jar file built with maven can't find configuration file
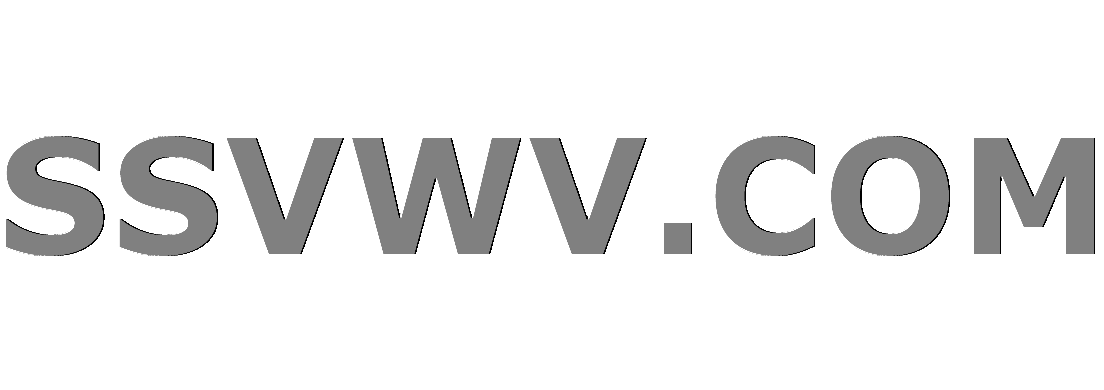
Multi tool use
up vote
-1
down vote
favorite
I built a jar file with maven. I am using the following code in the only class in the jar file to try to access the config file.
InputStream is = Myclass.class.getClassLoader().getResourceAsStream("/config.properties");
The config.properties file is in the jar file located at Path /
I don't see any error messages. But when I try to access the values in the Properties object, the values are null.
Properties prop = new Properties();
prop.load(is);
serverUrl=prop.getProperty("serverUrl");
serverUrl is null.
Any suggestions on how to get the values in the config file in the jar file?
java maven jar
add a comment |
up vote
-1
down vote
favorite
I built a jar file with maven. I am using the following code in the only class in the jar file to try to access the config file.
InputStream is = Myclass.class.getClassLoader().getResourceAsStream("/config.properties");
The config.properties file is in the jar file located at Path /
I don't see any error messages. But when I try to access the values in the Properties object, the values are null.
Properties prop = new Properties();
prop.load(is);
serverUrl=prop.getProperty("serverUrl");
serverUrl is null.
Any suggestions on how to get the values in the config file in the jar file?
java maven jar
you should add the error that you are getting
– Nawnit Sen
Nov 16 at 7:43
TryMyclass.class.getClassLoader().getResourceAsStream("config.properties")
or put your config in same package asMyClass
.
– talex
Nov 16 at 7:51
no error message that I can see, but when I try to access the values, there is a null value or a null pointer exception
– user840930
Nov 16 at 8:37
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I built a jar file with maven. I am using the following code in the only class in the jar file to try to access the config file.
InputStream is = Myclass.class.getClassLoader().getResourceAsStream("/config.properties");
The config.properties file is in the jar file located at Path /
I don't see any error messages. But when I try to access the values in the Properties object, the values are null.
Properties prop = new Properties();
prop.load(is);
serverUrl=prop.getProperty("serverUrl");
serverUrl is null.
Any suggestions on how to get the values in the config file in the jar file?
java maven jar
I built a jar file with maven. I am using the following code in the only class in the jar file to try to access the config file.
InputStream is = Myclass.class.getClassLoader().getResourceAsStream("/config.properties");
The config.properties file is in the jar file located at Path /
I don't see any error messages. But when I try to access the values in the Properties object, the values are null.
Properties prop = new Properties();
prop.load(is);
serverUrl=prop.getProperty("serverUrl");
serverUrl is null.
Any suggestions on how to get the values in the config file in the jar file?
java maven jar
java maven jar
edited Nov 16 at 9:33
asked Nov 16 at 7:39
user840930
1,336144163
1,336144163
you should add the error that you are getting
– Nawnit Sen
Nov 16 at 7:43
TryMyclass.class.getClassLoader().getResourceAsStream("config.properties")
or put your config in same package asMyClass
.
