Initiating a flow session from a flow that is annoted with InitiatedBy to a flow which is also InitiatedBy
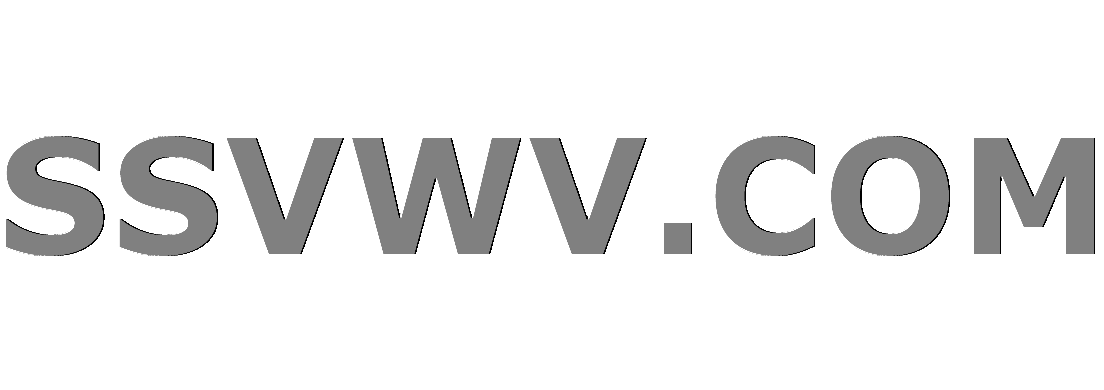
Multi tool use
Is it possible to initiate a flow session from a flow that is annoted with InitiatedBy to a flow which is also annoted with InitiatedBy?
For example:
@InitiatingFlow
Class FlowA
@InitiatedBy(FlowA.class)
Class FlowB
@InitiatedBy(FlowB.class)
Class FlowC
is it possible to achieve sequence of flow session like:
A->B->C ?
corda
add a comment |
Is it possible to initiate a flow session from a flow that is annoted with InitiatedBy to a flow which is also annoted with InitiatedBy?
For example:
@InitiatingFlow
Class FlowA
@InitiatedBy(FlowA.class)
Class FlowB
@InitiatedBy(FlowB.class)
Class FlowC
is it possible to achieve sequence of flow session like:
A->B->C ?
corda
add a comment |
Is it possible to initiate a flow session from a flow that is annoted with InitiatedBy to a flow which is also annoted with InitiatedBy?
For example:
@InitiatingFlow
Class FlowA
@InitiatedBy(FlowA.class)
Class FlowB
@InitiatedBy(FlowB.class)
Class FlowC
is it possible to achieve sequence of flow session like:
A->B->C ?
corda
Is it possible to initiate a flow session from a flow that is annoted with InitiatedBy to a flow which is also annoted with InitiatedBy?
For example:
@InitiatingFlow
Class FlowA
@InitiatedBy(FlowA.class)
Class FlowB
@InitiatedBy(FlowB.class)
Class FlowC
is it possible to achieve sequence of flow session like:
A->B->C ?
corda
corda
edited Nov 23 '18 at 12:58


