Take events from Database to FullCalendar
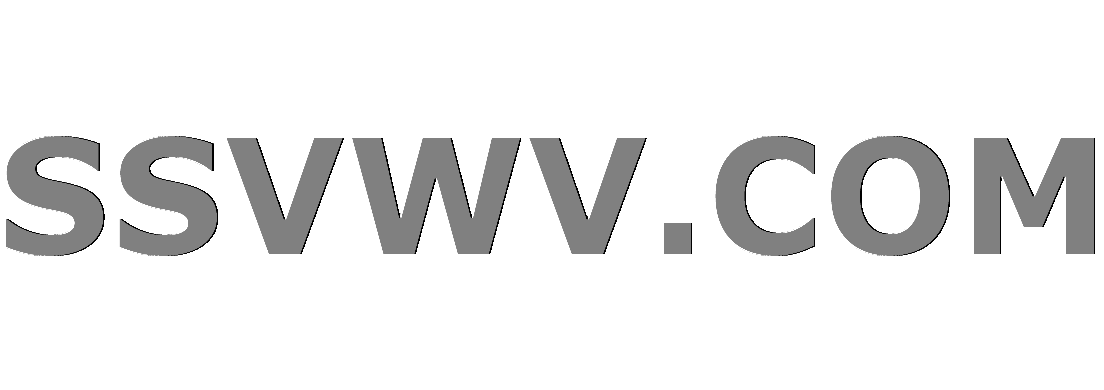
Multi tool use
So, I searched this everywhere and couldn't implement a working method.
I'm building a C#, ASP.NET system and in this project it's being used Full Calendar v3.9, I also have a Database on SQLServer where the events are save. I'm triyng to load these events in the Calendar, but I couldn't manage to do it.
My Class (ModeloTarefas):
private int id;
private string title, start , end;
public int Id
{
get { return id; }
set { id = value; }
}
public string Title
{
get{ return title; }
set{ this.title = value; }
}
public string Start
{
get { return start; }
set { start = value; }
}
public string End
{
get { return end; }
set { end = value; }
}
//CONSTRUCTOR
public ModeloTarefas(int id, String title, String start, String end)
{
this.id = id;
this.title = title;
this.start = start;
this.end = end;
}
WebServer Method:
[WebMethod]
public String ListarTarefas()
{
//Criar um array para guardar as informações que virão do BD
List<ModeloTarefas> lista = new List<ModeloTarefas>();
string tarefasJsonString = "";
try
{
con.Open();
//Comando para selecionar os itens da tabela peixes
SqlCommand listar = new SqlCommand("SELECT * FROM Tarefas", con);
SqlDataReader leitor = listar.ExecuteReader();
//Realiza a captura dos dados caso haja algo na tabela
if (leitor.HasRows)
{
var indexOfId = leitor.GetOrdinal("ID");
var indexOfTitle = leitor.GetOrdinal("Title");
var indexOfStart = leitor.GetOrdinal("start");
var indexOfEnd = leitor.GetOrdinal("end");
while (leitor.Read())
{
var id = (int)leitor.GetValue(indexOfId);
var title = leitor.GetValue(indexOfTitle).ToString();
var start = leitor.GetValue(indexOfStart);
var end = leitor.GetValue(indexOfEnd);
//Convert Implicity typed var to Date Time
DateTime RealData_Inicio = Convert.ToDateTime(start);
DateTime RealData_Fim = Convert.ToDateTime(end);
//Convert Date Time to ISO
String ReadyData_Inicio = RealData_Inicio.ToString("s");
String ReadyData_Fim = RealData_Fim.ToString("s");
ModeloTarefas tarefa = new ModeloTarefas(id, title, ReadyData_Inicio, ReadyData_Fim);
lista.Add(tarefa);
}
tarefasJsonString = (new JavaScriptSerializer()).Serialize(lista);
}
}
catch (Exception e)
{
lista = null;
}
if (con.State == ConnectionState.Open)
con.Close();
return tarefasJsonString;
}
JavaScript to load the Calendar:
<script>
//Get current Date
var today = new Date();
var dd = today.getDate();
var mm = today.getMonth() + 1; //January is 0!
