RemoteDisconnected(“Remote end closed connection without” http.client.RemoteDisconnected: Remote end...
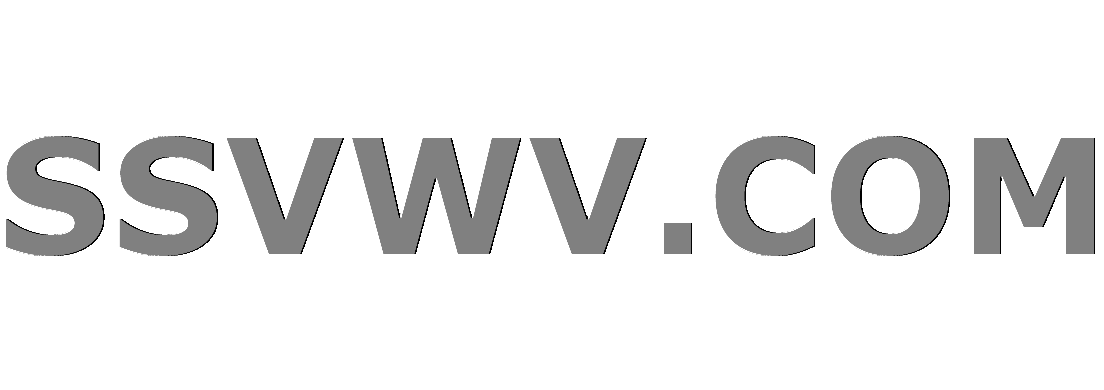
Multi tool use
up vote
2
down vote
favorite
Since Google denies access to API key of Google MyBusiness to all but established firms, I attempted to automate the process of changing my business information using selenium webdriver.
What works?
Logging in to Google Mybusiness by automating the login form.
What doesnt work?
After logging in, I need to open the small modal of editing working hours. I attempted to automate a click on the edit button, but unfortunately I am getting this error: http.client.RemoteDisconnected: Remote end closed connection without response
My code:
from selenium import webdriver
options = webdriver.ChromeOptions()
options.add_argument("start-maximized")
driver = webdriver.Chrome(chrome_options=options)
def LoginGMB(driver):
(myemail, mypassword) = AuthenticationDetails()
driver.find_element_by_id('identifierId').send_keys(myemail)
driver.find_element_by_id('identifierNext').click()
time.sleep(2)
driver.find_element_by_name('password').send_keys(mypassword)
driver.find_element_by_id('passwordNext').click()
time.sleep(2)
def OpenGMB(url):
driver.get(url)
print(driver.current_url)
pattern = re.compile(".*accounts.google.com/signin.*")
match = re.search(pattern, cururl)
if match:
LoginGMB(driver)
print("Ok we're back")
driver.find_element_by_id('ow50').click()
OpenGMB('https://business.google.com/edit/l/001?hl=en')
Stacktrace:
https://accounts.google.com/signin/v2/identifier?service=lbc&passive=1209600&continue
We need to login as we are presented login page
Ok we're back
Traceback (most recent call last):
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 600, in urlopen
chunked=chunked)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 384, in _make_request
six.raise_from(e, None)
File "<string>", line 2, in raise_from
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 380, in _make_request
httplib_response = conn.getresponse()
File "/usr/lib/python3.6/http/client.py", line 1331, in getresponse
response.begin()
File "/usr/lib/python3.6/http/client.py", line 297, in begin
version, status, reason = self._read_status()
File "/usr/lib/python3.6/http/client.py", line 266, in _read_status
raise RemoteDisconnected("Remote end closed connection without"
http.client.RemoteDisconnected: Remote end closed connection without response
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "gmb.py", line 77, in <module>
OpenGMB(url)
File "gmb.py", line 62, in OpenGMB
el = driver.find_element_by_id('ow50')
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 360, in find_element_by_id
return self.find_element(by=By.ID, value=id_)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 978, in find_element
'value': value})['value']
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 319, in execute
response = self.command_executor.execute(driver_command, params)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/remote_connection.py", line 376, in execute
return self._request(command_info[0], url, body=data)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/remote_connection.py", line 399, in _request
resp = self._conn.request(method, url, body=body, headers=headers)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/request.py", line 72, in request
**urlopen_kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/request.py", line 150, in request_encode_body
return self.urlopen(method, url, **extra_kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/poolmanager.py", line 323, in urlopen
response = conn.urlopen(method, u.request_uri, **kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 638, in urlopen
_stacktrace=sys.exc_info()[2])
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/util/retry.py", line 367, in increment
raise six.reraise(type(error), error, _stacktrace)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/packages/six.py", line 685, in reraise
raise value.with_traceback(tb)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 600, in urlopen
chunked=chunked)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 384, in _make_request
six.raise_from(e, None)
File "<string>", line 2, in raise_from
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 380, in _make_request
httplib_response = conn.getresponse()
File "/usr/lib/python3.6/http/client.py", line 1331, in getresponse
response.begin()
File "/usr/lib/python3.6/http/client.py", line 297, in begin
version, status, reason = self._read_status()
File "/usr/lib/python3.6/http/client.py", line 266, in _read_status
raise RemoteDisconnected("Remote end closed connection without"
urllib3.exceptions.ProtocolError: ('Connection aborted.', RemoteDisconnected('Remote end closed connection without response',))
python selenium

add a comment |
up vote
2
down vote
favorite
Since Google denies access to API key of Google MyBusiness to all but established firms, I attempted to automate the process of changing my business information using selenium webdriver.