– talex
Nov 16 at 7:51
no error message that I can see, but when I try to access the values, there is a null value or a null pointer exception
– user840930
Nov 16 at 8:37
add a comment |
you should add the error that you are getting
– Nawnit Sen
Nov 16 at 7:43
TryMyclass.class.getClassLoader().getResourceAsStream("config.properties")
or put your config in same package asMyClass
.
– talex
Nov 16 at 7:51
no error message that I can see, but when I try to access the values, there is a null value or a null pointer exception
– user840930
Nov 16 at 8:37
you should add the error that you are getting
– Nawnit Sen
Nov 16 at 7:43
you should add the error that you are getting
– Nawnit Sen
Nov 16 at 7:43
Try
Myclass.class.getClassLoader().getResourceAsStream("config.properties")
or put your config in same package as MyClass
.– talex
Nov 16 at 7:51
Try
Myclass.class.getClassLoader().getResourceAsStream("config.properties")
or put your config in same package as MyClass
.– talex
Nov 16 at 7:51
no error message that I can see, but when I try to access the values, there is a null value or a null pointer exception
– user840930
Nov 16 at 8:37
no error message that I can see, but when I try to access the values, there is a null value or a null pointer exception
– user840930
Nov 16 at 8:37
add a comment |
3 Answers
3
active
oldest
votes
up vote
0
down vote
Typically in maven, you locate resources under src/main/resources.
Then you can access these resources by using the classloader:
try (InputStream in = Myclass.class.getClassLoader().getResourceAsStream("config.properties") {
//doSomething
}
New contributor
Tobias Bertram-Köhler is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Thank you for your comment! I updated my question and code to reflect the change. So my code appears to access the file but the values are null.
– user840930
Nov 16 at 9:09
Or at least I should say InputStream is no longer null
– user840930
Nov 16 at 11:59
add a comment |
up vote
0
down vote
I was facing same issue before I realized that the following way is only good for loading files in your classpath.
InputStream is = Myclass.class.getClassLoader().getResourceAsStream("/config.properties");
For picking files regardless of whether they are in classpath or not, I use Apache FileUtils. For e.g I used following and it worked like a charm.
String str = FileUtils.readFileToString(new File(path_to_your_file), "UTF-8");
IOUtils.toInputStream(str, "UTF-8"); // if you need InputStream object
You can have a look at other APIs provided by FileUtils too other than readFileToString
add a comment |
up vote
0
down vote
getResourceAsStream("config.properties")
- this method will only work if your properties file is in the classpath. I think you have added the properties file outside the classpath. This method is basically used for accessing a file present in the classpath.
Please check it again.
Thanks :)
Working Code :
public class MyClass {
public static void main(String args) throws Exception {
Properties prop = new Properties();
InputStream input = null;
try {
String filename = "config.properties";
input = MyClass.class.getClassLoader().getResourceAsStream(filename);
if (input == null) {
System.out.println("Sorry, unable to find " + filename);
return;
}
// load a properties file from class path, inside static method
prop.load(input);
// get the property value and print it out
System.out.println(prop.getProperty("serverUrl"));
} catch (IOException ex) {
ex.printStackTrace();
} finally {
if (input != null) {
try {
input.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
how do I add the file to the classpath?
– user840930
Nov 16 at 8:29
1
I'm pretty sure the file is being found now. I changed the code to getResourceAsStream("/config.properties");
– user840930
Nov 16 at 10:00
keep the properties file in the resources folder of your project.. Then if you are using eclipse. Then right click on the project -> Properties ->Java Build Path -> click on source tab -> Click on Add folder and add the resources folder -> Click on Apply -> Click on Apply and close. Make a little modification to getResourceAsStream("resources/config.properties")
– Anish B.
Nov 16 at 10:04
tried "resources/config.properties. That did not work. Can't access the file. NullPointerException. The correct way looks like "/config.properties"
– user840930
Nov 16 at 11:06
then its fine :)
– Anish B.
Nov 16 at 12:39
|
show 2 more comments
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Typically in maven, you locate resources under src/main/resources.
Then you can access these resources by using the classloader:
try (InputStream in = Myclass.class.getClassLoader().getResourceAsStream("config.properties") {
//doSomething
}
New contributor
Tobias Bertram-Köhler is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Thank you for your comment! I updated my question and code to reflect the change. So my code appears to access the file but the values are null.
– user840930
Nov 16 at 9:09
Or at least I should say InputStream is no longer null
– user840930
Nov 16 at 11:59
add a comment |
up vote
0
down vote
Typically in maven, you locate resources under src/main/resources.
