unable to unpack information between custom Preamble in Python and telnetlib
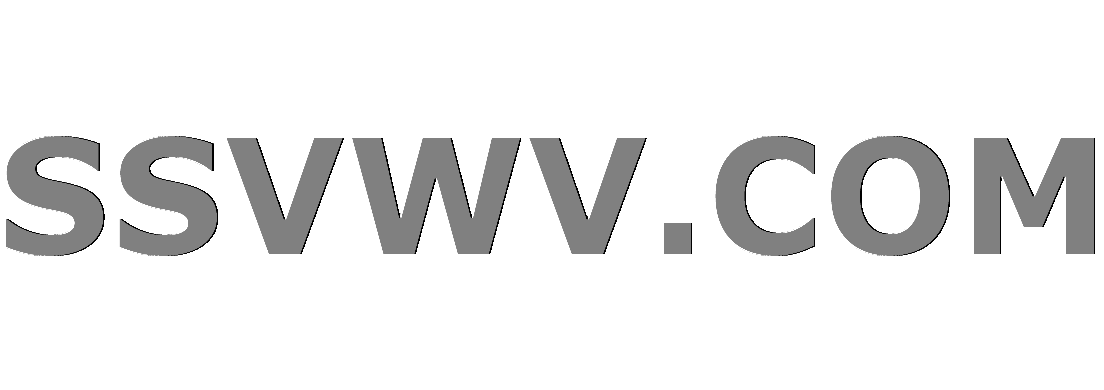
Multi tool use
I have an industrial sensor which provides me information via telnet
over port 10001
.
It has a Data Format as follows:
Also the manual:
All the measuring values are transmitted int32 or uint32 or float depending on the sensors
Code
import telnetlib
import struct
import time
# IP Address, Port, timeout for Telnet
tn = telnetlib.Telnet("169.254.168.150", 10001, 10)
while True:
op = tn.read_eager() # currently read information limit this till preamble
print(op[::-1]) # make little-endian
if not len(op[::-1]) == 0: # initially an empty bit starts (b'')
data = struct.unpack('!4c', op[::-1]) # unpacking `MEAS`
time.sleep(0.1)
my initial attempt:
Connect to the sensor
read data
make it to little-endian
OUTPUT
b''
b'MEASx85x8cx8cx07xa7x9dx01x0cx15x04xf6MEAS'
b'x04xf6MEASx86x8cx8cx07xa7x9ex01x0cx15x04xf6'
b'x15x04xf6MEASx85x8cx8cx07xa7x9fx01x0cx15'
b'x15x04xf6MEASx87x8cx8cx07xa7xa0x01x0c'
b'xa7xa2x01x0cx15x04xf6MEASx87x8cx8cx07xa7xa1x01x0c'
b'x8cx07xa7xa3x01x0cx15x04xf6MEASx87x8cx8cx07'
b'x88x8cx8cx07xa7xa4x01x0cx15x04xf6MEASx88x8c'
b'MEASx8bx8cx8cx07xa7xa5x01x0cx15x04xf6MEAS'
b'x04xf6MEASx8bx8cx8cx07xa7xa6x01x0cx15x04xf6'
b'x15x04xf6MEASx8ax8cx8cx07xa7xa7x01x0cx15'
b'x15x04xf6MEASx88x8cx8cx07xa7xa8x01x0c'
b'x01x0cx15x04xf6MEASx88x8cx8cx07xa7xa9x01x0c'
b'x8cx07xa7xabx01x0cx15x04xf6MEASx8bx8cx8cx07xa7xaa'
b'x8cx8cx07xa7xacx01x0cx15x04xf6MEASx8cx8c'
b'ASx89x8cx8cx07xa7xadx01x0cx15x04xf6MEASx8a'
b'MEASx88x8cx8cx07xa7xaex01x0cx15x04xf6ME'
b'x15x04xf6MEASx87x8cx8cx07xa7xafx01x0cx15x04xf6'
b'x15x04xf6MEASx8ax8cx8cx07xa7xb0x01x0c'
b'x0cx15x04xf6MEASx8ax8cx8cx07xa7xb1x01x0c'
b'x07xa7xb3x01x0cx15x04xf6MEASx89x8cx8cx07xa7xb2x01'
b'x8cx8cx07xa7xb4x01x0cx15x04xf6MEASx89x8cx8c'
b'x85x8cx8cx07xa7xb5x01x0cx15x04xf6MEASx84'
b'MEASx87x8cx8cx07xa7xb6x01x0cx15x04xf6MEAS'
b'x04xf6MEASx8bx8cx8cx07xa7xb7x01x0cx15x04xf6'
b'x15x04xf6MEASx8bx8cx8cx07xa7xb8x01x0cx15'
b'x15x04xf6MEASx8ax8cx8cx07xa7xb9x01x0c'
b'xa7xbbx01x0cx15x04xf6MEASx87x8cx8cx07xa7xbax01x0c'
- try to unpack the preamble !?