What works?
Logging in to Google Mybusiness by automating the login form.
What doesnt work?
After logging in, I need to open the small modal of editing working hours. I attempted to automate a click on the edit button, but unfortunately I am getting this error: http.client.RemoteDisconnected: Remote end closed connection without response
My code:
from selenium import webdriver
options = webdriver.ChromeOptions()
options.add_argument("start-maximized")
driver = webdriver.Chrome(chrome_options=options)
def LoginGMB(driver):
(myemail, mypassword) = AuthenticationDetails()
driver.find_element_by_id('identifierId').send_keys(myemail)
driver.find_element_by_id('identifierNext').click()
time.sleep(2)
driver.find_element_by_name('password').send_keys(mypassword)
driver.find_element_by_id('passwordNext').click()
time.sleep(2)
def OpenGMB(url):
driver.get(url)
print(driver.current_url)
pattern = re.compile(".*accounts.google.com/signin.*")
match = re.search(pattern, cururl)
if match:
LoginGMB(driver)
print("Ok we're back")
driver.find_element_by_id('ow50').click()
OpenGMB('https://business.google.com/edit/l/001?hl=en')
Stacktrace:
https://accounts.google.com/signin/v2/identifier?service=lbc&passive=1209600&continue
We need to login as we are presented login page
Ok we're back
Traceback (most recent call last):
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 600, in urlopen
chunked=chunked)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 384, in _make_request
six.raise_from(e, None)
File "<string>", line 2, in raise_from
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 380, in _make_request
httplib_response = conn.getresponse()
File "/usr/lib/python3.6/http/client.py", line 1331, in getresponse
response.begin()
File "/usr/lib/python3.6/http/client.py", line 297, in begin
version, status, reason = self._read_status()
File "/usr/lib/python3.6/http/client.py", line 266, in _read_status
raise RemoteDisconnected("Remote end closed connection without"
http.client.RemoteDisconnected: Remote end closed connection without response
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "gmb.py", line 77, in <module>
OpenGMB(url)
File "gmb.py", line 62, in OpenGMB
el = driver.find_element_by_id('ow50')
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 360, in find_element_by_id
return self.find_element(by=By.ID, value=id_)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 978, in find_element
'value': value})['value']
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 319, in execute
response = self.command_executor.execute(driver_command, params)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/remote_connection.py", line 376, in execute
return self._request(command_info[0], url, body=data)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/remote_connection.py", line 399, in _request
resp = self._conn.request(method, url, body=body, headers=headers)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/request.py", line 72, in request
**urlopen_kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/request.py", line 150, in request_encode_body
return self.urlopen(method, url, **extra_kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/poolmanager.py", line 323, in urlopen
response = conn.urlopen(method, u.request_uri, **kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 638, in urlopen
_stacktrace=sys.exc_info()[2])
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/util/retry.py", line 367, in increment
raise six.reraise(type(error), error, _stacktrace)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/packages/six.py", line 685, in reraise
raise value.with_traceback(tb)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 600, in urlopen
chunked=chunked)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 384, in _make_request
six.raise_from(e, None)
File "<string>", line 2, in raise_from
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 380, in _make_request
httplib_response = conn.getresponse()
File "/usr/lib/python3.6/http/client.py", line 1331, in getresponse
response.begin()
File "/usr/lib/python3.6/http/client.py", line 297, in begin
version, status, reason = self._read_status()
File "/usr/lib/python3.6/http/client.py", line 266, in _read_status
raise RemoteDisconnected("Remote end closed connection without"
urllib3.exceptions.ProtocolError: ('Connection aborted.', RemoteDisconnected('Remote end closed connection without response',))
python selenium

add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
Since Google denies access to API key of Google MyBusiness to all but established firms, I attempted to automate the process of changing my business information using selenium webdriver.
