How to hover on list items to change map marker styling in a more efficient way?
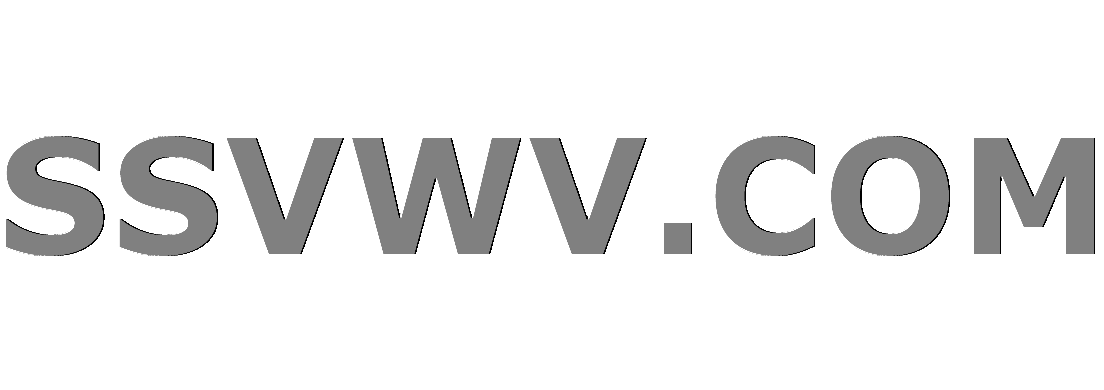
Multi tool use
up vote
0
down vote
favorite
I’m struggling to make a React/JS map I have more efficient. Essentially, I have a list of cards, and then a map of markers. When I hover on one of the cards, it changes the styling of the marker. Here’s what it looks like:
But the way I’m doing this...I’m pretty sure is really inefficient.
So here’s how I have it setup in a couple of components:
//LocationsGrid.js
class LocationsGrid extends React.Component {
state = {
hoveredCardId: ""
};
setCardMarkerHover = location => {
this.setState({
hoveredCardId: location.pageid
});
};
resetCardMarkerHover = () => {
this.setState({
hoveredCardId: ""
});
};
render() {
const { locations } = this.props;
{locations.map((location, index) => (
<LocationCard
setCardMarkerHover={this.setCardMarkerHover}
resetCardMarkerHover={this.resetCardMarkerHover}
location={location}
/>
))}
// map stuff
<div className={classes.mapDiv}>
<MapAndMarkers
locations={locations}
hoveredCardId={this.state.hoveredCardId}
/>
</div>
//LocationCard.js
class LocationCard extends React.Component {
render() {
const { classes, location } = this.props;
return (
<Card
className={classes.card}
onMouseEnter={e => this.props.setCardMarkerHover(location)}
onMouseLeave={e => this.props.resetCardMarkerHover()}
>
<CardContent className={classes.cardContentArea}>
</CardContent>
</Card>
);
}
}
// MapAndMarkers.js
class MapAndMarkers extends React.Component {
render() {
const { locations, hoveredCardId, pageid } = this.props;
let MapMarkers = locations.map((location, index) => {
return (
<MapMarker
key={location.id}
lat={location.lat}
lng={location.lng}
pageid={location.pageid}
hoveredCardId={hoveredCardId}
/>
);
});
return (
<div>
<GoogleMapReact>
{MapMarkers}
</GoogleMapReact>
</div>
);
}
}
// MapMarker.js
import classNames from 'classnames';
class MapMarker extends React.Component {
render() {
const { classes, pageid, hoveredCardId } = this.props;
return (
<div className={classes.markerParent}>
<span className={classNames(classes.tooltips_span, pageid == hoveredCardId && classes.niftyHoverBackground)}>{this.props.name}</span>
</div>
);
}
}
The problem with doing it this way is I’m pretty sure there’s a whole bunch of wasted rendering. When I pulled out the mouse events from my LocationCard, everything else runs faster.
What would be a more efficient way of tracking hovered list items so that I can change the map marker style?
Here’s the code: https://github.com/kpennell/airnyt
Demo: http://airnyt.surge.sh
javascript react.js google-maps
New contributor
Kyle Pennell is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
I’m struggling to make a React/JS map I have more efficient. Essentially, I have a list of cards, and then a map of markers. When I hover on one of the cards, it changes the styling of the marker. Here’s what it looks like:
But the way I’m doing this...I’m pretty sure is really inefficient.
