Access to folder Denied C#
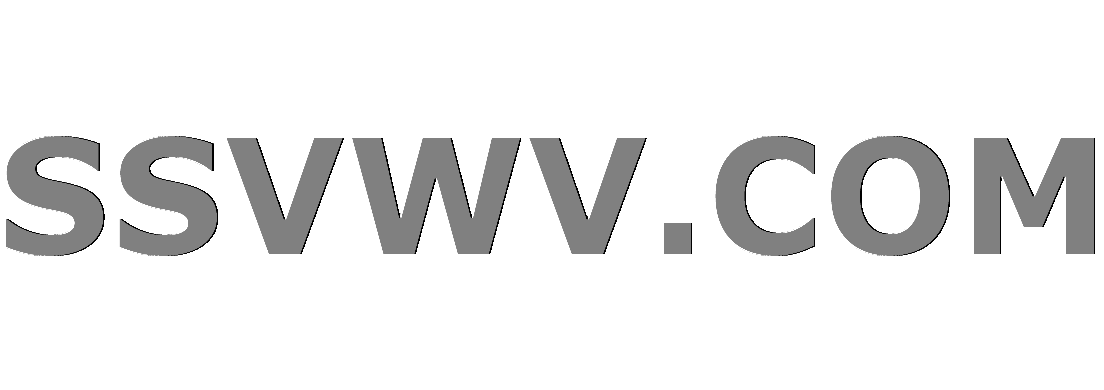
Multi tool use
I'm a fairly new C# Programmer, and I've run into an error beyond my ability to fix.
Currently I'm working on coding a discord bot, and upon trying to instantiate and Program
object, it returns an "Access is denied error".
The issue is that the error is referring to a folder, not a file and I've tried a bunch of stuff to fix it.
- Running Visual Studio as administrator
- Ensuring my account has permissions to access the files and folder
- Changing the location of the project files
- Restarting visual Studio
- Recoding the project off a clean sheet
- Ensuring the folder and files are not "Read Only"
The error is thrown at this line: => new Program().MainAsync().GetAwaiter().GetResult();
I'm basically out of ideas at this point. The full details of the exception message are as follows:
System.UnauthorizedAccessException
HResult=0x80070005
Message=Access to the path 'C:UsersXXXsourcereposdiscordBotdiscordBotbinDebug' is denied.
Source=mscorlib
StackTrace:
at System.IO.__Error.WinIOError(Int32 errorCode, String maybeFullPath)
at System.IO.FileStream.Init(String path, FileMode mode, FileAccess access, Int32 rights, Boolean useRights, FileShare share, Int32 bufferSize, FileOptions options, SECURITY_ATTRIBUTES secAttrs, String msgPath, Boolean bFromProxy, Boolean useLongPath, Boolean checkHost)
at System.IO.FileStream..ctor(String path, FileMode mode, FileAccess access)
at train_bot.Program.d__3.MoveNext() in C:UsersXXXsourcereposdiscordBotdiscordBotProgram.cs:line 46
at System.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.GetResult()
at train_bot.Program.Main(String args) in C:UsersXXXsourcereposdiscordBotdiscordBotProgram.cs:line 21
The less detailed version
System.UnauthorizedAccessException: 'Access to the path 'C:UsersSettingAdminsourcereposdiscordBotdiscordBotbinDebug' is denied.'
using System;
using System.IO;
using System.Reflection;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Discord;
using Discord.Commands;
using Discord.WebSocket;
namespace train_bot
{
class Program
{
private DiscordSocketClient Client;
private CommandService Commands;
static void Main(string args)
=> new Program().MainAsync().GetAwaiter().GetResult();
private async Task MainAsync()
{
//configuring client
Client = new DiscordSocketClient(new DiscordSocketConfig
{
LogLevel = LogSeverity.Debug //changes detail in log
});
Commands = new CommandService(new CommandServiceConfig
{
CaseSensitiveCommands = true,
DefaultRunMode = RunMode.Async,
LogLevel = LogSeverity.Debug
});
Client.MessageReceived += Client_MessageReceived;
await Commands.AddModulesAsync(Assembly.GetEntryAssembly());
Client.Ready += Client_Ready;
Client.Log += Client_Log;
string Token = "";
using (var Steam = new FileStream(Path.GetDirectoryName(Assembly.GetEntryAssembly().Location).Replace(@"binDebugnetcoreapp2.0", @"Token.txt"), FileMode.Open, FileAccess.Read))using (var ReadToken = new StreamReader(Steam))
{
Token = ReadToken.ReadToEnd();
}
await Client.LoginAsync(TokenType.Bot, Token);
await Client.StartAsync();
await Task.Delay(-1);
}
private async Task Client_Log(LogMessage Message)
{
Console.WriteLine($"{DateTime.Now} at {Message.Source}] {Message.Message}");
}
private async Task Client_Ready()
{
await Client.SetGameAsync("Hentai King 2018", "", StreamType.NotStreaming);
}
private async Task Client_MessageReceived(SocketMessage arg)
{
//Configure the commands
}
}
}
c# access-denied
add a comment |
I'm a fairly new C# Programmer, and I've run into an error beyond my ability to fix.
