c++, how do I copy a vector into a vector of vectors?
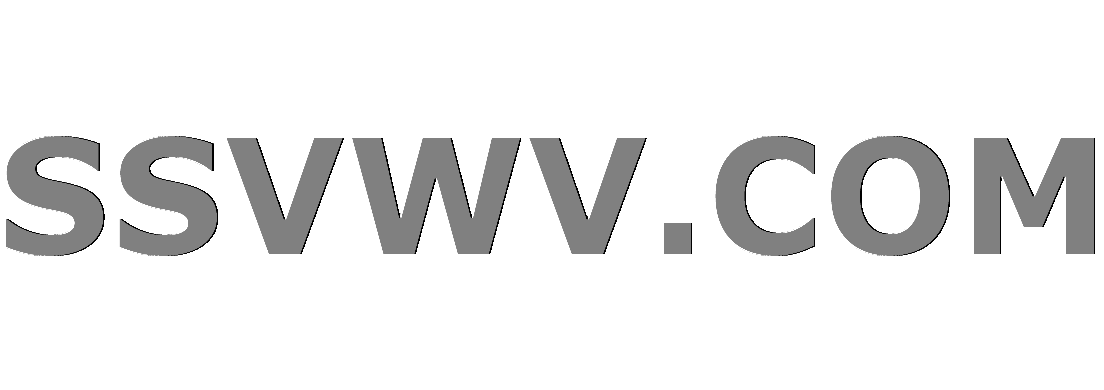
Multi tool use
up vote
1
down vote
favorite
I figured the following code would work, Here's an abridged version of my code:
#include <iostream>
#include <vector>
int main()
{
int xCoordMovement, yCoordMovement;
int rows, cols;
char point = '*';
std::cout << "enter number of rows";
std::cin >> rows;
cols = rows;
std::vector<std::vector<char>> grid(rows, std::vector<char>(cols, '0'));
std::vector<char> flattenedGrid;
for (int x = 0; x < rows; x++)
{
for (int y = 0; y < cols; y++)
std::cout << grid[x][y];
std::cout << std::endl;
}
std::cout << "input for x coord: ";
std::cin >> xCoordMovement;
std::cout << "input for y coord: ";
std::cin >> yCoordMovement;
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
flattenedGrid.push_back(grid[x][y]);
flattenedGrid[((cols * yCoordMovement) - (rows - xCoordMovement)) - 1] = point;
std::cout << flattenedGrid.size() << std::endl;
for(int i = 0; i < flattenedGrid.size(); i++)
std::cout << flattenedGrid[i];
std::cout << std::endl;
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
for (int x = 0; x < rows; x++)
{
for (int y = 0; y < cols; y++)
std::cout << grid[x][y];
std::cout << std::endl;
}
std::cin.ignore();
std::cin.get();
return 0;
}
However it does not seem to change the values of the grid, it should now have a value that is a star at one of it's coordinates, but alas, all grid contains is it's original values.
relevant output:
00000
00000
00000
00000
00000
desired output:
00000
000*0
00000
00000
00000
Here is the bit I was hoping would assign the values of my vector into my vector of vectors:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
c++ vector copy
add a comment |
up vote
1
down vote
favorite
I figured the following code would work, Here's an abridged version of my code:
#include <iostream>
#include <vector>
int main()
{
int xCoordMovement, yCoordMovement;
int rows, cols;
char point = '*';
std::cout << "enter number of rows";
std::cin >> rows;
cols = rows;
std::vector<std::vector<char>> grid(rows, std::vector<char>(cols, '0'));
std::vector<char> flattenedGrid;
for (int x = 0; x < rows; x++)
{
for (int y = 0; y < cols; y++)
std::cout << grid[x][y];
std::cout << std::endl;
}
std::cout << "input for x coord: ";
std::cin >> xCoordMovement;
std::cout << "input for y coord: ";
std::cin >> yCoordMovement;
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
flattenedGrid.push_back(grid[x][y]);
flattenedGrid[((cols * yCoordMovement) - (rows - xCoordMovement)) - 1] = point;
std::cout << flattenedGrid.size() << std::endl;
for(int i = 0; i < flattenedGrid.size(); i++)
std::cout << flattenedGrid[i];
std::cout << std::endl;
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
for (int x = 0; x < rows; x++)
{
for (int y = 0; y < cols; y++)
std::cout << grid[x][y];
std::cout << std::endl;
}
std::cin.ignore();
std::cin.get();
return 0;
}
However it does not seem to change the values of the grid, it should now have a value that is a star at one of it's coordinates, but alas, all grid contains is it's original values.
relevant output:
00000
00000
00000
00000
00000
desired output:
00000
000*0
00000
00000
00000
Here is the bit I was hoping would assign the values of my vector into my vector of vectors:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
c++ vector copy
Welcome to StackOverflow. Be sure to read about the Minimal, Complete, Verifiable Example. If at all possible, your code should have a main() and be standalone compilable, such as in an online compiler. Anything that's not central to your question should be removed. You should be able to show the output that you get, and explain the output that you wanted. (Saying things like "it should now have a value that's a star at one of it's coordinates" when there is no*
in your code isn't actionable.)
