Unit testing parallel function using moq
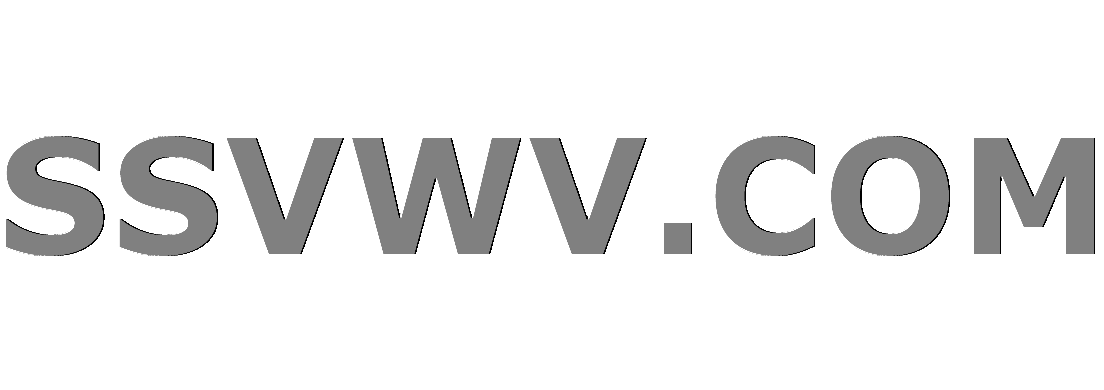
Multi tool use
up vote
0
down vote
favorite
I've got basic interface, and a class that takes it implementation in constructor, then fetches some data sequentialy or parallely depending on input.
public interface ISomeService
{
List<int> FetchSomeData(int a);
}
public class SomeClass
{
private ISomeService sr { get; }
public SomeClass(ISomeService sr) => this.sr = sr;
public int DoMagic(bool paralell)
{
int sum = 0;
if (paralell)
{
Parallel.For(0, 10, (x) =>
{
sum += sr.FetchSomeData(x).Sum(z => z);
});
}
else
{
for (int i = 0; i < 10; i++)
{
sum += sr.FetchSomeData(i).Sum(z => z);
}
}
return sum;
}
}
then i've got test:
[Test]
public void DoTest()
{
var service = new Mock<ISomeService>(MockBehavior.Strict);
service.Setup(x => x.FetchSomeData(It.IsAny<int>()))
.Returns(() => new List<int> { 3 });
var someClass = new SomeClass(service.Object);
var notParallel = someClass.DoMagic(false);
var parallel = someClass.DoMagic(true);
Assert.AreEqual(30, notParallel);
Assert.AreEqual(30, parallel);
}
And the problem:
NotParallel results are always the same ( 30 ), but in parallel case there results vary ( sometimes 15, other time 21 etc ).
What may be the problem? How to solve this ?
c# .net unit-testing mocking moq
add a comment |
up vote
0
down vote
favorite
I've got basic interface, and a class that takes it implementation in constructor, then fetches some data sequentialy or parallely depending on input.
public interface ISomeService
{
List<int> FetchSomeData(int a);
}
public class SomeClass
{
private ISomeService sr { get; }
public SomeClass(ISomeService sr) => this.sr = sr;
public int DoMagic(bool paralell)
{
int sum = 0;
if (paralell)
{
Parallel.For(0, 10, (x) =>
{
sum += sr.FetchSomeData(x).Sum(z => z);
});
}
else
{
for (int i = 0; i < 10; i++)
{
sum += sr.FetchSomeData(i).Sum(z => z);
}
}
return sum;
}
}
then i've got test:
[Test]
public void DoTest()
{
var service = new Mock<ISomeService>(MockBehavior.Strict);
service.Setup(x => x.FetchSomeData(It.IsAny<int>()))
.Returns(() => new List<int> { 3 });
var someClass = new SomeClass(service.Object);
var notParallel = someClass.DoMagic(false);
var parallel = someClass.DoMagic(true);
Assert.AreEqual(30, notParallel);
Assert.AreEqual(30, parallel);
}
And the problem:
NotParallel results are always the same ( 30 ), but in parallel case there results vary ( sometimes 15, other time 21 etc ).
