Is it considered good practice to shorten elements passed to props in react? [closed]
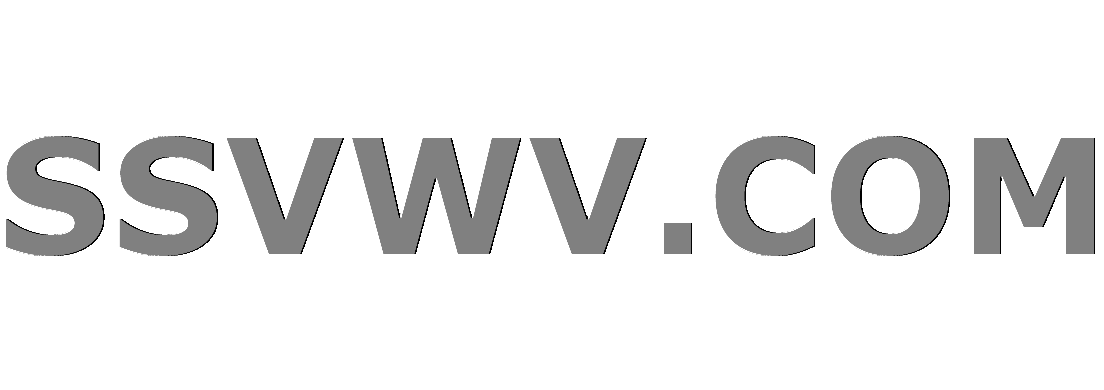
Multi tool use
I have the following Code:
class Parent extends PureComponent {
userInformation = {"id" => 3,
"name" => "Sarah",
"Address" => "Palmenweg",
// Some more random values
}
render() {
// Method 1
return <ComponentWhichOnlyNeedsUserId UserId={this.userInformation.id}>
// Method 2
return <ComponentWhichOnlyNeedsUserId UserInformation={this.userInformation}>
}
}
As you can see, I got a child component which only needs the id of the userInformation, most of the time I split the Object up then(Method 1), however I think this makes my code structure worse, as I got some components in my project which receive 30+ props. I want to restructure my code to always pass in the full object, as this will be cleaner.
Here are my 2 questions:
1. Is there any downside of using method 2 when it comes to performance?
2. Which of the 2 methods would you consider better practice?
Thanks for any answers in advance!
javascript reactjs
closed as primarily opinion-based by charlietfl, Colin, Mayank Shukla, Patrick Hund, JJJ Nov 25 '18 at 16:44
Many good questions generate some degree of opinion based on expert experience, but answers to this question will tend to be almost entirely based on opinions, rather than facts, references, or specific expertise. If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
I have the following Code:
class Parent extends PureComponent {
userInformation = {"id" => 3,
"name" => "Sarah",
"Address" => "Palmenweg",
// Some more random values
}
render() {
// Method 1
return <ComponentWhichOnlyNeedsUserId UserId={this.userInformation.id}>
// Method 2
return <ComponentWhichOnlyNeedsUserId UserInformation={this.userInformation}>
}
}
As you can see, I got a child component which only needs the id of the userInformation, most of the time I split the Object up then(Method 1), however I think this makes my code structure worse, as I got some components in my project which receive 30+ props. I want to restructure my code to always pass in the full object, as this will be cleaner.
Here are my 2 questions:
1. Is there any downside of using method 2 when it comes to performance?
2. Which of the 2 methods would you consider better practice?
Thanks for any answers in advance!
javascript reactjs
closed as primarily opinion-based by charlietfl, Colin, Mayank Shukla, Patrick Hund, JJJ Nov 25 '18 at 16:44
Many good questions generate some degree of opinion based on expert experience, but answers to this question will tend to be almost entirely based on opinions, rather than facts, references, or specific expertise. If this question can be reworded to fit the rules in the help center, please edit the question.
If some components receive 30 plus passed props, then maybe these components need to be split up? If no.. you could use the spread operator to pass in all props at the same time on one time. So<ComponentWhichOnlyNeedsUserId {... userInformation} />
But if the component only needs the ID then I would only pass in the ID as more props being passed in DOES effect performance
– zeduke
Nov 25 '18 at 19:28
add a comment |
I have the following Code:
class Parent extends PureComponent {
userInformation = {"id" => 3,
"name" => "Sarah",
"Address" => "Palmenweg",
// Some more random values
}
render() {
// Method 1
return <ComponentWhichOnlyNeedsUserId UserId={this.userInformation.id}>
// Method 2
return <ComponentWhichOnlyNeedsUserId UserInformation={this.userInformation}>
}
}
As you can see, I got a child component which only needs the id of the userInformation, most of the time I split the Object up then(Method 1), however I think this makes my code structure worse, as I got some components in my project which receive 30+ props. I want to restructure my code to always pass in the full object, as this will be cleaner.
Here are my 2 questions:
1. Is there any downside of using method 2 when it comes to performance?
2. Which of the 2 methods would you consider better practice?
Thanks for any answers in advance!
javascript reactjs
I have the following Code:
class Parent extends PureComponent {
userInformation = {"id" => 3,
"name" => "Sarah",
"Address" => "Palmenweg",
// Some more random values
}
render() {
// Method 1
return <ComponentWhichOnlyNeedsUserId UserId={this.userInformation.id}>
// Method 2
return <ComponentWhichOnlyNeedsUserId UserInformation={this.userInformation}>
}
}
As you can see, I got a child component which only needs the id of the userInformation, most of the time I split the Object up then(Method 1), however I think this makes my code structure worse, as I got some components in my project which receive 30+ props. I want to restructure my code to always pass in the full object, as this will be cleaner.