So here’s how I have it setup in a couple of components:
//LocationsGrid.js
class LocationsGrid extends React.Component {
state = {
hoveredCardId: ""
};
setCardMarkerHover = location => {
this.setState({
hoveredCardId: location.pageid
});
};
resetCardMarkerHover = () => {
this.setState({
hoveredCardId: ""
});
};
render() {
const { locations } = this.props;
{locations.map((location, index) => (
<LocationCard
setCardMarkerHover={this.setCardMarkerHover}
resetCardMarkerHover={this.resetCardMarkerHover}
location={location}
/>
))}
// map stuff
<div className={classes.mapDiv}>
<MapAndMarkers
locations={locations}
hoveredCardId={this.state.hoveredCardId}
/>
</div>
//LocationCard.js
class LocationCard extends React.Component {
render() {
const { classes, location } = this.props;
return (
<Card
className={classes.card}
onMouseEnter={e => this.props.setCardMarkerHover(location)}
onMouseLeave={e => this.props.resetCardMarkerHover()}
>
<CardContent className={classes.cardContentArea}>
</CardContent>
</Card>
);
}
}
// MapAndMarkers.js
class MapAndMarkers extends React.Component {
render() {
const { locations, hoveredCardId, pageid } = this.props;
let MapMarkers = locations.map((location, index) => {
return (
<MapMarker
key={location.id}
lat={location.lat}
lng={location.lng}
pageid={location.pageid}
hoveredCardId={hoveredCardId}
/>
);
});
return (
<div>
<GoogleMapReact>
{MapMarkers}
</GoogleMapReact>
</div>
);
}
}
// MapMarker.js
import classNames from 'classnames';
class MapMarker extends React.Component {
render() {
const { classes, pageid, hoveredCardId } = this.props;
return (
<div className={classes.markerParent}>
<span className={classNames(classes.tooltips_span, pageid == hoveredCardId && classes.niftyHoverBackground)}>{this.props.name}</span>
</div>
);
}
}
The problem with doing it this way is I’m pretty sure there’s a whole bunch of wasted rendering. When I pulled out the mouse events from my LocationCard, everything else runs faster.
What would be a more efficient way of tracking hovered list items so that I can change the map marker style?
Here’s the code: https://github.com/kpennell/airnyt
Demo: http://airnyt.surge.sh
javascript react.js google-maps
New contributor
Kyle Pennell is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I’m struggling to make a React/JS map I have more efficient. Essentially, I have a list of cards, and then a map of markers. When I hover on one of the cards, it changes the styling of the marker. Here’s what it looks like:
But the way I’m doing this...I’m pretty sure is really inefficient.
So here’s how I have it setup in a couple of components:
//LocationsGrid.js
class LocationsGrid extends React.Component {
state = {
hoveredCardId: ""
};
setCardMarkerHover = location => {
this.setState({
hoveredCardId: location.pageid
});
};
resetCardMarkerHover = () => {
this.setState({
hoveredCardId: ""
});
};
render() {
const { locations } = this.props;
{locations.map((location, index) => (
<LocationCard
setCardMarkerHover={this.setCardMarkerHover}
resetCardMarkerHover={this.resetCardMarkerHover}
location={location}
/>
))}
// map stuff
<div className={classes.mapDiv}>
<MapAndMarkers
locations={locations}
hoveredCardId={this.state.hoveredCardId}
/>
</div>
//LocationCard.js
class LocationCard extends React.Component {
render() {
const { classes, location } = this.props;
return (
<Card
className={classes.card}
onMouseEnter={e => this.props.setCardMarkerHover(location)}
onMouseLeave={e => this.props.resetCardMarkerHover()}
>
<CardContent className={classes.cardContentArea}>
</CardContent>
</Card>
);
}
}
// MapAndMarkers.js
class MapAndMarkers extends React.Component {
render() {
const { locations, hoveredCardId, pageid } = this.props;
let MapMarkers = locations.map((location, index) => {
return (
<MapMarker
key={location.id}
lat={location.lat}
lng={location.lng}
pageid={location.pageid}
hoveredCardId={hoveredCardId}
/>
);
});
return (
<div>
<GoogleMapReact>
{MapMarkers}
</GoogleMapReact>
</div>
);
}
}
// MapMarker.js
import classNames from 'classnames';
class MapMarker extends React.Component {
render() {
const { classes, pageid, hoveredCardId } = this.props;
return (
<div className={classes.markerParent}>
<span className={classNames(classes.tooltips_span, pageid == hoveredCardId && classes.niftyHoverBackground)}>{this.props.name}</span>
</div>
);
}
}
The problem with doing it this way is I’m pretty sure there’s a whole bunch of wasted rendering. When I pulled out the mouse events from my LocationCard, everything else runs faster.
