Flask-socketio: Payload of sent/emit messages not shown in devtools correctly
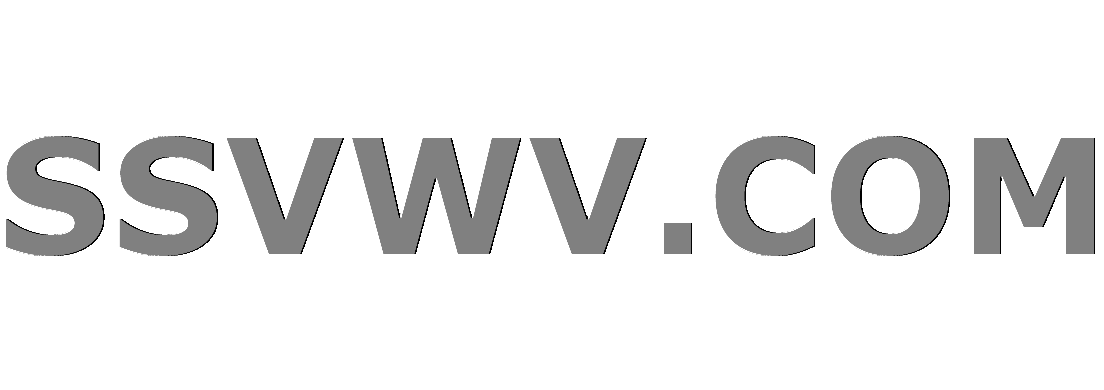
Multi tool use
I just started to play around with websockets in conjuction with Flask. For doing so I decided to use the flask-socketio
module. The code looks like this:
#!/usr/bin/env python3
from flask import Flask, send_from_directory
from flask_socketio import SocketIO, emit, send
app = Flask(__name__, static_url_path='')
socketio = SocketIO(app)
@app.route('/')
def hello_world():
return send_from_directory('html' ,'ws.html')
@socketio.on('connect')
def client_connected():
# log new messages
print('client connected')
send('You are connected. Nice to have you here')
@socketio.on('message')
def handle_message(message):
# echo received messages
print(message)
send(message)
if __name__ == '__main__':
socketio.run(app)
For the client side I copied a rather ugly ws.html
(which resides inside a folder called html
) from the flask-socketio docs and updated it to the latest version of socket.io:
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.slim.js"></script>
<script type="text/javascript" charset="utf-8">
var socket = io.connect('http://' + document.domain + ':' + location.port);
socket.on('connect', function() {
socket.emit('message', {data: 'I'm connected!'});
});
</script>
So the overall dir structure is:
.
├── srv.py
└── html
└── ws.html
I then had a look at the WebSocket traffic via Chrome's developer tools. However, the output does not seem to be correct. Neither the welcome message upon connect nor the message sent from within the ws.html
are shown in the frame's payload:
In order to narrow things a bit down I installed the npm module socketio-debugger
. I am not quite sure about its core functionality/usage, but at least flask's welcome message seems to be sent correctly:
$ socketio-debugger http://localhost:5000
● start Event › connect
Connected to http://localhost:5000
❯ log Event › message
You are connected. Nice to have you here
What is going on here? Why do I get incorrect payload in Chrome's dev tools?
python flask websocket

add a comment |
I just started to play around with websockets in conjuction with Flask. For doing so I decided to use the flask-socketio
module. The code looks like this:
#!/usr/bin/env python3
from flask import Flask, send_from_directory
from flask_socketio import SocketIO, emit, send
app = Flask(__name__, static_url_path='')
socketio = SocketIO(app)
@app.route('/')
def hello_world():
return send_from_directory('html' ,'ws.html')
@socketio.on('connect')
def client_connected():
# log new messages
print('client connected')
send('You are connected. Nice to have you here')
@socketio.on('message')
def handle_message(message):
# echo received messages
print(message)
send(message)
if __name__ == '__main__':
socketio.run(app)
For the client side I copied a rather ugly ws.html
(which resides inside a folder called html
) from the flask-socketio docs and updated it to the latest version of socket.io:
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.slim.js"></script>
<script type="text/javascript" charset="utf-8">
var socket = io.connect('http://' + document.domain + ':' + location.port);
socket.on('connect', function() {
socket.emit('message', {data: 'I'm connected!'});
});
</script>
So the overall dir structure is:
.
