Pytest: use parametrize output of fixture as class instance name in test
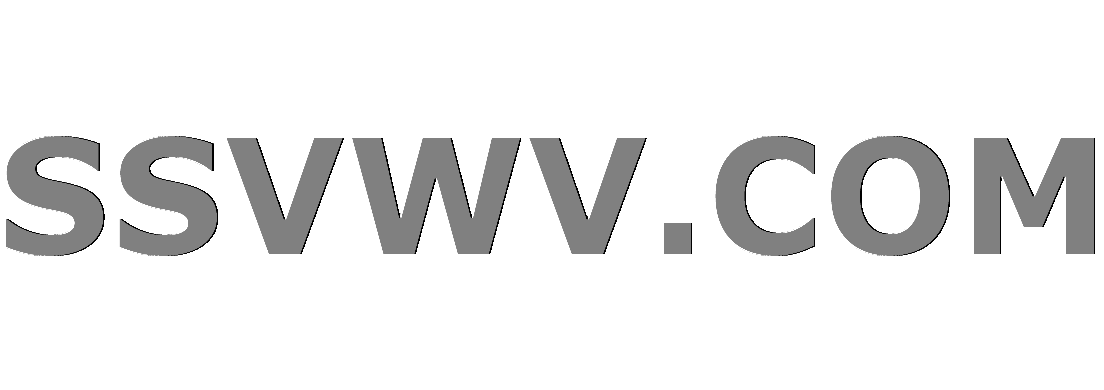
Multi tool use
I have a problem with using data provided by pytest_generate_tests
. The point is that data is provided as tuple of string values, and further in tests i need one of them to be used as name of the tested class, and i can't find solution how to initialize class with this str value as it's name
Here is a simple class for tests:
class Grade(Enum):
TRAINEE = 1
JUNIOR = 2
MIDDLE = 5
SENIOR = 10
class Employee(metaclass=ABCMeta):
def __init__(self, name, surname, grade: Grade):
self.name = name
self.surname = surname
base = 300
self.salary = int(base * grade.value)
class QAEngineer(Employee):
def __init__(self, name, surname, salary):
super().__init__(name, surname, salary)
and a simple test to check if instances are initialized correctly.
For this test fixture with pytest_generate_tests
method is written. Fixture uses data from simple input txt file in the form:
QAEngineer, Mary, Mills, SENIOR
and create from it param_list
in the following way:
[['QAEngineer', ('Mary', 'Mills', 'SENIOR')]]
and return as:
metafunc.parametrize("emplopyee_class, employee_parameters", tuple(params_list2))
so employee_class = QAEngineer, employee_parameters = ('Mary', 'Mills', 'SENIOR')
then i need to provide emplopyee_class, employee_parameters
values in my test, and here the problem is - since emplopyee_class
is str, i can't use it as class name when create QAEngineer instance for test, so i need to hardcode it, i.e.:
def test_emp_append_file(emplopyee_class, employee_parameters):
grade = Grade.__getattr__(employee_parameters[2])
employee = QAEngineer(employee_parameters[0], employee_parameters[1], grade)
assert employee.name == employee_parameters[0]
assert employee.surname == employee_parameters[1]
How can i change emplopyee_class
type and to what type to make it possible to use as a class name, so to wrote something like this instead:
employee = emplopyee_class(employee_parameters[0], employee_parameters[1], grade)
?
python pytest
|
show 2 more comments
I have a problem with using data provided by pytest_generate_tests
. The point is that data is provided as tuple of string values, and further in tests i need one of them to be used as name of the tested class, and i can't find solution how to initialize class with this str value as it's name
Here is a simple class for tests:
class Grade(Enum):
TRAINEE = 1
JUNIOR = 2
MIDDLE = 5
SENIOR = 10
class Employee(metaclass=ABCMeta):
def __init__(self, name, surname, grade: Grade):
self.name = name
self.surname = surname
base = 300
self.salary = int(base * grade.value)
class QAEngineer(Employee):
def __init__(self, name, surname, salary):
super().__init__(name, surname, salary)
and a simple test to check if instances are initialized correctly.
