Promises & TypeScript
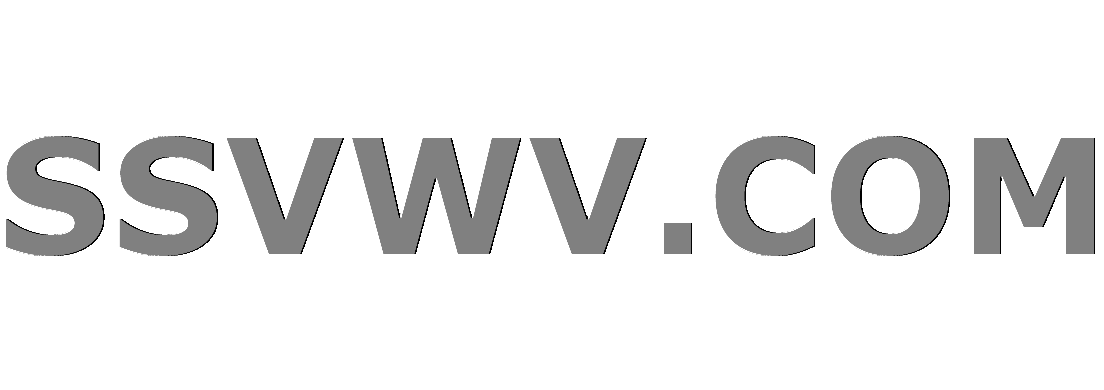
Multi tool use
up vote
1
down vote
favorite
I have a method that I must implement through an abstract class, which has a signature like this:
isAuthenticated(path: string): boolean
In the implementation I'm calling a promise from an authorization server
isAuthenticated(path: string): boolean {
this.authorization.isAuthenticated().then((res) => {
if(res == true) {
return true;
}
return false;
});
}
But the method gives me a error/warning like this:
A function whose type is neither declared type is neither 'void' nor 'any' must return a value
typescript promise angular6
add a comment |
up vote
1
down vote
favorite
I have a method that I must implement through an abstract class, which has a signature like this:
isAuthenticated(path: string): boolean
In the implementation I'm calling a promise from an authorization server
isAuthenticated(path: string): boolean {
this.authorization.isAuthenticated().then((res) => {
if(res == true) {
return true;
}
return false;
});
}
But the method gives me a error/warning like this:
A function whose type is neither declared type is neither 'void' nor 'any' must return a value
typescript promise angular6
your promise return something in your function. you need to return the result with maybereturn this.authorisation....
– charles Lgn
Nov 20 at 14:59
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a method that I must implement through an abstract class, which has a signature like this:
isAuthenticated(path: string): boolean
In the implementation I'm calling a promise from an authorization server
isAuthenticated(path: string): boolean {
this.authorization.isAuthenticated().then((res) => {
if(res == true) {
return true;
}
return false;
});
}
But the method gives me a error/warning like this:
A function whose type is neither declared type is neither 'void' nor 'any' must return a value
typescript promise angular6
I have a method that I must implement through an abstract class, which has a signature like this:
isAuthenticated(path: string): boolean
In the implementation I'm calling a promise from an authorization server
isAuthenticated(path: string): boolean {
this.authorization.isAuthenticated().then((res) => {
if(res == true) {
return true;
}
return false;
});
}
But the method gives me a error/warning like this:
A function whose type is neither declared type is neither 'void' nor 'any' must return a value
typescript promise angular6
typescript promise angular6
asked Nov 20 at 14:57
Tom Kurian
358
358
your promise return something in your function. you need to return the result with maybereturn this.authorisation....
– charles Lgn
Nov 20 at 14:59
add a comment |
your promise return something in your function. you need to return the result with maybereturn this.authorisation....
– charles Lgn
Nov 20 at 14:59
your promise return something in your function. you need to return the result with maybe
return this.authorisation....
– charles Lgn
Nov 20 at 14:59
your promise return something in your function. you need to return the result with maybe
return this.authorisation....
– charles Lgn
Nov 20 at 14:59
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
You are not returning anything from isAuthenticated
. You also cannot just "wait" here for the result that simply.
You can do this:
isAuthenticated(path: string): Promise<boolean> {
// return the ".then" to return a promise of the type returned
// in the .then
return this.authorization.isAuthenticated().then((res) => {
if(res === true) {
return true;
}
return false;
});
}
And allow the caller to "wait" for the boolean result.
Note: Assuming that this.authorization.isAuthenticated
returns a Promise<boolean>
and you don't need to do any other actions in the .then
, the code could be simplified to:
isAuthenticated(path: string): Promise<boolean> {
return this.authorization.isAuthenticated();
}
1
You could also use async/wait typescriptlang.org/play/…
– Titian Cernicova-Dragomir
Nov 20 at 15:05
@JohnnyHK Probably, but I did not want to assume too much or change too much from what they had. But I did realize that the.then
is probably unnecessary.
