php socket client not receiving continuous data
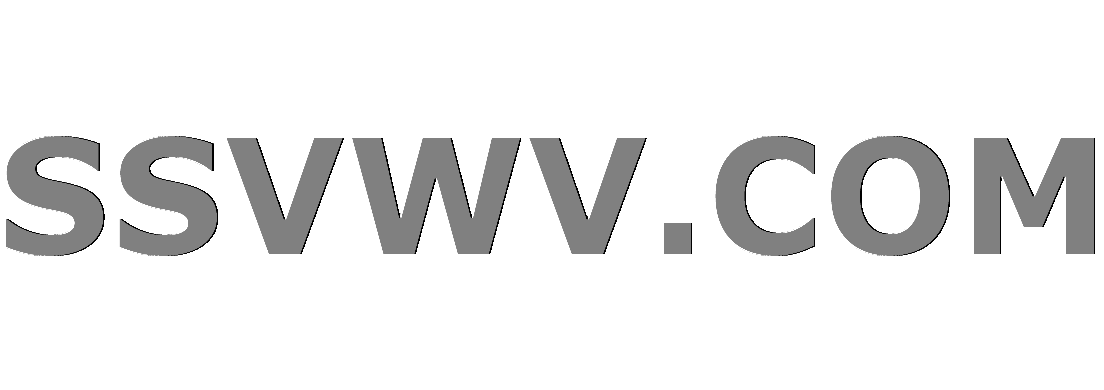
Multi tool use
My goal is capture messages being generated in real time by a Java server socket and display these messages on the webpage.
I am trying to use php to connect to the socket and receive data from the server. The php client should continuously listen to the server for messages.
Here is my php code
<?php
set_time_limit(0);
$serverAddress=SERVER_ADDRESS;
$serverListeningPort=SERVER_PORT;
//make a connection and get a socket object
if ( ($socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP)) === FALSE )
{
echo "socket_create() failed: reason: " .socket_strerror(socket_last_error());
}
else
{
echo("socket create was successful");
echo("<br>");
}
echo ("Attempting to connect to host");
echo("<br>");
if ( ($result = socket_connect($socket, $serverAddress, $serverListeningPort)) === FALSE )
{
echo ("socket_connect() failed. Reason:".socket_strerror(socket_last_error($socket)));
}
echo ("Reading response:");
$message="";
while(true)//listen for ever
{
$message=socket_read($socket, 300);
if($message!=='')
{
print_r($message);
}
}
?>
When I load this php page, most of the time I get a 504 gateway time out error. I have verified that the Java server is picking up the client connection.
Sometimes, I get a few messages only and then the page stops getting messages from the server. Not sure why, as I have a while(true) loop.
Am I using the php socket correctly? How can I accomplish my goal.
Thank you
php
add a comment |
My goal is capture messages being generated in real time by a Java server socket and display these messages on the webpage.
I am trying to use php to connect to the socket and receive data from the server. The php client should continuously listen to the server for messages.
Here is my php code
<?php
set_time_limit(0);
$serverAddress=SERVER_ADDRESS;
$serverListeningPort=SERVER_PORT;
//make a connection and get a socket object
if ( ($socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP)) === FALSE )
{
echo "socket_create() failed: reason: " .socket_strerror(socket_last_error());
}
else
{
echo("socket create was successful");
echo("<br>");
}
echo ("Attempting to connect to host");
echo("<br>");
if ( ($result = socket_connect($socket, $serverAddress, $serverListeningPort)) === FALSE )
{
echo ("socket_connect() failed. Reason:".socket_strerror(socket_last_error($socket)));
}
echo ("Reading response:");
$message="";
while(true)//listen for ever
{
$message=socket_read($socket, 300);
if($message!=='')
{
print_r($message);
}
}
?>
When I load this php page, most of the time I get a 504 gateway time out error. I have verified that the Java server is picking up the client connection.
Sometimes, I get a few messages only and then the page stops getting messages from the server. Not sure why, as I have a while(true) loop.
Am I using the php socket correctly? How can I accomplish my goal.