├── srv.py
└── html
└── ws.html
I then had a look at the WebSocket traffic via Chrome's developer tools. However, the output does not seem to be correct. Neither the welcome message upon connect nor the message sent from within the ws.html
are shown in the frame's payload:
In order to narrow things a bit down I installed the npm module socketio-debugger
. I am not quite sure about its core functionality/usage, but at least flask's welcome message seems to be sent correctly:
$ socketio-debugger http://localhost:5000
● start Event › connect
Connected to http://localhost:5000
❯ log Event › message
You are connected. Nice to have you here
What is going on here? Why do I get incorrect payload in Chrome's dev tools?
python flask websocket

You have omitted a very important detail. Does theprint(message)
statement execute? Or in other words: is the problem that the exchange doesn't work, or that the exchange does work but you don't understand why you don't see it in the dev tools?
– Miguel
Dec 5 '18 at 10:46
add a comment |
I just started to play around with websockets in conjuction with Flask. For doing so I decided to use the flask-socketio
module. The code looks like this:
#!/usr/bin/env python3
from flask import Flask, send_from_directory
from flask_socketio import SocketIO, emit, send
app = Flask(__name__, static_url_path='')
socketio = SocketIO(app)
@app.route('/')
def hello_world():
return send_from_directory('html' ,'ws.html')
@socketio.on('connect')
def client_connected():
# log new messages
print('client connected')
send('You are connected. Nice to have you here')
@socketio.on('message')
def handle_message(message):
# echo received messages
print(message)
send(message)
if __name__ == '__main__':
socketio.run(app)
For the client side I copied a rather ugly ws.html
(which resides inside a folder called html
) from the flask-socketio docs and updated it to the latest version of socket.io:
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.slim.js"></script>
<script type="text/javascript" charset="utf-8">
var socket = io.connect('http://' + document.domain + ':' + location.port);
socket.on('connect', function() {
socket.emit('message', {data: 'I'm connected!'});
});
</script>
So the overall dir structure is:
.
├── srv.py
└── html
└── ws.html
I then had a look at the WebSocket traffic via Chrome's developer tools. However, the output does not seem to be correct. Neither the welcome message upon connect nor the message sent from within the ws.html
are shown in the frame's payload:
In order to narrow things a bit down I installed the npm module socketio-debugger
. I am not quite sure about its core functionality/usage, but at least flask's welcome message seems to be sent correctly:
$ socketio-debugger http://localhost:5000
● start Event › connect
Connected to http://localhost:5000
❯ log Event › message
You are connected. Nice to have you here
What is going on here? Why do I get incorrect payload in Chrome's dev tools?
python flask websocket

I just started to play around with websockets in conjuction with Flask. For doing so I decided to use the flask-socketio
module. The code looks like this:
#!/usr/bin/env python3
from flask import Flask, send_from_directory
from flask_socketio import SocketIO, emit, send
app = Flask(__name__, static_url_path='')
socketio = SocketIO(app)
@app.route('/')
def hello_world():
return send_from_directory('html' ,'ws.html')
@socketio.on('connect')
def client_connected():
# log new messages
print('client connected')
send('You are connected. Nice to have you here')
@socketio.on('message')
def handle_message(message):
# echo received messages
print(message)
send(message)
if __name__ == '__main__':
socketio.run(app)
For the client side I copied a rather ugly ws.html
(which resides inside a folder called html
) from the flask-socketio docs and updated it to the latest version of socket.io:
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.slim.js"></script>
<script type="text/javascript" charset="utf-8">
var socket = io.connect('http://' + document.domain + ':' + location.port);
socket.on('connect', function() {
socket.emit('message', {data: 'I'm connected!'});
});
</script>
So the overall dir structure is:
.