What works?
Logging in to Google Mybusiness by automating the login form.
What doesnt work?
After logging in, I need to open the small modal of editing working hours. I attempted to automate a click on the edit button, but unfortunately I am getting this error: http.client.RemoteDisconnected: Remote end closed connection without response
My code:
from selenium import webdriver
options = webdriver.ChromeOptions()
options.add_argument("start-maximized")
driver = webdriver.Chrome(chrome_options=options)
def LoginGMB(driver):
(myemail, mypassword) = AuthenticationDetails()
driver.find_element_by_id('identifierId').send_keys(myemail)
driver.find_element_by_id('identifierNext').click()
time.sleep(2)
driver.find_element_by_name('password').send_keys(mypassword)
driver.find_element_by_id('passwordNext').click()
time.sleep(2)
def OpenGMB(url):
driver.get(url)
print(driver.current_url)
pattern = re.compile(".*accounts.google.com/signin.*")
match = re.search(pattern, cururl)
if match:
LoginGMB(driver)
print("Ok we're back")
driver.find_element_by_id('ow50').click()
OpenGMB('https://business.google.com/edit/l/001?hl=en')
Stacktrace:
https://accounts.google.com/signin/v2/identifier?service=lbc&passive=1209600&continue
We need to login as we are presented login page
Ok we're back
Traceback (most recent call last):
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 600, in urlopen
chunked=chunked)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 384, in _make_request
six.raise_from(e, None)
File "<string>", line 2, in raise_from
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 380, in _make_request
httplib_response = conn.getresponse()
File "/usr/lib/python3.6/http/client.py", line 1331, in getresponse
response.begin()
File "/usr/lib/python3.6/http/client.py", line 297, in begin
version, status, reason = self._read_status()
File "/usr/lib/python3.6/http/client.py", line 266, in _read_status
raise RemoteDisconnected("Remote end closed connection without"
http.client.RemoteDisconnected: Remote end closed connection without response
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "gmb.py", line 77, in <module>
OpenGMB(url)
File "gmb.py", line 62, in OpenGMB
el = driver.find_element_by_id('ow50')
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 360, in find_element_by_id
return self.find_element(by=By.ID, value=id_)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 978, in find_element
'value': value})['value']
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 319, in execute
response = self.command_executor.execute(driver_command, params)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/remote_connection.py", line 376, in execute
return self._request(command_info[0], url, body=data)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/remote_connection.py", line 399, in _request
resp = self._conn.request(method, url, body=body, headers=headers)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/request.py", line 72, in request
**urlopen_kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/request.py", line 150, in request_encode_body
return self.urlopen(method, url, **extra_kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/poolmanager.py", line 323, in urlopen
response = conn.urlopen(method, u.request_uri, **kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 638, in urlopen
_stacktrace=sys.exc_info()[2])
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/util/retry.py", line 367, in increment
raise six.reraise(type(error), error, _stacktrace)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/packages/six.py", line 685, in reraise
raise value.with_traceback(tb)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 600, in urlopen
chunked=chunked)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 384, in _make_request
six.raise_from(e, None)
File "<string>", line 2, in raise_from
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 380, in _make_request
httplib_response = conn.getresponse()
File "/usr/lib/python3.6/http/client.py", line 1331, in getresponse
response.begin()
File "/usr/lib/python3.6/http/client.py", line 297, in begin
version, status, reason = self._read_status()
File "/usr/lib/python3.6/http/client.py", line 266, in _read_status
raise RemoteDisconnected("Remote end closed connection without"
urllib3.exceptions.ProtocolError: ('Connection aborted.', RemoteDisconnected('Remote end closed connection without response',))
python selenium

Since Google denies access to API key of Google MyBusiness to all but established firms, I attempted to automate the process of changing my business information using selenium webdriver.
What works?
Logging in to Google Mybusiness by automating the login form.
What doesnt work?
