Print content if first ACF Repeater field is empty
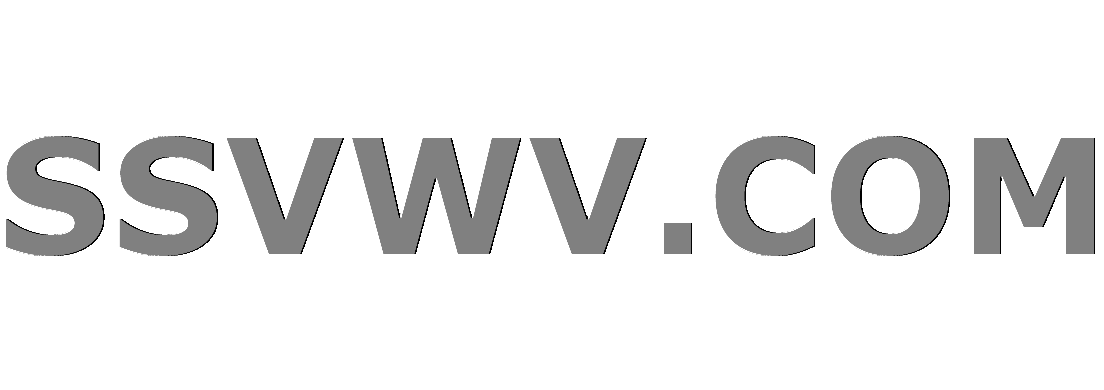
Multi tool use
I’m trying to make a simple document management system with ACF's repeater field. I need to print a button to download a file attached to the the top repeater field (with the size and filetype of the download). But if the top repeater field is empty, it should print “file not available” content.
I’m pretty new to PHP but this mostly works so far:
$repeater = get_field( 'document' )[0];
if( $repeater ) {
$fileurl = $repeater[ 'document' ][ 'url' ];
$filesize = filesize( get_attached_file ($repeater[ 'file' ][ 'id' ]) );
$filesize = size_format($filesize);
$filetype = wp_check_filetype( get_attached_file ($repeater[ 'file' ][ 'id' ]));
$download = '<div><a href="' . $repeater[ 'file' ][ 'url' ] . '">Download</a><div>' . $filesize . ' <span>' . $filetype[ 'ext' ] .'</span></div></div>' ;
echo $download;
}
This prints a button to the attached file in the top repeater, when there is an attached file in the top repeater. Only it prints out a dead link if there is nothing in the top repeater. This won’t do. I need to add an else condition or something so that it prints "file not available" content if there is nothing in the first repeater.
if(empty( $repeater )) {
$unavailable = '<div>Unavailable<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
}
I've tried a lot of different ways to do this, such as above, and I don't know what I'm doing wrong. Can you help?
php repeater advanced-custom-fields
add a comment |
I’m trying to make a simple document management system with ACF's repeater field. I need to print a button to download a file attached to the the top repeater field (with the size and filetype of the download). But if the top repeater field is empty, it should print “file not available” content.
I’m pretty new to PHP but this mostly works so far:
$repeater = get_field( 'document' )[0];
if( $repeater ) {
$fileurl = $repeater[ 'document' ][ 'url' ];
$filesize = filesize( get_attached_file ($repeater[ 'file' ][ 'id' ]) );
$filesize = size_format($filesize);
$filetype = wp_check_filetype( get_attached_file ($repeater[ 'file' ][ 'id' ]));
$download = '<div><a href="' . $repeater[ 'file' ][ 'url' ] . '">Download</a><div>' . $filesize . ' <span>' . $filetype[ 'ext' ] .'</span></div></div>' ;
echo $download;
}
This prints a button to the attached file in the top repeater, when there is an attached file in the top repeater. Only it prints out a dead link if there is nothing in the top repeater. This won’t do. I need to add an else condition or something so that it prints "file not available" content if there is nothing in the first repeater.
if(empty( $repeater )) {
$unavailable = '<div>Unavailable<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
}
I've tried a lot of different ways to do this, such as above, and I don't know what I'm doing wrong. Can you help?
php repeater advanced-custom-fields
add a comment |
I’m trying to make a simple document management system with ACF's repeater field. I need to print a button to download a file attached to the the top repeater field (with the size and filetype of the download). But if the top repeater field is empty, it should print “file not available” content.
