Creating fluent api issue
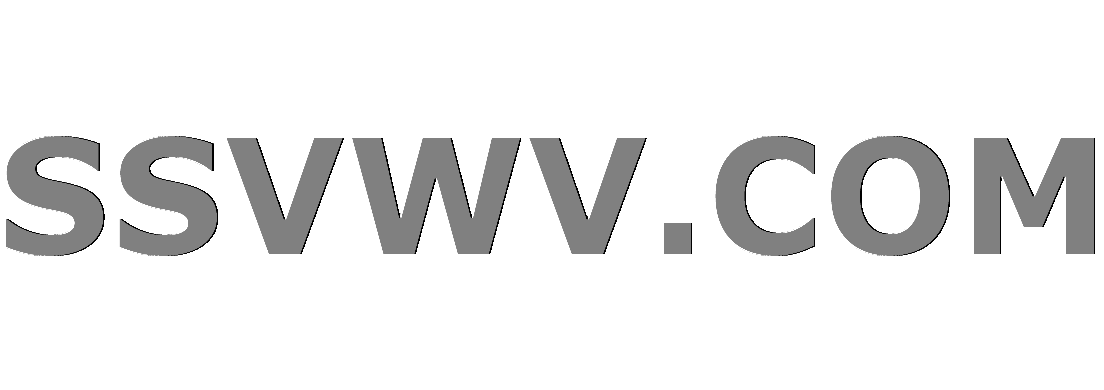
Multi tool use
I'm playing around with creating a fluent api. For my learning, I'm just going to stub out a simple rest client.
What I've got so far is this:
public interface IOAuthAuthentication
{
void AddParameter(string name, string value);
}
public interface IRestClient
{
IRestClient WithBasicAuthentication(string username, string password);
IRestClient WithNetworkAuthentication(string username, string password);
IOAuthAuthentication WithOAuthAuthentication(Uri authorizationUrl, string clientId, string clientSecret);
}
public class RestClient : IRestClient
{
public RestClient(Uri root)
{
}
public IRestClient WithBasicAuthentication(string username, string password)
{
return this;
}
public IRestClient WithNetworkAuthentication(string username, string password)
{
return this;
}
public IOAuthAuthentication WithOAuthAuthentication(Uri authorizationUrl, string clientId, string clientSecret)
{
return null;
}
}
I kind of imagined using it like this:
IRestClient restClient = new RestClient(new Uri(""))
.WithBasicAuthentication("", "")
.WithNetworkAuthentication("", "")
.WithOAuthAuthentication(new Uri(""), "", "")
.AddParameter("", "");
Now, obviously, you wouldn't have 3 different authentication methods, but that would be handled in the methods themselves I suppose.
For the OAuth authentication, the user might need to add a custom parameter, so I defined a different return type... however, this has the side affect of you can't end the chain with WithOAuthAuthentication()
or .AddParameter()
.
What's the correct way to handle that in fluent? I've seen some .net core stuff and was maybe thinking off something like:
WithOAuthAuthentication("", "", "", x => x.AddParameter("", ""))
and have the WithOAuthAuthentication()
return IRestClient.
Would that be more "fluenty"?
But then the code doesn't look as cool if you have to add a bunch of params:
.WithOAuthAuthentication("", "", "", x =>
{
x.AddParameter("", "")
.AddParameter("", "")
});
c#
|
show 8 more comments
I'm playing around with creating a fluent api. For my learning, I'm just going to stub out a simple rest client.
