Java socket: two newlines faster than one newline
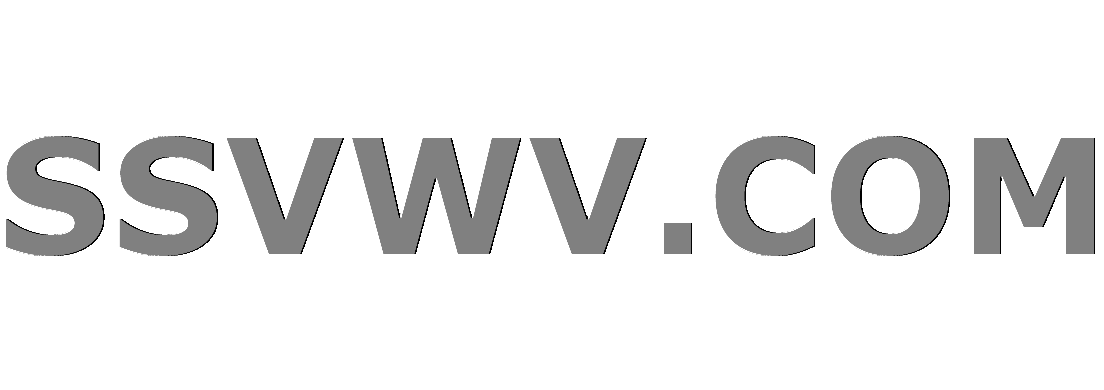
Multi tool use
up vote
3
down vote
favorite
I have a simple client-server application. Server is in Java, client in Python3. The server waits for a single client, then communicates with it for eternity in the following way. Server reads a line from the client, and the sends the message "yyyy" back. Thus, from the client side, the conversation could look something like this:
xxxx
> yyyy
xxxx
> yyyy
xxxx
> yyyy
...
The problem I have is with the speed of the communication. I measure the time it takes the server to read the client's request, and it is about 40ms. What is most surprising though, is that if the client appends an additional newline 'n' to each of its messages, the duration drops to ~0ms. What could be the cause of this, and how can I fix it so that I do not have to include an artificial newline to each client's request?
For completeness, I also provide the server side and client side code.
Server side:
import java.io.*;
import java.net.*;
import java.time.*;
import java.util.*;
class Server {
public static void main (String args) {
Clock clock = Clock.systemDefaultZone();
try {
ServerSocket server = new ServerSocket();
server.bind(new InetSocketAddress("127.0.0.1", 4248));
Socket client = server.accept();
Scanner sc = new Scanner(client.getInputStream());
PrintStream ps = new PrintStream(client.getOutputStream());
while (true) {
Instant start = clock.instant();
String msg = sc.nextLine();
Instant end = clock.instant();
System.err.format("Took me %d ms to receive the messagen", Duration.between(start, end).getNano() / 1000000);
ps.println("yyyy");
}
}
catch (IOException exc) {}
}
}
Client side:
import socket, sys
sock = socket.create_connection(("127.0.0.1", 4248))
fin = sock.makefile('r')
fout = sock.makefile('w')
while True:
print("xxxx", file = fout, flush = True)
msg = fin.readline().rstrip('n');
print(msg, file = sys.stderr)
We obtain the "fast" client by replacing "xxxx" with "xxxxn". Note that Python3 automatically prints a newline after each message, so the the former really is "xxxxn" and the latter is "xxxxnn".
java networking
|
show 2 more comments
up vote
3
down vote
favorite
I have a simple client-server application. Server is in Java, client in Python3. The server waits for a single client, then communicates with it for eternity in the following way. Server reads a line from the client, and the sends the message "yyyy" back. Thus, from the client side, the conversation could look something like this:
xxxx
> yyyy
xxxx
> yyyy
xxxx
> yyyy
...
The problem I have is with the speed of the communication. I measure the time it takes the server to read the client's request, and it is about 40ms. What is most surprising though, is that if the client appends an additional newline 'n' to each of its messages, the duration drops to ~0ms. What could be the cause of this, and how can I fix it so that I do not have to include an artificial newline to each client's request?
For completeness, I also provide the server side and client side code.