How do I read information like Article number
, Serial number
, Channel
, Status
, Measuring Value
between the preamble?
The payload size seems to be fixed here for 22 Bytes (via Wireshark)
python python-3.x tcp telnetlib
add a comment |
I have an industrial sensor which provides me information via telnet
over port 10001
.
It has a Data Format as follows:
Also the manual:
All the measuring values are transmitted int32 or uint32 or float depending on the sensors
Code
import telnetlib
import struct
import time
# IP Address, Port, timeout for Telnet
tn = telnetlib.Telnet("169.254.168.150", 10001, 10)
while True:
op = tn.read_eager() # currently read information limit this till preamble
print(op[::-1]) # make little-endian
if not len(op[::-1]) == 0: # initially an empty bit starts (b'')
data = struct.unpack('!4c', op[::-1]) # unpacking `MEAS`
time.sleep(0.1)
my initial attempt:
Connect to the sensor
read data
make it to little-endian
OUTPUT
b''
b'MEASx85x8cx8cx07xa7x9dx01x0cx15x04xf6MEAS'
b'x04xf6MEASx86x8cx8cx07xa7x9ex01x0cx15x04xf6'
b'x15x04xf6MEASx85x8cx8cx07xa7x9fx01x0cx15'
b'x15x04xf6MEASx87x8cx8cx07xa7xa0x01x0c'
b'xa7xa2x01x0cx15x04xf6MEASx87x8cx8cx07xa7xa1x01x0c'
b'x8cx07xa7xa3x01x0cx15x04xf6MEASx87x8cx8cx07'
b'x88x8cx8cx07xa7xa4x01x0cx15x04xf6MEASx88x8c'
b'MEASx8bx8cx8cx07xa7xa5x01x0cx15x04xf6MEAS'
b'x04xf6MEASx8bx8cx8cx07xa7xa6x01x0cx15x04xf6'
b'x15x04xf6MEASx8ax8cx8cx07xa7xa7x01x0cx15'
b'x15x04xf6MEASx88x8cx8cx07xa7xa8x01x0c'
b'x01x0cx15x04xf6MEASx88x8cx8cx07xa7xa9x01x0c'
b'x8cx07xa7xabx01x0cx15x04xf6MEASx8bx8cx8cx07xa7xaa'
b'x8cx8cx07xa7xacx01x0cx15x04xf6MEASx8cx8c'
b'ASx89x8cx8cx07xa7xadx01x0cx15x04xf6MEASx8a'
b'MEASx88x8cx8cx07xa7xaex01x0cx15x04xf6ME'
b'x15x04xf6MEASx87x8cx8cx07xa7xafx01x0cx15x04xf6'
b'x15x04xf6MEASx8ax8cx8cx07xa7xb0x01x0c'
b'x0cx15x04xf6MEASx8ax8cx8cx07xa7xb1x01x0c'
b'x07xa7xb3x01x0cx15x04xf6MEASx89x8cx8cx07xa7xb2x01'
b'x8cx8cx07xa7xb4x01x0cx15x04xf6MEASx89x8cx8c'
b'x85x8cx8cx07xa7xb5x01x0cx15x04xf6MEASx84'
b'MEASx87x8cx8cx07xa7xb6x01x0cx15x04xf6MEAS'
b'x04xf6MEASx8bx8cx8cx07xa7xb7x01x0cx15x04xf6'
b'x15x04xf6MEASx8bx8cx8cx07xa7xb8x01x0cx15'
b'x15x04xf6MEASx8ax8cx8cx07xa7xb9x01x0c'
b'xa7xbbx01x0cx15x04xf6MEASx87x8cx8cx07xa7xbax01x0c'
- try to unpack the preamble !?