What I've got so far is this:
public interface IOAuthAuthentication
{
void AddParameter(string name, string value);
}
public interface IRestClient
{
IRestClient WithBasicAuthentication(string username, string password);
IRestClient WithNetworkAuthentication(string username, string password);
IOAuthAuthentication WithOAuthAuthentication(Uri authorizationUrl, string clientId, string clientSecret);
}
public class RestClient : IRestClient
{
public RestClient(Uri root)
{
}
public IRestClient WithBasicAuthentication(string username, string password)
{
return this;
}
public IRestClient WithNetworkAuthentication(string username, string password)
{
return this;
}
public IOAuthAuthentication WithOAuthAuthentication(Uri authorizationUrl, string clientId, string clientSecret)
{
return null;
}
}
I kind of imagined using it like this:
IRestClient restClient = new RestClient(new Uri(""))
.WithBasicAuthentication("", "")
.WithNetworkAuthentication("", "")
.WithOAuthAuthentication(new Uri(""), "", "")
.AddParameter("", "");
Now, obviously, you wouldn't have 3 different authentication methods, but that would be handled in the methods themselves I suppose.
For the OAuth authentication, the user might need to add a custom parameter, so I defined a different return type... however, this has the side affect of you can't end the chain with WithOAuthAuthentication()
or .AddParameter()
.
What's the correct way to handle that in fluent? I've seen some .net core stuff and was maybe thinking off something like:
WithOAuthAuthentication("", "", "", x => x.AddParameter("", ""))
and have the WithOAuthAuthentication()
return IRestClient.
Would that be more "fluenty"?
But then the code doesn't look as cool if you have to add a bunch of params:
.WithOAuthAuthentication("", "", "", x =>
{
x.AddParameter("", "")
.AddParameter("", "")
});
c#
For the OAuth authentication, the user might need to add a custom parameter, so I defined a different return type
-- I don't understand what you mean. For Fluent Interfaces, the return type of a method is always going to bethis
. You'll have to work within that constraint.
– Robert Harvey♦
Nov 21 '18 at 18:42
@RobertHarvey -- what I mean is.. with OAuth authentication, the user might need to add stuff specific to that authentication method... AddParameter() doesn't apply to the other types... so does it make sense to put it in RestClient? Then the user could just blindly call the method without it even making sense.
– SledgeHammer
Nov 21 '18 at 18:44
You probably want that to be anew OAuth(something)
somewhere in your fluent calls, then.
– Robert Harvey♦
Nov 21 '18 at 18:48
You could do what ASP.NET Core Identity does. It returns you anISomething
that's not theIServiceCollection
used by the .NET Core Fluent Interface
– Camilo Terevinto
Nov 21 '18 at 18:50
@RobertHarvey looking at some .net core code, it seems like they do my last example where they just have the outer object return this and include a Action / Func lambda if you need to set stuff specific to that... is that fluent or more of a .net core thing?
– SledgeHammer
Nov 21 '18 at 18:50
|
show 8 more comments
I'm playing around with creating a fluent api. For my learning, I'm just going to stub out a simple rest client.
What I've got so far is this:
public interface IOAuthAuthentication
{
void AddParameter(string name, string value);
}
public interface IRestClient
{
IRestClient WithBasicAuthentication(string username, string password);
IRestClient WithNetworkAuthentication(string username, string password);
IOAuthAuthentication WithOAuthAuthentication(Uri authorizationUrl, string clientId, string clientSecret);
}
public class RestClient : IRestClient
{
public RestClient(Uri root)
{
}
public IRestClient WithBasicAuthentication(string username, string password)
{
return this;
}
public IRestClient WithNetworkAuthentication(string username, string password)
{
return this;
}
public IOAuthAuthentication WithOAuthAuthentication(Uri authorizationUrl, string clientId, string clientSecret)
{
return null;
}
}
I kind of imagined using it like this:
IRestClient restClient = new RestClient(new Uri(""))
.WithBasicAuthentication("", "")
.WithNetworkAuthentication("", "")
.WithOAuthAuthentication(new Uri(""), "", "")
.AddParameter("", "");
Now, obviously, you wouldn't have 3 different authentication methods, but that would be handled in the methods themselves I suppose.
For the OAuth authentication, the user might need to add a custom parameter, so I defined a different return type... however, this has the side affect of you can't end the chain with WithOAuthAuthentication()
or .AddParameter()
.
