How do I test the Elements value in React Native unit testing using Enzyme (Jest)
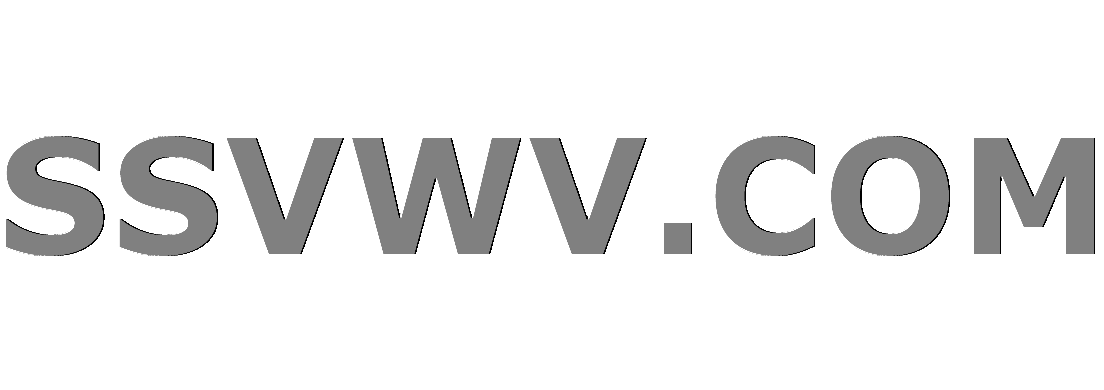
Multi tool use
I have the following Flash.js component to Test
export default class SnapshotChild extends Component {
render() {
return (
<View>
<Text>First Text</Text>
<Text>Second Text</Text>
</View>
);
}}
And My test cases is
describe('Flash Card', () => {
const flash = shallow(<Flash />);
it('test the `<Text/>` value',()=>{
console.log(flash.debug());
expect(flash.find('Component Text').at(0).text()).toEqual('First Text');
})});
Now when I run this code using npm test the result is showing
Expected value to equal:
"First Text"
Received:
"<Text />"
My expected result is "First Text" and getting "Text tag".
What's the wrong, please please somebody help me on this. Thanks in advance.
reactjs react-native jestjs enzyme
add a comment |
I have the following Flash.js component to Test
export default class SnapshotChild extends Component {
render() {
return (
<View>
<Text>First Text</Text>
<Text>Second Text</Text>
</View>
);
}}
And My test cases is
describe('Flash Card', () => {
const flash = shallow(<Flash />);
it('test the `<Text/>` value',()=>{
console.log(flash.debug());
expect(flash.find('Component Text').at(0).text()).toEqual('First Text');
})});
Now when I run this code using npm test the result is showing
Expected value to equal:
"First Text"
Received:
"<Text />"
My expected result is "First Text" and getting "Text tag".
What's the wrong, please please somebody help me on this. Thanks in advance.
reactjs react-native jestjs enzyme
add a comment |
I have the following Flash.js component to Test
export default class SnapshotChild extends Component {
render() {
return (
<View>
<Text>First Text</Text>
<Text>Second Text</Text>
</View>
);
}}
And My test cases is
describe('Flash Card', () => {
const flash = shallow(<Flash />);
it('test the `<Text/>` value',()=>{
console.log(flash.debug());
expect(flash.find('Component Text').at(0).text()).toEqual('First Text');
})});
Now when I run this code using npm test the result is showing
Expected value to equal:
"First Text"
Received:
"<Text />"
My expected result is "First Text" and getting "Text tag".
What's the wrong, please please somebody help me on this. Thanks in advance.
reactjs react-native jestjs enzyme
I have the following Flash.js component to Test
export default class SnapshotChild extends Component {
render() {
return (
<View>
<Text>First Text</Text>
<Text>Second Text</Text>
</View>
);
}}
And My test cases is
describe('Flash Card', () => {
const flash = shallow(<Flash />);
it('test the `<Text/>` value',()=>{
console.log(flash.debug());
expect(flash.find('Component Text').at(0).text()).toEqual('First Text');
})});
Now when I run this code using npm test the result is showing
Expected value to equal:
"First Text"
Received:
"<Text />"
My expected result is "First Text" and getting "Text tag".
