Persist relation to a class (and not a class instance) in Ruby on Rails with Mongoid
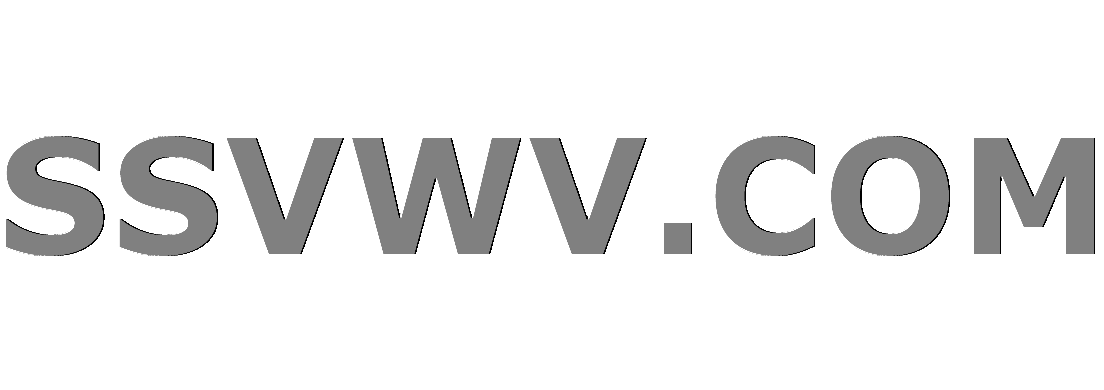
Multi tool use
up vote
1
down vote
favorite
Assume my users can subscribe to several plan_types. I want to define those plans as classes, and be able to keep, for each user, a reference to a subscription_plan and also a presubscription_plan. This is like a State pattern :
class Payment::SubscriptionPlan
def self.max_subscription_quantity
120
end
end
class Payment::ConcretePlan1 < Payment::SubscriptionPlan
def self.commitment_period
2.months
end
end
class Payment::ConcretePlan2 < Payment::SubscriptionPlan
def self.max_subscription_quantity
50
end
end
What is the best way to "persist" the relation to a class in the database ? Currently I was doing the following using the as_enum
gem
class Settings
setting :subscription_plans, type: Array, default: [
Payment::ConcretePlan1,
Payment::ConcretePlan2
]
end
class User
as_enum :subscription_plan, Settings.subscription_plans, map: :string
as_enum :presubscription_plan, Settings.subscription_plans, map: :string
def subscription_plan
return nil if super.nil?
Payment.const_get(super.to_s.demodulize)
end
def presubscription_plan
return nil if super.nil?
Payment.const_get(super.to_s.demodulize)
end
end
The current implementation works but I'm not sure this is the best way to tackle the problem
ruby ruby-on-rails mongodb
bumped to the homepage by Community♦ 5 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
up vote
1
down vote
favorite
Assume my users can subscribe to several plan_types. I want to define those plans as classes, and be able to keep, for each user, a reference to a subscription_plan and also a presubscription_plan. This is like a State pattern :
class Payment::SubscriptionPlan
def self.max_subscription_quantity
120
end
end
class Payment::ConcretePlan1 < Payment::SubscriptionPlan
def self.commitment_period
2.months
end
end
class Payment::ConcretePlan2 < Payment::SubscriptionPlan
def self.max_subscription_quantity
50
end
end
What is the best way to "persist" the relation to a class in the database ? Currently I was doing the following using the as_enum
gem
class Settings
setting :subscription_plans, type: Array, default: [
Payment::ConcretePlan1,
Payment::ConcretePlan2
]
end
class User
as_enum :subscription_plan, Settings.subscription_plans, map: :string
as_enum :presubscription_plan, Settings.subscription_plans, map: :string
def subscription_plan
return nil if super.nil?
Payment.const_get(super.to_s.demodulize)
end
def presubscription_plan
return nil if super.nil?
Payment.const_get(super.to_s.demodulize)
end
end
The current implementation works but I'm not sure this is the best way to tackle the problem
ruby ruby-on-rails mongodb
bumped to the homepage by Community♦ 5 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
ConcretePlan1 has a subscription period where as the other plans do not. Shouldn't all plans also have a subscription period? If these classes are just placeholders for returning a particular value, you can have that same functionality?
