Rotating an Image using own algorithm in python
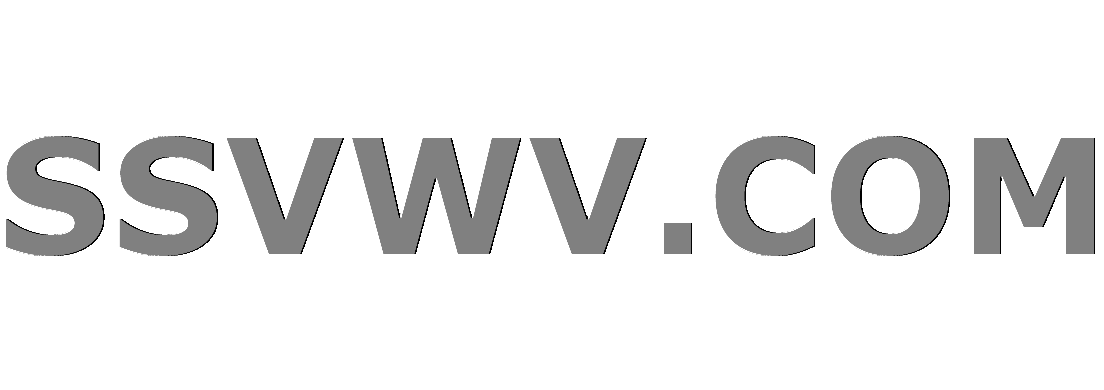
Multi tool use
up vote
4
down vote
favorite
For an uni assignment I have been giving the task of making my own rotating algorithm in python. This is my code so far.
import cv2
import math
import numpy as np
class rotator:
angle = 20.0
x = 330
y = 330
radians = float(angle*(math.pi/180))
img = cv2.imread('FNAF.png',0)
width,height = img.shape
def showImg(name, self):
cv2.imshow(name, self.img)
self.img = np.pad(self.img, (self.height) ,'constant', constant_values=0)
self.width,self.height = self.img.shape
def printWH(self):
print(self.width)
print(self.height)
def rotate(self):
emptyF = np.zeros((self.width,self.height),dtype="uint8")
emptyB = np.zeros((self.width,self.height),dtype="uint8")
emptyBB = np.zeros((self.width,self.height),dtype="uint8")
for i in range(self.width):
for j in range(self.height):
temp = self.img[i,j]
#forward mapping
xf = (i-self.x)*math.cos(self.radians)-(j-self.y)*math.sin(self.radians)+self.x
yf = (i-self.x)*math.sin(self.radians)+(j-self.y)*math.cos(self.radians)+self.x
#backward mapping should change the forward mapping to the original image
xbb = (i-self.x)*math.cos(self.radians)+(j-self.y)*math.sin(self.radians)+self.x
ybb = -(i-self.x)*math.sin(self.radians)+(j-self.y)*math.cos(self.radians)+self.x
xbb = int(xbb)
ybb = int(ybb)
if xf < 660 and yf < 660 and xf>0 and yf > 0:
emptyF[int(xf),int(yf)] = temp
else:
pass
if xbb < 660 and ybb < 660 and xbb>0 and ybb > 0:
emptyBB[(xbb),(ybb)] = temp
else:
pass
cv2.imshow('Forward', emptyF)
cv2.imshow('Backward', emptyBB)
def main():
rotator.showImg('normal', rotator)
rotator.printWH(rotator)
rotator.rotate(rotator)
cv2.waitKey(0)
cv2.destroyAllWindows
if __name__ == '__main__':
main()
Is there any way to improve this code or my algorithm? Any advise would be awesome.
NB. I also hav a problem with my backwards mapping. The output image has a lot of small black dots. Any suggestions to what that might be?
Please let me know.
python algorithm image numpy opencv
New contributor
Zorn01 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
4
down vote
favorite
For an uni assignment I have been giving the task of making my own rotating algorithm in python. This is my code so far.
import cv2
import math
import numpy as np
class rotator:
angle = 20.0
x = 330
y = 330
radians = float(angle*(math.pi/180))
img = cv2.imread('FNAF.png',0)
width,height = img.shape
def showImg(name, self):
cv2.imshow(name, self.img)
self.img = np.pad(self.img, (self.height) ,'constant', constant_values=0)
self.width,self.height = self.img.shape
def printWH(self):
print(self.width)
print(self.height)
def rotate(self):
emptyF = np.zeros((self.width,self.height),dtype="uint8")
emptyB = np.zeros((self.width,self.height),dtype="uint8")
emptyBB = np.zeros((self.width,self.height),dtype="uint8")
for i in range(self.width):
for j in range(self.height):
temp = self.img[i,j]
#forward mapping
xf = (i-self.x)*math.cos(self.radians)-(j-self.y)*math.sin(self.radians)+self.x
yf = (i-self.x)*math.sin(self.radians)+(j-self.y)*math.cos(self.radians)+self.x
#backward mapping should change the forward mapping to the original image
xbb = (i-self.x)*math.cos(self.radians)+(j-self.y)*math.sin(self.radians)+self.x
ybb = -(i-self.x)*math.sin(self.radians)+(j-self.y)*math.cos(self.radians)+self.x
xbb = int(xbb)
ybb = int(ybb)
if xf < 660 and yf < 660 and xf>0 and yf > 0:
emptyF[int(xf),int(yf)] = temp
else:
pass
if xbb < 660 and ybb < 660 and xbb>0 and ybb > 0:
emptyBB[(xbb),(ybb)] = temp
else:
pass
cv2.imshow('Forward', emptyF)
cv2.imshow('Backward', emptyBB)
def main():
rotator.showImg('normal', rotator)
rotator.printWH(rotator)
rotator.rotate(rotator)
cv2.waitKey(0)
cv2.destroyAllWindows
if __name__ == '__main__':
main()
Is there any way to improve this code or my algorithm? Any advise would be awesome.
