Find the minimum and maximum values in the nested object
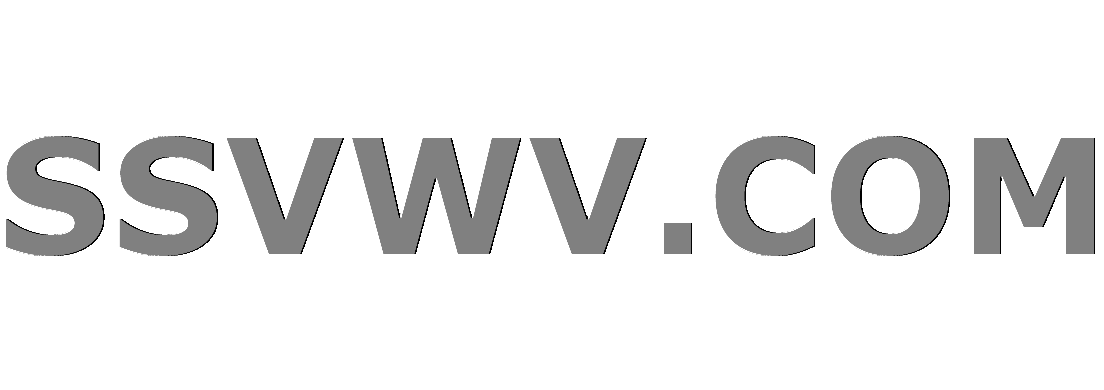
Multi tool use
up vote
0
down vote
favorite
I have a deeply nested javascript object with an unlimited amout of children. Every child has a value.
var object = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {...}
}
}
}
All attempts to make a recursive function did not succeed, it turned out to go down only to a lower level.
javascript reduce
add a comment |
up vote
0
down vote
favorite
I have a deeply nested javascript object with an unlimited amout of children. Every child has a value.
var object = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {...}
}
}
}
All attempts to make a recursive function did not succeed, it turned out to go down only to a lower level.
javascript reduce
3
What was your previous unsuccessful attempt?
– Robin Zigmond
Nov 19 at 16:39
Reopening. The linked question is quite different: no recursion is needed in that one..
– Scott Sauyet
Nov 19 at 17:00
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a deeply nested javascript object with an unlimited amout of children. Every child has a value.
var object = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {...}
}
}
}
All attempts to make a recursive function did not succeed, it turned out to go down only to a lower level.
javascript reduce
I have a deeply nested javascript object with an unlimited amout of children. Every child has a value.
var object = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {...}
}
}
}
All attempts to make a recursive function did not succeed, it turned out to go down only to a lower level.
javascript reduce
javascript reduce
asked Nov 19 at 16:38
LuisDemn
42
42
3
What was your previous unsuccessful attempt?
– Robin Zigmond
Nov 19 at 16:39
Reopening. The linked question is quite different: no recursion is needed in that one..
– Scott Sauyet
Nov 19 at 17:00
add a comment |
3
What was your previous unsuccessful attempt?
– Robin Zigmond
Nov 19 at 16:39
Reopening. The linked question is quite different: no recursion is needed in that one..
– Scott Sauyet
Nov 19 at 17:00
3
3
What was your previous unsuccessful attempt?
– Robin Zigmond
Nov 19 at 16:39
What was your previous unsuccessful attempt?
– Robin Zigmond
Nov 19 at 16:39
Reopening. The linked question is quite different: no recursion is needed in that one..
– Scott Sauyet
Nov 19 at 17:00
Reopening. The linked question is quite different: no recursion is needed in that one..