– HostileFork
2 days ago
grid[x][y] = flattenedGrid[y]
is just overwriting every row with the firstcols
entries offlattenedGrid
. Also, your linear index((cols * yCoordMovement) - (rows - xCoordMovement)) - 1
looks very suspicious -- you should check that it's calculating the value you expect. It's not clear why you need to flatten the whole grid just to do this one operation. Why not set the value directly intogrid
?
– paddy
2 days ago
I put up my whole program. I tried figuring out how to user cin coordinates for x and y, essentially allowing them to pick points on a coordinate plane. I could not think of a way of doing it without first flattening the grid and adjusting the index for the entered xCoord and yCoord.
– Ben Jenney
2 days ago
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I figured the following code would work, Here's an abridged version of my code:
#include <iostream>
#include <vector>
int main()
{
int xCoordMovement, yCoordMovement;
int rows, cols;
char point = '*';
std::cout << "enter number of rows";
std::cin >> rows;
cols = rows;
std::vector<std::vector<char>> grid(rows, std::vector<char>(cols, '0'));
std::vector<char> flattenedGrid;
for (int x = 0; x < rows; x++)
{
for (int y = 0; y < cols; y++)
std::cout << grid[x][y];
std::cout << std::endl;
}
std::cout << "input for x coord: ";
std::cin >> xCoordMovement;
std::cout << "input for y coord: ";
std::cin >> yCoordMovement;
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
flattenedGrid.push_back(grid[x][y]);
flattenedGrid[((cols * yCoordMovement) - (rows - xCoordMovement)) - 1] = point;
std::cout << flattenedGrid.size() << std::endl;
for(int i = 0; i < flattenedGrid.size(); i++)
std::cout << flattenedGrid[i];
std::cout << std::endl;
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
for (int x = 0; x < rows; x++)
{
for (int y = 0; y < cols; y++)
std::cout << grid[x][y];
std::cout << std::endl;
}
std::cin.ignore();
std::cin.get();
return 0;
}
However it does not seem to change the values of the grid, it should now have a value that is a star at one of it's coordinates, but alas, all grid contains is it's original values.
relevant output:
00000
00000
00000
00000
00000
desired output:
00000
000*0
00000
00000
00000
Here is the bit I was hoping would assign the values of my vector into my vector of vectors:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
c++ vector copy
I figured the following code would work, Here's an abridged version of my code:
#include <iostream>
#include <vector>
int main()
{
int xCoordMovement, yCoordMovement;
int rows, cols;
char point = '*';
std::cout << "enter number of rows";
std::cin >> rows;
cols = rows;
std::vector<std::vector<char>> grid(rows, std::vector<char>(cols, '0'));
std::vector<char> flattenedGrid;
for (int x = 0; x < rows; x++)
{
for (int y = 0; y < cols; y++)
std::cout << grid[x][y];
std::cout << std::endl;
}
std::cout << "input for x coord: ";
std::cin >> xCoordMovement;
std::cout << "input for y coord: ";
std::cin >> yCoordMovement;
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
flattenedGrid.push_back(grid[x][y]);
flattenedGrid[((cols * yCoordMovement) - (rows - xCoordMovement)) - 1] = point;
std::cout << flattenedGrid.size() << std::endl;
for(int i = 0; i < flattenedGrid.size(); i++)
std::cout << flattenedGrid[i];
std::cout << std::endl;
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
for (int x = 0; x < rows; x++)
{
for (int y = 0; y < cols; y++)
std::cout << grid[x][y];
std::cout << std::endl;
}
std::cin.ignore();
std::cin.get();
return 0;
}
However it does not seem to change the values of the grid, it should now have a value that is a star at one of it's coordinates, but alas, all grid contains is it's original values.
relevant output:
00000
00000
00000
00000
00000
desired output:
00000
000*0
00000
00000
00000
Here is the bit I was hoping would assign the values of my vector into my vector of vectors:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
c++ vector copy
c++ vector copy
edited yesterday
Shrikanth N
504210
504210
asked 2 days ago


Ben Jenney
154
154
Welcome to StackOverflow. Be sure to read about the Minimal, Complete, Verifiable Example. If at all possible, your code should have a main() and be standalone compilable, such as in an online compiler. Anything that's not central to your question should be removed. You should be able to show the output that you get, and explain the output that you wanted. (Saying things like "it should now have a value that's a star at one of it's coordinates" when there is no*
in your code isn't actionable.)