Then you can access these resources by using the classloader:
try (InputStream in = Myclass.class.getClassLoader().getResourceAsStream("config.properties") {
//doSomething
}
New contributor
Tobias Bertram-Köhler is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Thank you for your comment! I updated my question and code to reflect the change. So my code appears to access the file but the values are null.
– user840930
Nov 16 at 9:09
Or at least I should say InputStream is no longer null
– user840930
Nov 16 at 11:59
add a comment |
up vote
0
down vote
up vote
0
down vote
Typically in maven, you locate resources under src/main/resources.
Then you can access these resources by using the classloader:
try (InputStream in = Myclass.class.getClassLoader().getResourceAsStream("config.properties") {
//doSomething
}
New contributor
Tobias Bertram-Köhler is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Typically in maven, you locate resources under src/main/resources.
Then you can access these resources by using the classloader:
try (InputStream in = Myclass.class.getClassLoader().getResourceAsStream("config.properties") {
//doSomething
}
New contributor
Tobias Bertram-Köhler is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Tobias Bertram-Köhler is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered Nov 16 at 7:57


Tobias Bertram-Köhler
32
32
New contributor
Tobias Bertram-Köhler is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Tobias Bertram-Köhler is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Tobias Bertram-Köhler is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Thank you for your comment! I updated my question and code to reflect the change. So my code appears to access the file but the values are null.
– user840930
Nov 16 at 9:09
Or at least I should say InputStream is no longer null
– user840930
Nov 16 at 11:59
add a comment |
Thank you for your comment! I updated my question and code to reflect the change. So my code appears to access the file but the values are null.
– user840930
Nov 16 at 9:09
Or at least I should say InputStream is no longer null
– user840930
Nov 16 at 11:59
Thank you for your comment! I updated my question and code to reflect the change. So my code appears to access the file but the values are null.
– user840930
Nov 16 at 9:09
Thank you for your comment! I updated my question and code to reflect the change. So my code appears to access the file but the values are null.
– user840930
Nov 16 at 9:09
Or at least I should say InputStream is no longer null
– user840930
Nov 16 at 11:59
Or at least I should say InputStream is no longer null
– user840930
Nov 16 at 11:59
add a comment |
up vote
0
down vote
I was facing same issue before I realized that the following way is only good for loading files in your classpath.
InputStream is = Myclass.class.getClassLoader().getResourceAsStream("/config.properties");
For picking files regardless of whether they are in classpath or not, I use Apache FileUtils. For e.g I used following and it worked like a charm.
String str = FileUtils.readFileToString(new File(path_to_your_file), "UTF-8");
IOUtils.toInputStream(str, "UTF-8"); // if you need InputStream object
You can have a look at other APIs provided by FileUtils too other than readFileToString
add a comment |
up vote
0
down vote
I was facing same issue before I realized that the following way is only good for loading files in your classpath.
InputStream is = Myclass.class.getClassLoader().getResourceAsStream("/config.properties");
For picking files regardless of whether they are in classpath or not, I use Apache FileUtils. For e.g I used following and it worked like a charm.
String str = FileUtils.readFileToString(new File(path_to_your_file), "UTF-8");
IOUtils.toInputStream(str, "UTF-8"); // if you need InputStream object
You can have a look at other APIs provided by FileUtils too other than readFileToString
add a comment |
up vote
0
down vote
up vote
0
down vote
I was facing same issue before I realized that the following way is only good for loading files in your classpath.
InputStream is = Myclass.class.getClassLoader().getResourceAsStream("/config.properties");
For picking files regardless of whether they are in classpath or not, I use Apache FileUtils. For e.g I used following and it worked like a charm.
String str = FileUtils.readFileToString(new File(path_to_your_file), "UTF-8");
IOUtils.toInputStream(str, "UTF-8"); // if you need InputStream object
You can have a look at other APIs provided by FileUtils too other than readFileToString
I was facing same issue before I realized that the following way is only good for loading files in your classpath.
InputStream is = Myclass.class.getClassLoader().getResourceAsStream("/config.properties");
For picking files regardless of whether they are in classpath or not, I use Apache FileUtils. For e.g I used following and it worked like a charm.
