Listing prime numbers up to the given number
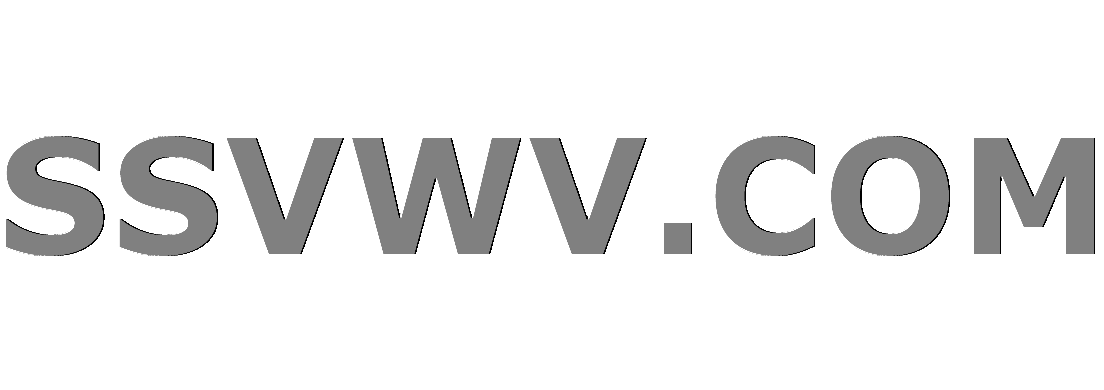
Multi tool use
up vote
0
down vote
favorite
import java.util.*;
public class PrimeNumber {
private static ArrayList<Integer> listOfPrime = new ArrayList<Integer>();
public static void main(String args) {
Scanner userInput = new Scanner(System.in);
System.out.print("Please enter a number to checked the list of prime number > ");
//Request user to input number.
String input = userInput.nextLine();
int number = 0;
boolean check = false;
//Check if integer entered is it integer
while(check != true) {
try {
// if input can be parse into integer, shows that its number so can change check into true to exit the while loop
number = Integer.parseInt(input);
check = true;
}
catch(NumberFormatException e) {
System.out.println("Please enter numbers only > ");
input = userInput.nextLine();
}
}
//Check if input enter is 0 or 1, as prime number cannot be those.
if(number < 2) {
System.out.println("Prime number cannot be 0 or 1");
}else {
checkPrime(number);
}
}
//Check for all prime numbers within the number user input.
private static void checkPrime(int input) {
boolean primeValidation = true;
//first for loop to check from small to biggest integer in the input
for(int i = 1; i <= input;i++) {
if(i !=1) {
//Second for loop to check from prime number in the small to big number.
//If the number i is 2 or 3, the loop will exit and add into the list as the default is prime, and no option changes it to not prime number.
for(int x = 2; x < i ; x++) {
if(i%x == 0) {
//If the number is divisable by any number to 0, set it to not prime.
primeValidation = false;
}
}
}
//if the input is either 0 or 1 set it to false and 0 and 1 is not prime
else {
primeValidation = false;
}
if(primeValidation == true) {
listOfPrime.add(i);
}else {
primeValidation = true;
}
}
System.out.println("The list of prime numbers of the number " + input + " are "+listOfPrime);
}
}
java primes
New contributor
Hau Jia Hung is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
import java.util.*;
public class PrimeNumber {
private static ArrayList<Integer> listOfPrime = new ArrayList<Integer>();
public static void main(String args) {
Scanner userInput = new Scanner(System.in);
System.out.print("Please enter a number to checked the list of prime number > ");
//Request user to input number.
String input = userInput.nextLine();
int number = 0;
boolean check = false;
//Check if integer entered is it integer
while(check != true) {
try {
// if input can be parse into integer, shows that its number so can change check into true to exit the while loop
number = Integer.parseInt(input);
check = true;
}
catch(NumberFormatException e) {
System.out.println("Please enter numbers only > ");
input = userInput.nextLine();
}
}
//Check if input enter is 0 or 1, as prime number cannot be those.
if(number < 2) {
System.out.println("Prime number cannot be 0 or 1");
}else {
checkPrime(number);
}
}
//Check for all prime numbers within the number user input.
private static void checkPrime(int input) {
boolean primeValidation = true;
//first for loop to check from small to biggest integer in the input
for(int i = 1; i <= input;i++) {
if(i !=1) {
//Second for loop to check from prime number in the small to big number.