After logging in, I need to open the small modal of editing working hours. I attempted to automate a click on the edit button, but unfortunately I am getting this error: http.client.RemoteDisconnected: Remote end closed connection without response
My code:
from selenium import webdriver
options = webdriver.ChromeOptions()
options.add_argument("start-maximized")
driver = webdriver.Chrome(chrome_options=options)
def LoginGMB(driver):
(myemail, mypassword) = AuthenticationDetails()
driver.find_element_by_id('identifierId').send_keys(myemail)
driver.find_element_by_id('identifierNext').click()
time.sleep(2)
driver.find_element_by_name('password').send_keys(mypassword)
driver.find_element_by_id('passwordNext').click()
time.sleep(2)
def OpenGMB(url):
driver.get(url)
print(driver.current_url)
pattern = re.compile(".*accounts.google.com/signin.*")
match = re.search(pattern, cururl)
if match:
LoginGMB(driver)
print("Ok we're back")
driver.find_element_by_id('ow50').click()
OpenGMB('https://business.google.com/edit/l/001?hl=en')
Stacktrace:
https://accounts.google.com/signin/v2/identifier?service=lbc&passive=1209600&continue
We need to login as we are presented login page
Ok we're back
Traceback (most recent call last):
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 600, in urlopen
chunked=chunked)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 384, in _make_request
six.raise_from(e, None)
File "<string>", line 2, in raise_from
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 380, in _make_request
httplib_response = conn.getresponse()
File "/usr/lib/python3.6/http/client.py", line 1331, in getresponse
response.begin()
File "/usr/lib/python3.6/http/client.py", line 297, in begin
version, status, reason = self._read_status()
File "/usr/lib/python3.6/http/client.py", line 266, in _read_status
raise RemoteDisconnected("Remote end closed connection without"
http.client.RemoteDisconnected: Remote end closed connection without response
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "gmb.py", line 77, in <module>
OpenGMB(url)
File "gmb.py", line 62, in OpenGMB
el = driver.find_element_by_id('ow50')
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 360, in find_element_by_id
return self.find_element(by=By.ID, value=id_)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 978, in find_element
'value': value})['value']
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/webdriver.py", line 319, in execute
response = self.command_executor.execute(driver_command, params)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/remote_connection.py", line 376, in execute
return self._request(command_info[0], url, body=data)
File "/home/joel/.local/lib/python3.6/site-packages/selenium/webdriver/remote/remote_connection.py", line 399, in _request
resp = self._conn.request(method, url, body=body, headers=headers)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/request.py", line 72, in request
**urlopen_kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/request.py", line 150, in request_encode_body
return self.urlopen(method, url, **extra_kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/poolmanager.py", line 323, in urlopen
response = conn.urlopen(method, u.request_uri, **kw)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 638, in urlopen
_stacktrace=sys.exc_info()[2])
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/util/retry.py", line 367, in increment
raise six.reraise(type(error), error, _stacktrace)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/packages/six.py", line 685, in reraise
raise value.with_traceback(tb)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 600, in urlopen
chunked=chunked)
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 384, in _make_request
six.raise_from(e, None)
File "<string>", line 2, in raise_from
File "/home/joel/.local/lib/python3.6/site-packages/urllib3/connectionpool.py", line 380, in _make_request
httplib_response = conn.getresponse()
File "/usr/lib/python3.6/http/client.py", line 1331, in getresponse
response.begin()
File "/usr/lib/python3.6/http/client.py", line 297, in begin
version, status, reason = self._read_status()
File "/usr/lib/python3.6/http/client.py", line 266, in _read_status
raise RemoteDisconnected("Remote end closed connection without"
urllib3.exceptions.ProtocolError: ('Connection aborted.', RemoteDisconnected('Remote end closed connection without response',))
python selenium

python selenium

edited Nov 20 at 7:22


DebanjanB
36k73372
36k73372
asked Nov 19 at 13:36
Joel G Mathew
1,85492643
1,85492643
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
These error messages...
RemoteDisconnected("Remote end closed connection without"
http.client.RemoteDisconnected: Remote end closed connection without response
and
RemoteDisconnected("Remote end closed connection without"
urllib3.exceptions.ProtocolError: ('Connection aborted.', RemoteDisconnected('Remote end closed connection without response',))
...implies that the Remote Connection was disconnected due to ProtocolError.
As per urllib3.exceptions.ProtocolError: ('Connection aborted.', error(10054, 'An existing connection was forcibly closed by the remote host')) This issue is pretty evident when there is a incompatibility between the version of the binaries you are using.