Thank you
php
How do you configure web server for processing PHP? You can't use reverse proxy for this. 2. PHP isn't good solution for you. You need extra layer who continuously get data from Java socket, store it and send to client when it request for it by AJAX. Or use websockets
– bato3
Nov 22 '18 at 22:12
Thanks for the note, @bato3. Not sure I follow 'How do you configure web server for processing PHP? '. Apache processes php. What could that extra layer be? Thanks
– dollarSquare
Nov 22 '18 at 23:15
add a comment |
My goal is capture messages being generated in real time by a Java server socket and display these messages on the webpage.
I am trying to use php to connect to the socket and receive data from the server. The php client should continuously listen to the server for messages.
Here is my php code
<?php
set_time_limit(0);
$serverAddress=SERVER_ADDRESS;
$serverListeningPort=SERVER_PORT;
//make a connection and get a socket object
if ( ($socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP)) === FALSE )
{
echo "socket_create() failed: reason: " .socket_strerror(socket_last_error());
}
else
{
echo("socket create was successful");
echo("<br>");
}
echo ("Attempting to connect to host");
echo("<br>");
if ( ($result = socket_connect($socket, $serverAddress, $serverListeningPort)) === FALSE )
{
echo ("socket_connect() failed. Reason:".socket_strerror(socket_last_error($socket)));
}
echo ("Reading response:");
$message="";
while(true)//listen for ever
{
$message=socket_read($socket, 300);
if($message!=='')
{
print_r($message);
}
}
?>
When I load this php page, most of the time I get a 504 gateway time out error. I have verified that the Java server is picking up the client connection.
Sometimes, I get a few messages only and then the page stops getting messages from the server. Not sure why, as I have a while(true) loop.
Am I using the php socket correctly? How can I accomplish my goal.
Thank you
php
My goal is capture messages being generated in real time by a Java server socket and display these messages on the webpage.
I am trying to use php to connect to the socket and receive data from the server. The php client should continuously listen to the server for messages.
Here is my php code
<?php
set_time_limit(0);
$serverAddress=SERVER_ADDRESS;
$serverListeningPort=SERVER_PORT;
//make a connection and get a socket object
if ( ($socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP)) === FALSE )
{
echo "socket_create() failed: reason: " .socket_strerror(socket_last_error());
}
else
{
echo("socket create was successful");
echo("<br>");
}
echo ("Attempting to connect to host");
echo("<br>");
if ( ($result = socket_connect($socket, $serverAddress, $serverListeningPort)) === FALSE )
{
echo ("socket_connect() failed. Reason:".socket_strerror(socket_last_error($socket)));
}
echo ("Reading response:");
$message="";
while(true)//listen for ever
{
$message=socket_read($socket, 300);
if($message!=='')
{
print_r($message);
}
}
?>
When I load this php page, most of the time I get a 504 gateway time out error. I have verified that the Java server is picking up the client connection.
Sometimes, I get a few messages only and then the page stops getting messages from the server. Not sure why, as I have a while(true) loop.
Am I using the php socket correctly? How can I accomplish my goal.
Thank you
php
php
asked Nov 22 '18 at 21:56
dollarSquaredollarSquare
133
133
How do you configure web server for processing PHP? You can't use reverse proxy for this. 2. PHP isn't good solution for you. You need extra layer who continuously get data from Java socket, store it and send to client when it request for it by AJAX. Or use websockets
– bato3
Nov 22 '18 at 22:12
Thanks for the note, @bato3. Not sure I follow 'How do you configure web server for processing PHP? '. Apache processes php. What could that extra layer be? Thanks
– dollarSquare
Nov 22 '18 at 23:15
add a comment |
How do you configure web server for processing PHP? You can't use reverse proxy for this. 2. PHP isn't good solution for you. You need extra layer who continuously get data from Java socket, store it and send to client when it request for it by AJAX. Or use websockets
– bato3
Nov 22 '18 at 22:12
Thanks for the note, @bato3. Not sure I follow 'How do you configure web server for processing PHP? '. Apache processes php. What could that extra layer be? Thanks
– dollarSquare
Nov 22 '18 at 23:15
How do you configure web server for processing PHP? You can't use reverse proxy for this. 2. PHP isn't good solution for you. You need extra layer who continuously get data from Java socket, store it and send to client when it request for it by AJAX. Or use websockets
– bato3
Nov 22 '18 at 22:12
How do you configure web server for processing PHP? You can't use reverse proxy for this. 2. PHP isn't good solution for you. You need extra layer who continuously get data from Java socket, store it and send to client when it request for it by AJAX. Or use websockets
– bato3
Nov 22 '18 at 22:12
Thanks for the note, @bato3. Not sure I follow 'How do you configure web server for processing PHP? '. Apache processes php. What could that extra layer be? Thanks
– dollarSquare
Nov 22 '18 at 23:15
Thanks for the note, @bato3. Not sure I follow 'How do you configure web server for processing PHP? '. Apache processes php. What could that extra layer be? Thanks
– dollarSquare
Nov 22 '18 at 23:15
add a comment |
1 Answer
1
active
oldest
votes
Try adding the below lines at the start of your code. This will output the result to browser as it is generated.
