String Compression
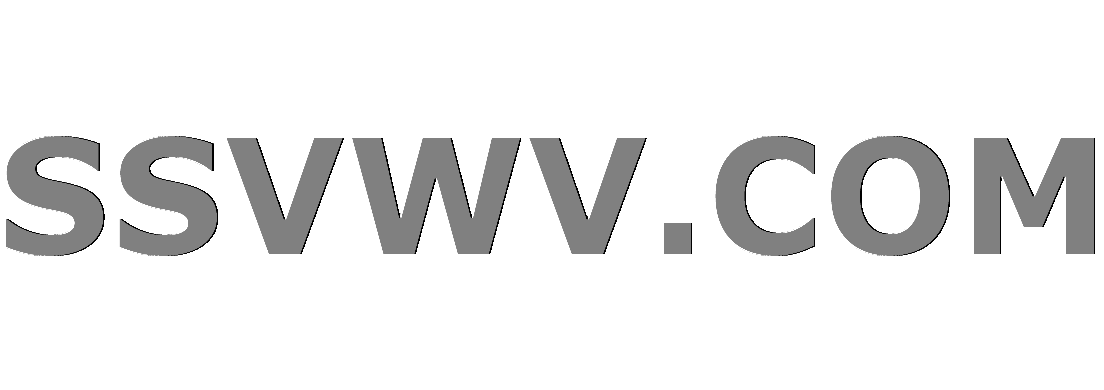
Multi tool use
up vote
1
down vote
favorite
Implement a method to perform basic string compression using the counts
of repeated characters.
For example, the string "aabcccccaaa" would become "a2b1c5a3". If the
"compressed" string would not become smaller than the original string, your method should return
the original string. You can assume the string has only uppercase and lowercase letters (a - z).
My solution is:
GitHub
public class StringCompression {
public static String compress(String input) {
if (input == null) {
return null;
}
StringBuilder output = new StringBuilder();
char prevChar = input.charAt(0);
int lengthSoFar = 1;
for (int index = 1; index < input.length(); index++) {
char currentChar = input.charAt(index);
if (prevChar == currentChar) {
lengthSoFar++;
} else {
output.append(prevChar);
output.append(lengthSoFar);
// reset counters
prevChar = currentChar;
lengthSoFar = 1;
}
}
output.append(prevChar);
output.append(lengthSoFar);
return output.toString().length() <= input.length() ? output.toString() : input;
}
}
java algorithm programming-challenge interview-questions compression
add a comment |
up vote
1
down vote
favorite
Implement a method to perform basic string compression using the counts
of repeated characters.
For example, the string "aabcccccaaa" would become "a2b1c5a3". If the
"compressed" string would not become smaller than the original string, your method should return
the original string. You can assume the string has only uppercase and lowercase letters (a - z).
My solution is:
GitHub
public class StringCompression {
public static String compress(String input) {
if (input == null) {
return null;
}
StringBuilder output = new StringBuilder();
char prevChar = input.charAt(0);
int lengthSoFar = 1;
for (int index = 1; index < input.length(); index++) {
char currentChar = input.charAt(index);
if (prevChar == currentChar) {
lengthSoFar++;
} else {
output.append(prevChar);
output.append(lengthSoFar);
// reset counters
prevChar = currentChar;
lengthSoFar = 1;
}
}
output.append(prevChar);
output.append(lengthSoFar);
return output.toString().length() <= input.length() ? output.toString() : input;
}
}
java algorithm programming-challenge interview-questions compression
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
Implement a method to perform basic string compression using the counts
of repeated characters.
For example, the string "aabcccccaaa" would become "a2b1c5a3". If the
"compressed" string would not become smaller than the original string, your method should return
the original string. You can assume the string has only uppercase and lowercase letters (a - z).
My solution is:
GitHub
public class StringCompression {
public static String compress(String input) {
if (input == null) {
return null;
}
StringBuilder output = new StringBuilder();
char prevChar = input.charAt(0);
int lengthSoFar = 1;
for (int index = 1; index < input.length(); index++) {
char currentChar = input.charAt(index);
if (prevChar == currentChar) {
lengthSoFar++;
} else {
output.append(prevChar);
output.append(lengthSoFar);
// reset counters
prevChar = currentChar;
lengthSoFar = 1;
}
}
output.append(prevChar);
output.append(lengthSoFar);
return output.toString().length() <= input.length() ? output.toString() : input;
}
}
java algorithm programming-challenge interview-questions compression
Implement a method to perform basic string compression using the counts
of repeated characters.
For example, the string "aabcccccaaa" would become "a2b1c5a3". If the
"compressed" string would not become smaller than the original string, your method should return
the original string. You can assume the string has only uppercase and lowercase letters (a - z).
My solution is:
GitHub
public class StringCompression {
public static String compress(String input) {
if (input == null) {
return null;
}
StringBuilder output = new StringBuilder();
char prevChar = input.charAt(0);
int lengthSoFar = 1;
for (int index = 1; index < input.length(); index++) {
char currentChar = input.charAt(index);
if (prevChar == currentChar) {
lengthSoFar++;
} else {
output.append(prevChar);
output.append(lengthSoFar);
// reset counters
prevChar = currentChar;
lengthSoFar = 1;
}
}
output.append(prevChar);
output.append(lengthSoFar);
return output.toString().length() <= input.length() ? output.toString() : input;
}
}
java algorithm programming-challenge interview-questions compression
java algorithm programming-challenge interview-questions compression
asked 7 hours ago
Exploring
21013
21013
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
For input "fooo", the implementation returns "f1o3",
which is the same length, and violates one of the requirements:
If the "compressed" string would not become smaller than the original string, your method should return the original string.
