PHP machine learning
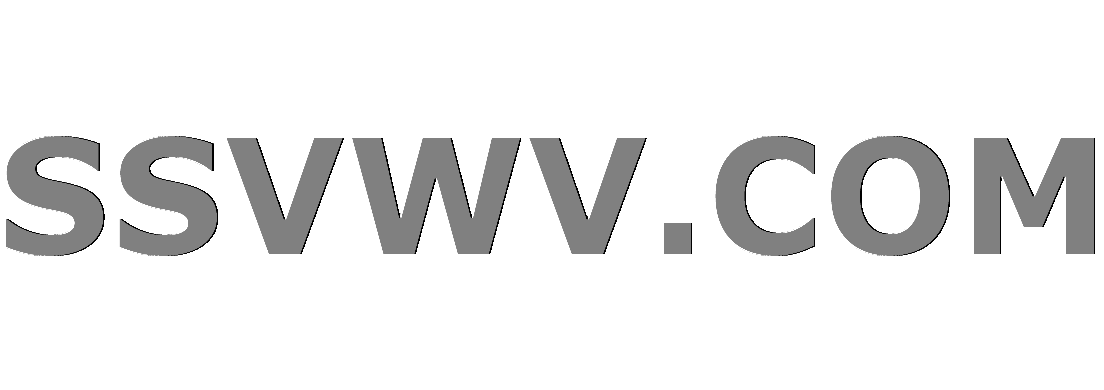
Multi tool use
I'm using this library to implement a basic machine learning in a project. I'm trying to loop over an array of teams of a competition and then use every single match as a label for a prediction about a percentage of success.
I'm training the script and all it seem to be ok in this part, but when I try to output the result of the prediction, there will be displayed only the first two team of the file. What I'm doing wrong with the loop?
This is the output of the machine learning library
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.413733) ["AC Chievo Verona"]=> float(0.586267) } }
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.58545) ["AC Chievo Verona"]=> float(0.41455) } }
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.58545) ["AC Chievo Verona"]=> float(0.41455) } }
But I expect something like this:
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.413733) ["AC Chievo Verona"]=> float(0.586267) } }
array(1) { [0]=> array(2) { ["Juventus"]=> float(0.58545) ["Milan"]=> float(0.41455) } }
array(1) { [0]=> array(2) { ["Sassuolo"]=> float(0.58545) ["Empoli"]=> float(0.41455) } }
// ecc...
Here is my code:
<?php
require_once __DIR__ . '/vendor/autoload.php';
use PhpmlClassificationSVC;
use PhpmlSupportVectorMachineKernel;
use PhpmlDatasetArrayDataset;
$data = json_decode(file_get_contents('serie_a.json'),true);
$classifier = new SVC(
Kernel::LINEAR, // $kernel
1.0, // $cost
3, // $degree
null, // $gamma
0.0, // $coef0
0.001, // $tolerance
100, // $cacheSize
true, // $shrinking
true // $probabilityEstimates, set to true
);
foreach($data['matches'] as $match){
$homeTeam = $match['homeTeam']['name'];
$awayTeam = $match['awayTeam']['name'];
#$matchday = $match['matchday'];
#$oldDate = new DateTime($match['utcDate']);
#$date = $oldDate->format('Y-m-d');
$labels = ["$homeTeam", "$awayTeam"];
}
$samples = [[1, 0], [1, 1], [0, 1]];
$classifier->train($samples, $labels);
echo '<pre>'.var_dump($classifier->predictProbability([[1, 0]])).'</pre>';
echo '<pre>'.var_dump($classifier->predictProbability([[1, 1]])).'</pre>';
echo '<pre>'.var_dump($classifier->predictProbability([[0, 1]])).'</pre>';
//$classifier->predictProbability([[3, 2], [1, 5]]);
// return [
// ['a' => 0.349833, 'b' => 0.650167],
// ['a' => 0.922664, 'b' => 0.0773364],
// ]
?>
php machine-learning foreach dataset
add a comment |
I'm using this library to implement a basic machine learning in a project. I'm trying to loop over an array of teams of a competition and then use every single match as a label for a prediction about a percentage of success.