What's the correct way to handle that in fluent? I've seen some .net core stuff and was maybe thinking off something like:
WithOAuthAuthentication("", "", "", x => x.AddParameter("", ""))
and have the WithOAuthAuthentication()
return IRestClient.
Would that be more "fluenty"?
But then the code doesn't look as cool if you have to add a bunch of params:
.WithOAuthAuthentication("", "", "", x =>
{
x.AddParameter("", "")
.AddParameter("", "")
});
c#
I'm playing around with creating a fluent api. For my learning, I'm just going to stub out a simple rest client.
What I've got so far is this:
public interface IOAuthAuthentication
{
void AddParameter(string name, string value);
}
public interface IRestClient
{
IRestClient WithBasicAuthentication(string username, string password);
IRestClient WithNetworkAuthentication(string username, string password);
IOAuthAuthentication WithOAuthAuthentication(Uri authorizationUrl, string clientId, string clientSecret);
}
public class RestClient : IRestClient
{
public RestClient(Uri root)
{
}
public IRestClient WithBasicAuthentication(string username, string password)
{
return this;
}
public IRestClient WithNetworkAuthentication(string username, string password)
{
return this;
}
public IOAuthAuthentication WithOAuthAuthentication(Uri authorizationUrl, string clientId, string clientSecret)
{
return null;
}
}
I kind of imagined using it like this:
IRestClient restClient = new RestClient(new Uri(""))
.WithBasicAuthentication("", "")
.WithNetworkAuthentication("", "")
.WithOAuthAuthentication(new Uri(""), "", "")
.AddParameter("", "");
Now, obviously, you wouldn't have 3 different authentication methods, but that would be handled in the methods themselves I suppose.
For the OAuth authentication, the user might need to add a custom parameter, so I defined a different return type... however, this has the side affect of you can't end the chain with WithOAuthAuthentication()
or .AddParameter()
.
What's the correct way to handle that in fluent? I've seen some .net core stuff and was maybe thinking off something like:
WithOAuthAuthentication("", "", "", x => x.AddParameter("", ""))
and have the WithOAuthAuthentication()
return IRestClient.
Would that be more "fluenty"?
But then the code doesn't look as cool if you have to add a bunch of params:
.WithOAuthAuthentication("", "", "", x =>
{
x.AddParameter("", "")
.AddParameter("", "")
});
c#
c#
asked Nov 21 '18 at 18:39
SledgeHammerSledgeHammer
3,57721131
3,57721131
For the OAuth authentication, the user might need to add a custom parameter, so I defined a different return type
-- I don't understand what you mean. For Fluent Interfaces, the return type of a method is always going to bethis
. You'll have to work within that constraint.
– Robert Harvey♦
Nov 21 '18 at 18:42
@RobertHarvey -- what I mean is.. with OAuth authentication, the user might need to add stuff specific to that authentication method... AddParameter() doesn't apply to the other types... so does it make sense to put it in RestClient? Then the user could just blindly call the method without it even making sense.
– SledgeHammer
Nov 21 '18 at 18:44
You probably want that to be anew OAuth(something)
somewhere in your fluent calls, then.
– Robert Harvey♦
Nov 21 '18 at 18:48
You could do what ASP.NET Core Identity does. It returns you anISomething
that's not theIServiceCollection
used by the .NET Core Fluent Interface
– Camilo Terevinto
Nov 21 '18 at 18:50
@RobertHarvey looking at some .net core code, it seems like they do my last example where they just have the outer object return this and include a Action / Func lambda if you need to set stuff specific to that... is that fluent or more of a .net core thing?
– SledgeHammer
Nov 21 '18 at 18:50
|
show 8 more comments
For the OAuth authentication, the user might need to add a custom parameter, so I defined a different return type
-- I don't understand what you mean. For Fluent Interfaces, the return type of a method is always going to bethis
. You'll have to work within that constraint.