//If the number i is 2 or 3, the loop will exit and add into the list as the default is prime, and no option changes it to not prime number.
for(int x = 2; x < i ; x++) {
if(i%x == 0) {
//If the number is divisable by any number to 0, set it to not prime.
primeValidation = false;
}
}
}
//if the input is either 0 or 1 set it to false and 0 and 1 is not prime
else {
primeValidation = false;
}
if(primeValidation == true) {
listOfPrime.add(i);
}else {
primeValidation = true;
}
}
System.out.println("The list of prime numbers of the number " + input + " are "+listOfPrime);
}
}
java primes
New contributor
Hau Jia Hung is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
Welcome to Code Review. Please fix your indentation. The easiest way to post core is to paste it into the question editor, highlight it, and press Ctrl-K to mark it as a code block. Also tell us what the code accomplishes — see How to Ask.
– 200_success
2 hours ago
1
Plus you have two " ` " character making your code unable to compile. Otherwise, a small advise: try to look on internet how to find prime numbers (in any language), you chose one of the worst methods and don't comment what's obvious, it make the reading harder (even with code cleaned ). Welcome on Code Review anyway :)
– Calak
1 hour ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
import java.util.*;
public class PrimeNumber {
private static ArrayList<Integer> listOfPrime = new ArrayList<Integer>();
public static void main(String args) {
Scanner userInput = new Scanner(System.in);
System.out.print("Please enter a number to checked the list of prime number > ");
//Request user to input number.
String input = userInput.nextLine();
int number = 0;
boolean check = false;
//Check if integer entered is it integer
while(check != true) {
try {
// if input can be parse into integer, shows that its number so can change check into true to exit the while loop
number = Integer.parseInt(input);
check = true;
}
catch(NumberFormatException e) {
System.out.println("Please enter numbers only > ");
input = userInput.nextLine();
}
}
//Check if input enter is 0 or 1, as prime number cannot be those.
if(number < 2) {
System.out.println("Prime number cannot be 0 or 1");
}else {
checkPrime(number);
}
}
//Check for all prime numbers within the number user input.
private static void checkPrime(int input) {
boolean primeValidation = true;
//first for loop to check from small to biggest integer in the input
for(int i = 1; i <= input;i++) {
if(i !=1) {
//Second for loop to check from prime number in the small to big number.
//If the number i is 2 or 3, the loop will exit and add into the list as the default is prime, and no option changes it to not prime number.
for(int x = 2; x < i ; x++) {
if(i%x == 0) {
//If the number is divisable by any number to 0, set it to not prime.
primeValidation = false;
}
}
}
//if the input is either 0 or 1 set it to false and 0 and 1 is not prime
else {
primeValidation = false;
}
if(primeValidation == true) {
listOfPrime.add(i);
}else {
primeValidation = true;
}
}
System.out.println("The list of prime numbers of the number " + input + " are "+listOfPrime);
}
}
java primes
New contributor
Hau Jia Hung is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
import java.util.*;
public class PrimeNumber {
private static ArrayList<Integer> listOfPrime = new ArrayList<Integer>();
public static void main(String args) {
Scanner userInput = new Scanner(System.in);
System.out.print("Please enter a number to checked the list of prime number > ");
//Request user to input number.
String input = userInput.nextLine();
int number = 0;
boolean check = false;
//Check if integer entered is it integer
while(check != true) {
try {
// if input can be parse into integer, shows that its number so can change check into true to exit the while loop
number = Integer.parseInt(input);
check = true;
}
catch(NumberFormatException e) {
System.out.println("Please enter numbers only > ");
input = userInput.nextLine();
}
}
//Check if input enter is 0 or 1, as prime number cannot be those.
if(number < 2) {
System.out.println("Prime number cannot be 0 or 1");
}else {
checkPrime(number);
}
}
//Check for all prime numbers within the number user input.
private static void checkPrime(int input) {
boolean primeValidation = true;
//first for loop to check from small to biggest integer in the input
for(int i = 1; i <= input;i++) {
if(i !=1) {
//Second for loop to check from prime number in the small to big number.