var yyyy = today.getFullYear();
if (dd < 10) {
dd = '0' + dd
}
if (mm < 10) {
mm = '0' + mm
}
today = yyyy + '/' + mm + '/' + dd;
//Load Calendar
$(document).ready(function () {
$('#calendar').fullCalendar({
header: {
left: 'prev,next today',
center: 'title',
right: 'listDay,listWeek,month'
},
// customize the button names,
// otherwise they'd all just say "list"
views: {
listDay: { buttonText: 'Dia' },
listWeek: { buttonText: 'Semana' }
},
defaultView: 'month',
defaultDate: today,
locale: 'pt-br',
navLinks: true, // can click day/week names to navigate views
editable: true,
selectable: true,
selectHelper: true,
eventLimit: true, // allow "more" link when too many events
//Create events when clicking
select: function (start, end) {
var title = prompt('Event Title:');
var eventData;
if (title) {
eventData = {
title: title,
start: start,
end: end
};
$('#calendar').fullCalendar('renderEvent', eventData, true); // stick? = true
}
$('#calendar').fullCalendar('unselect');
},
// Traz os eventos do Banco de Dados
eventSources: [
{
url: '../WebService1.asmx/ListarTarefas',
type: 'POST',
error: function () {
alert('Ocorreu um erro ao retornar as Tarefas. Por favor, entre em contato conosco.');
console.log(MediaError)
},
color: 'yellow', // a non-ajax option
textColor: 'black' // a non-ajax option
}
]
});
});
</script>
I'm not a professional in Javascript (actually this is my first big project with JS). I tried some solutions I've found here on StackOverflow but couldn't make it work.
This is the JSON that the WEB Method return:
<string xmlns="http://tempuri.org/">
[{"Id":3,"Title":"Teste Tarefa 1","Start":"2018-11-13T00:00:00","End":"2018-11-13T00:00:00"},{"Id":4,"Title":"Teste Tarefa 2","Start":"2018-11-13T00:00:00","End":"2018-11-13T00:00:00"}]
</string>
javascript c# asp.net-mvc fullcalendar fullcalendar-3
add a comment |
So, I searched this everywhere and couldn't implement a working method.
I'm building a C#, ASP.NET system and in this project it's being used Full Calendar v3.9, I also have a Database on SQLServer where the events are save. I'm triyng to load these events in the Calendar, but I couldn't manage to do it.
My Class (ModeloTarefas):
private int id;
private string title, start , end;
public int Id
{
get { return id; }
set { id = value; }
}
public string Title
{
get{ return title; }
set{ this.title = value; }
}
public string Start
{
get { return start; }
set { start = value; }
}
public string End
{
get { return end; }
set { end = value; }
}
//CONSTRUCTOR
public ModeloTarefas(int id, String title, String start, String end)
{
this.id = id;
this.title = title;
this.start = start;
this.end = end;
}
WebServer Method:
[WebMethod]
public String ListarTarefas()
{
//Criar um array para guardar as informações que virão do BD
List<ModeloTarefas> lista = new List<ModeloTarefas>();
string tarefasJsonString = "";
try
{
con.Open();
//Comando para selecionar os itens da tabela peixes
SqlCommand listar = new SqlCommand("SELECT * FROM Tarefas", con);
SqlDataReader leitor = listar.ExecuteReader();
//Realiza a captura dos dados caso haja algo na tabela
if (leitor.HasRows)
{
var indexOfId = leitor.GetOrdinal("ID");
var indexOfTitle = leitor.GetOrdinal("Title");
var indexOfStart = leitor.GetOrdinal("start");
var indexOfEnd = leitor.GetOrdinal("end");
while (leitor.Read())
{
var id = (int)leitor.GetValue(indexOfId);
var title = leitor.GetValue(indexOfTitle).ToString();
var start = leitor.GetValue(indexOfStart);
var end = leitor.GetValue(indexOfEnd);
//Convert Implicity typed var to Date Time
DateTime RealData_Inicio = Convert.ToDateTime(start);
DateTime RealData_Fim = Convert.ToDateTime(end);
//Convert Date Time to ISO
String ReadyData_Inicio = RealData_Inicio.ToString("s");
String ReadyData_Fim = RealData_Fim.ToString("s");
ModeloTarefas tarefa = new ModeloTarefas(id, title, ReadyData_Inicio, ReadyData_Fim);
lista.Add(tarefa);
}
tarefasJsonString = (new JavaScriptSerializer()).Serialize(lista);
}
}
catch (Exception e)
{
lista = null;
}
if (con.State == ConnectionState.Open)
con.Close();
return tarefasJsonString;
}
JavaScript to load the Calendar:
<script>
//Get current Date
var today = new Date();
var dd = today.getDate();
var mm = today.getMonth() + 1; //January is 0!