– BKSpurgeon
May 19 '17 at 13:21
Some payment systems involve automatic payment where I need to specify a period (eg. Stripe), whereas some other subscriptions are managed from the outside. I have factories to handle the subscriptions but I need to save the type of subscription for that :D And actually no commitment_period is a bit different than the subscription period : for monthly payment, our offer stipulates that you have to pay for at least 3 months when you start a subscription. When paying yearly, no such commitment period apply.
– Cyril Duchon-Doris
May 19 '17 at 13:27
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
Assume my users can subscribe to several plan_types. I want to define those plans as classes, and be able to keep, for each user, a reference to a subscription_plan and also a presubscription_plan. This is like a State pattern :
class Payment::SubscriptionPlan
def self.max_subscription_quantity
120
end
end
class Payment::ConcretePlan1 < Payment::SubscriptionPlan
def self.commitment_period
2.months
end
end
class Payment::ConcretePlan2 < Payment::SubscriptionPlan
def self.max_subscription_quantity
50
end
end
What is the best way to "persist" the relation to a class in the database ? Currently I was doing the following using the as_enum
gem
class Settings
setting :subscription_plans, type: Array, default: [
Payment::ConcretePlan1,
Payment::ConcretePlan2
]
end
class User
as_enum :subscription_plan, Settings.subscription_plans, map: :string
as_enum :presubscription_plan, Settings.subscription_plans, map: :string
def subscription_plan
return nil if super.nil?
Payment.const_get(super.to_s.demodulize)
end
def presubscription_plan
return nil if super.nil?
Payment.const_get(super.to_s.demodulize)
end
end
The current implementation works but I'm not sure this is the best way to tackle the problem
ruby ruby-on-rails mongodb
Assume my users can subscribe to several plan_types. I want to define those plans as classes, and be able to keep, for each user, a reference to a subscription_plan and also a presubscription_plan. This is like a State pattern :
class Payment::SubscriptionPlan
def self.max_subscription_quantity
120
end
end
class Payment::ConcretePlan1 < Payment::SubscriptionPlan
def self.commitment_period
2.months
end
end
class Payment::ConcretePlan2 < Payment::SubscriptionPlan
def self.max_subscription_quantity
50
end
end
What is the best way to "persist" the relation to a class in the database ? Currently I was doing the following using the as_enum
gem
class Settings
setting :subscription_plans, type: Array, default: [
Payment::ConcretePlan1,
Payment::ConcretePlan2
]
end
class User
as_enum :subscription_plan, Settings.subscription_plans, map: :string
as_enum :presubscription_plan, Settings.subscription_plans, map: :string
def subscription_plan
return nil if super.nil?
Payment.const_get(super.to_s.demodulize)
end
def presubscription_plan
return nil if super.nil?
Payment.const_get(super.to_s.demodulize)
end
end
The current implementation works but I'm not sure this is the best way to tackle the problem
ruby ruby-on-rails mongodb
ruby ruby-on-rails mongodb
edited May 17 '17 at 10:53
asked May 13 '17 at 15:11


Cyril Duchon-Doris
1114
1114
bumped to the homepage by Community♦ 5 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
bumped to the homepage by Community♦ 5 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
ConcretePlan1 has a subscription period where as the other plans do not. Shouldn't all plans also have a subscription period? If these classes are just placeholders for returning a particular value, you can have that same functionality?
– BKSpurgeon
May 19 '17 at 13:21
Some payment systems involve automatic payment where I need to specify a period (eg. Stripe), whereas some other subscriptions are managed from the outside. I have factories to handle the subscriptions but I need to save the type of subscription for that :D And actually no commitment_period is a bit different than the subscription period : for monthly payment, our offer stipulates that you have to pay for at least 3 months when you start a subscription. When paying yearly, no such commitment period apply.
– Cyril Duchon-Doris
May 19 '17 at 13:27
add a comment |
ConcretePlan1 has a subscription period where as the other plans do not. Shouldn't all plans also have a subscription period? If these classes are just placeholders for returning a particular value, you can have that same functionality?
– BKSpurgeon
May 19 '17 at 13:21
Some payment systems involve automatic payment where I need to specify a period (eg. Stripe), whereas some other subscriptions are managed from the outside. I have factories to handle the subscriptions but I need to save the type of subscription for that :D And actually no commitment_period is a bit different than the subscription period : for monthly payment, our offer stipulates that you have to pay for at least 3 months when you start a subscription. When paying yearly, no such commitment period apply.