Server side:
import java.io.*;
import java.net.*;
import java.time.*;
import java.util.*;
class Server {
public static void main (String args) {
Clock clock = Clock.systemDefaultZone();
try {
ServerSocket server = new ServerSocket();
server.bind(new InetSocketAddress("127.0.0.1", 4248));
Socket client = server.accept();
Scanner sc = new Scanner(client.getInputStream());
PrintStream ps = new PrintStream(client.getOutputStream());
while (true) {
Instant start = clock.instant();
String msg = sc.nextLine();
Instant end = clock.instant();
System.err.format("Took me %d ms to receive the messagen", Duration.between(start, end).getNano() / 1000000);
ps.println("yyyy");
}
}
catch (IOException exc) {}
}
}
Client side:
import socket, sys
sock = socket.create_connection(("127.0.0.1", 4248))
fin = sock.makefile('r')
fout = sock.makefile('w')
while True:
print("xxxx", file = fout, flush = True)
msg = fin.readline().rstrip('n');
print(msg, file = sys.stderr)
We obtain the "fast" client by replacing "xxxx" with "xxxxn". Note that Python3 automatically prints a newline after each message, so the the former really is "xxxxn" and the latter is "xxxxnn".
java networking
Are you sure you always get 0ms with the change? I expected alternation between 40ms and 0ms: 40ms, 0ms, 40ms, 0ms, 40ms, 0ms ...
– Socowi
Nov 20 at 10:09
Yes, that is what makes it so surprising. As if the socket had an issue with rapidly alternating input and output, but not so much if the ratio is 1:2 ...
– buj
Nov 20 at 10:11
Interesting enough I tested it here but with python 2 (that's what I have installed) and java 8 on a windows machine. I got no difference between xxxx and xxxxn - always 0-1ms
– Veselin Davidov
Nov 20 at 10:37
I could replicate OP's findings with Java 8 and Python 3 on an old linux laptop.
– Socowi
Nov 20 at 10:39
1
When I putprint("xxxx", ...)
beforewhile True
in the client, the measured time repeats in the following pattern: 40, 0, 0, 40, 0 0, ... With twoprints
before the loop, the pattern becomes 40, 0, 0, 0, 40, 0, 0, 0, ...
– Socowi
Nov 20 at 12:08
|
show 2 more comments
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I have a simple client-server application. Server is in Java, client in Python3. The server waits for a single client, then communicates with it for eternity in the following way. Server reads a line from the client, and the sends the message "yyyy" back. Thus, from the client side, the conversation could look something like this:
xxxx
> yyyy
xxxx
> yyyy
xxxx
> yyyy
...
The problem I have is with the speed of the communication. I measure the time it takes the server to read the client's request, and it is about 40ms. What is most surprising though, is that if the client appends an additional newline 'n' to each of its messages, the duration drops to ~0ms. What could be the cause of this, and how can I fix it so that I do not have to include an artificial newline to each client's request?
For completeness, I also provide the server side and client side code.
Server side:
import java.io.*;
import java.net.*;
import java.time.*;
import java.util.*;
class Server {
public static void main (String args) {
Clock clock = Clock.systemDefaultZone();
try {
ServerSocket server = new ServerSocket();
server.bind(new InetSocketAddress("127.0.0.1", 4248));
Socket client = server.accept();
Scanner sc = new Scanner(client.getInputStream());
PrintStream ps = new PrintStream(client.getOutputStream());
while (true) {
Instant start = clock.instant();
String msg = sc.nextLine();
Instant end = clock.instant();
System.err.format("Took me %d ms to receive the messagen", Duration.between(start, end).getNano() / 1000000);
ps.println("yyyy");
}
}
catch (IOException exc) {}
}
}
Client side:
import socket, sys
sock = socket.create_connection(("127.0.0.1", 4248))
fin = sock.makefile('r')
fout = sock.makefile('w')
while True:
print("xxxx", file = fout, flush = True)
msg = fin.readline().rstrip('n');
print(msg, file = sys.stderr)
We obtain the "fast" client by replacing "xxxx" with "xxxxn". Note that Python3 automatically prints a newline after each message, so the the former really is "xxxxn" and the latter is "xxxxnn".
java networking
I have a simple client-server application. Server is in Java, client in Python3. The server waits for a single client, then communicates with it for eternity in the following way. Server reads a line from the client, and the sends the message "yyyy" back. Thus, from the client side, the conversation could look something like this:
xxxx
> yyyy
xxxx
> yyyy
xxxx
> yyyy
...