Currently I'm working on coding a discord bot, and upon trying to instantiate and Program
object, it returns an "Access is denied error".
The issue is that the error is referring to a folder, not a file and I've tried a bunch of stuff to fix it.
- Running Visual Studio as administrator
- Ensuring my account has permissions to access the files and folder
- Changing the location of the project files
- Restarting visual Studio
- Recoding the project off a clean sheet
- Ensuring the folder and files are not "Read Only"
The error is thrown at this line: => new Program().MainAsync().GetAwaiter().GetResult();
I'm basically out of ideas at this point. The full details of the exception message are as follows:
System.UnauthorizedAccessException
HResult=0x80070005
Message=Access to the path 'C:UsersXXXsourcereposdiscordBotdiscordBotbinDebug' is denied.
Source=mscorlib
StackTrace:
at System.IO.__Error.WinIOError(Int32 errorCode, String maybeFullPath)
at System.IO.FileStream.Init(String path, FileMode mode, FileAccess access, Int32 rights, Boolean useRights, FileShare share, Int32 bufferSize, FileOptions options, SECURITY_ATTRIBUTES secAttrs, String msgPath, Boolean bFromProxy, Boolean useLongPath, Boolean checkHost)
at System.IO.FileStream..ctor(String path, FileMode mode, FileAccess access)
at train_bot.Program.d__3.MoveNext() in C:UsersXXXsourcereposdiscordBotdiscordBotProgram.cs:line 46
at System.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.GetResult()
at train_bot.Program.Main(String args) in C:UsersXXXsourcereposdiscordBotdiscordBotProgram.cs:line 21
The less detailed version
System.UnauthorizedAccessException: 'Access to the path 'C:UsersSettingAdminsourcereposdiscordBotdiscordBotbinDebug' is denied.'
using System;
using System.IO;
using System.Reflection;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Discord;
using Discord.Commands;
using Discord.WebSocket;
namespace train_bot
{
class Program
{
private DiscordSocketClient Client;
private CommandService Commands;
static void Main(string args)
=> new Program().MainAsync().GetAwaiter().GetResult();
private async Task MainAsync()
{
//configuring client
Client = new DiscordSocketClient(new DiscordSocketConfig
{
LogLevel = LogSeverity.Debug //changes detail in log
});
Commands = new CommandService(new CommandServiceConfig
{
CaseSensitiveCommands = true,
DefaultRunMode = RunMode.Async,
LogLevel = LogSeverity.Debug
});
Client.MessageReceived += Client_MessageReceived;
await Commands.AddModulesAsync(Assembly.GetEntryAssembly());
Client.Ready += Client_Ready;
Client.Log += Client_Log;
string Token = "";
using (var Steam = new FileStream(Path.GetDirectoryName(Assembly.GetEntryAssembly().Location).Replace(@"binDebugnetcoreapp2.0", @"Token.txt"), FileMode.Open, FileAccess.Read))using (var ReadToken = new StreamReader(Steam))
{
Token = ReadToken.ReadToEnd();
}
await Client.LoginAsync(TokenType.Bot, Token);
await Client.StartAsync();
await Task.Delay(-1);
}
private async Task Client_Log(LogMessage Message)
{
Console.WriteLine($"{DateTime.Now} at {Message.Source}] {Message.Message}");
}
private async Task Client_Ready()
{
await Client.SetGameAsync("Hentai King 2018", "", StreamType.NotStreaming);
}
private async Task Client_MessageReceived(SocketMessage arg)
{
//Configure the commands
}
}
}
c# access-denied
Set access permission to that folder from folder properties!