– Cyril Duchon-Doris
May 19 '17 at 13:27
ConcretePlan1 has a subscription period where as the other plans do not. Shouldn't all plans also have a subscription period? If these classes are just placeholders for returning a particular value, you can have that same functionality?
– BKSpurgeon
May 19 '17 at 13:21
ConcretePlan1 has a subscription period where as the other plans do not. Shouldn't all plans also have a subscription period? If these classes are just placeholders for returning a particular value, you can have that same functionality?
– BKSpurgeon
May 19 '17 at 13:21
Some payment systems involve automatic payment where I need to specify a period (eg. Stripe), whereas some other subscriptions are managed from the outside. I have factories to handle the subscriptions but I need to save the type of subscription for that :D And actually no commitment_period is a bit different than the subscription period : for monthly payment, our offer stipulates that you have to pay for at least 3 months when you start a subscription. When paying yearly, no such commitment period apply.
– Cyril Duchon-Doris
May 19 '17 at 13:27
Some payment systems involve automatic payment where I need to specify a period (eg. Stripe), whereas some other subscriptions are managed from the outside. I have factories to handle the subscriptions but I need to save the type of subscription for that :D And actually no commitment_period is a bit different than the subscription period : for monthly payment, our offer stipulates that you have to pay for at least 3 months when you start a subscription. When paying yearly, no such commitment period apply.
– Cyril Duchon-Doris
May 19 '17 at 13:27
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
It works that's for sure.
But enums could just as easily be defined in the database. Now that you've already got it working with enums, I would say stick with enums untill you have a good reason to change it.
anyways, here's how i probably would have done it:
e.g.
- user has_many subscription_plans
- subscription_plan can belong to many users.
- Limit the ability for a user to have a max of two, one of each type. Or the user must have two at any one time etc.
You get the picture. You can change the subscription plan table to suit your needs.
Hope this helps.
Hey thanks. I'm using a class object on my server since I need to define methods on the subscription_plan (subscription_plan.max_quantity
, etc.) which is why I want to initialize some Ruby object where I can add whatever methods I want. I edited my example to include this. I'm not just using this as string enum.
– Cyril Duchon-Doris
May 17 '17 at 10:50
@CyrilDuchon-Doris: You should be able to still add methods and behavior specific to a kind of plan. ActiveRecord allows you to specify a "descriminator" column to instantiate a particular concrete class, giving you some of the benefits of polymorphism.
– Greg Burghardt
Jul 11 at 21:48
Also just realized the OP has a "mongo-db" tag.
– Greg Burghardt
Jul 11 at 21:58
add a comment |
up vote
0
down vote
It sounds like you really need subscription plans to be data-driven. The methods you are defining on the concrete types are really just data. No need for concrete types. You need a better database table (and I use the term "table" loosely since you tagged the question with mongodb):
| id | name | max_subscription_quantity | commitment_period_num | commitment_period_type |
| 1 | Plan 1 | 120 | 2 | Month |
| 2 | Plan 2 | 50 | NULL | NULL |
There really isn't a need for sub classes. Just one class ought to do it:
class Payment::SubscriptionPlan
def commitment_period
# return period based on type and number
end
end
The only thing you need to define is commitment_period
which does the calculation of the commitment_period_num
and commitment_period_type
columns.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
It works that's for sure.
But enums could just as easily be defined in the database. Now that you've already got it working with enums, I would say stick with enums untill you have a good reason to change it.
anyways, here's how i probably would have done it:
e.g.
- user has_many subscription_plans
- subscription_plan can belong to many users.
- Limit the ability for a user to have a max of two, one of each type. Or the user must have two at any one time etc.
You get the picture. You can change the subscription plan table to suit your needs.
Hope this helps.
Hey thanks. I'm using a class object on my server since I need to define methods on the subscription_plan (subscription_plan.max_quantity
, etc.) which is why I want to initialize some Ruby object where I can add whatever methods I want. I edited my example to include this. I'm not just using this as string enum.
– Cyril Duchon-Doris
May 17 '17 at 10:50
@CyrilDuchon-Doris: You should be able to still add methods and behavior specific to a kind of plan. ActiveRecord allows you to specify a "descriminator" column to instantiate a particular concrete class, giving you some of the benefits of polymorphism.
– Greg Burghardt
Jul 11 at 21:48
Also just realized the OP has a "mongo-db" tag.
– Greg Burghardt
Jul 11 at 21:58
add a comment |
up vote
0
down vote
It works that's for sure.