What's the wrong, please please somebody help me on this. Thanks in advance.
reactjs react-native jestjs enzyme
reactjs react-native jestjs enzyme
edited Nov 23 '18 at 12:49
Bikash
asked Nov 23 '18 at 12:48


BikashBikash
3041213
3041213
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Since you use shallow()
nested <Text />
are not rendered. So method .text()
does not know how text will look like. So it seems just returning element name even without all its props.
Sure you can replace shallow()
with mount()
but I suggest you using amazing .toMatchSnapshot()
here.
Then your tests would look like
const flash = shallow(<Flash />);
it('has valid default output',()=>{
expect(flash).toMatchSnapshot();
})});
and that would check for both <Text />
elements and text inside as well.
[UPD] additionaly on why .text()
works this way. Image you had the same code with slightly different(but equal!) syntax:
<Text children={'First Text'} />
How could enzyme's helper .text()
know what to return here without rendering node?
[UPD2] if there is no way to use toMatchSnapshot()
it's still possible to test props
directly:
expect(flash.find(Text).at(0).props().children).toEqual('First Text')
But toMatchSnapshot
is much better in several ways(readability, maintainability, flexbility and other *abilities)
Hi @skyboyer, thank you so much for your quick response. Actually I have a task to test with elements value. How can I do this without using toMatchSnapshot()
– Bikash
Nov 23 '18 at 13:47
the same as with any other prop:expect(flash.find(Text).at(0).props().children).toEqual('First Text')
– skyboyer
Nov 23 '18 at 13:48
Thanks buddy its working.
– Bikash
Nov 23 '18 at 13:58
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447022%2fhow-do-i-test-the-elements-value-in-react-native-unit-testing-using-enzyme-jest%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Since you use shallow()
nested <Text />
are not rendered. So method .text()
does not know how text will look like. So it seems just returning element name even without all its props.
Sure you can replace shallow()
with mount()
but I suggest you using amazing .toMatchSnapshot()
here.
Then your tests would look like
const flash = shallow(<Flash />);
it('has valid default output',()=>{
expect(flash).toMatchSnapshot();
})});
and that would check for both <Text />
elements and text inside as well.
[UPD] additionaly on why .text()
works this way. Image you had the same code with slightly different(but equal!) syntax:
<Text children={'First Text'} />
How could enzyme's helper .text()
know what to return here without rendering node?
[UPD2] if there is no way to use toMatchSnapshot()
it's still possible to test props
directly:
expect(flash.find(Text).at(0).props().children).toEqual('First Text')
But toMatchSnapshot
is much better in several ways(readability, maintainability, flexbility and other *abilities)
Hi @skyboyer, thank you so much for your quick response. Actually I have a task to test with elements value. How can I do this without using toMatchSnapshot()
– Bikash
Nov 23 '18 at 13:47
the same as with any other prop:expect(flash.find(Text).at(0).props().children).toEqual('First Text')
– skyboyer
Nov 23 '18 at 13:48
Thanks buddy its working.
– Bikash
Nov 23 '18 at 13:58
add a comment |
Since you use shallow()
nested <Text />
are not rendered. So method .text()
does not know how text will look like. So it seems just returning element name even without all its props.
Sure you can replace shallow()
with mount()
but I suggest you using amazing .toMatchSnapshot()
here.
Then your tests would look like
const flash = shallow(<Flash />);
it('has valid default output',()=>{
expect(flash).toMatchSnapshot();
})});
and that would check for both <Text />
elements and text inside as well.
[UPD] additionaly on why .text()
works this way. Image you had the same code with slightly different(but equal!) syntax:
<Text children={'First Text'} />
How could enzyme's helper .text()
know what to return here without rendering node?
[UPD2] if there is no way to use toMatchSnapshot()
it's still possible to test props
directly:
expect(flash.find(Text).at(0).props().children).toEqual('First Text')
But toMatchSnapshot
is much better in several ways(readability, maintainability, flexbility and other *abilities)
Hi @skyboyer, thank you so much for your quick response. Actually I have a task to test with elements value. How can I do this without using toMatchSnapshot()
– Bikash
Nov 23 '18 at 13:47
the same as with any other prop:expect(flash.find(Text).at(0).props().children).toEqual('First Text')
– skyboyer
Nov 23 '18 at 13:48
Thanks buddy its working.