What may be the problem? How to solve this ?
c# .net unit-testing mocking moq
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I've got basic interface, and a class that takes it implementation in constructor, then fetches some data sequentialy or parallely depending on input.
public interface ISomeService
{
List<int> FetchSomeData(int a);
}
public class SomeClass
{
private ISomeService sr { get; }
public SomeClass(ISomeService sr) => this.sr = sr;
public int DoMagic(bool paralell)
{
int sum = 0;
if (paralell)
{
Parallel.For(0, 10, (x) =>
{
sum += sr.FetchSomeData(x).Sum(z => z);
});
}
else
{
for (int i = 0; i < 10; i++)
{
sum += sr.FetchSomeData(i).Sum(z => z);
}
}
return sum;
}
}
then i've got test:
[Test]
public void DoTest()
{
var service = new Mock<ISomeService>(MockBehavior.Strict);
service.Setup(x => x.FetchSomeData(It.IsAny<int>()))
.Returns(() => new List<int> { 3 });
var someClass = new SomeClass(service.Object);
var notParallel = someClass.DoMagic(false);
var parallel = someClass.DoMagic(true);
Assert.AreEqual(30, notParallel);
Assert.AreEqual(30, parallel);
}
And the problem:
NotParallel results are always the same ( 30 ), but in parallel case there results vary ( sometimes 15, other time 21 etc ).
What may be the problem? How to solve this ?
c# .net unit-testing mocking moq
I've got basic interface, and a class that takes it implementation in constructor, then fetches some data sequentialy or parallely depending on input.
public interface ISomeService
{
List<int> FetchSomeData(int a);
}
public class SomeClass
{
private ISomeService sr { get; }
public SomeClass(ISomeService sr) => this.sr = sr;
public int DoMagic(bool paralell)
{
int sum = 0;
if (paralell)
{
Parallel.For(0, 10, (x) =>
{
sum += sr.FetchSomeData(x).Sum(z => z);
});
}
else
{
for (int i = 0; i < 10; i++)
{
sum += sr.FetchSomeData(i).Sum(z => z);
}
}
return sum;
}
}
then i've got test:
[Test]
public void DoTest()
{
var service = new Mock<ISomeService>(MockBehavior.Strict);
service.Setup(x => x.FetchSomeData(It.IsAny<int>()))
.Returns(() => new List<int> { 3 });
var someClass = new SomeClass(service.Object);
var notParallel = someClass.DoMagic(false);
var parallel = someClass.DoMagic(true);
Assert.AreEqual(30, notParallel);
Assert.AreEqual(30, parallel);
}
And the problem:
NotParallel results are always the same ( 30 ), but in parallel case there results vary ( sometimes 15, other time 21 etc ).
What may be the problem? How to solve this ?
c# .net unit-testing mocking moq
c# .net unit-testing mocking moq
asked Nov 19 at 17:06
Rabur
12
12
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
You are updating the variable sum from multiple threads.
Here are some options how to make this thread-safe: https://social.msdn.microsoft.com/Forums/vstudio/en-US/d87c1085-cacb-4d82-826f-4151bf967f86/parallelfor-with-sum?forum=parallelextensions
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
You are updating the variable sum from multiple threads.
Here are some options how to make this thread-safe: https://social.msdn.microsoft.com/Forums/vstudio/en-US/d87c1085-cacb-4d82-826f-4151bf967f86/parallelfor-with-sum?forum=parallelextensions
add a comment |
up vote
1
down vote
You are updating the variable sum from multiple threads.
Here are some options how to make this thread-safe: https://social.msdn.microsoft.com/Forums/vstudio/en-US/d87c1085-cacb-4d82-826f-4151bf967f86/parallelfor-with-sum?forum=parallelextensions
add a comment |
up vote
1
down vote
up vote
1
down vote
You are updating the variable sum from multiple threads.
Here are some options how to make this thread-safe: https://social.msdn.microsoft.com/Forums/vstudio/en-US/d87c1085-cacb-4d82-826f-4151bf967f86/parallelfor-with-sum?forum=parallelextensions
You are updating the variable sum from multiple threads.
Here are some options how to make this thread-safe: https://social.msdn.microsoft.com/Forums/vstudio/en-US/d87c1085-cacb-4d82-826f-4151bf967f86/parallelfor-with-sum?forum=parallelextensions
answered Nov 19 at 17:08


Klaus Gütter
1,142612
1,142612
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53379512%2funit-testing-parallel-function-using-moq%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EkVBu,iES5hVMAY8,zkDRJlOnmXUOgqa4