As you are using ChromeDriver and Chrome Browser you must ensure that the binaries are compatible as per the entries below:
ChromeDriver v2.27 : Supports Chrome v54-56
ChromeDriver v2.28 : Supports Chrome v55-57
ChromeDriver v2.29 : Supports Chrome v56-58
ChromeDriver v2.30 : Supports Chrome v58-60
ChromeDriver v2.31 : Supports Chrome v58-60
ChromeDriver v2.32 : Supports Chrome v59-61
ChromeDriver v2.33 : Supports Chrome v60-62
ChromeDriver v2.34 : Supports Chrome v61-63
ChromeDriver v2.35 : Supports Chrome v62-64
ChromeDriver v2.36 : Supports Chrome v63-65
ChromeDriver v2.37 : Supports Chrome v64-66
ChromeDriver v2.38 : Supports Chrome v65-67
ChromeDriver v2.39 : Supports Chrome v66-68
ChromeDriver v2.40 : Supports Chrome v66-68
ChromeDriver v2.41 : Supports Chrome v67-69
ChromeDriver v2.42 : Supports Chrome v68-70
ChromeDriver v2.43 : Supports Chrome v69-71
ChromeDriver v2.44 : Supports Chrome v69-71 (same as ChromeDriver 2.43, but with additional bug fixes)
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
These error messages...
RemoteDisconnected("Remote end closed connection without"
http.client.RemoteDisconnected: Remote end closed connection without response
and
RemoteDisconnected("Remote end closed connection without"
urllib3.exceptions.ProtocolError: ('Connection aborted.', RemoteDisconnected('Remote end closed connection without response',))
...implies that the Remote Connection was disconnected due to ProtocolError.
As per urllib3.exceptions.ProtocolError: ('Connection aborted.', error(10054, 'An existing connection was forcibly closed by the remote host')) This issue is pretty evident when there is a incompatibility between the version of the binaries you are using.
As you are using ChromeDriver and Chrome Browser you must ensure that the binaries are compatible as per the entries below:
ChromeDriver v2.27 : Supports Chrome v54-56
ChromeDriver v2.28 : Supports Chrome v55-57
ChromeDriver v2.29 : Supports Chrome v56-58
ChromeDriver v2.30 : Supports Chrome v58-60
ChromeDriver v2.31 : Supports Chrome v58-60
ChromeDriver v2.32 : Supports Chrome v59-61
ChromeDriver v2.33 : Supports Chrome v60-62
ChromeDriver v2.34 : Supports Chrome v61-63
ChromeDriver v2.35 : Supports Chrome v62-64
ChromeDriver v2.36 : Supports Chrome v63-65
ChromeDriver v2.37 : Supports Chrome v64-66
ChromeDriver v2.38 : Supports Chrome v65-67
ChromeDriver v2.39 : Supports Chrome v66-68
ChromeDriver v2.40 : Supports Chrome v66-68
ChromeDriver v2.41 : Supports Chrome v67-69
ChromeDriver v2.42 : Supports Chrome v68-70
ChromeDriver v2.43 : Supports Chrome v69-71
ChromeDriver v2.44 : Supports Chrome v69-71 (same as ChromeDriver 2.43, but with additional bug fixes)
add a comment |
up vote
1
down vote
accepted
These error messages...
RemoteDisconnected("Remote end closed connection without"
http.client.RemoteDisconnected: Remote end closed connection without response
and
RemoteDisconnected("Remote end closed connection without"
urllib3.exceptions.ProtocolError: ('Connection aborted.', RemoteDisconnected('Remote end closed connection without response',))
...implies that the Remote Connection was disconnected due to ProtocolError.
As per urllib3.exceptions.ProtocolError: ('Connection aborted.', error(10054, 'An existing connection was forcibly closed by the remote host')) This issue is pretty evident when there is a incompatibility between the version of the binaries you are using.
As you are using ChromeDriver and Chrome Browser you must ensure that the binaries are compatible as per the entries below:
ChromeDriver v2.27 : Supports Chrome v54-56
ChromeDriver v2.28 : Supports Chrome v55-57
ChromeDriver v2.29 : Supports Chrome v56-58
ChromeDriver v2.30 : Supports Chrome v58-60
ChromeDriver v2.31 : Supports Chrome v58-60
ChromeDriver v2.32 : Supports Chrome v59-61
ChromeDriver v2.33 : Supports Chrome v60-62
ChromeDriver v2.34 : Supports Chrome v61-63
ChromeDriver v2.35 : Supports Chrome v62-64
ChromeDriver v2.36 : Supports Chrome v63-65
ChromeDriver v2.37 : Supports Chrome v64-66
ChromeDriver v2.38 : Supports Chrome v65-67
ChromeDriver v2.39 : Supports Chrome v66-68
ChromeDriver v2.40 : Supports Chrome v66-68
ChromeDriver v2.41 : Supports Chrome v67-69
ChromeDriver v2.42 : Supports Chrome v68-70
ChromeDriver v2.43 : Supports Chrome v69-71
ChromeDriver v2.44 : Supports Chrome v69-71 (same as ChromeDriver 2.43, but with additional bug fixes)
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
These error messages...