The fix is in the return
statement: change <=
to <
.
Another corner case is when the input is empty, the statement char prevChar = input.charAt(0);
will throw StringIndexOutOfBoundsException
.
Lastly, a performance pitfall in the return statement,
output.toString()
creates a new string,
therefore the following may result in double computation:
return output.toString().length() < input.length() ? output.toString() : input;
You could instead benefit from the length()
method of StringBuilder
:
return output.length() < input.length() ? output.toString() : input;
add a comment |
up vote
0
down vote
Generates an out of bounds exception if passed an empty (but not null
) string.
The new StringBuilder()
should be passed an initial size for the character buffer, to avoid continuous reallocations. Lacking any other reasonable value, I’d recommend passing in the original string length.
StringBuilder output = new StringBuilder(input.length());
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
For input "fooo", the implementation returns "f1o3",
which is the same length, and violates one of the requirements:
If the "compressed" string would not become smaller than the original string, your method should return the original string.
The fix is in the return
statement: change <=
to <
.
Another corner case is when the input is empty, the statement char prevChar = input.charAt(0);
will throw StringIndexOutOfBoundsException
.
Lastly, a performance pitfall in the return statement,
output.toString()
creates a new string,
therefore the following may result in double computation:
return output.toString().length() < input.length() ? output.toString() : input;
You could instead benefit from the length()
method of StringBuilder
:
return output.length() < input.length() ? output.toString() : input;
add a comment |
up vote
2
down vote
For input "fooo", the implementation returns "f1o3",
which is the same length, and violates one of the requirements:
If the "compressed" string would not become smaller than the original string, your method should return the original string.
The fix is in the return
statement: change <=
to <
.
Another corner case is when the input is empty, the statement char prevChar = input.charAt(0);
will throw StringIndexOutOfBoundsException
.
Lastly, a performance pitfall in the return statement,
output.toString()
creates a new string,
therefore the following may result in double computation:
return output.toString().length() < input.length() ? output.toString() : input;
You could instead benefit from the length()
method of StringBuilder
:
return output.length() < input.length() ? output.toString() : input;
add a comment |
up vote
2
down vote
up vote
2
down vote
For input "fooo", the implementation returns "f1o3",
which is the same length, and violates one of the requirements:
If the "compressed" string would not become smaller than the original string, your method should return the original string.
The fix is in the return
statement: change <=
to <
.
Another corner case is when the input is empty, the statement char prevChar = input.charAt(0);
will throw StringIndexOutOfBoundsException
.
Lastly, a performance pitfall in the return statement,
output.toString()
creates a new string,
therefore the following may result in double computation:
return output.toString().length() < input.length() ? output.toString() : input;
You could instead benefit from the length()
method of StringBuilder
:
return output.length() < input.length() ? output.toString() : input;
For input "fooo", the implementation returns "f1o3",
which is the same length, and violates one of the requirements:
If the "compressed" string would not become smaller than the original string, your method should return the original string.
The fix is in the return
statement: change <=
to <
.
Another corner case is when the input is empty, the statement char prevChar = input.charAt(0);
will throw StringIndexOutOfBoundsException
.
Lastly, a performance pitfall in the return statement,
output.toString()
creates a new string,
therefore the following may result in double computation:
return output.toString().length() < input.length() ? output.toString() : input;
You could instead benefit from the length()
method of StringBuilder
:
return output.length() < input.length() ? output.toString() : input;
answered 6 hours ago


janos
96.5k12122349
96.5k12122349
add a comment |
add a comment |
up vote
0
down vote
Generates an out of bounds exception if passed an empty (but not null
) string.
The new StringBuilder()
should be passed an initial size for the character buffer, to avoid continuous reallocations. Lacking any other reasonable value, I’d recommend passing in the original string length.
StringBuilder output = new StringBuilder(input.length());
add a comment |
up vote
0
down vote
Generates an out of bounds exception if passed an empty (but not null
) string.
The new StringBuilder()
should be passed an initial size for the character buffer, to avoid continuous reallocations. Lacking any other reasonable value, I’d recommend passing in the original string length.
StringBuilder output = new StringBuilder(input.length());
add a comment |
up vote
0
down vote
up vote
0
down vote
Generates an out of bounds exception if passed an empty (but not null
) string.
The new StringBuilder()
should be passed an initial size for the character buffer, to avoid continuous reallocations. Lacking any other reasonable value, I’d recommend passing in the original string length.
StringBuilder output = new StringBuilder(input.length());
Generates an out of bounds exception if passed an empty (but not null
) string.
The new StringBuilder()
should be passed an initial size for the character buffer, to avoid continuous reallocations. Lacking any other reasonable value, I’d recommend passing in the original string length.
StringBuilder output = new StringBuilder(input.length());
answered 6 hours ago
AJNeufeld
3,585317
3,585317
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f208089%2fstring-compression%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xY2nZVxGxVnS9 11 ZvG9CGLdpBlfvL,s Mz vx5E