String str = FileUtils.readFileToString(new File(path_to_your_file), "UTF-8");
IOUtils.toInputStream(str, "UTF-8"); // if you need InputStream object
You can have a look at other APIs provided by FileUtils too other than readFileToString
answered yesterday


tryingToLearn
2,33922546
2,33922546
add a comment |
add a comment |
up vote
0
down vote
getResourceAsStream("config.properties")
- this method will only work if your properties file is in the classpath. I think you have added the properties file outside the classpath. This method is basically used for accessing a file present in the classpath.
Please check it again.
Thanks :)
Working Code :
public class MyClass {
public static void main(String args) throws Exception {
Properties prop = new Properties();
InputStream input = null;
try {
String filename = "config.properties";
input = MyClass.class.getClassLoader().getResourceAsStream(filename);
if (input == null) {
System.out.println("Sorry, unable to find " + filename);
return;
}
// load a properties file from class path, inside static method
prop.load(input);
// get the property value and print it out
System.out.println(prop.getProperty("serverUrl"));
} catch (IOException ex) {
ex.printStackTrace();
} finally {
if (input != null) {
try {
input.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
how do I add the file to the classpath?
– user840930
Nov 16 at 8:29
1
I'm pretty sure the file is being found now. I changed the code to getResourceAsStream("/config.properties");
– user840930
Nov 16 at 10:00
keep the properties file in the resources folder of your project.. Then if you are using eclipse. Then right click on the project -> Properties ->Java Build Path -> click on source tab -> Click on Add folder and add the resources folder -> Click on Apply -> Click on Apply and close. Make a little modification to getResourceAsStream("resources/config.properties")
– Anish B.
Nov 16 at 10:04
tried "resources/config.properties. That did not work. Can't access the file. NullPointerException. The correct way looks like "/config.properties"
– user840930
Nov 16 at 11:06
then its fine :)
– Anish B.
Nov 16 at 12:39
|
show 2 more comments
up vote
0
down vote
getResourceAsStream("config.properties")
- this method will only work if your properties file is in the classpath. I think you have added the properties file outside the classpath. This method is basically used for accessing a file present in the classpath.
Please check it again.
Thanks :)
Working Code :
public class MyClass {
public static void main(String args) throws Exception {
Properties prop = new Properties();
InputStream input = null;
try {
String filename = "config.properties";
input = MyClass.class.getClassLoader().getResourceAsStream(filename);
if (input == null) {
System.out.println("Sorry, unable to find " + filename);
return;
}
// load a properties file from class path, inside static method
prop.load(input);
// get the property value and print it out
System.out.println(prop.getProperty("serverUrl"));
} catch (IOException ex) {
ex.printStackTrace();
} finally {
if (input != null) {
try {
input.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
how do I add the file to the classpath?
– user840930
Nov 16 at 8:29
1
I'm pretty sure the file is being found now. I changed the code to getResourceAsStream("/config.properties");
– user840930
Nov 16 at 10:00
keep the properties file in the resources folder of your project.. Then if you are using eclipse. Then right click on the project -> Properties ->Java Build Path -> click on source tab -> Click on Add folder and add the resources folder -> Click on Apply -> Click on Apply and close. Make a little modification to getResourceAsStream("resources/config.properties")
– Anish B.
Nov 16 at 10:04
tried "resources/config.properties. That did not work. Can't access the file. NullPointerException. The correct way looks like "/config.properties"
– user840930
Nov 16 at 11:06
then its fine :)
– Anish B.
Nov 16 at 12:39
|
show 2 more comments
up vote
0
down vote
up vote
0
down vote
getResourceAsStream("config.properties")
- this method will only work if your properties file is in the classpath. I think you have added the properties file outside the classpath. This method is basically used for accessing a file present in the classpath.
Please check it again.
Thanks :)
Working Code :
public class MyClass {
public static void main(String args) throws Exception {
Properties prop = new Properties();
InputStream input = null;
try {
String filename = "config.properties";
input = MyClass.class.getClassLoader().getResourceAsStream(filename);
if (input == null) {
System.out.println("Sorry, unable to find " + filename);
return;
}
// load a properties file from class path, inside static method
prop.load(input);
// get the property value and print it out
System.out.println(prop.getProperty("serverUrl"));
} catch (IOException ex) {
ex.printStackTrace();
} finally {
if (input != null) {
try {
input.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
getResourceAsStream("config.properties")
- this method will only work if your properties file is in the classpath. I think you have added the properties file outside the classpath. This method is basically used for accessing a file present in the classpath.
