The best way to use full-text search in django-filters' FilterSet?
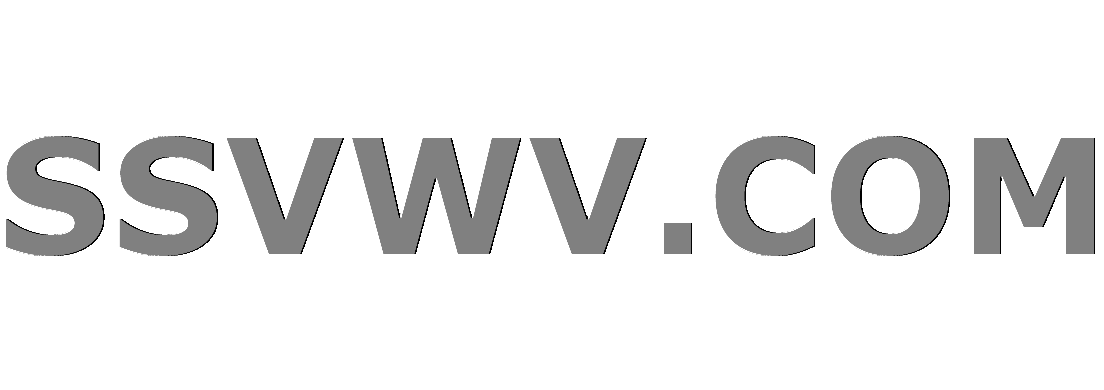
Multi tool use
My app uses rest-framework
and django-filter
. I use this FilterSet
to provide filtering list of articles (ModelViewSet
) by date
:
from django_filters import rest_framework as filters
class ArticleFilter(filters.FilterSet):
start_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='gte')
end_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='lte')
class Meta:
model = Article
fields = ['pub_date']
I want to add search box to my app, so I need another filter, that will use full-text search at my Article
model. Fields I would to search are title
and description
. I decided to add search
field with method
parameter like that:
from django_filters import rest_framework as filters
from django.contrib.postgres.search import SearchVector
class ArticleFilter(filters.FilterSet):
start_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='gte')
end_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='lte')
search = filters.CharFilter(method='filter_search')
def filter_search(self, queryset, name, value):
return queryset.annotate(search=SearchVector('title', 'description')).filter(search=value)
class Meta:
model = Article
fields = ['pub_date']
It works, but I'm not sure that it's the best way of using full-text search filtering. Am I missing something or this approach is ok?
django django-rest-framework django-filters
add a comment |
My app uses rest-framework
and django-filter
. I use this FilterSet
to provide filtering list of articles (ModelViewSet
) by date
:
from django_filters import rest_framework as filters
class ArticleFilter(filters.FilterSet):
start_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='gte')
end_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='lte')
class Meta:
model = Article
fields = ['pub_date']
I want to add search box to my app, so I need another filter, that will use full-text search at my Article
model. Fields I would to search are title
and description
. I decided to add search
field with method
parameter like that:
from django_filters import rest_framework as filters
from django.contrib.postgres.search import SearchVector
class ArticleFilter(filters.FilterSet):
start_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='gte')
end_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='lte')
search = filters.CharFilter(method='filter_search')
def filter_search(self, queryset, name, value):
return queryset.annotate(search=SearchVector('title', 'description')).filter(search=value)
class Meta:
model = Article
fields = ['pub_date']
It works, but I'm not sure that it's the best way of using full-text search filtering. Am I missing something or this approach is ok?
django django-rest-framework django-filters
add a comment |
My app uses rest-framework
and django-filter
. I use this FilterSet
to provide filtering list of articles (ModelViewSet
) by date
:
from django_filters import rest_framework as filters
class ArticleFilter(filters.FilterSet):
start_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='gte')
end_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='lte')
class Meta:
model = Article
fields = ['pub_date']
I want to add search box to my app, so I need another filter, that will use full-text search at my Article
model. Fields I would to search are title
and description
. I decided to add search
field with method
parameter like that:
from django_filters import rest_framework as filters
from django.contrib.postgres.search import SearchVector
class ArticleFilter(filters.FilterSet):
start_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='gte')
end_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='lte')
search = filters.CharFilter(method='filter_search')
def filter_search(self, queryset, name, value):
return queryset.annotate(search=SearchVector('title', 'description')).filter(search=value)
class Meta:
model = Article
fields = ['pub_date']
It works, but I'm not sure that it's the best way of using full-text search filtering. Am I missing something or this approach is ok?
django django-rest-framework django-filters
My app uses rest-framework
and django-filter
. I use this FilterSet
to provide filtering list of articles (ModelViewSet
) by date
:
from django_filters import rest_framework as filters
class ArticleFilter(filters.FilterSet):
start_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='gte')
end_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='lte')
class Meta:
model = Article
fields = ['pub_date']
I want to add search box to my app, so I need another filter, that will use full-text search at my Article
model. Fields I would to search are title
and description
. I decided to add search
field with method
parameter like that:
from django_filters import rest_framework as filters
from django.contrib.postgres.search import SearchVector
class ArticleFilter(filters.FilterSet):
start_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='gte')
end_date = filters.DateTimeFilter(field_name='pub_date', lookup_expr='lte')
search = filters.CharFilter(method='filter_search')
def filter_search(self, queryset, name, value):
return queryset.annotate(search=SearchVector('title', 'description')).filter(search=value)
class Meta:
model = Article
fields = ['pub_date']
It works, but I'm not sure that it's the best way of using full-text search filtering. Am I missing something or this approach is ok?
django django-rest-framework django-filters
django django-rest-framework django-filters
asked Nov 25 '18 at 11:38
vadimbvadimb
387
387
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
This is ok for a naive text search where you don't have much text to look into in a small-ish database.
When you get into bigger areas you need to either optimize your db for this or move to a dedicated search engine like Solr or ElasticSearch. Postgres has a section on full text searching, as does mysql.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467051%2fthe-best-way-to-use-full-text-search-in-django-filters-filterset%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is ok for a naive text search where you don't have much text to look into in a small-ish database.
When you get into bigger areas you need to either optimize your db for this or move to a dedicated search engine like Solr or ElasticSearch. Postgres has a section on full text searching, as does mysql.
add a comment |
This is ok for a naive text search where you don't have much text to look into in a small-ish database.
When you get into bigger areas you need to either optimize your db for this or move to a dedicated search engine like Solr or ElasticSearch. Postgres has a section on full text searching, as does mysql.
add a comment |
This is ok for a naive text search where you don't have much text to look into in a small-ish database.
When you get into bigger areas you need to either optimize your db for this or move to a dedicated search engine like Solr or ElasticSearch. Postgres has a section on full text searching, as does mysql.
This is ok for a naive text search where you don't have much text to look into in a small-ish database.
When you get into bigger areas you need to either optimize your db for this or move to a dedicated search engine like Solr or ElasticSearch. Postgres has a section on full text searching, as does mysql.
answered Nov 25 '18 at 12:52
JasonJason
6,2851770149
6,2851770149
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467051%2fthe-best-way-to-use-full-text-search-in-django-filters-filterset%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0Fq,hF4ax5VzHtqMCB mXKjH6N,U9UwWu45SonPF02CCPxXFY59k5PANrZVWF98 oNon5RymI