NB. I also hav a problem with my backwards mapping. The output image has a lot of small black dots. Any suggestions to what that might be?
Please let me know.
python algorithm image numpy opencv
New contributor
Zorn01 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
I suspect that the black dots might be due to integer rounding of trigonometric results.
– 200_success
6 hours ago
add a comment |
up vote
4
down vote
favorite
up vote
4
down vote
favorite
For an uni assignment I have been giving the task of making my own rotating algorithm in python. This is my code so far.
import cv2
import math
import numpy as np
class rotator:
angle = 20.0
x = 330
y = 330
radians = float(angle*(math.pi/180))
img = cv2.imread('FNAF.png',0)
width,height = img.shape
def showImg(name, self):
cv2.imshow(name, self.img)
self.img = np.pad(self.img, (self.height) ,'constant', constant_values=0)
self.width,self.height = self.img.shape
def printWH(self):
print(self.width)
print(self.height)
def rotate(self):
emptyF = np.zeros((self.width,self.height),dtype="uint8")
emptyB = np.zeros((self.width,self.height),dtype="uint8")
emptyBB = np.zeros((self.width,self.height),dtype="uint8")
for i in range(self.width):
for j in range(self.height):
temp = self.img[i,j]
#forward mapping
xf = (i-self.x)*math.cos(self.radians)-(j-self.y)*math.sin(self.radians)+self.x
yf = (i-self.x)*math.sin(self.radians)+(j-self.y)*math.cos(self.radians)+self.x
#backward mapping should change the forward mapping to the original image
xbb = (i-self.x)*math.cos(self.radians)+(j-self.y)*math.sin(self.radians)+self.x
ybb = -(i-self.x)*math.sin(self.radians)+(j-self.y)*math.cos(self.radians)+self.x
xbb = int(xbb)
ybb = int(ybb)
if xf < 660 and yf < 660 and xf>0 and yf > 0:
emptyF[int(xf),int(yf)] = temp
else:
pass
if xbb < 660 and ybb < 660 and xbb>0 and ybb > 0:
emptyBB[(xbb),(ybb)] = temp
else:
pass
cv2.imshow('Forward', emptyF)
cv2.imshow('Backward', emptyBB)
def main():
rotator.showImg('normal', rotator)
rotator.printWH(rotator)
rotator.rotate(rotator)
cv2.waitKey(0)
cv2.destroyAllWindows
if __name__ == '__main__':
main()
Is there any way to improve this code or my algorithm? Any advise would be awesome.
NB. I also hav a problem with my backwards mapping. The output image has a lot of small black dots. Any suggestions to what that might be?
Please let me know.
python algorithm image numpy opencv
New contributor
Zorn01 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
For an uni assignment I have been giving the task of making my own rotating algorithm in python. This is my code so far.
import cv2
import math
import numpy as np
class rotator:
angle = 20.0
x = 330
y = 330
radians = float(angle*(math.pi/180))
img = cv2.imread('FNAF.png',0)
width,height = img.shape
def showImg(name, self):
cv2.imshow(name, self.img)
self.img = np.pad(self.img, (self.height) ,'constant', constant_values=0)
self.width,self.height = self.img.shape
def printWH(self):
print(self.width)
print(self.height)
def rotate(self):
emptyF = np.zeros((self.width,self.height),dtype="uint8")
emptyB = np.zeros((self.width,self.height),dtype="uint8")
emptyBB = np.zeros((self.width,self.height),dtype="uint8")
for i in range(self.width):
for j in range(self.height):
temp = self.img[i,j]
#forward mapping
xf = (i-self.x)*math.cos(self.radians)-(j-self.y)*math.sin(self.radians)+self.x
yf = (i-self.x)*math.sin(self.radians)+(j-self.y)*math.cos(self.radians)+self.x
#backward mapping should change the forward mapping to the original image
xbb = (i-self.x)*math.cos(self.radians)+(j-self.y)*math.sin(self.radians)+self.x
ybb = -(i-self.x)*math.sin(self.radians)+(j-self.y)*math.cos(self.radians)+self.x
xbb = int(xbb)
ybb = int(ybb)
if xf < 660 and yf < 660 and xf>0 and yf > 0:
emptyF[int(xf),int(yf)] = temp
else:
pass
if xbb < 660 and ybb < 660 and xbb>0 and ybb > 0:
emptyBB[(xbb),(ybb)] = temp
else:
pass
cv2.imshow('Forward', emptyF)
cv2.imshow('Backward', emptyBB)
def main():
rotator.showImg('normal', rotator)
rotator.printWH(rotator)
rotator.rotate(rotator)
cv2.waitKey(0)
cv2.destroyAllWindows
if __name__ == '__main__':
main()
Is there any way to improve this code or my algorithm? Any advise would be awesome.