– Bikash
Nov 23 '18 at 13:58
add a comment |
Since you use shallow()
nested <Text />
are not rendered. So method .text()
does not know how text will look like. So it seems just returning element name even without all its props.
Sure you can replace shallow()
with mount()
but I suggest you using amazing .toMatchSnapshot()
here.
Then your tests would look like
const flash = shallow(<Flash />);
it('has valid default output',()=>{
expect(flash).toMatchSnapshot();
})});
and that would check for both <Text />
elements and text inside as well.
[UPD] additionaly on why .text()
works this way. Image you had the same code with slightly different(but equal!) syntax:
<Text children={'First Text'} />
How could enzyme's helper .text()
know what to return here without rendering node?
[UPD2] if there is no way to use toMatchSnapshot()
it's still possible to test props
directly:
expect(flash.find(Text).at(0).props().children).toEqual('First Text')
But toMatchSnapshot
is much better in several ways(readability, maintainability, flexbility and other *abilities)
Since you use shallow()
nested <Text />
are not rendered. So method .text()
does not know how text will look like. So it seems just returning element name even without all its props.
Sure you can replace shallow()
with mount()
but I suggest you using amazing .toMatchSnapshot()
here.
Then your tests would look like
const flash = shallow(<Flash />);
it('has valid default output',()=>{
expect(flash).toMatchSnapshot();
})});
and that would check for both <Text />
elements and text inside as well.
[UPD] additionaly on why .text()
works this way. Image you had the same code with slightly different(but equal!) syntax:
<Text children={'First Text'} />
How could enzyme's helper .text()
know what to return here without rendering node?
[UPD2] if there is no way to use toMatchSnapshot()
it's still possible to test props
directly:
expect(flash.find(Text).at(0).props().children).toEqual('First Text')
But toMatchSnapshot
is much better in several ways(readability, maintainability, flexbility and other *abilities)
edited Nov 23 '18 at 14:04
answered Nov 23 '18 at 12:54
skyboyerskyboyer
3,61611128
3,61611128
Hi @skyboyer, thank you so much for your quick response. Actually I have a task to test with elements value. How can I do this without using toMatchSnapshot()
– Bikash
Nov 23 '18 at 13:47
the same as with any other prop:expect(flash.find(Text).at(0).props().children).toEqual('First Text')
– skyboyer
Nov 23 '18 at 13:48
Thanks buddy its working.
– Bikash
Nov 23 '18 at 13:58
add a comment |
Hi @skyboyer, thank you so much for your quick response. Actually I have a task to test with elements value. How can I do this without using toMatchSnapshot()
– Bikash
Nov 23 '18 at 13:47
the same as with any other prop:expect(flash.find(Text).at(0).props().children).toEqual('First Text')
– skyboyer
Nov 23 '18 at 13:48
Thanks buddy its working.
– Bikash
Nov 23 '18 at 13:58
Hi @skyboyer, thank you so much for your quick response. Actually I have a task to test with elements value. How can I do this without using toMatchSnapshot()
– Bikash
Nov 23 '18 at 13:47
Hi @skyboyer, thank you so much for your quick response. Actually I have a task to test with elements value. How can I do this without using toMatchSnapshot()
– Bikash
Nov 23 '18 at 13:47
the same as with any other prop:
expect(flash.find(Text).at(0).props().children).toEqual('First Text')
– skyboyer
Nov 23 '18 at 13:48
the same as with any other prop:
expect(flash.find(Text).at(0).props().children).toEqual('First Text')
– skyboyer
Nov 23 '18 at 13:48
Thanks buddy its working.
– Bikash
Nov 23 '18 at 13:58
Thanks buddy its working.
– Bikash
Nov 23 '18 at 13:58
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447022%2fhow-do-i-test-the-elements-value-in-react-native-unit-testing-using-enzyme-jest%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CLzWYiFP WGx2YwE 5p04za,xPms5K 7UGIph7lzw2DKEMbrRTX