var yyyy = today.getFullYear();
if (dd < 10) {
dd = '0' + dd
}
if (mm < 10) {
mm = '0' + mm
}
today = yyyy + '/' + mm + '/' + dd;
//Load Calendar
$(document).ready(function () {
$('#calendar').fullCalendar({
header: {
left: 'prev,next today',
center: 'title',
right: 'listDay,listWeek,month'
},
// customize the button names,
// otherwise they'd all just say "list"
views: {
listDay: { buttonText: 'Dia' },
listWeek: { buttonText: 'Semana' }
},
defaultView: 'month',
defaultDate: today,
locale: 'pt-br',
navLinks: true, // can click day/week names to navigate views
editable: true,
selectable: true,
selectHelper: true,
eventLimit: true, // allow "more" link when too many events
//Create events when clicking
select: function (start, end) {
var title = prompt('Event Title:');
var eventData;
if (title) {
eventData = {
title: title,
start: start,
end: end
};
$('#calendar').fullCalendar('renderEvent', eventData, true); // stick? = true
}
$('#calendar').fullCalendar('unselect');
},
// Traz os eventos do Banco de Dados
eventSources: [
{
url: '../WebService1.asmx/ListarTarefas',
type: 'POST',
error: function () {
alert('Ocorreu um erro ao retornar as Tarefas. Por favor, entre em contato conosco.');
console.log(MediaError)
},
color: 'yellow', // a non-ajax option
textColor: 'black' // a non-ajax option
}
]
});
});
</script>
I'm not a professional in Javascript (actually this is my first big project with JS). I tried some solutions I've found here on StackOverflow but couldn't make it work.
This is the JSON that the WEB Method return:
<string xmlns="http://tempuri.org/">
[{"Id":3,"Title":"Teste Tarefa 1","Start":"2018-11-13T00:00:00","End":"2018-11-13T00:00:00"},{"Id":4,"Title":"Teste Tarefa 2","Start":"2018-11-13T00:00:00","End":"2018-11-13T00:00:00"}]
</string>
javascript c# asp.net-mvc fullcalendar fullcalendar-3
"This is the JSON"...problem is, it appears to be JSON wrapped in XML, for some reason.
– ADyson
Nov 22 '18 at 9:55
I think this is because you're using a legacy ASMX web service which returns XML by default. They have not been the standard thing to use for about 10 years now. Any reason you used that instead of a WCF webservice or Web API? If you want to stick with ASMX I think you can maybe change the configuration somewhere to stop it wrapping the XML, you'd have to google it though, it's been years since I worked with ASMX.
– ADyson
Nov 22 '18 at 10:01
I've changed succefully and now it returns just the JSON. But the Calendar still doesn't load the event.
