$(xml).find('someElement') : pulling values with jquery from xml with Namespaces
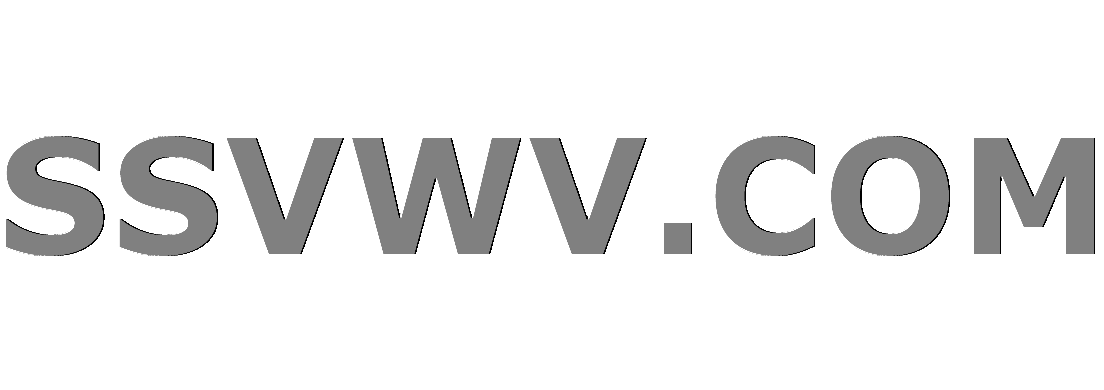
Multi tool use
up vote
6
down vote
favorite
The following code works in Chrome, but not IE, or FireFox. Does someone know the appropriate cross browser code?
<s:Envelope xmlns:s="http://www.w3.org/2003/05/soap-envelope" xmlns:a="http://www.w3.org/2005/08/addressing">
<s:Header>
<a:Action s:mustUnderstand="1">http://tempuri.org/SubscriptionService/Update</a:Action>
<netdx:Duplex xmlns:netdx="http://schemas.microsoft.com/2008/04/netduplex">
<netdx:Address>http://docs.oasis-open.org/ws-rx/wsmc/200702/anonymous?id=4ed8a7ee-b124-e03e-abf0-a294e99cff73</netdx:Address>
<netdx:SessionId>177b4f47-5664-d96c-7ffa-0a8d879b67dd</netdx:SessionId>
</netdx:Duplex>
</s:Header>
<s:Body>
<Update xmlns="http://tempuri.org/">
<lstResponseStruct xmlns:b="http://schemas.datacontract.org/2004/07/FSS.Libs.Core.InterprocessData.RMS" xmlns:i="http://www.w3.org/2001/XMLSchema-instance">
<b:DataA>
<b:ValueA>1.339565</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>120.3717</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>133.563116</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>-0.0059159999999999994</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
</lstResponseStruct>
</Update>
</s:Body>
Here are the JavaScript snippets...
<script src="http://code.jquery.com/jquery.min.js" type="text/javascript"></script>
var nodes;
if (typeof DOMParser != "undefined")
nodes = ((new DOMParser()).parseFromString(request.responseText, "application/xml")).getElementsByTagName("*");
else {
request.responseXML.loadXML(request.responseText);
nodes = request.responseXML.getElementsByTagName("*");
}
for (var i = 0; i < nodes.length; i++) {
var element = nodes[i];
...
if ((element.localName == "Body" || element.baseName == "Body") && element.namespaceURI == "http://www.w3.org/2003/05/soap-envelope") {
body = element;
break;
}
$(body).find('DataA').each(function () {
... Do something
}
for some reason, in each browser "body" definitely contains the body xml, however the $(body).find('DataA') doesn't return results for IE or FireFox.
Update:
Adding the namespace $(body).find('b\:DataA') works well for FireFox and IE, but breaks Chrome!
jquery xml internet-explorer firefox

add a comment |
up vote
6
down vote
favorite
The following code works in Chrome, but not IE, or FireFox. Does someone know the appropriate cross browser code?