Joel
10.8k11229
10.8k11229
asked Nov 23 '18 at 12:49


nitesh solankinitesh solanki
10617
10617
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Yes, this is possible, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
val newFlowSession = initiateFlow(secondCounterparty)
val int = newFlowSession.receive<Int>().unwrap { it }
flowSession.send(int)
}
}
@InitiatingFlow
@InitiatedBy(Responder::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
However, there is one major caveat. In Corda 3.x, you can't have two FlowSession
s with the same counterparty in the same flow. So either you need to disallow the case where A -> B -> A, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
if (secondCounterparty == ourIdentity) {
throw FlowException("In Corda 3.x, you can't have two flow sessions with the same party.")
}
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
if (secondCounterparty == flowSession.counterparty) {
throw FlowException("In Corda 3.x, you can't have two flow sessions with the same party.")
}
val newFlowSession = initiateFlow(secondCounterparty)
val int = newFlowSession.receive<Int>().unwrap { it }
flowSession.send(int)
}
}
@InitiatingFlow
@InitiatedBy(Responder::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
Or you need to drop into an InitiatingFlow
subflow in Responder
before starting the flow that starts ResponderResponder
, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
val int = subFlow(ResponderInitiator(secondCounterparty))
flowSession.send(int)
}
}
@InitiatingFlow
class ResponderInitiator(val counterparty: Party) : FlowLogic<Int>() {
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(counterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(ResponderInitiator::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447038%2finitiating-a-flow-session-from-a-flow-that-is-annoted-with-initiatedby-to-a-flow%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Yes, this is possible, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
val newFlowSession = initiateFlow(secondCounterparty)
val int = newFlowSession.receive<Int>().unwrap { it }
flowSession.send(int)
}
}
@InitiatingFlow
@InitiatedBy(Responder::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
However, there is one major caveat. In Corda 3.x, you can't have two FlowSession
s with the same counterparty in the same flow. So either you need to disallow the case where A -> B -> A, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
if (secondCounterparty == ourIdentity) {
throw FlowException("In Corda 3.x, you can't have two flow sessions with the same party.")
}
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
if (secondCounterparty == flowSession.counterparty) {
throw FlowException("In Corda 3.x, you can't have two flow sessions with the same party.")
}
val newFlowSession = initiateFlow(secondCounterparty)
val int = newFlowSession.receive<Int>().unwrap { it }
flowSession.send(int)
}
}
@InitiatingFlow
@InitiatedBy(Responder::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
Or you need to drop into an InitiatingFlow
subflow in Responder
before starting the flow that starts ResponderResponder
, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
val int = subFlow(ResponderInitiator(secondCounterparty))
flowSession.send(int)
}
}
@InitiatingFlow
class ResponderInitiator(val counterparty: Party) : FlowLogic<Int>() {
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(counterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(ResponderInitiator::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
add a comment |
Yes, this is possible, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
val newFlowSession = initiateFlow(secondCounterparty)
val int = newFlowSession.receive<Int>().unwrap { it }
flowSession.send(int)
}
}
@InitiatingFlow
@InitiatedBy(Responder::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
However, there is one major caveat. In Corda 3.x, you can't have two FlowSession
s with the same counterparty in the same flow. So either you need to disallow the case where A -> B -> A, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
if (secondCounterparty == ourIdentity) {
throw FlowException("In Corda 3.x, you can't have two flow sessions with the same party.")
}
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
if (secondCounterparty == flowSession.counterparty) {
throw FlowException("In Corda 3.x, you can't have two flow sessions with the same party.")
}
val newFlowSession = initiateFlow(secondCounterparty)
val int = newFlowSession.receive<Int>().unwrap { it }
flowSession.send(int)
}
}
@InitiatingFlow
@InitiatedBy(Responder::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
Or you need to drop into an InitiatingFlow
subflow in Responder
before starting the flow that starts ResponderResponder
, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
val int = subFlow(ResponderInitiator(secondCounterparty))
flowSession.send(int)
}
}
@InitiatingFlow
class ResponderInitiator(val counterparty: Party) : FlowLogic<Int>() {
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(counterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(ResponderInitiator::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
add a comment |
Yes, this is possible, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
val newFlowSession = initiateFlow(secondCounterparty)
val int = newFlowSession.receive<Int>().unwrap { it }
flowSession.send(int)
}
}
@InitiatingFlow
@InitiatedBy(Responder::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
However, there is one major caveat. In Corda 3.x, you can't have two FlowSession
s with the same counterparty in the same flow. So either you need to disallow the case where A -> B -> A, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
if (secondCounterparty == ourIdentity) {
throw FlowException("In Corda 3.x, you can't have two flow sessions with the same party.")
}
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
if (secondCounterparty == flowSession.counterparty) {
throw FlowException("In Corda 3.x, you can't have two flow sessions with the same party.")
}
val newFlowSession = initiateFlow(secondCounterparty)
val int = newFlowSession.receive<Int>().unwrap { it }
flowSession.send(int)
}
}
@InitiatingFlow
@InitiatedBy(Responder::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
Or you need to drop into an InitiatingFlow
subflow in Responder
before starting the flow that starts ResponderResponder
, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
val int = subFlow(ResponderInitiator(secondCounterparty))
flowSession.send(int)
}
}
@InitiatingFlow
class ResponderInitiator(val counterparty: Party) : FlowLogic<Int>() {
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(counterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(ResponderInitiator::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
Yes, this is possible, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
val newFlowSession = initiateFlow(secondCounterparty)
val int = newFlowSession.receive<Int>().unwrap { it }
flowSession.send(int)
}
}
@InitiatingFlow
@InitiatedBy(Responder::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
However, there is one major caveat. In Corda 3.x, you can't have two FlowSession
s with the same counterparty in the same flow. So either you need to disallow the case where A -> B -> A, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
if (secondCounterparty == ourIdentity) {
throw FlowException("In Corda 3.x, you can't have two flow sessions with the same party.")
}
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
if (secondCounterparty == flowSession.counterparty) {
throw FlowException("In Corda 3.x, you can't have two flow sessions with the same party.")
}
val newFlowSession = initiateFlow(secondCounterparty)
val int = newFlowSession.receive<Int>().unwrap { it }
flowSession.send(int)
}
}
@InitiatingFlow
@InitiatedBy(Responder::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
Or you need to drop into an InitiatingFlow
subflow in Responder
before starting the flow that starts ResponderResponder
, as follows:
@InitiatingFlow
@StartableByRPC
class Initiator(val firstCounterparty: Party, val secondCounterparty: Party) : FlowLogic<Int>() {
override val progressTracker = ProgressTracker()
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(firstCounterparty)
flowSession.send(secondCounterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(Initiator::class)
class Responder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
val secondCounterparty = flowSession.receive<Party>().unwrap { it }
val int = subFlow(ResponderInitiator(secondCounterparty))
flowSession.send(int)
}
}
@InitiatingFlow
class ResponderInitiator(val counterparty: Party) : FlowLogic<Int>() {
@Suspendable
override fun call(): Int {
val flowSession = initiateFlow(counterparty)
return flowSession.receive<Int>().unwrap { it }
}
}
@InitiatingFlow
@InitiatedBy(ResponderInitiator::class)
class ResponderResponder(val flowSession: FlowSession) : FlowLogic<Unit>() {
@Suspendable
override fun call() {
flowSession.send(3)
}
}
answered Nov 26 '18 at 11:47


JoelJoel
10.8k11229
10.8k11229
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447038%2finitiating-a-flow-session-from-a-flow-that-is-annoted-with-initiatedby-to-a-flow%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
e5W w3OZvkJm8Niq9A1yfXedhDAfzJh9 VSYPXrAOlY1L,5tI5bIgMJhNDWYv9ZTZNmvavol3,40Zle9sK