MochaJS: Rejected promises not triggering a failed test
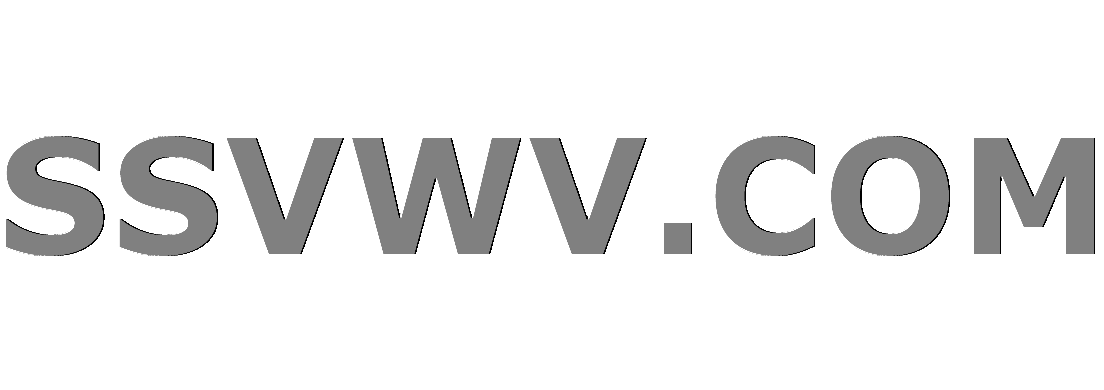
Multi tool use
I'm using async-await syntax with Mocha and Supertest. This is my problematic test:
it('Test POST/friends route: should add a friendship', async function () {
const lastFriendship = await models.Friendship.findAll({limit: 1,where: {},order: [ [ 'createdAt', 'DESC' ]]})
const lastFriendId = lastFriendship[0].id
await request(app)
.post('/friends')
.set('Authorization', token)
.send({ friendId: 998 })
.expect(200)
.expect(async (res) => {
console.log('res.data', res.body.data.id)
const newFriendId = res.body.data.id
expect(res.body.data.friendId).toEqual(998)
expect(res.body).toHaveProperty('error', null);
expect(newFriendId - lastFriendId).toBe(2)//This should fail the test.
})
})
The last assertion,as it's written now, should fail the test, but instead i receive this error:
UnhandledPromiseRejectionWarning: Error:
expect(received).toBe(expected) // Object.is equality
I tried putting the entire thing in try-catch block, with no success. I'm obviously missing something about the mechanics here.
What is wrong with my code?
EDIT: removing the "async" from the callback function of expect, solves it, though i don't know why throwing an exception in a callback doesn't get caught here.
node.js async-await mocha supertest
add a comment |
I'm using async-await syntax with Mocha and Supertest. This is my problematic test:
it('Test POST/friends route: should add a friendship', async function () {
const lastFriendship = await models.Friendship.findAll({limit: 1,where: {},order: [ [ 'createdAt', 'DESC' ]]})
const lastFriendId = lastFriendship[0].id
await request(app)
.post('/friends')
.set('Authorization', token)
.send({ friendId: 998 })
.expect(200)
.expect(async (res) => {
console.log('res.data', res.body.data.id)
const newFriendId = res.body.data.id
expect(res.body.data.friendId).toEqual(998)
expect(res.body).toHaveProperty('error', null);
expect(newFriendId - lastFriendId).toBe(2)//This should fail the test.
})
})
The last assertion,as it's written now, should fail the test, but instead i receive this error:
UnhandledPromiseRejectionWarning: Error:
expect(received).toBe(expected) // Object.is equality
I tried putting the entire thing in try-catch block, with no success. I'm obviously missing something about the mechanics here.
What is wrong with my code?