– crashmstr
Nov 20 at 15:06
@TitianCernicova-Dragomir Yes. Not as familiar yet with that syntax, but the ultimate point is that the code won't stop and wait for the result: you need to have the caller "wait" for it with some syntax.
– crashmstr
Nov 20 at 15:08
Thanks! But the problem is that I'm implementing an abstract method that just returns a boolean not Promise<boolean>.
– Tom Kurian
Nov 20 at 15:21
@TomKurian Sincethis.authorization.isAuthenticated
returns a promise, you can only get the boolean result by.then
,await
in anasync
function, or a callback function. All of which will be completed asynchronously. Thus, there is no way of returning "just a boolean" from this function.
– crashmstr
Nov 20 at 15:24
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53395729%2fpromises-typescript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
You are not returning anything from isAuthenticated
. You also cannot just "wait" here for the result that simply.
You can do this:
isAuthenticated(path: string): Promise<boolean> {
// return the ".then" to return a promise of the type returned
// in the .then
return this.authorization.isAuthenticated().then((res) => {
if(res === true) {
return true;
}
return false;
});
}
And allow the caller to "wait" for the boolean result.
Note: Assuming that this.authorization.isAuthenticated
returns a Promise<boolean>
and you don't need to do any other actions in the .then
, the code could be simplified to:
isAuthenticated(path: string): Promise<boolean> {
return this.authorization.isAuthenticated();
}
1
You could also use async/wait typescriptlang.org/play/…
– Titian Cernicova-Dragomir
Nov 20 at 15:05
@JohnnyHK Probably, but I did not want to assume too much or change too much from what they had. But I did realize that the.then
is probably unnecessary.
– crashmstr
Nov 20 at 15:06
@TitianCernicova-Dragomir Yes. Not as familiar yet with that syntax, but the ultimate point is that the code won't stop and wait for the result: you need to have the caller "wait" for it with some syntax.
– crashmstr
Nov 20 at 15:08
Thanks! But the problem is that I'm implementing an abstract method that just returns a boolean not Promise<boolean>.
– Tom Kurian
Nov 20 at 15:21
@TomKurian Sincethis.authorization.isAuthenticated
returns a promise, you can only get the boolean result by.then
,await
in anasync
function, or a callback function. All of which will be completed asynchronously. Thus, there is no way of returning "just a boolean" from this function.
– crashmstr
Nov 20 at 15:24
add a comment |
up vote
1
down vote
You are not returning anything from isAuthenticated
. You also cannot just "wait" here for the result that simply.
You can do this:
isAuthenticated(path: string): Promise<boolean> {
// return the ".then" to return a promise of the type returned
// in the .then
return this.authorization.isAuthenticated().then((res) => {
if(res === true) {
return true;
}
return false;
});
}
And allow the caller to "wait" for the boolean result.
Note: Assuming that this.authorization.isAuthenticated
returns a Promise<boolean>
and you don't need to do any other actions in the .then
, the code could be simplified to:
isAuthenticated(path: string): Promise<boolean> {
return this.authorization.isAuthenticated();
}
1
You could also use async/wait typescriptlang.org/play/…
– Titian Cernicova-Dragomir
Nov 20 at 15:05
@JohnnyHK Probably, but I did not want to assume too much or change too much from what they had. But I did realize that the.then
is probably unnecessary.
– crashmstr
Nov 20 at 15:06
@TitianCernicova-Dragomir Yes. Not as familiar yet with that syntax, but the ultimate point is that the code won't stop and wait for the result: you need to have the caller "wait" for it with some syntax.
– crashmstr
Nov 20 at 15:08
Thanks! But the problem is that I'm implementing an abstract method that just returns a boolean not Promise<boolean>.
– Tom Kurian
Nov 20 at 15:21
@TomKurian Sincethis.authorization.isAuthenticated
returns a promise, you can only get the boolean result by.then
,await
in anasync
function, or a callback function. All of which will be completed asynchronously. Thus, there is no way of returning "just a boolean" from this function.
– crashmstr
Nov 20 at 15:24
add a comment |
up vote
1
down vote
up vote
1
down vote
You are not returning anything from isAuthenticated
. You also cannot just "wait" here for the result that simply.
You can do this:
isAuthenticated(path: string): Promise<boolean> {
// return the ".then" to return a promise of the type returned
// in the .then
return this.authorization.isAuthenticated().then((res) => {
if(res === true) {
return true;
}
return false;
});
}
And allow the caller to "wait" for the boolean result.
Note: Assuming that this.authorization.isAuthenticated
returns a Promise<boolean>
and you don't need to do any other actions in the .then
, the code could be simplified to:
isAuthenticated(path: string): Promise<boolean> {
return this.authorization.isAuthenticated();
}
You are not returning anything from isAuthenticated
. You also cannot just "wait" here for the result that simply.