– Robert Harvey♦
Nov 21 '18 at 18:42
@RobertHarvey -- what I mean is.. with OAuth authentication, the user might need to add stuff specific to that authentication method... AddParameter() doesn't apply to the other types... so does it make sense to put it in RestClient? Then the user could just blindly call the method without it even making sense.
– SledgeHammer
Nov 21 '18 at 18:44
You probably want that to be anew OAuth(something)
somewhere in your fluent calls, then.
– Robert Harvey♦
Nov 21 '18 at 18:48
You could do what ASP.NET Core Identity does. It returns you anISomething
that's not theIServiceCollection
used by the .NET Core Fluent Interface
– Camilo Terevinto
Nov 21 '18 at 18:50
@RobertHarvey looking at some .net core code, it seems like they do my last example where they just have the outer object return this and include a Action / Func lambda if you need to set stuff specific to that... is that fluent or more of a .net core thing?
– SledgeHammer
Nov 21 '18 at 18:50
For the OAuth authentication, the user might need to add a custom parameter, so I defined a different return type
-- I don't understand what you mean. For Fluent Interfaces, the return type of a method is always going to be this
. You'll have to work within that constraint.– Robert Harvey♦
Nov 21 '18 at 18:42
For the OAuth authentication, the user might need to add a custom parameter, so I defined a different return type
-- I don't understand what you mean. For Fluent Interfaces, the return type of a method is always going to be this
. You'll have to work within that constraint.– Robert Harvey♦
Nov 21 '18 at 18:42
@RobertHarvey -- what I mean is.. with OAuth authentication, the user might need to add stuff specific to that authentication method... AddParameter() doesn't apply to the other types... so does it make sense to put it in RestClient? Then the user could just blindly call the method without it even making sense.
– SledgeHammer
Nov 21 '18 at 18:44
@RobertHarvey -- what I mean is.. with OAuth authentication, the user might need to add stuff specific to that authentication method... AddParameter() doesn't apply to the other types... so does it make sense to put it in RestClient? Then the user could just blindly call the method without it even making sense.
– SledgeHammer
Nov 21 '18 at 18:44
You probably want that to be a
new OAuth(something)
somewhere in your fluent calls, then.– Robert Harvey♦
Nov 21 '18 at 18:48
You probably want that to be a
new OAuth(something)
somewhere in your fluent calls, then.– Robert Harvey♦
Nov 21 '18 at 18:48
You could do what ASP.NET Core Identity does. It returns you an
ISomething
that's not the IServiceCollection
used by the .NET Core Fluent Interface– Camilo Terevinto
Nov 21 '18 at 18:50
You could do what ASP.NET Core Identity does. It returns you an
ISomething
that's not the IServiceCollection
used by the .NET Core Fluent Interface– Camilo Terevinto
Nov 21 '18 at 18:50
@RobertHarvey looking at some .net core code, it seems like they do my last example where they just have the outer object return this and include a Action / Func lambda if you need to set stuff specific to that... is that fluent or more of a .net core thing?
– SledgeHammer
Nov 21 '18 at 18:50
@RobertHarvey looking at some .net core code, it seems like they do my last example where they just have the outer object return this and include a Action / Func lambda if you need to set stuff specific to that... is that fluent or more of a .net core thing?
– SledgeHammer
Nov 21 '18 at 18:50
|
show 8 more comments
1 Answer
1
active
oldest
votes
This is a pretty subjective question, because there are a lot of styles of Fluent APIs out there. Some may even be fine with mutation of the this
context in which case you could use a Begin/End pattern for your OAuth and between those two method calls would be a mutated version of this
that is your custom OAuth provider.