How do I read information like Article number
, Serial number
, Channel
, Status
, Measuring Value
between the preamble?
The payload size seems to be fixed here for 22 Bytes (via Wireshark)
python python-3.x tcp telnetlib
add a comment |
I have an industrial sensor which provides me information via telnet
over port 10001
.
It has a Data Format as follows:
Also the manual:
All the measuring values are transmitted int32 or uint32 or float depending on the sensors
Code
import telnetlib
import struct
import time
# IP Address, Port, timeout for Telnet
tn = telnetlib.Telnet("169.254.168.150", 10001, 10)
while True:
op = tn.read_eager() # currently read information limit this till preamble
print(op[::-1]) # make little-endian
if not len(op[::-1]) == 0: # initially an empty bit starts (b'')
data = struct.unpack('!4c', op[::-1]) # unpacking `MEAS`
time.sleep(0.1)
my initial attempt:
Connect to the sensor
read data
make it to little-endian
OUTPUT
b''
b'MEASx85x8cx8cx07xa7x9dx01x0cx15x04xf6MEAS'
b'x04xf6MEASx86x8cx8cx07xa7x9ex01x0cx15x04xf6'
b'x15x04xf6MEASx85x8cx8cx07xa7x9fx01x0cx15'
b'x15x04xf6MEASx87x8cx8cx07xa7xa0x01x0c'
b'xa7xa2x01x0cx15x04xf6MEASx87x8cx8cx07xa7xa1x01x0c'
b'x8cx07xa7xa3x01x0cx15x04xf6MEASx87x8cx8cx07'
b'x88x8cx8cx07xa7xa4x01x0cx15x04xf6MEASx88x8c'
b'MEASx8bx8cx8cx07xa7xa5x01x0cx15x04xf6MEAS'
b'x04xf6MEASx8bx8cx8cx07xa7xa6x01x0cx15x04xf6'
b'x15x04xf6MEASx8ax8cx8cx07xa7xa7x01x0cx15'
b'x15x04xf6MEASx88x8cx8cx07xa7xa8x01x0c'
b'x01x0cx15x04xf6MEASx88x8cx8cx07xa7xa9x01x0c'
b'x8cx07xa7xabx01x0cx15x04xf6MEASx8bx8cx8cx07xa7xaa'
b'x8cx8cx07xa7xacx01x0cx15x04xf6MEASx8cx8c'
b'ASx89x8cx8cx07xa7xadx01x0cx15x04xf6MEASx8a'
b'MEASx88x8cx8cx07xa7xaex01x0cx15x04xf6ME'
b'x15x04xf6MEASx87x8cx8cx07xa7xafx01x0cx15x04xf6'
b'x15x04xf6MEASx8ax8cx8cx07xa7xb0x01x0c'
b'x0cx15x04xf6MEASx8ax8cx8cx07xa7xb1x01x0c'
b'x07xa7xb3x01x0cx15x04xf6MEASx89x8cx8cx07xa7xb2x01'
b'x8cx8cx07xa7xb4x01x0cx15x04xf6MEASx89x8cx8c'
b'x85x8cx8cx07xa7xb5x01x0cx15x04xf6MEASx84'
b'MEASx87x8cx8cx07xa7xb6x01x0cx15x04xf6MEAS'
b'x04xf6MEASx8bx8cx8cx07xa7xb7x01x0cx15x04xf6'
b'x15x04xf6MEASx8bx8cx8cx07xa7xb8x01x0cx15'
b'x15x04xf6MEASx8ax8cx8cx07xa7xb9x01x0c'
b'xa7xbbx01x0cx15x04xf6MEASx87x8cx8cx07xa7xbax01x0c'
- try to unpack the preamble !?
How do I read information like Article number
, Serial number
, Channel
, Status
, Measuring Value
between the preamble?