For this test fixture with pytest_generate_tests
method is written. Fixture uses data from simple input txt file in the form:
QAEngineer, Mary, Mills, SENIOR
and create from it param_list
in the following way:
[['QAEngineer', ('Mary', 'Mills', 'SENIOR')]]
and return as:
metafunc.parametrize("emplopyee_class, employee_parameters", tuple(params_list2))
so employee_class = QAEngineer, employee_parameters = ('Mary', 'Mills', 'SENIOR')
then i need to provide emplopyee_class, employee_parameters
values in my test, and here the problem is - since emplopyee_class
is str, i can't use it as class name when create QAEngineer instance for test, so i need to hardcode it, i.e.:
def test_emp_append_file(emplopyee_class, employee_parameters):
grade = Grade.__getattr__(employee_parameters[2])
employee = QAEngineer(employee_parameters[0], employee_parameters[1], grade)
assert employee.name == employee_parameters[0]
assert employee.surname == employee_parameters[1]
How can i change emplopyee_class
type and to what type to make it possible to use as a class name, so to wrote something like this instead:
employee = emplopyee_class(employee_parameters[0], employee_parameters[1], grade)
?
python pytest
Why not useQAEngineer
class object instead of class name inparam_list
?
– hoefling
Nov 21 at 0:08
@hoefling there are few more similar classes, and they all should be given in input file in the way given above. Or you meant readQAEngineer
from file and then pass it toparam_list
not as simple str value, but as class object?Have no ideas how to do it either
– Назарій Кушнір
Nov 21 at 10:25
Oh, somehow I overlooked the input data reading from text file. However, you can invoke theimportlib
machinery for dynamic importing of classes, given their names; you would just need to store the qualified class name (likepkg.module.QAEngineer
- same as you use to import the class in code). If you need a working example, complete your code to a Minimal, Complete, and Verifiable example so I can run it from my machine.
– hoefling
Nov 21 at 10:42
@hoefling, will it be ok? .py file with classes, fixture and simple test - pastebin.com/HhqFkQrH input_data.txt with test data for test - pastebin.com/ACatrd1w
– Назарій Кушнір
Nov 21 at 11:23
If you want to keep the tested code in the same file as the tests, then you can simply take the class fromglobals()
: changeparams_list1.append([vals[0])
toparams_list1.append(globals()[vals[0]])
and your example should work already.
– hoefling
Nov 22 at 1:41
|
show 2 more comments
I have a problem with using data provided by pytest_generate_tests
. The point is that data is provided as tuple of string values, and further in tests i need one of them to be used as name of the tested class, and i can't find solution how to initialize class with this str value as it's name
Here is a simple class for tests:
class Grade(Enum):
TRAINEE = 1
JUNIOR = 2
MIDDLE = 5
SENIOR = 10
class Employee(metaclass=ABCMeta):
def __init__(self, name, surname, grade: Grade):
self.name = name
self.surname = surname
base = 300
self.salary = int(base * grade.value)
class QAEngineer(Employee):
def __init__(self, name, surname, salary):
super().__init__(name, surname, salary)
and a simple test to check if instances are initialized correctly.
For this test fixture with pytest_generate_tests
method is written. Fixture uses data from simple input txt file in the form:
QAEngineer, Mary, Mills, SENIOR
and create from it param_list
in the following way:
[['QAEngineer', ('Mary', 'Mills', 'SENIOR')]]
and return as:
metafunc.parametrize("emplopyee_class, employee_parameters", tuple(params_list2))
so employee_class = QAEngineer, employee_parameters = ('Mary', 'Mills', 'SENIOR')
then i need to provide emplopyee_class, employee_parameters
values in my test, and here the problem is - since emplopyee_class
is str, i can't use it as class name when create QAEngineer instance for test, so i need to hardcode it, i.e.:
def test_emp_append_file(emplopyee_class, employee_parameters):
grade = Grade.__getattr__(employee_parameters[2])
employee = QAEngineer(employee_parameters[0], employee_parameters[1], grade)
assert employee.name == employee_parameters[0]
assert employee.surname == employee_parameters[1]
How can i change emplopyee_class
type and to what type to make it possible to use as a class name, so to wrote something like this instead:
employee = emplopyee_class(employee_parameters[0], employee_parameters[1], grade)
?
python pytest
I have a problem with using data provided by pytest_generate_tests
. The point is that data is provided as tuple of string values, and further in tests i need one of them to be used as name of the tested class, and i can't find solution how to initialize class with this str value as it's name
Here is a simple class for tests:
class Grade(Enum):
TRAINEE = 1
JUNIOR = 2
MIDDLE = 5
SENIOR = 10
class Employee(metaclass=ABCMeta):
def __init__(self, name, surname, grade: Grade):
self.name = name
self.surname = surname
base = 300
self.salary = int(base * grade.value)
class QAEngineer(Employee):
def __init__(self, name, surname, salary):
super().__init__(name, surname, salary)
and a simple test to check if instances are initialized correctly.
