Javascript while loop count higher than expected
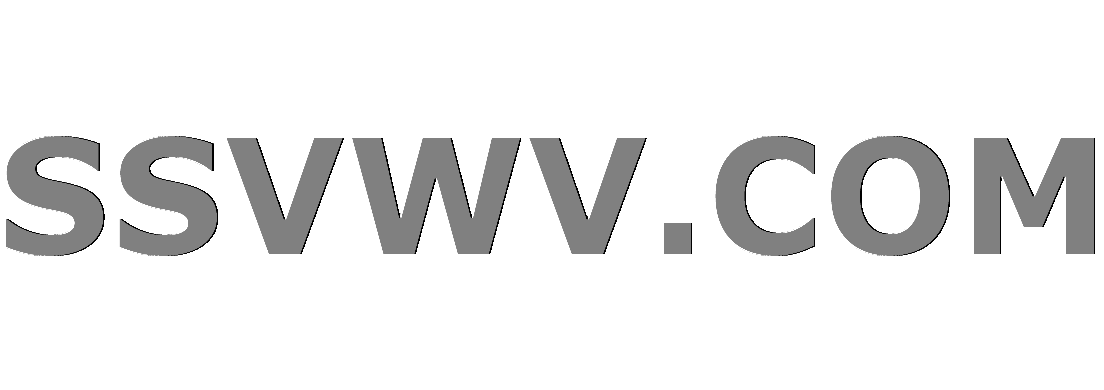
Multi tool use
up vote
0
down vote
favorite
I'm writing a function which takes in a positive number and returns the number of times you must multiply the digits in num until you reach a single digit.
example:
test(39) === 3 // because 3*9 = 27, 2*7 = 14, 1*4=4
// and 4 has only one digit//if single digit return num
The code:
function test(num) {
if (num > 10) { return num} else {
var j = num;var a;var count = 0;
while ( j > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
j--;
}
return count;
}}
test(39) //outputs 29 expected 3;
I have fixed the above by adding an array and filtering for unique values but would still like to know why the code is giving me a much higher count than expected.
javascript
add a comment |
up vote
0
down vote
favorite
I'm writing a function which takes in a positive number and returns the number of times you must multiply the digits in num until you reach a single digit.
example:
test(39) === 3 // because 3*9 = 27, 2*7 = 14, 1*4=4
// and 4 has only one digit//if single digit return num
The code:
function test(num) {
if (num > 10) { return num} else {
var j = num;var a;var count = 0;
while ( j > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
j--;
}
return count;
}}
test(39) //outputs 29 expected 3;
I have fixed the above by adding an array and filtering for unique values but would still like to know why the code is giving me a much higher count than expected.
javascript
1
Ifnum
is already below 10, shouldn't it return0
and not the number itself? I mean you describe your function as returning the number of iterations, not a result from a calculation.
– Lennholm
Nov 20 at 10:21
2
Thej--
part is the bit that's making the result far too high. You can addconsole.log(a,num,j)
inside the while to see what it's doing (or step through with the debugger). Alsoreturn num
at the start makes no sense, should bereturn 0
as num<10 means it's already single digit so doesn't need to run your algorithm.
– freedomn-m
Nov 20 at 10:24
You're right,dunno why i even put that j part and forgot about it.Works without it.
– dan brown
Nov 20 at 11:44
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm writing a function which takes in a positive number and returns the number of times you must multiply the digits in num until you reach a single digit.
example:
test(39) === 3 // because 3*9 = 27, 2*7 = 14, 1*4=4
// and 4 has only one digit//if single digit return num
The code:
function test(num) {
if (num > 10) { return num} else {
var j = num;var a;var count = 0;
while ( j > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
j--;
}
return count;
}}
test(39) //outputs 29 expected 3;
I have fixed the above by adding an array and filtering for unique values but would still like to know why the code is giving me a much higher count than expected.
javascript
I'm writing a function which takes in a positive number and returns the number of times you must multiply the digits in num until you reach a single digit.
example:
test(39) === 3 // because 3*9 = 27, 2*7 = 14, 1*4=4
// and 4 has only one digit//if single digit return num
The code:
function test(num) {
if (num > 10) { return num} else {
var j = num;var a;var count = 0;
while ( j > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
j--;
}
return count;
}}
test(39) //outputs 29 expected 3;
I have fixed the above by adding an array and filtering for unique values but would still like to know why the code is giving me a much higher count than expected.
javascript
javascript
edited Nov 20 at 11:41
asked Nov 20 at 10:12
dan brown
5616
5616
1
Ifnum
is already below 10, shouldn't it return0
and not the number itself? I mean you describe your function as returning the number of iterations, not a result from a calculation.