EDIT: removing the "async" from the callback function of expect, solves it, though i don't know why throwing an exception in a callback doesn't get caught here.
node.js async-await mocha supertest
add a comment |
I'm using async-await syntax with Mocha and Supertest. This is my problematic test:
it('Test POST/friends route: should add a friendship', async function () {
const lastFriendship = await models.Friendship.findAll({limit: 1,where: {},order: [ [ 'createdAt', 'DESC' ]]})
const lastFriendId = lastFriendship[0].id
await request(app)
.post('/friends')
.set('Authorization', token)
.send({ friendId: 998 })
.expect(200)
.expect(async (res) => {
console.log('res.data', res.body.data.id)
const newFriendId = res.body.data.id
expect(res.body.data.friendId).toEqual(998)
expect(res.body).toHaveProperty('error', null);
expect(newFriendId - lastFriendId).toBe(2)//This should fail the test.
})
})
The last assertion,as it's written now, should fail the test, but instead i receive this error:
UnhandledPromiseRejectionWarning: Error:
expect(received).toBe(expected) // Object.is equality
I tried putting the entire thing in try-catch block, with no success. I'm obviously missing something about the mechanics here.
What is wrong with my code?
EDIT: removing the "async" from the callback function of expect, solves it, though i don't know why throwing an exception in a callback doesn't get caught here.
node.js async-await mocha supertest
I'm using async-await syntax with Mocha and Supertest. This is my problematic test:
it('Test POST/friends route: should add a friendship', async function () {
const lastFriendship = await models.Friendship.findAll({limit: 1,where: {},order: [ [ 'createdAt', 'DESC' ]]})
const lastFriendId = lastFriendship[0].id
await request(app)
.post('/friends')
.set('Authorization', token)
.send({ friendId: 998 })
.expect(200)
.expect(async (res) => {
console.log('res.data', res.body.data.id)
const newFriendId = res.body.data.id
expect(res.body.data.friendId).toEqual(998)
expect(res.body).toHaveProperty('error', null);
expect(newFriendId - lastFriendId).toBe(2)//This should fail the test.
})
})
The last assertion,as it's written now, should fail the test, but instead i receive this error:
UnhandledPromiseRejectionWarning: Error:
expect(received).toBe(expected) // Object.is equality
I tried putting the entire thing in try-catch block, with no success. I'm obviously missing something about the mechanics here.
What is wrong with my code?
EDIT: removing the "async" from the callback function of expect, solves it, though i don't know why throwing an exception in a callback doesn't get caught here.
node.js async-await mocha supertest
node.js async-await mocha supertest
edited Nov 20 at 19:50
asked Nov 20 at 18:50
sheff2k1
366210
366210
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I think you need to parse your res.body as JSON.
Nah, the problem is that im using an async function as the expect callback. Once i removed it - it works. But i do not have the knowledge/understanding as to why it happens.
– sheff2k1
Nov 20 at 19:49
I use async functions in mocha. They're fine. I don't see how you can do an await without an async in the function signature.
– Jeff Lowery
Nov 20 at 19:54
Yes, but i'm talkign about the callback to the last expect call. I do not use "await" there. Anyway, removing the async from it solves it, which implies that i do not really understand how exceptions and promises work :D
– sheff2k1
Nov 20 at 20:02
Yes, I have a similar problem. Just when I think I know how they work, one bites me.
– Jeff Lowery
Nov 20 at 20:12
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53399622%2fmochajs-rejected-promises-not-triggering-a-failed-test%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think you need to parse your res.body as JSON.
Nah, the problem is that im using an async function as the expect callback. Once i removed it - it works. But i do not have the knowledge/understanding as to why it happens.
– sheff2k1
Nov 20 at 19:49
I use async functions in mocha. They're fine. I don't see how you can do an await without an async in the function signature.
– Jeff Lowery
Nov 20 at 19:54
Yes, but i'm talkign about the callback to the last expect call. I do not use "await" there. Anyway, removing the async from it solves it, which implies that i do not really understand how exceptions and promises work :D
– sheff2k1
Nov 20 at 20:02
Yes, I have a similar problem. Just when I think I know how they work, one bites me.