The payload size seems to be fixed here for 22 Bytes (via Wireshark)
python python-3.x tcp telnetlib
I have an industrial sensor which provides me information via telnet
over port 10001
.
It has a Data Format as follows:
Also the manual:
All the measuring values are transmitted int32 or uint32 or float depending on the sensors
Code
import telnetlib
import struct
import time
# IP Address, Port, timeout for Telnet
tn = telnetlib.Telnet("169.254.168.150", 10001, 10)
while True:
op = tn.read_eager() # currently read information limit this till preamble
print(op[::-1]) # make little-endian
if not len(op[::-1]) == 0: # initially an empty bit starts (b'')
data = struct.unpack('!4c', op[::-1]) # unpacking `MEAS`
time.sleep(0.1)
my initial attempt:
Connect to the sensor
read data
make it to little-endian
OUTPUT
b''
b'MEASx85x8cx8cx07xa7x9dx01x0cx15x04xf6MEAS'
b'x04xf6MEASx86x8cx8cx07xa7x9ex01x0cx15x04xf6'
b'x15x04xf6MEASx85x8cx8cx07xa7x9fx01x0cx15'
b'x15x04xf6MEASx87x8cx8cx07xa7xa0x01x0c'
b'xa7xa2x01x0cx15x04xf6MEASx87x8cx8cx07xa7xa1x01x0c'
b'x8cx07xa7xa3x01x0cx15x04xf6MEASx87x8cx8cx07'
b'x88x8cx8cx07xa7xa4x01x0cx15x04xf6MEASx88x8c'
b'MEASx8bx8cx8cx07xa7xa5x01x0cx15x04xf6MEAS'
b'x04xf6MEASx8bx8cx8cx07xa7xa6x01x0cx15x04xf6'
b'x15x04xf6MEASx8ax8cx8cx07xa7xa7x01x0cx15'
b'x15x04xf6MEASx88x8cx8cx07xa7xa8x01x0c'
b'x01x0cx15x04xf6MEASx88x8cx8cx07xa7xa9x01x0c'
b'x8cx07xa7xabx01x0cx15x04xf6MEASx8bx8cx8cx07xa7xaa'
b'x8cx8cx07xa7xacx01x0cx15x04xf6MEASx8cx8c'
b'ASx89x8cx8cx07xa7xadx01x0cx15x04xf6MEASx8a'
b'MEASx88x8cx8cx07xa7xaex01x0cx15x04xf6ME'
b'x15x04xf6MEASx87x8cx8cx07xa7xafx01x0cx15x04xf6'
b'x15x04xf6MEASx8ax8cx8cx07xa7xb0x01x0c'
b'x0cx15x04xf6MEASx8ax8cx8cx07xa7xb1x01x0c'
b'x07xa7xb3x01x0cx15x04xf6MEASx89x8cx8cx07xa7xb2x01'
b'x8cx8cx07xa7xb4x01x0cx15x04xf6MEASx89x8cx8c'
b'x85x8cx8cx07xa7xb5x01x0cx15x04xf6MEASx84'
b'MEASx87x8cx8cx07xa7xb6x01x0cx15x04xf6MEAS'
b'x04xf6MEASx8bx8cx8cx07xa7xb7x01x0cx15x04xf6'
b'x15x04xf6MEASx8bx8cx8cx07xa7xb8x01x0cx15'
b'x15x04xf6MEASx8ax8cx8cx07xa7xb9x01x0c'
b'xa7xbbx01x0cx15x04xf6MEASx87x8cx8cx07xa7xbax01x0c'
- try to unpack the preamble !?
How do I read information like Article number
, Serial number
, Channel
, Status
, Measuring Value
between the preamble?
The payload size seems to be fixed here for 22 Bytes (via Wireshark)
python python-3.x tcp telnetlib
python python-3.x tcp telnetlib
asked Nov 22 '18 at 14:10
Shan-DesaiShan-Desai
836933
836933
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Parsing the reversed buffer is just weird; please use struct
's support for endianess. Using big-endian '!' in a little-endian context is also odd.