If it were me, I would modify your base method signature to:
public IRestClient WithOAuthAuthentication(Action<IOAuthAuthentication> oauthHandler)
{
oauthHandler?.Invoke(new OAuthAuthentication(this));
return this;
}
Then I would see your usage pattern looking something like this:
restClient.WithOAuthAuthentication(
oauth =>
{
oauth.SetEndpoint("https://www.example.com")
.AddParameter("Param1", "CoolValue")
.AddParameter("Param2", "CoolValue2")
.Authenticate();
}
)
.YourNextFluentAction();
This is also called "Hadouken" style, because chaining them like this is very similar to Lisp coding style and their pyramide functions =) google.com/search?q=hadouken+coding&tbm=isch
– eocron
Nov 21 '18 at 18:59
Thanks... I'll probably go the action route since that's all over the place in .net core it seems, but probably keep the uri, username, password as params and add an overload for the optional action since that's probably an edge case.
– SledgeHammer
Nov 21 '18 at 19:01
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53418593%2fcreating-fluent-api-issue%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is a pretty subjective question, because there are a lot of styles of Fluent APIs out there. Some may even be fine with mutation of the this
context in which case you could use a Begin/End pattern for your OAuth and between those two method calls would be a mutated version of this
that is your custom OAuth provider.
If it were me, I would modify your base method signature to:
public IRestClient WithOAuthAuthentication(Action<IOAuthAuthentication> oauthHandler)
{
oauthHandler?.Invoke(new OAuthAuthentication(this));
return this;
}
Then I would see your usage pattern looking something like this:
restClient.WithOAuthAuthentication(
oauth =>
{
oauth.SetEndpoint("https://www.example.com")
.AddParameter("Param1", "CoolValue")
.AddParameter("Param2", "CoolValue2")
.Authenticate();
}
)
.YourNextFluentAction();
This is also called "Hadouken" style, because chaining them like this is very similar to Lisp coding style and their pyramide functions =) google.com/search?q=hadouken+coding&tbm=isch
– eocron
Nov 21 '18 at 18:59
Thanks... I'll probably go the action route since that's all over the place in .net core it seems, but probably keep the uri, username, password as params and add an overload for the optional action since that's probably an edge case.
– SledgeHammer
Nov 21 '18 at 19:01
add a comment |
This is a pretty subjective question, because there are a lot of styles of Fluent APIs out there. Some may even be fine with mutation of the this
context in which case you could use a Begin/End pattern for your OAuth and between those two method calls would be a mutated version of this
that is your custom OAuth provider.
If it were me, I would modify your base method signature to:
public IRestClient WithOAuthAuthentication(Action<IOAuthAuthentication> oauthHandler)
{
oauthHandler?.Invoke(new OAuthAuthentication(this));
return this;
}
Then I would see your usage pattern looking something like this:
restClient.WithOAuthAuthentication(
oauth =>
{
oauth.SetEndpoint("https://www.example.com")
.AddParameter("Param1", "CoolValue")
.AddParameter("Param2", "CoolValue2")
.Authenticate();
}
)
.YourNextFluentAction();
This is also called "Hadouken" style, because chaining them like this is very similar to Lisp coding style and their pyramide functions =) google.com/search?q=hadouken+coding&tbm=isch
– eocron
Nov 21 '18 at 18:59
Thanks... I'll probably go the action route since that's all over the place in .net core it seems, but probably keep the uri, username, password as params and add an overload for the optional action since that's probably an edge case.
– SledgeHammer
Nov 21 '18 at 19:01
add a comment |
This is a pretty subjective question, because there are a lot of styles of Fluent APIs out there. Some may even be fine with mutation of the this
context in which case you could use a Begin/End pattern for your OAuth and between those two method calls would be a mutated version of this
that is your custom OAuth provider.
If it were me, I would modify your base method signature to:
public IRestClient WithOAuthAuthentication(Action<IOAuthAuthentication> oauthHandler)
{
oauthHandler?.Invoke(new OAuthAuthentication(this));
return this;
}
Then I would see your usage pattern looking something like this:
restClient.WithOAuthAuthentication(
oauth =>
{
oauth.SetEndpoint("https://www.example.com")
.AddParameter("Param1", "CoolValue")
.AddParameter("Param2", "CoolValue2")
.Authenticate();
}
)
.YourNextFluentAction();
This is a pretty subjective question, because there are a lot of styles of Fluent APIs out there. Some may even be fine with mutation of the this
context in which case you could use a Begin/End pattern for your OAuth and between those two method calls would be a mutated version of this
that is your custom OAuth provider.