What would be a more efficient way of tracking hovered list items so that I can change the map marker style?
Here’s the code: https://github.com/kpennell/airnyt
Demo: http://airnyt.surge.sh
javascript react.js google-maps
New contributor
Kyle Pennell is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I’m struggling to make a React/JS map I have more efficient. Essentially, I have a list of cards, and then a map of markers. When I hover on one of the cards, it changes the styling of the marker. Here’s what it looks like:
But the way I’m doing this...I’m pretty sure is really inefficient.
So here’s how I have it setup in a couple of components:
//LocationsGrid.js
class LocationsGrid extends React.Component {
state = {
hoveredCardId: ""
};
setCardMarkerHover = location => {
this.setState({
hoveredCardId: location.pageid
});
};
resetCardMarkerHover = () => {
this.setState({
hoveredCardId: ""
});
};
render() {
const { locations } = this.props;
{locations.map((location, index) => (
<LocationCard
setCardMarkerHover={this.setCardMarkerHover}
resetCardMarkerHover={this.resetCardMarkerHover}
location={location}
/>
))}
// map stuff
<div className={classes.mapDiv}>
<MapAndMarkers
locations={locations}
hoveredCardId={this.state.hoveredCardId}
/>
</div>
//LocationCard.js
class LocationCard extends React.Component {
render() {
const { classes, location } = this.props;
return (
<Card
className={classes.card}
onMouseEnter={e => this.props.setCardMarkerHover(location)}
onMouseLeave={e => this.props.resetCardMarkerHover()}
>
<CardContent className={classes.cardContentArea}>
</CardContent>
</Card>
);
}
}
// MapAndMarkers.js
class MapAndMarkers extends React.Component {
render() {
const { locations, hoveredCardId, pageid } = this.props;
let MapMarkers = locations.map((location, index) => {
return (
<MapMarker
key={location.id}
lat={location.lat}
lng={location.lng}
pageid={location.pageid}
hoveredCardId={hoveredCardId}
/>
);
});
return (
<div>
<GoogleMapReact>
{MapMarkers}
</GoogleMapReact>
</div>
);
}
}
// MapMarker.js
import classNames from 'classnames';
class MapMarker extends React.Component {
render() {
const { classes, pageid, hoveredCardId } = this.props;
return (
<div className={classes.markerParent}>
<span className={classNames(classes.tooltips_span, pageid == hoveredCardId && classes.niftyHoverBackground)}>{this.props.name}</span>
</div>
);
}
}
The problem with doing it this way is I’m pretty sure there’s a whole bunch of wasted rendering. When I pulled out the mouse events from my LocationCard, everything else runs faster.
What would be a more efficient way of tracking hovered list items so that I can change the map marker style?
Here’s the code: https://github.com/kpennell/airnyt
Demo: http://airnyt.surge.sh
javascript react.js google-maps
javascript react.js google-maps
New contributor
Kyle Pennell is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Kyle Pennell is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Kyle Pennell is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 12 mins ago
Kyle Pennell
1011
1011
New contributor
Kyle Pennell is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Kyle Pennell is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Kyle Pennell is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Kyle Pennell is a new contributor. Be nice, and check out our Code of Conduct.
Kyle Pennell is a new contributor. Be nice, and check out our Code of Conduct.
Kyle Pennell is a new contributor. Be nice, and check out our Code of Conduct.
Kyle Pennell is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f208665%2fhow-to-hover-on-list-items-to-change-map-marker-styling-in-a-more-efficient-way%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bSKOSa DUl8eTSWi,2qsDxmqcGKduvVPax,i2E5V6F0 sXZQh,9,lq7seYG,A5t