– Scott Sauyet
Nov 19 at 17:00
add a comment |
3 Answers
3
active
oldest
votes
up vote
3
down vote
After flattening your linked list into an array, you can use Array.prototype.reduce()
with an accumulator that is a tuple of min
and max
, starting with initial values of Infinity
and -Infinity
respectively to match the implementations of Math.min()
and Math.max()
:
const object = {
value: 1,
children: {
value: 10,
children: {
value: 2,
children: {
value: 5,
children: null
}
}
}
}
const flat = o => o == null || o.value == null ? : [o.value, ...flat(o.children)]
const [min, max] = flat(object).reduce(
([min, max], value) => [Math.min(min, value), Math.max(max, value)],
[Infinity, -Infinity]
)
console.log(min, max)
add a comment |
up vote
2
down vote
Since children
is an object with only one value (vs an array with potentially many), this is a pretty simple recursive function. The base case is when there are no children in which case both min and max are just the value. Otherwise recurse on the children to find the min and max:
var object = {
value: -10,
children: {
value: 4,
children:{
value: 200,
children: {
value: -100,
children: null
}
}
}
}
function getMinMax(obj) {
if (!obj.children || obj.children.value == undefined)
return {min: obj.value, max: obj.value}
else {
let m = getMinMax(obj.children)
return {min: Math.min(obj.value, m.min), max: Math.max(obj.value, m.max)}
}
}
console.log(getMinMax(object))
add a comment |
up vote
1
down vote
Short and simple, for min change Math.max
to Math.min
var test = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {}
}
}
}
function findMaxValue(obj) {
if (Object.keys(obj.children).length === 0) {
return obj.value;
}
return Math.max(obj.value, findMaxValue(obj.children))
}
console.log(findMaxValue(test))
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
After flattening your linked list into an array, you can use Array.prototype.reduce()
with an accumulator that is a tuple of min
and max
, starting with initial values of Infinity
and -Infinity
respectively to match the implementations of Math.min()
and Math.max()
:
const object = {
value: 1,
children: {
value: 10,
children: {
value: 2,
children: {
value: 5,
children: null
}
}
}
}
const flat = o => o == null || o.value == null ? : [o.value, ...flat(o.children)]
const [min, max] = flat(object).reduce(
([min, max], value) => [Math.min(min, value), Math.max(max, value)],
[Infinity, -Infinity]
)
console.log(min, max)
add a comment |
up vote
3
down vote
After flattening your linked list into an array, you can use Array.prototype.reduce()
with an accumulator that is a tuple of min
and max
, starting with initial values of Infinity
and -Infinity
respectively to match the implementations of Math.min()
and Math.max()
:
const object = {
value: 1,
children: {
value: 10,
children: {
value: 2,
children: {
value: 5,
children: null
}
}
}
}
const flat = o => o == null || o.value == null ? : [o.value, ...flat(o.children)]
const [min, max] = flat(object).reduce(
([min, max], value) => [Math.min(min, value), Math.max(max, value)],
[Infinity, -Infinity]
)
console.log(min, max)
add a comment |
up vote
3
down vote
up vote
3
down vote
After flattening your linked list into an array, you can use Array.prototype.reduce()
with an accumulator that is a tuple of min
and max
, starting with initial values of Infinity
and -Infinity
respectively to match the implementations of Math.min()
and Math.max()
:
const object = {
value: 1,
children: {
value: 10,
children: {
value: 2,
children: {
value: 5,
children: null
}
}
}
}
const flat = o => o == null || o.value == null ? : [o.value, ...flat(o.children)]
const [min, max] = flat(object).reduce(
([min, max], value) => [Math.min(min, value), Math.max(max, value)],
[Infinity, -Infinity]
)
console.log(min, max)
After flattening your linked list into an array, you can use Array.prototype.reduce()
with an accumulator that is a tuple of min
and max
, starting with initial values of Infinity
and -Infinity
respectively to match the implementations of Math.min()
and Math.max()
:
const object = {
value: 1,
children: {
value: 10,
children: {
value: 2,
children: {
value: 5,
children: null
}
}
}
}
const flat = o => o == null || o.value == null ? : [o.value, ...flat(o.children)]
const [min, max] = flat(object).reduce(
([min, max], value) => [Math.min(min, value), Math.max(max, value)],
[Infinity, -Infinity]
)
console.log(min, max)
const object = {
value: 1,
children: {
value: 10,
children: {
value: 2,
children: {
value: 5,
children: null
}
}
}
}
const flat = o => o == null || o.value == null ? : [o.value, ...flat(o.children)]
const [min, max] = flat(object).reduce(
([min, max], value) => [Math.min(min, value), Math.max(max, value)],
[Infinity, -Infinity]
)
console.log(min, max)
const object = {
value: 1,
children: {
value: 10,
children: {
value: 2,
children: {
value: 5,
children: null
}
}
}
}
const flat = o => o == null || o.value == null ? : [o.value, ...flat(o.children)]
const [min, max] = flat(object).reduce(
([min, max], value) => [Math.min(min, value), Math.max(max, value)],
[Infinity, -Infinity]
)
console.log(min, max)
answered Nov 19 at 16:50
Patrick Roberts
18.3k23372
18.3k23372
add a comment |
add a comment |
up vote
2
down vote
Since children
is an object with only one value (vs an array with potentially many), this is a pretty simple recursive function. The base case is when there are no children in which case both min and max are just the value. Otherwise recurse on the children to find the min and max:
var object = {
value: -10,
children: {
value: 4,
children:{
value: 200,
children: {
value: -100,