– Lennholm
Nov 20 at 10:21
2
Thej--
part is the bit that's making the result far too high. You can addconsole.log(a,num,j)
inside the while to see what it's doing (or step through with the debugger). Alsoreturn num
at the start makes no sense, should bereturn 0
as num<10 means it's already single digit so doesn't need to run your algorithm.
– freedomn-m
Nov 20 at 10:24
You're right,dunno why i even put that j part and forgot about it.Works without it.
– dan brown
Nov 20 at 11:44
add a comment |
1
Ifnum
is already below 10, shouldn't it return0
and not the number itself? I mean you describe your function as returning the number of iterations, not a result from a calculation.
– Lennholm
Nov 20 at 10:21
2
Thej--
part is the bit that's making the result far too high. You can addconsole.log(a,num,j)
inside the while to see what it's doing (or step through with the debugger). Alsoreturn num
at the start makes no sense, should bereturn 0
as num<10 means it's already single digit so doesn't need to run your algorithm.
– freedomn-m
Nov 20 at 10:24
You're right,dunno why i even put that j part and forgot about it.Works without it.
– dan brown
Nov 20 at 11:44
1
1
If
num
is already below 10, shouldn't it return 0
and not the number itself? I mean you describe your function as returning the number of iterations, not a result from a calculation.– Lennholm
Nov 20 at 10:21
If
num
is already below 10, shouldn't it return 0
and not the number itself? I mean you describe your function as returning the number of iterations, not a result from a calculation.– Lennholm
Nov 20 at 10:21
2
2
The
j--
part is the bit that's making the result far too high. You can add console.log(a,num,j)
inside the while to see what it's doing (or step through with the debugger). Also return num
at the start makes no sense, should be return 0
as num<10 means it's already single digit so doesn't need to run your algorithm.– freedomn-m
Nov 20 at 10:24
The
j--
part is the bit that's making the result far too high. You can add console.log(a,num,j)
inside the while to see what it's doing (or step through with the debugger). Also return num
at the start makes no sense, should be return 0
as num<10 means it's already single digit so doesn't need to run your algorithm.– freedomn-m
Nov 20 at 10:24
You're right,dunno why i even put that j part and forgot about it.Works without it.
– dan brown
Nov 20 at 11:44
You're right,dunno why i even put that j part and forgot about it.Works without it.
– dan brown
Nov 20 at 11:44
add a comment |
4 Answers
4
active
oldest
votes
up vote
2
down vote
accepted
You might be looking to use recursion here - if the num
is smaller than 10
, return 0, otherwise, perform the needed multiplication, and then return 1 +
the result of calling test
on the product:
function test(num) {
return num < 10
? 0
: 1 + test(
num.toString().split('').reduce((a, c) => a * c)
)
}
console.log(test(39));
(note that *
will coerce strings to numbers already, no need for parseInt
)
What happened toif (num < 10)
{ return num }
?
– Peter B
Nov 20 at 10:22
return num
doesn't make sense if OP is trying to figure out the number of iterations required to produce a single digit number
– CertainPerformance
Nov 20 at 10:23
@PeterB that's handled with the?:
=if (num<10) return 0;
– freedomn-m
Nov 20 at 10:26
True, description by OP does not seem to match what they tried.