I'm training the script and all it seem to be ok in this part, but when I try to output the result of the prediction, there will be displayed only the first two team of the file. What I'm doing wrong with the loop?
This is the output of the machine learning library
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.413733) ["AC Chievo Verona"]=> float(0.586267) } }
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.58545) ["AC Chievo Verona"]=> float(0.41455) } }
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.58545) ["AC Chievo Verona"]=> float(0.41455) } }
But I expect something like this:
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.413733) ["AC Chievo Verona"]=> float(0.586267) } }
array(1) { [0]=> array(2) { ["Juventus"]=> float(0.58545) ["Milan"]=> float(0.41455) } }
array(1) { [0]=> array(2) { ["Sassuolo"]=> float(0.58545) ["Empoli"]=> float(0.41455) } }
// ecc...
Here is my code:
<?php
require_once __DIR__ . '/vendor/autoload.php';
use PhpmlClassificationSVC;
use PhpmlSupportVectorMachineKernel;
use PhpmlDatasetArrayDataset;
$data = json_decode(file_get_contents('serie_a.json'),true);
$classifier = new SVC(
Kernel::LINEAR, // $kernel
1.0, // $cost
3, // $degree
null, // $gamma
0.0, // $coef0
0.001, // $tolerance
100, // $cacheSize
true, // $shrinking
true // $probabilityEstimates, set to true
);
foreach($data['matches'] as $match){
$homeTeam = $match['homeTeam']['name'];
$awayTeam = $match['awayTeam']['name'];
#$matchday = $match['matchday'];
#$oldDate = new DateTime($match['utcDate']);
#$date = $oldDate->format('Y-m-d');
$labels = ["$homeTeam", "$awayTeam"];
}
$samples = [[1, 0], [1, 1], [0, 1]];
$classifier->train($samples, $labels);
echo '<pre>'.var_dump($classifier->predictProbability([[1, 0]])).'</pre>';
echo '<pre>'.var_dump($classifier->predictProbability([[1, 1]])).'</pre>';
echo '<pre>'.var_dump($classifier->predictProbability([[0, 1]])).'</pre>';
//$classifier->predictProbability([[3, 2], [1, 5]]);
// return [
// ['a' => 0.349833, 'b' => 0.650167],
// ['a' => 0.922664, 'b' => 0.0773364],
// ]
?>
php machine-learning foreach dataset
add a comment |
I'm using this library to implement a basic machine learning in a project. I'm trying to loop over an array of teams of a competition and then use every single match as a label for a prediction about a percentage of success.
I'm training the script and all it seem to be ok in this part, but when I try to output the result of the prediction, there will be displayed only the first two team of the file. What I'm doing wrong with the loop?
This is the output of the machine learning library
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.413733) ["AC Chievo Verona"]=> float(0.586267) } }
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.58545) ["AC Chievo Verona"]=> float(0.41455) } }
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.58545) ["AC Chievo Verona"]=> float(0.41455) } }
But I expect something like this:
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.413733) ["AC Chievo Verona"]=> float(0.586267) } }
array(1) { [0]=> array(2) { ["Juventus"]=> float(0.58545) ["Milan"]=> float(0.41455) } }
array(1) { [0]=> array(2) { ["Sassuolo"]=> float(0.58545) ["Empoli"]=> float(0.41455) } }
// ecc...