//If the number i is 2 or 3, the loop will exit and add into the list as the default is prime, and no option changes it to not prime number.
for(int x = 2; x < i ; x++) {
if(i%x == 0) {
//If the number is divisable by any number to 0, set it to not prime.
primeValidation = false;
}
}
}
//if the input is either 0 or 1 set it to false and 0 and 1 is not prime
else {
primeValidation = false;
}
if(primeValidation == true) {
listOfPrime.add(i);
}else {
primeValidation = true;
}
}
System.out.println("The list of prime numbers of the number " + input + " are "+listOfPrime);
}
}
java primes
java primes
New contributor
Hau Jia Hung is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Hau Jia Hung is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 19 mins ago


200_success
127k15148411
127k15148411
New contributor
Hau Jia Hung is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 2 hours ago
Hau Jia Hung
6
6
New contributor
Hau Jia Hung is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Hau Jia Hung is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Hau Jia Hung is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
Welcome to Code Review. Please fix your indentation. The easiest way to post core is to paste it into the question editor, highlight it, and press Ctrl-K to mark it as a code block. Also tell us what the code accomplishes — see How to Ask.
– 200_success
2 hours ago
1
Plus you have two " ` " character making your code unable to compile. Otherwise, a small advise: try to look on internet how to find prime numbers (in any language), you chose one of the worst methods and don't comment what's obvious, it make the reading harder (even with code cleaned ). Welcome on Code Review anyway :)
– Calak
1 hour ago
add a comment |
1
Welcome to Code Review. Please fix your indentation. The easiest way to post core is to paste it into the question editor, highlight it, and press Ctrl-K to mark it as a code block. Also tell us what the code accomplishes — see How to Ask.
– 200_success
2 hours ago
1
Plus you have two " ` " character making your code unable to compile. Otherwise, a small advise: try to look on internet how to find prime numbers (in any language), you chose one of the worst methods and don't comment what's obvious, it make the reading harder (even with code cleaned ). Welcome on Code Review anyway :)
– Calak
1 hour ago
1
1
Welcome to Code Review. Please fix your indentation. The easiest way to post core is to paste it into the question editor, highlight it, and press Ctrl-K to mark it as a code block. Also tell us what the code accomplishes — see How to Ask.
– 200_success
2 hours ago
Welcome to Code Review. Please fix your indentation. The easiest way to post core is to paste it into the question editor, highlight it, and press Ctrl-K to mark it as a code block. Also tell us what the code accomplishes — see How to Ask.
– 200_success
2 hours ago
1
1
Plus you have two " ` " character making your code unable to compile. Otherwise, a small advise: try to look on internet how to find prime numbers (in any language), you chose one of the worst methods and don't comment what's obvious, it make the reading harder (even with code cleaned ). Welcome on Code Review anyway :)
– Calak
1 hour ago
Plus you have two " ` " character making your code unable to compile. Otherwise, a small advise: try to look on internet how to find prime numbers (in any language), you chose one of the worst methods and don't comment what's obvious, it make the reading harder (even with code cleaned ). Welcome on Code Review anyway :)
– Calak
1 hour ago
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Hau Jia Hung is a new contributor. Be nice, and check out our Code of Conduct.
draft saved
draft discarded
Hau Jia Hung is a new contributor. Be nice, and check out our Code of Conduct.
Hau Jia Hung is a new contributor. Be nice, and check out our Code of Conduct.
Hau Jia Hung is a new contributor. Be nice, and check out our Code of Conduct.
draft saved
draft discarded
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f208353%2flisting-prime-numbers-up-to-the-given-number%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
W6NKj9K,pCLsmim6BKYeh,Drv8 ROt3Mm
1
Welcome to Code Review. Please fix your indentation. The easiest way to post core is to paste it into the question editor, highlight it, and press Ctrl-K to mark it as a code block. Also tell us what the code accomplishes — see How to Ask.
– 200_success
2 hours ago
1
Plus you have two " ` " character making your code unable to compile. Otherwise, a small advise: try to look on internet how to find prime numbers (in any language), you chose one of the worst methods and don't comment what's obvious, it make the reading harder (even with code cleaned ). Welcome on Code Review anyway :)
– Calak
1 hour ago