– Peter B
Nov 20 at 10:28
Thanks guys.This works.Also the > sign in the first line was a type,now corrected
– dan brown
Nov 20 at 11:42
add a comment |
up vote
1
down vote
Correction in your code, you can compare
function test(num) {
if (num < 10) { return num} else {
var a;var count = 0;
while ( num > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
}
return count;
}}
test(39) //outputs 3;
add a comment |
up vote
0
down vote
You're decrementing j, which is initially 39. It will reach 10, then it will return the number of times it was decremented (29 times, which coincides with your result). You need to do the following, assuming the split part is correct:
function test(num) {
if (num < 10) { return num} else {
var j = num;var a = 11; // just to enter the while loop at least once
var count = 0;
while ( a > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
j--;
}
return count;
}}
add a comment |
up vote
0
down vote
You decremented your num
by 1 each iteration. It gives you 29
because 39 - 10
is 29
. You didn't update the actual num
which you need to compare if it's still greater than 10.
Do this instead:
function test(num) {
if (num < 10) {
return num
} else {
var count = 0;
while (num > 10) {
num = num.toString().split('').map(e => parseInt(e)).reduce((a, c) => a * c);
count++;
}
return count;
}
}
console.log(test(39))
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You might be looking to use recursion here - if the num
is smaller than 10
, return 0, otherwise, perform the needed multiplication, and then return 1 +
the result of calling test
on the product:
function test(num) {
return num < 10
? 0
: 1 + test(
num.toString().split('').reduce((a, c) => a * c)
)
}
console.log(test(39));
(note that *
will coerce strings to numbers already, no need for parseInt
)
What happened toif (num < 10)
{ return num }
?
– Peter B
Nov 20 at 10:22
return num
doesn't make sense if OP is trying to figure out the number of iterations required to produce a single digit number
– CertainPerformance
Nov 20 at 10:23
@PeterB that's handled with the?:
=if (num<10) return 0;
– freedomn-m
Nov 20 at 10:26
True, description by OP does not seem to match what they tried.
– Peter B
Nov 20 at 10:28
Thanks guys.This works.Also the > sign in the first line was a type,now corrected
– dan brown
Nov 20 at 11:42
add a comment |
up vote
2
down vote
accepted
You might be looking to use recursion here - if the num
is smaller than 10
, return 0, otherwise, perform the needed multiplication, and then return 1 +
the result of calling test
on the product:
function test(num) {
return num < 10
? 0
: 1 + test(
num.toString().split('').reduce((a, c) => a * c)
)
}
console.log(test(39));
(note that *
will coerce strings to numbers already, no need for parseInt
)
What happened toif (num < 10)
{ return num }
?
– Peter B
Nov 20 at 10:22
return num
doesn't make sense if OP is trying to figure out the number of iterations required to produce a single digit number
– CertainPerformance
Nov 20 at 10:23
@PeterB that's handled with the?:
=if (num<10) return 0;
– freedomn-m
Nov 20 at 10:26
True, description by OP does not seem to match what they tried.
– Peter B
Nov 20 at 10:28
Thanks guys.This works.Also the > sign in the first line was a type,now corrected
– dan brown
Nov 20 at 11:42
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You might be looking to use recursion here - if the num
is smaller than 10
, return 0, otherwise, perform the needed multiplication, and then return 1 +
the result of calling test
on the product:
function test(num) {
return num < 10
? 0
: 1 + test(
num.toString().split('').reduce((a, c) => a * c)
)
}
console.log(test(39));
(note that *
will coerce strings to numbers already, no need for parseInt
)
You might be looking to use recursion here - if the num
is smaller than 10
, return 0, otherwise, perform the needed multiplication, and then return 1 +
the result of calling test
on the product:
function test(num) {
return num < 10
? 0
: 1 + test(
num.toString().split('').reduce((a, c) => a * c)
)
}
console.log(test(39));
(note that *
will coerce strings to numbers already, no need for parseInt
)
function test(num) {
return num < 10
? 0
: 1 + test(
num.toString().split('').reduce((a, c) => a * c)
)
}
console.log(test(39));
function test(num) {
return num < 10
? 0
: 1 + test(
num.toString().split('').reduce((a, c) => a * c)
)
}
console.log(test(39));
answered Nov 20 at 10:15
CertainPerformance
71.3k143453
71.3k143453
What happened toif (num < 10)
{ return num }
?
