Typescript wrapping types
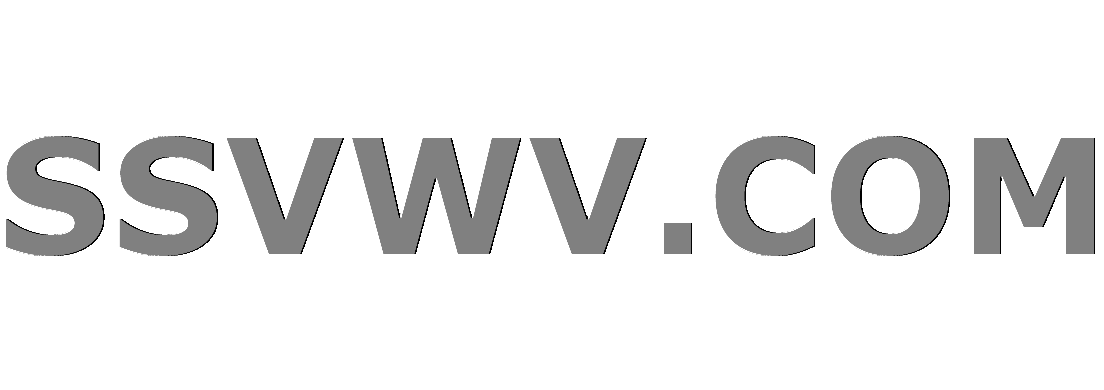
Multi tool use
I have some factory method which is making some computations and manipulations. Also I have a generic method doSomething. I don't wanna to specify type for doSomething each time. I would like to do it once for factory method and then get it each time with already assigned type
function factory<T>(t: T){
// some computations
return {method: doSomething<T>} <- this is what I wanna do
}
// Generic
function<T extends object>doSomething(): T{
//complex stuff, a lot of lambdas
}
How from factory method I can return doSomething with allready assigned type?
javascript angular reactjs typescript
add a comment |
I have some factory method which is making some computations and manipulations. Also I have a generic method doSomething. I don't wanna to specify type for doSomething each time. I would like to do it once for factory method and then get it each time with already assigned type
function factory<T>(t: T){
// some computations
return {method: doSomething<T>} <- this is what I wanna do
}
// Generic
function<T extends object>doSomething(): T{
//complex stuff, a lot of lambdas
}
How from factory method I can return doSomething with allready assigned type?
javascript angular reactjs typescript
add a comment |
I have some factory method which is making some computations and manipulations. Also I have a generic method doSomething. I don't wanna to specify type for doSomething each time. I would like to do it once for factory method and then get it each time with already assigned type
function factory<T>(t: T){
// some computations
return {method: doSomething<T>} <- this is what I wanna do
}
// Generic
function<T extends object>doSomething(): T{
//complex stuff, a lot of lambdas
}
How from factory method I can return doSomething with allready assigned type?
javascript angular reactjs typescript
I have some factory method which is making some computations and manipulations. Also I have a generic method doSomething. I don't wanna to specify type for doSomething each time. I would like to do it once for factory method and then get it each time with already assigned type
function factory<T>(t: T){
// some computations
return {method: doSomething<T>} <- this is what I wanna do
}
// Generic
function<T extends object>doSomething(): T{
//complex stuff, a lot of lambdas
}
How from factory method I can return doSomething with allready assigned type?
javascript angular reactjs typescript
javascript angular reactjs typescript
asked Nov 22 '18 at 22:11
Oskar WoźniakOskar Woźniak
119113
119113
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can't specify the type parameter of a generic function without calling it. So doSomething<T>
is not acceptable; only doSomething<T>()
is allowed. Luckily, you can just return a concrete function that calls the generic function with the proper type parameter specified. Like this:
function factory<T extends object>(t: T) {
// some computations
return { method: ()=>doSomething<T>() }
}
// Generic, note generic parameter comes after the function name
declare function doSomething<T extends object>(): T;
Let's see if it works:
const ret = factory({a: "hey"}).method();
// const ret: { a: string }
Looks good to me. Hope that helps; good luck!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438523%2ftypescript-wrapping-types%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can't specify the type parameter of a generic function without calling it. So doSomething<T>
is not acceptable; only doSomething<T>()
is allowed. Luckily, you can just return a concrete function that calls the generic function with the proper type parameter specified. Like this:
function factory<T extends object>(t: T) {
// some computations
return { method: ()=>doSomething<T>() }
}
// Generic, note generic parameter comes after the function name
declare function doSomething<T extends object>(): T;
Let's see if it works:
const ret = factory({a: "hey"}).method();
// const ret: { a: string }
Looks good to me. Hope that helps; good luck!
add a comment |
You can't specify the type parameter of a generic function without calling it. So doSomething<T>
is not acceptable; only doSomething<T>()
is allowed. Luckily, you can just return a concrete function that calls the generic function with the proper type parameter specified. Like this:
function factory<T extends object>(t: T) {
// some computations
return { method: ()=>doSomething<T>() }
}
// Generic, note generic parameter comes after the function name
declare function doSomething<T extends object>(): T;
Let's see if it works:
const ret = factory({a: "hey"}).method();
// const ret: { a: string }
Looks good to me. Hope that helps; good luck!
add a comment |
You can't specify the type parameter of a generic function without calling it. So doSomething<T>
is not acceptable; only doSomething<T>()
is allowed. Luckily, you can just return a concrete function that calls the generic function with the proper type parameter specified. Like this:
function factory<T extends object>(t: T) {
// some computations
return { method: ()=>doSomething<T>() }
}
// Generic, note generic parameter comes after the function name
declare function doSomething<T extends object>(): T;
Let's see if it works:
const ret = factory({a: "hey"}).method();
// const ret: { a: string }
Looks good to me. Hope that helps; good luck!
You can't specify the type parameter of a generic function without calling it. So doSomething<T>
is not acceptable; only doSomething<T>()
is allowed. Luckily, you can just return a concrete function that calls the generic function with the proper type parameter specified. Like this:
function factory<T extends object>(t: T) {
// some computations
return { method: ()=>doSomething<T>() }
}
// Generic, note generic parameter comes after the function name
declare function doSomething<T extends object>(): T;
Let's see if it works:
const ret = factory({a: "hey"}).method();
// const ret: { a: string }
Looks good to me. Hope that helps; good luck!
answered Nov 23 '18 at 1:50


jcalzjcalz
24.1k22142
24.1k22142
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438523%2ftypescript-wrapping-types%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qF,P 4wGPIsrxt,bR,5CP,YjLfc1VEUux,6f98xX7w 43qVpD OnSoWdKbTI g8AX,6CxYg,TG8SqQKL6Xw6DObI,9z