– HostileFork
2 days ago
grid[x][y] = flattenedGrid[y]
is just overwriting every row with the firstcols
entries offlattenedGrid
. Also, your linear index((cols * yCoordMovement) - (rows - xCoordMovement)) - 1
looks very suspicious -- you should check that it's calculating the value you expect. It's not clear why you need to flatten the whole grid just to do this one operation. Why not set the value directly intogrid
?
– paddy
2 days ago
I put up my whole program. I tried figuring out how to user cin coordinates for x and y, essentially allowing them to pick points on a coordinate plane. I could not think of a way of doing it without first flattening the grid and adjusting the index for the entered xCoord and yCoord.
– Ben Jenney
2 days ago
add a comment |
Welcome to StackOverflow. Be sure to read about the Minimal, Complete, Verifiable Example. If at all possible, your code should have a main() and be standalone compilable, such as in an online compiler. Anything that's not central to your question should be removed. You should be able to show the output that you get, and explain the output that you wanted. (Saying things like "it should now have a value that's a star at one of it's coordinates" when there is no*
in your code isn't actionable.)
– HostileFork
2 days ago
grid[x][y] = flattenedGrid[y]
is just overwriting every row with the firstcols
entries offlattenedGrid
. Also, your linear index((cols * yCoordMovement) - (rows - xCoordMovement)) - 1
looks very suspicious -- you should check that it's calculating the value you expect. It's not clear why you need to flatten the whole grid just to do this one operation. Why not set the value directly intogrid
?
– paddy
2 days ago
I put up my whole program. I tried figuring out how to user cin coordinates for x and y, essentially allowing them to pick points on a coordinate plane. I could not think of a way of doing it without first flattening the grid and adjusting the index for the entered xCoord and yCoord.
– Ben Jenney
2 days ago
Welcome to StackOverflow. Be sure to read about the Minimal, Complete, Verifiable Example. If at all possible, your code should have a main() and be standalone compilable, such as in an online compiler. Anything that's not central to your question should be removed. You should be able to show the output that you get, and explain the output that you wanted. (Saying things like "it should now have a value that's a star at one of it's coordinates" when there is no
*
in your code isn't actionable.)– HostileFork
2 days ago
Welcome to StackOverflow. Be sure to read about the Minimal, Complete, Verifiable Example. If at all possible, your code should have a main() and be standalone compilable, such as in an online compiler. Anything that's not central to your question should be removed. You should be able to show the output that you get, and explain the output that you wanted. (Saying things like "it should now have a value that's a star at one of it's coordinates" when there is no
*
in your code isn't actionable.)– HostileFork
2 days ago
grid[x][y] = flattenedGrid[y]
is just overwriting every row with the first cols
entries of flattenedGrid
. Also, your linear index ((cols * yCoordMovement) - (rows - xCoordMovement)) - 1
looks very suspicious -- you should check that it's calculating the value you expect. It's not clear why you need to flatten the whole grid just to do this one operation. Why not set the value directly into grid
?– paddy
2 days ago
grid[x][y] = flattenedGrid[y]
is just overwriting every row with the first cols
entries of flattenedGrid
. Also, your linear index ((cols * yCoordMovement) - (rows - xCoordMovement)) - 1
looks very suspicious -- you should check that it's calculating the value you expect. It's not clear why you need to flatten the whole grid just to do this one operation. Why not set the value directly into grid
?– paddy
2 days ago
I put up my whole program. I tried figuring out how to user cin coordinates for x and y, essentially allowing them to pick points on a coordinate plane. I could not think of a way of doing it without first flattening the grid and adjusting the index for the entered xCoord and yCoord.
– Ben Jenney
2 days ago
I put up my whole program. I tried figuring out how to user cin coordinates for x and y, essentially allowing them to pick points on a coordinate plane. I could not think of a way of doing it without first flattening the grid and adjusting the index for the entered xCoord and yCoord.
– Ben Jenney
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
There are a couple of changes you have to make to the code:
- First, when you assign
point
to an element offlattenedGrid
Change:
flattenedGrid[((cols * yCoordMovement) - (rows - xCoordMovement)) - 1] = point;
to:
flattenedGrid[(yCoordMovement) + (rows * xCoordMovement)] = point;
- Second, when you refill
grid
with elements offlattenedGrid
Change:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
to:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
grid[x][y] = flattenedGrid[rows * x + y];
Thank you! this is definitely superior to what I had.