– M. Robert
Nov 22 '18 at 13:13
I believe the property names in the JSON need to be lower case, likeevents: [{ "id": 3, "title": "Teste Tarefa 1", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }, { "id": 4, "title": "Teste Tarefa 2", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }]
Demo: Not working, Working
– ADyson
Nov 22 '18 at 13:31
It worked, I changed to lower and is showing now. Thank You !
– M. Robert
Nov 22 '18 at 13:52
add a comment |
So, I searched this everywhere and couldn't implement a working method.
I'm building a C#, ASP.NET system and in this project it's being used Full Calendar v3.9, I also have a Database on SQLServer where the events are save. I'm triyng to load these events in the Calendar, but I couldn't manage to do it.
My Class (ModeloTarefas):
private int id;
private string title, start , end;
public int Id
{
get { return id; }
set { id = value; }
}
public string Title
{
get{ return title; }
set{ this.title = value; }
}
public string Start
{
get { return start; }
set { start = value; }
}
public string End
{
get { return end; }
set { end = value; }
}
//CONSTRUCTOR
public ModeloTarefas(int id, String title, String start, String end)
{
this.id = id;
this.title = title;
this.start = start;
this.end = end;
}
WebServer Method:
[WebMethod]
public String ListarTarefas()
{
//Criar um array para guardar as informações que virão do BD
List<ModeloTarefas> lista = new List<ModeloTarefas>();
string tarefasJsonString = "";
try
{
con.Open();
//Comando para selecionar os itens da tabela peixes
SqlCommand listar = new SqlCommand("SELECT * FROM Tarefas", con);
SqlDataReader leitor = listar.ExecuteReader();
//Realiza a captura dos dados caso haja algo na tabela
if (leitor.HasRows)
{
var indexOfId = leitor.GetOrdinal("ID");
var indexOfTitle = leitor.GetOrdinal("Title");
var indexOfStart = leitor.GetOrdinal("start");
var indexOfEnd = leitor.GetOrdinal("end");
while (leitor.Read())
{
var id = (int)leitor.GetValue(indexOfId);
var title = leitor.GetValue(indexOfTitle).ToString();
var start = leitor.GetValue(indexOfStart);
var end = leitor.GetValue(indexOfEnd);
//Convert Implicity typed var to Date Time
DateTime RealData_Inicio = Convert.ToDateTime(start);
DateTime RealData_Fim = Convert.ToDateTime(end);
//Convert Date Time to ISO
String ReadyData_Inicio = RealData_Inicio.ToString("s");
String ReadyData_Fim = RealData_Fim.ToString("s");
ModeloTarefas tarefa = new ModeloTarefas(id, title, ReadyData_Inicio, ReadyData_Fim);
lista.Add(tarefa);
}
tarefasJsonString = (new JavaScriptSerializer()).Serialize(lista);
}
}
catch (Exception e)
{
lista = null;
}
if (con.State == ConnectionState.Open)
con.Close();
return tarefasJsonString;
}
JavaScript to load the Calendar:
<script>
//Get current Date
var today = new Date();
var dd = today.getDate();
var mm = today.getMonth() + 1; //January is 0!
var yyyy = today.getFullYear();
if (dd < 10) {
dd = '0' + dd
}
if (mm < 10) {
mm = '0' + mm
}
today = yyyy + '/' + mm + '/' + dd;
//Load Calendar
$(document).ready(function () {
$('#calendar').fullCalendar({
header: {
left: 'prev,next today',
center: 'title',
right: 'listDay,listWeek,month'
},
// customize the button names,
// otherwise they'd all just say "list"
views: {
listDay: { buttonText: 'Dia' },
listWeek: { buttonText: 'Semana' }
},
defaultView: 'month',
defaultDate: today,
locale: 'pt-br',
navLinks: true, // can click day/week names to navigate views
editable: true,
selectable: true,
selectHelper: true,
eventLimit: true, // allow "more" link when too many events
//Create events when clicking
select: function (start, end) {
var title = prompt('Event Title:');
var eventData;
if (title) {
eventData = {
title: title,
start: start,
end: end
};
$('#calendar').fullCalendar('renderEvent', eventData, true); // stick? = true
}
$('#calendar').fullCalendar('unselect');
},
// Traz os eventos do Banco de Dados
eventSources: [
{
url: '../WebService1.asmx/ListarTarefas',
type: 'POST',
error: function () {
alert('Ocorreu um erro ao retornar as Tarefas. Por favor, entre em contato conosco.');
console.log(MediaError)
},
color: 'yellow', // a non-ajax option
textColor: 'black' // a non-ajax option
}
]
});
});
</script>
I'm not a professional in Javascript (actually this is my first big project with JS). I tried some solutions I've found here on StackOverflow but couldn't make it work.