The problem I have is with the speed of the communication. I measure the time it takes the server to read the client's request, and it is about 40ms. What is most surprising though, is that if the client appends an additional newline 'n' to each of its messages, the duration drops to ~0ms. What could be the cause of this, and how can I fix it so that I do not have to include an artificial newline to each client's request?
For completeness, I also provide the server side and client side code.
Server side:
import java.io.*;
import java.net.*;
import java.time.*;
import java.util.*;
class Server {
public static void main (String args) {
Clock clock = Clock.systemDefaultZone();
try {
ServerSocket server = new ServerSocket();
server.bind(new InetSocketAddress("127.0.0.1", 4248));
Socket client = server.accept();
Scanner sc = new Scanner(client.getInputStream());
PrintStream ps = new PrintStream(client.getOutputStream());
while (true) {
Instant start = clock.instant();
String msg = sc.nextLine();
Instant end = clock.instant();
System.err.format("Took me %d ms to receive the messagen", Duration.between(start, end).getNano() / 1000000);
ps.println("yyyy");
}
}
catch (IOException exc) {}
}
}
Client side:
import socket, sys
sock = socket.create_connection(("127.0.0.1", 4248))
fin = sock.makefile('r')
fout = sock.makefile('w')
while True:
print("xxxx", file = fout, flush = True)
msg = fin.readline().rstrip('n');
print(msg, file = sys.stderr)
We obtain the "fast" client by replacing "xxxx" with "xxxxn". Note that Python3 automatically prints a newline after each message, so the the former really is "xxxxn" and the latter is "xxxxnn".
java networking
java networking
edited Nov 20 at 10:21
asked Nov 20 at 10:03


buj
335
335
Are you sure you always get 0ms with the change? I expected alternation between 40ms and 0ms: 40ms, 0ms, 40ms, 0ms, 40ms, 0ms ...
– Socowi
Nov 20 at 10:09
Yes, that is what makes it so surprising. As if the socket had an issue with rapidly alternating input and output, but not so much if the ratio is 1:2 ...
– buj
Nov 20 at 10:11
Interesting enough I tested it here but with python 2 (that's what I have installed) and java 8 on a windows machine. I got no difference between xxxx and xxxxn - always 0-1ms
– Veselin Davidov
Nov 20 at 10:37
I could replicate OP's findings with Java 8 and Python 3 on an old linux laptop.
– Socowi
Nov 20 at 10:39
1
When I putprint("xxxx", ...)
beforewhile True
in the client, the measured time repeats in the following pattern: 40, 0, 0, 40, 0 0, ... With twoprints
before the loop, the pattern becomes 40, 0, 0, 0, 40, 0, 0, 0, ...
– Socowi
Nov 20 at 12:08
|
show 2 more comments
Are you sure you always get 0ms with the change? I expected alternation between 40ms and 0ms: 40ms, 0ms, 40ms, 0ms, 40ms, 0ms ...
– Socowi
Nov 20 at 10:09
Yes, that is what makes it so surprising. As if the socket had an issue with rapidly alternating input and output, but not so much if the ratio is 1:2 ...
– buj
Nov 20 at 10:11
Interesting enough I tested it here but with python 2 (that's what I have installed) and java 8 on a windows machine. I got no difference between xxxx and xxxxn - always 0-1ms
– Veselin Davidov
Nov 20 at 10:37
I could replicate OP's findings with Java 8 and Python 3 on an old linux laptop.
– Socowi
Nov 20 at 10:39
1
When I putprint("xxxx", ...)
beforewhile True
in the client, the measured time repeats in the following pattern: 40, 0, 0, 40, 0 0, ... With twoprints
before the loop, the pattern becomes 40, 0, 0, 0, 40, 0, 0, 0, ...
– Socowi
Nov 20 at 12:08
Are you sure you always get 0ms with the change? I expected alternation between 40ms and 0ms: 40ms, 0ms, 40ms, 0ms, 40ms, 0ms ...
– Socowi
Nov 20 at 10:09
Are you sure you always get 0ms with the change? I expected alternation between 40ms and 0ms: 40ms, 0ms, 40ms, 0ms, 40ms, 0ms ...