Here is my code:
<?php
require_once __DIR__ . '/vendor/autoload.php';
use PhpmlClassificationSVC;
use PhpmlSupportVectorMachineKernel;
use PhpmlDatasetArrayDataset;
$data = json_decode(file_get_contents('serie_a.json'),true);
$classifier = new SVC(
Kernel::LINEAR, // $kernel
1.0, // $cost
3, // $degree
null, // $gamma
0.0, // $coef0
0.001, // $tolerance
100, // $cacheSize
true, // $shrinking
true // $probabilityEstimates, set to true
);
foreach($data['matches'] as $match){
$homeTeam = $match['homeTeam']['name'];
$awayTeam = $match['awayTeam']['name'];
#$matchday = $match['matchday'];
#$oldDate = new DateTime($match['utcDate']);
#$date = $oldDate->format('Y-m-d');
$labels = ["$homeTeam", "$awayTeam"];
}
$samples = [[1, 0], [1, 1], [0, 1]];
$classifier->train($samples, $labels);
echo '<pre>'.var_dump($classifier->predictProbability([[1, 0]])).'</pre>';
echo '<pre>'.var_dump($classifier->predictProbability([[1, 1]])).'</pre>';
echo '<pre>'.var_dump($classifier->predictProbability([[0, 1]])).'</pre>';
//$classifier->predictProbability([[3, 2], [1, 5]]);
// return [
// ['a' => 0.349833, 'b' => 0.650167],
// ['a' => 0.922664, 'b' => 0.0773364],
// ]
?>
php machine-learning foreach dataset
I'm using this library to implement a basic machine learning in a project. I'm trying to loop over an array of teams of a competition and then use every single match as a label for a prediction about a percentage of success.
I'm training the script and all it seem to be ok in this part, but when I try to output the result of the prediction, there will be displayed only the first two team of the file. What I'm doing wrong with the loop?
This is the output of the machine learning library
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.413733) ["AC Chievo Verona"]=> float(0.586267) } }
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.58545) ["AC Chievo Verona"]=> float(0.41455) } }
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.58545) ["AC Chievo Verona"]=> float(0.41455) } }
But I expect something like this:
array(1) { [0]=> array(2) { ["Frosinone Calcio"]=> float(0.413733) ["AC Chievo Verona"]=> float(0.586267) } }
array(1) { [0]=> array(2) { ["Juventus"]=> float(0.58545) ["Milan"]=> float(0.41455) } }
array(1) { [0]=> array(2) { ["Sassuolo"]=> float(0.58545) ["Empoli"]=> float(0.41455) } }
// ecc...
Here is my code:
<?php
require_once __DIR__ . '/vendor/autoload.php';
use PhpmlClassificationSVC;
use PhpmlSupportVectorMachineKernel;
use PhpmlDatasetArrayDataset;
$data = json_decode(file_get_contents('serie_a.json'),true);
$classifier = new SVC(
Kernel::LINEAR, // $kernel
1.0, // $cost
3, // $degree
null, // $gamma
0.0, // $coef0
0.001, // $tolerance
100, // $cacheSize
true, // $shrinking
true // $probabilityEstimates, set to true
);
foreach($data['matches'] as $match){
$homeTeam = $match['homeTeam']['name'];
$awayTeam = $match['awayTeam']['name'];
#$matchday = $match['matchday'];
#$oldDate = new DateTime($match['utcDate']);
#$date = $oldDate->format('Y-m-d');
$labels = ["$homeTeam", "$awayTeam"];
}
$samples = [[1, 0], [1, 1], [0, 1]];
$classifier->train($samples, $labels);
echo '<pre>'.var_dump($classifier->predictProbability([[1, 0]])).'</pre>';
echo '<pre>'.var_dump($classifier->predictProbability([[1, 1]])).'</pre>';
echo '<pre>'.var_dump($classifier->predictProbability([[0, 1]])).'</pre>';
//$classifier->predictProbability([[3, 2], [1, 5]]);
// return [
// ['a' => 0.349833, 'b' => 0.650167],
// ['a' => 0.922664, 'b' => 0.0773364],
// ]
?>
php machine-learning foreach dataset
php machine-learning foreach dataset
edited Nov 23 '18 at 13:08
desertnaut
17.5k63869
17.5k63869
asked Nov 23 '18 at 12:53
user10479680user10479680
668
668
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447105%2fphp-machine-learning%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447105%2fphp-machine-learning%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ie5oH6zpuOLH 4VxXi1XeYolNr3nO6x2w5ym5