ob_end_flush();
ob_implicit_flush(1);
awesome, this works. But this is causing the other pages of my website to not load- they give a 504 error. Any idea why. Thanks a lot.
– dollarSquare
Nov 23 '18 at 11:54
not sure either, could be because of memory leak caused by the current script. which php version are you using
– subroutines
Nov 26 '18 at 0:17
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438394%2fphp-socket-client-not-receiving-continuous-data%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try adding the below lines at the start of your code. This will output the result to browser as it is generated.
ob_end_flush();
ob_implicit_flush(1);
awesome, this works. But this is causing the other pages of my website to not load- they give a 504 error. Any idea why. Thanks a lot.
– dollarSquare
Nov 23 '18 at 11:54
not sure either, could be because of memory leak caused by the current script. which php version are you using
– subroutines
Nov 26 '18 at 0:17
add a comment |
Try adding the below lines at the start of your code. This will output the result to browser as it is generated.
ob_end_flush();
ob_implicit_flush(1);
awesome, this works. But this is causing the other pages of my website to not load- they give a 504 error. Any idea why. Thanks a lot.
– dollarSquare
Nov 23 '18 at 11:54
not sure either, could be because of memory leak caused by the current script. which php version are you using
– subroutines
Nov 26 '18 at 0:17
add a comment |
Try adding the below lines at the start of your code. This will output the result to browser as it is generated.
ob_end_flush();
ob_implicit_flush(1);
Try adding the below lines at the start of your code. This will output the result to browser as it is generated.
ob_end_flush();
ob_implicit_flush(1);
answered Nov 23 '18 at 0:53
subroutinessubroutines
1,38311115
1,38311115
awesome, this works. But this is causing the other pages of my website to not load- they give a 504 error. Any idea why. Thanks a lot.
– dollarSquare
Nov 23 '18 at 11:54
not sure either, could be because of memory leak caused by the current script. which php version are you using
– subroutines
Nov 26 '18 at 0:17
add a comment |
awesome, this works. But this is causing the other pages of my website to not load- they give a 504 error. Any idea why. Thanks a lot.
– dollarSquare
Nov 23 '18 at 11:54
not sure either, could be because of memory leak caused by the current script. which php version are you using
– subroutines
Nov 26 '18 at 0:17
awesome, this works. But this is causing the other pages of my website to not load- they give a 504 error. Any idea why. Thanks a lot.
– dollarSquare
Nov 23 '18 at 11:54
awesome, this works. But this is causing the other pages of my website to not load- they give a 504 error. Any idea why. Thanks a lot.
– dollarSquare
Nov 23 '18 at 11:54
not sure either, could be because of memory leak caused by the current script. which php version are you using
– subroutines
Nov 26 '18 at 0:17
not sure either, could be because of memory leak caused by the current script. which php version are you using
– subroutines
Nov 26 '18 at 0:17
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438394%2fphp-socket-client-not-receiving-continuous-data%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PL0iha y1U 3TGTc3htADpSAHY7k,NJqbTGMCzHey4A4Cm,gO VJS,RH0iQEw32iNcKj
How do you configure web server for processing PHP? You can't use reverse proxy for this. 2. PHP isn't good solution for you. You need extra layer who continuously get data from Java socket, store it and send to client when it request for it by AJAX. Or use websockets
– bato3
Nov 22 '18 at 22:12
Thanks for the note, @bato3. Not sure I follow 'How do you configure web server for processing PHP? '. Apache processes php. What could that extra layer be? Thanks
– dollarSquare
Nov 22 '18 at 23:15