– TanvirArjel
Nov 25 '18 at 16:40
add a comment |
I'm a fairly new C# Programmer, and I've run into an error beyond my ability to fix.
Currently I'm working on coding a discord bot, and upon trying to instantiate and Program
object, it returns an "Access is denied error".
The issue is that the error is referring to a folder, not a file and I've tried a bunch of stuff to fix it.
- Running Visual Studio as administrator
- Ensuring my account has permissions to access the files and folder
- Changing the location of the project files
- Restarting visual Studio
- Recoding the project off a clean sheet
- Ensuring the folder and files are not "Read Only"
The error is thrown at this line: => new Program().MainAsync().GetAwaiter().GetResult();
I'm basically out of ideas at this point. The full details of the exception message are as follows:
System.UnauthorizedAccessException
HResult=0x80070005
Message=Access to the path 'C:UsersXXXsourcereposdiscordBotdiscordBotbinDebug' is denied.
Source=mscorlib
StackTrace:
at System.IO.__Error.WinIOError(Int32 errorCode, String maybeFullPath)
at System.IO.FileStream.Init(String path, FileMode mode, FileAccess access, Int32 rights, Boolean useRights, FileShare share, Int32 bufferSize, FileOptions options, SECURITY_ATTRIBUTES secAttrs, String msgPath, Boolean bFromProxy, Boolean useLongPath, Boolean checkHost)
at System.IO.FileStream..ctor(String path, FileMode mode, FileAccess access)
at train_bot.Program.d__3.MoveNext() in C:UsersXXXsourcereposdiscordBotdiscordBotProgram.cs:line 46
at System.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.GetResult()
at train_bot.Program.Main(String args) in C:UsersXXXsourcereposdiscordBotdiscordBotProgram.cs:line 21
The less detailed version
System.UnauthorizedAccessException: 'Access to the path 'C:UsersSettingAdminsourcereposdiscordBotdiscordBotbinDebug' is denied.'
using System;
using System.IO;
using System.Reflection;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Discord;
using Discord.Commands;
using Discord.WebSocket;
namespace train_bot
{
class Program
{
private DiscordSocketClient Client;
private CommandService Commands;
static void Main(string args)
=> new Program().MainAsync().GetAwaiter().GetResult();
private async Task MainAsync()
{
//configuring client
Client = new DiscordSocketClient(new DiscordSocketConfig
{
LogLevel = LogSeverity.Debug //changes detail in log
});
Commands = new CommandService(new CommandServiceConfig
{
CaseSensitiveCommands = true,
DefaultRunMode = RunMode.Async,
LogLevel = LogSeverity.Debug
});
Client.MessageReceived += Client_MessageReceived;
await Commands.AddModulesAsync(Assembly.GetEntryAssembly());
Client.Ready += Client_Ready;
Client.Log += Client_Log;
string Token = "";
using (var Steam = new FileStream(Path.GetDirectoryName(Assembly.GetEntryAssembly().Location).Replace(@"binDebugnetcoreapp2.0", @"Token.txt"), FileMode.Open, FileAccess.Read))using (var ReadToken = new StreamReader(Steam))
{
Token = ReadToken.ReadToEnd();
}
await Client.LoginAsync(TokenType.Bot, Token);
await Client.StartAsync();
await Task.Delay(-1);
}
private async Task Client_Log(LogMessage Message)
{
Console.WriteLine($"{DateTime.Now} at {Message.Source}] {Message.Message}");
}
private async Task Client_Ready()
{
await Client.SetGameAsync("Hentai King 2018", "", StreamType.NotStreaming);
}
private async Task Client_MessageReceived(SocketMessage arg)
{
//Configure the commands
}
}
}
c# access-denied
I'm a fairly new C# Programmer, and I've run into an error beyond my ability to fix.
Currently I'm working on coding a discord bot, and upon trying to instantiate and Program
object, it returns an "Access is denied error".
The issue is that the error is referring to a folder, not a file and I've tried a bunch of stuff to fix it.