– Peter B
Nov 20 at 10:22
return num
doesn't make sense if OP is trying to figure out the number of iterations required to produce a single digit number
– CertainPerformance
Nov 20 at 10:23
@PeterB that's handled with the?:
=if (num<10) return 0;
– freedomn-m
Nov 20 at 10:26
True, description by OP does not seem to match what they tried.
– Peter B
Nov 20 at 10:28
Thanks guys.This works.Also the > sign in the first line was a type,now corrected
– dan brown
Nov 20 at 11:42
add a comment |
What happened toif (num < 10)
{ return num }
?
– Peter B
Nov 20 at 10:22
return num
doesn't make sense if OP is trying to figure out the number of iterations required to produce a single digit number
– CertainPerformance
Nov 20 at 10:23
@PeterB that's handled with the?:
=if (num<10) return 0;
– freedomn-m
Nov 20 at 10:26
True, description by OP does not seem to match what they tried.
– Peter B
Nov 20 at 10:28
Thanks guys.This works.Also the > sign in the first line was a type,now corrected
– dan brown
Nov 20 at 11:42
What happened to
if (num < 10)
{ return num }
?– Peter B
Nov 20 at 10:22
What happened to
if (num < 10)
{ return num }
?– Peter B
Nov 20 at 10:22
return num
doesn't make sense if OP is trying to figure out the number of iterations required to produce a single digit number– CertainPerformance
Nov 20 at 10:23
return num
doesn't make sense if OP is trying to figure out the number of iterations required to produce a single digit number– CertainPerformance
Nov 20 at 10:23
@PeterB that's handled with the
?:
= if (num<10) return 0;
– freedomn-m
Nov 20 at 10:26
@PeterB that's handled with the
?:
= if (num<10) return 0;
– freedomn-m
Nov 20 at 10:26
True, description by OP does not seem to match what they tried.
– Peter B
Nov 20 at 10:28
True, description by OP does not seem to match what they tried.
– Peter B
Nov 20 at 10:28
Thanks guys.This works.Also the > sign in the first line was a type,now corrected
– dan brown
Nov 20 at 11:42
Thanks guys.This works.Also the > sign in the first line was a type,now corrected
– dan brown
Nov 20 at 11:42
add a comment |
up vote
1
down vote
Correction in your code, you can compare
function test(num) {
if (num < 10) { return num} else {
var a;var count = 0;
while ( num > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
}
return count;
}}
test(39) //outputs 3;
add a comment |
up vote
1
down vote
Correction in your code, you can compare
function test(num) {
if (num < 10) { return num} else {
var a;var count = 0;
while ( num > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
}
return count;
}}
test(39) //outputs 3;
add a comment |
up vote
1
down vote
up vote
1
down vote
Correction in your code, you can compare
function test(num) {
if (num < 10) { return num} else {
var a;var count = 0;
while ( num > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
}
return count;
}}
test(39) //outputs 3;
Correction in your code, you can compare
function test(num) {
if (num < 10) { return num} else {
var a;var count = 0;
while ( num > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
}
return count;
}}
test(39) //outputs 3;
answered Nov 20 at 10:21


Anubrij Chandra
7311719
7311719
add a comment |
add a comment |
up vote
0
down vote
You're decrementing j, which is initially 39. It will reach 10, then it will return the number of times it was decremented (29 times, which coincides with your result). You need to do the following, assuming the split part is correct:
function test(num) {
if (num < 10) { return num} else {
var j = num;var a = 11; // just to enter the while loop at least once
var count = 0;
while ( a > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
j--;
}
return count;
}}
add a comment |
up vote
0
down vote
You're decrementing j, which is initially 39. It will reach 10, then it will return the number of times it was decremented (29 times, which coincides with your result). You need to do the following, assuming the split part is correct:
function test(num) {
if (num < 10) { return num} else {
var j = num;var a = 11; // just to enter the while loop at least once
var count = 0;
while ( a > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
j--;
}
return count;
}}
add a comment |
up vote
0
down vote
up vote
0
down vote
You're decrementing j, which is initially 39. It will reach 10, then it will return the number of times it was decremented (29 times, which coincides with your result). You need to do the following, assuming the split part is correct:
function test(num) {
if (num < 10) { return num} else {
var j = num;var a = 11; // just to enter the while loop at least once
var count = 0;
while ( a > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
j--;
}
return count;
}}
You're decrementing j, which is initially 39. It will reach 10, then it will return the number of times it was decremented (29 times, which coincides with your result). You need to do the following, assuming the split part is correct:
function test(num) {
if (num < 10) { return num} else {
var j = num;var a = 11; // just to enter the while loop at least once
var count = 0;
while ( a > 10){
a = num.toString().split('').map( e=> parseInt(e)).reduce((a,c)=> a*c);
num = a;
count++;
j--;
}
return count;
}}
answered Nov 20 at 10:24
Bogdan
225
225
add a comment |
add a comment |
up vote
0
down vote
You decremented your num
by 1 each iteration. It gives you 29
because 39 - 10
is 29
. You didn't update the actual num
which you need to compare if it's still greater than 10.