The first four bytes are a text constant. Ok, fine perhaps you'll need to reverse those. But just those, please.
After that, use struct.unpack
to parse out 'IIQI'
. So far, that was kind of working OK with your approach, since all fields consume 4 bytes or a pair of 4 bytes. But finding frame M's length is the fly in the ointment since it is just 2 bytes, so parse it with 'H'
, giving you a combined 'IIQIH'
. After that, you'll need to advance by only that many bytes, and then expect another 'MEAS' text constant once you've exhausted that set of measurements.
add a comment |
I managed to avoid TelnetLib
altogether and created a tcp
client using python3
. I had the payload size already from my wireshark dump (22 Bytes) hence I keep receiving 22 bytes of Information. Apparently the module sends two distinct 22 Bytes payload
- First (frame) payload has the
preamble
,serial
,article
,channel
information - Second (frame) payload has the information like
bytes per frame
,measuring value counter
,measuring value Channel 1
,measuring value Channel 2
,measuring value Channel 3
The information is in int32
and thus needs a formula to be converted to real readings (mentioned in the instruction manual)
(as mentioned by @J_H the unpacking was as He mentioned in his answer with small changes)
Code
import socket
import time
import struct
DRANGEMIN = 3261
DRANGEMAX = 15853
MEASRANGE = 50
OFFSET = 35
# Create a TCP/IP socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_address = ('169.254.168.150', 10001)
print('connecting to %s port %s' % server_address)
sock.connect(server_address)
def value_mm(raw_val):
return (((raw_val - DRANGEMIN) * MEASRANGE) / (DRANGEMAX - DRANGEMIN) + OFFSET)
if __name__ == '__main__':
while True:
Laser_Value = 0
data = sock.recv(22)
preamble, article, serial, x1, x2 = struct.unpack('<4sIIQH', data)
if not preamble == b'SAEM':
status, bpf, mValCounter, CH1, CH2, CH3 = struct.unpack('<hIIIII',data)
#print(CH1, CH2, CH3)
Laser_Value = CH3
print(str(value_mm(Laser_Value)) + " mm")
#print('RAW: ' + str(len(data)))
print('n')
#time.sleep(0.1)
Sure enough, this provides me the information that is needed and I compared the information via the propreitary software which the company provides.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53432812%2funable-to-unpack-information-between-custom-preamble-in-python-and-telnetlib%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Parsing the reversed buffer is just weird; please use struct
's support for endianess. Using big-endian '!' in a little-endian context is also odd.
The first four bytes are a text constant. Ok, fine perhaps you'll need to reverse those. But just those, please.
After that, use struct.unpack
to parse out 'IIQI'
. So far, that was kind of working OK with your approach, since all fields consume 4 bytes or a pair of 4 bytes. But finding frame M's length is the fly in the ointment since it is just 2 bytes, so parse it with 'H'
, giving you a combined 'IIQIH'
. After that, you'll need to advance by only that many bytes, and then expect another 'MEAS' text constant once you've exhausted that set of measurements.
add a comment |
Parsing the reversed buffer is just weird; please use struct
's support for endianess. Using big-endian '!' in a little-endian context is also odd.
The first four bytes are a text constant. Ok, fine perhaps you'll need to reverse those. But just those, please.
After that, use struct.unpack
to parse out 'IIQI'
. So far, that was kind of working OK with your approach, since all fields consume 4 bytes or a pair of 4 bytes. But finding frame M's length is the fly in the ointment since it is just 2 bytes, so parse it with 'H'
, giving you a combined 'IIQIH'
. After that, you'll need to advance by only that many bytes, and then expect another 'MEAS' text constant once you've exhausted that set of measurements.
add a comment |
Parsing the reversed buffer is just weird; please use struct
's support for endianess. Using big-endian '!' in a little-endian context is also odd.
The first four bytes are a text constant. Ok, fine perhaps you'll need to reverse those. But just those, please.
After that, use struct.unpack
to parse out 'IIQI'
. So far, that was kind of working OK with your approach, since all fields consume 4 bytes or a pair of 4 bytes. But finding frame M's length is the fly in the ointment since it is just 2 bytes, so parse it with 'H'
, giving you a combined 'IIQIH'
. After that, you'll need to advance by only that many bytes, and then expect another 'MEAS' text constant once you've exhausted that set of measurements.