- Running Visual Studio as administrator
- Ensuring my account has permissions to access the files and folder
- Changing the location of the project files
- Restarting visual Studio
- Recoding the project off a clean sheet
- Ensuring the folder and files are not "Read Only"
The error is thrown at this line: => new Program().MainAsync().GetAwaiter().GetResult();
I'm basically out of ideas at this point. The full details of the exception message are as follows:
System.UnauthorizedAccessException
HResult=0x80070005
Message=Access to the path 'C:UsersXXXsourcereposdiscordBotdiscordBotbinDebug' is denied.
Source=mscorlib
StackTrace:
at System.IO.__Error.WinIOError(Int32 errorCode, String maybeFullPath)
at System.IO.FileStream.Init(String path, FileMode mode, FileAccess access, Int32 rights, Boolean useRights, FileShare share, Int32 bufferSize, FileOptions options, SECURITY_ATTRIBUTES secAttrs, String msgPath, Boolean bFromProxy, Boolean useLongPath, Boolean checkHost)
at System.IO.FileStream..ctor(String path, FileMode mode, FileAccess access)
at train_bot.Program.d__3.MoveNext() in C:UsersXXXsourcereposdiscordBotdiscordBotProgram.cs:line 46
at System.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at System.Runtime.CompilerServices.TaskAwaiter.GetResult()
at train_bot.Program.Main(String args) in C:UsersXXXsourcereposdiscordBotdiscordBotProgram.cs:line 21
The less detailed version
System.UnauthorizedAccessException: 'Access to the path 'C:UsersSettingAdminsourcereposdiscordBotdiscordBotbinDebug' is denied.'
using System;
using System.IO;
using System.Reflection;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Discord;
using Discord.Commands;
using Discord.WebSocket;
namespace train_bot
{
class Program
{
private DiscordSocketClient Client;
private CommandService Commands;
static void Main(string args)
=> new Program().MainAsync().GetAwaiter().GetResult();
private async Task MainAsync()
{
//configuring client
Client = new DiscordSocketClient(new DiscordSocketConfig
{
LogLevel = LogSeverity.Debug //changes detail in log
});
Commands = new CommandService(new CommandServiceConfig
{
CaseSensitiveCommands = true,
DefaultRunMode = RunMode.Async,
LogLevel = LogSeverity.Debug
});
Client.MessageReceived += Client_MessageReceived;
await Commands.AddModulesAsync(Assembly.GetEntryAssembly());
Client.Ready += Client_Ready;
Client.Log += Client_Log;
string Token = "";
using (var Steam = new FileStream(Path.GetDirectoryName(Assembly.GetEntryAssembly().Location).Replace(@"binDebugnetcoreapp2.0", @"Token.txt"), FileMode.Open, FileAccess.Read))using (var ReadToken = new StreamReader(Steam))
{
Token = ReadToken.ReadToEnd();
}
await Client.LoginAsync(TokenType.Bot, Token);
await Client.StartAsync();
await Task.Delay(-1);
}
private async Task Client_Log(LogMessage Message)
{
Console.WriteLine($"{DateTime.Now} at {Message.Source}] {Message.Message}");
}
private async Task Client_Ready()
{
await Client.SetGameAsync("Hentai King 2018", "", StreamType.NotStreaming);
}
private async Task Client_MessageReceived(SocketMessage arg)
{
//Configure the commands
}
}
}
c# access-denied
c# access-denied
asked Nov 25 '18 at 16:33
Angus LongmoreAngus Longmore
61
61
Set access permission to that folder from folder properties!
– TanvirArjel
Nov 25 '18 at 16:40
add a comment |
Set access permission to that folder from folder properties!
– TanvirArjel
Nov 25 '18 at 16:40
Set access permission to that folder from folder properties!
– TanvirArjel
Nov 25 '18 at 16:40
Set access permission to that folder from folder properties!
– TanvirArjel
Nov 25 '18 at 16:40
add a comment |
2 Answers
2
active
oldest
votes
The problem may be that you try to open a directory as file. The path you construct is:
Path.GetDirectoryName(Assembly.GetEntryAssembly().Location).Replace(@"binDebugnetcoreapp2.0", @"Token.txt")
This would only work if Assembly.GetEntryAssembly().Location indeed contains the string @"binDebugnetcoreapp2.0".