<s:Envelope xmlns:s="http://www.w3.org/2003/05/soap-envelope" xmlns:a="http://www.w3.org/2005/08/addressing">
<s:Header>
<a:Action s:mustUnderstand="1">http://tempuri.org/SubscriptionService/Update</a:Action>
<netdx:Duplex xmlns:netdx="http://schemas.microsoft.com/2008/04/netduplex">
<netdx:Address>http://docs.oasis-open.org/ws-rx/wsmc/200702/anonymous?id=4ed8a7ee-b124-e03e-abf0-a294e99cff73</netdx:Address>
<netdx:SessionId>177b4f47-5664-d96c-7ffa-0a8d879b67dd</netdx:SessionId>
</netdx:Duplex>
</s:Header>
<s:Body>
<Update xmlns="http://tempuri.org/">
<lstResponseStruct xmlns:b="http://schemas.datacontract.org/2004/07/FSS.Libs.Core.InterprocessData.RMS" xmlns:i="http://www.w3.org/2001/XMLSchema-instance">
<b:DataA>
<b:ValueA>1.339565</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>120.3717</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>133.563116</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>-0.0059159999999999994</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
</lstResponseStruct>
</Update>
</s:Body>
Here are the JavaScript snippets...
<script src="http://code.jquery.com/jquery.min.js" type="text/javascript"></script>
var nodes;
if (typeof DOMParser != "undefined")
nodes = ((new DOMParser()).parseFromString(request.responseText, "application/xml")).getElementsByTagName("*");
else {
request.responseXML.loadXML(request.responseText);
nodes = request.responseXML.getElementsByTagName("*");
}
for (var i = 0; i < nodes.length; i++) {
var element = nodes[i];
...
if ((element.localName == "Body" || element.baseName == "Body") && element.namespaceURI == "http://www.w3.org/2003/05/soap-envelope") {
body = element;
break;
}
$(body).find('DataA').each(function () {
... Do something
}
for some reason, in each browser "body" definitely contains the body xml, however the $(body).find('DataA') doesn't return results for IE or FireFox.
Update:
Adding the namespace $(body).find('b\:DataA') works well for FireFox and IE, but breaks Chrome!
jquery xml internet-explorer firefox

4
Tip: Use jQuery's$.parseXML
method. It returns anXMLDocument
object (not a jQuery object). To get a jQuery object, wrap the return value:$($.parseXML('<x>Test</x>'));
– Rob W
Apr 16 '12 at 21:14
Better yet, since this appears to be an ajax request, use jquery'sajax
and set thedataType
appropriately (or let jquery figure it out) and you won't even need to callparseXML
directly.
– James Montagne
Apr 16 '12 at 21:24
$(xData.responseXML).find("z\:row, row").each(function() { // Do Stuff }); Seems to work for IE, FireFox, and Chrome.
– antwarpes
Apr 16 '12 at 21:48
add a comment |
up vote
6
down vote
favorite
up vote
6
down vote
favorite
The following code works in Chrome, but not IE, or FireFox. Does someone know the appropriate cross browser code?
<s:Envelope xmlns:s="http://www.w3.org/2003/05/soap-envelope" xmlns:a="http://www.w3.org/2005/08/addressing">
<s:Header>
<a:Action s:mustUnderstand="1">http://tempuri.org/SubscriptionService/Update</a:Action>
<netdx:Duplex xmlns:netdx="http://schemas.microsoft.com/2008/04/netduplex">
<netdx:Address>http://docs.oasis-open.org/ws-rx/wsmc/200702/anonymous?id=4ed8a7ee-b124-e03e-abf0-a294e99cff73</netdx:Address>
<netdx:SessionId>177b4f47-5664-d96c-7ffa-0a8d879b67dd</netdx:SessionId>
</netdx:Duplex>
</s:Header>
<s:Body>
<Update xmlns="http://tempuri.org/">
<lstResponseStruct xmlns:b="http://schemas.datacontract.org/2004/07/FSS.Libs.Core.InterprocessData.RMS" xmlns:i="http://www.w3.org/2001/XMLSchema-instance">
<b:DataA>
<b:ValueA>1.339565</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>120.3717</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>133.563116</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>-0.0059159999999999994</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
</lstResponseStruct>
</Update>
</s:Body>
Here are the JavaScript snippets...
