How do I play music without requiring user action when a notification fires in iOS while the device is...
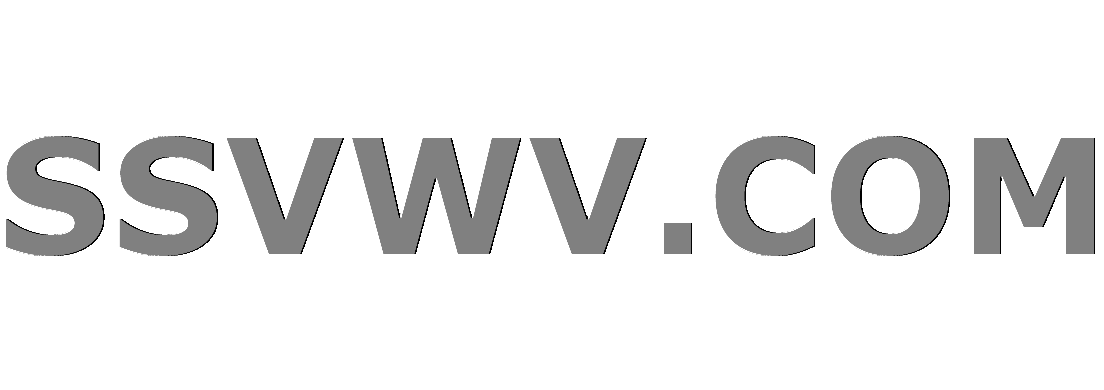
Multi tool use
How do I play music without requiring user action when a notification fires in iOS while the device is locked? The music needs to be a significantly long audio -- longer than the 30-second limit placed on the notification tone. Other posts on stackoverflow indicate it can't be done, but I have seen an app that is able to do it. Perhaps it can be done by a roundabout way, though I would like to learn the preferred way.
Here is my code so far:
let content = UNMutableNotificationContent()
content.categoryIdentifier = "HELLO"
content.title = "Hello World!"
content.body = "May the Force be with you."
var dateComponents = DateComponents()
dateComponents.second = 0
let trigger = UNCalendarNotificationTrigger(dateMatching: dateComponents, repeats: true)
// Create the request
let uuidString = UUID().uuidString
let request = UNNotificationRequest(identifier: uuidString,
content: content, trigger: trigger)
// Schedule the request with the system.
center.add(request) { (error) in
if error != nil {
print("error=", error?.localizedDescription as Any)
}
}
// MARK: - UNUserNotificationCenterDelegate
extension ViewController: UNUserNotificationCenterDelegate {
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
print("willPresent")
completionHandler([.alert, .badge, .sound])
}
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
print("didReceive")
print(response.actionIdentifier)
completionHandler()
}
}
The delegate callback methods don't fire when the device is locked.
ios audio notifications
add a comment |
How do I play music without requiring user action when a notification fires in iOS while the device is locked? The music needs to be a significantly long audio -- longer than the 30-second limit placed on the notification tone. Other posts on stackoverflow indicate it can't be done, but I have seen an app that is able to do it. Perhaps it can be done by a roundabout way, though I would like to learn the preferred way.
Here is my code so far:
let content = UNMutableNotificationContent()
content.categoryIdentifier = "HELLO"
content.title = "Hello World!"
content.body = "May the Force be with you."
var dateComponents = DateComponents()
dateComponents.second = 0
let trigger = UNCalendarNotificationTrigger(dateMatching: dateComponents, repeats: true)
// Create the request
let uuidString = UUID().uuidString
let request = UNNotificationRequest(identifier: uuidString,
content: content, trigger: trigger)
// Schedule the request with the system.
center.add(request) { (error) in
if error != nil {
print("error=", error?.localizedDescription as Any)
}
}
// MARK: - UNUserNotificationCenterDelegate
extension ViewController: UNUserNotificationCenterDelegate {
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
print("willPresent")
completionHandler([.alert, .badge, .sound])
}
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
print("didReceive")
print(response.actionIdentifier)
completionHandler()
}
}
The delegate callback methods don't fire when the device is locked.
ios audio notifications
Delegates will be called once user click on the notification. However you can add custom audio files in the settings of the application to change the notification tone.
– Vatsal K
Nov 22 '18 at 22:22
@VatsalK I actually need to audio to be a full-blown mp3 file longer than the 30-second limit placed on the notification tone. I will add that detail in my post.
– Daniel Brower
Nov 22 '18 at 22:25
add a comment |
How do I play music without requiring user action when a notification fires in iOS while the device is locked? The music needs to be a significantly long audio -- longer than the 30-second limit placed on the notification tone. Other posts on stackoverflow indicate it can't be done, but I have seen an app that is able to do it. Perhaps it can be done by a roundabout way, though I would like to learn the preferred way.