For this test fixture with pytest_generate_tests
method is written. Fixture uses data from simple input txt file in the form:
QAEngineer, Mary, Mills, SENIOR
and create from it param_list
in the following way:
[['QAEngineer', ('Mary', 'Mills', 'SENIOR')]]
and return as:
metafunc.parametrize("emplopyee_class, employee_parameters", tuple(params_list2))
so employee_class = QAEngineer, employee_parameters = ('Mary', 'Mills', 'SENIOR')
then i need to provide emplopyee_class, employee_parameters
values in my test, and here the problem is - since emplopyee_class
is str, i can't use it as class name when create QAEngineer instance for test, so i need to hardcode it, i.e.:
def test_emp_append_file(emplopyee_class, employee_parameters):
grade = Grade.__getattr__(employee_parameters[2])
employee = QAEngineer(employee_parameters[0], employee_parameters[1], grade)
assert employee.name == employee_parameters[0]
assert employee.surname == employee_parameters[1]
How can i change emplopyee_class
type and to what type to make it possible to use as a class name, so to wrote something like this instead:
employee = emplopyee_class(employee_parameters[0], employee_parameters[1], grade)
?
python pytest
python pytest
edited Nov 20 at 22:58


dustinos3
4691616
4691616
asked Nov 20 at 21:46


Назарій Кушнір
256
256
Why not useQAEngineer
class object instead of class name inparam_list
?
– hoefling
Nov 21 at 0:08
@hoefling there are few more similar classes, and they all should be given in input file in the way given above. Or you meant readQAEngineer
from file and then pass it toparam_list
not as simple str value, but as class object?Have no ideas how to do it either
– Назарій Кушнір
Nov 21 at 10:25
Oh, somehow I overlooked the input data reading from text file. However, you can invoke theimportlib
machinery for dynamic importing of classes, given their names; you would just need to store the qualified class name (likepkg.module.QAEngineer
- same as you use to import the class in code). If you need a working example, complete your code to a Minimal, Complete, and Verifiable example so I can run it from my machine.
– hoefling
Nov 21 at 10:42
@hoefling, will it be ok? .py file with classes, fixture and simple test - pastebin.com/HhqFkQrH input_data.txt with test data for test - pastebin.com/ACatrd1w
– Назарій Кушнір
Nov 21 at 11:23
If you want to keep the tested code in the same file as the tests, then you can simply take the class fromglobals()
: changeparams_list1.append([vals[0])
toparams_list1.append(globals()[vals[0]])
and your example should work already.
– hoefling
Nov 22 at 1:41
|
show 2 more comments
Why not useQAEngineer
class object instead of class name inparam_list
?
– hoefling
Nov 21 at 0:08
@hoefling there are few more similar classes, and they all should be given in input file in the way given above. Or you meant readQAEngineer
from file and then pass it toparam_list
not as simple str value, but as class object?Have no ideas how to do it either
– Назарій Кушнір
Nov 21 at 10:25
Oh, somehow I overlooked the input data reading from text file. However, you can invoke theimportlib
machinery for dynamic importing of classes, given their names; you would just need to store the qualified class name (likepkg.module.QAEngineer
- same as you use to import the class in code). If you need a working example, complete your code to a Minimal, Complete, and Verifiable example so I can run it from my machine.
– hoefling
Nov 21 at 10:42
@hoefling, will it be ok? .py file with classes, fixture and simple test - pastebin.com/HhqFkQrH input_data.txt with test data for test - pastebin.com/ACatrd1w
– Назарій Кушнір
Nov 21 at 11:23
If you want to keep the tested code in the same file as the tests, then you can simply take the class fromglobals()
: changeparams_list1.append([vals[0])
toparams_list1.append(globals()[vals[0]])
and your example should work already.