├── srv.py
└── html
└── ws.html
I then had a look at the WebSocket traffic via Chrome's developer tools. However, the output does not seem to be correct. Neither the welcome message upon connect nor the message sent from within the ws.html
are shown in the frame's payload:
In order to narrow things a bit down I installed the npm module socketio-debugger
. I am not quite sure about its core functionality/usage, but at least flask's welcome message seems to be sent correctly:
$ socketio-debugger http://localhost:5000
● start Event › connect
Connected to http://localhost:5000
❯ log Event › message
You are connected. Nice to have you here
What is going on here? Why do I get incorrect payload in Chrome's dev tools?
python flask websocket

python flask websocket

asked Nov 25 '18 at 14:33


albertalbert
3,65442253
3,65442253
You have omitted a very important detail. Does theprint(message)
statement execute? Or in other words: is the problem that the exchange doesn't work, or that the exchange does work but you don't understand why you don't see it in the dev tools?
– Miguel
Dec 5 '18 at 10:46
add a comment |
You have omitted a very important detail. Does theprint(message)
statement execute? Or in other words: is the problem that the exchange doesn't work, or that the exchange does work but you don't understand why you don't see it in the dev tools?
– Miguel
Dec 5 '18 at 10:46
You have omitted a very important detail. Does the
print(message)
statement execute? Or in other words: is the problem that the exchange doesn't work, or that the exchange does work but you don't understand why you don't see it in the dev tools?– Miguel
Dec 5 '18 at 10:46
You have omitted a very important detail. Does the
print(message)
statement execute? Or in other words: is the problem that the exchange doesn't work, or that the exchange does work but you don't understand why you don't see it in the dev tools?– Miguel
Dec 5 '18 at 10:46
add a comment |
2 Answers
2
active
oldest
votes
Based on how you phrased your question, I'm going to guess that the test application that you built works correctly, and you are only asking why you don't see the exchange of events in the Chrome dev tools.
The reason is that in the default way of starting a Socket.IO connection, clients connect via long-polling first, and once that connection is established they attempt an upgrade to WebSocket. So always the first few things that are exchanged go through long-polling. If you look at the HTTP requests in your dev tools you will see this event that your client is sending in a POST
request.
If you want the connection to be established strictly on WebSocket, you can do so from the client as follows:
var socket = io.connect(
'http://' + document.domain + ':' + location.port,
{transports: ['websocket']}
);
Hope this helps!
add a comment |
You need to handle sent messages on client side as well.
When you receive message
from client side, you can emit some random message again and handle it on client side.
Flask side:
@socketio.on('message')
def handle_message(message):
# echo received messages
print(message)
emit('custom_msg', {'msg': 'hi'})
Client side (your ws.html)
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.slim.js"></script>
<script type="text/javascript" charset="utf-8">
var socket = io.connect('http://' + document.domain + ':' + location.port);
socket.on('connect', function() {
socket.emit('message', {data: 'I'm connected!'});
});
socket.on('custom_msg', function(data){
console.log(data['msg'])
}
</script>
1
Your code shows that the messages are correctly transferred from client to server and vice versa. However, Chrome dev tools Websocket output for the frame contents is unaffected and still the same.
– albert
Nov 25 '18 at 21:15
I don't want to brag, but I've built something with flask socketio. If you implement websockets correctly, you should see them in frames. aukcije-online.herokuapp.com/article/1 ---> This is something I've built, if you open Chrome dev tools and go to frames, and open same article in an other tab, you will see messages. If you want to learn from my project, you can check it here: github.com/PinkFrojd/material_aukcije_online .
– Dinko Pehar
Nov 25 '18 at 23:02
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53468545%2fflask-socketio-payload-of-sent-emit-messages-not-shown-in-devtools-correctly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Based on how you phrased your question, I'm going to guess that the test application that you built works correctly, and you are only asking why you don't see the exchange of events in the Chrome dev tools.
The reason is that in the default way of starting a Socket.IO connection, clients connect via long-polling first, and once that connection is established they attempt an upgrade to WebSocket. So always the first few things that are exchanged go through long-polling. If you look at the HTTP requests in your dev tools you will see this event that your client is sending in a POST
request.
If you want the connection to be established strictly on WebSocket, you can do so from the client as follows:
var socket = io.connect(
'http://' + document.domain + ':' + location.port,
{transports: ['websocket']}
);
Hope this helps!
add a comment |
Based on how you phrased your question, I'm going to guess that the test application that you built works correctly, and you are only asking why you don't see the exchange of events in the Chrome dev tools.