Here are my 2 questions:
1. Is there any downside of using method 2 when it comes to performance?
2. Which of the 2 methods would you consider better practice?
Thanks for any answers in advance!
javascript reactjs
javascript reactjs
edited Nov 25 '18 at 14:57
rehan
6619
6619
asked Nov 25 '18 at 14:50
Tim von KänelTim von Känel
669
669
closed as primarily opinion-based by charlietfl, Colin, Mayank Shukla, Patrick Hund, JJJ Nov 25 '18 at 16:44
Many good questions generate some degree of opinion based on expert experience, but answers to this question will tend to be almost entirely based on opinions, rather than facts, references, or specific expertise. If this question can be reworded to fit the rules in the help center, please edit the question.
closed as primarily opinion-based by charlietfl, Colin, Mayank Shukla, Patrick Hund, JJJ Nov 25 '18 at 16:44
Many good questions generate some degree of opinion based on expert experience, but answers to this question will tend to be almost entirely based on opinions, rather than facts, references, or specific expertise. If this question can be reworded to fit the rules in the help center, please edit the question.
If some components receive 30 plus passed props, then maybe these components need to be split up? If no.. you could use the spread operator to pass in all props at the same time on one time. So<ComponentWhichOnlyNeedsUserId {... userInformation} />
But if the component only needs the ID then I would only pass in the ID as more props being passed in DOES effect performance
– zeduke
Nov 25 '18 at 19:28
add a comment |
If some components receive 30 plus passed props, then maybe these components need to be split up? If no.. you could use the spread operator to pass in all props at the same time on one time. So<ComponentWhichOnlyNeedsUserId {... userInformation} />
But if the component only needs the ID then I would only pass in the ID as more props being passed in DOES effect performance
– zeduke
Nov 25 '18 at 19:28
If some components receive 30 plus passed props, then maybe these components need to be split up? If no.. you could use the spread operator to pass in all props at the same time on one time. So
<ComponentWhichOnlyNeedsUserId {... userInformation} />
But if the component only needs the ID then I would only pass in the ID as more props being passed in DOES effect performance– zeduke
Nov 25 '18 at 19:28
If some components receive 30 plus passed props, then maybe these components need to be split up? If no.. you could use the spread operator to pass in all props at the same time on one time. So
<ComponentWhichOnlyNeedsUserId {... userInformation} />
But if the component only needs the ID then I would only pass in the ID as more props being passed in DOES effect performance– zeduke
Nov 25 '18 at 19:28
add a comment |
2 Answers
2
active
oldest
votes
In regards to the object being passed to the child component, you are actually passing a reference of the object, so there isn't any real downside to do this from a performance perspective, even if it's a huge object.
i personally believe one is not better than the other, as long as you follow the guidelines of not changing the value of props of reference types (should be immutable)
add a comment |
It's good to pass only props which it requires. Because, it might create a set of values for each single component and an extra useless prop might use up some memory. So, better be a miser and send only what it wants :)
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
In regards to the object being passed to the child component, you are actually passing a reference of the object, so there isn't any real downside to do this from a performance perspective, even if it's a huge object.
i personally believe one is not better than the other, as long as you follow the guidelines of not changing the value of props of reference types (should be immutable)
add a comment |
In regards to the object being passed to the child component, you are actually passing a reference of the object, so there isn't any real downside to do this from a performance perspective, even if it's a huge object.
i personally believe one is not better than the other, as long as you follow the guidelines of not changing the value of props of reference types (should be immutable)
add a comment |
In regards to the object being passed to the child component, you are actually passing a reference of the object, so there isn't any real downside to do this from a performance perspective, even if it's a huge object.
i personally believe one is not better than the other, as long as you follow the guidelines of not changing the value of props of reference types (should be immutable)
In regards to the object being passed to the child component, you are actually passing a reference of the object, so there isn't any real downside to do this from a performance perspective, even if it's a huge object.
i personally believe one is not better than the other, as long as you follow the guidelines of not changing the value of props of reference types (should be immutable)
answered Nov 25 '18 at 15:21
EliranEliran
1657
1657
add a comment |
add a comment |
It's good to pass only props which it requires. Because, it might create a set of values for each single component and an extra useless prop might use up some memory. So, better be a miser and send only what it wants :)
add a comment |
It's good to pass only props which it requires. Because, it might create a set of values for each single component and an extra useless prop might use up some memory. So, better be a miser and send only what it wants :)
add a comment |
It's good to pass only props which it requires. Because, it might create a set of values for each single component and an extra useless prop might use up some memory. So, better be a miser and send only what it wants :)
It's good to pass only props which it requires. Because, it might create a set of values for each single component and an extra useless prop might use up some memory. So, better be a miser and send only what it wants :)
answered Nov 25 '18 at 15:18
klvenkyklvenky
129211
129211
add a comment |
add a comment |
kYJARh F1QDhZhQ KjF URrtLeQi kRjAU3Mvn4QvMqL9WaU4tPwg0fmdgmv CheYSZLBe6TTrvSSqrbY8uQfxh4PicCw LPAlCVcj
If some components receive 30 plus passed props, then maybe these components need to be split up? If no.. you could use the spread operator to pass in all props at the same time on one time. So
<ComponentWhichOnlyNeedsUserId {... userInformation} />
But if the component only needs the ID then I would only pass in the ID as more props being passed in DOES effect performance– zeduke
Nov 25 '18 at 19:28