<script src="http://code.jquery.com/jquery.min.js" type="text/javascript"></script>
var nodes;
if (typeof DOMParser != "undefined")
nodes = ((new DOMParser()).parseFromString(request.responseText, "application/xml")).getElementsByTagName("*");
else {
request.responseXML.loadXML(request.responseText);
nodes = request.responseXML.getElementsByTagName("*");
}
for (var i = 0; i < nodes.length; i++) {
var element = nodes[i];
...
if ((element.localName == "Body" || element.baseName == "Body") && element.namespaceURI == "http://www.w3.org/2003/05/soap-envelope") {
body = element;
break;
}
$(body).find('DataA').each(function () {
... Do something
}
for some reason, in each browser "body" definitely contains the body xml, however the $(body).find('DataA') doesn't return results for IE or FireFox.
Update:
Adding the namespace $(body).find('b\:DataA') works well for FireFox and IE, but breaks Chrome!
jquery xml internet-explorer firefox

The following code works in Chrome, but not IE, or FireFox. Does someone know the appropriate cross browser code?
<s:Envelope xmlns:s="http://www.w3.org/2003/05/soap-envelope" xmlns:a="http://www.w3.org/2005/08/addressing">
<s:Header>
<a:Action s:mustUnderstand="1">http://tempuri.org/SubscriptionService/Update</a:Action>
<netdx:Duplex xmlns:netdx="http://schemas.microsoft.com/2008/04/netduplex">
<netdx:Address>http://docs.oasis-open.org/ws-rx/wsmc/200702/anonymous?id=4ed8a7ee-b124-e03e-abf0-a294e99cff73</netdx:Address>
<netdx:SessionId>177b4f47-5664-d96c-7ffa-0a8d879b67dd</netdx:SessionId>
</netdx:Duplex>
</s:Header>
<s:Body>
<Update xmlns="http://tempuri.org/">
<lstResponseStruct xmlns:b="http://schemas.datacontract.org/2004/07/FSS.Libs.Core.InterprocessData.RMS" xmlns:i="http://www.w3.org/2001/XMLSchema-instance">
<b:DataA>
<b:ValueA>1.339565</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>120.3717</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>133.563116</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
<b:DataA>
<b:ValueA>-0.0059159999999999994</b:ValueA>
<b:Status>None</b:Status>
</b:DataA>
</lstResponseStruct>
</Update>
</s:Body>
Here are the JavaScript snippets...
<script src="http://code.jquery.com/jquery.min.js" type="text/javascript"></script>
var nodes;
if (typeof DOMParser != "undefined")
nodes = ((new DOMParser()).parseFromString(request.responseText, "application/xml")).getElementsByTagName("*");
else {
request.responseXML.loadXML(request.responseText);
nodes = request.responseXML.getElementsByTagName("*");
}
for (var i = 0; i < nodes.length; i++) {
var element = nodes[i];
...
if ((element.localName == "Body" || element.baseName == "Body") && element.namespaceURI == "http://www.w3.org/2003/05/soap-envelope") {
body = element;
break;
}
$(body).find('DataA').each(function () {
... Do something
}
for some reason, in each browser "body" definitely contains the body xml, however the $(body).find('DataA') doesn't return results for IE or FireFox.
Update:
Adding the namespace $(body).find('b\:DataA') works well for FireFox and IE, but breaks Chrome!
jquery xml internet-explorer firefox

jquery xml internet-explorer firefox

edited Apr 18 '12 at 16:04
asked Apr 16 '12 at 20:18
antwarpes
1,24721217
1,24721217
4
Tip: Use jQuery's$.parseXML
method. It returns anXMLDocument
object (not a jQuery object). To get a jQuery object, wrap the return value:$($.parseXML('<x>Test</x>'));
– Rob W
Apr 16 '12 at 21:14
Better yet, since this appears to be an ajax request, use jquery'sajax
and set thedataType
appropriately (or let jquery figure it out) and you won't even need to callparseXML
directly.