Parsing the reversed buffer is just weird; please use struct
's support for endianess. Using big-endian '!' in a little-endian context is also odd.
The first four bytes are a text constant. Ok, fine perhaps you'll need to reverse those. But just those, please.
After that, use struct.unpack
to parse out 'IIQI'
. So far, that was kind of working OK with your approach, since all fields consume 4 bytes or a pair of 4 bytes. But finding frame M's length is the fly in the ointment since it is just 2 bytes, so parse it with 'H'
, giving you a combined 'IIQIH'
. After that, you'll need to advance by only that many bytes, and then expect another 'MEAS' text constant once you've exhausted that set of measurements.
answered Nov 22 '18 at 21:43


J_HJ_H
3,3201617
3,3201617
add a comment |
add a comment |
I managed to avoid TelnetLib
altogether and created a tcp
client using python3
. I had the payload size already from my wireshark dump (22 Bytes) hence I keep receiving 22 bytes of Information. Apparently the module sends two distinct 22 Bytes payload
- First (frame) payload has the
preamble
,serial
,article
,channel
information - Second (frame) payload has the information like
bytes per frame
,measuring value counter
,measuring value Channel 1
,measuring value Channel 2
,measuring value Channel 3
The information is in int32
and thus needs a formula to be converted to real readings (mentioned in the instruction manual)
(as mentioned by @J_H the unpacking was as He mentioned in his answer with small changes)
Code
import socket
import time
import struct
DRANGEMIN = 3261
DRANGEMAX = 15853
MEASRANGE = 50
OFFSET = 35
# Create a TCP/IP socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_address = ('169.254.168.150', 10001)
print('connecting to %s port %s' % server_address)
sock.connect(server_address)
def value_mm(raw_val):
return (((raw_val - DRANGEMIN) * MEASRANGE) / (DRANGEMAX - DRANGEMIN) + OFFSET)
if __name__ == '__main__':
while True:
Laser_Value = 0
data = sock.recv(22)
preamble, article, serial, x1, x2 = struct.unpack('<4sIIQH', data)
if not preamble == b'SAEM':
status, bpf, mValCounter, CH1, CH2, CH3 = struct.unpack('<hIIIII',data)
#print(CH1, CH2, CH3)
Laser_Value = CH3
print(str(value_mm(Laser_Value)) + " mm")
#print('RAW: ' + str(len(data)))
print('n')
#time.sleep(0.1)
Sure enough, this provides me the information that is needed and I compared the information via the propreitary software which the company provides.
add a comment |
I managed to avoid TelnetLib
altogether and created a tcp
client using python3
. I had the payload size already from my wireshark dump (22 Bytes) hence I keep receiving 22 bytes of Information. Apparently the module sends two distinct 22 Bytes payload
- First (frame) payload has the
preamble
,serial
,article
,channel
information - Second (frame) payload has the information like
bytes per frame
,measuring value counter
,measuring value Channel 1
,measuring value Channel 2
,measuring value Channel 3
The information is in int32
and thus needs a formula to be converted to real readings (mentioned in the instruction manual)
(as mentioned by @J_H the unpacking was as He mentioned in his answer with small changes)
Code
import socket
import time
import struct
DRANGEMIN = 3261
DRANGEMAX = 15853
MEASRANGE = 50
OFFSET = 35
# Create a TCP/IP socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_address = ('169.254.168.150', 10001)
print('connecting to %s port %s' % server_address)
sock.connect(server_address)
def value_mm(raw_val):
return (((raw_val - DRANGEMIN) * MEASRANGE) / (DRANGEMAX - DRANGEMIN) + OFFSET)
if __name__ == '__main__':
while True:
Laser_Value = 0
data = sock.recv(22)
preamble, article, serial, x1, x2 = struct.unpack('<4sIIQH', data)
if not preamble == b'SAEM':
status, bpf, mValCounter, CH1, CH2, CH3 = struct.unpack('<hIIIII',data)
#print(CH1, CH2, CH3)
Laser_Value = CH3
print(str(value_mm(Laser_Value)) + " mm")
#print('RAW: ' + str(len(data)))
print('n')
#time.sleep(0.1)
Sure enough, this provides me the information that is needed and I compared the information via the propreitary software which the company provides.