You probably intended something like
Path.Combine(Path.GetDirectoryName(Assembly.GetEntryAssembly().Location)), @"Token.txt")
agree. You can reproduce the Access Denied error with any directory:using (var stream = new FileStream( Directory.GetCurrentDirectory() , FileMode.Open, FileAccess.Read)) {}
. To my mind, the framework should really give a more explicit error saying "can't open a directory as a file"
– Chris F Carroll
Nov 25 '18 at 16:49
@ChrisFCarroll: It was established as the "fallback error" decades ago. Same way "file not found" would be the response of Windows 95 era programms if it ran into NTFS rights. So now they can not change it anymore without risking to break code. Maybe at least .NET Core has a bit more telling exception? Asuming they did not put the exact exceptions into the .NET Standard itself, of course.
– Christopher
Nov 25 '18 at 16:54
Okay, apparently Filestream is part of the .NET Standard since 1.3. So that behavior will not change. however the description is interesting: "The access requested is not permitted by the operating system for the specified path, such as when access is Write or ReadWrite and the file or directory is set for read-only access." It always took a path, not a filepath. And for many purposes, folders are realized as a sort-off file on the disk. And then there are Zip containers that are largely treated as a literal folder on the disk since Vista.
– Christopher
Nov 25 '18 at 16:59
Thank you :), I implemented what you suggested, changed the location of the .txt file and it worked.. Really appreciate it
– Angus Longmore
Nov 25 '18 at 17:01
add a comment |
Like the error said, you don't have access to that folder. If you are running it on debug mode make sure you run visual studio as administrator so you get to ignore all that on dev environment. If its deployed, make sure the the account running your program has appropriate rights to the folder.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53469576%2faccess-to-folder-denied-c-sharp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The problem may be that you try to open a directory as file. The path you construct is:
Path.GetDirectoryName(Assembly.GetEntryAssembly().Location).Replace(@"binDebugnetcoreapp2.0", @"Token.txt")
This would only work if Assembly.GetEntryAssembly().Location indeed contains the string @"binDebugnetcoreapp2.0".
You probably intended something like
Path.Combine(Path.GetDirectoryName(Assembly.GetEntryAssembly().Location)), @"Token.txt")
agree. You can reproduce the Access Denied error with any directory:using (var stream = new FileStream( Directory.GetCurrentDirectory() , FileMode.Open, FileAccess.Read)) {}
. To my mind, the framework should really give a more explicit error saying "can't open a directory as a file"
– Chris F Carroll
Nov 25 '18 at 16:49
@ChrisFCarroll: It was established as the "fallback error" decades ago. Same way "file not found" would be the response of Windows 95 era programms if it ran into NTFS rights. So now they can not change it anymore without risking to break code. Maybe at least .NET Core has a bit more telling exception? Asuming they did not put the exact exceptions into the .NET Standard itself, of course.
– Christopher
Nov 25 '18 at 16:54
Okay, apparently Filestream is part of the .NET Standard since 1.3. So that behavior will not change. however the description is interesting: "The access requested is not permitted by the operating system for the specified path, such as when access is Write or ReadWrite and the file or directory is set for read-only access." It always took a path, not a filepath. And for many purposes, folders are realized as a sort-off file on the disk. And then there are Zip containers that are largely treated as a literal folder on the disk since Vista.
– Christopher
Nov 25 '18 at 16:59
Thank you :), I implemented what you suggested, changed the location of the .txt file and it worked.. Really appreciate it
– Angus Longmore
Nov 25 '18 at 17:01
add a comment |
The problem may be that you try to open a directory as file. The path you construct is:
Path.GetDirectoryName(Assembly.GetEntryAssembly().Location).Replace(@"binDebugnetcoreapp2.0", @"Token.txt")
This would only work if Assembly.GetEntryAssembly().Location indeed contains the string @"binDebugnetcoreapp2.0".
You probably intended something like
Path.Combine(Path.GetDirectoryName(Assembly.GetEntryAssembly().Location)), @"Token.txt")
agree. You can reproduce the Access Denied error with any directory:using (var stream = new FileStream( Directory.GetCurrentDirectory() , FileMode.Open, FileAccess.Read)) {}
. To my mind, the framework should really give a more explicit error saying "can't open a directory as a file"
– Chris F Carroll
Nov 25 '18 at 16:49
@ChrisFCarroll: It was established as the "fallback error" decades ago. Same way "file not found" would be the response of Windows 95 era programms if it ran into NTFS rights. So now they can not change it anymore without risking to break code. Maybe at least .NET Core has a bit more telling exception? Asuming they did not put the exact exceptions into the .NET Standard itself, of course.