But enums could just as easily be defined in the database. Now that you've already got it working with enums, I would say stick with enums untill you have a good reason to change it.
anyways, here's how i probably would have done it:
e.g.
- user has_many subscription_plans
- subscription_plan can belong to many users.
- Limit the ability for a user to have a max of two, one of each type. Or the user must have two at any one time etc.
You get the picture. You can change the subscription plan table to suit your needs.
Hope this helps.
Hey thanks. I'm using a class object on my server since I need to define methods on the subscription_plan (subscription_plan.max_quantity
, etc.) which is why I want to initialize some Ruby object where I can add whatever methods I want. I edited my example to include this. I'm not just using this as string enum.
– Cyril Duchon-Doris
May 17 '17 at 10:50
@CyrilDuchon-Doris: You should be able to still add methods and behavior specific to a kind of plan. ActiveRecord allows you to specify a "descriminator" column to instantiate a particular concrete class, giving you some of the benefits of polymorphism.
– Greg Burghardt
Jul 11 at 21:48
Also just realized the OP has a "mongo-db" tag.
– Greg Burghardt
Jul 11 at 21:58
add a comment |
up vote
0
down vote
up vote
0
down vote
It works that's for sure.
But enums could just as easily be defined in the database. Now that you've already got it working with enums, I would say stick with enums untill you have a good reason to change it.
anyways, here's how i probably would have done it:
e.g.
- user has_many subscription_plans
- subscription_plan can belong to many users.
- Limit the ability for a user to have a max of two, one of each type. Or the user must have two at any one time etc.
You get the picture. You can change the subscription plan table to suit your needs.
Hope this helps.
It works that's for sure.
But enums could just as easily be defined in the database. Now that you've already got it working with enums, I would say stick with enums untill you have a good reason to change it.
anyways, here's how i probably would have done it:
e.g.
- user has_many subscription_plans
- subscription_plan can belong to many users.
- Limit the ability for a user to have a max of two, one of each type. Or the user must have two at any one time etc.
You get the picture. You can change the subscription plan table to suit your needs.
Hope this helps.
answered May 17 '17 at 4:10
BKSpurgeon
96129
96129
Hey thanks. I'm using a class object on my server since I need to define methods on the subscription_plan (subscription_plan.max_quantity
, etc.) which is why I want to initialize some Ruby object where I can add whatever methods I want. I edited my example to include this. I'm not just using this as string enum.
– Cyril Duchon-Doris
May 17 '17 at 10:50
@CyrilDuchon-Doris: You should be able to still add methods and behavior specific to a kind of plan. ActiveRecord allows you to specify a "descriminator" column to instantiate a particular concrete class, giving you some of the benefits of polymorphism.
– Greg Burghardt
Jul 11 at 21:48
Also just realized the OP has a "mongo-db" tag.
– Greg Burghardt
Jul 11 at 21:58
add a comment |
Hey thanks. I'm using a class object on my server since I need to define methods on the subscription_plan (subscription_plan.max_quantity
, etc.) which is why I want to initialize some Ruby object where I can add whatever methods I want. I edited my example to include this. I'm not just using this as string enum.
– Cyril Duchon-Doris
May 17 '17 at 10:50
@CyrilDuchon-Doris: You should be able to still add methods and behavior specific to a kind of plan. ActiveRecord allows you to specify a "descriminator" column to instantiate a particular concrete class, giving you some of the benefits of polymorphism.
– Greg Burghardt
Jul 11 at 21:48
Also just realized the OP has a "mongo-db" tag.
– Greg Burghardt
Jul 11 at 21:58
Hey thanks. I'm using a class object on my server since I need to define methods on the subscription_plan (
subscription_plan.max_quantity
, etc.) which is why I want to initialize some Ruby object where I can add whatever methods I want. I edited my example to include this. I'm not just using this as string enum.– Cyril Duchon-Doris
May 17 '17 at 10:50
Hey thanks. I'm using a class object on my server since I need to define methods on the subscription_plan (
subscription_plan.max_quantity
, etc.) which is why I want to initialize some Ruby object where I can add whatever methods I want. I edited my example to include this. I'm not just using this as string enum.– Cyril Duchon-Doris
May 17 '17 at 10:50
@CyrilDuchon-Doris: You should be able to still add methods and behavior specific to a kind of plan. ActiveRecord allows you to specify a "descriminator" column to instantiate a particular concrete class, giving you some of the benefits of polymorphism.