add a comment |
I managed to avoid TelnetLib
altogether and created a tcp
client using python3
. I had the payload size already from my wireshark dump (22 Bytes) hence I keep receiving 22 bytes of Information. Apparently the module sends two distinct 22 Bytes payload
- First (frame) payload has the
preamble
,serial
,article
,channel
information - Second (frame) payload has the information like
bytes per frame
,measuring value counter
,measuring value Channel 1
,measuring value Channel 2
,measuring value Channel 3
The information is in int32
and thus needs a formula to be converted to real readings (mentioned in the instruction manual)
(as mentioned by @J_H the unpacking was as He mentioned in his answer with small changes)
Code
import socket
import time
import struct
DRANGEMIN = 3261
DRANGEMAX = 15853
MEASRANGE = 50
OFFSET = 35
# Create a TCP/IP socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_address = ('169.254.168.150', 10001)
print('connecting to %s port %s' % server_address)
sock.connect(server_address)
def value_mm(raw_val):
return (((raw_val - DRANGEMIN) * MEASRANGE) / (DRANGEMAX - DRANGEMIN) + OFFSET)
if __name__ == '__main__':
while True:
Laser_Value = 0
data = sock.recv(22)
preamble, article, serial, x1, x2 = struct.unpack('<4sIIQH', data)
if not preamble == b'SAEM':
status, bpf, mValCounter, CH1, CH2, CH3 = struct.unpack('<hIIIII',data)
#print(CH1, CH2, CH3)
Laser_Value = CH3
print(str(value_mm(Laser_Value)) + " mm")
#print('RAW: ' + str(len(data)))
print('n')
#time.sleep(0.1)
Sure enough, this provides me the information that is needed and I compared the information via the propreitary software which the company provides.
I managed to avoid TelnetLib
altogether and created a tcp
client using python3
. I had the payload size already from my wireshark dump (22 Bytes) hence I keep receiving 22 bytes of Information. Apparently the module sends two distinct 22 Bytes payload
- First (frame) payload has the
preamble
,serial
,article
,channel
information - Second (frame) payload has the information like
bytes per frame
,measuring value counter
,measuring value Channel 1
,measuring value Channel 2
,measuring value Channel 3
The information is in int32
and thus needs a formula to be converted to real readings (mentioned in the instruction manual)
(as mentioned by @J_H the unpacking was as He mentioned in his answer with small changes)
Code
import socket
import time
import struct
DRANGEMIN = 3261
DRANGEMAX = 15853
MEASRANGE = 50
OFFSET = 35
# Create a TCP/IP socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_address = ('169.254.168.150', 10001)
print('connecting to %s port %s' % server_address)
sock.connect(server_address)
def value_mm(raw_val):
return (((raw_val - DRANGEMIN) * MEASRANGE) / (DRANGEMAX - DRANGEMIN) + OFFSET)
if __name__ == '__main__':
while True:
Laser_Value = 0
data = sock.recv(22)
preamble, article, serial, x1, x2 = struct.unpack('<4sIIQH', data)
if not preamble == b'SAEM':
status, bpf, mValCounter, CH1, CH2, CH3 = struct.unpack('<hIIIII',data)
#print(CH1, CH2, CH3)
Laser_Value = CH3
print(str(value_mm(Laser_Value)) + " mm")
#print('RAW: ' + str(len(data)))
print('n')
#time.sleep(0.1)
Sure enough, this provides me the information that is needed and I compared the information via the propreitary software which the company provides.
answered Nov 26 '18 at 15:51
Shan-DesaiShan-Desai
836933
836933
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53432812%2funable-to-unpack-information-between-custom-preamble-in-python-and-telnetlib%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8dtE eN14rMvZDJXqz6jxP N9oet6ktpYRz0lHlp,X CJsnQ2SrXACI,EJ9bg x2IUTwR0nSOS,L41iam lOW