– James Montagne
Apr 16 '12 at 21:24
$(xData.responseXML).find("z\:row, row").each(function() { // Do Stuff }); Seems to work for IE, FireFox, and Chrome.
– antwarpes
Apr 16 '12 at 21:48
add a comment |
4
Tip: Use jQuery's$.parseXML
method. It returns anXMLDocument
object (not a jQuery object). To get a jQuery object, wrap the return value:$($.parseXML('<x>Test</x>'));
– Rob W
Apr 16 '12 at 21:14
Better yet, since this appears to be an ajax request, use jquery'sajax
and set thedataType
appropriately (or let jquery figure it out) and you won't even need to callparseXML
directly.
– James Montagne
Apr 16 '12 at 21:24
$(xData.responseXML).find("z\:row, row").each(function() { // Do Stuff }); Seems to work for IE, FireFox, and Chrome.
– antwarpes
Apr 16 '12 at 21:48
4
4
Tip: Use jQuery's
$.parseXML
method. It returns an XMLDocument
object (not a jQuery object). To get a jQuery object, wrap the return value: $($.parseXML('<x>Test</x>'));
– Rob W
Apr 16 '12 at 21:14
Tip: Use jQuery's
$.parseXML
method. It returns an XMLDocument
object (not a jQuery object). To get a jQuery object, wrap the return value: $($.parseXML('<x>Test</x>'));
– Rob W
Apr 16 '12 at 21:14
Better yet, since this appears to be an ajax request, use jquery's
ajax
and set the dataType
appropriately (or let jquery figure it out) and you won't even need to call parseXML
directly.– James Montagne
Apr 16 '12 at 21:24
Better yet, since this appears to be an ajax request, use jquery's
ajax
and set the dataType
appropriately (or let jquery figure it out) and you won't even need to call parseXML
directly.– James Montagne
Apr 16 '12 at 21:24
$(xData.responseXML).find("z\:row, row").each(function() { // Do Stuff }); Seems to work for IE, FireFox, and Chrome.
– antwarpes
Apr 16 '12 at 21:48
$(xData.responseXML).find("z\:row, row").each(function() { // Do Stuff }); Seems to work for IE, FireFox, and Chrome.
– antwarpes
Apr 16 '12 at 21:48
add a comment |
2 Answers
2
active
oldest
votes
up vote
12
down vote
accepted
It was a problem accessing XML nodes without the namespace specified. For some reason Chrome doesn't want to see the namespace.
I found that the "b:DataA" selector works for FireFox and IE, and the "DataA" selector works for Chrome.
so...
$(xData.responseXML).find("b\:DataA, DataA").each(function() { // Do Stuff });
Seems to work for IE, FireFox, and Chrome.
see http://www.steveworkman.com/html5-2/javascript/2011/improving-javascript-xml-node-finding-performance-by-2000/ for more information and ways to improve XML node finding performance.
1
Thanks, we have the same problem. +1 for solving my problem.
– Dexter Huinda
Jul 19 '12 at 13:40
Thanks, this solved my problem. Oh, and it works in Safari too for that matter.. :-)
– Drew
Oct 13 '14 at 16:03
add a comment |
up vote
0
down vote
It's working fine!!! Try this,
Chrome/Firefox:
xml.children[0].childNodes[1].innerHTML;
IE8+/Safari:
xml.childNodes[0].childNodes[1].textContent;
IE8:
xml.documentElement.childNodes[1].text;
Sample code here,
var xml = $.parseXML(XMLDOC);
Var xmlNodeValue = "";
if(userAgent.match("firbox")){
xml.children[0].childNodes[1].innerHTML;
}else{ // IE8+
xmlNodeValue = xml.childNodes[0].childNodes[1].textContent;
}
referencing using hard coded position index is not flexible.