– Socowi
Nov 20 at 10:09
Yes, that is what makes it so surprising. As if the socket had an issue with rapidly alternating input and output, but not so much if the ratio is 1:2 ...
– buj
Nov 20 at 10:11
Yes, that is what makes it so surprising. As if the socket had an issue with rapidly alternating input and output, but not so much if the ratio is 1:2 ...
– buj
Nov 20 at 10:11
Interesting enough I tested it here but with python 2 (that's what I have installed) and java 8 on a windows machine. I got no difference between xxxx and xxxxn - always 0-1ms
– Veselin Davidov
Nov 20 at 10:37
Interesting enough I tested it here but with python 2 (that's what I have installed) and java 8 on a windows machine. I got no difference between xxxx and xxxxn - always 0-1ms
– Veselin Davidov
Nov 20 at 10:37
I could replicate OP's findings with Java 8 and Python 3 on an old linux laptop.
– Socowi
Nov 20 at 10:39
I could replicate OP's findings with Java 8 and Python 3 on an old linux laptop.
– Socowi
Nov 20 at 10:39
1
1
When I put
print("xxxx", ...)
before while True
in the client, the measured time repeats in the following pattern: 40, 0, 0, 40, 0 0, ... With two prints
before the loop, the pattern becomes 40, 0, 0, 0, 40, 0, 0, 0, ...– Socowi
Nov 20 at 12:08
When I put
print("xxxx", ...)
before while True
in the client, the measured time repeats in the following pattern: 40, 0, 0, 40, 0 0, ... With two prints
before the loop, the pattern becomes 40, 0, 0, 0, 40, 0, 0, 0, ...– Socowi
Nov 20 at 12:08
|
show 2 more comments
1 Answer
1
active
oldest
votes
up vote
0
down vote
I measure the time it takes the server to read the client's request
Not only that, but you also measure (at least partially) the time until the client sends its request.
Just a guess
The Python client is the bottle neck and cannot keep up with the Java server. The server's nextLine()
always waits for the client to print something.
When you change print("xxxx")
to print("xxxxn")
two lines are printed at once, which doubles the line output rate of the client. Now it's the other way around: The client sends messages faster than the server can process them and the client's readline()
has to wait for the server.
Note that in the latter case, the messages alternate between "xxxx"
and ""
. That's probably not what you want. However, you could use print("xxxxnxxxx")
.
You could test this theory by writing a Java client that uses println("xxxx")
. Assuming the Java client is faster than the python client, I would expect the measured time to be less than 40ms but maybe a bit more than 0ms.
I have just tested this (with Java 8 both client and server). With a single newline, it is exactly two times slower (80ms). With two newlines, it's still 0ms super-fast. The thing is, even if the client was slower than the server, it would not explain why processing "xxxx" and then "" is faster than processing "xxxx" only.
– buj
Nov 20 at 10:54
Then I must have guessed wrong. To clear up a possible misunderstanding:why processing "xxxx" and then "" is faster than processing "xxxx"
. I did not talk about the time it takes to process something on the server, but rather the time the server spends waiting for input, that is the time the client takes to send something. Withprint("xxxxn")
two lines per loop iteration are sent; withprint("xxxx")
only one line is sent – but both calls should take around the same time. Also withxxxxn
the next input""
for the server is already there before the server even sent its reply.
– Socowi
Nov 20 at 12:03
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53390519%2fjava-socket-two-newlines-faster-than-one-newline%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
I measure the time it takes the server to read the client's request
Not only that, but you also measure (at least partially) the time until the client sends its request.
Just a guess
The Python client is the bottle neck and cannot keep up with the Java server. The server's nextLine()
always waits for the client to print something.
When you change print("xxxx")
to print("xxxxn")
two lines are printed at once, which doubles the line output rate of the client. Now it's the other way around: The client sends messages faster than the server can process them and the client's readline()
has to wait for the server.
Note that in the latter case, the messages alternate between "xxxx"
and ""
. That's probably not what you want. However, you could use print("xxxxnxxxx")
.
You could test this theory by writing a Java client that uses println("xxxx")
. Assuming the Java client is faster than the python client, I would expect the measured time to be less than 40ms but maybe a bit more than 0ms.