RemoteDisconnected("Remote end closed connection without"
http.client.RemoteDisconnected: Remote end closed connection without response
and
RemoteDisconnected("Remote end closed connection without"
urllib3.exceptions.ProtocolError: ('Connection aborted.', RemoteDisconnected('Remote end closed connection without response',))
...implies that the Remote Connection was disconnected due to ProtocolError.
As per urllib3.exceptions.ProtocolError: ('Connection aborted.', error(10054, 'An existing connection was forcibly closed by the remote host')) This issue is pretty evident when there is a incompatibility between the version of the binaries you are using.
As you are using ChromeDriver and Chrome Browser you must ensure that the binaries are compatible as per the entries below:
ChromeDriver v2.27 : Supports Chrome v54-56
ChromeDriver v2.28 : Supports Chrome v55-57
ChromeDriver v2.29 : Supports Chrome v56-58
ChromeDriver v2.30 : Supports Chrome v58-60
ChromeDriver v2.31 : Supports Chrome v58-60
ChromeDriver v2.32 : Supports Chrome v59-61
ChromeDriver v2.33 : Supports Chrome v60-62
ChromeDriver v2.34 : Supports Chrome v61-63
ChromeDriver v2.35 : Supports Chrome v62-64
ChromeDriver v2.36 : Supports Chrome v63-65
ChromeDriver v2.37 : Supports Chrome v64-66
ChromeDriver v2.38 : Supports Chrome v65-67
ChromeDriver v2.39 : Supports Chrome v66-68
ChromeDriver v2.40 : Supports Chrome v66-68
ChromeDriver v2.41 : Supports Chrome v67-69
ChromeDriver v2.42 : Supports Chrome v68-70
ChromeDriver v2.43 : Supports Chrome v69-71
ChromeDriver v2.44 : Supports Chrome v69-71 (same as ChromeDriver 2.43, but with additional bug fixes)
These error messages...
RemoteDisconnected("Remote end closed connection without"
http.client.RemoteDisconnected: Remote end closed connection without response
and
RemoteDisconnected("Remote end closed connection without"
urllib3.exceptions.ProtocolError: ('Connection aborted.', RemoteDisconnected('Remote end closed connection without response',))
...implies that the Remote Connection was disconnected due to ProtocolError.
As per urllib3.exceptions.ProtocolError: ('Connection aborted.', error(10054, 'An existing connection was forcibly closed by the remote host')) This issue is pretty evident when there is a incompatibility between the version of the binaries you are using.
As you are using ChromeDriver and Chrome Browser you must ensure that the binaries are compatible as per the entries below:
ChromeDriver v2.27 : Supports Chrome v54-56
ChromeDriver v2.28 : Supports Chrome v55-57
ChromeDriver v2.29 : Supports Chrome v56-58
ChromeDriver v2.30 : Supports Chrome v58-60
ChromeDriver v2.31 : Supports Chrome v58-60
ChromeDriver v2.32 : Supports Chrome v59-61
ChromeDriver v2.33 : Supports Chrome v60-62
ChromeDriver v2.34 : Supports Chrome v61-63
ChromeDriver v2.35 : Supports Chrome v62-64
ChromeDriver v2.36 : Supports Chrome v63-65
ChromeDriver v2.37 : Supports Chrome v64-66
ChromeDriver v2.38 : Supports Chrome v65-67
ChromeDriver v2.39 : Supports Chrome v66-68
ChromeDriver v2.40 : Supports Chrome v66-68
ChromeDriver v2.41 : Supports Chrome v67-69
ChromeDriver v2.42 : Supports Chrome v68-70
ChromeDriver v2.43 : Supports Chrome v69-71
ChromeDriver v2.44 : Supports Chrome v69-71 (same as ChromeDriver 2.43, but with additional bug fixes)
edited Nov 20 at 10:55
answered Nov 20 at 7:24


DebanjanB
36k73372
36k73372
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53375824%2fremotedisconnectedremote-end-closed-connection-without-http-client-remotedisc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eQ,fCaD MZG z8CNjpW Z Nz4 Bxgi