– Christopher
Nov 25 '18 at 16:54
Okay, apparently Filestream is part of the .NET Standard since 1.3. So that behavior will not change. however the description is interesting: "The access requested is not permitted by the operating system for the specified path, such as when access is Write or ReadWrite and the file or directory is set for read-only access." It always took a path, not a filepath. And for many purposes, folders are realized as a sort-off file on the disk. And then there are Zip containers that are largely treated as a literal folder on the disk since Vista.
– Christopher
Nov 25 '18 at 16:59
Thank you :), I implemented what you suggested, changed the location of the .txt file and it worked.. Really appreciate it
– Angus Longmore
Nov 25 '18 at 17:01
add a comment |
The problem may be that you try to open a directory as file. The path you construct is:
Path.GetDirectoryName(Assembly.GetEntryAssembly().Location).Replace(@"binDebugnetcoreapp2.0", @"Token.txt")
This would only work if Assembly.GetEntryAssembly().Location indeed contains the string @"binDebugnetcoreapp2.0".
You probably intended something like
Path.Combine(Path.GetDirectoryName(Assembly.GetEntryAssembly().Location)), @"Token.txt")
The problem may be that you try to open a directory as file. The path you construct is:
Path.GetDirectoryName(Assembly.GetEntryAssembly().Location).Replace(@"binDebugnetcoreapp2.0", @"Token.txt")
This would only work if Assembly.GetEntryAssembly().Location indeed contains the string @"binDebugnetcoreapp2.0".
You probably intended something like
Path.Combine(Path.GetDirectoryName(Assembly.GetEntryAssembly().Location)), @"Token.txt")
answered Nov 25 '18 at 16:43


Klaus GütterKlaus Gütter
2,56721321
2,56721321
agree. You can reproduce the Access Denied error with any directory:using (var stream = new FileStream( Directory.GetCurrentDirectory() , FileMode.Open, FileAccess.Read)) {}
. To my mind, the framework should really give a more explicit error saying "can't open a directory as a file"
– Chris F Carroll
Nov 25 '18 at 16:49
@ChrisFCarroll: It was established as the "fallback error" decades ago. Same way "file not found" would be the response of Windows 95 era programms if it ran into NTFS rights. So now they can not change it anymore without risking to break code. Maybe at least .NET Core has a bit more telling exception? Asuming they did not put the exact exceptions into the .NET Standard itself, of course.
– Christopher
Nov 25 '18 at 16:54
Okay, apparently Filestream is part of the .NET Standard since 1.3. So that behavior will not change. however the description is interesting: "The access requested is not permitted by the operating system for the specified path, such as when access is Write or ReadWrite and the file or directory is set for read-only access." It always took a path, not a filepath. And for many purposes, folders are realized as a sort-off file on the disk. And then there are Zip containers that are largely treated as a literal folder on the disk since Vista.
– Christopher
Nov 25 '18 at 16:59
Thank you :), I implemented what you suggested, changed the location of the .txt file and it worked.. Really appreciate it
– Angus Longmore
Nov 25 '18 at 17:01
add a comment |
agree. You can reproduce the Access Denied error with any directory:using (var stream = new FileStream( Directory.GetCurrentDirectory() , FileMode.Open, FileAccess.Read)) {}
. To my mind, the framework should really give a more explicit error saying "can't open a directory as a file"
– Chris F Carroll
Nov 25 '18 at 16:49
@ChrisFCarroll: It was established as the "fallback error" decades ago. Same way "file not found" would be the response of Windows 95 era programms if it ran into NTFS rights. So now they can not change it anymore without risking to break code. Maybe at least .NET Core has a bit more telling exception? Asuming they did not put the exact exceptions into the .NET Standard itself, of course.
– Christopher
Nov 25 '18 at 16:54
Okay, apparently Filestream is part of the .NET Standard since 1.3. So that behavior will not change. however the description is interesting: "The access requested is not permitted by the operating system for the specified path, such as when access is Write or ReadWrite and the file or directory is set for read-only access." It always took a path, not a filepath. And for many purposes, folders are realized as a sort-off file on the disk. And then there are Zip containers that are largely treated as a literal folder on the disk since Vista.