I have just tested this (with Java 8 both client and server). With a single newline, it is exactly two times slower (80ms). With two newlines, it's still 0ms super-fast. The thing is, even if the client was slower than the server, it would not explain why processing "xxxx" and then "" is faster than processing "xxxx" only.
– buj
Nov 20 at 10:54
Then I must have guessed wrong. To clear up a possible misunderstanding:why processing "xxxx" and then "" is faster than processing "xxxx"
. I did not talk about the time it takes to process something on the server, but rather the time the server spends waiting for input, that is the time the client takes to send something. Withprint("xxxxn")
two lines per loop iteration are sent; withprint("xxxx")
only one line is sent – but both calls should take around the same time. Also withxxxxn
the next input""
for the server is already there before the server even sent its reply.
– Socowi
Nov 20 at 12:03
add a comment |
up vote
0
down vote
I measure the time it takes the server to read the client's request
Not only that, but you also measure (at least partially) the time until the client sends its request.
Just a guess
The Python client is the bottle neck and cannot keep up with the Java server. The server's nextLine()
always waits for the client to print something.
When you change print("xxxx")
to print("xxxxn")
two lines are printed at once, which doubles the line output rate of the client. Now it's the other way around: The client sends messages faster than the server can process them and the client's readline()
has to wait for the server.
Note that in the latter case, the messages alternate between "xxxx"
and ""
. That's probably not what you want. However, you could use print("xxxxnxxxx")
.
You could test this theory by writing a Java client that uses println("xxxx")
. Assuming the Java client is faster than the python client, I would expect the measured time to be less than 40ms but maybe a bit more than 0ms.
I have just tested this (with Java 8 both client and server). With a single newline, it is exactly two times slower (80ms). With two newlines, it's still 0ms super-fast. The thing is, even if the client was slower than the server, it would not explain why processing "xxxx" and then "" is faster than processing "xxxx" only.
– buj
Nov 20 at 10:54
Then I must have guessed wrong. To clear up a possible misunderstanding:why processing "xxxx" and then "" is faster than processing "xxxx"
. I did not talk about the time it takes to process something on the server, but rather the time the server spends waiting for input, that is the time the client takes to send something. Withprint("xxxxn")
two lines per loop iteration are sent; withprint("xxxx")
only one line is sent – but both calls should take around the same time. Also withxxxxn
the next input""
for the server is already there before the server even sent its reply.
– Socowi
Nov 20 at 12:03
add a comment |
up vote
0
down vote
up vote
0
down vote
I measure the time it takes the server to read the client's request
Not only that, but you also measure (at least partially) the time until the client sends its request.
Just a guess
The Python client is the bottle neck and cannot keep up with the Java server. The server's nextLine()
always waits for the client to print something.
When you change print("xxxx")
to print("xxxxn")
two lines are printed at once, which doubles the line output rate of the client. Now it's the other way around: The client sends messages faster than the server can process them and the client's readline()
has to wait for the server.
Note that in the latter case, the messages alternate between "xxxx"
and ""
. That's probably not what you want. However, you could use print("xxxxnxxxx")
.
You could test this theory by writing a Java client that uses println("xxxx")
. Assuming the Java client is faster than the python client, I would expect the measured time to be less than 40ms but maybe a bit more than 0ms.
I measure the time it takes the server to read the client's request
Not only that, but you also measure (at least partially) the time until the client sends its request.
Just a guess
The Python client is the bottle neck and cannot keep up with the Java server. The server's nextLine()
always waits for the client to print something.
When you change print("xxxx")
to print("xxxxn")
two lines are printed at once, which doubles the line output rate of the client. Now it's the other way around: The client sends messages faster than the server can process them and the client's readline()
has to wait for the server.
Note that in the latter case, the messages alternate between "xxxx"
and ""
. That's probably not what you want. However, you could use print("xxxxnxxxx")
.
You could test this theory by writing a Java client that uses println("xxxx")
. Assuming the Java client is faster than the python client, I would expect the measured time to be less than 40ms but maybe a bit more than 0ms.
edited Nov 20 at 10:49
answered Nov 20 at 10:35


Socowi
5,9062724
5,9062724
I have just tested this (with Java 8 both client and server). With a single newline, it is exactly two times slower (80ms). With two newlines, it's still 0ms super-fast. The thing is, even if the client was slower than the server, it would not explain why processing "xxxx" and then "" is faster than processing "xxxx" only.