– Greg Burghardt
Jul 11 at 21:48
@CyrilDuchon-Doris: You should be able to still add methods and behavior specific to a kind of plan. ActiveRecord allows you to specify a "descriminator" column to instantiate a particular concrete class, giving you some of the benefits of polymorphism.
– Greg Burghardt
Jul 11 at 21:48
Also just realized the OP has a "mongo-db" tag.
– Greg Burghardt
Jul 11 at 21:58
Also just realized the OP has a "mongo-db" tag.
– Greg Burghardt
Jul 11 at 21:58
add a comment |
up vote
0
down vote
It sounds like you really need subscription plans to be data-driven. The methods you are defining on the concrete types are really just data. No need for concrete types. You need a better database table (and I use the term "table" loosely since you tagged the question with mongodb):
| id | name | max_subscription_quantity | commitment_period_num | commitment_period_type |
| 1 | Plan 1 | 120 | 2 | Month |
| 2 | Plan 2 | 50 | NULL | NULL |
There really isn't a need for sub classes. Just one class ought to do it:
class Payment::SubscriptionPlan
def commitment_period
# return period based on type and number
end
end
The only thing you need to define is commitment_period
which does the calculation of the commitment_period_num
and commitment_period_type
columns.
add a comment |
up vote
0
down vote
It sounds like you really need subscription plans to be data-driven. The methods you are defining on the concrete types are really just data. No need for concrete types. You need a better database table (and I use the term "table" loosely since you tagged the question with mongodb):
| id | name | max_subscription_quantity | commitment_period_num | commitment_period_type |
| 1 | Plan 1 | 120 | 2 | Month |
| 2 | Plan 2 | 50 | NULL | NULL |
There really isn't a need for sub classes. Just one class ought to do it:
class Payment::SubscriptionPlan
def commitment_period
# return period based on type and number
end
end
The only thing you need to define is commitment_period
which does the calculation of the commitment_period_num
and commitment_period_type
columns.
add a comment |
up vote
0
down vote
up vote
0
down vote
It sounds like you really need subscription plans to be data-driven. The methods you are defining on the concrete types are really just data. No need for concrete types. You need a better database table (and I use the term "table" loosely since you tagged the question with mongodb):
| id | name | max_subscription_quantity | commitment_period_num | commitment_period_type |
| 1 | Plan 1 | 120 | 2 | Month |
| 2 | Plan 2 | 50 | NULL | NULL |
There really isn't a need for sub classes. Just one class ought to do it:
class Payment::SubscriptionPlan
def commitment_period
# return period based on type and number
end
end
The only thing you need to define is commitment_period
which does the calculation of the commitment_period_num
and commitment_period_type
columns.
It sounds like you really need subscription plans to be data-driven. The methods you are defining on the concrete types are really just data. No need for concrete types. You need a better database table (and I use the term "table" loosely since you tagged the question with mongodb):
| id | name | max_subscription_quantity | commitment_period_num | commitment_period_type |
| 1 | Plan 1 | 120 | 2 | Month |
| 2 | Plan 2 | 50 | NULL | NULL |
There really isn't a need for sub classes. Just one class ought to do it:
class Payment::SubscriptionPlan
def commitment_period
# return period based on type and number
end
end
The only thing you need to define is commitment_period
which does the calculation of the commitment_period_num
and commitment_period_type
columns.
answered Jul 11 at 21:58
Greg Burghardt
4,746619
4,746619
add a comment |
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f163259%2fpersist-relation-to-a-class-and-not-a-class-instance-in-ruby-on-rails-with-mon%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pafDk,ubIk3 0FwHidzW QZ1cb88D5WsBozQZHHNxB89vd54R AO8x,MHAj8JiXJ9vrcckV,mM
ConcretePlan1 has a subscription period where as the other plans do not. Shouldn't all plans also have a subscription period? If these classes are just placeholders for returning a particular value, you can have that same functionality?
– BKSpurgeon
May 19 '17 at 13:21
Some payment systems involve automatic payment where I need to specify a period (eg. Stripe), whereas some other subscriptions are managed from the outside. I have factories to handle the subscriptions but I need to save the type of subscription for that :D And actually no commitment_period is a bit different than the subscription period : for monthly payment, our offer stipulates that you have to pay for at least 3 months when you start a subscription. When paying yearly, no such commitment period apply.
– Cyril Duchon-Doris
May 19 '17 at 13:27