I’m pretty new to PHP but this mostly works so far:
$repeater = get_field( 'document' )[0];
if( $repeater ) {
$fileurl = $repeater[ 'document' ][ 'url' ];
$filesize = filesize( get_attached_file ($repeater[ 'file' ][ 'id' ]) );
$filesize = size_format($filesize);
$filetype = wp_check_filetype( get_attached_file ($repeater[ 'file' ][ 'id' ]));
$download = '<div><a href="' . $repeater[ 'file' ][ 'url' ] . '">Download</a><div>' . $filesize . ' <span>' . $filetype[ 'ext' ] .'</span></div></div>' ;
echo $download;
}
This prints a button to the attached file in the top repeater, when there is an attached file in the top repeater. Only it prints out a dead link if there is nothing in the top repeater. This won’t do. I need to add an else condition or something so that it prints "file not available" content if there is nothing in the first repeater.
if(empty( $repeater )) {
$unavailable = '<div>Unavailable<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
}
I've tried a lot of different ways to do this, such as above, and I don't know what I'm doing wrong. Can you help?
php repeater advanced-custom-fields
I’m trying to make a simple document management system with ACF's repeater field. I need to print a button to download a file attached to the the top repeater field (with the size and filetype of the download). But if the top repeater field is empty, it should print “file not available” content.
I’m pretty new to PHP but this mostly works so far:
$repeater = get_field( 'document' )[0];
if( $repeater ) {
$fileurl = $repeater[ 'document' ][ 'url' ];
$filesize = filesize( get_attached_file ($repeater[ 'file' ][ 'id' ]) );
$filesize = size_format($filesize);
$filetype = wp_check_filetype( get_attached_file ($repeater[ 'file' ][ 'id' ]));
$download = '<div><a href="' . $repeater[ 'file' ][ 'url' ] . '">Download</a><div>' . $filesize . ' <span>' . $filetype[ 'ext' ] .'</span></div></div>' ;
echo $download;
}
This prints a button to the attached file in the top repeater, when there is an attached file in the top repeater. Only it prints out a dead link if there is nothing in the top repeater. This won’t do. I need to add an else condition or something so that it prints "file not available" content if there is nothing in the first repeater.
if(empty( $repeater )) {
$unavailable = '<div>Unavailable<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
}
I've tried a lot of different ways to do this, such as above, and I don't know what I'm doing wrong. Can you help?
php repeater advanced-custom-fields
php repeater advanced-custom-fields
asked Nov 23 '18 at 2:45
ahateleybrowneahateleybrowne
11
11
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
You have to check for a value before displaying field like that :
if( get_field('document'){
... // there is an attached file
}
else {
$unavailable = '<div>Unavailable<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
}
I think I declared that value already:$repeater = get_field( 'document' )[0]; if( $repeater ) {
– ahateleybrowne
Nov 25 '18 at 0:20
add a comment |
I think they had your case at the ACF support forum: https://support.advancedcustomfields.com/forums/topic/if-repeater-field-if-empty-else-doesnt-work/
add a comment |
I finally got it! I think I wasn't declaring my variables clearly enough.