This is the JSON that the WEB Method return:
<string xmlns="http://tempuri.org/">
[{"Id":3,"Title":"Teste Tarefa 1","Start":"2018-11-13T00:00:00","End":"2018-11-13T00:00:00"},{"Id":4,"Title":"Teste Tarefa 2","Start":"2018-11-13T00:00:00","End":"2018-11-13T00:00:00"}]
</string>
javascript c# asp.net-mvc fullcalendar fullcalendar-3
So, I searched this everywhere and couldn't implement a working method.
I'm building a C#, ASP.NET system and in this project it's being used Full Calendar v3.9, I also have a Database on SQLServer where the events are save. I'm triyng to load these events in the Calendar, but I couldn't manage to do it.
My Class (ModeloTarefas):
private int id;
private string title, start , end;
public int Id
{
get { return id; }
set { id = value; }
}
public string Title
{
get{ return title; }
set{ this.title = value; }
}
public string Start
{
get { return start; }
set { start = value; }
}
public string End
{
get { return end; }
set { end = value; }
}
//CONSTRUCTOR
public ModeloTarefas(int id, String title, String start, String end)
{
this.id = id;
this.title = title;
this.start = start;
this.end = end;
}
WebServer Method:
[WebMethod]
public String ListarTarefas()
{
//Criar um array para guardar as informações que virão do BD
List<ModeloTarefas> lista = new List<ModeloTarefas>();
string tarefasJsonString = "";
try
{
con.Open();
//Comando para selecionar os itens da tabela peixes
SqlCommand listar = new SqlCommand("SELECT * FROM Tarefas", con);
SqlDataReader leitor = listar.ExecuteReader();
//Realiza a captura dos dados caso haja algo na tabela
if (leitor.HasRows)
{
var indexOfId = leitor.GetOrdinal("ID");
var indexOfTitle = leitor.GetOrdinal("Title");
var indexOfStart = leitor.GetOrdinal("start");
var indexOfEnd = leitor.GetOrdinal("end");
while (leitor.Read())
{
var id = (int)leitor.GetValue(indexOfId);
var title = leitor.GetValue(indexOfTitle).ToString();
var start = leitor.GetValue(indexOfStart);
var end = leitor.GetValue(indexOfEnd);
//Convert Implicity typed var to Date Time
DateTime RealData_Inicio = Convert.ToDateTime(start);
DateTime RealData_Fim = Convert.ToDateTime(end);
//Convert Date Time to ISO
String ReadyData_Inicio = RealData_Inicio.ToString("s");
String ReadyData_Fim = RealData_Fim.ToString("s");
ModeloTarefas tarefa = new ModeloTarefas(id, title, ReadyData_Inicio, ReadyData_Fim);
lista.Add(tarefa);
}
tarefasJsonString = (new JavaScriptSerializer()).Serialize(lista);
}
}
catch (Exception e)
{
lista = null;
}
if (con.State == ConnectionState.Open)
con.Close();
return tarefasJsonString;
}
JavaScript to load the Calendar:
<script>
//Get current Date
var today = new Date();
var dd = today.getDate();
var mm = today.getMonth() + 1; //January is 0!