Do this instead:
function test(num) {
if (num < 10) {
return num
} else {
var count = 0;
while (num > 10) {
num = num.toString().split('').map(e => parseInt(e)).reduce((a, c) => a * c);
count++;
}
return count;
}
}
console.log(test(39))
add a comment |
up vote
0
down vote
You decremented your num
by 1 each iteration. It gives you 29
because 39 - 10
is 29
. You didn't update the actual num
which you need to compare if it's still greater than 10.
Do this instead:
function test(num) {
if (num < 10) {
return num
} else {
var count = 0;
while (num > 10) {
num = num.toString().split('').map(e => parseInt(e)).reduce((a, c) => a * c);
count++;
}
return count;
}
}
console.log(test(39))
add a comment |
up vote
0
down vote
up vote
0
down vote
You decremented your num
by 1 each iteration. It gives you 29
because 39 - 10
is 29
. You didn't update the actual num
which you need to compare if it's still greater than 10.
Do this instead:
function test(num) {
if (num < 10) {
return num
} else {
var count = 0;
while (num > 10) {
num = num.toString().split('').map(e => parseInt(e)).reduce((a, c) => a * c);
count++;
}
return count;
}
}
console.log(test(39))
You decremented your num
by 1 each iteration. It gives you 29
because 39 - 10
is 29
. You didn't update the actual num
which you need to compare if it's still greater than 10.
Do this instead:
function test(num) {
if (num < 10) {
return num
} else {
var count = 0;
while (num > 10) {
num = num.toString().split('').map(e => parseInt(e)).reduce((a, c) => a * c);
count++;
}
return count;
}
}
console.log(test(39))
function test(num) {
if (num < 10) {
return num
} else {
var count = 0;
while (num > 10) {
num = num.toString().split('').map(e => parseInt(e)).reduce((a, c) => a * c);
count++;
}
return count;
}
}
console.log(test(39))
function test(num) {
if (num < 10) {
return num
} else {
var count = 0;
while (num > 10) {
num = num.toString().split('').map(e => parseInt(e)).reduce((a, c) => a * c);
count++;
}
return count;
}
}
console.log(test(39))
answered Nov 20 at 10:39


ACD
714111
714111
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53390682%2fjavascript-while-loop-count-higher-than-expected%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
g68jQ5hW QS8l7PSD0aWnw,KX6BHqqQ8
1
If
num
is already below 10, shouldn't it return0
and not the number itself? I mean you describe your function as returning the number of iterations, not a result from a calculation.– Lennholm
Nov 20 at 10:21
2
The
j--
part is the bit that's making the result far too high. You can addconsole.log(a,num,j)
inside the while to see what it's doing (or step through with the debugger). Alsoreturn num
at the start makes no sense, should bereturn 0
as num<10 means it's already single digit so doesn't need to run your algorithm.– freedomn-m
Nov 20 at 10:24
You're right,dunno why i even put that j part and forgot about it.Works without it.
– dan brown
Nov 20 at 11:44