– Christopher
Nov 25 '18 at 16:59
Thank you :), I implemented what you suggested, changed the location of the .txt file and it worked.. Really appreciate it
– Angus Longmore
Nov 25 '18 at 17:01
agree. You can reproduce the Access Denied error with any directory:
using (var stream = new FileStream( Directory.GetCurrentDirectory() , FileMode.Open, FileAccess.Read)) {}
. To my mind, the framework should really give a more explicit error saying "can't open a directory as a file"– Chris F Carroll
Nov 25 '18 at 16:49
agree. You can reproduce the Access Denied error with any directory:
using (var stream = new FileStream( Directory.GetCurrentDirectory() , FileMode.Open, FileAccess.Read)) {}
. To my mind, the framework should really give a more explicit error saying "can't open a directory as a file"– Chris F Carroll
Nov 25 '18 at 16:49
@ChrisFCarroll: It was established as the "fallback error" decades ago. Same way "file not found" would be the response of Windows 95 era programms if it ran into NTFS rights. So now they can not change it anymore without risking to break code. Maybe at least .NET Core has a bit more telling exception? Asuming they did not put the exact exceptions into the .NET Standard itself, of course.
– Christopher
Nov 25 '18 at 16:54
@ChrisFCarroll: It was established as the "fallback error" decades ago. Same way "file not found" would be the response of Windows 95 era programms if it ran into NTFS rights. So now they can not change it anymore without risking to break code. Maybe at least .NET Core has a bit more telling exception? Asuming they did not put the exact exceptions into the .NET Standard itself, of course.
– Christopher
Nov 25 '18 at 16:54
Okay, apparently Filestream is part of the .NET Standard since 1.3. So that behavior will not change. however the description is interesting: "The access requested is not permitted by the operating system for the specified path, such as when access is Write or ReadWrite and the file or directory is set for read-only access." It always took a path, not a filepath. And for many purposes, folders are realized as a sort-off file on the disk. And then there are Zip containers that are largely treated as a literal folder on the disk since Vista.
– Christopher
Nov 25 '18 at 16:59
Okay, apparently Filestream is part of the .NET Standard since 1.3. So that behavior will not change. however the description is interesting: "The access requested is not permitted by the operating system for the specified path, such as when access is Write or ReadWrite and the file or directory is set for read-only access." It always took a path, not a filepath. And for many purposes, folders are realized as a sort-off file on the disk. And then there are Zip containers that are largely treated as a literal folder on the disk since Vista.
– Christopher
Nov 25 '18 at 16:59
Thank you :), I implemented what you suggested, changed the location of the .txt file and it worked.. Really appreciate it
– Angus Longmore
Nov 25 '18 at 17:01
Thank you :), I implemented what you suggested, changed the location of the .txt file and it worked.. Really appreciate it
– Angus Longmore
Nov 25 '18 at 17:01
add a comment |
Like the error said, you don't have access to that folder. If you are running it on debug mode make sure you run visual studio as administrator so you get to ignore all that on dev environment. If its deployed, make sure the the account running your program has appropriate rights to the folder.
add a comment |
Like the error said, you don't have access to that folder. If you are running it on debug mode make sure you run visual studio as administrator so you get to ignore all that on dev environment. If its deployed, make sure the the account running your program has appropriate rights to the folder.
add a comment |
Like the error said, you don't have access to that folder. If you are running it on debug mode make sure you run visual studio as administrator so you get to ignore all that on dev environment. If its deployed, make sure the the account running your program has appropriate rights to the folder.
Like the error said, you don't have access to that folder. If you are running it on debug mode make sure you run visual studio as administrator so you get to ignore all that on dev environment. If its deployed, make sure the the account running your program has appropriate rights to the folder.
answered Nov 25 '18 at 16:49
calingasancalingasan
31423
31423
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53469576%2faccess-to-folder-denied-c-sharp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bir,hs Lfm7 1YVhpMH kC3zig3uCiizKO,RzuUNU Xv7KOlHz wW
Set access permission to that folder from folder properties!
– TanvirArjel
Nov 25 '18 at 16:40