NB. I also hav a problem with my backwards mapping. The output image has a lot of small black dots. Any suggestions to what that might be?
Please let me know.
python algorithm image numpy opencv
python algorithm image numpy opencv
New contributor
Zorn01 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Zorn01 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Zorn01 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 6 hours ago
Zorn01
211
211
New contributor
Zorn01 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Zorn01 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Zorn01 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
I suspect that the black dots might be due to integer rounding of trigonometric results.
– 200_success
6 hours ago
add a comment |
1
I suspect that the black dots might be due to integer rounding of trigonometric results.
– 200_success
6 hours ago
1
1
I suspect that the black dots might be due to integer rounding of trigonometric results.
– 200_success
6 hours ago
I suspect that the black dots might be due to integer rounding of trigonometric results.
– 200_success
6 hours ago
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
The most common method for getting rid of the black dots is to paint over each pixel of the entire destination image with pixels copied from computed positions in the source image, instead of painting the source image pixels into computed positions in the destination image.
for i in range(660):
for j in range(660):
xb = int(...)
yb = int(...)
if xb in range(self.width) and yb in range(self.height):
emptyF[i, j] = self.img[xb, yb]
Computing destination pixels positions from the source pixel positions may result in tiny areas unpainted in the destination due to numeric errors/limits.
Computing source pixels positions from the destination pixel position may result in a pixel value being copied to two adjacent locations, and other pixels never being copied from, but this is usually/often unnoticeable.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
The most common method for getting rid of the black dots is to paint over each pixel of the entire destination image with pixels copied from computed positions in the source image, instead of painting the source image pixels into computed positions in the destination image.
for i in range(660):
for j in range(660):
xb = int(...)
yb = int(...)
if xb in range(self.width) and yb in range(self.height):
emptyF[i, j] = self.img[xb, yb]
Computing destination pixels positions from the source pixel positions may result in tiny areas unpainted in the destination due to numeric errors/limits.
Computing source pixels positions from the destination pixel position may result in a pixel value being copied to two adjacent locations, and other pixels never being copied from, but this is usually/often unnoticeable.
add a comment |
up vote
0
down vote
The most common method for getting rid of the black dots is to paint over each pixel of the entire destination image with pixels copied from computed positions in the source image, instead of painting the source image pixels into computed positions in the destination image.
for i in range(660):
for j in range(660):
xb = int(...)
yb = int(...)
if xb in range(self.width) and yb in range(self.height):
emptyF[i, j] = self.img[xb, yb]
Computing destination pixels positions from the source pixel positions may result in tiny areas unpainted in the destination due to numeric errors/limits.
Computing source pixels positions from the destination pixel position may result in a pixel value being copied to two adjacent locations, and other pixels never being copied from, but this is usually/often unnoticeable.
add a comment |
up vote
0
down vote
up vote
0
down vote
The most common method for getting rid of the black dots is to paint over each pixel of the entire destination image with pixels copied from computed positions in the source image, instead of painting the source image pixels into computed positions in the destination image.
for i in range(660):
for j in range(660):
xb = int(...)
yb = int(...)
if xb in range(self.width) and yb in range(self.height):
emptyF[i, j] = self.img[xb, yb]
Computing destination pixels positions from the source pixel positions may result in tiny areas unpainted in the destination due to numeric errors/limits.
Computing source pixels positions from the destination pixel position may result in a pixel value being copied to two adjacent locations, and other pixels never being copied from, but this is usually/often unnoticeable.
The most common method for getting rid of the black dots is to paint over each pixel of the entire destination image with pixels copied from computed positions in the source image, instead of painting the source image pixels into computed positions in the destination image.
for i in range(660):
for j in range(660):
xb = int(...)
yb = int(...)
if xb in range(self.width) and yb in range(self.height):
emptyF[i, j] = self.img[xb, yb]
Computing destination pixels positions from the source pixel positions may result in tiny areas unpainted in the destination due to numeric errors/limits.
Computing source pixels positions from the destination pixel position may result in a pixel value being copied to two adjacent locations, and other pixels never being copied from, but this is usually/often unnoticeable.
answered 2 hours ago
AJNeufeld
3,595317
3,595317
add a comment |
add a comment |
Zorn01 is a new contributor. Be nice, and check out our Code of Conduct.
Zorn01 is a new contributor. Be nice, and check out our Code of Conduct.
Zorn01 is a new contributor. Be nice, and check out our Code of Conduct.
Zorn01 is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f208101%2frotating-an-image-using-own-algorithm-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IzzQ 8KyKXnIQMzmVJLm Zt4DxUD3HqTs
1
I suspect that the black dots might be due to integer rounding of trigonometric results.
– 200_success
6 hours ago