggested approach; this will require you to invest more time into your project but will be beneficial in the long run. It involves investing into a good architecture for your app.
Firstly, if you have not yet, then invest time familiarising yourself with app architectures like MVP, MVVM and more and make a decision on using one for your app. If you do not want to read all, then see Android Arch Components.
I'm sure you will have the right way of transforming your fragments once you are done with the above. You can still refactor the app using approach (1), but you will be doing more. Depending upon how much business logic you have housed in the individual activities, it will make sense to keep the NavigationActivity very lean, and then create ViewModel (or Presenter or something similar) for each new functionality (perhaps for each new fragment).
In summary, essentially, this approach is all about taking a more mature engineering route.
answered Nov 21 at 0:43


codeFood
8661111
8661111
Thank you! The first approache helped me achieve my goal but there is something tricky about my app right now. When i play a song and click on another fragment e.gCollection
then the app still continues to play and when i get back to myMain
(where my song list is) theplay button
is onpause
visibily but it still plays. Any fix? EDIT: Im still using aCustomAdapter
who is not inside any fragment.
– Delice
Nov 21 at 15:07
I believe this should be easily fixable. May I remind you that when you switch fragments, they do not get destroyed. Things work a bit differently than mere finishing and creating the activity. And so you will need to make their UI slightly stateful, such as persist the state of button when fragment was switched and then restore it when the same fragment was switched back in.
– codeFood
Nov 22 at 0:33
So i should use something likeonPause()
?
– Delice
Nov 23 at 13:37
add a comment |
Thank you! The first approache helped me achieve my goal but there is something tricky about my app right now. When i play a song and click on another fragment e.gCollection
then the app still continues to play and when i get back to myMain
(where my song list is) theplay button
is onpause
visibily but it still plays. Any fix? EDIT: Im still using aCustomAdapter
who is not inside any fragment.
– Delice
Nov 21 at 15:07
I believe this should be easily fixable. May I remind you that when you switch fragments, they do not get destroyed. Things work a bit differently than mere finishing and creating the activity. And so you will need to make their UI slightly stateful, such as persist the state of button when fragment was switched and then restore it when the same fragment was switched back in.
– codeFood
Nov 22 at 0:33
So i should use something likeonPause()
?
– Delice
Nov 23 at 13:37
Thank you! The first approache helped me achieve my goal but there is something tricky about my app right now. When i play a song and click on another fragment e.g
Collection
then the app still continues to play and when i get back to my Main
(where my song list is) the play button
is on pause
visibily but it still plays. Any fix? EDIT: Im still using a CustomAdapter
who is not inside any fragment.– Delice
Nov 21 at 15:07
Thank you! The first approache helped me achieve my goal but there is something tricky about my app right now. When i play a song and click on another fragment e.g
Collection
then the app still continues to play and when i get back to my Main
(where my song list is) the play button
is on pause
visibily but it still plays. Any fix? EDIT: Im still using a CustomAdapter
who is not inside any fragment.– Delice
Nov 21 at 15:07
I believe this should be easily fixable. May I remind you that when you switch fragments, they do not get destroyed. Things work a bit differently than mere finishing and creating the activity. And so you will need to make their UI slightly stateful, such as persist the state of button when fragment was switched and then restore it when the same fragment was switched back in.
– codeFood
Nov 22 at 0:33
I believe this should be easily fixable. May I remind you that when you switch fragments, they do not get destroyed. Things work a bit differently than mere finishing and creating the activity. And so you will need to make their UI slightly stateful, such as persist the state of button when fragment was switched and then restore it when the same fragment was switched back in.
– codeFood
Nov 22 at 0:33
So i should use something like
onPause()
?– Delice
Nov 23 at 13:37
So i should use something like
onPause()
?– Delice
Nov 23 at 13:37
add a comment |
netP Ns3J2Pe0