Here is my code so far:
let content = UNMutableNotificationContent()
content.categoryIdentifier = "HELLO"
content.title = "Hello World!"
content.body = "May the Force be with you."
var dateComponents = DateComponents()
dateComponents.second = 0
let trigger = UNCalendarNotificationTrigger(dateMatching: dateComponents, repeats: true)
// Create the request
let uuidString = UUID().uuidString
let request = UNNotificationRequest(identifier: uuidString,
content: content, trigger: trigger)
// Schedule the request with the system.
center.add(request) { (error) in
if error != nil {
print("error=", error?.localizedDescription as Any)
}
}
// MARK: - UNUserNotificationCenterDelegate
extension ViewController: UNUserNotificationCenterDelegate {
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
print("willPresent")
completionHandler([.alert, .badge, .sound])
}
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
print("didReceive")
print(response.actionIdentifier)
completionHandler()
}
}
The delegate callback methods don't fire when the device is locked.
ios audio notifications
How do I play music without requiring user action when a notification fires in iOS while the device is locked? The music needs to be a significantly long audio -- longer than the 30-second limit placed on the notification tone. Other posts on stackoverflow indicate it can't be done, but I have seen an app that is able to do it. Perhaps it can be done by a roundabout way, though I would like to learn the preferred way.
Here is my code so far:
let content = UNMutableNotificationContent()
content.categoryIdentifier = "HELLO"
content.title = "Hello World!"
content.body = "May the Force be with you."
var dateComponents = DateComponents()
dateComponents.second = 0
let trigger = UNCalendarNotificationTrigger(dateMatching: dateComponents, repeats: true)
// Create the request
let uuidString = UUID().uuidString
let request = UNNotificationRequest(identifier: uuidString,
content: content, trigger: trigger)
// Schedule the request with the system.
center.add(request) { (error) in
if error != nil {
print("error=", error?.localizedDescription as Any)
}
}
// MARK: - UNUserNotificationCenterDelegate
extension ViewController: UNUserNotificationCenterDelegate {
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
print("willPresent")
completionHandler([.alert, .badge, .sound])
}
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
print("didReceive")
print(response.actionIdentifier)
completionHandler()
}
}
The delegate callback methods don't fire when the device is locked.
ios audio notifications
ios audio notifications
edited Nov 22 '18 at 22:27
Daniel Brower
asked Nov 22 '18 at 22:13
Daniel BrowerDaniel Brower
10610
10610
Delegates will be called once user click on the notification. However you can add custom audio files in the settings of the application to change the notification tone.
– Vatsal K
Nov 22 '18 at 22:22
@VatsalK I actually need to audio to be a full-blown mp3 file longer than the 30-second limit placed on the notification tone. I will add that detail in my post.
– Daniel Brower
Nov 22 '18 at 22:25
add a comment |
Delegates will be called once user click on the notification. However you can add custom audio files in the settings of the application to change the notification tone.
– Vatsal K
Nov 22 '18 at 22:22
@VatsalK I actually need to audio to be a full-blown mp3 file longer than the 30-second limit placed on the notification tone. I will add that detail in my post.
– Daniel Brower
Nov 22 '18 at 22:25
Delegates will be called once user click on the notification. However you can add custom audio files in the settings of the application to change the notification tone.
– Vatsal K
Nov 22 '18 at 22:22
Delegates will be called once user click on the notification. However you can add custom audio files in the settings of the application to change the notification tone.
– Vatsal K
Nov 22 '18 at 22:22
@VatsalK I actually need to audio to be a full-blown mp3 file longer than the 30-second limit placed on the notification tone. I will add that detail in my post.
– Daniel Brower
Nov 22 '18 at 22:25
@VatsalK I actually need to audio to be a full-blown mp3 file longer than the 30-second limit placed on the notification tone. I will add that detail in my post.
– Daniel Brower
Nov 22 '18 at 22:25
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438536%2fhow-do-i-play-music-without-requiring-user-action-when-a-notification-fires-in-i%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438536%2fhow-do-i-play-music-without-requiring-user-action-when-a-notification-fires-in-i%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
77fU3VXzeFQQtUsTrWRYoSrAj5mzH7NsOTFc7PL2y1 w7pKe2TYC6ONUwcf4fQ
Delegates will be called once user click on the notification. However you can add custom audio files in the settings of the application to change the notification tone.
– Vatsal K
Nov 22 '18 at 22:22
@VatsalK I actually need to audio to be a full-blown mp3 file longer than the 30-second limit placed on the notification tone. I will add that detail in my post.
– Daniel Brower
Nov 22 '18 at 22:25