How should data be loaded asynchronously in Blazor
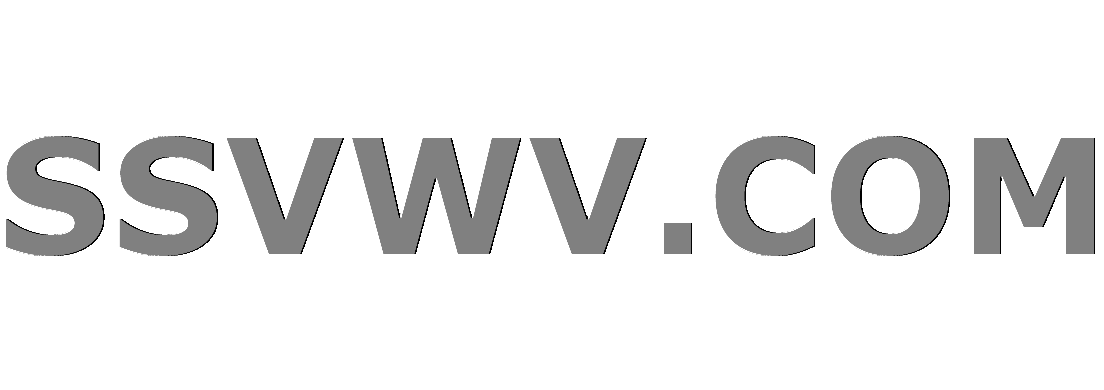
Multi tool use
I have been playing with Blazor and trying to build a simple application. One of the first things I tried to do was to load data asynchronously (in my case from LocalStorage).
protected override async Task OnInitAsync()
{
await Log.Log($"{nameof(IndexComponent)}.{nameof(OnInitAsync)}");
Model = await LocalStorage.GetItem<DataModel>("model");
}
My rendering code looked like this:
<div>@Model.Attribute</div>
I was getting a NullReferenceException when rendering the page, plus the browser went into an unresponsive state at which point all I could was to close the browser tab and restart the Blazor application.
Even though it turned out this behaviour is by-design, I haven't found it explained on any piece of documentation and I think it's unexpected enough to grant sharing here. Hopefully it will help someone else (see my own answer below).
blazor
add a comment |
I have been playing with Blazor and trying to build a simple application. One of the first things I tried to do was to load data asynchronously (in my case from LocalStorage).
protected override async Task OnInitAsync()
{
await Log.Log($"{nameof(IndexComponent)}.{nameof(OnInitAsync)}");
Model = await LocalStorage.GetItem<DataModel>("model");
}
My rendering code looked like this:
<div>@Model.Attribute</div>
I was getting a NullReferenceException when rendering the page, plus the browser went into an unresponsive state at which point all I could was to close the browser tab and restart the Blazor application.
Even though it turned out this behaviour is by-design, I haven't found it explained on any piece of documentation and I think it's unexpected enough to grant sharing here. Hopefully it will help someone else (see my own answer below).
blazor
add a comment |
I have been playing with Blazor and trying to build a simple application. One of the first things I tried to do was to load data asynchronously (in my case from LocalStorage).
protected override async Task OnInitAsync()
{
await Log.Log($"{nameof(IndexComponent)}.{nameof(OnInitAsync)}");
Model = await LocalStorage.GetItem<DataModel>("model");
}
My rendering code looked like this:
<div>@Model.Attribute</div>
I was getting a NullReferenceException when rendering the page, plus the browser went into an unresponsive state at which point all I could was to close the browser tab and restart the Blazor application.
Even though it turned out this behaviour is by-design, I haven't found it explained on any piece of documentation and I think it's unexpected enough to grant sharing here. Hopefully it will help someone else (see my own answer below).
blazor
I have been playing with Blazor and trying to build a simple application. One of the first things I tried to do was to load data asynchronously (in my case from LocalStorage).
protected override async Task OnInitAsync()
{
await Log.Log($"{nameof(IndexComponent)}.{nameof(OnInitAsync)}");
Model = await LocalStorage.GetItem<DataModel>("model");
}
My rendering code looked like this:
<div>@Model.Attribute</div>
I was getting a NullReferenceException when rendering the page, plus the browser went into an unresponsive state at which point all I could was to close the browser tab and restart the Blazor application.