– Jeff Lowery
Nov 20 at 20:12
add a comment |
I think you need to parse your res.body as JSON.
Nah, the problem is that im using an async function as the expect callback. Once i removed it - it works. But i do not have the knowledge/understanding as to why it happens.
– sheff2k1
Nov 20 at 19:49
I use async functions in mocha. They're fine. I don't see how you can do an await without an async in the function signature.
– Jeff Lowery
Nov 20 at 19:54
Yes, but i'm talkign about the callback to the last expect call. I do not use "await" there. Anyway, removing the async from it solves it, which implies that i do not really understand how exceptions and promises work :D
– sheff2k1
Nov 20 at 20:02
Yes, I have a similar problem. Just when I think I know how they work, one bites me.
– Jeff Lowery
Nov 20 at 20:12
add a comment |
I think you need to parse your res.body as JSON.
I think you need to parse your res.body as JSON.
answered Nov 20 at 19:46
Jeff Lowery
1,0411527
1,0411527
Nah, the problem is that im using an async function as the expect callback. Once i removed it - it works. But i do not have the knowledge/understanding as to why it happens.
– sheff2k1
Nov 20 at 19:49
I use async functions in mocha. They're fine. I don't see how you can do an await without an async in the function signature.
– Jeff Lowery
Nov 20 at 19:54
Yes, but i'm talkign about the callback to the last expect call. I do not use "await" there. Anyway, removing the async from it solves it, which implies that i do not really understand how exceptions and promises work :D
– sheff2k1
Nov 20 at 20:02
Yes, I have a similar problem. Just when I think I know how they work, one bites me.
– Jeff Lowery
Nov 20 at 20:12
add a comment |
Nah, the problem is that im using an async function as the expect callback. Once i removed it - it works. But i do not have the knowledge/understanding as to why it happens.
– sheff2k1
Nov 20 at 19:49
I use async functions in mocha. They're fine. I don't see how you can do an await without an async in the function signature.
– Jeff Lowery
Nov 20 at 19:54
Yes, but i'm talkign about the callback to the last expect call. I do not use "await" there. Anyway, removing the async from it solves it, which implies that i do not really understand how exceptions and promises work :D
– sheff2k1
Nov 20 at 20:02
Yes, I have a similar problem. Just when I think I know how they work, one bites me.
– Jeff Lowery
Nov 20 at 20:12
Nah, the problem is that im using an async function as the expect callback. Once i removed it - it works. But i do not have the knowledge/understanding as to why it happens.
– sheff2k1
Nov 20 at 19:49
Nah, the problem is that im using an async function as the expect callback. Once i removed it - it works. But i do not have the knowledge/understanding as to why it happens.
– sheff2k1
Nov 20 at 19:49
I use async functions in mocha. They're fine. I don't see how you can do an await without an async in the function signature.
– Jeff Lowery
Nov 20 at 19:54
I use async functions in mocha. They're fine. I don't see how you can do an await without an async in the function signature.
– Jeff Lowery
Nov 20 at 19:54
Yes, but i'm talkign about the callback to the last expect call. I do not use "await" there. Anyway, removing the async from it solves it, which implies that i do not really understand how exceptions and promises work :D
– sheff2k1
Nov 20 at 20:02
Yes, but i'm talkign about the callback to the last expect call. I do not use "await" there. Anyway, removing the async from it solves it, which implies that i do not really understand how exceptions and promises work :D
– sheff2k1
Nov 20 at 20:02
Yes, I have a similar problem. Just when I think I know how they work, one bites me.
– Jeff Lowery
Nov 20 at 20:12
Yes, I have a similar problem. Just when I think I know how they work, one bites me.
– Jeff Lowery
Nov 20 at 20:12
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53399622%2fmochajs-rejected-promises-not-triggering-a-failed-test%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7zPCwbOJBkHQHu,RczBSyKJ6YL4El