– lulalala
Mar 11 '15 at 2:25
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
12
down vote
accepted
It was a problem accessing XML nodes without the namespace specified. For some reason Chrome doesn't want to see the namespace.
I found that the "b:DataA" selector works for FireFox and IE, and the "DataA" selector works for Chrome.
so...
$(xData.responseXML).find("b\:DataA, DataA").each(function() { // Do Stuff });
Seems to work for IE, FireFox, and Chrome.
see http://www.steveworkman.com/html5-2/javascript/2011/improving-javascript-xml-node-finding-performance-by-2000/ for more information and ways to improve XML node finding performance.
1
Thanks, we have the same problem. +1 for solving my problem.
– Dexter Huinda
Jul 19 '12 at 13:40
Thanks, this solved my problem. Oh, and it works in Safari too for that matter.. :-)
– Drew
Oct 13 '14 at 16:03
add a comment |
up vote
12
down vote
accepted
It was a problem accessing XML nodes without the namespace specified. For some reason Chrome doesn't want to see the namespace.
I found that the "b:DataA" selector works for FireFox and IE, and the "DataA" selector works for Chrome.
so...
$(xData.responseXML).find("b\:DataA, DataA").each(function() { // Do Stuff });
Seems to work for IE, FireFox, and Chrome.
see http://www.steveworkman.com/html5-2/javascript/2011/improving-javascript-xml-node-finding-performance-by-2000/ for more information and ways to improve XML node finding performance.
1
Thanks, we have the same problem. +1 for solving my problem.
– Dexter Huinda
Jul 19 '12 at 13:40
Thanks, this solved my problem. Oh, and it works in Safari too for that matter.. :-)
– Drew
Oct 13 '14 at 16:03
add a comment |
up vote
12
down vote
accepted
up vote
12
down vote
accepted
It was a problem accessing XML nodes without the namespace specified. For some reason Chrome doesn't want to see the namespace.
I found that the "b:DataA" selector works for FireFox and IE, and the "DataA" selector works for Chrome.
so...
$(xData.responseXML).find("b\:DataA, DataA").each(function() { // Do Stuff });
Seems to work for IE, FireFox, and Chrome.
see http://www.steveworkman.com/html5-2/javascript/2011/improving-javascript-xml-node-finding-performance-by-2000/ for more information and ways to improve XML node finding performance.
It was a problem accessing XML nodes without the namespace specified. For some reason Chrome doesn't want to see the namespace.
I found that the "b:DataA" selector works for FireFox and IE, and the "DataA" selector works for Chrome.
so...
$(xData.responseXML).find("b\:DataA, DataA").each(function() { // Do Stuff });
Seems to work for IE, FireFox, and Chrome.
see http://www.steveworkman.com/html5-2/javascript/2011/improving-javascript-xml-node-finding-performance-by-2000/ for more information and ways to improve XML node finding performance.
answered Apr 17 '12 at 14:14
antwarpes
1,24721217
1,24721217
1
Thanks, we have the same problem. +1 for solving my problem.
– Dexter Huinda
Jul 19 '12 at 13:40
Thanks, this solved my problem. Oh, and it works in Safari too for that matter.. :-)
– Drew
Oct 13 '14 at 16:03
add a comment |
1
Thanks, we have the same problem. +1 for solving my problem.
– Dexter Huinda
Jul 19 '12 at 13:40
Thanks, this solved my problem. Oh, and it works in Safari too for that matter.. :-)
– Drew
Oct 13 '14 at 16:03
1
1
Thanks, we have the same problem. +1 for solving my problem.
– Dexter Huinda
Jul 19 '12 at 13:40
Thanks, we have the same problem. +1 for solving my problem.