$row = get_field( 'document' );
$first_row = $row[0];
$first_row_file = $first_row[ 'file' ];
if( $first_row_file ) :
$download = '<div>Available!<div>This document is ready for download.</div></div>' ;
echo $download;
else :
$unavailable = '<div>Unavailable!<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
endif;
Now I can add more sophisticated content (like a download button) to display when there is a file to download, and a helpful message when there isn't.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440069%2fprint-content-if-first-acf-repeater-field-is-empty%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You have to check for a value before displaying field like that :
if( get_field('document'){
... // there is an attached file
}
else {
$unavailable = '<div>Unavailable<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
}
I think I declared that value already:$repeater = get_field( 'document' )[0]; if( $repeater ) {
– ahateleybrowne
Nov 25 '18 at 0:20
add a comment |
You have to check for a value before displaying field like that :
if( get_field('document'){
... // there is an attached file
}
else {
$unavailable = '<div>Unavailable<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
}
I think I declared that value already:$repeater = get_field( 'document' )[0]; if( $repeater ) {
– ahateleybrowne
Nov 25 '18 at 0:20
add a comment |
You have to check for a value before displaying field like that :
if( get_field('document'){
... // there is an attached file
}
else {
$unavailable = '<div>Unavailable<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
}
You have to check for a value before displaying field like that :
if( get_field('document'){
... // there is an attached file
}
else {
$unavailable = '<div>Unavailable<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
}
answered Nov 23 '18 at 15:47
Nicolas LorandNicolas Lorand
268
268
I think I declared that value already:$repeater = get_field( 'document' )[0]; if( $repeater ) {
– ahateleybrowne
Nov 25 '18 at 0:20
add a comment |
I think I declared that value already:$repeater = get_field( 'document' )[0]; if( $repeater ) {
– ahateleybrowne
Nov 25 '18 at 0:20
I think I declared that value already:
$repeater = get_field( 'document' )[0]; if( $repeater ) {
– ahateleybrowne
Nov 25 '18 at 0:20
I think I declared that value already:
$repeater = get_field( 'document' )[0]; if( $repeater ) {
– ahateleybrowne
Nov 25 '18 at 0:20
add a comment |
I think they had your case at the ACF support forum: https://support.advancedcustomfields.com/forums/topic/if-repeater-field-if-empty-else-doesnt-work/
add a comment |
I think they had your case at the ACF support forum: https://support.advancedcustomfields.com/forums/topic/if-repeater-field-if-empty-else-doesnt-work/
add a comment |
I think they had your case at the ACF support forum: https://support.advancedcustomfields.com/forums/topic/if-repeater-field-if-empty-else-doesnt-work/
I think they had your case at the ACF support forum: https://support.advancedcustomfields.com/forums/topic/if-repeater-field-if-empty-else-doesnt-work/
answered Nov 23 '18 at 16:07
muka.gergelymuka.gergely
604412
604412
add a comment |
add a comment |
I finally got it! I think I wasn't declaring my variables clearly enough.
$row = get_field( 'document' );
$first_row = $row[0];
$first_row_file = $first_row[ 'file' ];
if( $first_row_file ) :
$download = '<div>Available!<div>This document is ready for download.</div></div>' ;
echo $download;
else :
$unavailable = '<div>Unavailable!<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
endif;
Now I can add more sophisticated content (like a download button) to display when there is a file to download, and a helpful message when there isn't.
add a comment |
I finally got it! I think I wasn't declaring my variables clearly enough.
$row = get_field( 'document' );
$first_row = $row[0];
$first_row_file = $first_row[ 'file' ];
if( $first_row_file ) :
$download = '<div>Available!<div>This document is ready for download.</div></div>' ;
echo $download;
else :
$unavailable = '<div>Unavailable!<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
endif;
Now I can add more sophisticated content (like a download button) to display when there is a file to download, and a helpful message when there isn't.
add a comment |
I finally got it! I think I wasn't declaring my variables clearly enough.
$row = get_field( 'document' );
$first_row = $row[0];
$first_row_file = $first_row[ 'file' ];
if( $first_row_file ) :
$download = '<div>Available!<div>This document is ready for download.</div></div>' ;
echo $download;
else :
$unavailable = '<div>Unavailable!<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
endif;
Now I can add more sophisticated content (like a download button) to display when there is a file to download, and a helpful message when there isn't.
I finally got it! I think I wasn't declaring my variables clearly enough.
$row = get_field( 'document' );
$first_row = $row[0];
$first_row_file = $first_row[ 'file' ];
if( $first_row_file ) :
$download = '<div>Available!<div>This document is ready for download.</div></div>' ;
echo $download;
else :
$unavailable = '<div>Unavailable!<div>This document isn't ready yet. Please check back later.</div></div>' ;
echo $unavailable;
endif;
Now I can add more sophisticated content (like a download button) to display when there is a file to download, and a helpful message when there isn't.
answered Nov 25 '18 at 23:30
ahateleybrowneahateleybrowne
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440069%2fprint-content-if-first-acf-repeater-field-is-empty%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4I6vOR a2nO5vsZGT aQEjPPCL