children: null
}
}
}
}
function getMinMax(obj) {
if (!obj.children || obj.children.value == undefined)
return {min: obj.value, max: obj.value}
else {
let m = getMinMax(obj.children)
return {min: Math.min(obj.value, m.min), max: Math.max(obj.value, m.max)}
}
}
console.log(getMinMax(object))
add a comment |
up vote
2
down vote
Since children
is an object with only one value (vs an array with potentially many), this is a pretty simple recursive function. The base case is when there are no children in which case both min and max are just the value. Otherwise recurse on the children to find the min and max:
var object = {
value: -10,
children: {
value: 4,
children:{
value: 200,
children: {
value: -100,
children: null
}
}
}
}
function getMinMax(obj) {
if (!obj.children || obj.children.value == undefined)
return {min: obj.value, max: obj.value}
else {
let m = getMinMax(obj.children)
return {min: Math.min(obj.value, m.min), max: Math.max(obj.value, m.max)}
}
}
console.log(getMinMax(object))
add a comment |
up vote
2
down vote
up vote
2
down vote
Since children
is an object with only one value (vs an array with potentially many), this is a pretty simple recursive function. The base case is when there are no children in which case both min and max are just the value. Otherwise recurse on the children to find the min and max:
var object = {
value: -10,
children: {
value: 4,
children:{
value: 200,
children: {
value: -100,
children: null
}
}
}
}
function getMinMax(obj) {
if (!obj.children || obj.children.value == undefined)
return {min: obj.value, max: obj.value}
else {
let m = getMinMax(obj.children)
return {min: Math.min(obj.value, m.min), max: Math.max(obj.value, m.max)}
}
}
console.log(getMinMax(object))
Since children
is an object with only one value (vs an array with potentially many), this is a pretty simple recursive function. The base case is when there are no children in which case both min and max are just the value. Otherwise recurse on the children to find the min and max:
var object = {
value: -10,
children: {
value: 4,
children:{
value: 200,
children: {
value: -100,
children: null
}
}
}
}
function getMinMax(obj) {
if (!obj.children || obj.children.value == undefined)
return {min: obj.value, max: obj.value}
else {
let m = getMinMax(obj.children)
return {min: Math.min(obj.value, m.min), max: Math.max(obj.value, m.max)}
}
}
console.log(getMinMax(object))
var object = {
value: -10,
children: {
value: 4,
children:{
value: 200,
children: {
value: -100,
children: null
}
}
}
}
function getMinMax(obj) {
if (!obj.children || obj.children.value == undefined)
return {min: obj.value, max: obj.value}
else {
let m = getMinMax(obj.children)
return {min: Math.min(obj.value, m.min), max: Math.max(obj.value, m.max)}
}
}
console.log(getMinMax(object))
var object = {
value: -10,
children: {
value: 4,
children:{
value: 200,
children: {
value: -100,
children: null
}
}
}
}
function getMinMax(obj) {
if (!obj.children || obj.children.value == undefined)
return {min: obj.value, max: obj.value}
else {
let m = getMinMax(obj.children)
return {min: Math.min(obj.value, m.min), max: Math.max(obj.value, m.max)}
}
}
console.log(getMinMax(object))
edited Nov 19 at 17:18
answered Nov 19 at 16:51


Mark Meyer
30.6k32550
30.6k32550
add a comment |
add a comment |
up vote
1
down vote
Short and simple, for min change Math.max
to Math.min
var test = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {}
}
}
}
function findMaxValue(obj) {
if (Object.keys(obj.children).length === 0) {
return obj.value;
}
return Math.max(obj.value, findMaxValue(obj.children))
}
console.log(findMaxValue(test))
add a comment |
up vote
1
down vote
Short and simple, for min change Math.max
to Math.min
var test = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {}
}
}
}
function findMaxValue(obj) {
if (Object.keys(obj.children).length === 0) {
return obj.value;
}
return Math.max(obj.value, findMaxValue(obj.children))
}
console.log(findMaxValue(test))
add a comment |
up vote
1
down vote
up vote
1
down vote
Short and simple, for min change Math.max
to Math.min
var test = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {}
}
}
}
function findMaxValue(obj) {
if (Object.keys(obj.children).length === 0) {
return obj.value;
}
return Math.max(obj.value, findMaxValue(obj.children))
}
console.log(findMaxValue(test))
Short and simple, for min change Math.max
to Math.min
var test = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {}
}
}
}
function findMaxValue(obj) {
if (Object.keys(obj.children).length === 0) {
return obj.value;
}
return Math.max(obj.value, findMaxValue(obj.children))
}
console.log(findMaxValue(test))
var test = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {}
}
}
}
function findMaxValue(obj) {
if (Object.keys(obj.children).length === 0) {
return obj.value;
}
return Math.max(obj.value, findMaxValue(obj.children))
}
console.log(findMaxValue(test))
var test = {
value: 1,
children: {
value: 10,
children:{
value: 2,
children: {}
}
}
}
function findMaxValue(obj) {
if (Object.keys(obj.children).length === 0) {
return obj.value;
}
return Math.max(obj.value, findMaxValue(obj.children))
}
console.log(findMaxValue(test))
answered Nov 19 at 16:51


dotconnor
87416
87416
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53379069%2ffind-the-minimum-and-maximum-values-in-the-nested-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oo,k 9DGau7 UmwT3vt0uRm
3
What was your previous unsuccessful attempt?
– Robin Zigmond
Nov 19 at 16:39
Reopening. The linked question is quite different: no recursion is needed in that one..
– Scott Sauyet
Nov 19 at 17:00