Even though it turned out this behaviour is by-design, I haven't found it explained on any piece of documentation and I think it's unexpected enough to grant sharing here. Hopefully it will help someone else (see my own answer below).
blazor
blazor
asked Nov 20 at 21:36


Rodolfo Grave
579511
579511
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
It all boils down to:
Blazor calls your OnInitAsync method and renders your page first time immediately after first suspension (await). Then it renders your page again when your method finishes.
There are two solutions. The first one is to make sure your rendering code handles the case in which Model is null. So, instead of:
<div>@Model.Attribute</div>
use:
@if (Model != null)
{
<div>@Model.Attribute</div>
}
The other, and simpler solution, is to make sure Model is never null:
protected DataModel Model { get; } = new DataModel();
Initially I raised an issue in Blazor's GitHub, from where I copied the answer almost verbatim: https://github.com/aspnet/Blazor/issues/1703
Very helpful members of the community very quickly pointed out what the issue was, and all the credit goes to them. I'm just trying to help other people by bringing the knowledge to StackOverflow.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53401935%2fhow-should-data-be-loaded-asynchronously-in-blazor%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
It all boils down to:
Blazor calls your OnInitAsync method and renders your page first time immediately after first suspension (await). Then it renders your page again when your method finishes.
There are two solutions. The first one is to make sure your rendering code handles the case in which Model is null. So, instead of:
<div>@Model.Attribute</div>
use:
@if (Model != null)
{
<div>@Model.Attribute</div>
}
The other, and simpler solution, is to make sure Model is never null:
protected DataModel Model { get; } = new DataModel();
Initially I raised an issue in Blazor's GitHub, from where I copied the answer almost verbatim: https://github.com/aspnet/Blazor/issues/1703
Very helpful members of the community very quickly pointed out what the issue was, and all the credit goes to them. I'm just trying to help other people by bringing the knowledge to StackOverflow.
add a comment |
It all boils down to:
Blazor calls your OnInitAsync method and renders your page first time immediately after first suspension (await). Then it renders your page again when your method finishes.
There are two solutions. The first one is to make sure your rendering code handles the case in which Model is null. So, instead of:
<div>@Model.Attribute</div>
use:
@if (Model != null)
{
<div>@Model.Attribute</div>
}
The other, and simpler solution, is to make sure Model is never null:
protected DataModel Model { get; } = new DataModel();
Initially I raised an issue in Blazor's GitHub, from where I copied the answer almost verbatim: https://github.com/aspnet/Blazor/issues/1703
Very helpful members of the community very quickly pointed out what the issue was, and all the credit goes to them. I'm just trying to help other people by bringing the knowledge to StackOverflow.
add a comment |
It all boils down to:
Blazor calls your OnInitAsync method and renders your page first time immediately after first suspension (await). Then it renders your page again when your method finishes.
There are two solutions. The first one is to make sure your rendering code handles the case in which Model is null. So, instead of:
<div>@Model.Attribute</div>
use:
@if (Model != null)
{
<div>@Model.Attribute</div>
}
The other, and simpler solution, is to make sure Model is never null:
protected DataModel Model { get; } = new DataModel();
Initially I raised an issue in Blazor's GitHub, from where I copied the answer almost verbatim: https://github.com/aspnet/Blazor/issues/1703
Very helpful members of the community very quickly pointed out what the issue was, and all the credit goes to them. I'm just trying to help other people by bringing the knowledge to StackOverflow.
It all boils down to:
Blazor calls your OnInitAsync method and renders your page first time immediately after first suspension (await). Then it renders your page again when your method finishes.
There are two solutions. The first one is to make sure your rendering code handles the case in which Model is null. So, instead of:
<div>@Model.Attribute</div>
use:
@if (Model != null)
{
<div>@Model.Attribute</div>
}
The other, and simpler solution, is to make sure Model is never null:
protected DataModel Model { get; } = new DataModel();
Initially I raised an issue in Blazor's GitHub, from where I copied the answer almost verbatim: https://github.com/aspnet/Blazor/issues/1703
Very helpful members of the community very quickly pointed out what the issue was, and all the credit goes to them. I'm just trying to help other people by bringing the knowledge to StackOverflow.
answered Nov 20 at 21:36


Rodolfo Grave
579511
579511
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53401935%2fhow-should-data-be-loaded-asynchronously-in-blazor%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xKc,l7q2GRalUoSSTHGx,FHYHTdE8qpS nbVza2y324CUoSDh 5UXA QE,HCeupX,JqdQKIKLUhXrBpCcPr9tvvlil1HQYDupHS,ugm8