– hoefling
Nov 22 at 1:41
Why not use
QAEngineer
class object instead of class name in param_list
?– hoefling
Nov 21 at 0:08
Why not use
QAEngineer
class object instead of class name in param_list
?– hoefling
Nov 21 at 0:08
@hoefling there are few more similar classes, and they all should be given in input file in the way given above. Or you meant read
QAEngineer
from file and then pass it to param_list
not as simple str value, but as class object?Have no ideas how to do it either– Назарій Кушнір
Nov 21 at 10:25
@hoefling there are few more similar classes, and they all should be given in input file in the way given above. Or you meant read
QAEngineer
from file and then pass it to param_list
not as simple str value, but as class object?Have no ideas how to do it either– Назарій Кушнір
Nov 21 at 10:25
Oh, somehow I overlooked the input data reading from text file. However, you can invoke the
importlib
machinery for dynamic importing of classes, given their names; you would just need to store the qualified class name (like pkg.module.QAEngineer
- same as you use to import the class in code). If you need a working example, complete your code to a Minimal, Complete, and Verifiable example so I can run it from my machine.– hoefling
Nov 21 at 10:42
Oh, somehow I overlooked the input data reading from text file. However, you can invoke the
importlib
machinery for dynamic importing of classes, given their names; you would just need to store the qualified class name (like pkg.module.QAEngineer
- same as you use to import the class in code). If you need a working example, complete your code to a Minimal, Complete, and Verifiable example so I can run it from my machine.– hoefling
Nov 21 at 10:42
@hoefling, will it be ok? .py file with classes, fixture and simple test - pastebin.com/HhqFkQrH input_data.txt with test data for test - pastebin.com/ACatrd1w
– Назарій Кушнір
Nov 21 at 11:23
@hoefling, will it be ok? .py file with classes, fixture and simple test - pastebin.com/HhqFkQrH input_data.txt with test data for test - pastebin.com/ACatrd1w
– Назарій Кушнір
Nov 21 at 11:23
If you want to keep the tested code in the same file as the tests, then you can simply take the class from
globals()
: change params_list1.append([vals[0])
to params_list1.append(globals()[vals[0]])
and your example should work already.– hoefling
Nov 22 at 1:41
If you want to keep the tested code in the same file as the tests, then you can simply take the class from
globals()
: change params_list1.append([vals[0])
to params_list1.append(globals()[vals[0]])
and your example should work already.– hoefling
Nov 22 at 1:41
|
show 2 more comments
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402062%2fpytest-use-parametrize-output-of-fixture-as-class-instance-name-in-test%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402062%2fpytest-use-parametrize-output-of-fixture-as-class-instance-name-in-test%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
j 4pg7ApAn,QxBl0Jf A2NWrxmjqWPbWuT9Eeh8PVjZ3VVNAplEA gy,vpbd,nNL,g6 V8 1 e
Why not use
QAEngineer
class object instead of class name inparam_list
?– hoefling
Nov 21 at 0:08
@hoefling there are few more similar classes, and they all should be given in input file in the way given above. Or you meant read
QAEngineer
from file and then pass it toparam_list
not as simple str value, but as class object?Have no ideas how to do it either– Назарій Кушнір
Nov 21 at 10:25
Oh, somehow I overlooked the input data reading from text file. However, you can invoke the
importlib
machinery for dynamic importing of classes, given their names; you would just need to store the qualified class name (likepkg.module.QAEngineer
- same as you use to import the class in code). If you need a working example, complete your code to a Minimal, Complete, and Verifiable example so I can run it from my machine.– hoefling
Nov 21 at 10:42
@hoefling, will it be ok? .py file with classes, fixture and simple test - pastebin.com/HhqFkQrH input_data.txt with test data for test - pastebin.com/ACatrd1w
– Назарій Кушнір
Nov 21 at 11:23
If you want to keep the tested code in the same file as the tests, then you can simply take the class from
globals()
: changeparams_list1.append([vals[0])
toparams_list1.append(globals()[vals[0]])
and your example should work already.– hoefling
Nov 22 at 1:41