If it were me, I would modify your base method signature to:
public IRestClient WithOAuthAuthentication(Action<IOAuthAuthentication> oauthHandler)
{
oauthHandler?.Invoke(new OAuthAuthentication(this));
return this;
}
Then I would see your usage pattern looking something like this:
restClient.WithOAuthAuthentication(
oauth =>
{
oauth.SetEndpoint("https://www.example.com")
.AddParameter("Param1", "CoolValue")
.AddParameter("Param2", "CoolValue2")
.Authenticate();
}
)
.YourNextFluentAction();
answered Nov 21 '18 at 18:54


Shawn LehnerShawn Lehner
1,260712
1,260712
This is also called "Hadouken" style, because chaining them like this is very similar to Lisp coding style and their pyramide functions =) google.com/search?q=hadouken+coding&tbm=isch
– eocron
Nov 21 '18 at 18:59
Thanks... I'll probably go the action route since that's all over the place in .net core it seems, but probably keep the uri, username, password as params and add an overload for the optional action since that's probably an edge case.
– SledgeHammer
Nov 21 '18 at 19:01
add a comment |
This is also called "Hadouken" style, because chaining them like this is very similar to Lisp coding style and their pyramide functions =) google.com/search?q=hadouken+coding&tbm=isch
– eocron
Nov 21 '18 at 18:59
Thanks... I'll probably go the action route since that's all over the place in .net core it seems, but probably keep the uri, username, password as params and add an overload for the optional action since that's probably an edge case.
– SledgeHammer
Nov 21 '18 at 19:01
This is also called "Hadouken" style, because chaining them like this is very similar to Lisp coding style and their pyramide functions =) google.com/search?q=hadouken+coding&tbm=isch
– eocron
Nov 21 '18 at 18:59
This is also called "Hadouken" style, because chaining them like this is very similar to Lisp coding style and their pyramide functions =) google.com/search?q=hadouken+coding&tbm=isch
– eocron
Nov 21 '18 at 18:59
Thanks... I'll probably go the action route since that's all over the place in .net core it seems, but probably keep the uri, username, password as params and add an overload for the optional action since that's probably an edge case.
– SledgeHammer
Nov 21 '18 at 19:01
Thanks... I'll probably go the action route since that's all over the place in .net core it seems, but probably keep the uri, username, password as params and add an overload for the optional action since that's probably an edge case.
– SledgeHammer
Nov 21 '18 at 19:01
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53418593%2fcreating-fluent-api-issue%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gM4D7 yx0 jFXsPCMuWpbBTn8k2,OWLZObsCi ts
For the OAuth authentication, the user might need to add a custom parameter, so I defined a different return type
-- I don't understand what you mean. For Fluent Interfaces, the return type of a method is always going to bethis
. You'll have to work within that constraint.– Robert Harvey♦
Nov 21 '18 at 18:42
@RobertHarvey -- what I mean is.. with OAuth authentication, the user might need to add stuff specific to that authentication method... AddParameter() doesn't apply to the other types... so does it make sense to put it in RestClient? Then the user could just blindly call the method without it even making sense.
– SledgeHammer
Nov 21 '18 at 18:44
You probably want that to be a
new OAuth(something)
somewhere in your fluent calls, then.– Robert Harvey♦
Nov 21 '18 at 18:48
You could do what ASP.NET Core Identity does. It returns you an
ISomething
that's not theIServiceCollection
used by the .NET Core Fluent Interface– Camilo Terevinto
Nov 21 '18 at 18:50
@RobertHarvey looking at some .net core code, it seems like they do my last example where they just have the outer object return this and include a Action / Func lambda if you need to set stuff specific to that... is that fluent or more of a .net core thing?
– SledgeHammer
Nov 21 '18 at 18:50