– Ben Jenney
22 hours ago
@BenJenney: You are welcome. :)
– P.W
22 hours ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
There are a couple of changes you have to make to the code:
- First, when you assign
point
to an element offlattenedGrid
Change:
flattenedGrid[((cols * yCoordMovement) - (rows - xCoordMovement)) - 1] = point;
to:
flattenedGrid[(yCoordMovement) + (rows * xCoordMovement)] = point;
- Second, when you refill
grid
with elements offlattenedGrid
Change:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
to:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
grid[x][y] = flattenedGrid[rows * x + y];
Thank you! this is definitely superior to what I had.
– Ben Jenney
22 hours ago
@BenJenney: You are welcome. :)
– P.W
22 hours ago
add a comment |
up vote
0
down vote
accepted
There are a couple of changes you have to make to the code:
- First, when you assign
point
to an element offlattenedGrid
Change:
flattenedGrid[((cols * yCoordMovement) - (rows - xCoordMovement)) - 1] = point;
to:
flattenedGrid[(yCoordMovement) + (rows * xCoordMovement)] = point;
- Second, when you refill
grid
with elements offlattenedGrid
Change:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
to:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
grid[x][y] = flattenedGrid[rows * x + y];
Thank you! this is definitely superior to what I had.
– Ben Jenney
22 hours ago
@BenJenney: You are welcome. :)
– P.W
22 hours ago
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
There are a couple of changes you have to make to the code:
- First, when you assign
point
to an element offlattenedGrid
Change:
flattenedGrid[((cols * yCoordMovement) - (rows - xCoordMovement)) - 1] = point;
to:
flattenedGrid[(yCoordMovement) + (rows * xCoordMovement)] = point;
- Second, when you refill
grid
with elements offlattenedGrid
Change:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
to:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
grid[x][y] = flattenedGrid[rows * x + y];
There are a couple of changes you have to make to the code:
- First, when you assign
point
to an element offlattenedGrid
Change:
flattenedGrid[((cols * yCoordMovement) - (rows - xCoordMovement)) - 1] = point;
to:
flattenedGrid[(yCoordMovement) + (rows * xCoordMovement)] = point;
- Second, when you refill
grid
with elements offlattenedGrid
Change:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
for (int i = 0; i < flattenedGrid.size(); i++)
grid[x][y] = flattenedGrid[i];
to:
for (int x = 0; x < rows; x++)
for (int y = 0; y < cols; y++)
grid[x][y] = flattenedGrid[rows * x + y];
edited yesterday
answered 2 days ago
P.W
7,6602438
7,6602438
Thank you! this is definitely superior to what I had.
– Ben Jenney
22 hours ago
@BenJenney: You are welcome. :)
– P.W
22 hours ago
add a comment |
Thank you! this is definitely superior to what I had.
– Ben Jenney
22 hours ago
@BenJenney: You are welcome. :)
– P.W
22 hours ago
Thank you! this is definitely superior to what I had.
– Ben Jenney
22 hours ago
Thank you! this is definitely superior to what I had.
– Ben Jenney
22 hours ago
@BenJenney: You are welcome. :)
– P.W
22 hours ago
@BenJenney: You are welcome. :)
– P.W
22 hours ago
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53367282%2fc-how-do-i-copy-a-vector-into-a-vector-of-vectors%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KTgOmxwaXM1TkfA47,ABzdG Fw FW4YVoiyAWl4,uvZdoox1UMh
Welcome to StackOverflow. Be sure to read about the Minimal, Complete, Verifiable Example. If at all possible, your code should have a main() and be standalone compilable, such as in an online compiler. Anything that's not central to your question should be removed. You should be able to show the output that you get, and explain the output that you wanted. (Saying things like "it should now have a value that's a star at one of it's coordinates" when there is no
*
in your code isn't actionable.)– HostileFork
2 days ago
grid[x][y] = flattenedGrid[y]
is just overwriting every row with the firstcols
entries offlattenedGrid
. Also, your linear index((cols * yCoordMovement) - (rows - xCoordMovement)) - 1
looks very suspicious -- you should check that it's calculating the value you expect. It's not clear why you need to flatten the whole grid just to do this one operation. Why not set the value directly intogrid
?– paddy
2 days ago
I put up my whole program. I tried figuring out how to user cin coordinates for x and y, essentially allowing them to pick points on a coordinate plane. I could not think of a way of doing it without first flattening the grid and adjusting the index for the entered xCoord and yCoord.
– Ben Jenney
2 days ago