var yyyy = today.getFullYear();
if (dd < 10) {
dd = '0' + dd
}
if (mm < 10) {
mm = '0' + mm
}
today = yyyy + '/' + mm + '/' + dd;
//Load Calendar
$(document).ready(function () {
$('#calendar').fullCalendar({
header: {
left: 'prev,next today',
center: 'title',
right: 'listDay,listWeek,month'
},
// customize the button names,
// otherwise they'd all just say "list"
views: {
listDay: { buttonText: 'Dia' },
listWeek: { buttonText: 'Semana' }
},
defaultView: 'month',
defaultDate: today,
locale: 'pt-br',
navLinks: true, // can click day/week names to navigate views
editable: true,
selectable: true,
selectHelper: true,
eventLimit: true, // allow "more" link when too many events
//Create events when clicking
select: function (start, end) {
var title = prompt('Event Title:');
var eventData;
if (title) {
eventData = {
title: title,
start: start,
end: end
};
$('#calendar').fullCalendar('renderEvent', eventData, true); // stick? = true
}
$('#calendar').fullCalendar('unselect');
},
// Traz os eventos do Banco de Dados
eventSources: [
{
url: '../WebService1.asmx/ListarTarefas',
type: 'POST',
error: function () {
alert('Ocorreu um erro ao retornar as Tarefas. Por favor, entre em contato conosco.');
console.log(MediaError)
},
color: 'yellow', // a non-ajax option
textColor: 'black' // a non-ajax option
}
]
});
});
</script>
I'm not a professional in Javascript (actually this is my first big project with JS). I tried some solutions I've found here on StackOverflow but couldn't make it work.
This is the JSON that the WEB Method return:
<string xmlns="http://tempuri.org/">
[{"Id":3,"Title":"Teste Tarefa 1","Start":"2018-11-13T00:00:00","End":"2018-11-13T00:00:00"},{"Id":4,"Title":"Teste Tarefa 2","Start":"2018-11-13T00:00:00","End":"2018-11-13T00:00:00"}]
</string>
javascript c# asp.net-mvc fullcalendar fullcalendar-3
javascript c# asp.net-mvc fullcalendar fullcalendar-3
asked Nov 21 '18 at 12:50
M. Robert
11
11
"This is the JSON"...problem is, it appears to be JSON wrapped in XML, for some reason.
– ADyson
Nov 22 '18 at 9:55
I think this is because you're using a legacy ASMX web service which returns XML by default. They have not been the standard thing to use for about 10 years now. Any reason you used that instead of a WCF webservice or Web API? If you want to stick with ASMX I think you can maybe change the configuration somewhere to stop it wrapping the XML, you'd have to google it though, it's been years since I worked with ASMX.
– ADyson
Nov 22 '18 at 10:01
I've changed succefully and now it returns just the JSON. But the Calendar still doesn't load the event.
– M. Robert
Nov 22 '18 at 13:13
I believe the property names in the JSON need to be lower case, likeevents: [{ "id": 3, "title": "Teste Tarefa 1", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }, { "id": 4, "title": "Teste Tarefa 2", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }]
Demo: Not working, Working
– ADyson
Nov 22 '18 at 13:31
It worked, I changed to lower and is showing now. Thank You !
– M. Robert
Nov 22 '18 at 13:52
add a comment |
"This is the JSON"...problem is, it appears to be JSON wrapped in XML, for some reason.
– ADyson
Nov 22 '18 at 9:55
I think this is because you're using a legacy ASMX web service which returns XML by default. They have not been the standard thing to use for about 10 years now. Any reason you used that instead of a WCF webservice or Web API? If you want to stick with ASMX I think you can maybe change the configuration somewhere to stop it wrapping the XML, you'd have to google it though, it's been years since I worked with ASMX.
– ADyson
Nov 22 '18 at 10:01
I've changed succefully and now it returns just the JSON. But the Calendar still doesn't load the event.