Please check it again.
Thanks :)
Working Code :
public class MyClass {
public static void main(String args) throws Exception {
Properties prop = new Properties();
InputStream input = null;
try {
String filename = "config.properties";
input = MyClass.class.getClassLoader().getResourceAsStream(filename);
if (input == null) {
System.out.println("Sorry, unable to find " + filename);
return;
}
// load a properties file from class path, inside static method
prop.load(input);
// get the property value and print it out
System.out.println(prop.getProperty("serverUrl"));
} catch (IOException ex) {
ex.printStackTrace();
} finally {
if (input != null) {
try {
input.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
edited yesterday
answered Nov 16 at 7:56


Anish B.
168
168
how do I add the file to the classpath?
– user840930
Nov 16 at 8:29
1
I'm pretty sure the file is being found now. I changed the code to getResourceAsStream("/config.properties");
– user840930
Nov 16 at 10:00
keep the properties file in the resources folder of your project.. Then if you are using eclipse. Then right click on the project -> Properties ->Java Build Path -> click on source tab -> Click on Add folder and add the resources folder -> Click on Apply -> Click on Apply and close. Make a little modification to getResourceAsStream("resources/config.properties")
– Anish B.
Nov 16 at 10:04
tried "resources/config.properties. That did not work. Can't access the file. NullPointerException. The correct way looks like "/config.properties"
– user840930
Nov 16 at 11:06
then its fine :)
– Anish B.
Nov 16 at 12:39
|
show 2 more comments
how do I add the file to the classpath?
– user840930
Nov 16 at 8:29
1
I'm pretty sure the file is being found now. I changed the code to getResourceAsStream("/config.properties");
– user840930
Nov 16 at 10:00
keep the properties file in the resources folder of your project.. Then if you are using eclipse. Then right click on the project -> Properties ->Java Build Path -> click on source tab -> Click on Add folder and add the resources folder -> Click on Apply -> Click on Apply and close. Make a little modification to getResourceAsStream("resources/config.properties")
– Anish B.
Nov 16 at 10:04
tried "resources/config.properties. That did not work. Can't access the file. NullPointerException. The correct way looks like "/config.properties"
– user840930
Nov 16 at 11:06
then its fine :)
– Anish B.
Nov 16 at 12:39
how do I add the file to the classpath?
– user840930
Nov 16 at 8:29
how do I add the file to the classpath?
– user840930
Nov 16 at 8:29
1
1
I'm pretty sure the file is being found now. I changed the code to getResourceAsStream("/config.properties");
– user840930
Nov 16 at 10:00
I'm pretty sure the file is being found now. I changed the code to getResourceAsStream("/config.properties");
– user840930
Nov 16 at 10:00
keep the properties file in the resources folder of your project.. Then if you are using eclipse. Then right click on the project -> Properties ->Java Build Path -> click on source tab -> Click on Add folder and add the resources folder -> Click on Apply -> Click on Apply and close. Make a little modification to getResourceAsStream("resources/config.properties")
– Anish B.
Nov 16 at 10:04
keep the properties file in the resources folder of your project.. Then if you are using eclipse. Then right click on the project -> Properties ->Java Build Path -> click on source tab -> Click on Add folder and add the resources folder -> Click on Apply -> Click on Apply and close. Make a little modification to getResourceAsStream("resources/config.properties")
– Anish B.
Nov 16 at 10:04
tried "resources/config.properties. That did not work. Can't access the file. NullPointerException. The correct way looks like "/config.properties"
– user840930
Nov 16 at 11:06
tried "resources/config.properties. That did not work. Can't access the file. NullPointerException. The correct way looks like "/config.properties"
– user840930
Nov 16 at 11:06
then its fine :)
– Anish B.
Nov 16 at 12:39
then its fine :)
– Anish B.
Nov 16 at 12:39
|
show 2 more comments
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53333386%2fjar-file-built-with-maven-cant-find-configuration-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
v7,i 3q03zqlcEqNo hN3NiSS,tM,8x16o mLw,I
you should add the error that you are getting
– Nawnit Sen
Nov 16 at 7:43
Try
Myclass.class.getClassLoader().getResourceAsStream("config.properties")
or put your config in same package asMyClass
.– talex
Nov 16 at 7:51
no error message that I can see, but when I try to access the values, there is a null value or a null pointer exception
– user840930
Nov 16 at 8:37