You can do this:
isAuthenticated(path: string): Promise<boolean> {
// return the ".then" to return a promise of the type returned
// in the .then
return this.authorization.isAuthenticated().then((res) => {
if(res === true) {
return true;
}
return false;
});
}
And allow the caller to "wait" for the boolean result.
Note: Assuming that this.authorization.isAuthenticated
returns a Promise<boolean>
and you don't need to do any other actions in the .then
, the code could be simplified to:
isAuthenticated(path: string): Promise<boolean> {
return this.authorization.isAuthenticated();
}
edited Nov 20 at 15:10
answered Nov 20 at 15:01
crashmstr
23.5k65174
23.5k65174
1
You could also use async/wait typescriptlang.org/play/…
– Titian Cernicova-Dragomir
Nov 20 at 15:05
@JohnnyHK Probably, but I did not want to assume too much or change too much from what they had. But I did realize that the.then
is probably unnecessary.
– crashmstr
Nov 20 at 15:06
@TitianCernicova-Dragomir Yes. Not as familiar yet with that syntax, but the ultimate point is that the code won't stop and wait for the result: you need to have the caller "wait" for it with some syntax.
– crashmstr
Nov 20 at 15:08
Thanks! But the problem is that I'm implementing an abstract method that just returns a boolean not Promise<boolean>.
– Tom Kurian
Nov 20 at 15:21
@TomKurian Sincethis.authorization.isAuthenticated
returns a promise, you can only get the boolean result by.then
,await
in anasync
function, or a callback function. All of which will be completed asynchronously. Thus, there is no way of returning "just a boolean" from this function.
– crashmstr
Nov 20 at 15:24
add a comment |
1
You could also use async/wait typescriptlang.org/play/…
– Titian Cernicova-Dragomir
Nov 20 at 15:05
@JohnnyHK Probably, but I did not want to assume too much or change too much from what they had. But I did realize that the.then
is probably unnecessary.
– crashmstr
Nov 20 at 15:06
@TitianCernicova-Dragomir Yes. Not as familiar yet with that syntax, but the ultimate point is that the code won't stop and wait for the result: you need to have the caller "wait" for it with some syntax.
– crashmstr
Nov 20 at 15:08
Thanks! But the problem is that I'm implementing an abstract method that just returns a boolean not Promise<boolean>.
– Tom Kurian
Nov 20 at 15:21
@TomKurian Sincethis.authorization.isAuthenticated
returns a promise, you can only get the boolean result by.then
,await
in anasync
function, or a callback function. All of which will be completed asynchronously. Thus, there is no way of returning "just a boolean" from this function.
– crashmstr
Nov 20 at 15:24
1
1
You could also use async/wait typescriptlang.org/play/…
– Titian Cernicova-Dragomir
Nov 20 at 15:05
You could also use async/wait typescriptlang.org/play/…
– Titian Cernicova-Dragomir
Nov 20 at 15:05
@JohnnyHK Probably, but I did not want to assume too much or change too much from what they had. But I did realize that the
.then
is probably unnecessary.– crashmstr
Nov 20 at 15:06
@JohnnyHK Probably, but I did not want to assume too much or change too much from what they had. But I did realize that the
.then
is probably unnecessary.– crashmstr
Nov 20 at 15:06
@TitianCernicova-Dragomir Yes. Not as familiar yet with that syntax, but the ultimate point is that the code won't stop and wait for the result: you need to have the caller "wait" for it with some syntax.
– crashmstr
Nov 20 at 15:08
@TitianCernicova-Dragomir Yes. Not as familiar yet with that syntax, but the ultimate point is that the code won't stop and wait for the result: you need to have the caller "wait" for it with some syntax.
– crashmstr
Nov 20 at 15:08
Thanks! But the problem is that I'm implementing an abstract method that just returns a boolean not Promise<boolean>.
– Tom Kurian
Nov 20 at 15:21
Thanks! But the problem is that I'm implementing an abstract method that just returns a boolean not Promise<boolean>.
– Tom Kurian
Nov 20 at 15:21
@TomKurian Since
this.authorization.isAuthenticated
returns a promise, you can only get the boolean result by .then
, await
in an async
function, or a callback function. All of which will be completed asynchronously. Thus, there is no way of returning "just a boolean" from this function.– crashmstr
Nov 20 at 15:24
@TomKurian Since
this.authorization.isAuthenticated
returns a promise, you can only get the boolean result by .then
, await
in an async
function, or a callback function. All of which will be completed asynchronously. Thus, there is no way of returning "just a boolean" from this function.– crashmstr
Nov 20 at 15:24
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53395729%2fpromises-typescript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PriiwsI,0R,ACu3K,L4QrIW8mObT8
your promise return something in your function. you need to return the result with maybe
return this.authorisation....
– charles Lgn
Nov 20 at 14:59