– Dexter Huinda
Jul 19 '12 at 13:40
Thanks, this solved my problem. Oh, and it works in Safari too for that matter.. :-)
– Drew
Oct 13 '14 at 16:03
Thanks, this solved my problem. Oh, and it works in Safari too for that matter.. :-)
– Drew
Oct 13 '14 at 16:03
add a comment |
up vote
0
down vote
It's working fine!!! Try this,
Chrome/Firefox:
xml.children[0].childNodes[1].innerHTML;
IE8+/Safari:
xml.childNodes[0].childNodes[1].textContent;
IE8:
xml.documentElement.childNodes[1].text;
Sample code here,
var xml = $.parseXML(XMLDOC);
Var xmlNodeValue = "";
if(userAgent.match("firbox")){
xml.children[0].childNodes[1].innerHTML;
}else{ // IE8+
xmlNodeValue = xml.childNodes[0].childNodes[1].textContent;
}
referencing using hard coded position index is not flexible.
– lulalala
Mar 11 '15 at 2:25
add a comment |
up vote
0
down vote
It's working fine!!! Try this,
Chrome/Firefox:
xml.children[0].childNodes[1].innerHTML;
IE8+/Safari:
xml.childNodes[0].childNodes[1].textContent;
IE8:
xml.documentElement.childNodes[1].text;
Sample code here,
var xml = $.parseXML(XMLDOC);
Var xmlNodeValue = "";
if(userAgent.match("firbox")){
xml.children[0].childNodes[1].innerHTML;
}else{ // IE8+
xmlNodeValue = xml.childNodes[0].childNodes[1].textContent;
}
referencing using hard coded position index is not flexible.
– lulalala
Mar 11 '15 at 2:25
add a comment |
up vote
0
down vote
up vote
0
down vote
It's working fine!!! Try this,
Chrome/Firefox:
xml.children[0].childNodes[1].innerHTML;
IE8+/Safari:
xml.childNodes[0].childNodes[1].textContent;
IE8:
xml.documentElement.childNodes[1].text;
Sample code here,
var xml = $.parseXML(XMLDOC);
Var xmlNodeValue = "";
if(userAgent.match("firbox")){
xml.children[0].childNodes[1].innerHTML;
}else{ // IE8+
xmlNodeValue = xml.childNodes[0].childNodes[1].textContent;
}
It's working fine!!! Try this,
Chrome/Firefox:
xml.children[0].childNodes[1].innerHTML;
IE8+/Safari:
xml.childNodes[0].childNodes[1].textContent;
IE8:
xml.documentElement.childNodes[1].text;
Sample code here,
var xml = $.parseXML(XMLDOC);
Var xmlNodeValue = "";
if(userAgent.match("firbox")){
xml.children[0].childNodes[1].innerHTML;
}else{ // IE8+
xmlNodeValue = xml.childNodes[0].childNodes[1].textContent;
}
edited Nov 19 at 21:17
answered Feb 21 '15 at 11:25
Muthu Selvam
364
364
referencing using hard coded position index is not flexible.
– lulalala
Mar 11 '15 at 2:25
add a comment |
referencing using hard coded position index is not flexible.
– lulalala
Mar 11 '15 at 2:25
referencing using hard coded position index is not flexible.
– lulalala
Mar 11 '15 at 2:25
referencing using hard coded position index is not flexible.
– lulalala
Mar 11 '15 at 2:25
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f10181087%2fxml-findsomeelement-pulling-values-with-jquery-from-xml-with-namespace%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KJlWV 47R
4
Tip: Use jQuery's
$.parseXML
method. It returns anXMLDocument
object (not a jQuery object). To get a jQuery object, wrap the return value:$($.parseXML('<x>Test</x>'));
– Rob W
Apr 16 '12 at 21:14
Better yet, since this appears to be an ajax request, use jquery's
ajax
and set thedataType
appropriately (or let jquery figure it out) and you won't even need to callparseXML
directly.– James Montagne
Apr 16 '12 at 21:24
$(xData.responseXML).find("z\:row, row").each(function() { // Do Stuff }); Seems to work for IE, FireFox, and Chrome.
– antwarpes
Apr 16 '12 at 21:48