– M. Robert
Nov 22 '18 at 13:13
I believe the property names in the JSON need to be lower case, likeevents: [{ "id": 3, "title": "Teste Tarefa 1", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }, { "id": 4, "title": "Teste Tarefa 2", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }]
Demo: Not working, Working
– ADyson
Nov 22 '18 at 13:31
It worked, I changed to lower and is showing now. Thank You !
– M. Robert
Nov 22 '18 at 13:52
"This is the JSON"...problem is, it appears to be JSON wrapped in XML, for some reason.
– ADyson
Nov 22 '18 at 9:55
"This is the JSON"...problem is, it appears to be JSON wrapped in XML, for some reason.
– ADyson
Nov 22 '18 at 9:55
I think this is because you're using a legacy ASMX web service which returns XML by default. They have not been the standard thing to use for about 10 years now. Any reason you used that instead of a WCF webservice or Web API? If you want to stick with ASMX I think you can maybe change the configuration somewhere to stop it wrapping the XML, you'd have to google it though, it's been years since I worked with ASMX.
– ADyson
Nov 22 '18 at 10:01
I think this is because you're using a legacy ASMX web service which returns XML by default. They have not been the standard thing to use for about 10 years now. Any reason you used that instead of a WCF webservice or Web API? If you want to stick with ASMX I think you can maybe change the configuration somewhere to stop it wrapping the XML, you'd have to google it though, it's been years since I worked with ASMX.
– ADyson
Nov 22 '18 at 10:01
I've changed succefully and now it returns just the JSON. But the Calendar still doesn't load the event.
– M. Robert
Nov 22 '18 at 13:13
I've changed succefully and now it returns just the JSON. But the Calendar still doesn't load the event.
– M. Robert
Nov 22 '18 at 13:13
I believe the property names in the JSON need to be lower case, like
events: [{ "id": 3, "title": "Teste Tarefa 1", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }, { "id": 4, "title": "Teste Tarefa 2", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }]
Demo: Not working, Working– ADyson
Nov 22 '18 at 13:31
I believe the property names in the JSON need to be lower case, like
events: [{ "id": 3, "title": "Teste Tarefa 1", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }, { "id": 4, "title": "Teste Tarefa 2", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }]
Demo: Not working, Working– ADyson
Nov 22 '18 at 13:31
It worked, I changed to lower and is showing now. Thank You !
– M. Robert
Nov 22 '18 at 13:52
It worked, I changed to lower and is showing now. Thank You !
– M. Robert
Nov 22 '18 at 13:52
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53412435%2ftake-events-from-database-to-fullcalendar%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53412435%2ftake-events-from-database-to-fullcalendar%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6Zld 9tQr OnOAazh99c1Tzz8Ib,B,mZetTT4LgBrGrA,8sMFG3cF
"This is the JSON"...problem is, it appears to be JSON wrapped in XML, for some reason.
– ADyson
Nov 22 '18 at 9:55
I think this is because you're using a legacy ASMX web service which returns XML by default. They have not been the standard thing to use for about 10 years now. Any reason you used that instead of a WCF webservice or Web API? If you want to stick with ASMX I think you can maybe change the configuration somewhere to stop it wrapping the XML, you'd have to google it though, it's been years since I worked with ASMX.
– ADyson
Nov 22 '18 at 10:01
I've changed succefully and now it returns just the JSON. But the Calendar still doesn't load the event.
– M. Robert
Nov 22 '18 at 13:13
I believe the property names in the JSON need to be lower case, like
events: [{ "id": 3, "title": "Teste Tarefa 1", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }, { "id": 4, "title": "Teste Tarefa 2", "start": "2018-11-13T00:00:00", "end": "2018-11-13T00:00:00" }]
Demo: Not working, Working– ADyson
Nov 22 '18 at 13:31
It worked, I changed to lower and is showing now. Thank You !
– M. Robert
Nov 22 '18 at 13:52