– buj
Nov 20 at 10:54
Then I must have guessed wrong. To clear up a possible misunderstanding:why processing "xxxx" and then "" is faster than processing "xxxx"
. I did not talk about the time it takes to process something on the server, but rather the time the server spends waiting for input, that is the time the client takes to send something. Withprint("xxxxn")
two lines per loop iteration are sent; withprint("xxxx")
only one line is sent – but both calls should take around the same time. Also withxxxxn
the next input""
for the server is already there before the server even sent its reply.
– Socowi
Nov 20 at 12:03
add a comment |
I have just tested this (with Java 8 both client and server). With a single newline, it is exactly two times slower (80ms). With two newlines, it's still 0ms super-fast. The thing is, even if the client was slower than the server, it would not explain why processing "xxxx" and then "" is faster than processing "xxxx" only.
– buj
Nov 20 at 10:54
Then I must have guessed wrong. To clear up a possible misunderstanding:why processing "xxxx" and then "" is faster than processing "xxxx"
. I did not talk about the time it takes to process something on the server, but rather the time the server spends waiting for input, that is the time the client takes to send something. Withprint("xxxxn")
two lines per loop iteration are sent; withprint("xxxx")
only one line is sent – but both calls should take around the same time. Also withxxxxn
the next input""
for the server is already there before the server even sent its reply.
– Socowi
Nov 20 at 12:03
I have just tested this (with Java 8 both client and server). With a single newline, it is exactly two times slower (80ms). With two newlines, it's still 0ms super-fast. The thing is, even if the client was slower than the server, it would not explain why processing "xxxx" and then "" is faster than processing "xxxx" only.
– buj
Nov 20 at 10:54
I have just tested this (with Java 8 both client and server). With a single newline, it is exactly two times slower (80ms). With two newlines, it's still 0ms super-fast. The thing is, even if the client was slower than the server, it would not explain why processing "xxxx" and then "" is faster than processing "xxxx" only.
– buj
Nov 20 at 10:54
Then I must have guessed wrong. To clear up a possible misunderstanding:
why processing "xxxx" and then "" is faster than processing "xxxx"
. I did not talk about the time it takes to process something on the server, but rather the time the server spends waiting for input, that is the time the client takes to send something. With print("xxxxn")
two lines per loop iteration are sent; with print("xxxx")
only one line is sent – but both calls should take around the same time. Also with xxxxn
the next input ""
for the server is already there before the server even sent its reply.– Socowi
Nov 20 at 12:03
Then I must have guessed wrong. To clear up a possible misunderstanding:
why processing "xxxx" and then "" is faster than processing "xxxx"
. I did not talk about the time it takes to process something on the server, but rather the time the server spends waiting for input, that is the time the client takes to send something. With print("xxxxn")
two lines per loop iteration are sent; with print("xxxx")
only one line is sent – but both calls should take around the same time. Also with xxxxn
the next input ""
for the server is already there before the server even sent its reply.– Socowi
Nov 20 at 12:03
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53390519%2fjava-socket-two-newlines-faster-than-one-newline%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Fx5si,HApGrs,OpRk CYJ0GTv3ql9UDdeaVfvmdK,Xs9EF,xDt
Are you sure you always get 0ms with the change? I expected alternation between 40ms and 0ms: 40ms, 0ms, 40ms, 0ms, 40ms, 0ms ...
– Socowi
Nov 20 at 10:09
Yes, that is what makes it so surprising. As if the socket had an issue with rapidly alternating input and output, but not so much if the ratio is 1:2 ...
– buj
Nov 20 at 10:11
Interesting enough I tested it here but with python 2 (that's what I have installed) and java 8 on a windows machine. I got no difference between xxxx and xxxxn - always 0-1ms
– Veselin Davidov
Nov 20 at 10:37
I could replicate OP's findings with Java 8 and Python 3 on an old linux laptop.
– Socowi
Nov 20 at 10:39
1
When I put
print("xxxx", ...)
beforewhile True
in the client, the measured time repeats in the following pattern: 40, 0, 0, 40, 0 0, ... With twoprints
before the loop, the pattern becomes 40, 0, 0, 0, 40, 0, 0, 0, ...– Socowi
Nov 20 at 12:08