The reason is that in the default way of starting a Socket.IO connection, clients connect via long-polling first, and once that connection is established they attempt an upgrade to WebSocket. So always the first few things that are exchanged go through long-polling. If you look at the HTTP requests in your dev tools you will see this event that your client is sending in a POST
request.
If you want the connection to be established strictly on WebSocket, you can do so from the client as follows:
var socket = io.connect(
'http://' + document.domain + ':' + location.port,
{transports: ['websocket']}
);
Hope this helps!
add a comment |
Based on how you phrased your question, I'm going to guess that the test application that you built works correctly, and you are only asking why you don't see the exchange of events in the Chrome dev tools.
The reason is that in the default way of starting a Socket.IO connection, clients connect via long-polling first, and once that connection is established they attempt an upgrade to WebSocket. So always the first few things that are exchanged go through long-polling. If you look at the HTTP requests in your dev tools you will see this event that your client is sending in a POST
request.
If you want the connection to be established strictly on WebSocket, you can do so from the client as follows:
var socket = io.connect(
'http://' + document.domain + ':' + location.port,
{transports: ['websocket']}
);
Hope this helps!
Based on how you phrased your question, I'm going to guess that the test application that you built works correctly, and you are only asking why you don't see the exchange of events in the Chrome dev tools.
The reason is that in the default way of starting a Socket.IO connection, clients connect via long-polling first, and once that connection is established they attempt an upgrade to WebSocket. So always the first few things that are exchanged go through long-polling. If you look at the HTTP requests in your dev tools you will see this event that your client is sending in a POST
request.
If you want the connection to be established strictly on WebSocket, you can do so from the client as follows:
var socket = io.connect(
'http://' + document.domain + ':' + location.port,
{transports: ['websocket']}
);
Hope this helps!
answered Dec 5 '18 at 11:01
MiguelMiguel
42.7k68399
42.7k68399
add a comment |
add a comment |
You need to handle sent messages on client side as well.
When you receive message
from client side, you can emit some random message again and handle it on client side.
Flask side:
@socketio.on('message')
def handle_message(message):
# echo received messages
print(message)
emit('custom_msg', {'msg': 'hi'})
Client side (your ws.html)
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.slim.js"></script>
<script type="text/javascript" charset="utf-8">
var socket = io.connect('http://' + document.domain + ':' + location.port);
socket.on('connect', function() {
socket.emit('message', {data: 'I'm connected!'});
});
socket.on('custom_msg', function(data){
console.log(data['msg'])
}
</script>
1
Your code shows that the messages are correctly transferred from client to server and vice versa. However, Chrome dev tools Websocket output for the frame contents is unaffected and still the same.
– albert
Nov 25 '18 at 21:15
I don't want to brag, but I've built something with flask socketio. If you implement websockets correctly, you should see them in frames. aukcije-online.herokuapp.com/article/1 ---> This is something I've built, if you open Chrome dev tools and go to frames, and open same article in an other tab, you will see messages. If you want to learn from my project, you can check it here: github.com/PinkFrojd/material_aukcije_online .
– Dinko Pehar
Nov 25 '18 at 23:02
add a comment |
You need to handle sent messages on client side as well.
When you receive message
from client side, you can emit some random message again and handle it on client side.
Flask side:
@socketio.on('message')
def handle_message(message):
# echo received messages
print(message)
emit('custom_msg', {'msg': 'hi'})
Client side (your ws.html)
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.slim.js"></script>
<script type="text/javascript" charset="utf-8">
var socket = io.connect('http://' + document.domain + ':' + location.port);
socket.on('connect', function() {
socket.emit('message', {data: 'I'm connected!'});
});
socket.on('custom_msg', function(data){
console.log(data['msg'])
}
</script>
1
Your code shows that the messages are correctly transferred from client to server and vice versa. However, Chrome dev tools Websocket output for the frame contents is unaffected and still the same.
– albert
Nov 25 '18 at 21:15
I don't want to brag, but I've built something with flask socketio. If you implement websockets correctly, you should see them in frames. aukcije-online.herokuapp.com/article/1 ---> This is something I've built, if you open Chrome dev tools and go to frames, and open same article in an other tab, you will see messages. If you want to learn from my project, you can check it here: github.com/PinkFrojd/material_aukcije_online .
– Dinko Pehar
Nov 25 '18 at 23:02
add a comment |
You need to handle sent messages on client side as well.
When you receive message
from client side, you can emit some random message again and handle it on client side.
Flask side:
@socketio.on('message')
def handle_message(message):
# echo received messages
print(message)
emit('custom_msg', {'msg': 'hi'})
Client side (your ws.html)
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.slim.js"></script>
<script type="text/javascript" charset="utf-8">
var socket = io.connect('http://' + document.domain + ':' + location.port);
socket.on('connect', function() {
socket.emit('message', {data: 'I'm connected!'});
});
socket.on('custom_msg', function(data){
console.log(data['msg'])
}
</script>
You need to handle sent messages on client side as well.
When you receive message
from client side, you can emit some random message again and handle it on client side.
Flask side:
@socketio.on('message')
def handle_message(message):
# echo received messages
print(message)
emit('custom_msg', {'msg': 'hi'})
Client side (your ws.html)
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.slim.js"></script>
<script type="text/javascript" charset="utf-8">
var socket = io.connect('http://' + document.domain + ':' + location.port);
socket.on('connect', function() {
socket.emit('message', {data: 'I'm connected!'});
});
socket.on('custom_msg', function(data){
console.log(data['msg'])
}
</script>
answered Nov 25 '18 at 15:53


Dinko PeharDinko Pehar
1,5313424
1,5313424
1
Your code shows that the messages are correctly transferred from client to server and vice versa. However, Chrome dev tools Websocket output for the frame contents is unaffected and still the same.
– albert
Nov 25 '18 at 21:15
I don't want to brag, but I've built something with flask socketio. If you implement websockets correctly, you should see them in frames. aukcije-online.herokuapp.com/article/1 ---> This is something I've built, if you open Chrome dev tools and go to frames, and open same article in an other tab, you will see messages. If you want to learn from my project, you can check it here: github.com/PinkFrojd/material_aukcije_online .
– Dinko Pehar
Nov 25 '18 at 23:02
add a comment |
1
Your code shows that the messages are correctly transferred from client to server and vice versa. However, Chrome dev tools Websocket output for the frame contents is unaffected and still the same.
– albert
Nov 25 '18 at 21:15
I don't want to brag, but I've built something with flask socketio. If you implement websockets correctly, you should see them in frames. aukcije-online.herokuapp.com/article/1 ---> This is something I've built, if you open Chrome dev tools and go to frames, and open same article in an other tab, you will see messages. If you want to learn from my project, you can check it here: github.com/PinkFrojd/material_aukcije_online .
– Dinko Pehar
Nov 25 '18 at 23:02
1
1
Your code shows that the messages are correctly transferred from client to server and vice versa. However, Chrome dev tools Websocket output for the frame contents is unaffected and still the same.
– albert
Nov 25 '18 at 21:15
Your code shows that the messages are correctly transferred from client to server and vice versa. However, Chrome dev tools Websocket output for the frame contents is unaffected and still the same.
– albert
Nov 25 '18 at 21:15
I don't want to brag, but I've built something with flask socketio. If you implement websockets correctly, you should see them in frames. aukcije-online.herokuapp.com/article/1 ---> This is something I've built, if you open Chrome dev tools and go to frames, and open same article in an other tab, you will see messages. If you want to learn from my project, you can check it here: github.com/PinkFrojd/material_aukcije_online .
– Dinko Pehar
Nov 25 '18 at 23:02
I don't want to brag, but I've built something with flask socketio. If you implement websockets correctly, you should see them in frames. aukcije-online.herokuapp.com/article/1 ---> This is something I've built, if you open Chrome dev tools and go to frames, and open same article in an other tab, you will see messages. If you want to learn from my project, you can check it here: github.com/PinkFrojd/material_aukcije_online .
– Dinko Pehar
Nov 25 '18 at 23:02
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53468545%2fflask-socketio-payload-of-sent-emit-messages-not-shown-in-devtools-correctly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
C,j,A7x17gaCiO4bfhm34vzFEQuVif985 7MCgdWTX3
You have omitted a very important detail. Does the
print(message)
statement execute? Or in other words: is the problem that the exchange doesn't work, or that the exchange does work but you don't understand why you don't see it in the dev tools?– Miguel
Dec 5 '18 at 10:46